Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdec.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdec.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDEC driver API function 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "r_vdec.h" 00035 #include "r_vdec_user.h" 00036 #include "r_vdec_register.h" 00037 #include "r_vdec_check_parameter.h" 00038 00039 00040 /****************************************************************************** 00041 Macro definitions 00042 ******************************************************************************/ 00043 00044 /****************************************************************************** 00045 Typedef definitions 00046 ******************************************************************************/ 00047 00048 /****************************************************************************** 00049 Private global variables and functions 00050 ******************************************************************************/ 00051 00052 /**************************************************************************//** 00053 * @brief VDEC driver initialization 00054 * 00055 * Description:<br> 00056 * This function performs the following processing: 00057 * - Calls the user-defined function specified in init_func. 00058 * - Sets up the input pins. 00059 * @param[in] ch : Channel 00060 * @param[in] vinsel : Input pin control 00061 * - VDEC_ADC_VINSEL_VIN1 00062 * - VDEC_ADC_VINSEL_VIN2 00063 * @param[in] init_func : Pointer to a user-defined function 00064 * @param[in] user_num : User defined number 00065 * @retval Error code 00066 *****************************************************************************/ 00067 vdec_error_t R_VDEC_Initialize ( 00068 const vdec_channel_t ch, 00069 const vdec_adc_vinsel_t vinsel, 00070 void (* const init_func)(uint32_t), 00071 const uint32_t user_num) 00072 { 00073 vdec_error_t vdec_error; 00074 00075 vdec_error = VDEC_OK ; 00076 #ifdef R_VDEC_CHECK_PARAMETERS 00077 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00078 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00079 } 00080 if (vdec_error == VDEC_OK ) { 00081 vdec_error = VDEC_InitializeCheckParam(vinsel); 00082 } 00083 #endif /* R_VDEC_CHECK_PARAMETERS */ 00084 if (vdec_error == VDEC_OK ) { 00085 /* User-defined function */ 00086 if (init_func != 0) { 00087 init_func(user_num); 00088 } 00089 VDEC_Initialize(ch, vinsel); 00090 } 00091 return vdec_error; 00092 } /* End of function R_VDEC_Initialize() */ 00093 00094 /**************************************************************************//** 00095 * @brief VDEC driver termination 00096 * 00097 * Description:<br> 00098 * This function performs the following processing: 00099 * - Calls the user-defined function specified in quit_func. 00100 * @param[in] ch : Channel 00101 * @param[in] quit_func : Pointer to a user-defined function 00102 * @param[in] user_num : User defined number 00103 * @retval Error code 00104 *****************************************************************************/ 00105 vdec_error_t R_VDEC_Terminate (const vdec_channel_t ch, void (* const quit_func)(uint32_t), const uint32_t user_num) 00106 { 00107 vdec_error_t vdec_error; 00108 00109 vdec_error = VDEC_OK ; 00110 #ifdef R_VDEC_CHECK_PARAMETERS 00111 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00112 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00113 } 00114 #endif /* R_VDEC_CHECK_PARAMETERS */ 00115 if (vdec_error == VDEC_OK ) { 00116 /* User-defined function */ 00117 if (quit_func != 0) { 00118 quit_func(user_num); 00119 } 00120 } 00121 return vdec_error; 00122 } /* End of function R_VDEC_Terminate() */ 00123 00124 /**************************************************************************//** 00125 * @brief Active image period setup 00126 * 00127 * Description:<br> 00128 * This function configures the active image period. 00129 * @param[in] ch : Channel 00130 * @param[in] param : Active image period parameter 00131 * @retval Error code 00132 *****************************************************************************/ 00133 vdec_error_t R_VDEC_ActivePeriod (const vdec_channel_t ch, const vdec_active_period_t * const param) 00134 { 00135 vdec_error_t vdec_error; 00136 00137 vdec_error = VDEC_OK ; 00138 #ifdef R_VDEC_CHECK_PARAMETERS 00139 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00140 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00141 } 00142 if (vdec_error == VDEC_OK ) { 00143 vdec_error = VDEC_ActivePeriodCheckParam(param); 00144 } 00145 #endif /* R_VDEC_CHECK_PARAMETERS */ 00146 if (vdec_error == VDEC_OK ) { 00147 VDEC_ActivePeriod(ch, param); 00148 } 00149 return vdec_error; 00150 } /* End of function R_VDEC_ActivePeriod() */ 00151 00152 /**************************************************************************//** 00153 * @brief Sync separation processing 00154 * 00155 * Description:<br> 00156 * This function performs the following processing: 00157 * - Configures the noise reduction LPF. 00158 * - Configures the auto level control sync slicer. 00159 * - Configures the horizontal AFC. 00160 * - Configures the vertical count-down. 00161 * - Configures the AGC. 00162 * - Configures the peak limiter control. 00163 * @param[in] ch : Channel 00164 * @param[in] param : Sync separation parameter 00165 * @retval Error code 00166 *****************************************************************************/ 00167 vdec_error_t R_VDEC_SyncSeparation (const vdec_channel_t ch, const vdec_sync_separation_t * const param) 00168 { 00169 vdec_error_t vdec_error; 00170 00171 vdec_error = VDEC_OK ; 00172 #ifdef R_VDEC_CHECK_PARAMETERS 00173 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00174 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00175 } 00176 if (vdec_error == VDEC_OK ) { 00177 vdec_error = VDEC_SyncSeparationCheckParam(param); 00178 } 00179 #endif /* R_VDEC_CHECK_PARAMETERS */ 00180 if (vdec_error == VDEC_OK ) { 00181 VDEC_SyncSeparation(ch, param); 00182 } 00183 return vdec_error; 00184 } /* End of function R_VDEC_SyncSeparation() */ 00185 00186 /**************************************************************************//** 00187 * @brief Y/C separation processing 00188 * 00189 * Description:<br> 00190 * This function performs the following processing: 00191 * - Configures the over-range control. 00192 * - Configures the Y/C separation control. 00193 * - Configures the chroma filter TAP coefficients for Y/C separation. 00194 * @param[in] ch : Channel 00195 * @param[in] param : Y/C separation parameter 00196 * @retval Error code 00197 *****************************************************************************/ 00198 vdec_error_t R_VDEC_YcSeparation (const vdec_channel_t ch, const vdec_yc_separation_t * const param) 00199 { 00200 vdec_error_t vdec_error; 00201 00202 vdec_error = VDEC_OK ; 00203 #ifdef R_VDEC_CHECK_PARAMETERS 00204 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00205 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00206 } 00207 if (vdec_error == VDEC_OK ) { 00208 vdec_error = VDEC_YcSeparationCheckParam(param); 00209 } 00210 #endif /* R_VDEC_CHECK_PARAMETERS */ 00211 if (vdec_error == VDEC_OK ) { 00212 VDEC_YcSeparation(ch, param); 00213 } 00214 return vdec_error; 00215 } /* End of function R_VDEC_YcSeparation() */ 00216 00217 /**************************************************************************//** 00218 * @brief Chroma decoding processing 00219 * 00220 * Description:<br> 00221 * This function performs the following processing: 00222 * - Configures the color system detection. 00223 * - Configures the BCO. 00224 * - Configures the ACC/color killer. 00225 * - Configures the TINT correction/R-Y axis correction. 00226 * @param[in] ch : Channel 00227 * @param[in] param : Chroma decoding parameter 00228 * @retval Error code 00229 *****************************************************************************/ 00230 vdec_error_t R_VDEC_ChromaDecoding (const vdec_channel_t ch, const vdec_chroma_decoding_t * const param) 00231 { 00232 vdec_error_t vdec_error; 00233 00234 vdec_error = VDEC_OK ; 00235 #ifdef R_VDEC_CHECK_PARAMETERS 00236 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00237 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00238 } 00239 if (vdec_error == VDEC_OK ) { 00240 vdec_error = VDEC_ChromaDecodingCheckParam(param); 00241 } 00242 #endif /* R_VDEC_CHECK_PARAMETERS */ 00243 if (vdec_error == VDEC_OK ) { 00244 VDEC_ChromaDecoding(ch, param); 00245 } 00246 return vdec_error; 00247 } /* End of function R_VDEC_ChromaDecoding() */ 00248 00249 /**************************************************************************//** 00250 * @brief Digital clamp processing 00251 * 00252 * Description:<br> 00253 * This function performs the following processing: 00254 * - Configures the digital clamp control. 00255 * - Configures the center clamp. 00256 * - Configures the pedestal clamp. 00257 * - Configures the noise detection control. 00258 * @param[in] ch : Channel 00259 * @param[in] param : Digital clamp parameter 00260 * @retval Error code 00261 *****************************************************************************/ 00262 vdec_error_t R_VDEC_DigitalClamp (const vdec_channel_t ch, const vdec_degital_clamp_t * const param) 00263 { 00264 vdec_error_t vdec_error; 00265 00266 vdec_error = VDEC_OK ; 00267 #ifdef R_VDEC_CHECK_PARAMETERS 00268 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00269 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00270 } 00271 if (vdec_error == VDEC_OK ) { 00272 vdec_error = VDEC_DigitalClampCheckParam(param); 00273 } 00274 #endif /* R_VDEC_CHECK_PARAMETERS */ 00275 if (vdec_error == VDEC_OK ) { 00276 VDEC_DigitalClamp(ch, param); 00277 } 00278 return vdec_error; 00279 } /* End of function R_VDEC_DigitalClamp() */ 00280 00281 /**************************************************************************//** 00282 * @brief Output adjustment processing 00283 * 00284 * Description:<br> 00285 * This function makes settings for output adjustment. 00286 * @param[in] ch : Channel 00287 * @param[in] param : Output adjustment parameter 00288 * @retval Error code 00289 *****************************************************************************/ 00290 vdec_error_t R_VDEC_Output (const vdec_channel_t ch, const vdec_output_t * const param) 00291 { 00292 vdec_error_t vdec_error; 00293 00294 vdec_error = VDEC_OK ; 00295 #ifdef R_VDEC_CHECK_PARAMETERS 00296 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00297 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00298 } 00299 if (vdec_error == VDEC_OK ) { 00300 vdec_error = VDEC_OutputCheckParam(param); 00301 } 00302 #endif /* R_VDEC_CHECK_PARAMETERS */ 00303 if (vdec_error == VDEC_OK ) { 00304 VDEC_Output(ch, param); 00305 } 00306 return vdec_error; 00307 } /* End of function R_VDEC_Output() */ 00308 00309 /**************************************************************************//** 00310 * @brief VDEC information acquisition processing 00311 * 00312 * Description:<br> 00313 * This gets the parameters of the VDEC modules. The parameters that can be obtained are listed below. 00314 * - Sync separation parameters 00315 * - AGC parameters 00316 * - Chroma decoding parameters 00317 * - Digital clamp parameters 00318 * @param[in] ch : Channel 00319 * @param[out] q_sync_sep : Pointer to the place where the sync separation parameters are stored. 00320 * @param[out] q_agc : Pointer to the place where the AGC parameters are stored. 00321 * @param[out] q_chroma_dec : Pointer to the place where the chroma decoding parameters are stored. 00322 * @param[out] q_digital_clamp : Pointer to the place where the digital clamp parameters are stored. 00323 * @retval Error code 00324 *****************************************************************************/ 00325 vdec_error_t R_VDEC_Query ( 00326 const vdec_channel_t ch, 00327 vdec_q_sync_sep_t * const q_sync_sep, 00328 vdec_q_agc_t * const q_agc, 00329 vdec_q_chroma_dec_t * const q_chroma_dec, 00330 vdec_q_digital_clamp_t * const q_digital_clamp) 00331 { 00332 vdec_error_t vdec_error; 00333 00334 vdec_error = VDEC_OK ; 00335 #ifdef R_VDEC_CHECK_PARAMETERS 00336 if ((ch != VDEC_CHANNEL_0 ) && (ch != VDEC_CHANNEL_1 )) { 00337 vdec_error = VDEC_ERR_CHANNEL ; /* Channel error */ 00338 } 00339 #endif /* R_VDEC_CHECK_PARAMETERS */ 00340 if (vdec_error == VDEC_OK ) { 00341 VDEC_Query(ch, q_sync_sep, q_agc, q_chroma_dec, q_digital_clamp); 00342 } 00343 return vdec_error; 00344 } /* End of function R_VDEC_Query() */ 00345
Generated on Tue Jul 12 2022 15:08:46 by
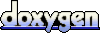