Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdec_register.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdec_register.h 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDEC driver register setup definitions 00029 ******************************************************************************/ 00030 00031 #ifndef R_VDEC_REGISTER_H 00032 #define R_VDEC_REGISTER_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include "r_vdec.h" 00038 #include "r_vdec_user.h" 00039 00040 00041 /****************************************************************************** 00042 Macro definitions 00043 ******************************************************************************/ 00044 00045 /****************************************************************************** 00046 Typedef definitions 00047 ******************************************************************************/ 00048 /*! VDEC register address list */ 00049 typedef struct { 00050 volatile uint16_t * adccr1; 00051 volatile uint16_t * tgcr1; 00052 volatile uint16_t * tgcr2; 00053 volatile uint16_t * tgcr3; 00054 volatile uint16_t * synscr1; 00055 volatile uint16_t * synscr2; 00056 volatile uint16_t * synscr3; 00057 volatile uint16_t * synscr4; 00058 volatile uint16_t * synscr5; 00059 volatile uint16_t * hafccr1; 00060 volatile uint16_t * hafccr2; 00061 volatile uint16_t * hafccr3; 00062 volatile uint16_t * vcdwcr1; 00063 volatile uint16_t * dcpcr1; 00064 volatile uint16_t * dcpcr2; 00065 volatile uint16_t * dcpcr3; 00066 volatile uint16_t * dcpcr4; 00067 volatile uint16_t * dcpcr5; 00068 volatile uint16_t * dcpcr6; 00069 volatile uint16_t * dcpcr7; 00070 volatile uint16_t * dcpcr8; 00071 volatile uint16_t * nsdcr; 00072 volatile uint16_t * btlcr; 00073 volatile uint16_t * btgpcr; 00074 volatile uint16_t * acccr1; 00075 volatile uint16_t * acccr2; 00076 volatile uint16_t * acccr3; 00077 volatile uint16_t * tintcr; 00078 volatile uint16_t * ycdcr; 00079 volatile uint16_t * agccr1; 00080 volatile uint16_t * agccr2; 00081 volatile uint16_t * pklimitcr; 00082 volatile uint16_t * rgorcr1; 00083 volatile uint16_t * rgorcr2; 00084 volatile uint16_t * rgorcr3; 00085 volatile uint16_t * rgorcr4; 00086 volatile uint16_t * rgorcr5; 00087 volatile uint16_t * rgorcr6; 00088 volatile uint16_t * rgorcr7; 00089 volatile uint16_t * afcpfcr; 00090 volatile uint16_t * rupdcr; 00091 volatile uint16_t * vsyncsr; 00092 volatile uint16_t * hsyncsr; 00093 volatile uint16_t * dcpsr1; 00094 volatile uint16_t * dcpsr2; 00095 volatile uint16_t * nsdsr; 00096 volatile uint16_t * cromasr1; 00097 volatile uint16_t * cromasr2; 00098 volatile uint16_t * syncssr; 00099 volatile uint16_t * agccsr1; 00100 volatile uint16_t * agccsr2; 00101 volatile uint16_t * ycscr3; 00102 volatile uint16_t * ycscr4; 00103 volatile uint16_t * ycscr5; 00104 volatile uint16_t * ycscr6; 00105 volatile uint16_t * ycscr7; 00106 volatile uint16_t * ycscr8; 00107 volatile uint16_t * ycscr9; 00108 volatile uint16_t * ycscr11; 00109 volatile uint16_t * ycscr12; 00110 volatile uint16_t * dcpcr9; 00111 volatile uint16_t * ygaincr; 00112 volatile uint16_t * cbgaincr; 00113 volatile uint16_t * crgaincr; 00114 volatile uint16_t * pga_update; 00115 volatile uint16_t * pgacr; 00116 volatile uint16_t * adccr2; 00117 } vdec_reg_address_t ; 00118 00119 /*! VDEC register address list (for 2D filter tap coefficient) */ 00120 typedef struct { 00121 volatile uint16_t * yctwa_f[VDEC_CHRFIL_TAPCOEF_NUM]; 00122 volatile uint16_t * yctwb_f[VDEC_CHRFIL_TAPCOEF_NUM]; 00123 volatile uint16_t * yctna_f[VDEC_CHRFIL_TAPCOEF_NUM]; 00124 volatile uint16_t * yctnb_f[VDEC_CHRFIL_TAPCOEF_NUM]; 00125 } vdec_filter_reg_address_t ; 00126 00127 00128 /****************************************************************************** 00129 Variable Externs 00130 ******************************************************************************/ 00131 extern const vdec_reg_address_t vdec_reg_address[VDEC_CHANNEL_NUM ]; 00132 extern const vdec_filter_reg_address_t vdec_filter_reg_address[VDEC_CHANNEL_NUM ]; 00133 00134 00135 /****************************************************************************** 00136 Functions Prototypes 00137 ******************************************************************************/ 00138 void VDEC_Initialize(const vdec_channel_t ch, const vdec_adc_vinsel_t vinsel); 00139 void VDEC_ActivePeriod(const vdec_channel_t ch, const vdec_active_period_t * const param); 00140 void VDEC_SyncSeparation(const vdec_channel_t ch, const vdec_sync_separation_t * const param); 00141 void VDEC_YcSeparation(const vdec_channel_t ch, const vdec_yc_separation_t * const param); 00142 void VDEC_ChromaDecoding(const vdec_channel_t ch, const vdec_chroma_decoding_t * const param); 00143 void VDEC_DigitalClamp(const vdec_channel_t ch, const vdec_degital_clamp_t * const param); 00144 void VDEC_Output(const vdec_channel_t ch, const vdec_output_t * const param); 00145 void VDEC_Query( 00146 const vdec_channel_t ch, 00147 vdec_q_sync_sep_t * const q_sync_sep, 00148 vdec_q_agc_t * const q_agc, 00149 vdec_q_chroma_dec_t * const q_chroma_dec, 00150 vdec_q_digital_clamp_t * const q_digital_clamp); 00151 00152 00153 #endif /* R_VDEC_REGISTER_H */
Generated on Tue Jul 12 2022 15:08:46 by
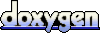