Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
lvds_pll_main.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file lvds_pll_main.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief lvds pll setting value 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include <stdio.h> 00035 #include <stdlib.h> 00036 #include <math.h> 00037 00038 #include "r_typedefs.h" 00039 #include "lvds_pll_calc.h" 00040 00041 /****************************************************************************** 00042 Macro definitions 00043 ******************************************************************************/ 00044 /* FIN: LVDS PLL input frequency */ 00045 #define FIN_MIN (9.0) /*!< The lower limit of the FIN */ 00046 #define FIN_MAX (30.0) /*!< The upper limit of the FIN */ 00047 /* FREF */ 00048 #define FREF_MIN (2.5) /*!< The lower limit of the FREF */ 00049 #define FREF_MAX (30.0) /*!< The upper limit of the FREF */ 00050 /* FVCO: VCO output frequency */ 00051 #define FVCO_MIN (750.0) /*!< The lower limit of the FVCO */ 00052 #define FVCO_MAX (1630.0) /*!< The upper limit of the FVCO */ 00053 /* FOUT: LVDS PLL output frequency */ 00054 #define FOUT_MIN (0.0) /*!< The lower limit of the FOUT */ 00055 #define FOUT_MAX (609.0) /*!< The upper limit of the FOUT */ 00056 00057 #define DIVIDER_3 (7.0) /*!< The frequency dividing value for frequency divider 3 */ 00058 00059 00060 /****************************************************************************** 00061 Typedef definitions 00062 ******************************************************************************/ 00063 00064 00065 /****************************************************************************** 00066 Variable Externs 00067 ******************************************************************************/ 00068 extern const double NIDIV_data[]; 00069 extern const double NRD_data[]; 00070 extern const double NFD_data[]; 00071 extern const double NOD_data[]; 00072 extern const double NODIV_data[]; 00073 00074 00075 /****************************************************************************** 00076 Private global variables and functions 00077 ******************************************************************************/ 00078 static double InputClock; 00079 static double OutputClock; 00080 static int LvdsUsed; 00081 00082 00083 00084 /**************************************************************************//** 00085 * @brief 00086 * @param [in,out]param 00087 * @param [in]fout 00088 * @retval int 00089 *****************************************************************************/ 00090 static int compare_nodiv (pll_parameter_t * param, double fout) 00091 { 00092 int change; 00093 const double * nodiv_list; 00094 double diff_freq; 00095 00096 change = 0; 00097 nodiv_list = NODIV_data; 00098 while (*nodiv_list != 0.0) { 00099 diff_freq = fabs(OutputClock - (fout / *nodiv_list)); 00100 if (param->comparison_freq > diff_freq) { 00101 param->comparison_freq = diff_freq; 00102 param->output_freq = fout / *nodiv_list; 00103 param->nodiv = *nodiv_list; 00104 change = 1; 00105 } 00106 nodiv_list++; 00107 } 00108 return change; 00109 } 00110 00111 /**************************************************************************//** 00112 * @brief 00113 * @param [in,out]param 00114 * @param [in]fout 00115 * @retval int 00116 *****************************************************************************/ 00117 static int compare_div3 (pll_parameter_t * param, double fout) 00118 { 00119 double diff_freq; 00120 int change; 00121 00122 change = 0; 00123 diff_freq = fabs(OutputClock - (fout / DIVIDER_3)); 00124 00125 if (param->comparison_freq > diff_freq) { 00126 param->comparison_freq = diff_freq; 00127 param->output_freq = fout / DIVIDER_3; 00128 change = 1; 00129 } 00130 return change; 00131 } 00132 00133 /**************************************************************************//** 00134 * @brief 00135 * @param [out]param 00136 * @retval None 00137 *****************************************************************************/ 00138 static void SeekValue (pll_parameter_t * param) 00139 { 00140 const double * nidiv_list; 00141 const double * nrd_list; 00142 const double * nfd_list; 00143 const double * nod_list; 00144 00145 double fin; 00146 double fref; 00147 double fvco; 00148 double fout; 00149 int ret; 00150 00151 nidiv_list = NIDIV_data; 00152 while (*nidiv_list != 0.0) { 00153 fin = InputClock / *nidiv_list; 00154 if ((fin < FIN_MIN) || (FIN_MAX < fin)) { 00155 nidiv_list++; 00156 continue; 00157 } 00158 nrd_list = NRD_data; 00159 while (*nrd_list != 0.0) { 00160 fref = fin / *nrd_list; 00161 if ((fref < FREF_MIN) || (FREF_MAX < fref)) { 00162 nrd_list++; 00163 continue; 00164 } 00165 nfd_list = NFD_data; 00166 while (*nfd_list != 0.0) { 00167 fvco = fref * (*nfd_list); 00168 if ((fvco < FVCO_MIN) || (FVCO_MAX < fvco)) { 00169 nfd_list++; 00170 continue; 00171 } 00172 nod_list = NOD_data; 00173 while (*nod_list != 0.0) { 00174 fout = fvco / *nod_list; 00175 if ((fout < FOUT_MIN) || (FOUT_MAX < fout)) { 00176 nod_list++; 00177 continue; 00178 } 00179 00180 if (LvdsUsed == 0) { 00181 ret = compare_nodiv (param, fout); 00182 } else { 00183 ret = compare_div3 (param, fout); 00184 } 00185 if (ret != 0) { 00186 param->nidiv = *nidiv_list; 00187 param->nrd = *nrd_list; 00188 param->nfd = *nfd_list; 00189 param->nod = *nod_list; 00190 } 00191 nod_list++; 00192 } 00193 nfd_list++; 00194 } 00195 nrd_list++; 00196 } 00197 nidiv_list++; 00198 } 00199 } 00200 /**************************************************************************//** 00201 * @brief PLL value Calculation function 00202 * @param[in] InClock : Input clock frequency [MHz] 00203 * @param[in] OuClock : Output clock frequency [MHz] 00204 * @param[in] Lvds : The LVDS output interface is used (=1) or not (=0) 00205 * @param[out] result : pll_parameter_t 00206 * @retval error (-1) 00207 ******************************************************************************/ 00208 int32_t lvds_pll_calc( 00209 const double InClock, 00210 const double OuClock, 00211 const uint32_t Lvds, 00212 pll_parameter_t * result ) 00213 { 00214 result->comparison_freq = 1000.0; 00215 result->output_freq = 0.0; 00216 result->nidiv = 0.0; 00217 result->nrd = 0.0; 00218 result->nfd = 0.0; 00219 result->nod = 0.0; 00220 result->nodiv = 0.0; 00221 00222 InputClock = InClock; 00223 OutputClock = OuClock; 00224 LvdsUsed = Lvds; 00225 00226 /* Calculation */ 00227 SeekValue (result); 00228 00229 result->nidiv = result->nidiv/2; 00230 result->nodiv = result->nodiv/2; 00231 if( result->nod <= 4 ) { 00232 result->nod = result->nod/2; 00233 } else if( result->nod == 8 ) { 00234 result->nod = 3; 00235 } else { 00236 } 00237 result->nrd -=1; /* nrd-1 */ 00238 return 0; 00239 }
Generated on Tue Jul 12 2022 15:08:46 by
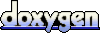