Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
RGA_API_typedef.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file RGA_API_typedef.h 00025 * @brief $Module: RGA $ $PublicVersion: 1.20 $ (=RGA_VERSION) 00026 * $Rev: $ 00027 * $Date:: $ 00028 */ 00029 00030 #ifndef RGA_API_TYPEDEF_H 00031 #define RGA_API_TYPEDEF_H 00032 00033 /****************************************************************************** 00034 Includes <System Includes> , "Project Includes" 00035 ******************************************************************************/ 00036 #include "Project_Config.h" 00037 #include "r_ospl_typedef.h" 00038 #include "locking_typedef.h" 00039 #include "clib_drivers_typedef.h " 00040 #include "frame_buffer_typedef.h " 00041 #include "RGA_raw_image_typedef.h " 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 00048 /****************************************************************************** 00049 Typedef definitions 00050 ******************************************************************************/ 00051 00052 /** 00053 * @enum graphics_quality_flag_t 00054 * @brief graphics_quality_flag_t 00055 */ 00056 typedef enum { 00057 /* Set to "ON" */ 00058 GRAPHICS_RENDERING_QUALITY_ANTIALIASED = 0x0001, 00059 GRAPHICS_IMAGE_QUALITY_ANTIALIASED = 0x0002, 00060 00061 /* Set to "OFF" */ 00062 GRAPHICS_RENDERING_QUALITY_NONANTIALIASED = GRAPHICS_RENDERING_QUALITY_ANTIALIASED << 16, 00063 GRAPHICS_IMAGE_QUALITY_NONANTIALIASED = GRAPHICS_IMAGE_QUALITY_ANTIALIASED << 16 00064 } 00065 graphics_quality_flag_t; 00066 00067 00068 /** 00069 * @enum graphics_jpeg_decoder_t 00070 * @brief graphics_jpeg_decoder_t 00071 */ 00072 typedef enum { 00073 GRAPHICS_JPEG_DECODER_NONE = 0, 00074 GRAPHICS_JPEG_DECODER_HARD = 1 00075 } graphics_jpeg_decoder_t; 00076 00077 00078 /** 00079 * @typedef graphics_quality_flags_t 00080 * @brief graphics_quality_flags_t 00081 */ 00082 typedef bit_flags_fast32_t /*<graphics_quality_flag_t>*/ graphics_quality_flags_t; 00083 00084 00085 /** 00086 * @struct graphics_config_t 00087 * @brief graphics_config_t 00088 */ 00089 typedef struct _GraphicsConfigClass graphics_config_t; 00090 struct _GraphicsConfigClass { 00091 bit_flags32_t flags; 00092 frame_buffer_t *frame_buffer; /* F_GRAPHICS_FRAME_BUFFER */ 00093 00094 /* for RGAH/RGAS hardware renderer */ 00095 void *work_buffer_address; /* F_GRAPHICS_WORK_BUFFER_ADDRESS */ 00096 size_t work_buffer_size; /* F_GRAPHICS_WORK_BUFFER_SIZE */ 00097 int_fast32_t max_width_of_frame_buffer; /* F_GRAPHICS_MAX_WIDTH_OF_FRAME_BUFFER */ 00098 int_fast32_t max_height_of_frame_buffer; /* F_GRAPHICS_MAX_HEIGHT_OF_FRAME_BUFFER */ 00099 00100 /* for any */ 00101 graphics_quality_flags_t quality_flags; /* F_GRAPHICS_QUALITY_FLAGS */ 00102 r8g8b8a8_t background_color; /* F_GRAPHICS_BACK_GROUND_COLOR */ 00103 bool_t is_fast_manual_flush; /* F_GRAPHICS_IS_FAST_MANUAL_FLUSH */ 00104 00105 /* work buffer B */ 00106 void *work_buffer_b_address; /* F_GRAPHICS_WORK_BUFFER_B_ADDRESS */ 00107 size_t work_buffer_b_size; /* F_GRAPHICS_WORK_BUFFER_B_SIZE */ 00108 00109 /* JPEG */ 00110 graphics_jpeg_decoder_t jpeg_decoder; /* F_GRAPHICS_JPEG_DECODER */ 00111 00112 /* PNG */ 00113 size_t work_size_for_libPNG; /* F_GRAPHICS_WORK_SIZE_FOR_LIBPNG */ 00114 00115 /* Asynchronous call */ 00116 bit_flags32_t internal_event_value; /* F_GRAPHICS_INTERNAL_EVENT_VALUE : 16bit */ 00117 00118 /* Thread safe */ 00119 BSP_CFG_USER_LOCKING_TYPE *lock_object; /* F_GRAPHICS_LOCK_OBJECT */ 00120 00121 /* for RGAS */ 00122 int_fast32_t channel_of_DMAC_1; /* F_GRAPHICS_CHANNEL_OF_DMAC_1 */ 00123 int_fast32_t channel_of_DMAC_2; /* F_GRAPHICS_CHANNEL_OF_DMAC_2 */ 00124 int_fast32_t event_value_of_DMAC_1; /* F_GRAPHICS_EVENT_VALUE_OF_DMAC_1 */ 00125 int_fast32_t event_value_of_DMAC_2; /* F_GRAPHICS_EVENT_VALUE_OF_DMAC_2 */ 00126 }; 00127 enum { 00128 F_GRAPHICS_FRAME_BUFFER = 0x0001, 00129 F_GRAPHICS_WORK_BUFFER_ADDRESS = 0x0002, 00130 F_GRAPHICS_WORK_BUFFER_SIZE = 0x0004, 00131 F_GRAPHICS_MAX_HEIGHT_OF_FRAME_BUFFER = 0x0008, 00132 F_GRAPHICS_MAX_WIDTH_OF_FRAME_BUFFER = 0x0080, 00133 F_GRAPHICS_QUALITY_FLAGS = 0x0010, 00134 F_GRAPHICS_BACK_GROUND_COLOR = 0x0020, 00135 F_GRAPHICS_IS_FAST_MANUAL_FLUSH = 0x0040, 00136 F_GRAPHICS_WORK_BUFFER_B_ADDRESS = 0x0100, 00137 F_GRAPHICS_WORK_BUFFER_B_SIZE = 0x0200, 00138 F_GRAPHICS_JPEG_DECODER = 0x1000, 00139 F_GRAPHICS_WORK_SIZE_FOR_LIBPNG = 0x8000, 00140 F_GRAPHICS_INTERNAL_EVENT_VALUE = 0x2000, 00141 F_GRAPHICS_LOCK_OBJECT = 0x4000, 00142 F_GRAPHICS_CHANNEL_OF_DMAC_1 = 0x0400, 00143 F_GRAPHICS_CHANNEL_OF_DMAC_2 = 0x0800, 00144 F_GRAPHICS_EVENT_VALUE_OF_DMAC_1 = 0x040000, 00145 F_GRAPHICS_EVENT_VALUE_OF_DMAC_2 = 0x080000 00146 }; 00147 00148 00149 /** 00150 * @typedef graphics_matrix_float_t 00151 * @brief graphics_matrix_float_t 00152 */ 00153 typedef float graphics_matrix_float_t; 00154 00155 00156 /** 00157 * @struct graphics_matrix3x3_t 00158 * @brief graphics_matrix3x3_t 00159 */ 00160 typedef union { 00161 graphics_matrix_float_t xy[3][3]; /* [x][y] */ 00162 graphics_matrix_float_t array[9]; /* { sx, shy, w0, shx, sy, w1, tx, ty, w2 } */ 00163 } graphics_matrix3x3_t; 00164 00165 00166 /** 00167 * @struct graphics_image_properties_t 00168 * @brief graphics_image_properties_t 00169 */ 00170 #if IS_SUPPORT_SAME_TYPEDEF || ! defined( graphics_image_properties_t ) 00171 typedef struct _GraphicsImagePropertiesClass graphics_image_properties_t; 00172 #define graphics_image_properties_t graphics_image_properties_t 00173 #endif 00174 struct _GraphicsImagePropertiesClass { 00175 int_fast32_t width; 00176 int_fast32_t height; 00177 uint8_t *data; /* NULL, if pixelFormat != PIXEL_FORMAT_R8G8B8A8 */ 00178 void *pixels; /* Same as "data" but not NULL */ 00179 pixel_format_t pixelFormat; 00180 uint32_t *CLUT; 00181 int_fast32_t CLUT_count; 00182 }; 00183 00184 00185 /** 00186 * @enum repetition_t 00187 * @brief repetition_t 00188 */ 00189 typedef enum st_repetition_t { 00190 GRAPHICS_REPEAT = 1 00191 } repetition_t; 00192 00193 00194 /** 00195 * @struct graphics_pattern_t 00196 * @brief graphics_pattern_t 00197 */ 00198 typedef struct st_graphics_pattern_t graphics_pattern_t; 00199 struct st_graphics_pattern_t { 00200 graphics_image_t *image; 00201 repetition_t repetition; 00202 }; 00203 00204 00205 /** 00206 * @enum graphics_composite_operation_t 00207 * @brief graphics_composite_operation_t 00208 */ 00209 typedef enum st_graphics_composite_operation_t { 00210 GRAPHICS_SOURCE_OVER = 1, 00211 GRAPHICS_DESTINATION_OUT = 7, 00212 GRAPHICS_COPY = 0 00213 } graphics_composite_operation_t; 00214 00215 00216 /** 00217 * @struct graphics_status_t 00218 * @brief graphics_status_t 00219 */ 00220 typedef struct st_graphics_status_t graphics_status_t; 00221 struct st_graphics_status_t { 00222 uint8_t __Members[0x30]; 00223 }; 00224 00225 00226 /** 00227 * @struct graphics_hard_status_t 00228 * @brief graphics_hard_status_t 00229 */ 00230 #ifdef RGAH_VERSION 00231 typedef struct st_graphics_hard_status_t graphics_hard_status_t; 00232 struct st_graphics_hard_status_t { 00233 volatile bool_t IsRenderingCompleted; 00234 }; 00235 #endif 00236 00237 00238 /** 00239 * @struct graphics_async_status_t 00240 * @brief graphics_async_status_t 00241 */ 00242 typedef struct st_graphics_async_status_t graphics_async_status_t; 00243 struct st_graphics_async_status_t { 00244 volatile r_ospl_async_state_t State; 00245 r_ospl_async_t *volatile Async; 00246 BSP_CFG_USER_LOCKING_TYPE *CLock; 00247 r_ospl_thread_id_t LockedThread; 00248 #ifdef RGAH_VERSION 00249 const graphics_hard_status_t *Hardware; 00250 #endif 00251 }; 00252 00253 00254 /** 00255 * @struct graphics_t 00256 * @brief graphics_t 00257 */ 00258 #if IS_SUPPORT_SAME_TYPEDEF || ! defined( graphics_t ) 00259 typedef struct st_graphics_t graphics_t; 00260 #endif 00261 struct st_graphics_t { 00262 uint8_t __Members[0x118]; 00263 }; 00264 00265 00266 /** 00267 * @brief R_GRAPHICS_OnInitialize_FuncType 00268 * 00269 * @par Parameters 00270 * None 00271 * @return None. 00272 */ 00273 typedef errnum_t (*R_GRAPHICS_OnInitialize_FuncType)( 00274 graphics_t *self, graphics_config_t *in_out_Config, void **out_CalleeDefined ); 00275 00276 /** 00277 * @brief R_GRAPHICS_OnFinalize_FuncType 00278 * 00279 * @par Parameters 00280 * None 00281 * @return None. 00282 */ 00283 typedef errnum_t (*R_GRAPHICS_OnFinalize_FuncType)( 00284 graphics_t *self, void *CalleeDefined, errnum_t e ); 00285 00286 00287 /** 00288 * @struct graphics_static_t 00289 * @brief graphics_static_t 00290 */ 00291 typedef struct st_graphics_static_t graphics_static_t; 00292 struct st_graphics_static_t { 00293 R_GRAPHICS_OnInitialize_FuncType OnInitialize; 00294 R_GRAPHICS_OnFinalize_FuncType OnFinalize; 00295 graphics_t *Contexts[1]; 00296 }; 00297 00298 00299 /** 00300 * @struct animation_timing_function_t 00301 * @brief animation_timing_function_t 00302 */ 00303 typedef struct _CSS_animation_timing_function_Class animation_timing_function_t; 00304 00305 00306 /****************************************************************************** 00307 Macro definitions 00308 ******************************************************************************/ 00309 00310 /****************************************************************************** 00311 Variable Externs 00312 ******************************************************************************/ 00313 00314 /****************************************************************************** 00315 Functions Prototypes 00316 ******************************************************************************/ 00317 00318 00319 #ifdef __cplusplus 00320 } /* extern "C" */ 00321 #endif 00322 00323 #endif /* RGA_API_TYPEDEF_H */
Generated on Tue Jul 12 2022 11:15:04 by
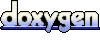