Graphics framework for GR-PEACH. When you use this program, we judge you have agreed to the following contents. https://developer.mbed.org/teams/Renesas/wiki/About-LICENSE
Dependents: ImageZoomInout_Sample ImageRotaion_Sample ImageScroll_Sample GR-PEACH_LCD_4_3inch_Save_to_USB ... more
r_ospl_debug.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /** 00024 * @file r_ospl_debug.c 00025 * @brief Debug tools provided by OSPL. 00026 * 00027 * $Module: OSPL $ $PublicVersion: 0.90 $ (=R_OSPL_VERSION) 00028 * $Rev: 35 $ 00029 * $Date:: 2014-04-15 21:38:18 +0900#$ 00030 */ 00031 00032 00033 /****************************************************************************** 00034 Includes <System Includes> , "Project Includes" 00035 ******************************************************************************/ 00036 #include "r_ospl.h" 00037 #include "r_ospl_debug.h" 00038 #if R_OSPL_DEBUG_TOOL 00039 #include <stdio.h> 00040 #endif 00041 00042 /****************************************************************************** 00043 Typedef definitions 00044 ******************************************************************************/ 00045 00046 /** 00047 * @struct r_ospl_debug_watch4_t 00048 * @brief Watch context 00049 * 00050 * @par Description 00051 * Member variables should not be accessed. 00052 */ 00053 #if R_OSPL_DEBUG_TOOL 00054 typedef struct st_r_ospl_debug_watch4_t r_ospl_debug_watch4_t; 00055 struct st_r_ospl_debug_watch4_t { 00056 volatile const uint32_t *Address; 00057 uint32_t BreakValue; 00058 bool_t IsPrintf; 00059 }; 00060 static r_ospl_debug_watch4_t g_r_ospl_debug_watch4[ 10 ]; 00061 #endif 00062 00063 00064 /****************************************************************************** 00065 Macro definitions 00066 ******************************************************************************/ 00067 00068 /****************************************************************************** 00069 Imported global variables and functions (from other files) 00070 ******************************************************************************/ 00071 00072 /****************************************************************************** 00073 Exported global variables and functions (to be accessed by other files) 00074 ******************************************************************************/ 00075 00076 /****************************************************************************** 00077 Private global variables and functions 00078 ******************************************************************************/ 00079 00080 00081 /*********************************************************************** 00082 * Implement: R_D_Add 00083 ************************************************************************/ 00084 #if R_OSPL_DEBUG_TOOL 00085 void R_D_Add( int_fast32_t IndexNum, volatile const void *in_Address, uint32_t BreakValue, bool_t IsPrintf ) 00086 { 00087 r_ospl_debug_watch4_t *self = &g_r_ospl_debug_watch4[ IndexNum ]; 00088 bool_t was_all_enabled; /* = false; */ /* QAC 3197 */ 00089 int_t r; 00090 00091 was_all_enabled = R_OSPL_DisableAllInterrupt(); 00092 00093 if ( IS( IsPrintf ) ) { 00094 r= printf( "Debug_add( %d, 0x%08X )\n", IndexNum, (uintptr_t) in_Address ); 00095 R_UNREFERENCED_VARIABLE( r ); /* QAC 3200 : This is not error information */ 00096 } 00097 self->Address = (uint32_t *) in_Address; 00098 self->BreakValue = BreakValue; 00099 self->IsPrintf = IsPrintf; 00100 00101 if ( IS( was_all_enabled ) ) { 00102 R_OSPL_EnableAllInterrupt(); 00103 } 00104 } 00105 #endif /* R_OSPL_DEBUG_TOOL */ 00106 00107 00108 /*********************************************************************** 00109 * Implement: R_D_Watch 00110 ************************************************************************/ 00111 #if R_OSPL_DEBUG_TOOL 00112 void R_D_Watch( int_fast32_t in_IndexNum ) 00113 { 00114 r_ospl_debug_watch4_t *self = &g_r_ospl_debug_watch4[ in_IndexNum ]; 00115 bool_t was_all_enabled; /* = false; */ /* QAC 3197 */ 00116 int_t r; 00117 00118 was_all_enabled = R_OSPL_DisableAllInterrupt(); 00119 00120 if ( self->Address != NULL ) { 00121 if ( IS( self->IsPrintf ) ) { 00122 r= printf( "Debug_watch( %d ): 0x%08X\n", in_IndexNum, (uintptr_t) *self->Address ); 00123 R_UNREFERENCED_VARIABLE( r ); /* QAC 3200 : This is not error information */ 00124 /* Cast of "uintptr_t" is for avoiding "format" warning of GNU_ARM */ 00125 } 00126 if ( *self->Address == self->BreakValue ) { 00127 R_DEBUG_BREAK(); 00128 } 00129 } 00130 00131 if ( IS( was_all_enabled ) ) { 00132 R_OSPL_EnableAllInterrupt(); 00133 } 00134 } 00135 #endif /* R_OSPL_DEBUG_TOOL */ 00136 00137 00138 /*********************************************************************** 00139 * Implement: R_D_AddToIntLog 00140 ************************************************************************/ 00141 #if R_OSPL_DEBUG_TOOL 00142 #define IntLogBreakID -1 /* -1=not break */ 00143 00144 volatile uint_fast32_t g_DebugVar[ g_DebugVarCount ]; 00145 volatile int_fast32_t g_IntLog[ g_IntLogCount ]; 00146 volatile int_fast32_t g_IntLogLength; 00147 00148 void R_D_AddToIntLog( int_fast32_t in_Value ) /* Add to g_IntLog, g_IntLogLength */ 00149 { 00150 g_IntLog[ (uint_fast32_t) g_IntLogLength % ( sizeof(g_IntLog) / sizeof(*g_IntLog) ) ] = in_Value; 00151 if ( g_IntLogLength == IntLogBreakID ) { 00152 R_DEBUG_BREAK(); 00153 } 00154 g_IntLogLength += 1; 00155 } 00156 #endif /* R_OSPL_DEBUG_TOOL */ 00157 00158 00159 /*********************************************************************** 00160 * Implement: R_D_Counter 00161 ************************************************************************/ 00162 #if R_OSPL_DEBUG_TOOL 00163 bool_t R_D_Counter( int_fast32_t *in_out_Counter, int_fast32_t TargetCount, char_t *in_Label ) 00164 { 00165 int_fast32_t counter; 00166 bool_t is_stop; 00167 int_t r; 00168 00169 counter = *in_out_Counter; 00170 counter += 1; 00171 is_stop = ( counter == TargetCount ); 00172 if ( in_Label != NULL ) { 00173 r= printf( "%s %d:\n", in_Label, counter ); 00174 R_UNREFERENCED_VARIABLE( r ); /* QAC 3200 : This is not error information */ 00175 } else { 00176 if ( counter == 1 ) { 00177 printf( "R_D_Counter address: 0x%08X\n", (uintptr_t) in_out_Counter ); 00178 } 00179 } 00180 *in_out_Counter = counter; 00181 00182 return is_stop; 00183 } 00184 #endif /* R_OSPL_DEBUG_TOOL */ 00185 00186
Generated on Tue Jul 12 2022 11:15:03 by
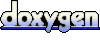