Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
jcu_para.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /******************************************************************************* 00024 * $FileName: jcu_para.c $ 00025 * $Module: JCU $ $PublicVersion: 1.00 $ (=JCU_VERSION) 00026 * $Rev: 38 $ 00027 * $Date:: 2014-03-18 16:14:45 +0900#$ 00028 * Description : JCU driver checking parameter 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include <string.h> 00035 #include "r_typedefs.h" 00036 #include "r_ospl.h" 00037 #include "r_jcu_api.h" 00038 #include "r_jcu_local.h" 00039 #include "r_jcu_user.h" 00040 #include "iodefine.h" 00041 00042 #ifdef JCU_PARAMETER_CHECK 00043 00044 /****************************************************************************** 00045 Typedef definitions 00046 ******************************************************************************/ 00047 00048 /****************************************************************************** 00049 Macro definitions 00050 ******************************************************************************/ 00051 #define MASK_LOW_3BIT (0x7u) 00052 #define MOD_8 (0x8u) 00053 #define MOD_16 (0x10u) 00054 00055 /****************************************************************************** 00056 Imported global variables and functions (from other files) 00057 ******************************************************************************/ 00058 00059 /****************************************************************************** 00060 Exported global variables and functions (to be accessed by other files) 00061 ******************************************************************************/ 00062 00063 /****************************************************************************** 00064 Private global variables and functions 00065 ******************************************************************************/ 00066 00067 /**************************************************************************//** 00068 * Function Name : [JCU_ParaCheckSelectCodec] 00069 * @brief SelectCodec api's parameter checking 00070 * @param [in] codec codec type 00071 * @retval jcu_errorcode_t 00072 *****************************************************************************/ 00073 jcu_errorcode_t JCU_ParaCheckSelectCodec( 00074 const jcu_codec_t codec) 00075 { 00076 jcu_errorcode_t returnValue; 00077 00078 IF_DS ((codec != JCU_ENCODE) 00079 && (codec != JCU_DECODE)) { 00080 returnValue = JCU_ERROR_PARAM; 00081 } 00082 else { 00083 returnValue = JCU_ERROR_OK; 00084 } /* end if */ 00085 00086 return (returnValue); 00087 } 00088 00089 /**************************************************************************//** 00090 * Function Name : [JCU_ParaCheckStart] 00091 * @brief Start api's parameter checking 00092 * @param [in] codec codec type 00093 * @retval jcu_errorcode_t 00094 *****************************************************************************/ 00095 jcu_errorcode_t JCU_ParaCheckStart( 00096 void) 00097 { 00098 jcu_errorcode_t returnValue; 00099 00100 /* If jcu already start, it cannot update this bit (this is limitation of hardware) */ 00101 IF ( (uint32_t)R_GET_REG_BIT_FIELD( JCU.JCCMD, JSRT ) == REG_F_SET ) { 00102 returnValue = JCU_ERROR_STATUS; 00103 } 00104 else { 00105 returnValue = JCU_ERROR_OK; 00106 } /* end if */ 00107 00108 return (returnValue); 00109 } 00110 00111 /**************************************************************************//** 00112 * Function Name : [JCU_ParaCheckSetCountMode] 00113 * @brief parameter check for the SetCountMode 00114 * @param [in] buffer the parameter for the Count Mode 00115 * @retval jcu_errorcode_t 00116 *****************************************************************************/ 00117 jcu_errorcode_t JCU_ParaCheckSetCountMode( 00118 const jcu_count_mode_param_t *const buffer) 00119 { 00120 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00121 00122 IF (buffer == NULL) { 00123 returnValue = JCU_ERROR_PARAM; 00124 goto fin; 00125 } /* end if */ 00126 00127 IF ((buffer->inputBuffer.isEnable != false) && (buffer->outputBuffer.isEnable != false)) { 00128 returnValue = JCU_ERROR_PARAM; 00129 goto fin; 00130 } 00131 00132 if (buffer->inputBuffer.isEnable != false) { 00133 /* When initAddress is true, restartAddress has to set the address */ 00134 if (buffer->inputBuffer.isInitAddress != false) { 00135 IF (buffer->inputBuffer.restartAddress == NULL) { 00136 returnValue = JCU_ERROR_PARAM; 00137 goto fin; 00138 } /* end if */ 00139 } /* end if */ 00140 00141 /* Datasize(JDATAS/LINES bit) have to 8byte alignment */ 00142 IF ((buffer->inputBuffer.dataCount & MASK_LOW_3BIT) != 0u) { 00143 returnValue = JCU_ERROR_PARAM; 00144 goto fin; 00145 } /* end if */ 00146 00147 /* Datasize have to bigger than 0 */ 00148 IF (buffer->inputBuffer.dataCount == 0u) { 00149 returnValue = JCU_ERROR_PARAM; 00150 goto fin; 00151 } /* end if */ 00152 00153 } /* end if */ 00154 00155 if (buffer->outputBuffer.isEnable != false) { 00156 /* When initAddress is true, restartAddress has to set the address */ 00157 if (buffer->outputBuffer.isInitAddress != false) { 00158 IF (buffer->outputBuffer.restartAddress == NULL) { 00159 returnValue = JCU_ERROR_PARAM; 00160 goto fin; 00161 } /* end if */ 00162 } /* end if */ 00163 00164 /* Datasize(JDATAS/LINES bit) have to 8byte alignment */ 00165 IF ((buffer->outputBuffer.dataCount & MASK_LOW_3BIT) != 0u) { 00166 returnValue = JCU_ERROR_PARAM; 00167 goto fin; 00168 } /* end if */ 00169 00170 /* Datasize have to bigger than 0 */ 00171 IF (buffer->outputBuffer.dataCount == 0u) { 00172 returnValue = JCU_ERROR_PARAM; 00173 goto fin; 00174 } /* end if */ 00175 00176 } /* end if */ 00177 fin: 00178 return (returnValue); 00179 } 00180 00181 /**************************************************************************//** 00182 * Function Name : [JCU_ParaCheckSetDecodeParam] 00183 * @brief SetDecodeParam api's parameter checking 00184 * @param [in] buffer input and output buffer settings 00185 * @param [in] interruptKind tye type of interrupt that use in this system 00186 * @retval jcu_errorcode_t 00187 *****************************************************************************/ 00188 jcu_errorcode_t JCU_ParaCheckSetDecodeParam( 00189 const jcu_decode_param_t *const decode, 00190 const jcu_buffer_param_t *const buffer) 00191 { 00192 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00193 00194 IF ((decode == NULL) || (buffer == NULL)) { 00195 returnValue = JCU_ERROR_PARAM; 00196 goto fin; 00197 } /* end if */ 00198 00199 /* NULL check for the address data */ 00200 IF ((buffer->source.address == NULL) 00201 || (buffer->destination.address == NULL)) { 00202 returnValue = JCU_ERROR_PARAM; 00203 goto fin; 00204 } /* end if */ 00205 00206 /* Check for the address alignment */ 00207 /* ->MISRA 11.3 */ /* ->SEC R2.7.1 */ 00208 IF ((((uint32_t) (buffer->source.address) % MOD_8) != 0u) || 00209 (((uint32_t) (buffer->destination.address) % MOD_8) != 0u)) 00210 /* <-MISRA 11.3 */ { /* <-SEC R2.7.1 */ 00211 returnValue = JCU_ERROR_PARAM; 00212 goto fin; 00213 } /* end if */ 00214 00215 /*Check for Cb/Cr offset */ 00216 if(decode->decodeFormat == JCU_OUTPUT_YCbCr422) { 00217 IF (((decode->outputCbCrOffset) != JCU_CBCR_OFFSET_0) && ((decode->outputCbCrOffset) != JCU_CBCR_OFFSET_128)) { 00218 returnValue = JCU_ERROR_PARAM; 00219 } /* end if */ 00220 } else { 00221 IF ((decode->outputCbCrOffset) != JCU_CBCR_OFFSET_0) { 00222 returnValue = JCU_ERROR_PARAM; 00223 } /* end if */ 00224 } /* end if */ 00225 fin: 00226 return (returnValue); 00227 } 00228 00229 /**************************************************************************//** 00230 * Function Name : [JCU_ParaCheckGetImageInfo] 00231 * @brief GetImageInfo api's parameter checking 00232 * @param [in] buffer buffer address that set the image information 00233 * @retval jcu_errorcode_t 00234 *****************************************************************************/ 00235 jcu_errorcode_t JCU_ParaCheckGetImageInfo( 00236 const jcu_image_info_t *const buffer) 00237 { 00238 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00239 00240 IF (buffer == NULL) { 00241 returnValue = JCU_ERROR_PARAM; 00242 } /* end if */ 00243 00244 return (returnValue); 00245 } 00246 00247 /**************************************************************************//** 00248 * Function Name : [JCU_ParaCheckSetQuantizationTbl] 00249 * @brief SetQuantizationTable api's parameter checking 00250 * @param [in] tableNo the table number that set the parameter 00251 * @retval jcu_errorcode_t 00252 *****************************************************************************/ 00253 jcu_errorcode_t JCU_ParaCheckSetQuantizationTbl( 00254 const jcu_table_no_t tableNo) 00255 { 00256 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00257 00258 /* ->MISRA 13.7 : For fail safe. */ 00259 IF_DS (tableNo > JCU_TABLE_NO_3) 00260 /* <-MISRA 13.7 */ 00261 /* ->MISRA 14.1 ->QAC 3201 : For fail safe. */ 00262 { 00263 returnValue = JCU_ERROR_PARAM; 00264 } /* end if */ 00265 /* <-MISRA 14.1 <-QAC 3201 */ 00266 00267 return (returnValue); 00268 } 00269 00270 /**************************************************************************//** 00271 * Function Name : [JCU_ParaCheckSetHuffmanTable] 00272 * @brief SetHuffmanTable api's parameter checking 00273 * @param [in] tableNo the table number that set the parameter 00274 * @param [in] type the type which table is set(AC or DC) 00275 * @retval jcu_errorcode_t 00276 *****************************************************************************/ 00277 jcu_errorcode_t JCU_ParaCheckSetHuffmanTable( 00278 const jcu_table_no_t tableNo, 00279 const jcu_huff_t type) 00280 { 00281 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00282 00283 /* ->MISRA 13.7 : For fail safe. */ 00284 IF (tableNo > JCU_TABLE_NO_1) 00285 /* <-MISRA 13.7 */ 00286 { 00287 returnValue = JCU_ERROR_PARAM; 00288 goto fin; 00289 } /* end if */ 00290 00291 /* ->MISRA 13.7 : For fail safe. */ 00292 IF_DS ((type != JCU_HUFFMAN_AC) 00293 && (type != JCU_HUFFMAN_DC)) 00294 /* <-MISRA 13.7 */ 00295 /* ->MISRA 14.1 ->QAC 3201 : For fail safe. */ 00296 { 00297 returnValue = JCU_ERROR_PARAM; 00298 } /* end if */ 00299 /* <-MISRA 14.1 <-QAC 3201 */ 00300 fin: 00301 return (returnValue); 00302 } 00303 00304 /**************************************************************************//** 00305 * Function Name : [JCU_ParaCheckEncodeParam] 00306 * @brief api's parameter checking 00307 * @param [in] 00308 * @retval jcu_errorcode_t 00309 *****************************************************************************/ 00310 jcu_errorcode_t JCU_ParaCheckEncodeParam( 00311 const jcu_encode_param_t *const encode, 00312 const jcu_buffer_param_t *const buffer) 00313 { 00314 jcu_errorcode_t returnValue = JCU_ERROR_OK; 00315 00316 IF ((encode == NULL) || (buffer == NULL)) { 00317 returnValue = JCU_ERROR_PARAM; 00318 goto fin; 00319 } /* end if */ 00320 00321 /* NULL check for the address data */ 00322 IF ((buffer->source.address == NULL) 00323 || (buffer->destination.address == NULL)) { 00324 returnValue = JCU_ERROR_PARAM; 00325 goto fin; 00326 } /* end if */ 00327 00328 00329 if (encode->encodeFormat == JCU_JPEG_YCbCr422) { 00330 /* Check the width of the image data */ 00331 IF ((encode->width % MOD_16) != 0u) { 00332 returnValue = JCU_ERROR_PARAM; 00333 goto fin; 00334 } /* end if */ 00335 00336 /* Check the height of the image data */ 00337 IF ((encode->height % MOD_8) != 0u) { 00338 returnValue = JCU_ERROR_PARAM; 00339 goto fin; 00340 } /* end if */ 00341 } else { 00342 returnValue = JCU_ERROR_PARAM; 00343 ASSERT_D( false, R_NOOP() ); 00344 goto fin; 00345 } /* end if */ 00346 00347 /*Check for Cb/Cr offset */ 00348 IF (((encode->inputCbCrOffset) != JCU_CBCR_OFFSET_0) && ((encode->inputCbCrOffset) != JCU_CBCR_OFFSET_128)) { 00349 returnValue = JCU_ERROR_PARAM; 00350 } /* end if */ 00351 00352 fin: 00353 return (returnValue); 00354 } 00355 00356 #endif
Generated on Tue Jul 12 2022 11:15:01 by
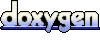