Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
RGA_Port_inline.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2013 - 2014 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /****************************************************************************** 00024 * $FileName: RGA_Port_inline.h $ 00025 * $Module: RGA $ $PublicVersion: 1.03 $ (=RGA_VERSION) 00026 * $Rev: $ 00027 * $Date:: $ 00028 * Description: 00029 ******************************************************************************/ 00030 00031 00032 /* This file is included from "RGA_Port.h" */ 00033 00034 00035 #ifndef RGA_PORT_INLINE_H 00036 #define RGA_PORT_INLINE_H 00037 #ifndef NOT_DEFINE_INLINE_FUNCTION 00038 00039 /****************************************************************************** 00040 Includes <System Includes> , "Project Includes" 00041 ******************************************************************************/ 00042 #include "clib_drivers.h " 00043 #include "frame_buffer.h " 00044 00045 #ifdef __cplusplus 00046 extern "C" { 00047 #endif /* __cplusplus */ 00048 00049 00050 /****************************************************************************** 00051 Typedef definitions 00052 ******************************************************************************/ 00053 /* in RGA_Port_typedef.h */ 00054 00055 /****************************************************************************** 00056 Macro definitions 00057 ******************************************************************************/ 00058 /* in RGA_Port_typedef.h */ 00059 00060 /****************************************************************************** 00061 Variable Externs 00062 ******************************************************************************/ 00063 /* in RGA_Port_typedef.h */ 00064 00065 /****************************************************************************** 00066 Functions Prototypes 00067 ******************************************************************************/ 00068 00069 00070 /** 00071 * @brief SwapEndian to 2Byte 00072 * 00073 * @param in_out_2byteVariable Address to 2 byte 00074 * @return None 00075 */ 00076 INLINE void SwapEndian2Byte( void *in_out_2byteVariable ) 00077 { 00078 byte_t buffer[2]; 00079 byte_t *data = (byte_t *) in_out_2byteVariable; 00080 00081 buffer[0] = data[0]; 00082 buffer[1] = data[1]; 00083 00084 data[0] = buffer[1]; 00085 data[1] = buffer[0]; 00086 } 00087 00088 00089 /** 00090 * @brief SwapEndian to 4Byte 00091 * 00092 * @param in_out_2byteVariable Address to 4 byte 00093 * @return None 00094 */ 00095 INLINE void SwapEndian4Byte( void *in_out_4byteVariable ) 00096 { 00097 byte_t buffer[4]; 00098 byte_t *data = (byte_t *) in_out_4byteVariable; 00099 00100 buffer[0] = data[0]; 00101 buffer[1] = data[1]; 00102 buffer[2] = data[2]; 00103 buffer[3] = data[3]; 00104 00105 data[0] = buffer[3]; 00106 data[1] = buffer[2]; 00107 data[2] = buffer[1]; 00108 data[3] = buffer[0]; 00109 } 00110 00111 00112 /** 00113 * @brief Sets the value to the address not aligned 4byte 00114 * 00115 * @param out_Variable <uint32_not_aligned_t> 00116 * @param Value Set value 00117 * @return None 00118 */ 00119 INLINE void uint32_not_aligned_t_set( uint32_not_aligned_t *out_Variable, uint32_t Value ) 00120 { 00121 #if BYTE_ENDIAN == BYTE_LITTLE_ENDIAN 00122 out_Variable->byte[0] = (byte_t)( ( Value & 0x000000FF ) ); 00123 out_Variable->byte[1] = (byte_t)( ( Value & 0x0000FF00 ) >> 8 ); 00124 out_Variable->byte[2] = (byte_t)( ( Value & 0x00FF0000 ) >> 16 ); 00125 out_Variable->byte[3] = (byte_t)( ( Value & 0xFF000000 ) >> 24 ); 00126 #else 00127 out_Variable->byte[0] = (byte_t)( ( Value & 0xFF000000 ) >> 24 ); 00128 out_Variable->byte[1] = (byte_t)( ( Value & 0x00FF0000 ) >> 16 ); 00129 out_Variable->byte[2] = (byte_t)( ( Value & 0x0000FF00 ) >> 8 ); 00130 out_Variable->byte[3] = (byte_t)( ( Value & 0x000000FF ) ); 00131 #endif 00132 } 00133 00134 00135 /*********************************************************************** 00136 * Class: byte_per_pixel_t 00137 ************************************************************************/ 00138 00139 00140 /** 00141 * @brief R_RGA_BitPerPixelType_To_BytePerPixelType 00142 * 00143 * @param BitPerPixel Input 00144 * @return byte_per_pixel_t 00145 */ 00146 INLINE byte_per_pixel_t R_RGA_BitPerPixelType_To_BytePerPixelType( int_fast32_t BitPerPixel ) 00147 { 00148 if ( R_Mod_8s( BitPerPixel ) == 0 ) { 00149 return (byte_per_pixel_t)( (uint_fast32_t) BitPerPixel / 8 ); 00150 } else { 00151 return BitPerPixel << R_BYTE_PER_PIXEL_SHIFT; 00152 } 00153 } 00154 00155 00156 /** 00157 * @brief R_RGA_BytePerPixelType_To_BitPerPixelType 00158 * 00159 * @param BytePerPixel byte_per_pixel_t 00160 * @return BitPerPixel 00161 */ 00162 INLINE int_fast32_t R_RGA_BytePerPixelType_To_BitPerPixelType( byte_per_pixel_t BytePerPixel ) 00163 { 00164 return ( ( BytePerPixel & R_BYTE_PER_PIXEL_MASK ) * 8 ) | 00165 ( BytePerPixel >> R_BYTE_PER_PIXEL_SHIFT ); 00166 } 00167 00168 00169 /** 00170 * @brief R_BYTE_PER_PIXEL_IsInteger 00171 * 00172 * @param BytePerPixel byte_per_pixel_t 00173 * @return Whether "BytePerPixel" is integer type. 00174 */ 00175 INLINE bool_t R_BYTE_PER_PIXEL_IsInteger( byte_per_pixel_t BytePerPixel ) 00176 { 00177 return ( BytePerPixel & R_BYTE_PER_PIXEL_MASK ) != 0; 00178 } 00179 00180 00181 /* Section: Global */ 00182 /** 00183 * @brief argb8888_t_to_rgb565_t 00184 * 00185 * @par Parameters 00186 * None 00187 * @return None. 00188 */ 00189 INLINE rgb565_t argb8888_t_to_rgb565_t( argb8888_t Color ) 00190 { 00191 rgb565_t ret = {0}; 00192 /* Warning: C4017W: ret may be used before being set */ 00193 /* This warning is not correct */ 00194 00195 ret.Value = (uint16_t)( 00196 ( ( Color.Value & 0x00F80000 ) >> 8 ) | 00197 ( ( Color.Value & 0x0000FC00 ) >> 5 ) | 00198 ( ( Color.Value & 0x000000F8 ) >> 3 ) ); 00199 00200 return ret; 00201 } 00202 00203 00204 /** 00205 * @brief argb8888_t_to_argb1555_t 00206 * 00207 * @par Parameters 00208 * None 00209 * @return None. 00210 */ 00211 INLINE argb1555_t argb8888_t_to_argb1555_t( argb8888_t Color ) 00212 { 00213 argb1555_t ret = {0}; 00214 /* Warning: C4017W: ret may be used before being set */ 00215 /* This warning is not correct */ 00216 00217 ret.Value = (uint16_t)( 00218 ( ( Color.Value & 0x80000000 ) >> 16 ) | /* A */ 00219 ( ( Color.Value & 0x00F80000 ) >> 9 ) | /* R */ 00220 ( ( Color.Value & 0x0000F800 ) >> 6 ) | /* G */ 00221 ( ( Color.Value & 0x000000F8 ) >> 3 ) ); /* B */ 00222 00223 return ret; 00224 } 00225 00226 00227 /** 00228 * @brief argb8888_t_to_argb4444_t 00229 * 00230 * @par Parameters 00231 * None 00232 * @return None. 00233 */ 00234 INLINE argb4444_t argb8888_t_to_argb4444_t( argb8888_t Color ) 00235 { 00236 argb4444_t ret = {0}; 00237 /* Warning: C4017W: ret may be used before being set */ 00238 /* This warning is not correct */ 00239 00240 ret.Value = (uint16_t)( 00241 ( ( Color.Value & 0xF0000000 ) >> 16 ) | 00242 ( ( Color.Value & 0x00F00000 ) >> 12 ) | 00243 ( ( Color.Value & 0x0000F000 ) >> 8 ) | 00244 ( ( Color.Value & 0x000000F0 ) >> 4 ) ); 00245 00246 return ret; 00247 } 00248 00249 00250 /** 00251 * @brief argb8888_t_to_r8g8b8a8_t 00252 * 00253 * @par Parameters 00254 * None 00255 * @return None. 00256 */ 00257 INLINE r8g8b8a8_t argb8888_t_to_r8g8b8a8_t( argb8888_t Color ) 00258 { 00259 r8g8b8a8_t ret; 00260 ret.Value = 0; /* Avoid Warning: C4017W: ret may be used before being set */ 00261 00262 ret.u.Red = Color.u.Red; 00263 ret.u.Green = Color.u.Green; 00264 ret.u.Blue = Color.u.Blue; 00265 ret.u.Alpha = Color.u.Alpha; 00266 00267 return ret; 00268 } 00269 00270 00271 /** 00272 * @brief rgb565_t_to_argb8888_t 00273 * 00274 * @par Parameters 00275 * None 00276 * @return None. 00277 */ 00278 INLINE argb8888_t rgb565_t_to_argb8888_t( rgb565_t Color ) 00279 { 00280 argb8888_t ret; 00281 00282 ret.Value = 0xFF000000 | /* A */ 00283 ( ( Color.Value & 0xF800 ) << 8 ) | ( ( Color.Value & 0xE000 ) << 3 ) | /* R */ 00284 ( ( Color.Value & 0x07E0 ) << 5 ) | ( ( Color.Value & 0x0600 ) >> 1 ) | /* G */ 00285 ( ( Color.Value & 0x001F ) << 3 ) | ( ( Color.Value & 0x001C ) >> 2 ); /* B */ 00286 00287 return ret; 00288 } 00289 00290 00291 /** 00292 * @brief argb1555_t_to_argb8888_t 00293 * 00294 * @par Parameters 00295 * None 00296 * @return None. 00297 */ 00298 INLINE argb8888_t argb1555_t_to_argb8888_t( argb1555_t Color ) 00299 { 00300 argb8888_t ret; 00301 00302 ret.Value = 00303 ( ( Color.Value & 0x7C00 ) << 9 ) | ( ( Color.Value & 0x7000 ) << 4 ) | /* R */ 00304 ( ( Color.Value & 0x03E0 ) << 6 ) | ( ( Color.Value & 0x0380 ) << 1 ) | /* G */ 00305 ( ( Color.Value & 0x001F ) << 3 ) | ( ( Color.Value & 0x001C ) >> 2 ); /* B */ 00306 00307 if ( Color.u.Alpha ) { 00308 ret.Value |= 0xFF000000; /* A */ 00309 } 00310 00311 return ret; 00312 } 00313 00314 00315 /** 00316 * @brief argb4444_t_to_argb8888_t 00317 * 00318 * @par Parameters 00319 * None 00320 * @return None. 00321 */ 00322 INLINE argb8888_t argb4444_t_to_argb8888_t( argb4444_t Color ) 00323 { 00324 argb8888_t ret; 00325 00326 ret.Value = 00327 ( ( Color.Value & 0xF000 ) << 16 ) | ( ( Color.Value & 0xF000 ) << 12 ) | /* A */ 00328 ( ( Color.Value & 0x0F00 ) << 12 ) | ( ( Color.Value & 0x0F00 ) << 8 ) | /* R */ 00329 ( ( Color.Value & 0x00F0 ) << 8 ) | ( ( Color.Value & 0x00F0 ) << 4 ) | /* G */ 00330 ( ( Color.Value & 0x000F ) << 4 ) | ( ( Color.Value & 0x000F ) ); /* B */ 00331 00332 return ret; 00333 } 00334 00335 00336 /** 00337 * @brief rgba8888_t_to_rgb565_t 00338 * 00339 * @par Parameters 00340 * None 00341 * @return None. 00342 */ 00343 INLINE rgb565_t rgba8888_t_to_rgb565_t( rgba8888_t Color ) 00344 { 00345 rgb565_t ret; 00346 00347 ret.Value = (uint16_t) ( 00348 ( ( Color.Value & 0xF8000000 ) >> 16 ) | /* R */ 00349 ( ( Color.Value & 0x00FC0000 ) >> 13 ) | /* G */ 00350 ( ( Color.Value & 0x0000F800 ) >> 11 ) ); /* B */ 00351 00352 return ret; 00353 } 00354 00355 00356 /** 00357 * @brief rgba8888_t_to_argb1555_t 00358 * 00359 * @par Parameters 00360 * None 00361 * @return None. 00362 */ 00363 INLINE argb1555_t rgba8888_t_to_argb1555_t( rgba8888_t Color ) 00364 { 00365 argb1555_t ret; 00366 00367 ret.Value = (uint16_t) ( 00368 ( ( Color.Value & 0xF8000000 ) >> 17 ) | /* R */ 00369 ( ( Color.Value & 0x00F80000 ) >> 14 ) | /* G */ 00370 ( ( Color.Value & 0x0000F800 ) >> 11 ) | /* B */ 00371 ( ( Color.Value & 0x00000080 ) << 8 ) ); /* A */ 00372 00373 return ret; 00374 } 00375 00376 00377 /** 00378 * @brief rgba8888_t_to_argb4444_t 00379 * 00380 * @par Parameters 00381 * None 00382 * @return None. 00383 */ 00384 INLINE argb4444_t rgba8888_t_to_argb4444_t( rgba8888_t Color ) 00385 { 00386 argb4444_t ret; 00387 00388 ret.Value = (uint16_t) ( 00389 ( ( Color.Value & 0xF0000000 ) >> 20 ) | /* R */ 00390 ( ( Color.Value & 0x00F00000 ) >> 16 ) | /* G */ 00391 ( ( Color.Value & 0x0000F000 ) >> 12 ) | /* B */ 00392 ( ( Color.Value & 0x000000F0 ) << 8 ) ); /* A */ 00393 00394 return ret; 00395 } 00396 00397 00398 /** 00399 * @brief r8g8b8a8_t_to_argb8888_t 00400 * 00401 * @par Parameters 00402 * None 00403 * @return None. 00404 */ 00405 INLINE argb8888_t r8g8b8a8_t_to_argb8888_t( r8g8b8a8_t Color ) 00406 { 00407 argb8888_t ret; 00408 00409 ret.Value = 00410 ( Color.u.Alpha << 24 ) | 00411 ( Color.u.Red << 16 ) | 00412 ( Color.u.Green << 8 ) | 00413 ( Color.u.Blue ); 00414 00415 return ret; 00416 } 00417 00418 00419 /** 00420 * @brief rgba8888_t_to_argb8888_t 00421 * 00422 * @par Parameters 00423 * None 00424 * @return None. 00425 */ 00426 INLINE argb8888_t rgba8888_t_to_argb8888_t( rgba8888_t Color ) 00427 { 00428 argb8888_t ret; 00429 00430 ret.Value = ( 00431 ( ( Color.Value & 0xFF000000 ) >> 8 ) | /* R */ 00432 ( ( Color.Value & 0x00FF0000 ) >> 8 ) | /* G */ 00433 ( ( Color.Value & 0x0000FF00 ) >> 8 ) | /* B */ 00434 ( ( Color.Value & 0x000000FF ) << 24 ) ); /* A */ 00435 00436 return ret; 00437 } 00438 00439 00440 #ifdef __cplusplus 00441 } /* extern "C" */ 00442 #endif /* __cplusplus */ 00443 00444 #endif /* NOT_DEFINE_INLINE_FUNCTION */ 00445 #endif /* RGA_PORT_INLINE_H */
Generated on Tue Jul 12 2022 11:15:04 by
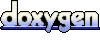