Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
clib_drivers_inline.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2013 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file clib_drivers_inline.h 00025 * @brief $Module: CLibCommon $ $PublicVersion: 0.90 $ (=CLIB_VERSION) 00026 * $Rev: 30 $ 00027 * $Date:: 2014-02-13 21:21:47 +0900#$ 00028 * - Description: Common code for drivers and more. 00029 */ 00030 00031 #ifndef CLIB_DRIVERS_INLINE_H 00032 #define CLIB_DRIVERS_INLINE_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 00038 #ifdef __cplusplus 00039 extern "C" { 00040 #endif /* __cplusplus */ 00041 00042 00043 /****************************************************************************** 00044 Typedef definitions 00045 ******************************************************************************/ 00046 /* in clib_drivers_typedef.h */ 00047 00048 /****************************************************************************** 00049 Macro definitions 00050 ******************************************************************************/ 00051 /* in clib_drivers_typedef.h */ 00052 00053 /****************************************************************************** 00054 Variable Externs 00055 ******************************************************************************/ 00056 /* in clib_drivers_typedef.h */ 00057 00058 /****************************************************************************** 00059 Functions Prototypes 00060 ******************************************************************************/ 00061 00062 00063 /** 00064 * @brief Cast with range check 00065 * 00066 * @param input Input value 00067 * @param output Output value 00068 * @return Error Code. 0=No Error. 00069 */ 00070 INLINE errnum_t R_int_t_to_int8_t( int_t input, int8_t *output ) 00071 { 00072 return R_int32_t_to_int8_t( input, output ); 00073 } 00074 00075 00076 /** 00077 * @brief Fast mod operation 00078 * 00079 * @param value Left operand 00080 * @return value % N 00081 * 00082 * @par Description 00083 * - This is shared description of 00084 * R_Mod_2s, R_Mod_4s, R_Mod_8s, R_Mod_16s, R_Mod_32s, R_Mod_64s, 00085 * R_Mod_2u, R_Mod_4u, R_Mod_8u, R_Mod_16u, R_Mod_32u, R_Mod_64u. 00086 * - Porting layer of fast % operator. 00087 * - MISRA & IPA SEC confirmed version. 00088 * - Minus value is expected 2's complement. 00089 * - Not same C99 % operator. 00090 * - Sample: R_Mod_4s( - 7 ) == +1. Sample: -7 % 4 == -3 00091 */ 00092 00093 00094 /** 00095 * @brief Fast mod operation. See <R_Mod_N>. 00096 * 00097 * @par Parameters 00098 * None 00099 * @return None. 00100 */ 00101 INLINE int_fast32_t R_Mod_2s( int_fast32_t const value ) 00102 { 00103 enum { mask = 1 }; 00104 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00105 } 00106 00107 00108 /** 00109 * @brief Fast mod operation. See <R_Mod_N>. 00110 * 00111 * @par Parameters 00112 * None 00113 * @return None. 00114 */ 00115 INLINE int_fast32_t R_Mod_4s( int_fast32_t const value ) 00116 { 00117 enum { mask = 3 }; 00118 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00119 } 00120 00121 00122 /** 00123 * @brief Fast mod operation. See <R_Mod_N>. 00124 * 00125 * @par Parameters 00126 * None 00127 * @return None. 00128 */ 00129 INLINE int_fast32_t R_Mod_8s( int_fast32_t const value ) 00130 { 00131 enum { mask = 7 }; 00132 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00133 } 00134 00135 00136 /** 00137 * @brief Fast mod operation. See <R_Mod_N>. 00138 * 00139 * @par Parameters 00140 * None 00141 * @return None. 00142 */ 00143 INLINE int_fast32_t R_Mod_16s( int_fast32_t const value ) 00144 { 00145 enum { mask = 15 }; 00146 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00147 } 00148 00149 00150 /** 00151 * @brief Fast mod operation. See <R_Mod_N>. 00152 * 00153 * @par Parameters 00154 * None 00155 * @return None. 00156 */ 00157 INLINE int_fast32_t R_Mod_32s( int_fast32_t const value ) 00158 { 00159 enum { mask = 31 }; 00160 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00161 } 00162 00163 00164 /** 00165 * @brief Fast mod operation. See <R_Mod_N>. 00166 * 00167 * @par Parameters 00168 * None 00169 * @return None. 00170 */ 00171 INLINE int_fast32_t R_Mod_64s( int_fast32_t const value ) 00172 { 00173 enum { mask = 63 }; 00174 return ((int_fast32_t)((uint_fast32_t)(value) & mask)); 00175 } 00176 00177 00178 /** 00179 * @brief Fast mod operation. See <R_Mod_N>. 00180 * 00181 * @par Parameters 00182 * None 00183 * @return None. 00184 */ 00185 INLINE uint_fast32_t R_Mod_2u( uint_fast32_t const value ) 00186 { 00187 return ((value) & 1u); 00188 } 00189 00190 00191 /** 00192 * @brief Fast mod operation. See <R_Mod_N>. 00193 * 00194 * @par Parameters 00195 * None 00196 * @return None. 00197 */ 00198 INLINE uint_fast32_t R_Mod_4u( uint_fast32_t const value ) 00199 { 00200 return ((value) & 3u); 00201 } 00202 00203 00204 /** 00205 * @brief Fast mod operation. See <R_Mod_N>. 00206 * 00207 * @par Parameters 00208 * None 00209 * @return None. 00210 */ 00211 INLINE uint_fast32_t R_Mod_8u( uint_fast32_t const value ) 00212 { 00213 return ((value) & 7u); 00214 } 00215 00216 00217 /** 00218 * @brief Fast mod operation. See <R_Mod_N>. 00219 * 00220 * @par Parameters 00221 * None 00222 * @return None. 00223 */ 00224 INLINE uint_fast32_t R_Mod_16u( uint_fast32_t const value ) 00225 { 00226 return ((value) & 15u); 00227 } 00228 00229 00230 /** 00231 * @brief Fast mod operation. See <R_Mod_N>. 00232 * 00233 * @par Parameters 00234 * None 00235 * @return None. 00236 */ 00237 INLINE uint_fast32_t R_Mod_32u( uint_fast32_t const value ) 00238 { 00239 return ((value) & 31u); 00240 } 00241 00242 00243 /** 00244 * @brief Fast mod operation. See <R_Mod_N>. 00245 * 00246 * @par Parameters 00247 * None 00248 * @return None. 00249 */ 00250 INLINE uint_fast32_t R_Mod_64u( uint_fast32_t const value ) 00251 { 00252 return ((value) & 63u); 00253 } 00254 00255 00256 /** 00257 * @brief Fast ceil operation 00258 * 00259 * @param value Left operand 00260 * @return Ceil( value / N ) 00261 * 00262 * @par Description 00263 * - This is shared description of 00264 * R_Ceil_2s, R_Ceil_4s, R_Ceil_8s, R_Ceil_16s, R_Ceil_32s, R_Ceil_64s, 00265 * R_Ceil_2u, R_Ceil_4u, R_Ceil_8u, R_Ceil_16u, R_Ceil_32u, R_Ceil_64u. 00266 * - Porting layer of fast ceil operation. 00267 * - Function version is confirmed with MISRA & IPA SEC. 00268 */ 00269 00270 00271 /** 00272 * @brief Fast ceil operation. See <R_Ceil_N>. 00273 * 00274 * @par Parameters 00275 * None 00276 * @return None. 00277 */ 00278 INLINE int_fast32_t R_Ceil_2s( int_fast32_t const value ) 00279 { 00280 static const uint_fast32_t mask = 1; 00281 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00282 } 00283 00284 00285 /** 00286 * @brief Fast ceil operation. See <R_Ceil_N>. 00287 * 00288 * @par Parameters 00289 * None 00290 * @return None. 00291 */ 00292 INLINE int_fast32_t R_Ceil_4s( int_fast32_t const value ) 00293 { 00294 static const uint_fast32_t mask = 3; 00295 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00296 } 00297 00298 00299 /** 00300 * @brief Fast ceil operation. See <R_Ceil_N>. 00301 * 00302 * @par Parameters 00303 * None 00304 * @return None. 00305 */ 00306 INLINE int_fast32_t R_Ceil_8s( int_fast32_t const value ) 00307 { 00308 static const uint_fast32_t mask = 7; 00309 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00310 } 00311 00312 00313 /** 00314 * @brief Fast ceil operation. See <R_Ceil_N>. 00315 * 00316 * @par Parameters 00317 * None 00318 * @return None. 00319 */ 00320 INLINE int_fast32_t R_Ceil_16s( int_fast32_t const value ) 00321 { 00322 static const uint_fast32_t mask = 15; 00323 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00324 } 00325 00326 00327 /** 00328 * @brief Fast ceil operation. See <R_Ceil_N>. 00329 * 00330 * @par Parameters 00331 * None 00332 * @return None. 00333 */ 00334 INLINE int_fast32_t R_Ceil_32s( int_fast32_t const value ) 00335 { 00336 static const uint_fast32_t mask = 31; 00337 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00338 } 00339 00340 00341 /** 00342 * @brief Fast ceil operation. See <R_Ceil_N>. 00343 * 00344 * @par Parameters 00345 * None 00346 * @return None. 00347 */ 00348 INLINE int_fast32_t R_Ceil_64s( int_fast32_t const value ) 00349 { 00350 static const uint_fast32_t mask = 63; 00351 return ((int_fast32_t)((uint_fast32_t)((value)+mask)&~mask)); 00352 } 00353 00354 00355 /** 00356 * @brief Fast ceil operation. See <R_Ceil_N>. 00357 * 00358 * @par Parameters 00359 * None 00360 * @return None. 00361 */ 00362 INLINE uint_fast32_t R_Ceil_2u( uint_fast32_t const value ) 00363 { 00364 static const uint_fast32_t mask = 1; 00365 return (((value)+mask)&~mask); 00366 } 00367 00368 00369 /** 00370 * @brief Fast ceil operation. See <R_Ceil_N>. 00371 * 00372 * @par Parameters 00373 * None 00374 * @return None. 00375 */ 00376 INLINE uint_fast32_t R_Ceil_4u( uint_fast32_t const value ) 00377 { 00378 static const uint_fast32_t mask = 3; 00379 return (((value)+mask)&~mask); 00380 } 00381 00382 00383 /** 00384 * @brief Fast ceil operation. See <R_Ceil_N>. 00385 * 00386 * @par Parameters 00387 * None 00388 * @return None. 00389 */ 00390 INLINE uint_fast32_t R_Ceil_8u( uint_fast32_t const value ) 00391 { 00392 static const uint_fast32_t mask = 7; 00393 return (((value)+mask)&~mask); 00394 } 00395 00396 00397 /** 00398 * @brief Fast ceil operation. See <R_Ceil_N>. 00399 * 00400 * @par Parameters 00401 * None 00402 * @return None. 00403 */ 00404 INLINE uint_fast32_t R_Ceil_16u( uint_fast32_t const value ) 00405 { 00406 static const uint_fast32_t mask = 15; 00407 return (((value)+mask)&~mask); 00408 } 00409 00410 00411 /** 00412 * @brief Fast ceil operation. See <R_Ceil_N>. 00413 * 00414 * @par Parameters 00415 * None 00416 * @return None. 00417 */ 00418 INLINE uint_fast32_t R_Ceil_32u( uint_fast32_t const value ) 00419 { 00420 static const uint_fast32_t mask = 31; 00421 return (((value)+mask)&~mask); 00422 } 00423 00424 00425 /** 00426 * @brief Fast ceil operation. See <R_Ceil_N>. 00427 * 00428 * @par Parameters 00429 * None 00430 * @return None. 00431 */ 00432 INLINE uint_fast32_t R_Ceil_64u( uint_fast32_t const value ) 00433 { 00434 static const uint_fast32_t mask = 63; 00435 return (((value)+mask)&~mask); 00436 } 00437 00438 00439 #ifdef __cplusplus 00440 } /* extern "C" */ 00441 #endif /* __cplusplus */ 00442 00443 #endif /* CLIB_DRIVERS_INLINE_H */ 00444 00445 00446
Generated on Tue Jul 12 2022 11:15:00 by
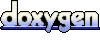