Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_jcu_api.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_jcu_api.h 00025 * @brief JCU (JPEG hardware) driver API. Main Header. 00026 * 00027 * $Module: JCU $ $PublicVersion: 1.00 $ (=JCU_VERSION) 00028 * $Rev: 38 $ 00029 * $Date:: 2014-03-18 16:14:45 +0900#$ 00030 */ 00031 00032 #ifndef JCU_API_H_ 00033 #define JCU_API_H_ 00034 00035 /****************************************************************************** 00036 Includes <System Includes> , "Project Includes" 00037 ******************************************************************************/ 00038 #include <stddef.h> 00039 #include "r_jcu_user.h" 00040 #include "r_ospl.h" 00041 #ifdef __cplusplus 00042 extern "C" 00043 { 00044 #endif /* __cplusplus */ 00045 00046 /****************************************************************************** 00047 Typedef definitions 00048 ******************************************************************************/ 00049 00050 /** 00051 * @enum jcu_status_information_t 00052 * @brief Status 00053 * 00054 * - JCU_STATUS_UNDEF - 0x00, Undefined 00055 * - JCU_STATUS_INIT - 0x01, Initialized 00056 * - JCU_STATUS_SELECTED - 0x02, Codec is selected 00057 * - JCU_STATUS_READY - 0x08, Ready 00058 * - JCU_STATUS_RUN - 0x10, Run 00059 * - JCU_STATUS_INTERRUPTING - 0x40, Interrupting 00060 * - JCU_STATUS_INTERRUPTED - 0x80, Interrupted 00061 */ 00062 typedef enum { 00063 JCU_STATUS_UNDEF = 0x00, 00064 JCU_STATUS_INIT = 0x01, 00065 JCU_STATUS_SELECTED = 0x02, 00066 JCU_STATUS_READY = 0x08, 00067 JCU_STATUS_RUN = 0x10, 00068 JCU_STATUS_INTERRUPTING = 0x40, 00069 JCU_STATUS_INTERRUPTED = 0x80 00070 } jcu_status_information_t; 00071 00072 00073 /** 00074 * @struct jcu_async_status_t 00075 * @brief Status of asynchronize 00076 */ 00077 typedef struct st_jcu_async_status_t jcu_async_status_t; 00078 struct st_jcu_async_status_t { 00079 jcu_status_information_t Status; 00080 bit_flags_fast32_t SubStatusFlags; 00081 bool_t IsPaused; 00082 bool_t IsEnabledInterrupt; 00083 r_ospl_flag32_t InterruptEnables; 00084 r_ospl_flag32_t InterruptFlags; 00085 r_ospl_flag32_t CancelFlags; /*<r_ospl_cancel_flag_t>*/ 00086 }; 00087 00088 00089 /* Section: Global */ 00090 /** 00091 * @enum SubStatusFlags 00092 * @brief Sub Status 00093 * 00094 * - JCU_SUB_INFOMATION_READY - 0x00000008 00095 * - JCU_SUB_DECODE_OUTPUT_PAUSE - 0x00000100 00096 * - JCU_SUB_DECODE_INPUT_PAUSE - 0x00000200 00097 * - JCU_SUB_ENCODE_OUTPUT_PAUSE - 0x00001000 00098 * - JCU_SUB_ENCODE_INPUT_PAUSE - 0x00002000 00099 */ 00100 #define JCU_SUB_INFOMATION_READY 0x00000008 00101 #define JCU_SUB_DECODE_OUTPUT_PAUSE 0x00000100 00102 #define JCU_SUB_DECODE_INPUT_PAUSE 0x00000200 00103 #define JCU_SUB_ENCODE_OUTPUT_PAUSE 0x00001000 00104 #define JCU_SUB_ENCODE_INPUT_PAUSE 0x00002000 00105 00106 00107 /** 00108 * @def jcu_interrupt_line_t 00109 * @brief Interrupt Lines 00110 * 00111 * - JCU_INTERRUPT_LINE_JEDI - 1 00112 * - JCU_INTERRUPT_LINE_JDTI - 2 00113 * - JCU_INTERRUPT_LINE_ALL - 1 | 2 00114 */ 00115 typedef uint_fast32_t jcu_interrupt_line_t; 00116 #define JCU_INTERRUPT_LINE_JEDI 0x00000001u 00117 #define JCU_INTERRUPT_LINE_JDTI 0x00000002u 00118 #define JCU_INTERRUPT_LINE_ALL \ 00119 ( JCU_INTERRUPT_LINE_JEDI | JCU_INTERRUPT_LINE_JDTI ) 00120 00121 00122 /** 00123 * @typedef jcu_interrupt_lines_t 00124 * @brief Bit flags of <jcu_interrupt_line_t> 00125 */ 00126 typedef bit_flags_fast32_t /*<jcu_interrupt_line_t>*/ jcu_interrupt_lines_t; 00127 00128 00129 /****************************************************************************** 00130 Macro definitions 00131 ******************************************************************************/ 00132 00133 00134 /** 00135 * @def JCU_VERSION 00136 * @brief VERSION 00137 * @par Parameters 00138 * None 00139 * @return None. 00140 */ 00141 #define JCU_VERSION 100 00142 00143 00144 /** 00145 * @def JCU_VERSION_STRING 00146 * @brief String of <JCU_VERSION> 00147 * @par Parameters 00148 * None 00149 * @return None. 00150 */ 00151 #define JCU_VERSION_STRING "1.00" 00152 00153 00154 /** 00155 * @def JCU_INT_TYPE_NUM 00156 * @brief The number of the interrupt source 00157 * @par Parameters 00158 * None 00159 * @return None. 00160 */ 00161 #define JCU_INT_TYPE_NUM 0x0009 00162 00163 00164 /** 00165 * @def JCU_COLOR_ELEMENT_NUM 00166 * @brief Y,Cb and Cr 00167 * @par Parameters 00168 * None 00169 * @return None. 00170 */ 00171 #define JCU_COLOR_ELEMENT_NUM 3 00172 00173 00174 /****************************************************************************** 00175 Struct & Enum definitions 00176 ******************************************************************************/ 00177 00178 /** 00179 * @enum jcu_errorcode_t 00180 * @brief Error code of JCU driver 00181 * 00182 * - JCU_ERROR_OK - 0 00183 * - JCU_ERROR_PARAM - 0x4501 00184 * - JCU_ERROR_STATUS - 0x4502 00185 * - JCU_ERROR_CODEC_TYPE - 0x4503 00186 * - JCU_ERROR_LIMITATION - 0x4504 00187 */ 00188 enum { E_CATEGORY_JCU_ERR = 0x4500 }; /* Offset of JCU error code */ 00189 typedef errnum_t jcu_errorcode_t; 00190 enum { 00191 JCU_ERROR_OK = 0x0000, 00192 JCU_ERROR_PARAM = 0x4501, 00193 JCU_ERROR_STATUS = 0x4502, 00194 JCU_ERROR_CODEC_TYPE = 0x4503, 00195 JCU_ERROR_LIMITATION = 0x4504 00196 }; 00197 00198 00199 /** 00200 * @enum jcu_detail_error_t 00201 * @brief Kind of detailed error for decoding 00202 * 00203 * - JCU_JCDERR_OK - 0x0000 00204 * - JCU_JCDERR_SOI_NOT_FOUND - 0x4521 00205 * - JCU_JCDERR_INVALID_SOF - 0x4522 00206 * - JCU_JCDERR_UNPROVIDED_SOF - 0x4523 00207 * - JCU_JCDERR_SOF_ACCURACY - 0x4524 00208 * - JCU_JCDERR_DQT_ACCURACY - 0x4525 00209 * - JCU_JCDERR_COMPONENT_1 - 0x4526 00210 * - JCU_JCDERR_COMPONENT_2 - 0x4527 00211 * - JCU_JCDERR_NO_SOF0_DQT_DHT - 0x4528 00212 * - JCU_JCDERR_SOS_NOT_FOUND - 0x4529 00213 * - JCU_JCDERR_EOI_NOT_FOUND - 0x452A 00214 * - JCU_JCDERR_RESTART_INTERVAL - 0x452B 00215 * - JCU_JCDERR_IMAGE_SIZE - 0x452C 00216 * - JCU_JCDERR_LAST_MCU_DATA - 0x452D 00217 * - JCU_JCDERR_BLOCK_DATA - 0x452E 00218 */ 00219 enum { E_CATEGORY_JCU_JCDERR = 0x4520 }; /* Offset of JCU detail error code */ 00220 typedef errnum_t jcu_detail_error_t; 00221 enum { 00222 JCU_JCDERR_OK = 0x0000, 00223 JCU_JCDERR_SOI_NOT_FOUND = 0x4521, 00224 JCU_JCDERR_INVALID_SOF = 0x4522, 00225 JCU_JCDERR_UNPROVIDED_SOF = 0x4523, 00226 JCU_JCDERR_SOF_ACCURACY = 0x4524, 00227 JCU_JCDERR_DQT_ACCURACY = 0x4525, 00228 JCU_JCDERR_COMPONENT_1 = 0x4526, 00229 JCU_JCDERR_COMPONENT_2 = 0x4527, 00230 JCU_JCDERR_NO_SOF0_DQT_DHT = 0x4528, 00231 JCU_JCDERR_SOS_NOT_FOUND = 0x4529, 00232 JCU_JCDERR_EOI_NOT_FOUND = 0x452A, 00233 JCU_JCDERR_RESTART_INTERVAL = 0x452B, 00234 JCU_JCDERR_IMAGE_SIZE = 0x452C, 00235 JCU_JCDERR_LAST_MCU_DATA = 0x452D, 00236 JCU_JCDERR_BLOCK_DATA = 0x452E 00237 }; 00238 00239 00240 /** 00241 * @enum jcu_codec_t 00242 * @brief The type of codec 00243 * 00244 * - JCU_ENCODE - 0 00245 * - JCU_DECODE - 1 00246 */ 00247 typedef enum { 00248 JCU_ENCODE = 0, 00249 JCU_DECODE = 1 00250 } jcu_codec_t; 00251 00252 00253 /** 00254 * @struct jcu_count_mode_param_t 00255 * @brief Buffer settings for separating decode or encode 00256 */ 00257 typedef struct { 00258 struct { 00259 /** true: It uses the count mode process. */ 00260 bool_t isEnable; 00261 00262 /** Address is changed when processing is stopped. */ 00263 bool_t isInitAddress; 00264 00265 /** This is enable when isInitAddrss is true. */ 00266 uint32_t *restartAddress; 00267 00268 /** Encode:8line Decode:8byte. */ 00269 uint32_t dataCount; 00270 00271 } inputBuffer; 00272 00273 struct { 00274 /** true: It uses the count mode process. */ 00275 bool_t isEnable; 00276 00277 /** Address is changed when processing is stopped. */ 00278 bool_t isInitAddress; /* */ 00279 00280 /** This is enable when isInitAddress is true. */ 00281 uint32_t *restartAddress; /* */ 00282 00283 /** Encode:8byte Decode:8line. */ 00284 uint32_t dataCount; 00285 00286 } outputBuffer; 00287 } jcu_count_mode_param_t; 00288 00289 00290 /* Section: Global */ 00291 /** 00292 * @enum jcu_continue_type_t 00293 * @brief Type of continue buffer 00294 * 00295 * - JCU_INPUT_BUFFER - 0 00296 * - JCU_OUTPUT_BUFFER - 1 00297 * - JCU_IMAGE_INFO - 2 00298 */ 00299 typedef enum { 00300 JCU_INPUT_BUFFER, 00301 JCU_OUTPUT_BUFFER, 00302 JCU_IMAGE_INFO 00303 } jcu_continue_type_t; 00304 00305 00306 /** 00307 * @def jcu_int_detail_error_t 00308 * @brief Bit flags of detail error 00309 * 00310 * - JCU_INT_ERROR_RESTART_INTERVAL_DATA - INT7_MASK 00311 * - JCU_INT_ERROR_SEGMENT_TOTAL_DATA - INT6_MASK 00312 * - JCU_INT_ERROR_MCU_BLOCK_DATA - INT5_MASK 00313 * - JCU_INT_ERROR_ALL - e.g. for mask 00314 */ 00315 typedef uint_fast32_t jcu_int_detail_error_t; 00316 #define JCU_INT_ERROR_RESTART_INTERVAL_DATA (0x80u) 00317 #define JCU_INT_ERROR_SEGMENT_TOTAL_DATA (0x40u) 00318 #define JCU_INT_ERROR_MCU_BLOCK_DATA (0x20u) 00319 #define JCU_INT_ERROR_ALL \ 00320 ( JCU_INT_ERROR_RESTART_INTERVAL_DATA | JCU_INT_ERROR_SEGMENT_TOTAL_DATA | \ 00321 JCU_INT_ERROR_MCU_BLOCK_DATA ) 00322 00323 00324 /** 00325 * @typedef jcu_int_detail_errors_t 00326 * @brief Bit flags of detail error 00327 */ 00328 typedef bit_flags_fast32_t /*<jcu_int_detail_error_t>*/ jcu_int_detail_errors_t; 00329 00330 00331 /** 00332 * @enum jcu_swap_t 00333 * @brief SampleEnum 00334 * 00335 * - SymbolA - 0, NULL 00336 * - SymbolB - 1 00337 */ 00338 typedef int_t jcu_swap_t; 00339 #define JCU_SWAP_NONE (0x00) 00340 #define JCU_SWAP_BYTE (0x01) 00341 #define JCU_SWAP_WORD (0x02) 00342 #define JCU_SWAP_WORD_AND_BYTE (0x03) 00343 #define JCU_SWAP_LONG_WORD (0x04) 00344 #define JCU_SWAP_LONG_WORD_AND_BYTE (0x05) 00345 #define JCU_SWAP_LONG_WORD_AND_WORD (0x06) 00346 #define JCU_SWAP_LONG_WORD_AND_WORD_AND_BYTE (0x07) 00347 00348 00349 /** 00350 * @struct jcu_buffer_t 00351 * @brief Buffer settings 00352 */ 00353 typedef struct { 00354 jcu_swap_t swapSetting; 00355 uint32_t *address; 00356 } jcu_buffer_t; 00357 00358 00359 /** 00360 * @struct jcu_buffer_param_t 00361 * @brief Buffer parameter for input and output 00362 */ 00363 typedef struct { 00364 jcu_buffer_t source; 00365 jcu_buffer_t destination; 00366 int16_t lineOffset; 00367 } jcu_buffer_param_t; 00368 00369 00370 /* Section: Global */ 00371 /** 00372 * @enum jcu_sub_sampling_t 00373 * @brief Sub sampling settings for decoding 00374 * 00375 * - JCU_SUB_SAMPLING_1_1 - 0x00 = 1/1 00376 * - JCU_SUB_SAMPLING_1_2 - 0x01 = 1/2 00377 * - JCU_SUB_SAMPLING_1_4 - 0x02 = 1/4 00378 * - JCU_SUB_SAMPLING_1_8 - 0x03 = 1/8 00379 */ 00380 typedef enum { 00381 JCU_SUB_SAMPLING_1_1 = 0x00, 00382 JCU_SUB_SAMPLING_1_2 = 0x01, 00383 JCU_SUB_SAMPLING_1_4 = 0x02, 00384 JCU_SUB_SAMPLING_1_8 = 0x03 00385 } jcu_sub_sampling_t; 00386 00387 00388 /** 00389 * @enum jcu_decode_format_t 00390 * @brief Kind of pixel format can process the decoding 00391 * 00392 * - JCU_OUTPUT_YCbCr422 - 0x00 00393 * - JCU_OUTPUT_ARGB8888 - 0x01 00394 * - JCU_OUTPUT_RGB565 - 0x02 00395 */ 00396 typedef enum { 00397 JCU_OUTPUT_YCbCr422 = 0x00, 00398 JCU_OUTPUT_ARGB8888 = 0x01, 00399 JCU_OUTPUT_RGB565 = 0x02 00400 } jcu_decode_format_t; 00401 00402 00403 /** 00404 * @enum jcu_cbcr_offset_t 00405 * @brief Cb/Cr offset 00406 * 00407 * - JCU_CBCR_OFFSET_0 - 0 = No offset 00408 * - JCU_CBCR_OFFSET_128 - 1 = +128 offset 00409 */ 00410 typedef enum { 00411 JCU_CBCR_OFFSET_0 = 0, 00412 JCU_CBCR_OFFSET_128 = 1 00413 } jcu_cbcr_offset_t; 00414 00415 00416 /** 00417 * @enum jcu_jpeg_format_t 00418 * @brief Kind of pixel format for the jpeg file format when decoding 00419 * 00420 * - JCU_JPEG_YCbCr444 - 0 00421 * - JCU_JPEG_YCbCr422 - 1 00422 * - JCU_JPEG_YCbCr420 - 2 00423 * - JCU_JPEG_YCbCr411 - 6 00424 */ 00425 typedef enum { 00426 JCU_JPEG_YCbCr444 = 0x00, 00427 JCU_JPEG_YCbCr422 = 0x01, 00428 JCU_JPEG_YCbCr420 = 0x02, 00429 JCU_JPEG_YCbCr411 = 0x06 00430 } jcu_jpeg_format_t; 00431 00432 00433 /** 00434 * @struct jcu_image_info_t 00435 * @brief Image information data store variable 00436 */ 00437 typedef struct { 00438 uint32_t width; 00439 uint32_t height; 00440 jcu_jpeg_format_t encodedFormat; 00441 } jcu_image_info_t; 00442 00443 00444 /* Section: Global */ 00445 /** 00446 * @enum jcu_table_no_t 00447 * @brief The index of the table 00448 * 00449 * - JCU_TABLE_NO_0 - 0 00450 * - JCU_TABLE_NO_1 - 1 00451 * - JCU_TABLE_NO_2 - 2 00452 * - JCU_TABLE_NO_3 - 3 00453 */ 00454 typedef enum { 00455 JCU_TABLE_NO_0 = 0, 00456 JCU_TABLE_NO_1 = 1, 00457 JCU_TABLE_NO_2 = 2, 00458 JCU_TABLE_NO_3 = 3 00459 } jcu_table_no_t; 00460 00461 00462 /** 00463 * @enum jcu_huff_t 00464 * @brief Kind of Huffman table 00465 * 00466 * - JCU_HUFFMAN_AC - 0 00467 * - JCU_HUFFMAN_DC - 1 00468 */ 00469 typedef enum { 00470 JCU_HUFFMAN_AC, 00471 JCU_HUFFMAN_DC 00472 } jcu_huff_t; 00473 00474 00475 /** 00476 * @enum jcu_color_element_t 00477 * @brief Kind of color data for using the encoder parameter 00478 * 00479 * - JCU_ELEMENT_Y - 0 00480 * - JCU_ELEMENT_Cb - 1 00481 * - JCU_ELEMENT_Cr - 2 00482 */ 00483 typedef enum { 00484 JCU_ELEMENT_Y, 00485 JCU_ELEMENT_Cb, 00486 JCU_ELEMENT_Cr 00487 } jcu_color_element_t; 00488 00489 00490 /** 00491 * @struct jcu_decode_param_t 00492 * @brief Setting parameter for the Decoding 00493 */ 00494 typedef struct { 00495 jcu_sub_sampling_t verticalSubSampling; 00496 jcu_sub_sampling_t horizontalSubSampling; 00497 jcu_decode_format_t decodeFormat; 00498 jcu_cbcr_offset_t outputCbCrOffset; 00499 uint8_t alpha; 00500 } jcu_decode_param_t; 00501 00502 00503 /** 00504 * @struct jcu_encode_param_t 00505 * @brief Setting parameter for the Encoding 00506 */ 00507 typedef struct { 00508 jcu_jpeg_format_t encodeFormat; 00509 int32_t QuantizationTable[JCU_COLOR_ELEMENT_NUM]; 00510 int32_t HuffmanTable[JCU_COLOR_ELEMENT_NUM]; 00511 uint32_t DRI_value; 00512 uint32_t width; 00513 uint32_t height; 00514 jcu_cbcr_offset_t inputCbCrOffset; 00515 } jcu_encode_param_t; 00516 00517 00518 /* Section: Global */ 00519 /** 00520 * @enum jcu_codec_status_t 00521 * @brief Codec type 00522 * 00523 * - JCU_CODEC_NOT_SELECTED - -1 00524 * - JCU_STATUS_ENCODE - 0 00525 * - JCU_STATUS_DECODE - 1 00526 */ 00527 typedef enum { 00528 JCU_CODEC_NOT_SELECTED = -1, 00529 JCU_STATUS_ENCODE = 0, 00530 JCU_STATUS_DECODE = 1 00531 } jcu_codec_status_t; 00532 00533 00534 /****************************************************************************** 00535 Variable Externs 00536 ******************************************************************************/ 00537 00538 /****************************************************************************** 00539 Imported global variables and functions (from other files) 00540 ******************************************************************************/ 00541 00542 /****************************************************************************** 00543 Functions Prototypes 00544 ******************************************************************************/ 00545 jcu_errorcode_t R_JCU_Initialize( void *const NullConfig ); 00546 jcu_errorcode_t R_JCU_Terminate(void); 00547 jcu_errorcode_t R_JCU_TerminateAsync( r_ospl_async_t *const async ); 00548 jcu_errorcode_t R_JCU_SelectCodec(const jcu_codec_t codec); 00549 jcu_errorcode_t R_JCU_Start(void); 00550 jcu_errorcode_t R_JCU_StartAsync( r_ospl_async_t *const async ); 00551 jcu_errorcode_t R_JCU_SetCountMode(const jcu_count_mode_param_t *const buffer); 00552 jcu_errorcode_t R_JCU_Continue(const jcu_continue_type_t type); 00553 jcu_errorcode_t R_JCU_ContinueAsync(const jcu_continue_type_t type, r_ospl_async_t *const async); 00554 void R_JCU_GetAsyncStatus(const jcu_async_status_t **const out_Status); 00555 jcu_errorcode_t R_JCU_SetDecodeParam(const jcu_decode_param_t *const decode, const jcu_buffer_param_t *const buffer); 00556 jcu_errorcode_t R_JCU_SetPauseForImageInfo(const bool_t is_pause); 00557 jcu_errorcode_t R_JCU_GetImageInfo(jcu_image_info_t *const buffer); 00558 jcu_errorcode_t R_JCU_SetErrorFilter(jcu_int_detail_errors_t filter); 00559 jcu_errorcode_t R_JCU_SetQuantizationTable(const jcu_table_no_t tableNo, const uint8_t *const table); 00560 jcu_errorcode_t R_JCU_SetHuffmanTable(const jcu_table_no_t tableNo, const jcu_huff_t type, const uint8_t *const table); 00561 jcu_errorcode_t R_JCU_SetEncodeParam(const jcu_encode_param_t *const encode, const jcu_buffer_param_t *const buffer); 00562 jcu_errorcode_t R_JCU_GetEncodedSize(size_t *const out_Size); 00563 jcu_errorcode_t R_JCU_Set2ndCacheAttribute( r_ospl_axi_cache_attribute_t const read_cache_attribute, 00564 r_ospl_axi_cache_attribute_t const write_cache_attribute ); 00565 00566 #ifndef R_OSPL_NDEBUG 00567 void R_JCU_PrintRegisters(void); 00568 #endif 00569 00570 /* For interrupt callback */ 00571 errnum_t R_JCU_OnInterrupting( const r_ospl_interrupt_t *const InterruptSource ); 00572 errnum_t R_JCU_OnInterrupted(void); 00573 #ifdef __cplusplus 00574 } 00575 #endif /* __cplusplus */ 00576 00577 #endif /* #define JCU_API_H_ */
Generated on Tue Jul 12 2022 11:15:02 by
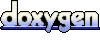