Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
video_input_typedef.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file video_input_typedef.h 00025 * @brief $Rev: 47 $ 00026 * $Date:: 2014-01-06 18:18:48 +0900#$ 00027 */ 00028 00029 #ifndef VIDEO_INPUT_TYPEDEF_H 00030 #define VIDEO_INPUT_TYPEDEF_H 00031 00032 /****************************************************************************** 00033 Includes <System Includes> , "Project Includes" 00034 ******************************************************************************/ 00035 #include "r_vdc5.h" 00036 #include "r_vdec.h" 00037 #include "frame_buffer_typedef.h " 00038 #include "r_ospl_typedef.h" 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif /* __cplusplus */ 00043 00044 00045 /****************************************************************************** 00046 Macro definitions 00047 ******************************************************************************/ 00048 00049 /*********************************************************************** 00050 * Macros: INTERRUPT_FUNCTION_TYPE 00051 * INTERRUPT_FUNCTION_TYPE 00052 * 00053 * : INTERRUPT_FUNCTION_TYPE_OS_RTX - RTX 00054 * : INTERRUPT_FUNCTION_TYPE_OS_LESS - OS less 00055 ************************************************************************/ 00056 #if R_OSPL_IS_PREEMPTION 00057 #define INTERRUPT_FUNCTION_TYPE INTERRUPT_FUNCTION_TYPE_OS_RTX 00058 #else 00059 #define INTERRUPT_FUNCTION_TYPE INTERRUPT_FUNCTION_TYPE_OS_LESS 00060 #endif 00061 #define INTERRUPT_FUNCTION_TYPE_OS_LESS 0 00062 #define INTERRUPT_FUNCTION_TYPE_OS_RTX 1 00063 00064 00065 /****************************************************************************** 00066 Typedef definitions 00067 ******************************************************************************/ 00068 00069 /** 00070 * @enum video_input_channel_enum_t 00071 * @brief Special integer value 00072 * 00073 * - VIDEO_INPUT_NOT_DISPLAY - 0xFF 00074 */ 00075 enum st_video_input_channel_enum_t { 00076 VIDEO_INPUT_NOT_DISPLAY = 0xFF 00077 }; 00078 typedef enum st_video_input_channel_enum_t video_input_channel_enum_t; 00079 /* "typedef" must be after "enum". Because GCC_ARM raises an error */ 00080 00081 00082 /** 00083 * @struct video_input_t 00084 * @brief video_input_t 00085 * 00086 * @par Description 00087 * Member variables can not be accessed by user. 00088 */ 00089 typedef struct st_video_input_t video_input_t; 00090 struct st_video_input_t { 00091 00092 /*-----------------------------------------------------------*/ 00093 /* Group: Cannel */ 00094 00095 /** video_input_channel */ 00096 vdc5_channel_t video_input_channel; 00097 00098 /** video_input_select */ 00099 vdec_adc_vinsel_t video_input_select; 00100 00101 /** is_data_control */ 00102 bool_t is_data_control; 00103 00104 /** data_control_ID */ 00105 vdc5_layer_id_t data_control_ID; 00106 00107 00108 /*-----------------------------------------------------------*/ 00109 /* Group: Interrupt */ 00110 00111 /** is_vsync_interrupt_registered */ 00112 bool_t is_vsync_interrupt_registered[3]; 00113 00114 /** interrupt_for_VDC5 */ 00115 vdc5_int_t interrupt_for_VDC5; 00116 00117 /** interrupt_ID */ 00118 bsp_int_src_t interrupt_ID; 00119 00120 /** interrupt_vector */ 00121 #if INTERRUPT_FUNCTION_TYPE == INTERRUPT_FUNCTION_TYPE_OS_RTX 00122 bsp_int_cb_t interrupt_vector; 00123 #endif 00124 00125 00126 /*-----------------------------------------------------------*/ 00127 /* Group: Buffer */ 00128 00129 /** frame_buffer */ 00130 frame_buffer_t frame_buffer; 00131 00132 /** captured_buffer_index */ 00133 int_fast32_t captured_buffer_index; 00134 00135 /** captured_count */ 00136 int_fast32_t captured_count; 00137 00138 /** captured_async */ 00139 r_ospl_async_t *captured_async; 00140 }; 00141 00142 00143 /** 00144 * @struct video_input_config_t 00145 * @brief Parameters of <R_VIDEO_INPUT_Initialize> 00146 */ 00147 typedef struct st_video_input_config_t video_input_config_t; 00148 struct st_video_input_config_t { 00149 00150 /** 0 | VIDEO_INPUT_CONFIG_T_layer_ID | ... */ 00151 bit_flags_fast32_t flags; 00152 00153 00154 /** 0=ch0.VIN1, 1=ch1.VIN1, 2=ch0.VIN2, 3=ch1.VIN2 */ 00155 int_fast32_t video_input_channel_num; 00156 00157 /** 0, 1 or VIDEO_INPUT_NOT_DISPLAY */ 00158 int_fast32_t display_channel_num; 00159 00160 /** -1 or VIDEO_INPUT_NOT_DISPLAY */ 00161 int_fast32_t display_layer_num; 00162 00163 /** frame_buffer */ 00164 frame_buffer_t *frame_buffer; 00165 00166 /** captured_async */ 00167 r_ospl_async_t *captured_async; 00168 }; 00169 /* video_input_config_t::flags */ 00170 #define VIDEO_INPUT_CONFIG_T_VIDEO_INPUT_CHANNEL_NUM 0x0001 00171 #define VIDEO_INPUT_CONFIG_T_DISPLAY_CHANNEL_NUM 0x0002 00172 #define VIDEO_INPUT_CONFIG_T_DISPLAY_LAYER_NUM 0x0004 00173 #define VIDEO_INPUT_CONFIG_T_FRAME_BUFFER 0x0008 00174 #define VIDEO_INPUT_CONFIG_T_CAPTURED_ASYNC 0x0010 00175 00176 00177 /****************************************************************************** 00178 Variable Externs 00179 ******************************************************************************/ 00180 00181 /****************************************************************************** 00182 Functions Prototypes 00183 ******************************************************************************/ 00184 /* In "video_input.h" */ 00185 00186 #ifdef __cplusplus 00187 } /* extern "C" */ 00188 #endif /* __cplusplus */ 00189 00190 #endif
Generated on Tue Jul 12 2022 11:15:05 by
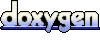