Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdc5_register.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdc5_register.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDC5 driver register setup processing 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "r_vdc5.h" 00035 #include "r_vdc5_user.h" 00036 #include "r_vdc5_register.h" 00037 #include "r_vdc5_shared_param.h" 00038 00039 00040 /****************************************************************************** 00041 Macro definitions 00042 ******************************************************************************/ 00043 #define INITIAL_SCALING_PHASE ((uint32_t)2048u) 00044 #define SCALING_RATIO_SAME_SIZE (4096u) 00045 /* Vsync signal delay control for OIR */ 00046 #define OIR_VSYNC_DELAY ((uint32_t)1u) 00047 00048 #define LVDS_LPHYACC_VALUE ((uint32_t)0x0001u) 00049 00050 /* Panel clock frequency division ratio */ 00051 #define VDC5_REG_CLKDIV_1_1 (1u) 00052 #define VDC5_REG_CLKDIV_1_2 (2u) 00053 #define VDC5_REG_CLKDIV_1_3 (3u) 00054 #define VDC5_REG_CLKDIV_1_4 (4u) 00055 #define VDC5_REG_CLKDIV_1_5 (5u) 00056 #define VDC5_REG_CLKDIV_1_6 (6u) 00057 #define VDC5_REG_CLKDIV_1_7 (7u) 00058 #define VDC5_REG_CLKDIV_1_8 (8u) 00059 #define VDC5_REG_CLKDIV_1_9 (9u) 00060 #define VDC5_REG_CLKDIV_1_12 (12u) 00061 #define VDC5_REG_CLKDIV_1_16 (16u) 00062 #define VDC5_REG_CLKDIV_1_24 (24u) 00063 #define VDC5_REG_CLKDIV_1_32 (32u) 00064 /* Panel clock select */ 00065 #define VDC5_REG_ICKSEL_IMG (0x0000u) 00066 #define VDC5_REG_ICKSEL_IMG_DV (0x0000u) 00067 #define VDC5_REG_ICKSEL_EXT_0 (0x1000u) 00068 #define VDC5_REG_ICKSEL_EXT_1 (0x2000u) 00069 #define VDC5_REG_ICKSEL_PERI (0x3000u) 00070 #define VDC5_REG_ICKSEL_LVDS (0x0400u) 00071 #define VDC5_REG_ICKSEL_LVDS_DIV7 (0x0800u) 00072 /* Output pin edge select */ 00073 #define VDC5_REG_TCON0_EDGE (0x0040u) 00074 #define VDC5_REG_TCON1_EDGE (0x0020u) 00075 #define VDC5_REG_TCON2_EDGE (0x0010u) 00076 #define VDC5_REG_TCON3_EDGE (0x0008u) 00077 #define VDC5_REG_TCON4_EDGE (0x0004u) 00078 #define VDC5_REG_TCON5_EDGE (0x0002u) 00079 #define VDC5_REG_TCON6_EDGE (0x0001u) 00080 /* Panel dither output format select */ 00081 #define VDC5_REG_PATH_FORMAT_RGB888 (0x00000000u) 00082 #define VDC5_REG_PATH_FORMAT_RGB666 (0x00010000u) 00083 #define VDC5_REG_PATH_FORMAT_RGB565 (0x00020000u) 00084 #define VDC5_REG_PATH_FORMAT_NON (0x00000000u) 00085 /* Calculate scaling ratio use value */ 00086 #define VDC5_REG_FLOAT_1_0 (1.0f) 00087 #define VDC5_REG_FLOAT_0_5 (0.5f) 00088 /* bits per pixel */ 00089 #define VDC5_REG_BIT_PER_PIXEL_RGB565 (16u) 00090 #define VDC5_REG_BIT_PER_PIXEL_RGB888 (32u) 00091 #define VDC5_REG_BIT_PER_PIXEL_ARGB1555 (16u) 00092 #define VDC5_REG_BIT_PER_PIXEL_ARGB4444 (16u) 00093 #define VDC5_REG_BIT_PER_PIXEL_ARGB8888 (32u) 00094 #define VDC5_REG_BIT_PER_PIXEL_CLUT8 (8u) 00095 #define VDC5_REG_BIT_PER_PIXEL_CLUT4 (4u) 00096 #define VDC5_REG_BIT_PER_PIXEL_CLUT1 (1u) 00097 #define VDC5_REG_BIT_PER_PIXEL_YCBCR422 (32u) 00098 #define VDC5_REG_BIT_PER_PIXEL_YCBCR444 (32u) 00099 #define VDC5_REG_BIT_PER_PIXEL_RGBA5551 (16u) 00100 #define VDC5_REG_BIT_PER_PIXEL_RGBA8888 (32u) 00101 #define VDC5_REG_BIT_PER_PIXEL_VALUE_8 (8u) 00102 /* Convert the color data from 4 bits value into 8 bits value */ 00103 #define VDC5_REG_EXTENSION_VALUE_17 (17u) 00104 /* Convert the color data from 5 bits value into 8 bits value */ 00105 #define VDC5_REG_EXTENSION_VALUE_263 (263u) 00106 #define VDC5_REG_EXTENSION_VALUE_32 (32u) 00107 /* Convert the color data from 6 bits value into 8 bits value */ 00108 #define VDC5_REG_EXTENSION_VALUE_259 (259u) 00109 #define VDC5_REG_EXTENSION_VALUE_64 (64u) 00110 /* Round off */ 00111 #define VDC5_REG_ROUND_OFF_VALUE_1 (1u) 00112 #define VDC5_REG_ROUND_OFF_VALUE_2 (2u) 00113 /* Color mask data */ 00114 #define VDC5_REG_ALPHA_8BIT (0xFF000000u) 00115 #define VDC5_REG_RGB_24BIT_MASK (0x00FFFFFFu) 00116 #define VDC5_REG_COLOR_8BIT_MASK (0x000000FFu) 00117 #define VDC5_REG_COLOR_6BIT_MASK (0x0000003Fu) 00118 #define VDC5_REG_COLOR_5BIT_MASK (0x0000001Fu) 00119 #define VDC5_REG_COLOR_4BIT_MASK (0x0000000Fu) 00120 #define VDC5_REG_ARGB8888_GB_MASK (0x00FFFF00u) 00121 00122 /* RGB565 bits mask data */ 00123 #define VDC5_REG_RGB565_R_MASK (0x0000F800u) 00124 #define VDC5_REG_RGB565_G_MASK (0x000007E0u) 00125 #define VDC5_REG_RGB565_B_MASK (0x0000001Fu) 00126 /* ARGB4444 bits mask data */ 00127 #define VDC5_REG_ARGB4444_R_MASK (0x00000F00u) 00128 #define VDC5_REG_ARGB4444_G_MASK (0x000000F0u) 00129 #define VDC5_REG_ARGB4444_B_MASK (0x0000000Fu) 00130 /* ARGB1555 bits mask data */ 00131 #define VDC5_REG_ARGB1555_R_MASK (0x00007C00u) 00132 #define VDC5_REG_ARGB1555_G_MASK (0x000003E0u) 00133 #define VDC5_REG_ARGB1555_B_MASK (0x0000001Fu) 00134 /* RGBA5551 bits mask data */ 00135 #define VDC5_REG_RGBA5551_R_MASK (0x0000F800u) 00136 #define VDC5_REG_RGBA5551_G_MASK (0x000007C0u) 00137 #define VDC5_REG_RGBA5551_B_MASK (0x0000003Eu) 00138 00139 /* bit pattern */ 00140 #define VDC5_REG_BIT31 (0x80000000u) 00141 #define VDC5_REG_BIT29 (0x20000000u) 00142 #define VDC5_REG_BIT28 (0x10000000u) 00143 #define VDC5_REG_BIT24 (0x01000000u) 00144 #define VDC5_REG_BIT20 (0x00100000u) 00145 #define VDC5_REG_BIT16 (0x00010000u) 00146 #define VDC5_REG_BIT15 (0x00008000u) 00147 #define VDC5_REG_BIT14 (0x00004000u) 00148 #define VDC5_REG_BIT13 (0x00002000u) 00149 #define VDC5_REG_BIT12 (0x00001000u) 00150 #define VDC5_REG_BIT8 (0x00000100u) 00151 #define VDC5_REG_BIT4 (0x00000010u) 00152 #define VDC5_REG_BIT2 (0x00000004u) 00153 #define VDC5_REG_BIT1 (0x00000002u) 00154 #define VDC5_REG_BIT0 (0x00000001u) 00155 00156 /* shift value */ 00157 #define VDC5_REG_SHIFT_28 (28u) 00158 #define VDC5_REG_SHIFT_24 (24u) 00159 #define VDC5_REG_SHIFT_20 (20u) 00160 #define VDC5_REG_SHIFT_16 (16u) 00161 #define VDC5_REG_SHIFT_13 (13u) 00162 #define VDC5_REG_SHIFT_12 (12u) 00163 #define VDC5_REG_SHIFT_11 (11u) 00164 #define VDC5_REG_SHIFT_10 (10u) 00165 #define VDC5_REG_SHIFT_8 (8u) 00166 #define VDC5_REG_SHIFT_6 (6u) 00167 #define VDC5_REG_SHIFT_5 (5u) 00168 #define VDC5_REG_SHIFT_4 (4u) 00169 #define VDC5_REG_SHIFT_2 (2u) 00170 #define VDC5_REG_SHIFT_1 (1u) 00171 00172 /* register set value */ 00173 #define VDC5_REG_BIT_0XFFFF0000 (0xFFFF0000u) /* scaler_0->scl0_frc2 */ 00174 /* scaler_1->scl0_frc2 */ 00175 /* register mask value */ 00176 #define VDC5_REG_MASK_0XF7FF1C3F (0xF7FF1C3Fu) /* mask img_synthesizer->gr_flm6 */ 00177 #define VDC5_REG_MASK_0X0000D000 (0x0000D000u) /* mask img_synthesizer->gr_ab1 */ 00178 #define VDC5_REG_MASK_0X01FF00FF (0x01FF00FFu) /* mask img_synthesizer->gr_ab6 */ 00179 #define VDC5_REG_MASK_0X0000007F (0x0000007Fu) /* mask output_ctrl->out_clk_phase */ 00180 #define VDC5_REG_MASK_0X11003313 (0x11003313u) /* mask output_ctrl->out_set */ 00181 #define VDC5_REG_MASK_0X00030000 (0x00030000u) /* mask output_ctrl->out_pdtha */ 00182 #define VDC5_REG_MASK_0X0007000D (0x0007000Du) /* mask scaler->scl1_wr1 */ 00183 #define VDC5_REG_MASK_0X00000300 (0x00000300u) /* mask scaler->scl1_wr5 */ 00184 #define VDC5_REG_MASK_0X0703FF02 (0x0703FF02u) /* mask regaddr_lvds->lclkselr */ 00185 #define VDC5_REG_MASK_0X07FF1F30 (0x07FF1F30u) /* mask regaddr_lvds->lpllsetr */ 00186 #define VDC5_REG_MASK_0X00010301 (0x00010301u) /* mask img_synthesizer->gr_flm1 */ 00187 #define VDC5_REG_MASK_0X7FFF03FF (0x7FFF03FFu) /* mask img_synthesizer->gr_flm3 */ 00188 #define VDC5_REG_MASK_0X0000E000 (0x0000E000u) /* mask img_synthesizer->gr_flm6 */ 00189 #define VDC5_REG_MASK_0X00007F33 (0x00007F33u) /* mask input_ctrl->imgcnt_nr_cnt0 */ 00190 #define VDC5_REG_MASK_0X00FF0000 (0x00FF0000u) /* mask img_synthesizer->gr_ab7 */ 00191 #define VDC5_REG_MASK_0X00300000 (0x00300000u) /* mask output_ctrl->out_pdtha */ 00192 #define VDC5_REG_MASK_0X00003333 (0x00003333u) /* mask output_ctrl->out_pdtha */ 00193 #define VDC5_REG_MASK_0X00007111 (0x00007111u) /* mask input_ctrl->inp_sel_cnt */ 00194 #define VDC5_REG_MASK_0X11110113 (0x11110113u) /* mask input_ctrl->inp_ext_sync_cnt */ 00195 #define VDC5_REG_MASK_0X000007FF (0x000007FFu) /* mask img_synthesizer->gr_flm5 */ 00196 #define VDC5_REG_MASK_0X0000FFFF (0x0000FFFFu) /* mask scaler->scl0_ds4 */ 00197 /* caler->scl0_us5 */ 00198 #define VDC5_REG_MASK_0X00000011 (0x00000011u) /* mask scaler->scl0_ds1 */ 00199 /* scaler->scl0_us1 */ 00200 #define VDC5_REG_MASK_0X00000070 (0x00000070u) /* mask scaler->scl1_wr1 */ 00201 #define VDC5_REG_MASK_0X00000080 (0x00000080u) /* mask scaler->scl1_wr1 */ 00202 #define VDC5_REG_MASK_0X00003110 (0x00003110u) /* mask output_ctrl->tcon_tim_pola2 */ 00203 /* output_ctrl->tcon_tim_polb2 */ 00204 #define VDC5_REG_MASK_0X000000FF (0x000000FFu) /* mask scaler_0->scl0_frc5 */ 00205 /* scaler_1->scl0_frc5 */ 00206 #define VDC5_REG_MASK_0X0000003F (0x0000003Fu) /* mask img_synthesizer->gr_flm6 */ 00207 /* img_qlty_imp->adj_enh_shp1 */ 00208 #define VDC5_REG_MASK_0XFF000000 (0xFF000000u) /* mask img_synthesizer->gr_ab10 */ 00209 /* img_synthesizer->gr_ab11 */ 00210 #define VDC5_REG_MASK_0X00000110 (0x00000110u) /* mask output_ctrl->tcon_tim_sth2 */ 00211 /* output_ctrl->tcon_tim_stb2 */ 00212 /* output_ctrl->tcon_tim_cpv2 */ 00213 #define VDC5_REG_MASK_0X00000007 (0x00000007u) /* mask *(output_pin_regaddr[tcon_pin]) */ 00214 /* img_synthesizer->gr_flm2 */ 00215 /* img_synthesizer->gr_flm2 */ 00216 #define VDC5_REG_MASK_0X07FF0000 (0x07FF0000u) /* mask scaler_0->scl0_frc4 */ 00217 /* scaler_1->scl0_frc4 */ 00218 /* img_synthesizer->gr_flm5 */ 00219 /* img_synthesizer->gr_flm6 */ 00220 #define VDC5_REG_MASK_0X0FFF0FFF (0x0FFF0FFFu) /* mask */ 00221 /* scaler->scl0_ds5, scaler->scl0_us6 */ 00222 /* scaler->scl0_ds5, scaler->scl0_us6 */ 00223 /* scaler->scl0_ds5, scaler->scl0_us6 */ 00224 /* scaler->scl0_ds5, scaler->scl0_us6 */ 00225 /* scaler->scl0_ds5, scaler->scl0_us6 */ 00226 #define VDC5_REG_MASK_0X00000003 (0x00000003u) /* mask img_synthesizer->gr_ab1 */ 00227 /* regaddr_lvds->lphyacc */ 00228 00229 00230 /****************************************************************************** 00231 Typedef definitions 00232 ******************************************************************************/ 00233 00234 /****************************************************************************** 00235 Private global variables and functions 00236 ******************************************************************************/ 00237 static void SetVideoExternalInput( 00238 const vdc5_ext_in_sig_t * const ext_sig_tmp, 00239 const vdc5_regaddr_input_ctrl_t * const input_ctrl); 00240 static void SetInitialConnection(const vdc5_channel_t ch); 00241 static void SetGrapicsBackgroundColor(const vdc5_channel_t ch); 00242 static void SetScalerBackgroundColor( 00243 const vdc5_channel_t ch, 00244 const vdc5_graphics_type_t graphics_id, 00245 const vdc5_color_space_t color_space); 00246 static void SetLcdTcon_STVA_VS( 00247 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00248 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00249 static void SetLcdTcon_STVB_VE( 00250 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00251 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00252 static void SetLcdTcon_STH_HS( 00253 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00254 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00255 static void SetLcdTcon_STB_HE( 00256 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00257 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00258 static void SetLcdTcon_CPV_GCK( 00259 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00260 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00261 static void SetLcdTcon_POLA( 00262 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00263 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00264 static void SetLcdTcon_POLB( 00265 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00266 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00267 static void SetLcdTcon_DE( 00268 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00269 const vdc5_regaddr_output_ctrl_t * const output_ctrl); 00270 static void SetScalingDown( 00271 const vdc5_scalingdown_rot_t * const scldw_rot, 00272 const vdc5_res_inter_t res_inter, 00273 const vdc5_regaddr_scaler_t * const scaler); 00274 static void SetScalingUp( 00275 const vdc5_width_read_fb_t * const w_read_fb, 00276 const vdc5_period_rect_t * const gr_grc, 00277 const vdc5_onoff_t adj_sel, 00278 const vdc5_onoff_t ip_conversion, 00279 const vdc5_wr_md_t res_ds_wr_md, 00280 const vdc5_gr_ln_off_dir_t ln_off_dir, 00281 const vdc5_regaddr_scaler_t * const scaler); 00282 static uint32_t CalcScalingRatio( 00283 const uint32_t before_scl, 00284 const uint32_t after_scl, 00285 const vdc5_onoff_t adj_sel, 00286 const vdc5_onoff_t round_up); 00287 static void SetInputCntrlColorMtx(const vdc5_channel_t ch, const vdc5_scaling_type_t scaling_id); 00288 static vdc5_onoff_t ConfirmGraphicsEnlargement( 00289 const vdc5_graphics_type_t graphics_id, 00290 const vdc5_gr_flm_sel_t gr_flm_sel, 00291 const vdc5_width_read_fb_t * const w_read_fb, 00292 const vdc5_period_rect_t * const gr_grc); 00293 static void SetScalerGraphics( 00294 const vdc5_channel_t ch, 00295 const vdc5_graphics_type_t graphics_id, 00296 const vdc5_width_read_fb_t * const w_read_fb, 00297 const vdc5_period_rect_t * const gr_grc, 00298 const vdc5_gr_flm_sel_t gr_flm_sel, 00299 const vdc5_onoff_t gr_enlarge); 00300 static uint32_t DisplayStartPixelSetting(const uint32_t gr_base, const vdc5_gr_format_t gr_format); 00301 static vdc5_onoff_t SetCascade( 00302 const vdc5_channel_t ch, 00303 const vdc5_graphics_type_t graphics_id, 00304 const vdc5_gr_flm_sel_t gr_flm_sel, 00305 const vdc5_onoff_t gr_enlarge); 00306 static void SetupGraphicsVIN( 00307 const vdc5_channel_t ch, 00308 const vdc5_graphics_type_t graphics_id, 00309 const vdc5_gr_flm_sel_t gr_flm_sel, 00310 const vdc5_onoff_t gr_enlarge); 00311 static void ChangeGraphicsVIN( 00312 const vdc5_channel_t ch, 00313 const vdc5_graphics_type_t graphics_id, 00314 const vdc5_gr_flm_sel_t gr_flm_sel, 00315 const vdc5_onoff_t gr_enlarge); 00316 static void SetImgQaImproverColorMtx( 00317 const vdc5_channel_t ch, 00318 const vdc5_graphics_type_t graphics_id, 00319 const vdc5_color_space_t color_space); 00320 00321 static void SetRwProcAbility_Write_0(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00322 static void SetRwProcAbility_Write_1(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00323 static void SetRwProcAbility_Write_OIR(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00324 static void SetRwProcAbility_Read_0(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00325 static void SetRwProcAbility_Read_1(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00326 static void SetRwProcAbility_Read_2(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00327 static void SetRwProcAbility_Read_3(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00328 static void SetRwProcAbility_Read_OIR(const vdc5_channel_t ch, const vdc5_onoff_t ability); 00329 static void SetRegUpdateRwEnable(const vdc5_channel_t ch, const vdc5_layer_id_t layer_id); 00330 00331 static void SetGraphicsDisplayMode( 00332 const vdc5_channel_t ch, 00333 const vdc5_layer_id_t layer_id, 00334 const vdc5_gr_disp_sel_t * const gr_disp_sel); 00335 static void SetColorMatrix( 00336 const vdc5_colormtx_mode_t mtx_mode, 00337 const uint16_t * const offset, 00338 const uint16_t * const gain, 00339 const vdc5_regaddr_color_matrix_t * const color_matrix); 00340 static void SetImageEnhancementSharpness( 00341 const vdc5_onoff_t shp_h_on, 00342 const vdc5_enhance_sharp_t * const sharp_param, 00343 const vdc5_regaddr_img_qlty_imp_t * const img_qlty_imp); 00344 static void SetImageEnhancementLti( 00345 const vdc5_onoff_t lti_h_on, 00346 const vdc5_enhance_lti_t * const lti_param, 00347 const vdc5_regaddr_img_qlty_imp_t * const img_qlty_imp); 00348 static void SetAlphaRectArea( 00349 const vdc5_pd_disp_rect_t * const pd_disp_rect, 00350 const vdc5_regaddr_img_synthesizer_t * const img_synthesizer); 00351 static void SetUndSel(const vdc5_channel_t ch, const vdc5_onoff_t und_sel); 00352 static uint32_t ColorConversion(const vdc5_gr_format_t gr_format, const uint32_t input_color); 00353 static uint32_t ColorConversionFrom4to8(const uint32_t color_value); 00354 static uint32_t ColorConversionFrom5to8(const uint32_t color_value); 00355 static uint32_t ColorConversionFrom6to8(const uint32_t color_value); 00356 static uint32_t ColorConversionIntoAgbr(const uint32_t color_value); 00357 static void Set_Clut(const vdc5_clut_t * const param, volatile uint32_t * regaddr_clut); 00358 static void Set_StartThreshold_Gamma(const uint8_t * gam_th, volatile uint32_t * const * const gam_area); 00359 static void Set_GainAdjustment_Gamma(const uint16_t * gam_gain, volatile uint32_t * const * const gam_lut); 00360 static void Wait_200_usec(void); 00361 00362 /*! Color matrix offset (DC) adjustment (YG, B, and R) */ 00363 static const uint16_t colormtx_offset_adj [VDC5_COLORMTX_OFFST_NUM ] = { 00364 (uint16_t)VDC5_COLORCONV_DC_OFFSET, (uint16_t)VDC5_COLORCONV_DC_OFFSET, (uint16_t)VDC5_COLORCONV_DC_OFFSET 00365 }; 00366 /*! Color matrix signal gain adjustment (GG, GB, GR, BG, BB, BR, RG, RB, and RR) */ 00367 static const uint16_t colormtx_gain_adj [VDC5_COLORMTX_MODE_NUM ][VDC5_COLORMTX_GAIN_NUM ] = { 00368 { /* GBR to GBR */ 00369 (uint16_t)VDC5_COLORCONV_1TIMES_GAIN, 0, 0, 00370 0, (uint16_t)VDC5_COLORCONV_1TIMES_GAIN, 0, 00371 0, 0, (uint16_t)VDC5_COLORCONV_1TIMES_GAIN 00372 }, 00373 { /* GBR to YCbCr */ 00374 (uint16_t)VDC5_COLORCONV_Y_G, (uint16_t)VDC5_COLORCONV_Y_B, (uint16_t)VDC5_COLORCONV_Y_R, 00375 (uint16_t)VDC5_COLORCONV_CB_G, (uint16_t)VDC5_COLORCONV_CB_B, (uint16_t)VDC5_COLORCONV_CB_R, 00376 (uint16_t)VDC5_COLORCONV_CR_G, (uint16_t)VDC5_COLORCONV_CR_B, (uint16_t)VDC5_COLORCONV_CR_R 00377 }, 00378 { /* YCbCr to GBR */ 00379 (uint16_t)VDC5_COLORCONV_G_Y, (uint16_t)VDC5_COLORCONV_G_CB, (uint16_t)VDC5_COLORCONV_G_CR, 00380 (uint16_t)VDC5_COLORCONV_B_Y, (uint16_t)VDC5_COLORCONV_B_CB, (uint16_t)VDC5_COLORCONV_B_CR, 00381 (uint16_t)VDC5_COLORCONV_R_Y, (uint16_t)VDC5_COLORCONV_R_CB, (uint16_t)VDC5_COLORCONV_R_CR 00382 }, 00383 { /* YCbCr to YCbCr */ 00384 (uint16_t)VDC5_COLORCONV_1TIMES_GAIN, 0, 0, 00385 0, (uint16_t)VDC5_COLORCONV_1TIMES_GAIN, 0, 00386 0, 0, (uint16_t)VDC5_COLORCONV_1TIMES_GAIN 00387 } 00388 }; 00389 00390 static void (* const rw_proc_function_tbl[VDC5_LAYER_ID_NUM ])(const vdc5_channel_t ch, const vdc5_onoff_t ability) = { 00391 &SetRwProcAbility_Write_0, /* Write scaler 0 */ 00392 &SetRwProcAbility_Write_1, /* Write scaler 1 */ 00393 &SetRwProcAbility_Write_OIR, /* Write OIR */ 00394 &SetRwProcAbility_Read_0, /* Read graphics 0 */ 00395 &SetRwProcAbility_Read_1, /* Read graphics 1 */ 00396 &SetRwProcAbility_Read_2, /* Read graphics 2 */ 00397 &SetRwProcAbility_Read_3, /* Read graphics 3 */ 00398 0, /* Read VIN */ 00399 &SetRwProcAbility_Read_OIR /* Read OIR */ 00400 }; 00401 00402 00403 /**************************************************************************//** 00404 * @brief Sets registers for initialization 00405 * @param[in] ch : Channel 00406 * @param[in] param : Initialization parameter 00407 * @retval None 00408 *****************************************************************************/ 00409 void VDC5_Initialize (const vdc5_channel_t ch, const vdc5_init_t * const param) 00410 { 00411 const vdc5_lvds_t * lvds_tmp; 00412 const vdc5_regaddr_lvds_t * regaddr_lvds; 00413 volatile uint32_t counter; 00414 uint16_t syscnt_panel_clk_tmp; 00415 volatile uint16_t * syscnt_panel_clk_reg; 00416 volatile uint32_t * inp_sel_cnt_reg; 00417 static const uint16_t panel_dcdr_tbl[VDC5_PANEL_CLKDIV_NUM ] = { 00418 /* Panel clock frequency division ratio */ 00419 (uint16_t)VDC5_REG_CLKDIV_1_1, (uint16_t)VDC5_REG_CLKDIV_1_2, (uint16_t)VDC5_REG_CLKDIV_1_3, (uint16_t)VDC5_REG_CLKDIV_1_4, 00420 (uint16_t)VDC5_REG_CLKDIV_1_5, (uint16_t)VDC5_REG_CLKDIV_1_6, (uint16_t)VDC5_REG_CLKDIV_1_7, (uint16_t)VDC5_REG_CLKDIV_1_8, 00421 (uint16_t)VDC5_REG_CLKDIV_1_9, (uint16_t)VDC5_REG_CLKDIV_1_12, (uint16_t)VDC5_REG_CLKDIV_1_16, (uint16_t)VDC5_REG_CLKDIV_1_24, 00422 (uint16_t)VDC5_REG_CLKDIV_1_32 00423 }; 00424 static const uint16_t panel_icksel_tbl[VDC5_PANEL_ICKSEL_NUM ] = { 00425 /* Panel clock select */ 00426 (uint16_t)VDC5_REG_ICKSEL_IMG, (uint16_t)VDC5_REG_ICKSEL_IMG_DV, (uint16_t)VDC5_REG_ICKSEL_EXT_0, (uint16_t)VDC5_REG_ICKSEL_EXT_1, 00427 (uint16_t)VDC5_REG_ICKSEL_PERI, (uint16_t)VDC5_REG_ICKSEL_LVDS, (uint16_t)VDC5_REG_ICKSEL_LVDS_DIV7 00428 }; 00429 00430 syscnt_panel_clk_reg = vdc5_regaddr_system_ctrl[ch].syscnt_panel_clk; 00431 /* Disable */ 00432 syscnt_panel_clk_tmp = (uint16_t)((uint32_t)*syscnt_panel_clk_reg & (~VDC5_REG_BIT8)); 00433 *syscnt_panel_clk_reg = syscnt_panel_clk_tmp; 00434 00435 /* LVDS */ 00436 lvds_tmp = param->lvds ; 00437 if (lvds_tmp != NULL) { 00438 regaddr_lvds = &vdc5_regaddr_lvds; 00439 00440 /* Output from the LVDS PLL is disabled. */ 00441 *(regaddr_lvds->lclkselr) &= (uint32_t)~VDC5_REG_BIT4; 00442 /* Controls power-down for the LVDS PLL: Power-down state */ 00443 *(regaddr_lvds->lpllsetr) |= (uint32_t)VDC5_REG_BIT0; 00444 for (counter = 0; counter < (uint32_t)VDC5_LVDS_PLL_WAIT_CYCLE; counter++) { 00445 /* This is a delay (1 usec) while waiting for PLL PD to settle. */ 00446 } 00447 00448 /* LPHYACC */ 00449 *(regaddr_lvds->lphyacc) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 00450 *(regaddr_lvds->lphyacc) |= LVDS_LPHYACC_VALUE; 00451 /* LCLKSELR: LVDS clock select register */ 00452 *(regaddr_lvds->lclkselr) &= (uint32_t)~VDC5_REG_MASK_0X0703FF02; 00453 /* The clock input to frequency divider 1 */ 00454 *(regaddr_lvds->lclkselr) |= (uint32_t)lvds_tmp->lvds_in_clk_sel << VDC5_REG_SHIFT_24; 00455 /* The frequency dividing value (NIDIV) for frequency divider 1 */ 00456 *(regaddr_lvds->lclkselr) |= (uint32_t)lvds_tmp->lvds_idiv_set << VDC5_REG_SHIFT_16; 00457 /* Internal parameter setting for LVDS PLL */ 00458 *(regaddr_lvds->lclkselr) |= (uint32_t)lvds_tmp->lvdspll_tst << VDC5_REG_SHIFT_10; 00459 /* The frequency dividing value (NODIV) for frequency divider 2 */ 00460 *(regaddr_lvds->lclkselr) |= (uint32_t)lvds_tmp->lvds_odiv_set << VDC5_REG_SHIFT_8; 00461 if (lvds_tmp->lvds_vdc_sel != VDC5_CHANNEL_0 ) { 00462 /* A channel in VDC5 whose data is to be output through the LVDS */ 00463 *(regaddr_lvds->lclkselr) |= (uint32_t)VDC5_REG_BIT1; 00464 } 00465 00466 /* LPLLSETR: LVDS PLL setting register */ 00467 *(regaddr_lvds->lpllsetr) &= (uint32_t)~VDC5_REG_MASK_0X07FF1F30; 00468 /* The frequency dividing value (NFD) for the feedback frequency */ 00469 *(regaddr_lvds->lpllsetr) |= (uint32_t)lvds_tmp->lvdspll_fd << VDC5_REG_SHIFT_16; 00470 /* The frequency dividing value (NRD) for the input frequency */ 00471 *(regaddr_lvds->lpllsetr) |= (uint32_t)lvds_tmp->lvdspll_rd << VDC5_REG_SHIFT_8; 00472 /* The frequency dividing value (NOD) for the output frequency */ 00473 *(regaddr_lvds->lpllsetr) |= (uint32_t)lvds_tmp->lvdspll_od << VDC5_REG_SHIFT_4; 00474 00475 for (counter = 0; counter < (uint32_t)VDC5_LVDS_PLL_WAIT_CYCLE; counter++) { 00476 /* This is a delay (1 usec) while waiting for PLL PD to settle. */ 00477 } 00478 /* Controls power-down for the LVDS PLL: Normal operation */ 00479 *(regaddr_lvds->lpllsetr) &= (uint32_t)~VDC5_REG_BIT0; 00480 00481 /* Wait for 200 usec. */ 00482 Wait_200_usec(); 00483 00484 /* Output from the LVDS PLL is enabled. */ 00485 *(regaddr_lvds->lclkselr) |= (uint32_t)VDC5_REG_BIT4; 00486 } 00487 00488 inp_sel_cnt_reg = vdc5_regaddr_input_ctrl[ch].inp_sel_cnt; 00489 /* Input select */ 00490 if (param->panel_icksel == VDC5_PANEL_ICKSEL_IMG_DV ) { 00491 *inp_sel_cnt_reg |= (uint32_t)VDC5_REG_BIT20; 00492 } else { 00493 *inp_sel_cnt_reg &= (uint32_t)~VDC5_REG_BIT20; 00494 } 00495 syscnt_panel_clk_tmp = panel_dcdr_tbl[param->panel_dcdr ]; 00496 syscnt_panel_clk_tmp = (uint16_t)((uint32_t)syscnt_panel_clk_tmp | 00497 (uint32_t)panel_icksel_tbl[param->panel_icksel ]); 00498 *syscnt_panel_clk_reg = syscnt_panel_clk_tmp; 00499 /* Enable */ 00500 syscnt_panel_clk_tmp = (uint16_t)((uint32_t)syscnt_panel_clk_tmp | VDC5_REG_BIT8); 00501 *syscnt_panel_clk_reg = syscnt_panel_clk_tmp; 00502 00503 } /* End of function VDC5_Initialize() */ 00504 00505 /**************************************************************************//** 00506 * @brief Sets registers for termination 00507 * @param[in] ch : Channel 00508 * @retval None 00509 ******************************************************************************/ 00510 void VDC5_Terminate (const vdc5_channel_t ch) 00511 { 00512 volatile uint16_t * syscnt_panel_clk_reg; 00513 uint16_t syscnt_panel_clk_tmp; 00514 vdc5_resource_state_t rsrc_state; 00515 vdc5_onoff_t lvds_ref; 00516 const vdc5_regaddr_lvds_t * regaddr_lvds; 00517 volatile uint32_t counter; 00518 00519 syscnt_panel_clk_reg = vdc5_regaddr_system_ctrl[ch].syscnt_panel_clk; 00520 /* Disable */ 00521 syscnt_panel_clk_tmp = (uint16_t)((uint32_t)*syscnt_panel_clk_reg & (~VDC5_REG_BIT8)); 00522 *syscnt_panel_clk_reg = syscnt_panel_clk_tmp; 00523 00524 rsrc_state = VDC5_ShrdPrmGetResource(ch, VDC5_RESOURCE_LVDS_CLK ); 00525 if (rsrc_state != VDC5_RESOURCE_ST_INVALID ) { /* LVDS PLL clock is set. */ 00526 lvds_ref = VDC5_ShrdPrmGetLvdsClkRef(); 00527 if (lvds_ref == VDC5_OFF ) { /* LVDS PLL clock is not referred. */ 00528 regaddr_lvds = &vdc5_regaddr_lvds; 00529 00530 /* Output from the LVDS PLL is disabled. */ 00531 *(regaddr_lvds->lclkselr) &= (uint32_t)~VDC5_REG_BIT4; 00532 /* Controls power-down for the LVDS PLL: Power-down state */ 00533 *(regaddr_lvds->lpllsetr) |= (uint32_t)VDC5_REG_BIT0; 00534 for (counter = 0; counter < (uint32_t)VDC5_LVDS_PLL_WAIT_CYCLE; counter++) { 00535 /* This is a delay (1 usec) while waiting for PLL PD to settle. */ 00536 } 00537 } 00538 } 00539 } /* End of function VDC5_Terminate() */ 00540 00541 /**************************************************************************//** 00542 * @brief Sets registers for video input 00543 * @param[in] ch : Channel 00544 * @param[in] param : Video input setup parameter 00545 * @retval None 00546 ******************************************************************************/ 00547 void VDC5_VideoInput (const vdc5_channel_t ch, const vdc5_input_t * const param) 00548 { 00549 const vdc5_regaddr_input_ctrl_t * input_ctrl; 00550 const vdc5_sync_delay_t * dly_tmp; 00551 const vdc5_ext_in_sig_t * ext_sig_tmp; 00552 00553 input_ctrl = &vdc5_regaddr_input_ctrl[ch]; 00554 00555 /* Input select */ 00556 if (param->inp_sel == VDC5_INPUT_SEL_VDEC ) { 00557 *(input_ctrl->inp_sel_cnt) &= (uint32_t)~VDC5_REG_BIT20; 00558 } else { 00559 *(input_ctrl->inp_sel_cnt) |= (uint32_t)VDC5_REG_BIT20; 00560 } 00561 /* Vsync signal 1/2fH and 1/4fH phase timing */ 00562 *(input_ctrl->inp_vsync_ph_adj) = (uint32_t)param->inp_fh50 << VDC5_REG_SHIFT_16; 00563 *(input_ctrl->inp_vsync_ph_adj) |= (uint32_t)param->inp_fh25 ; 00564 00565 /* Sync signal delay adjustment */ 00566 dly_tmp = param->dly ; 00567 if (dly_tmp != NULL) { 00568 /* Number of lines for delaying Vsync signal and field differentiation signal */ 00569 *(input_ctrl->inp_dly_adj) = (uint32_t)dly_tmp->inp_vs_dly_l << VDC5_REG_SHIFT_24; 00570 /* Field differentiation signal delay amount */ 00571 *(input_ctrl->inp_dly_adj) |= (uint32_t)dly_tmp->inp_fld_dly << VDC5_REG_SHIFT_16; 00572 /* Vsync signal delay amount */ 00573 *(input_ctrl->inp_dly_adj) |= (uint32_t)dly_tmp->inp_vs_dly << VDC5_REG_SHIFT_8; 00574 /* Hsync signal delay amount */ 00575 *(input_ctrl->inp_dly_adj) |= (uint32_t)dly_tmp->inp_hs_dly ; 00576 } 00577 00578 /* Horizontal noise reduction operating mode, Y/Cb/Cr mode */ 00579 *(input_ctrl->imgcnt_nr_cnt0) |= (uint32_t)VDC5_REG_BIT20; 00580 00581 /* External input signal */ 00582 ext_sig_tmp = param->ext_sig ; 00583 if (ext_sig_tmp != NULL) { 00584 /* Setting external input video signal */ 00585 SetVideoExternalInput(ext_sig_tmp, input_ctrl); 00586 00587 /* Horizontal noise reduction operating mode, G/B/R mode */ 00588 if ((ext_sig_tmp->inp_format == VDC5_EXTIN_FORMAT_RGB888 ) || 00589 (ext_sig_tmp->inp_format == VDC5_EXTIN_FORMAT_RGB666 ) || 00590 (ext_sig_tmp->inp_format == VDC5_EXTIN_FORMAT_RGB565 )) { 00591 *(input_ctrl->imgcnt_nr_cnt0) &= (uint32_t)~VDC5_REG_BIT20; 00592 } 00593 } 00594 00595 /* Register update control register (INP_UPDATE) 00596 b4 INP_EXT_UPDATE 00597 b0 INP_IMG_UPDATE */ 00598 *(input_ctrl->inp_update) |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 00599 /* Register update control register (IMGCNT_UPDATE) 00600 b0 IMGCNT_VEN */ 00601 *(input_ctrl->imgcnt_update) |= (uint32_t)VDC5_REG_BIT0; 00602 } /* End of function VDC5_VideoInput() */ 00603 00604 /**************************************************************************//** 00605 * @brief Sets registers for sync control 00606 * @param[in] ch : Channel 00607 * @param[in] param : Sync signal control parameter 00608 * @retval None 00609 ******************************************************************************/ 00610 void VDC5_SyncControl (const vdc5_channel_t ch, const vdc5_sync_ctrl_t * const param) 00611 { 00612 const vdc5_regaddr_scaler_t * scaler_0; 00613 const vdc5_regaddr_scaler_t * scaler_1; 00614 const vdc5_regaddr_scaler_t * scaler_oir; 00615 uint32_t full_scr; 00616 00617 scaler_0 = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ]; 00618 scaler_1 = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ]; 00619 scaler_oir = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ]; 00620 00621 if (param->vsync_cpmpe != NULL) { 00622 /* Frequent Vsync signal masking period */ 00623 *(scaler_0->scl0_frc1) = (uint32_t)param->vsync_cpmpe ->res_vmask << VDC5_REG_SHIFT_16; 00624 *(scaler_1->scl0_frc1) = (uint32_t)param->vsync_cpmpe ->res_vmask << VDC5_REG_SHIFT_16; 00625 /* Missing-Sync compensating pulse output wait time */ 00626 *(scaler_0->scl0_frc2) = (uint32_t)param->vsync_cpmpe ->res_vlack << VDC5_REG_SHIFT_16; 00627 *(scaler_1->scl0_frc2) = (uint32_t)param->vsync_cpmpe ->res_vlack << VDC5_REG_SHIFT_16; 00628 /* Frequent Vsync signal masking and missing Vsync signal compensation */ 00629 *(scaler_0->scl0_frc1) |= (uint32_t)VDC5_REG_BIT0; 00630 *(scaler_1->scl0_frc1) |= (uint32_t)VDC5_REG_BIT0; 00631 *(scaler_0->scl0_frc2) |= (uint32_t)VDC5_REG_BIT0; 00632 *(scaler_1->scl0_frc2) |= (uint32_t)VDC5_REG_BIT0; 00633 } else { 00634 /* Frequent Vsync signal masking and missing Vsync signal compensation are disabled, if unnecessary. */ 00635 *(scaler_0->scl0_frc1) &= (uint32_t)~VDC5_REG_BIT0; 00636 *(scaler_1->scl0_frc1) &= (uint32_t)~VDC5_REG_BIT0; 00637 *(scaler_0->scl0_frc2) &= (uint32_t)~VDC5_REG_BIT0; 00638 *(scaler_0->scl0_frc2) |= (uint32_t)VDC5_REG_BIT_0XFFFF0000; 00639 *(scaler_1->scl0_frc2) &= (uint32_t)~VDC5_REG_BIT0; 00640 *(scaler_1->scl0_frc2) |= (uint32_t)VDC5_REG_BIT_0XFFFF0000; 00641 } 00642 /* In OIR, frequent Vsync signal masking and missing Vsync signal compensation are set to off. */ 00643 *(scaler_oir->scl0_frc1) &= (uint32_t)~VDC5_REG_BIT0; 00644 *(scaler_oir->scl0_frc2) &= (uint32_t)~VDC5_REG_BIT0; 00645 00646 /* Hsync period setting and free-running Vsync period initialization */ 00647 *(scaler_0->scl0_frc4) = (uint32_t)param->res_fh ; 00648 *(scaler_1->scl0_frc4) = (uint32_t)param->res_fh ; 00649 *(scaler_oir->scl0_frc4) = (uint32_t)param->res_fh ; 00650 /* Vsync signal output select (free-running Vsync on/off control) */ 00651 if (param->res_vs_sel == VDC5_OFF ) { 00652 *(scaler_0->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT0; 00653 *(scaler_1->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT0; 00654 } else { 00655 *(scaler_0->scl0_frc3) |= (uint32_t)VDC5_REG_BIT0; 00656 *(scaler_1->scl0_frc3) |= (uint32_t)VDC5_REG_BIT0; 00657 *(scaler_0->scl0_frc4) &= (uint32_t)~VDC5_REG_MASK_0X07FF0000; 00658 *(scaler_0->scl0_frc4) |= (uint32_t)param->res_fv << VDC5_REG_SHIFT_16; 00659 *(scaler_1->scl0_frc4) &= (uint32_t)~VDC5_REG_MASK_0X07FF0000; 00660 *(scaler_1->scl0_frc4) |= (uint32_t)param->res_fv << VDC5_REG_SHIFT_16; 00661 } 00662 /* In OIR, external input Vsync signal is selected automatically. */ 00663 *(scaler_oir->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT0; 00664 00665 /* Sync signal output and full-screen enable signal select: Scaler 0 */ 00666 *(scaler_0->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT8; 00667 *(scaler_1->scl0_frc3) |= (uint32_t)VDC5_REG_BIT8; 00668 00669 /* Vsync signal delay control */ 00670 *(scaler_0->scl0_frc5) &= (uint32_t)~VDC5_REG_MASK_0X000000FF; 00671 *(scaler_0->scl0_frc5) |= (uint32_t)param->res_vsdly ; 00672 *(scaler_1->scl0_frc5) &= (uint32_t)~VDC5_REG_MASK_0X000000FF; 00673 *(scaler_1->scl0_frc5) |= (uint32_t)param->res_vsdly ; 00674 *(scaler_oir->scl0_frc5) &= (uint32_t)~VDC5_REG_MASK_0X000000FF; 00675 *(scaler_oir->scl0_frc5) |= OIR_VSYNC_DELAY; 00676 00677 /* Full-screen enable signal */ 00678 full_scr = (uint32_t)param->res_f .vs << VDC5_REG_SHIFT_16; 00679 full_scr |= (uint32_t)param->res_f .vw ; 00680 00681 *(scaler_0->scl0_frc6) = full_scr; 00682 *(scaler_1->scl0_frc6) = full_scr; 00683 *(scaler_oir->scl0_frc6) = full_scr; 00684 00685 full_scr = (uint32_t)param->res_f .hs << VDC5_REG_SHIFT_16; 00686 full_scr |= (uint32_t)param->res_f .hw ; 00687 00688 *(scaler_0->scl0_frc7) = full_scr; 00689 *(scaler_1->scl0_frc7) = full_scr; 00690 *(scaler_oir->scl0_frc7) = full_scr; 00691 00692 /* Register update control register (SC0_SCL0_UPDATE, SC1_SCL0_UPDATE, OIR_SCL0_UPDATE) 00693 b8 SCL0_UPDATE 00694 b4 SCL0_VEN_B */ 00695 *(scaler_0->scl0_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 00696 *(scaler_1->scl0_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 00697 *(scaler_oir->scl0_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 00698 00699 SetInitialConnection(ch); 00700 00701 VDC5_ShrdPrmSetUndSel(ch, VDC5_OFF ); 00702 } /* End of function VDC5_SyncControl() */ 00703 00704 /**************************************************************************//** 00705 * @brief Sets registers for display output 00706 * @param[in] ch : Channel 00707 * @param[in] param : Display output configuration parameter 00708 * @retval None 00709 ******************************************************************************/ 00710 void VDC5_DisplayOutput (const vdc5_channel_t ch, const vdc5_output_t * const param) 00711 { 00712 const vdc5_regaddr_output_ctrl_t * output_ctrl; 00713 volatile uint32_t * output_pin_regaddr[VDC5_LCD_TCON_PIN_NUM]; 00714 uint32_t tcon_sig_index; 00715 const vdc5_lcd_tcon_timing_t * const * lcd_tcon_timing; 00716 static void (* const tcon_function_tbl[VDC5_LCD_TCONSIG_NUM])( 00717 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 00718 const vdc5_regaddr_output_ctrl_t * const output_ctrl) = { 00719 &SetLcdTcon_STVA_VS, /* STVA/VS */ 00720 &SetLcdTcon_STVB_VE, /* STVB/VE */ 00721 &SetLcdTcon_STH_HS, /* STH_HS */ 00722 &SetLcdTcon_STB_HE, /* STB_HE */ 00723 &SetLcdTcon_CPV_GCK, /* CPV_GCK */ 00724 &SetLcdTcon_POLA, /* POLA */ 00725 &SetLcdTcon_POLB, /* POLB */ 00726 &SetLcdTcon_DE /* DE */ 00727 }; 00728 const uint32_t output_pin_edge[VDC5_LCD_TCON_PIN_NUM] = { 00729 VDC5_REG_TCON0_EDGE, VDC5_REG_TCON1_EDGE, VDC5_REG_TCON2_EDGE, VDC5_REG_TCON3_EDGE, 00730 VDC5_REG_TCON4_EDGE, VDC5_REG_TCON5_EDGE, VDC5_REG_TCON6_EDGE 00731 }; 00732 vdc5_lcd_tcon_pin_t tcon_pin_tmp; 00733 const vdc5_gr_disp_sel_t gr_disp_sel_tbl[VDC5_GR_TYPE_NUM ] = { 00734 /* Graphics display mode for initialization */ 00735 VDC5_DISPSEL_BACK , /* Graphics 0 */ 00736 VDC5_DISPSEL_LOWER , /* Graphics 1 */ 00737 VDC5_DISPSEL_LOWER , /* Graphics 2 */ 00738 VDC5_DISPSEL_LOWER , /* Graphics 3 */ 00739 VDC5_DISPSEL_IGNORED , /* VIN */ 00740 VDC5_DISPSEL_BACK /* OIR */ 00741 }; 00742 const uint32_t dither_format[VDC5_LCD_OUTFORMAT_NUM] = { 00743 /* Panel dither output format select */ 00744 VDC5_REG_PATH_FORMAT_RGB888, VDC5_REG_PATH_FORMAT_RGB666, 00745 VDC5_REG_PATH_FORMAT_RGB565, VDC5_REG_PATH_FORMAT_NON 00746 }; 00747 00748 output_ctrl = &vdc5_regaddr_output_ctrl[ch]; 00749 00750 /* 1/2fH timing */ 00751 *(output_ctrl->tcon_tim) = (uint32_t)param->tcon_half << VDC5_REG_SHIFT_16; 00752 /* Offset Hsync signal timing */ 00753 *(output_ctrl->tcon_tim) |= (uint32_t)param->tcon_offset ; 00754 00755 /* Create output pin register address table */ 00756 output_pin_regaddr[VDC5_LCD_TCON_PIN_0 ] = output_ctrl->tcon_tim_stva2; 00757 output_pin_regaddr[VDC5_LCD_TCON_PIN_1 ] = output_ctrl->tcon_tim_stvb2; 00758 output_pin_regaddr[VDC5_LCD_TCON_PIN_2 ] = output_ctrl->tcon_tim_sth2; 00759 output_pin_regaddr[VDC5_LCD_TCON_PIN_3 ] = output_ctrl->tcon_tim_stb2; 00760 output_pin_regaddr[VDC5_LCD_TCON_PIN_4 ] = output_ctrl->tcon_tim_cpv2; 00761 output_pin_regaddr[VDC5_LCD_TCON_PIN_5 ] = output_ctrl->tcon_tim_pola2; 00762 output_pin_regaddr[VDC5_LCD_TCON_PIN_6 ] = output_ctrl->tcon_tim_polb2; 00763 00764 /* Clear output phase control of LCT_TCON0~6 pin */ 00765 *(output_ctrl->out_clk_phase) &= (uint32_t)~VDC5_REG_MASK_0X0000007F; 00766 /* LCD TCON */ 00767 lcd_tcon_timing = param->outctrl ; 00768 for (tcon_sig_index = 0; tcon_sig_index < VDC5_LCD_TCONSIG_NUM; tcon_sig_index++) { 00769 if (*lcd_tcon_timing != NULL) { 00770 /* Setting LCD TCON */ 00771 tcon_function_tbl[tcon_sig_index](*lcd_tcon_timing, output_ctrl); 00772 00773 tcon_pin_tmp = (*lcd_tcon_timing)->tcon_pin ; 00774 if (tcon_pin_tmp != VDC5_LCD_TCON_PIN_NON ) { 00775 /* LCD TCON output pin select */ 00776 *(output_pin_regaddr[tcon_pin_tmp]) &= (uint32_t)~VDC5_REG_MASK_0X00000007; 00777 *(output_pin_regaddr[tcon_pin_tmp]) |= tcon_sig_index; 00778 /* Output phase control of LCT_TCON pin */ 00779 if ((*lcd_tcon_timing)->outcnt_edge != VDC5_EDGE_RISING ) { 00780 *(output_ctrl->out_clk_phase) |= output_pin_edge[tcon_pin_tmp]; 00781 } 00782 } 00783 } 00784 lcd_tcon_timing++; 00785 } 00786 00787 *(output_ctrl->out_set) &= (uint32_t)~VDC5_REG_MASK_0X11003313; 00788 /* Bit endian change ON/OFF control */ 00789 if (param->out_endian_on != VDC5_OFF ) { 00790 *(output_ctrl->out_set) |= (uint32_t)VDC5_REG_BIT28; 00791 } 00792 /* B/R signal swap ON/OFF control */ 00793 if (param->out_swap_on != VDC5_OFF ) { 00794 *(output_ctrl->out_set) |= (uint32_t)VDC5_REG_BIT24; 00795 } 00796 /* Output format select */ 00797 *(output_ctrl->out_set) |= (uint32_t)param->out_format << VDC5_REG_SHIFT_12; 00798 00799 /* Panel dither output format select */ 00800 *(output_ctrl->out_pdtha) &= (uint32_t)~VDC5_REG_MASK_0X00030000; 00801 *(output_ctrl->out_pdtha) |= dither_format[param->out_format ]; 00802 00803 if (param->out_format == VDC5_LCD_OUTFORMAT_SERIAL_RGB ) { 00804 /* Clock frequency control */ 00805 *(output_ctrl->out_set) |= (uint32_t)param->out_frq_sel << VDC5_REG_SHIFT_8; 00806 /* Scan direction select */ 00807 if (param->out_dir_sel != VDC5_LCD_SERIAL_SCAN_FORWARD ) { 00808 *(output_ctrl->out_set) |= (uint32_t)VDC5_REG_BIT4; 00809 } 00810 /* Clock phase adjustment */ 00811 *(output_ctrl->out_set) |= (uint32_t)param->out_phase ; 00812 } else { 00813 /* Clock frequency control */ 00814 *(output_ctrl->out_set) |= (uint32_t)VDC5_LCD_PARALLEL_CLKFRQ_1 << VDC5_REG_SHIFT_8; 00815 } 00816 00817 /* Output phase control of LCD_DATA23 to LCD_DATA0 pin */ 00818 if (param->outcnt_lcd_edge == VDC5_EDGE_RISING ) { 00819 *(output_ctrl->out_clk_phase) &= (uint32_t)~VDC5_REG_BIT8; 00820 } else { 00821 *(output_ctrl->out_clk_phase) |= (uint32_t)VDC5_REG_BIT8; 00822 } 00823 00824 /* Set background color */ 00825 SetGrapicsBackgroundColor(ch); 00826 /* Initialize graphics display mode */ 00827 SetGraphicsDisplayMode(ch, VDC5_LAYER_ID_ALL , gr_disp_sel_tbl); 00828 00829 /* TCON register update control register (TCON_UPDATE) 00830 b0 TCON_VEN */ 00831 *(output_ctrl->tcon_update) |= (uint32_t)VDC5_REG_BIT0; 00832 /* Register update control register (OUT_UPDATE) 00833 b0 OUTCNT_VEN */ 00834 *(output_ctrl->out_update) |= (uint32_t)VDC5_REG_BIT0; 00835 00836 } /* End of function VDC5_DisplayOutput() */ 00837 00838 /**************************************************************************//** 00839 * @brief Sets registers for data write control processing 00840 * @param[in] ch : Channel 00841 * @param[in] scaling_id : Scaling type ID 00842 * @param[in] param : Data write control parameter 00843 * @retval None 00844 ******************************************************************************/ 00845 void VDC5_WriteDataControl ( 00846 const vdc5_channel_t ch, 00847 const vdc5_scaling_type_t scaling_id, 00848 const vdc5_write_t * const param) 00849 { 00850 const vdc5_regaddr_scaler_t * scaler; 00851 const vdc5_scalingdown_rot_t * scldw_rot; 00852 00853 scaler = &vdc5_regaddr_scaler[ch][scaling_id]; 00854 scldw_rot = ¶m->scalingdown_rot ; 00855 00856 /* Image area to be captured */ 00857 *(scaler->scl0_ds2) = (uint32_t)scldw_rot->res .vs << VDC5_REG_SHIFT_16; 00858 *(scaler->scl0_ds2) |= (uint32_t)scldw_rot->res .vw ; 00859 *(scaler->scl0_ds3) = (uint32_t)scldw_rot->res .hs << VDC5_REG_SHIFT_16; 00860 *(scaler->scl0_ds3) |= (uint32_t)scldw_rot->res .hw ; 00861 00862 *(scaler->scl1_wr1) &= (uint32_t)~VDC5_REG_MASK_0X0007000D; 00863 /* Swap setting in frame buffer writing */ 00864 *(scaler->scl1_wr1) |= (uint32_t)param->res_wrswa << VDC5_REG_SHIFT_16; 00865 /* Frame buffer video-signal writing format */ 00866 *(scaler->scl1_wr1) |= (uint32_t)param->res_md << VDC5_REG_SHIFT_2; 00867 /* Transfer burst length for frame buffer writing */ 00868 if (param->res_bst_md != VDC5_BST_MD_32BYTE ) { 00869 *(scaler->scl1_wr1) |= (uint32_t)VDC5_REG_BIT0; 00870 } 00871 00872 /* Frame buffer base address */ 00873 *(scaler->scl1_wr2) = (uint32_t)param->base ; 00874 /* Frame buffer line offset address[byte] */ 00875 *(scaler->scl1_wr3) = param->ln_off << VDC5_REG_SHIFT_16; 00876 /* Number of frames of buffer to be written to (res_flm_num + 1) */ 00877 *(scaler->scl1_wr3) |= param->flm_num ; 00878 /* Frame buffer frame offset address [byte] */ 00879 if (param->flm_num != 0u) { 00880 *(scaler->scl1_wr4) = param->flm_off ; 00881 } 00882 00883 /* Writing rate */ 00884 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_MASK_0X00000300; 00885 *(scaler->scl1_wr5) |= (uint32_t)param->res_fs_rate << VDC5_REG_SHIFT_8; 00886 00887 /* Dither correction on/off */ 00888 if (param->res_dth_on == VDC5_OFF ) { 00889 *(scaler->scl1_wr6) &= (uint32_t)~VDC5_REG_BIT4; 00890 } else { 00891 *(scaler->scl1_wr6) |= (uint32_t)VDC5_REG_BIT4; 00892 } 00893 /* Bit Reduction */ 00894 if (param->res_md == VDC5_RES_MD_RGB565 ) { 00895 /* On */ 00896 *(scaler->scl1_wr6) |= (uint32_t)VDC5_REG_BIT0; 00897 } else { 00898 /* Off */ 00899 *(scaler->scl1_wr6) &= (uint32_t)~VDC5_REG_BIT0; 00900 } 00901 00902 if (scaling_id == VDC5_SC_TYPE_OIR ) { 00903 /* OIR */ 00904 /* Vertical and horizontal width after scaling-down control block */ 00905 *(scaler->scl0_ds7) = (uint32_t)scldw_rot->res .vw << VDC5_REG_SHIFT_16; 00906 *(scaler->scl0_ds7) |= (uint32_t)scldw_rot->res .hw ; 00907 00908 /* Vertical scaling-down and horizontal scaling-down : OFF */ 00909 *(scaler->scl0_ds1) &= (uint32_t)~VDC5_REG_MASK_0X00000011; 00910 00911 /* Field operating mode select is fixed to progressive */ 00912 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT12; 00913 } else { 00914 /* Scaler 0 or 1 */ 00915 /* Field determination signal delay control */ 00916 if (param->flm_num == 0u) { 00917 /* Frame buffer: One plane */ 00918 *(scaler->scl0_frc5) &= (uint32_t)~VDC5_REG_BIT8; 00919 } else { 00920 /* Frame buffer: Two planes or more */ 00921 *(scaler->scl0_frc5) |= (uint32_t)VDC5_REG_BIT8; 00922 } 00923 00924 /* Horizontal prefilter */ 00925 if (scldw_rot->res_pfil_sel == VDC5_OFF ) { 00926 *(scaler->scl0_ds4) &= (uint32_t)~VDC5_REG_BIT29; 00927 } else { 00928 *(scaler->scl0_ds4) |= (uint32_t)VDC5_REG_BIT29; 00929 } 00930 /* Number of valid lines in vertical direction output by scaling-down control block */ 00931 *(scaler->scl0_ds7) = (uint32_t)scldw_rot->res_out_vw << VDC5_REG_SHIFT_16; 00932 /* Number of valid horizontal pixels output by scaling-down control block */ 00933 *(scaler->scl0_ds7) |= (uint32_t)scldw_rot->res_out_hw ; 00934 00935 /* Scaling-down */ 00936 SetScalingDown(scldw_rot, param->res_inter , scaler); 00937 00938 /* Frame buffer writing mode for image processing */ 00939 *(scaler->scl1_wr1) &= (uint32_t)~VDC5_REG_MASK_0X00000070; 00940 *(scaler->scl1_wr1) |= (uint32_t)scldw_rot->res_ds_wr_md << VDC5_REG_SHIFT_4; 00941 00942 /* Field operating mode select */ 00943 if (param->res_inter == VDC5_RES_INTER_PROGRESSIVE ) { 00944 /* Progressive */ 00945 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT12; 00946 } else { 00947 /* Interlace */ 00948 *(scaler->scl1_wr5) |= (uint32_t)VDC5_REG_BIT12; 00949 } 00950 /* Write field select */ 00951 if (param->res_fld_sel == VDC5_RES_FLD_SEL_TOP ) { 00952 /* Top field */ 00953 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT4; 00954 } else { 00955 /* Bottom field */ 00956 *(scaler->scl1_wr5) |= (uint32_t)VDC5_REG_BIT4; 00957 } 00958 00959 /* Frame buffer for bottom */ 00960 if (param->btm_base != NULL) { 00961 /* SC_RES_TB_ADD_MOD */ 00962 *(scaler->scl1_wr1) |= (uint32_t)VDC5_REG_MASK_0X00000080; 00963 00964 /* Frame buffer base address */ 00965 *(scaler->scl1_wr8) = (uint32_t)param->btm_base ; 00966 /* Frame buffer line offset address[byte] */ 00967 *(scaler->scl1_wr9) = param->ln_off << VDC5_REG_SHIFT_16; 00968 /* Number of frames of buffer to be written to (defined by res_flm_num + 1) */ 00969 *(scaler->scl1_wr9) |= param->flm_num ; 00970 /* Frame buffer frame offset address */ 00971 if (param->flm_num != 0u) { 00972 *(scaler->scl1_wr10) = param->flm_off ; 00973 } 00974 } else { 00975 /* SC_RES_TB_ADD_MOD */ 00976 *(scaler->scl1_wr1) &= (uint32_t)~VDC5_REG_MASK_0X00000080; 00977 } 00978 } 00979 00980 /* Register update control register (SC0/SC1/OIR_SCL0_UPDATE) 00981 b12 SCL0_VEN_C 00982 b4 SCL0_VEN_B 00983 b0 SCL0_VEN_A */ 00984 *(scaler->scl0_update) |= (uint32_t)(VDC5_REG_BIT12 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 00985 /* Register update control register (SC0/SC1/OIR_SCL1_UPDATE) 00986 b16 SCL1_UPDATE_A 00987 b4 SCL1_VEN_B 00988 b0 SCL1_VEN_A */ 00989 *(scaler->scl1_update) |= (uint32_t)(VDC5_REG_BIT16 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 00990 00991 if (scaling_id != VDC5_SC_TYPE_OIR ) { 00992 SetInputCntrlColorMtx(ch, scaling_id); 00993 } 00994 } /* End of function VDC5_WriteDataControl() */ 00995 00996 /**************************************************************************//** 00997 * @brief Sets registers for data write change processing 00998 * @param[in] ch : Channel 00999 * @param[in] scaling_id : Scaling type ID 01000 * @param[in] param : Data write change parameter 01001 * @retval None 01002 ******************************************************************************/ 01003 void VDC5_ChangeWriteProcess ( 01004 const vdc5_channel_t ch, 01005 const vdc5_scaling_type_t scaling_id, 01006 const vdc5_write_chg_t * const param) 01007 { 01008 const vdc5_regaddr_scaler_t * scaler; 01009 const vdc5_scalingdown_rot_t * scldw_rot; 01010 vdc5_res_inter_t res_inter_tmp; 01011 01012 scaler = &vdc5_regaddr_scaler[ch][scaling_id]; 01013 scldw_rot = ¶m->scalingdown_rot ; 01014 01015 /* Image area to be captured */ 01016 *(scaler->scl0_ds2) = (uint32_t)scldw_rot->res .vs << VDC5_REG_SHIFT_16; 01017 *(scaler->scl0_ds2) |= (uint32_t)scldw_rot->res .vw ; 01018 *(scaler->scl0_ds3) = (uint32_t)scldw_rot->res .hs << VDC5_REG_SHIFT_16; 01019 *(scaler->scl0_ds3) |= (uint32_t)scldw_rot->res .hw ; 01020 01021 if (scaling_id == VDC5_SC_TYPE_OIR ) { 01022 /* OIR */ 01023 /* Vertical and horizontal width after scaling-down control block */ 01024 *(scaler->scl0_ds7) = (uint32_t)scldw_rot->res .vw << VDC5_REG_SHIFT_16; 01025 *(scaler->scl0_ds7) |= (uint32_t)scldw_rot->res .hw ; 01026 } else { 01027 /* Scaler 0 or 1 */ 01028 /* Horizontal prefilter */ 01029 if (scldw_rot->res_pfil_sel == VDC5_OFF ) { 01030 *(scaler->scl0_ds4) &= (uint32_t)~VDC5_REG_BIT29; 01031 } else { 01032 *(scaler->scl0_ds4) |= (uint32_t)VDC5_REG_BIT29; 01033 } 01034 /* Number of valid lines in vertical direction output by scaling-down control block */ 01035 *(scaler->scl0_ds7) = (uint32_t)scldw_rot->res_out_vw << VDC5_REG_SHIFT_16; 01036 /* Number of valid horizontal pixels output by scaling-down control block */ 01037 *(scaler->scl0_ds7) |= (uint32_t)scldw_rot->res_out_hw ; 01038 01039 /* Progressive or interlace */ 01040 res_inter_tmp = VDC5_ShrdPrmGetInterlace(ch, scaling_id); 01041 /* Scaling-down */ 01042 SetScalingDown(scldw_rot, res_inter_tmp, scaler); 01043 01044 /* Frame buffer writing mode for image processing */ 01045 *(scaler->scl1_wr1) &= (uint32_t)~VDC5_REG_MASK_0X00000070; 01046 *(scaler->scl1_wr1) |= (uint32_t)scldw_rot->res_ds_wr_md << VDC5_REG_SHIFT_4; 01047 01048 /* Register update control register (SC0/SC1) 01049 b4 SCL1_VEN_B 01050 b0 SCL1_VEN_A */ 01051 *(scaler->scl1_update) |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 01052 } 01053 01054 /* Register update control register (SC0/SC1/OIR_SCL0_UPDATE) 01055 b12 SCL0_VEN_C 01056 b4 SCL0_VEN_B 01057 b0 SCL0_VEN_A */ 01058 *(scaler->scl0_update) |= (uint32_t)(VDC5_REG_BIT12 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 01059 01060 } /* End of function VDC5_ChangeWriteProcess() */ 01061 01062 /**************************************************************************//** 01063 * @brief Sets registers for data read control processing 01064 * @param[in] ch : Channel 01065 * @param[in] graphics_id : Graphics type ID 01066 * @param[in] param : Data read control parameter 01067 * @retval None 01068 ******************************************************************************/ 01069 void VDC5_ReadDataControl ( 01070 const vdc5_channel_t ch, 01071 const vdc5_graphics_type_t graphics_id, 01072 const vdc5_read_t * const param) 01073 { 01074 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01075 const vdc5_regaddr_img_qlty_imp_t * img_qlty_imp; 01076 vdc5_width_read_fb_t * w_read_fb; 01077 vdc5_onoff_t graphics_enlargement; 01078 vdc5_color_space_t color_space; 01079 vdc5_resource_state_t rsrc_state; 01080 uint32_t reg_data; 01081 01082 w_read_fb = VDC5_ShrdPrmGetFrBuffWidth_Rd(ch, graphics_id); 01083 01084 graphics_enlargement = ConfirmGraphicsEnlargement(graphics_id, param->gr_flm_sel , w_read_fb, ¶m->gr_grc ); 01085 01086 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || 01087 (graphics_id == VDC5_GR_TYPE_GR1 ) || 01088 (graphics_id == VDC5_GR_TYPE_OIR )) { 01089 SetScalerGraphics(ch, graphics_id, w_read_fb, ¶m->gr_grc , param->gr_flm_sel , graphics_enlargement); 01090 } 01091 01092 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01093 01094 *(img_synthesizer->gr_flm1) &= (uint32_t)~VDC5_REG_MASK_0X00010301; 01095 /* Line offset address direction of the frame buffer */ 01096 if (param->gr_ln_off_dir != VDC5_GR_LN_OFF_DIR_INC ) { 01097 *(img_synthesizer->gr_flm1) |= (uint32_t)VDC5_REG_BIT16; 01098 } 01099 /* Frame buffer address setting signal */ 01100 *(img_synthesizer->gr_flm1) |= (uint32_t)param->gr_flm_sel << VDC5_REG_SHIFT_8; 01101 /* Frame buffer burst transfer mode */ 01102 if (param->gr_bst_md != VDC5_BST_MD_32BYTE ) { 01103 *(img_synthesizer->gr_flm1) |= (uint32_t)VDC5_REG_BIT0; 01104 } 01105 /* Sets the frame buffer number for distortion correction */ 01106 if (param->gr_flm_sel == VDC5_GR_FLM_SEL_DISTORTION ) { 01107 if (param->gr_imr_flm_inv == VDC5_OFF ) { 01108 *(img_synthesizer->gr_flm1) &= (uint32_t)~VDC5_REG_BIT4; 01109 } else { 01110 *(img_synthesizer->gr_flm1) |= (uint32_t)VDC5_REG_BIT4; 01111 } 01112 } 01113 01114 /* Frame buffer base address */ 01115 *(img_synthesizer->gr_flm2) = (uint32_t)param->gr_base & (uint32_t)~VDC5_REG_MASK_0X00000007; 01116 01117 /* Frame buffer line offset address[byte] */ 01118 *(img_synthesizer->gr_flm3) &= (uint32_t)~VDC5_REG_MASK_0X7FFF03FF; 01119 *(img_synthesizer->gr_flm3) |= param->gr_ln_off << VDC5_REG_SHIFT_16; 01120 01121 /* The number of lines when reading the addresses repeatedly */ 01122 *(img_synthesizer->gr_flm5) = (uint32_t)VDC5_REG_MASK_0X000007FF; 01123 /* Number of lines in a frame */ 01124 *(img_synthesizer->gr_flm5) |= ((uint32_t)w_read_fb->in_vw - 1u) << VDC5_REG_SHIFT_16; 01125 01126 *(img_synthesizer->gr_flm6) &= (uint32_t)~VDC5_REG_MASK_0XF7FF1C3F; 01127 /* Format of the frame buffer read signal */ 01128 *(img_synthesizer->gr_flm6) |= (uint32_t)param->gr_format << VDC5_REG_SHIFT_28; 01129 /* Width of the horizontal valid period */ 01130 *(img_synthesizer->gr_flm6) |= ((uint32_t)w_read_fb->in_hw - 1u) << VDC5_REG_SHIFT_16; 01131 /* Swap setting in frame buffer reading */ 01132 *(img_synthesizer->gr_flm6) |= (uint32_t)param->gr_rdswa << VDC5_REG_SHIFT_10; 01133 01134 if ((param->gr_flm_sel == VDC5_GR_FLM_SEL_SCALE_DOWN ) || (param->gr_flm_sel == VDC5_GR_FLM_SEL_FLM_NUM )) { 01135 /* Sets the amount of data to be skipped through */ 01136 *(img_synthesizer->gr_flm6) |= DisplayStartPixelSetting((uint32_t)param->gr_base , param->gr_format ); 01137 } 01138 01139 /* Graphics display mode */ 01140 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 01141 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || 01142 (graphics_id == VDC5_GR_TYPE_OIR )) { 01143 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_BACK ; 01144 } else { 01145 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 01146 } 01147 01148 /* Graphics display area */ 01149 *(img_synthesizer->gr_ab2) = (uint32_t)param->gr_grc .vs << VDC5_REG_SHIFT_16; 01150 *(img_synthesizer->gr_ab2) |= (uint32_t)param->gr_grc .vw ; 01151 *(img_synthesizer->gr_ab3) = (uint32_t)param->gr_grc .hs << VDC5_REG_SHIFT_16; 01152 *(img_synthesizer->gr_ab3) |= (uint32_t)param->gr_grc .hw ; 01153 01154 /* Initialize alpha blending in a rectangular area */ 01155 if ((graphics_id == VDC5_GR_TYPE_GR1 ) || 01156 (graphics_id == VDC5_GR_TYPE_GR2 ) || 01157 (graphics_id == VDC5_GR_TYPE_GR3 )) { 01158 /* Turns off alpha blending in a rectangular area */ 01159 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X0000D000; 01160 /* The valid image area for alpha blending in a rectangular area */ 01161 reg_data = *(img_synthesizer->gr_ab2); 01162 *(img_synthesizer->gr_ab4) = reg_data; 01163 reg_data = *(img_synthesizer->gr_ab3); 01164 *(img_synthesizer->gr_ab5) = reg_data; 01165 /* Disable fade-in and fade-out */ 01166 *(img_synthesizer->gr_ab6) &= (uint32_t)~VDC5_REG_MASK_0X01FF00FF; 01167 /* Set the initial alpha value to '255' */ 01168 *(img_synthesizer->gr_ab7) |= (uint32_t)VDC5_REG_MASK_0X00FF0000; 01169 } 01170 /* Turns off chroma-key processing */ 01171 *(img_synthesizer->gr_ab7) &= (uint32_t)~VDC5_REG_BIT0; 01172 01173 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_OIR )) { 01174 /* Alpha value is set to 255. */ 01175 *(img_synthesizer->gr_ab10) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01176 *(img_synthesizer->gr_ab11) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01177 } 01178 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_GR1 )) { 01179 /* Swapping of data read from buffer in the YCbCr422 format */ 01180 *(img_synthesizer->gr_flm6) &= (uint32_t)~VDC5_REG_MASK_0X0000E000; 01181 if (param->gr_format == VDC5_GR_FORMAT_YCBCR422 ) { 01182 *(img_synthesizer->gr_flm6) |= (uint32_t)param->gr_ycc_swap << VDC5_REG_SHIFT_13; 01183 /* The interpolation mode for YCC422 to YCbCr444 conversion is fixed to average interpolation */ 01184 *(img_synthesizer->gr_flm6) |= (uint32_t)VDC5_REG_BIT8; 01185 } else { 01186 /* If the format of the frame buffer read signal is not YCbCr422, 01187 initialize the swapping of data read from buffer in the YCbCr422 format. */ 01188 *(img_synthesizer->gr_flm6) |= (uint32_t)VDC5_GR_YCCSWAP_CBY0CRY1 << VDC5_REG_SHIFT_13; 01189 } 01190 01191 SetupGraphicsVIN(ch, graphics_id, param->gr_flm_sel , graphics_enlargement); 01192 01193 color_space = VDC5_ShrdPrmGetColorSpaceFbRd(ch, graphics_id); 01194 01195 SetScalerBackgroundColor(ch, graphics_id, color_space); 01196 SetImgQaImproverColorMtx(ch, graphics_id, color_space); 01197 01198 if (graphics_id == VDC5_GR_TYPE_GR0 ) { 01199 img_qlty_imp = &vdc5_regaddr_img_qlty_imp[ch][VDC5_IMG_IMPRV_0 ]; 01200 } else { 01201 img_qlty_imp = &vdc5_regaddr_img_qlty_imp[ch][VDC5_IMG_IMPRV_1 ]; 01202 } 01203 /* Operating mode */ 01204 if (color_space == VDC5_COLOR_SPACE_GBR ) { 01205 *(img_qlty_imp->adj_enh_tim1) &= (uint32_t)~VDC5_REG_BIT4; 01206 } else { 01207 *(img_qlty_imp->adj_enh_tim1) |= (uint32_t)VDC5_REG_BIT4; 01208 } 01209 /* Register update control register (ADJx_UPDATE) 01210 b0 ADJx_VEN */ 01211 *(img_qlty_imp->adj_update) |= (uint32_t)VDC5_REG_BIT0; 01212 } 01213 01214 rsrc_state = VDC5_ShrdPrmGetLayerResource(ch, VDC5_LAYER_ID_1_RD ); 01215 if ((graphics_id == VDC5_GR_TYPE_GR0 ) && (rsrc_state == VDC5_RESOURCE_ST_INVALID )) { 01216 /* Graphics 0 is specified when graphics 1 is not used. */ 01217 SetImgQaImproverColorMtx(ch, VDC5_GR_TYPE_GR1 , VDC5_COLOR_SPACE_GBR ); 01218 } 01219 /* Graphics register update control register (GRx_UPDATE) 01220 b8 GRx_UPDATE 01221 b4 GRx_P_VEN 01222 b0 GRx_IBUS_VEN */ 01223 *(img_synthesizer->gr_update) |= (uint32_t)(VDC5_REG_BIT8 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 01224 01225 } /* End of function VDC5_ReadDataControl() */ 01226 01227 /**************************************************************************//** 01228 * @brief Sets registers for data read change processing 01229 * @param[in] ch : Channel 01230 * @param[in] graphics_id : Graphics type ID 01231 * @param[in] param : Data read change parameter 01232 * @retval None 01233 ******************************************************************************/ 01234 void VDC5_ChangeReadProcess ( 01235 const vdc5_channel_t ch, 01236 const vdc5_graphics_type_t graphics_id, 01237 const vdc5_read_chg_t * const param) 01238 { 01239 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01240 vdc5_gr_flm_sel_t gr_flm_sel_tmp; 01241 vdc5_period_rect_t * gr_grc_tmp; 01242 vdc5_width_read_fb_t * w_read_fb; 01243 vdc5_onoff_t graphics_enlargement; 01244 vdc5_gr_format_t gr_format_tmp; 01245 01246 gr_flm_sel_tmp = VDC5_ShrdPrmGetSelFbAddrSig(ch, graphics_id); 01247 gr_grc_tmp = VDC5_ShrdPrmGetDisplayArea(ch, graphics_id); 01248 w_read_fb = VDC5_ShrdPrmGetFrBuffWidth_Rd(ch, graphics_id); 01249 01250 graphics_enlargement = ConfirmGraphicsEnlargement(graphics_id, gr_flm_sel_tmp, w_read_fb, gr_grc_tmp); 01251 01252 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || 01253 (graphics_id == VDC5_GR_TYPE_GR1 ) || 01254 (graphics_id == VDC5_GR_TYPE_OIR )) { 01255 SetScalerGraphics(ch, graphics_id, w_read_fb, gr_grc_tmp, gr_flm_sel_tmp, graphics_enlargement); 01256 } 01257 01258 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01259 01260 /* Frame buffer base address */ 01261 if (param->gr_base != NULL) { 01262 *(img_synthesizer->gr_flm2) = (uint32_t)param->gr_base & (uint32_t)~VDC5_REG_MASK_0X00000007; 01263 01264 if ((gr_flm_sel_tmp == VDC5_GR_FLM_SEL_SCALE_DOWN ) || (gr_flm_sel_tmp == VDC5_GR_FLM_SEL_FLM_NUM )) { 01265 gr_format_tmp = VDC5_ShrdPrmGetGraphicsFormat(ch, graphics_id); 01266 /* Sets the amount of data to be skipped through */ 01267 *(img_synthesizer->gr_flm6) &= (uint32_t)~VDC5_REG_MASK_0X0000003F; 01268 *(img_synthesizer->gr_flm6) |= DisplayStartPixelSetting((uint32_t)param->gr_base , gr_format_tmp); 01269 } 01270 } 01271 01272 /* Size of the frame buffer to be read */ 01273 if (param->width_read_fb != NULL) { 01274 /* Number of lines in a frame */ 01275 *(img_synthesizer->gr_flm5) &= (uint32_t)~VDC5_REG_MASK_0X07FF0000; 01276 *(img_synthesizer->gr_flm5) |= ((uint32_t)param->width_read_fb ->in_vw - 1u) << VDC5_REG_SHIFT_16; 01277 /* Width of the horizontal valid period */ 01278 *(img_synthesizer->gr_flm6) &= (uint32_t)~VDC5_REG_MASK_0X07FF0000; 01279 *(img_synthesizer->gr_flm6) |= ((uint32_t)param->width_read_fb ->in_hw - 1u) << VDC5_REG_SHIFT_16; 01280 } 01281 01282 /* Graphics display mode */ 01283 if (param->gr_disp_sel != NULL) { 01284 if (*(param->gr_disp_sel ) != VDC5_DISPSEL_IGNORED ) { 01285 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 01286 *(img_synthesizer->gr_ab1) |= (uint32_t)*(param->gr_disp_sel ); 01287 } 01288 } 01289 01290 /* Graphics display area */ 01291 if (param->gr_grc != NULL) { 01292 *(img_synthesizer->gr_ab2) = (uint32_t)param->gr_grc ->vs << VDC5_REG_SHIFT_16; 01293 *(img_synthesizer->gr_ab2) |= (uint32_t)param->gr_grc ->vw ; 01294 *(img_synthesizer->gr_ab3) = (uint32_t)param->gr_grc ->hs << VDC5_REG_SHIFT_16; 01295 *(img_synthesizer->gr_ab3) |= (uint32_t)param->gr_grc ->hw ; 01296 } 01297 01298 /* Graphics register update control register (GRx_UPDATE) 01299 b4 GRx_P_VEN 01300 b0 GRx_IBUS_VEN */ 01301 *(img_synthesizer->gr_update) |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 01302 01303 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_GR1 )) { 01304 ChangeGraphicsVIN(ch, graphics_id, gr_flm_sel_tmp, graphics_enlargement); 01305 } 01306 } /* End of function VDC5_ChangeReadProcess() */ 01307 01308 /**************************************************************************//** 01309 * @brief Sets registers for data write/read start processing 01310 * @param[in] ch : Channel 01311 * @param[in] layer_id : Layer ID 01312 * @param[in] param : Data write/read start parameter 01313 * @retval None 01314 ******************************************************************************/ 01315 void VDC5_StartProcess (const vdc5_channel_t ch, const vdc5_layer_id_t layer_id, const vdc5_start_t * const param) 01316 { 01317 uint32_t layer_id_num; 01318 vdc5_resource_state_t resource_state; 01319 vdc5_resource_state_t oir_resrc_st_before; 01320 vdc5_resource_state_t oir_resrc_st_after; 01321 const vdc5_regaddr_scaler_t * scaler; 01322 01323 if (param->gr_disp_sel != NULL) { 01324 /* Set graphics display mode */ 01325 SetGraphicsDisplayMode(ch, layer_id, param->gr_disp_sel ); 01326 } 01327 01328 oir_resrc_st_before = VDC5_ShrdPrmGetOirRwProcEnabled(ch); 01329 01330 if (layer_id != VDC5_LAYER_ID_ALL ) { 01331 rw_proc_function_tbl[layer_id](ch, VDC5_ON ); 01332 } else { 01333 for (layer_id_num = 0; layer_id_num < (uint32_t)VDC5_LAYER_ID_NUM; layer_id_num++) { 01334 resource_state = VDC5_ShrdPrmGetRwProcReady(ch, (vdc5_layer_id_t )layer_id_num); 01335 if (resource_state != VDC5_RESOURCE_ST_INVALID ) { 01336 rw_proc_function_tbl[layer_id_num](ch, VDC5_ON ); 01337 } 01338 } 01339 } 01340 SetRegUpdateRwEnable(ch, layer_id); 01341 01342 oir_resrc_st_after = VDC5_ShrdPrmGetOirRwProcEnabled(ch); 01343 01344 /* OIR */ 01345 if ((oir_resrc_st_before == VDC5_RESOURCE_ST_INVALID ) && 01346 (oir_resrc_st_after != VDC5_RESOURCE_ST_INVALID )) { 01347 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ]; 01348 *(scaler->scl0_frc3) |= (uint32_t)VDC5_REG_BIT16; 01349 /* Register update control register (OIR_SCL0_UPDATE) 01350 b8 SCL0_UPDATE */ 01351 *(scaler->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 01352 } 01353 } /* End of function VDC5_StartProcess() */ 01354 01355 /**************************************************************************//** 01356 * @brief Sets registers for data write/read stop processing 01357 * @param[in] ch : Channel 01358 * @param[in] layer_id : Layer ID 01359 * @retval None 01360 ******************************************************************************/ 01361 void VDC5_StopProcess (const vdc5_channel_t ch, const vdc5_layer_id_t layer_id) 01362 { 01363 uint32_t layer_id_num; 01364 vdc5_resource_state_t resource_state; 01365 vdc5_resource_state_t oir_resrc_st_before; 01366 vdc5_resource_state_t oir_resrc_st_after; 01367 const vdc5_regaddr_scaler_t * scaler; 01368 01369 oir_resrc_st_before = VDC5_ShrdPrmGetOirRwProcEnabled(ch); 01370 01371 if (layer_id != VDC5_LAYER_ID_ALL ) { 01372 rw_proc_function_tbl[layer_id](ch, VDC5_OFF ); 01373 } else { 01374 for (layer_id_num = 0; layer_id_num < (uint32_t)VDC5_LAYER_ID_NUM; layer_id_num++) { 01375 resource_state = VDC5_ShrdPrmGetRwProcEnabled(ch, (vdc5_layer_id_t )layer_id_num); 01376 if (resource_state != VDC5_RESOURCE_ST_INVALID ) { 01377 rw_proc_function_tbl[layer_id_num](ch, VDC5_OFF ); 01378 } 01379 } 01380 } 01381 SetRegUpdateRwEnable(ch, layer_id); 01382 01383 oir_resrc_st_after = VDC5_ShrdPrmGetOirRwProcEnabled(ch); 01384 01385 /* OIR */ 01386 if ((oir_resrc_st_before != VDC5_RESOURCE_ST_INVALID ) && 01387 (oir_resrc_st_after == VDC5_RESOURCE_ST_INVALID )) { 01388 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ]; 01389 *(scaler->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT16; 01390 /* Register update control register (OIR_SCL0_UPDATE) 01391 b8 SCL0_UPDATE */ 01392 *(scaler->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 01393 } 01394 } /* End of function VDC5_StopProcess() */ 01395 01396 /**************************************************************************//** 01397 * @brief Sets registers for data write/read control release processing 01398 * @param[in] ch : Channel 01399 * @param[in] layer_id : Layer ID 01400 * @retval None 01401 ******************************************************************************/ 01402 void VDC5_ReleaseDataControl (const vdc5_channel_t ch, const vdc5_layer_id_t layer_id) 01403 { 01404 const vdc5_regaddr_scaler_t * scaler; 01405 vdc5_resource_state_t resource_state; 01406 const vdc5_regaddr_img_synthesizer_t * img_synthesizer_vin; 01407 01408 resource_state = VDC5_ShrdPrmGetRwProcReady(ch, VDC5_LAYER_ID_1_RD ); 01409 if ((layer_id == VDC5_LAYER_ID_1_RD ) || 01410 ((layer_id == VDC5_LAYER_ID_ALL ) && (resource_state!= VDC5_RESOURCE_ST_INVALID ))) { 01411 SetInitialConnection(ch); 01412 01413 /* Use Vsync and enable signal output from scaler 0 */ 01414 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ]; 01415 *(scaler->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT8; 01416 /* Register update control register (SC0_SCL0_UPDATE) 01417 b8 SCL0_UPDATE */ 01418 *(scaler->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 01419 01420 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ]; 01421 *(scaler->scl0_frc3) |= (uint32_t)VDC5_REG_BIT8; 01422 /* Register update control register (SC1_SCL0_UPDATE) 01423 b8 SCL0_UPDATE */ 01424 *(scaler->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 01425 01426 /* Initialize the color matrix in the image quality improver (scaler 1 output) */ 01427 SetImgQaImproverColorMtx(ch, VDC5_GR_TYPE_GR1 , VDC5_COLOR_SPACE_GBR ); 01428 } 01429 01430 resource_state = VDC5_ShrdPrmGetRwProcReady(ch, VDC5_LAYER_ID_0_RD ); 01431 if ((layer_id == VDC5_LAYER_ID_0_RD ) || 01432 ((layer_id == VDC5_LAYER_ID_ALL ) && (resource_state!= VDC5_RESOURCE_ST_INVALID ))) { 01433 img_synthesizer_vin = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_VIN ]; 01434 01435 /* Turns off alpha blending in a rectangular area */ 01436 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_BIT12; 01437 /* Selection of lower-layer plane in scaler, graphics 1 is used as lower-layer graphics. */ 01438 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 01439 /* Graphics display mode in VIN */ 01440 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 01441 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 01442 /* Disable fade-in and fade-out */ 01443 *(img_synthesizer_vin->gr_ab6) &= (uint32_t)~VDC5_REG_MASK_0X01FF00FF; 01444 /* Set the initial alpha value to '255' */ 01445 *(img_synthesizer_vin->gr_ab7) |= (uint32_t)VDC5_REG_MASK_0X00FF0000; 01446 01447 /* Graphics register update control register (GR_VIN_UPDATE) 01448 b8 GR_VIN_UPDATE 01449 b4 GR_VIN_P_VEN */ 01450 *(img_synthesizer_vin->gr_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 01451 } 01452 } /* End of function VDC5_ReleaseDataControl() */ 01453 01454 /**************************************************************************//** 01455 * @brief Sets registers for noise reduction 01456 * @param[in] ch : Channel 01457 * @param[in] nr1d_on : Noise reduction ON/OFF setting 01458 * @param[in] param : Noise reduction setup parameter 01459 * @retval None 01460 ******************************************************************************/ 01461 void VDC5_VideoNoiseReduction ( 01462 const vdc5_channel_t ch, 01463 const vdc5_onoff_t nr1d_on, 01464 const vdc5_noise_reduction_t * const param) 01465 { 01466 const vdc5_regaddr_input_ctrl_t * input_ctrl; 01467 const vdc5_nr_param_t * nr_param; 01468 01469 input_ctrl = &vdc5_regaddr_input_ctrl[ch]; 01470 01471 if (param != NULL) { 01472 /* Y/G signal noise reduction parameter */ 01473 nr_param = ¶m->y ; 01474 *(input_ctrl->imgcnt_nr_cnt0) &= (uint32_t)~VDC5_REG_MASK_0X00007F33; 01475 /* Maximum value of coring (absolute value) */ 01476 *(input_ctrl->imgcnt_nr_cnt0) |= nr_param->nr1d_th << VDC5_REG_SHIFT_8; 01477 /* TAP select */ 01478 *(input_ctrl->imgcnt_nr_cnt0) |= (uint32_t)nr_param->nr1d_tap << VDC5_REG_SHIFT_4; 01479 /* Noise reduction gain adjustment */ 01480 *(input_ctrl->imgcnt_nr_cnt0) |= (uint32_t)nr_param->nr1d_gain ; 01481 01482 /* Cb/B signal noise reduction parameter */ 01483 nr_param = ¶m->cb ; 01484 /* Maximum value of coring (absolute value) */ 01485 *(input_ctrl->imgcnt_nr_cnt1) = nr_param->nr1d_th << VDC5_REG_SHIFT_24; 01486 /* TAP select */ 01487 *(input_ctrl->imgcnt_nr_cnt1) |= (uint32_t)nr_param->nr1d_tap << VDC5_REG_SHIFT_20; 01488 /* Noise reduction gain adjustment */ 01489 *(input_ctrl->imgcnt_nr_cnt1) |= (uint32_t)nr_param->nr1d_gain << VDC5_REG_SHIFT_16; 01490 01491 /* Cr/R signal noise reduction parameter */ 01492 nr_param = ¶m->cr ; 01493 /* Maximum value of coring (absolute value) */ 01494 *(input_ctrl->imgcnt_nr_cnt1) |= nr_param->nr1d_th << VDC5_REG_SHIFT_8; 01495 /* TAP select */ 01496 *(input_ctrl->imgcnt_nr_cnt1) |= (uint32_t)nr_param->nr1d_tap << VDC5_REG_SHIFT_4; 01497 /* Noise reduction gain adjustment */ 01498 *(input_ctrl->imgcnt_nr_cnt1) |= (uint32_t)nr_param->nr1d_gain ; 01499 } 01500 01501 if (nr1d_on == VDC5_OFF ) { 01502 *(input_ctrl->imgcnt_nr_cnt0) &= (uint32_t)~VDC5_REG_BIT16; 01503 } else { 01504 *(input_ctrl->imgcnt_nr_cnt0) |= (uint32_t)VDC5_REG_BIT16; 01505 } 01506 01507 /* Register update control register (IMGCNT_UPDATE) 01508 b0 IMGCNT_VEN */ 01509 *(input_ctrl->imgcnt_update) |= (uint32_t)VDC5_REG_BIT0; 01510 01511 } /* End of function VDC5_VideoNoiseReduction() */ 01512 01513 /**************************************************************************//** 01514 * @brief Sets registers for color matrix 01515 * @param[in] ch : Channel 01516 * @param[in] param : Color matrix setup parameter 01517 * @retval None 01518 ******************************************************************************/ 01519 void VDC5_ImageColorMatrix (const vdc5_channel_t ch, const vdc5_color_matrix_t * const param) 01520 { 01521 SetColorMatrix(param->mtx_mode , param->offset , param->gain , &vdc5_regaddr_color_matrix[ch][param->module ]); 01522 } /* End of function VDC5_ImageColorMatrix() */ 01523 01524 /**************************************************************************//** 01525 * @brief Sets registers for image enhancement processing 01526 * @param[in] ch : Channel 01527 * @param[in] imgimprv_id : Image quality improver ID 01528 * @param[in] shp_h_on : Sharpness ON/OFF setting 01529 * @param[in] sharp_param : Sharpness setup parameter 01530 * @param[in] lti_h_on : LTI ON/OFF setting 01531 * @param[in] lti_param : LTI setup parameter 01532 * @param[in] enh_area : Enhancer-enabled area setup parameter 01533 * @retval None 01534 ******************************************************************************/ 01535 void VDC5_ImageEnhancement ( 01536 const vdc5_channel_t ch, 01537 const vdc5_imgimprv_id_t imgimprv_id, 01538 const vdc5_onoff_t shp_h_on, 01539 const vdc5_enhance_sharp_t * const sharp_param, 01540 const vdc5_onoff_t lti_h_on, 01541 const vdc5_enhance_lti_t * const lti_param, 01542 const vdc5_period_rect_t * const enh_area) 01543 { 01544 const vdc5_regaddr_img_qlty_imp_t * img_qlty_imp; 01545 01546 img_qlty_imp = &vdc5_regaddr_img_qlty_imp[ch][imgimprv_id]; 01547 01548 /* Sharpness */ 01549 SetImageEnhancementSharpness(shp_h_on, sharp_param, img_qlty_imp); 01550 01551 /* LTI */ 01552 SetImageEnhancementLti(lti_h_on, lti_param, img_qlty_imp); 01553 01554 /* Enhancer area */ 01555 if (enh_area != NULL) { 01556 *(img_qlty_imp->adj_enh_tim2) = (uint32_t)enh_area->vs << VDC5_REG_SHIFT_16; 01557 *(img_qlty_imp->adj_enh_tim2) |= (uint32_t)enh_area->vw ; 01558 *(img_qlty_imp->adj_enh_tim3) = (uint32_t)enh_area->hs << VDC5_REG_SHIFT_16; 01559 *(img_qlty_imp->adj_enh_tim3) |= (uint32_t)enh_area->hw ; 01560 } 01561 01562 /* Register update control register (ADJx_UPDATE) 01563 b0 ADJx_VEN */ 01564 *(img_qlty_imp->adj_update) |= (uint32_t)VDC5_REG_BIT0; 01565 01566 } /* End of function VDC5_ImageEnhancement() */ 01567 01568 /**************************************************************************//** 01569 * @brief Sets registers for black stretch 01570 * @param[in] ch : Channel 01571 * @param[in] imgimprv_id : Image quality improver ID 01572 * @param[in] bkstr_on : Black stretch ON/OFF setting 01573 * @param[in] param : Black stretch setup parameter 01574 * @retval None 01575 ******************************************************************************/ 01576 void VDC5_ImageBlackStretch ( 01577 const vdc5_channel_t ch, 01578 const vdc5_imgimprv_id_t imgimprv_id, 01579 const vdc5_onoff_t bkstr_on, 01580 const vdc5_black_t * const param) 01581 { 01582 const vdc5_regaddr_img_qlty_imp_t * img_qlty_imp; 01583 01584 img_qlty_imp = &vdc5_regaddr_img_qlty_imp[ch][imgimprv_id]; 01585 01586 if (param != NULL) { 01587 /* Black stretch start point */ 01588 *(img_qlty_imp->adj_bkstr_set) = (uint32_t)((uint32_t)param->bkstr_st << VDC5_REG_SHIFT_20); 01589 /* Black stretch depth */ 01590 *(img_qlty_imp->adj_bkstr_set) |= (uint32_t)((uint32_t)param->bkstr_d << VDC5_REG_SHIFT_16); 01591 /* Black stretch time constant (T1) */ 01592 *(img_qlty_imp->adj_bkstr_set) |= (uint32_t)((uint32_t)param->bkstr_t1 << VDC5_REG_SHIFT_8); 01593 /* Black stretch time constant (T2) */ 01594 *(img_qlty_imp->adj_bkstr_set) |= (uint32_t)param->bkstr_t2 ; 01595 } 01596 01597 if (bkstr_on == VDC5_OFF ) { 01598 *(img_qlty_imp->adj_bkstr_set) &= (uint32_t)~VDC5_REG_BIT24; 01599 } else { 01600 *(img_qlty_imp->adj_bkstr_set) |= (uint32_t)VDC5_REG_BIT24; 01601 } 01602 01603 /* Register update control register (ADJx_UPDATE) 01604 b0 ADJx_VEN */ 01605 *(img_qlty_imp->adj_update) |= (uint32_t)VDC5_REG_BIT0; 01606 01607 } /* End of function VDC5_ImageBlackStretch() */ 01608 01609 /**************************************************************************//** 01610 * @brief Sets registers for alpha blending 01611 * @param[in] ch : Channel 01612 * @param[in] graphics_id : Graphics type ID 01613 * @param[in] param : Alpha blending setup parameter 01614 * @retval None 01615 ******************************************************************************/ 01616 void VDC5_AlphaBlending ( 01617 const vdc5_channel_t ch, 01618 const vdc5_graphics_type_t graphics_id, 01619 const vdc5_alpha_blending_t * const param) 01620 { 01621 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01622 01623 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01624 01625 /* Alpha signal of the ARGB1555/ARGB5551 format */ 01626 if (param->alpha_1bit != NULL) { 01627 *(img_synthesizer->gr_ab10) &= (uint32_t)~VDC5_REG_MASK_0XFF000000; 01628 *(img_synthesizer->gr_ab10) |= (uint32_t)param->alpha_1bit ->gr_a0 << VDC5_REG_SHIFT_24; 01629 *(img_synthesizer->gr_ab11) &= (uint32_t)~VDC5_REG_MASK_0XFF000000; 01630 *(img_synthesizer->gr_ab11) |= (uint32_t)param->alpha_1bit ->gr_a1 << VDC5_REG_SHIFT_24; 01631 } 01632 01633 /* Premultiplication processing at alpha blending in one-pixel */ 01634 if (param->alpha_pixel != NULL) { 01635 if (param->alpha_pixel ->gr_acalc_md == VDC5_OFF ) { 01636 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_BIT14; 01637 } else { 01638 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT14; 01639 } 01640 } 01641 01642 /* Graphics register update control register (GRx_UPDATE) 01643 b4 GRx_P_VEN */ 01644 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 01645 01646 } /* End of function VDC5_AlphaBlending() */ 01647 01648 /**************************************************************************//** 01649 * @brief Sets registers for rectangle alpha blending 01650 * @param[in] ch : Channel 01651 * @param[in] graphics_id : Graphics type ID 01652 * @param[in] gr_arc_on : ON/OFF setting for alpha blending in a rectangular area 01653 * @param[in] param : Setup parameter for alpha blending in a rectangular area 01654 * @retval None 01655 ******************************************************************************/ 01656 void VDC5_AlphaBlendingRect ( 01657 const vdc5_channel_t ch, 01658 const vdc5_graphics_type_t graphics_id, 01659 const vdc5_onoff_t gr_arc_on, 01660 const vdc5_alpha_blending_rect_t * const param) 01661 { 01662 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01663 const vdc5_alpha_rect_t * alpha_rect_tmp; 01664 uint32_t arc_coef; 01665 01666 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01667 01668 /* Alpha blending on/off control in a rectangular area */ 01669 if (gr_arc_on == VDC5_OFF ) { 01670 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_BIT12; 01671 } else { 01672 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT12; 01673 } 01674 01675 if (param != NULL) { 01676 /* Selection of lower-layer plane in scaler */ 01677 if (param->scl_und_sel != NULL) { 01678 SetUndSel(ch, param->scl_und_sel ->gr_vin_scl_und_sel ); 01679 } 01680 /* Rectangular area subjected to alpha blending */ 01681 SetAlphaRectArea(param->gr_arc , img_synthesizer); 01682 01683 /* Alpha blending in a rectangular area */ 01684 alpha_rect_tmp = param->alpha_rect ; 01685 if (alpha_rect_tmp != NULL) { 01686 if (graphics_id != VDC5_GR_TYPE_VIN ) { 01687 /* Multiplication processing with current alpha at alpha blending in a rectangular area (on/off) */ 01688 if (alpha_rect_tmp->gr_arc_mul == VDC5_OFF ) { 01689 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_BIT15; 01690 } else { 01691 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT15; 01692 } 01693 } 01694 /* Alpha coefficient for alpha blending in a rectangular area (-255 to 255) */ 01695 if (alpha_rect_tmp->gr_arc_coef < 0) { 01696 arc_coef = (uint32_t)(alpha_rect_tmp->gr_arc_coef * (-1)); /* Conversion into absolute value */ 01697 /* Subtraction of the alpha coefficient */ 01698 *(img_synthesizer->gr_ab6) = (uint32_t)VDC5_REG_BIT24; 01699 } else { 01700 arc_coef = (uint32_t)alpha_rect_tmp->gr_arc_coef ; 01701 /* Addition of the alpha coefficient */ 01702 *(img_synthesizer->gr_ab6) = (uint32_t)0x00000000u; 01703 } 01704 *(img_synthesizer->gr_ab6) |= arc_coef << VDC5_REG_SHIFT_16; 01705 /* Frame rate for alpha blending in a rectangular area (gr_arc_rate + 1) */ 01706 *(img_synthesizer->gr_ab6) |= (uint32_t)alpha_rect_tmp->gr_arc_rate ; 01707 /* Initial alpha value for alpha blending in a rectangular area */ 01708 *(img_synthesizer->gr_ab7) &= (uint32_t)~VDC5_REG_MASK_0X00FF0000; 01709 *(img_synthesizer->gr_ab7) |= (uint32_t)alpha_rect_tmp->gr_arc_def << VDC5_REG_SHIFT_16; 01710 } 01711 } 01712 /* Graphics register update control register (GRx_UPDATE) 01713 b4 GRx_P_VEN */ 01714 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 01715 01716 } /* End of function VDC5_AlphaBlending() */ 01717 01718 /**************************************************************************//** 01719 * @brief Sets registers for chroma-key 01720 * @param[in] ch : Channel 01721 * @param[in] graphics_id : Graphics type ID 01722 * @param[in] gr_ck_on : Chroma-key ON/OFF setting 01723 * @param[in] param : Chroma-key setup parameter 01724 * @retval None 01725 ******************************************************************************/ 01726 void VDC5_Chromakey ( 01727 const vdc5_channel_t ch, 01728 const vdc5_graphics_type_t graphics_id, 01729 const vdc5_onoff_t gr_ck_on, 01730 const vdc5_chromakey_t * const param) 01731 { 01732 vdc5_gr_format_t gr_format_tmp; 01733 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01734 01735 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01736 01737 if (param != NULL) { 01738 gr_format_tmp = VDC5_ShrdPrmGetGraphicsFormat(ch, graphics_id); 01739 if ((gr_format_tmp == VDC5_GR_FORMAT_CLUT1 ) || 01740 (gr_format_tmp == VDC5_GR_FORMAT_CLUT4 ) || 01741 (gr_format_tmp == VDC5_GR_FORMAT_CLUT8 )) { 01742 *(img_synthesizer->gr_ab8) = (uint32_t)(param->ck_color << VDC5_REG_SHIFT_24); 01743 *(img_synthesizer->gr_ab9) = ColorConversionIntoAgbr(param->rep_color & VDC5_REG_RGB_24BIT_MASK); 01744 } else { 01745 /* Color conversion from gr_format_tmp into RGB888 format */ 01746 *(img_synthesizer->gr_ab8) = ColorConversion(gr_format_tmp, param->ck_color ); 01747 *(img_synthesizer->gr_ab9) = ColorConversion(gr_format_tmp, param->rep_color ); 01748 } 01749 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_OIR )) { 01750 /* Alpha value is set to 255. */ 01751 *(img_synthesizer->gr_ab9) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01752 } else { 01753 *(img_synthesizer->gr_ab9) |= (uint32_t)param->rep_alpha << VDC5_REG_SHIFT_24; 01754 } 01755 } 01756 01757 if (gr_ck_on == VDC5_OFF ) { 01758 *(img_synthesizer->gr_ab7) &= (uint32_t)~VDC5_REG_BIT0; 01759 } else { 01760 *(img_synthesizer->gr_ab7) |= (uint32_t)VDC5_REG_BIT0; 01761 } 01762 01763 /* Graphics register update control register (GRx_UPDATE) 01764 b4 GRx_P_VEN */ 01765 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 01766 01767 } /* End of function VDC5_Chromakey() */ 01768 01769 /**************************************************************************//** 01770 * @brief Sets registers for CLUT 01771 * @param[in] ch : Channel 01772 * @param[in] graphics_id : Graphics type ID 01773 * @param[in] param : CLUT setup parameter 01774 * @retval None 01775 ******************************************************************************/ 01776 void VDC5_CLUT (const vdc5_channel_t ch, const vdc5_graphics_type_t graphics_id, const vdc5_clut_t * const param) 01777 { 01778 vdc5_gr_format_t gr_format_tmp; 01779 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01780 01781 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 01782 01783 gr_format_tmp = VDC5_ShrdPrmGetGraphicsFormat(ch, graphics_id); 01784 if (gr_format_tmp == VDC5_GR_FORMAT_CLUT1 ) { 01785 if (param->color_num == ((uint32_t)1u)) { 01786 *(img_synthesizer->gr_ab10) = ColorConversionIntoAgbr(param->clut [0]); 01787 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_OIR )) { 01788 /* Alpha value is set to 255. */ 01789 *(img_synthesizer->gr_ab10) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01790 } 01791 } else { 01792 *(img_synthesizer->gr_ab10) = ColorConversionIntoAgbr(param->clut [0]); 01793 *(img_synthesizer->gr_ab11) = ColorConversionIntoAgbr(param->clut [1]); 01794 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_OIR )) { 01795 /* Alpha value is set to 255. */ 01796 *(img_synthesizer->gr_ab10) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01797 *(img_synthesizer->gr_ab11) |= (uint32_t)VDC5_REG_ALPHA_8BIT; 01798 } 01799 } 01800 } else { /* CLUT4 or CLUT8 */ 01801 Set_Clut(param, vdc5_regaddr_clut[ch][graphics_id]); 01802 01803 /* CLUT table control register (GRx_CLUT) 01804 b16 GRx_CLT_SEL - CLUT table select signal - XOR, flip signal */ 01805 *(img_synthesizer->gr_clut) ^= (uint32_t)VDC5_REG_BIT16; 01806 } 01807 /* Graphics register update control register (GRx_UPDATE) 01808 b4 GRx_P_VEN */ 01809 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 01810 01811 } /* End of function VDC5_CLUT() */ 01812 01813 /**************************************************************************//** 01814 * @brief Sets registers for display calibration 01815 * @param[in] ch : Channel 01816 * @param[in] param : Display calibration parameter 01817 * @retval None 01818 ******************************************************************************/ 01819 void VDC5_DisplayCalibration (const vdc5_channel_t ch, const vdc5_disp_calibration_t * const param) 01820 { 01821 const vdc5_calibr_bright_t * bright_tmp; 01822 const vdc5_calibr_contrast_t * contrast_tmp; 01823 const vdc5_calibr_dither_t * panel_dither_tmp; 01824 const vdc5_regaddr_output_ctrl_t * output_ctrl; 01825 01826 output_ctrl = &vdc5_regaddr_output_ctrl[ch]; 01827 01828 /* Correction circuit sequence control */ 01829 if (param->route == VDC5_CALIBR_ROUTE_BCG ) { 01830 *(output_ctrl->out_clk_phase) &= (uint32_t)~VDC5_REG_BIT12; 01831 } else { 01832 *(output_ctrl->out_clk_phase) |= (uint32_t)VDC5_REG_BIT12; 01833 } 01834 01835 /* Brightness (DC) adjustment */ 01836 bright_tmp = param->bright ; 01837 if (bright_tmp != NULL) { 01838 *(output_ctrl->out_bright1) = (uint32_t)bright_tmp->pbrt_g ; 01839 *(output_ctrl->out_bright2) = (uint32_t)bright_tmp->pbrt_b << VDC5_REG_SHIFT_16; 01840 *(output_ctrl->out_bright2) |= (uint32_t)bright_tmp->pbrt_r ; 01841 } 01842 01843 /* Contrast (gain) adjustment */ 01844 contrast_tmp = param->contrast ; 01845 if (contrast_tmp != NULL) { 01846 *(output_ctrl->out_contrast) = (uint32_t)contrast_tmp->cont_g << VDC5_REG_SHIFT_16; 01847 *(output_ctrl->out_contrast) |= (uint32_t)contrast_tmp->cont_b << VDC5_REG_SHIFT_8; 01848 *(output_ctrl->out_contrast) |= (uint32_t)contrast_tmp->cont_r ; 01849 } 01850 01851 /* Panel dithering */ 01852 panel_dither_tmp = param->panel_dither ; 01853 if (panel_dither_tmp != NULL) { 01854 *(output_ctrl->out_pdtha) &= (uint32_t)~VDC5_REG_MASK_0X00300000; 01855 *(output_ctrl->out_pdtha) |= (uint32_t)panel_dither_tmp->pdth_sel << VDC5_REG_SHIFT_20; 01856 if (panel_dither_tmp->pdth_sel == VDC5_PDTH_MD_2X2 ) { 01857 *(output_ctrl->out_pdtha) &= (uint32_t)~VDC5_REG_MASK_0X00003333; 01858 *(output_ctrl->out_pdtha) |= (uint32_t)panel_dither_tmp->pdth_pa << VDC5_REG_SHIFT_12; 01859 *(output_ctrl->out_pdtha) |= (uint32_t)panel_dither_tmp->pdth_pb << VDC5_REG_SHIFT_8; 01860 *(output_ctrl->out_pdtha) |= (uint32_t)panel_dither_tmp->pdth_pc << VDC5_REG_SHIFT_4; 01861 *(output_ctrl->out_pdtha) |= (uint32_t)panel_dither_tmp->pdth_pd ; 01862 } 01863 } 01864 01865 /* Register update control register (OUT_UPDATE) 01866 b0 OUTCNT_VEN */ 01867 *(output_ctrl->out_update) |= (uint32_t)VDC5_REG_BIT0; 01868 01869 } /* End of function VDC5_DisplayCalibration() */ 01870 01871 /**************************************************************************//** 01872 * @brief Sets registers for gamma correction 01873 * @param[in] ch : Channel 01874 * @param[in] gam_on : Gamma correction ON/OFF setting 01875 * @param[in] param : Gamma correction setup parameter 01876 * @retval None 01877 ******************************************************************************/ 01878 void VDC5_GammaCorrection ( 01879 const vdc5_channel_t ch, 01880 const vdc5_onoff_t gam_on, 01881 const vdc5_gamma_correction_t * const param) 01882 { 01883 const vdc5_regaddr_gamma_t * gamma; 01884 01885 gamma = &vdc5_regaddr_gamma[ch]; 01886 01887 /* Gamma correction on/off control */ 01888 *(gamma->gam_sw) = (gam_on == VDC5_OFF ) ? 0x0000u : 0x0001u; 01889 01890 if (param != NULL) { 01891 /* Start threshold of area 1 to 31 of G signal */ 01892 Set_StartThreshold_Gamma(param->gam_g_th , gamma->gam_g_area); 01893 /* Gain adjustment of area 0 to 31 of G signal */ 01894 Set_GainAdjustment_Gamma(param->gam_g_gain , gamma->gam_g_lut); 01895 /* Register update control register G (GAM_G_UPDATE) 01896 b0 GAM_G_VEN */ 01897 *(gamma->gam_g_update) |= (uint32_t)VDC5_REG_BIT0; 01898 01899 /* Start threshold of area 1 to 31 of B signal */ 01900 Set_StartThreshold_Gamma(param->gam_b_th , gamma->gam_b_area); 01901 /* Gain adjustment of area 0 to 31 of B signal */ 01902 Set_GainAdjustment_Gamma(param->gam_b_gain , gamma->gam_b_lut); 01903 /* Register update control register B (GAM_B_UPDATE) 01904 b0 GAM_B_VEN */ 01905 *(gamma->gam_b_update) |= (uint32_t)VDC5_REG_BIT0; 01906 01907 /* Start threshold of area 1 to 31 of R signal */ 01908 Set_StartThreshold_Gamma(param->gam_r_th , gamma->gam_r_area); 01909 /* Gain adjustment of area 0 to 31 of R signal */ 01910 Set_GainAdjustment_Gamma(param->gam_r_gain , gamma->gam_r_lut); 01911 /* Register update control register R (GAM_R_UPDATE) 01912 b0 GAM_R_VEN */ 01913 *(gamma->gam_r_update) |= (uint32_t)VDC5_REG_BIT0; 01914 } 01915 } /* End of function VDC5_GammaCorrection() */ 01916 01917 /****************************************************************************** 01918 Local Functions 01919 ******************************************************************************/ 01920 /**************************************************************************//** 01921 * @brief Sets external input video signal 01922 * @param[in] ext_sig_tmp : External input signal parameter 01923 * @param[in] input_ctrl : Input controller registers 01924 * @retval None 01925 ******************************************************************************/ 01926 static void SetVideoExternalInput ( 01927 const vdc5_ext_in_sig_t * const ext_sig_tmp, 01928 const vdc5_regaddr_input_ctrl_t * const input_ctrl) 01929 { 01930 *(input_ctrl->inp_sel_cnt) &= (uint32_t)~VDC5_REG_MASK_0X00007111; 01931 /* External input format select */ 01932 if (ext_sig_tmp->inp_format == VDC5_EXTIN_FORMAT_YCBCR444 ) { 01933 *(input_ctrl->inp_sel_cnt) |= (uint32_t)VDC5_EXTIN_FORMAT_RGB888 << VDC5_REG_SHIFT_12; 01934 } else { 01935 *(input_ctrl->inp_sel_cnt) |= (uint32_t)ext_sig_tmp->inp_format << VDC5_REG_SHIFT_12; 01936 } 01937 /* Clock edge select for capturing external input video image signals */ 01938 if (ext_sig_tmp->inp_pxd_edge != VDC5_EDGE_RISING ) { 01939 *(input_ctrl->inp_sel_cnt) |= (uint32_t)VDC5_REG_BIT8; 01940 } 01941 /* Clock edge select for capturing external input Vsync signals */ 01942 if (ext_sig_tmp->inp_vs_edge != VDC5_EDGE_RISING ) { 01943 *(input_ctrl->inp_sel_cnt) |= (uint32_t)VDC5_REG_BIT4; 01944 } 01945 /* Clock edge select for capturing external input Hsync signals */ 01946 if (ext_sig_tmp->inp_hs_edge != VDC5_EDGE_RISING ) { 01947 *(input_ctrl->inp_sel_cnt) |= (uint32_t)VDC5_REG_BIT0; 01948 } 01949 01950 *(input_ctrl->inp_ext_sync_cnt) &= (uint32_t)~VDC5_REG_MASK_0X11110113; 01951 /* External input bit endian change on/off control */ 01952 if (ext_sig_tmp->inp_endian_on != VDC5_OFF ) { 01953 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT28; 01954 } 01955 /* External input B/R signal swap on/off control */ 01956 if (ext_sig_tmp->inp_swap_on != VDC5_OFF ) { 01957 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT24; 01958 } 01959 /* External input Vsync signal DV_VSYNC inversion control */ 01960 if (ext_sig_tmp->inp_vs_inv != VDC5_SIG_POL_NOT_INVERTED ) { 01961 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT20; 01962 } 01963 /* External input Hsync signal DV_HSYNC inversion control */ 01964 if (ext_sig_tmp->inp_hs_inv != VDC5_SIG_POL_NOT_INVERTED ) { 01965 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT16; 01966 } 01967 /* Reference select for external input BT.656 Hsync signal */ 01968 if (ext_sig_tmp->inp_h_edge_sel != VDC5_EXTIN_REF_H_EAV ) { 01969 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT8; 01970 } 01971 /* Number of lines for BT.656 external input */ 01972 if (ext_sig_tmp->inp_f525_625 != VDC5_EXTIN_LINE_525 ) { 01973 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)VDC5_REG_BIT4; 01974 } 01975 /* Y/Cb/Y/Cr data string start timing to Hsync reference */ 01976 *(input_ctrl->inp_ext_sync_cnt) |= (uint32_t)ext_sig_tmp->inp_h_pos ; 01977 } /* End of function SetVideoExternalInput() */ 01978 01979 /**************************************************************************//** 01980 * @brief Initializes cascaded connection 01981 * @param[in] ch : Channel 01982 * @retval None 01983 ******************************************************************************/ 01984 static void SetInitialConnection (const vdc5_channel_t ch) 01985 { 01986 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 01987 01988 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 01989 01990 /* Cascade ON */ 01991 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT28; 01992 /* Graphics register update control register (GR1_UPDATE) 01993 b8 GR1_UPDATE */ 01994 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT8; 01995 01996 VDC5_ShrdPrmSetCascade(ch, VDC5_ON ); 01997 01998 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_VIN ]; 01999 02000 /* Turns off alpha blending in a rectangular area */ 02001 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_BIT12; 02002 /* Selection of lower-layer plane in scaler, graphics 1 is used as lower-layer graphics. */ 02003 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 02004 /* Graphics display mode in VIN */ 02005 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 02006 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 02007 /* Disable fade-in and fade-out */ 02008 *(img_synthesizer->gr_ab6) &= (uint32_t)~VDC5_REG_MASK_0X01FF00FF; 02009 /* Set the initial alpha value to '255' */ 02010 *(img_synthesizer->gr_ab7) |= (uint32_t)VDC5_REG_MASK_0X00FF0000; 02011 /* Graphics register update control register (GR_VIN_UPDATE) 02012 b8 GR_VIN_UPDATE 02013 b4 GR_VIN_P_VEN */ 02014 *(img_synthesizer->gr_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 02015 02016 } /* End of function SetInitialConnection() */ 02017 02018 /**************************************************************************//** 02019 * @brief Sets background color for graphics 02020 * @param[in] ch : Channel 02021 * @retval None 02022 ******************************************************************************/ 02023 static void SetGrapicsBackgroundColor (const vdc5_channel_t ch) 02024 { 02025 uint32_t bg_color_tmp; 02026 uint32_t bg_color_conv; 02027 volatile uint32_t * bg_color_reg; 02028 volatile uint32_t * update_reg; 02029 vdc5_graphics_type_t gr_type_index; 02030 02031 /* Background color in 24-bit RGB color format or CrYCb format */ 02032 bg_color_tmp = VDC5_ShrdPrmGetBgColor(ch, VDC5_COLOR_SPACE_GBR ); 02033 /* Conversion from RGB into GBR / from CrYCb into YCbCr */ 02034 bg_color_conv = ColorConversionIntoAgbr(bg_color_tmp); 02035 bg_color_conv &= (uint32_t)VDC5_REG_RGB_24BIT_MASK; 02036 02037 /* GR2, GR3, VIN, OIR */ 02038 for (gr_type_index = VDC5_GR_TYPE_GR2 ; gr_type_index < VDC5_GR_TYPE_NUM ; gr_type_index++) { 02039 /* Background color in GBR format (GRx_BASE) */ 02040 bg_color_reg = vdc5_regaddr_img_synthesizer[ch][gr_type_index].gr_base; 02041 *bg_color_reg = bg_color_conv; 02042 /* Graphics register update control register (GRx_UPDATE) 02043 b4 GRx_P_VEN */ 02044 update_reg = vdc5_regaddr_img_synthesizer[ch][gr_type_index].gr_update; 02045 *update_reg |= (uint32_t)VDC5_REG_BIT4; 02046 } 02047 /* For OIR */ 02048 /* Background color in RGB format (OIR_SCL0_OVR1) */ 02049 bg_color_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ].scl0_ovr1; 02050 *bg_color_reg = bg_color_tmp; 02051 /* Register update control register (OIR_SCL0_UPDATE) 02052 b4 SCL0_VEN_B */ 02053 update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ].scl0_update; 02054 *update_reg |= (uint32_t)VDC5_REG_BIT4; 02055 } /* End of function SetGrapicsBackgroundColor() */ 02056 02057 /**************************************************************************//** 02058 * @brief Sets background color for scaler 0, scaler 1, graphics 0, and graphics 1 02059 * 02060 * Description:<br> 02061 * This function should be called only when graphics 0 or graphics 1 is selected. 02062 * @param[in] ch : Channel 02063 * @param[in] graphics_id : Graphics type ID 02064 * @param[in] color_space : Color space (GBR or YCbCr) 02065 * @retval None 02066 ******************************************************************************/ 02067 static void SetScalerBackgroundColor ( 02068 const vdc5_channel_t ch, 02069 const vdc5_graphics_type_t graphics_id, 02070 const vdc5_color_space_t color_space) 02071 { 02072 volatile uint32_t * scl0_update_reg; 02073 volatile uint32_t * scl0_ovr1_reg; 02074 volatile uint32_t * gr_base_reg; 02075 volatile uint32_t * gr_update_reg; 02076 uint32_t bg_color_tmp; 02077 uint32_t bg_color_conv; 02078 02079 if (graphics_id == VDC5_GR_TYPE_GR0 ) { 02080 scl0_ovr1_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl0_ovr1; 02081 scl0_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl0_update; 02082 gr_base_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ].gr_base; 02083 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ].gr_update; 02084 } else { 02085 /* graphics_id == VDC5_GR_TYPE_GR1 */ 02086 scl0_ovr1_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl0_ovr1; 02087 scl0_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl0_update; 02088 gr_base_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ].gr_base; 02089 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ].gr_update; 02090 } 02091 /* Background color in 24-bit RGB color format or CrYCb format */ 02092 bg_color_tmp = VDC5_ShrdPrmGetBgColor(ch, color_space); 02093 /* Conversion from RGB into GBR / from CrYCb into YCbCr */ 02094 bg_color_conv = ColorConversionIntoAgbr(bg_color_tmp); 02095 bg_color_conv &= (uint32_t)VDC5_REG_RGB_24BIT_MASK; 02096 02097 /* Background color in RGB format or CrYCb format */ 02098 *scl0_ovr1_reg = bg_color_tmp; 02099 /* Register update control register 02100 b4 SCL0_VEN_B */ 02101 *scl0_update_reg |= (uint32_t)VDC5_REG_BIT4; 02102 02103 /* Background color in GBR format or YCbCr format */ 02104 *gr_base_reg = bg_color_conv; 02105 /* Graphics register update control register 02106 b4 GRx_P_VEN */ 02107 *gr_update_reg |= (uint32_t)VDC5_REG_BIT4; 02108 } /* End of function SetScalerBackgroundColor() */ 02109 02110 /**************************************************************************//** 02111 * @brief Sets LCD TCON - STVA/VS 02112 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02113 * @param[in] output_ctrl : Output controller registers 02114 * @retval None 02115 ******************************************************************************/ 02116 static void SetLcdTcon_STVA_VS ( 02117 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02118 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02119 { 02120 /* Signal pulse start position */ 02121 *(output_ctrl->tcon_tim_stva1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02122 /* Pulse width */ 02123 *(output_ctrl->tcon_tim_stva1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02124 02125 /* Polarity inversion control of signal */ 02126 if (lcd_tcon->tcon_inv == VDC5_SIG_POL_NOT_INVERTED ) { 02127 *(output_ctrl->tcon_tim_stva2) &= (uint32_t)~VDC5_REG_BIT4; 02128 } else { 02129 *(output_ctrl->tcon_tim_stva2) |= (uint32_t)VDC5_REG_BIT4; 02130 } 02131 } /* End of function SetLcdTcon_STVA_VS() */ 02132 02133 /**************************************************************************//** 02134 * @brief Sets LCD TCON - STVB/VE 02135 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02136 * @param[in] output_ctrl : Output controller registers 02137 * @retval None 02138 ******************************************************************************/ 02139 static void SetLcdTcon_STVB_VE ( 02140 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02141 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02142 { 02143 /* Signal pulse start position */ 02144 *(output_ctrl->tcon_tim_stvb1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02145 /* Pulse width */ 02146 *(output_ctrl->tcon_tim_stvb1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02147 02148 /* Polarity inversion control of signal */ 02149 if (lcd_tcon->tcon_inv == VDC5_SIG_POL_NOT_INVERTED ) { 02150 *(output_ctrl->tcon_tim_stvb2) &= (uint32_t)~VDC5_REG_BIT4; 02151 } else { 02152 *(output_ctrl->tcon_tim_stvb2) |= (uint32_t)VDC5_REG_BIT4; 02153 } 02154 } /* End of function SetLcdTcon_STVB_VE() */ 02155 02156 /**************************************************************************//** 02157 * @brief Sets LCD TCON - STH/HS 02158 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02159 * @param[in] output_ctrl : Output controller registers 02160 * @retval None 02161 ******************************************************************************/ 02162 static void SetLcdTcon_STH_HS ( 02163 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02164 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02165 { 02166 /* Signal pulse start position */ 02167 *(output_ctrl->tcon_tim_sth1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02168 /* Pulse width */ 02169 *(output_ctrl->tcon_tim_sth1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02170 02171 *(output_ctrl->tcon_tim_sth2) &= (uint32_t)~VDC5_REG_MASK_0X00000110; 02172 /* Signal operating reference select */ 02173 if (lcd_tcon->tcon_hs_sel != VDC5_LCD_TCON_REFSEL_HSYNC ) { 02174 *(output_ctrl->tcon_tim_sth2) |= (uint32_t)VDC5_REG_BIT8; 02175 } 02176 /* Polarity inversion control of signal */ 02177 if (lcd_tcon->tcon_inv != VDC5_SIG_POL_NOT_INVERTED ) { 02178 *(output_ctrl->tcon_tim_sth2) |= (uint32_t)VDC5_REG_BIT4; 02179 } 02180 } /* End of function SetLcdTcon_STH_HS() */ 02181 02182 /**************************************************************************//** 02183 * @brief Sets LCD TCON - STB/HE 02184 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02185 * @param[in] output_ctrl : Output controller registers 02186 * @retval None 02187 ******************************************************************************/ 02188 static void SetLcdTcon_STB_HE ( 02189 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02190 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02191 { 02192 /* Signal pulse start position */ 02193 *(output_ctrl->tcon_tim_stb1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02194 /* Pulse width */ 02195 *(output_ctrl->tcon_tim_stb1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02196 02197 *(output_ctrl->tcon_tim_stb2) &= (uint32_t)~VDC5_REG_MASK_0X00000110; 02198 /* Signal operating reference select */ 02199 if (lcd_tcon->tcon_hs_sel != VDC5_LCD_TCON_REFSEL_HSYNC ) { 02200 *(output_ctrl->tcon_tim_stb2) |= (uint32_t)VDC5_REG_BIT8; 02201 } 02202 /* Polarity inversion control of signal */ 02203 if (lcd_tcon->tcon_inv != VDC5_SIG_POL_NOT_INVERTED ) { 02204 *(output_ctrl->tcon_tim_stb2) |= (uint32_t)VDC5_REG_BIT4; 02205 } 02206 } /* End of function SetLcdTcon_STB_HE() */ 02207 02208 /**************************************************************************//** 02209 * @brief Sets LCD TCON - CPV/GCK 02210 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02211 * @param[in] output_ctrl : Output controller registers 02212 * @retval None 02213 ******************************************************************************/ 02214 static void SetLcdTcon_CPV_GCK ( 02215 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02216 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02217 { 02218 /* Signal pulse start position */ 02219 *(output_ctrl->tcon_tim_cpv1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02220 /* Pulse width */ 02221 *(output_ctrl->tcon_tim_cpv1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02222 02223 *(output_ctrl->tcon_tim_cpv2) &= (uint32_t)~VDC5_REG_MASK_0X00000110; 02224 /* Signal operating reference select */ 02225 if (lcd_tcon->tcon_hs_sel != VDC5_LCD_TCON_REFSEL_HSYNC ) { 02226 *(output_ctrl->tcon_tim_cpv2) |= (uint32_t)VDC5_REG_BIT8; 02227 } 02228 /* Polarity inversion control of signal */ 02229 if (lcd_tcon->tcon_inv != VDC5_SIG_POL_NOT_INVERTED ) { 02230 *(output_ctrl->tcon_tim_cpv2) |= (uint32_t)VDC5_REG_BIT4; 02231 } 02232 } /* End of function SetLcdTcon_CPV_GCK() */ 02233 02234 /**************************************************************************//** 02235 * @brief Sets LCD TCON - POLA 02236 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02237 * @param[in] output_ctrl : Output controller registers 02238 * @retval None 02239 ******************************************************************************/ 02240 static void SetLcdTcon_POLA ( 02241 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02242 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02243 { 02244 /* Signal pulse start position */ 02245 *(output_ctrl->tcon_tim_pola1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02246 /* Pulse width */ 02247 *(output_ctrl->tcon_tim_pola1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02248 02249 *(output_ctrl->tcon_tim_pola2) &= (uint32_t)~VDC5_REG_MASK_0X00003110; 02250 /* POLA/POLB signal generation mode select */ 02251 *(output_ctrl->tcon_tim_pola2) |= (uint32_t)lcd_tcon->tcon_md << VDC5_REG_SHIFT_12; 02252 /* Signal operating reference select */ 02253 if (lcd_tcon->tcon_hs_sel != VDC5_LCD_TCON_REFSEL_HSYNC ) { 02254 *(output_ctrl->tcon_tim_pola2) |= (uint32_t)VDC5_REG_BIT8; 02255 } 02256 /* Polarity inversion control of signal */ 02257 if (lcd_tcon->tcon_inv != VDC5_SIG_POL_NOT_INVERTED ) { 02258 *(output_ctrl->tcon_tim_pola2) |= (uint32_t)VDC5_REG_BIT4; 02259 } 02260 } /* End of function SetLcdTcon_POLA() */ 02261 02262 /**************************************************************************//** 02263 * @brief Sets LCD TCON - POLB 02264 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02265 * @param[in] output_ctrl : Output controller registers 02266 * @retval None 02267 ******************************************************************************/ 02268 static void SetLcdTcon_POLB ( 02269 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02270 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02271 { 02272 /* Signal pulse start position */ 02273 *(output_ctrl->tcon_tim_polb1) = (uint32_t)lcd_tcon->tcon_hsvs << VDC5_REG_SHIFT_16; 02274 /* Pulse width */ 02275 *(output_ctrl->tcon_tim_polb1) |= (uint32_t)lcd_tcon->tcon_hwvw ; 02276 02277 *(output_ctrl->tcon_tim_polb2) &= (uint32_t)~VDC5_REG_MASK_0X00003110; 02278 /* POLA/POLB signal generation mode select */ 02279 *(output_ctrl->tcon_tim_polb2) |= (uint32_t)lcd_tcon->tcon_md << VDC5_REG_SHIFT_12; 02280 /* Signal operating reference select */ 02281 if (lcd_tcon->tcon_hs_sel != VDC5_LCD_TCON_REFSEL_HSYNC ) { 02282 *(output_ctrl->tcon_tim_polb2) |= (uint32_t)VDC5_REG_BIT8; 02283 } 02284 /* Polarity inversion control of signal */ 02285 if (lcd_tcon->tcon_inv != VDC5_SIG_POL_NOT_INVERTED ) { 02286 *(output_ctrl->tcon_tim_polb2) |= (uint32_t)VDC5_REG_BIT4; 02287 } 02288 } /* End of function SetLcdTcon_POLB() */ 02289 02290 /**************************************************************************//** 02291 * @brief Sets LCD TCON - DE 02292 * @param[in] lcd_tcon : LCD TCON timing signal parameter 02293 * @param[in] output_ctrl : Output controller registers 02294 * @retval None 02295 ******************************************************************************/ 02296 static void SetLcdTcon_DE ( 02297 const vdc5_lcd_tcon_timing_t * const lcd_tcon, 02298 const vdc5_regaddr_output_ctrl_t * const output_ctrl) 02299 { 02300 /* Polarity inversion control of signal */ 02301 *(output_ctrl->tcon_tim_de) = (lcd_tcon->tcon_inv == VDC5_SIG_POL_NOT_INVERTED ) ? (uint32_t)0u : (uint32_t)1u; 02302 02303 } /* End of function SetLcdTcon_DE() */ 02304 02305 /**************************************************************************//** 02306 * @brief Sets scaling-down 02307 * @param[in] scldw_rot : Scaling-down and rotation parameter 02308 * @param[in] res_inter : Field operating mode select 02309 * @param[in] scaler : Scaler registers 02310 * @retval None 02311 ******************************************************************************/ 02312 static void SetScalingDown ( 02313 const vdc5_scalingdown_rot_t * const scldw_rot, 02314 const vdc5_res_inter_t res_inter, 02315 const vdc5_regaddr_scaler_t * const scaler) 02316 { 02317 uint32_t vw_before_scld; /* Vertical width before scaling-down */ 02318 uint32_t hw_before_scld; /* Horizontal width before scaling-down */ 02319 uint32_t vw_after_scld; /* Vertical width after scaling-down */ 02320 uint32_t hw_after_scld; /* Horizontal width after scaling-down */ 02321 uint32_t scaling_ratio; 02322 02323 vw_before_scld = (uint32_t)scldw_rot->res .vw ; 02324 hw_before_scld = (uint32_t)scldw_rot->res .hw ; 02325 02326 vw_after_scld = (uint32_t)scldw_rot->res_out_vw ; 02327 hw_after_scld = (uint32_t)scldw_rot->res_out_hw ; 02328 02329 /* Vertical scaling-down: ON, vertical scaling-up: OFF */ 02330 *(scaler->scl0_ds1) |= (uint32_t)VDC5_REG_BIT4; 02331 *(scaler->scl0_us1) &= (uint32_t)~VDC5_REG_BIT4; 02332 if (vw_before_scld > vw_after_scld) { 02333 scaling_ratio = CalcScalingRatio(vw_before_scld, vw_after_scld, scldw_rot->adj_sel , VDC5_OFF ); 02334 02335 *(scaler->scl0_ds6) = scaling_ratio; 02336 } else { 02337 *(scaler->scl0_ds6) = (uint32_t)SCALING_RATIO_SAME_SIZE; 02338 } 02339 02340 /* Horizontal scaling-down */ 02341 if (hw_before_scld > hw_after_scld) { 02342 /* ON */ 02343 *(scaler->scl0_ds1) |= (uint32_t)VDC5_REG_BIT0; 02344 02345 scaling_ratio = CalcScalingRatio(hw_before_scld, hw_after_scld, VDC5_ON , VDC5_ON ); 02346 02347 *(scaler->scl0_ds4) &= (uint32_t)~VDC5_REG_MASK_0X0000FFFF; 02348 *(scaler->scl0_ds4) |= scaling_ratio; 02349 } else { 02350 /* OFF */ 02351 *(scaler->scl0_ds1) &= (uint32_t)~VDC5_REG_BIT0; 02352 } 02353 02354 /* Initial scaling phase */ 02355 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02356 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02357 if (res_inter != VDC5_RES_INTER_PROGRESSIVE ) { 02358 /* Top */ 02359 *(scaler->scl0_ds5) |= INITIAL_SCALING_PHASE << VDC5_REG_SHIFT_16; 02360 } 02361 02362 } /* End of function SetScalingDown() */ 02363 02364 /**************************************************************************//** 02365 * @brief Sets scaling-up 02366 * @param[in] w_read_fb : Size of the frame buffer to be read 02367 * @param[in] gr_grc : Graphics display area 02368 * @param[in] adj_sel : Folding handling (on/off) 02369 * @param[in] ip_conversion : IP conversion on/off 02370 * @param[in] res_ds_wr_md : Frame buffer writing mode for image processing 02371 * @param[in] ln_off_dir : Line offset address direction of the frame buffer 02372 * @param[in] scaler : Scaler registers 02373 * @retval None 02374 ******************************************************************************/ 02375 static void SetScalingUp ( 02376 const vdc5_width_read_fb_t * const w_read_fb, 02377 const vdc5_period_rect_t * const gr_grc, 02378 const vdc5_onoff_t adj_sel, 02379 const vdc5_onoff_t ip_conversion, 02380 const vdc5_wr_md_t res_ds_wr_md, 02381 const vdc5_gr_ln_off_dir_t ln_off_dir, 02382 const vdc5_regaddr_scaler_t * const scaler) 02383 { 02384 uint32_t w_before_scl; /* Width before scaling-up */ 02385 uint32_t w_after_scl; /* Width after scaling-up */ 02386 uint32_t scaling_ratio; 02387 vdc5_onoff_t vscl_up; /* Vertical scaling-up */ 02388 02389 vscl_up = VDC5_OFF ; 02390 02391 /* Vertical scaling-up */ 02392 w_before_scl = (uint32_t)w_read_fb->in_vw ; 02393 w_after_scl = (uint32_t)gr_grc->vw ; 02394 if (w_before_scl < w_after_scl) { 02395 /* Vertical scaling-down: OFF, vertical scaling-up: ON */ 02396 *(scaler->scl0_ds1) &= (uint32_t)~VDC5_REG_BIT4; 02397 *(scaler->scl0_us1) |= (uint32_t)VDC5_REG_BIT4; 02398 02399 scaling_ratio = CalcScalingRatio(w_before_scl, w_after_scl, adj_sel, VDC5_OFF ); 02400 *(scaler->scl0_ds6) = scaling_ratio; 02401 02402 vscl_up = VDC5_ON ; 02403 } else { 02404 /* Vertical scaling-up: OFF */ 02405 *(scaler->scl0_us1) &= (uint32_t)~VDC5_REG_BIT4; 02406 } 02407 02408 /* Horizontal scaling-up */ 02409 w_before_scl = (uint32_t)w_read_fb->in_hw ; 02410 w_after_scl = (uint32_t)gr_grc->hw ; 02411 if (w_before_scl < w_after_scl) { 02412 /* Horizontal scaling-up: ON */ 02413 *(scaler->scl0_us1) |= (uint32_t)VDC5_REG_BIT0; 02414 02415 scaling_ratio = CalcScalingRatio(w_before_scl, w_after_scl, adj_sel, VDC5_OFF ); 02416 02417 *(scaler->scl0_us5) &= (uint32_t)~VDC5_REG_MASK_0X0000FFFF; 02418 *(scaler->scl0_us5) |= scaling_ratio; 02419 } else { 02420 /* Horizontal scaling-up: OFF */ 02421 *(scaler->scl0_us1) &= (uint32_t)~VDC5_REG_BIT0; 02422 } 02423 02424 /* Initial scaling phase */ 02425 if (ip_conversion == VDC5_OFF ) { 02426 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02427 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02428 } else { 02429 if (vscl_up != VDC5_OFF ) { 02430 switch (res_ds_wr_md) { 02431 case VDC5_WR_MD_ROT_90DEG : 02432 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02433 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02434 /* US_HB */ 02435 *(scaler->scl0_us6) |= INITIAL_SCALING_PHASE; 02436 break; 02437 case VDC5_WR_MD_ROT_180DEG : 02438 if (ln_off_dir == VDC5_GR_LN_OFF_DIR_INC ) { 02439 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02440 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02441 /* BTM */ 02442 *(scaler->scl0_ds5) |= INITIAL_SCALING_PHASE; 02443 } 02444 break; 02445 case VDC5_WR_MD_ROT_270DEG : 02446 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02447 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02448 /* US_HT */ 02449 *(scaler->scl0_us6) |= INITIAL_SCALING_PHASE << VDC5_REG_SHIFT_16; 02450 break; 02451 default: /* Normal or horizontal mirroring */ 02452 if (ln_off_dir == VDC5_GR_LN_OFF_DIR_DEC ) { 02453 *(scaler->scl0_ds5) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02454 *(scaler->scl0_us6) &= (uint32_t)~VDC5_REG_MASK_0X0FFF0FFF; 02455 /* BTM */ 02456 *(scaler->scl0_ds5) |= INITIAL_SCALING_PHASE; 02457 } 02458 break; 02459 } 02460 } 02461 } 02462 } /* End of function SetScalingUp() */ 02463 02464 /**************************************************************************//** 02465 * @brief Calculates scaling ratio 02466 * 02467 * Description:<br> 02468 * In this function, the overflow in calculation is not considered. 02469 * before_scl and after_scl are 11bit width, so the overflow does not occur. 02470 * @param[in] before_scl : Size before scaling 02471 * @param[in] after_scl : Size after scaling 02472 * @param[in] adj_sel : Handling for lack of last-input pixel/line, or folding handling 02473 * @param[in] round_up : Round-up on/off 02474 * @retval Scaling ratio 02475 ******************************************************************************/ 02476 static uint32_t CalcScalingRatio ( 02477 const uint32_t before_scl, 02478 const uint32_t after_scl, 02479 const vdc5_onoff_t adj_sel, 02480 const vdc5_onoff_t round_up) 02481 { 02482 float32_t ratio; 02483 float32_t sigma; 02484 int32_t ratio_int; 02485 uint32_t scaling_ratio; 02486 02487 /* When parameter checking is not performed, 02488 following checks are necessary to avoid a division by zero exception and a negative number. */ 02489 if ((after_scl == 0u) || (after_scl == 1u)) { 02490 scaling_ratio = (uint32_t)SCALING_RATIO_SAME_SIZE; 02491 } else { 02492 /* ratio = before_scl * 4096.0 / after_scl */ 02493 ratio = (float32_t)before_scl * (float32_t)SCALING_RATIO_SAME_SIZE; 02494 ratio /= (float32_t)after_scl; 02495 02496 if (adj_sel != VDC5_OFF ) { 02497 sigma = ratio * ((float32_t)after_scl - (float32_t)VDC5_REG_FLOAT_1_0); 02498 sigma -= ((float32_t)before_scl - (float32_t)VDC5_REG_FLOAT_1_0) * (float32_t)SCALING_RATIO_SAME_SIZE; 02499 sigma /= ((float32_t)after_scl - (float32_t)VDC5_REG_FLOAT_1_0); 02500 ratio -= sigma; 02501 } 02502 02503 if (round_up == VDC5_OFF ) { 02504 /* Round off */ 02505 ratio += (float32_t)VDC5_REG_FLOAT_0_5; 02506 ratio_int = (int32_t)ratio; 02507 } else { 02508 /* Round up */ 02509 ratio_int = (int32_t)ratio; 02510 if ((float32_t)ratio_int < ratio) { 02511 ratio_int++; 02512 } 02513 } 02514 scaling_ratio = (uint32_t)ratio_int; 02515 } 02516 return scaling_ratio; 02517 } /* End of function CalcScalingRatio() */ 02518 02519 /**************************************************************************//** 02520 * @brief Sets color matrix in the input controller 02521 * @param[in] ch : Channel 02522 * @param[in] scaling_id : Scaling type ID 02523 * @retval None 02524 ******************************************************************************/ 02525 static void SetInputCntrlColorMtx (const vdc5_channel_t ch, const vdc5_scaling_type_t scaling_id) 02526 { 02527 vdc5_channel_t channel; 02528 vdc5_color_space_t color_space_input; 02529 vdc5_color_space_t color_space_fb; 02530 vdc5_colormtx_mode_t mtx_mode_tmp; 02531 const uint16_t * offset_tmp; 02532 const uint16_t * gain_tmp; 02533 02534 if (scaling_id == VDC5_SC_TYPE_SC0 ) { 02535 channel = ch; 02536 } else { 02537 channel = (ch == VDC5_CHANNEL_0 ) ? VDC5_CHANNEL_1 : VDC5_CHANNEL_0 ; 02538 } 02539 color_space_input = VDC5_ShrdPrmGetColorSpace(channel); 02540 color_space_fb = VDC5_ShrdPrmGetColorSpaceFbWr(ch, scaling_id); 02541 /* Color matrix operating mode */ 02542 if (color_space_input == VDC5_COLOR_SPACE_GBR ) { 02543 if (color_space_fb == VDC5_COLOR_SPACE_GBR ) { 02544 /* GBR to GBR */ 02545 mtx_mode_tmp = VDC5_COLORMTX_GBR_GBR ; 02546 } else { 02547 /* GBR to YCbCr */ 02548 mtx_mode_tmp = VDC5_COLORMTX_GBR_YCBCR ; 02549 } 02550 } else { 02551 if (color_space_fb == VDC5_COLOR_SPACE_GBR ) { 02552 /* YCbCr to GBR */ 02553 mtx_mode_tmp = VDC5_COLORMTX_YCBCR_GBR ; 02554 } else { 02555 /* YCbCr to YCbCr */ 02556 mtx_mode_tmp = VDC5_COLORMTX_YCBCR_YCBCR ; 02557 } 02558 } 02559 /* Color matrix offset (DC) adjustment (YG, B, and R) */ 02560 offset_tmp = colormtx_offset_adj ; 02561 /* Color matrix signal gain adjustment (GG, GB, GR, BG, BB, BR, RG, RB, and RR) */ 02562 gain_tmp = colormtx_gain_adj[mtx_mode_tmp]; 02563 02564 SetColorMatrix(mtx_mode_tmp, offset_tmp, gain_tmp, &vdc5_regaddr_color_matrix[channel][VDC5_COLORMTX_IMGCNT ]); 02565 02566 } /* End of function SetInputCntrlColorMtx() */ 02567 02568 /**************************************************************************//** 02569 * @brief Confirms whether the graphics enlargement will occur or not 02570 * @param[in] graphics_id : Graphics type ID 02571 * @param[in] gr_flm_sel : Frame buffer address setting signal 02572 * @param[in] w_read_fb : Size of the frame buffer to be read 02573 * @param[in] gr_grc : Graphics display area 02574 * @retval Graphics enlargement on/off 02575 ******************************************************************************/ 02576 static vdc5_onoff_t ConfirmGraphicsEnlargement ( 02577 const vdc5_graphics_type_t graphics_id, 02578 const vdc5_gr_flm_sel_t gr_flm_sel, 02579 const vdc5_width_read_fb_t * const w_read_fb, 02580 const vdc5_period_rect_t * const gr_grc) 02581 { 02582 vdc5_onoff_t graphics_enlargement; 02583 02584 graphics_enlargement = VDC5_OFF ; 02585 if (gr_flm_sel == VDC5_GR_FLM_SEL_FLM_NUM ) { 02586 if ((graphics_id == VDC5_GR_TYPE_GR0 ) || (graphics_id == VDC5_GR_TYPE_GR1 )) { 02587 if (((uint32_t)w_read_fb->in_vw < (uint32_t)gr_grc->vw ) || 02588 ((uint32_t)w_read_fb->in_hw < (uint32_t)gr_grc->hw )) { 02589 graphics_enlargement = VDC5_ON ; 02590 } 02591 } 02592 } else { 02593 if (((uint32_t)w_read_fb->in_vw < (uint32_t)gr_grc->vw ) || ((uint32_t)w_read_fb->in_hw < (uint32_t)gr_grc->hw )) { 02594 graphics_enlargement = VDC5_ON ; 02595 } 02596 } 02597 return graphics_enlargement; 02598 } /* End of function ConfirmGraphicsEnlargement() */ 02599 02600 /**************************************************************************//** 02601 * @brief Sets scaler for graphics 02602 * @param[in] ch : Channel 02603 * @param[in] graphics_id : Graphics type ID 02604 * @param[in] w_read_fb : Size of the frame buffer to be read 02605 * @param[in] gr_grc : Graphics display area 02606 * @param[in] gr_flm_sel : Frame buffer address setting signal 02607 * @param[in] gr_enlarge : Graphics enlargement on/off 02608 * @retval None 02609 ******************************************************************************/ 02610 static void SetScalerGraphics ( 02611 const vdc5_channel_t ch, 02612 const vdc5_graphics_type_t graphics_id, 02613 const vdc5_width_read_fb_t * const w_read_fb, 02614 const vdc5_period_rect_t * const gr_grc, 02615 const vdc5_gr_flm_sel_t gr_flm_sel, 02616 const vdc5_onoff_t gr_enlarge) 02617 { 02618 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 02619 const vdc5_regaddr_scaler_t * scaler; 02620 vdc5_scaling_type_t scaling_id; 02621 vdc5_res_inter_t res_inter_tmp; 02622 vdc5_wr_md_t res_ds_wr_md_tmp; 02623 vdc5_onoff_t adj_sel_tmp; 02624 vdc5_onoff_t ip_conversion; 02625 uint32_t reg_data; 02626 vdc5_gr_ln_off_dir_t ln_off_dir; 02627 02628 if (graphics_id == VDC5_GR_TYPE_GR0 ) { 02629 /* Scaler 0 */ 02630 scaling_id = VDC5_SC_TYPE_SC0 ; 02631 } else if (graphics_id == VDC5_GR_TYPE_GR1 ) { 02632 /* Scaler 1 */ 02633 scaling_id = VDC5_SC_TYPE_SC1 ; 02634 } else { 02635 /* OIR */ 02636 scaling_id = VDC5_SC_TYPE_OIR ; 02637 } 02638 02639 scaler = &vdc5_regaddr_scaler[ch][scaling_id]; 02640 02641 if ((gr_flm_sel == VDC5_GR_FLM_SEL_FLM_NUM ) && (gr_enlarge == VDC5_OFF )) { 02642 /* Normal graphics display */ 02643 /* Sync signals from the graphics processing block */ 02644 *(scaler->scl0_us8) |= (uint32_t)VDC5_REG_BIT4; 02645 02646 /* Register update control register (SC0_SCL0_UPDATE, SC1_SCL0_UPDATE, OIR_SCL0_UPDATE) 02647 b13 SCL0_VEN_D 02648 b4 SCL0_VEN_B */ 02649 *(scaler->scl0_update) |= (uint32_t)(VDC5_REG_BIT13 | VDC5_REG_BIT4); 02650 } else { 02651 /* Video image or graphics enlargement */ 02652 if (graphics_id == VDC5_GR_TYPE_OIR ) { 02653 /* Turn the vertical scaling-up and the horizontal scaling-up off */ 02654 *(scaler->scl0_us1) &= (uint32_t)~VDC5_REG_MASK_0X00000011; 02655 /* Sync signals from the graphics processing block */ 02656 *(scaler->scl0_us8) |= (uint32_t)VDC5_REG_BIT4; 02657 } else { 02658 /* Sync signals from the scaling-up control block */ 02659 *(scaler->scl0_us8) &= (uint32_t)~VDC5_REG_BIT4; 02660 02661 /* Graphics display area */ 02662 *(scaler->scl0_us2) = (uint32_t)gr_grc->vs << VDC5_REG_SHIFT_16; 02663 *(scaler->scl0_us2) |= (uint32_t)gr_grc->vw ; 02664 *(scaler->scl0_us3) = (uint32_t)gr_grc->hs << VDC5_REG_SHIFT_16; 02665 *(scaler->scl0_us3) |= (uint32_t)gr_grc->hw ; 02666 02667 *(scaler->scl0_us4) = (uint32_t)w_read_fb->in_vw << VDC5_REG_SHIFT_16; 02668 *(scaler->scl0_us4) |= (uint32_t)w_read_fb->in_hw ; 02669 02670 res_ds_wr_md_tmp = VDC5_ShrdPrmGetWritingMode(ch, scaling_id); 02671 adj_sel_tmp = VDC5_ShrdPrmGetMeasureFolding(ch, graphics_id); 02672 ip_conversion = VDC5_OFF ; 02673 if ((gr_flm_sel == VDC5_GR_FLM_SEL_SCALE_DOWN ) || (gr_flm_sel == VDC5_GR_FLM_SEL_POINTER_BUFF )) { 02674 res_inter_tmp = VDC5_ShrdPrmGetInterlace(ch, scaling_id); 02675 if (res_inter_tmp != VDC5_RES_INTER_PROGRESSIVE ) { 02676 ip_conversion = VDC5_ON ; 02677 } 02678 } 02679 02680 ln_off_dir = VDC5_ShrdPrmGetLineOfsAddrDir(ch, graphics_id); 02681 /* Scaling-up */ 02682 SetScalingUp(w_read_fb, gr_grc, adj_sel_tmp, ip_conversion, res_ds_wr_md_tmp, ln_off_dir, scaler); 02683 } 02684 /* Register update control register (SC0_SCL0_UPDATE, SC1_SCL0_UPDATE, OIR_SCL0_UPDATE) 02685 b13 SCL0_VEN_D 02686 b8 SCL0_UPDATE 02687 b4 SCL0_VEN_B 02688 b0 SCL0_VEN_A */ 02689 *(scaler->scl0_update) |= (uint32_t)(VDC5_REG_BIT13 | VDC5_REG_BIT8 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 02690 } 02691 02692 if (gr_flm_sel != VDC5_GR_FLM_SEL_FLM_NUM ) { 02693 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 02694 /* Frame buffer frame offset address */ 02695 reg_data = *(scaler->scl1_wr4); 02696 *(img_synthesizer->gr_flm4) = reg_data; 02697 02698 /* Graphics register update control register (GRx_UPDATE) 02699 b0 GRx_IBUS_VEN */ 02700 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT0; 02701 } 02702 } /* End of function SetScalerGraphics() */ 02703 02704 /**************************************************************************//** 02705 * @brief Gets the amount of data to be skipped through 02706 * @param[in] gr_base : Frame buffer base address 02707 * @param[in] gr_format : Graphics format 02708 * @retval The amount of data to be skipped through 02709 ******************************************************************************/ 02710 static uint32_t DisplayStartPixelSetting (const uint32_t gr_base, const vdc5_gr_format_t gr_format) 02711 { 02712 uint32_t gr_sta_pos; 02713 static const uint32_t bits_per_pixel[VDC5_GR_FORMAT_NUM ] = { 02714 VDC5_REG_BIT_PER_PIXEL_RGB565, /* RGB565 */ 02715 VDC5_REG_BIT_PER_PIXEL_RGB888, /* RGB888 */ 02716 VDC5_REG_BIT_PER_PIXEL_ARGB1555, /* ARGB1555 */ 02717 VDC5_REG_BIT_PER_PIXEL_ARGB4444, /* ARGB4444 */ 02718 VDC5_REG_BIT_PER_PIXEL_ARGB8888, /* ARGB8888 */ 02719 VDC5_REG_BIT_PER_PIXEL_CLUT8, /* CLUT8 */ 02720 VDC5_REG_BIT_PER_PIXEL_CLUT4, /* CLUT4 */ 02721 VDC5_REG_BIT_PER_PIXEL_CLUT1, /* CLUT1 */ 02722 VDC5_REG_BIT_PER_PIXEL_YCBCR422, /* YCbCr422: In the YCbCr422 format, 32 bits are used for two pixels. */ 02723 VDC5_REG_BIT_PER_PIXEL_YCBCR444, /* YCbCr444 */ 02724 VDC5_REG_BIT_PER_PIXEL_RGBA5551, /* RGBA5551 */ 02725 VDC5_REG_BIT_PER_PIXEL_RGBA8888 /* RGBA8888 */ 02726 }; 02727 02728 gr_sta_pos = gr_base & (uint32_t)VDC5_REG_MASK_0X00000007; 02729 gr_sta_pos *= (uint32_t)VDC5_REG_BIT_PER_PIXEL_VALUE_8; 02730 gr_sta_pos /= bits_per_pixel[gr_format]; 02731 gr_sta_pos &= (uint32_t)VDC5_REG_MASK_0X0000003F; 02732 02733 return gr_sta_pos; 02734 } /* End of function DisplayStartPixelSetting() */ 02735 02736 /**************************************************************************//** 02737 * @brief Sets cascaded connection 02738 * @param[in] ch : Channel 02739 * @param[in] graphics_id : Graphics type ID 02740 * @param[in] gr_flm_sel : Frame buffer address setting signal 02741 * @param[in] gr_enlarge : Graphics enlargement on/off 02742 * @retval Cascade ON/OFF 02743 ******************************************************************************/ 02744 static vdc5_onoff_t SetCascade ( 02745 const vdc5_channel_t ch, 02746 const vdc5_graphics_type_t graphics_id, 02747 const vdc5_gr_flm_sel_t gr_flm_sel, 02748 const vdc5_onoff_t gr_enlarge) 02749 { 02750 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 02751 const vdc5_regaddr_scaler_t * scaler_0; 02752 const vdc5_regaddr_scaler_t * scaler_1; 02753 vdc5_res_vs_in_sel_t res_vs_in_sel_tmp; 02754 vdc5_onoff_t cascade; 02755 02756 cascade = VDC5_ShrdPrmGetCascade(ch); 02757 /* Cascade */ 02758 if (graphics_id == VDC5_GR_TYPE_GR1 ) { 02759 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 02760 scaler_0 = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ]; 02761 scaler_1 = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ]; 02762 02763 if ((gr_flm_sel != VDC5_GR_FLM_SEL_FLM_NUM ) || (gr_enlarge != VDC5_OFF )) { 02764 /* Video image or graphics enlargement */ 02765 /* Cascade OFF */ 02766 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_BIT28; 02767 02768 cascade = VDC5_OFF ; 02769 /* Get sync signal output and full-screen enable signal select */ 02770 res_vs_in_sel_tmp = VDC5_ShrdPrmGetVsInSel(ch); 02771 if (res_vs_in_sel_tmp == VDC5_RES_VS_IN_SEL_SC0 ) { 02772 /* Use Vsync and enable signal output from scaler 0 */ 02773 *(scaler_0->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT8; 02774 *(scaler_1->scl0_frc3) |= (uint32_t)VDC5_REG_BIT8; 02775 } else { 02776 /* Use Vsync and enable signal output from scaler 1 */ 02777 *(scaler_0->scl0_frc3) |= (uint32_t)VDC5_REG_BIT8; 02778 *(scaler_1->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT8; 02779 } 02780 } else { 02781 /* Cascade ON */ 02782 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_REG_BIT28; 02783 02784 cascade = VDC5_ON ; 02785 /* Use Vsync and enable signal output from scaler 0 */ 02786 *(scaler_0->scl0_frc3) &= (uint32_t)~VDC5_REG_BIT8; 02787 } 02788 VDC5_ShrdPrmSetCascade(ch, cascade); 02789 02790 /* Register update control register (SC0_SCL0_UPDATE) 02791 b8 SCL0_UPDATE */ 02792 *(scaler_0->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 02793 /* Register update control register (SC1_SCL0_UPDATE) 02794 b8 SCL0_UPDATE */ 02795 *(scaler_1->scl0_update) |= (uint32_t)VDC5_REG_BIT8; 02796 /* Graphics register update control register (GR1_UPDATE) 02797 b8 GR1_UPDATE */ 02798 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT8; 02799 } 02800 return cascade; 02801 } /* End of function SetCascade() */ 02802 02803 /**************************************************************************//** 02804 * @brief Sets VIN synthesizer 02805 * @param[in] ch : Channel 02806 * @param[in] graphics_id : Graphics type ID 02807 * @param[in] gr_flm_sel : Frame buffer address setting signal 02808 * @param[in] gr_enlarge : Graphics enlargement on/off 02809 * @retval None 02810 ******************************************************************************/ 02811 static void SetupGraphicsVIN ( 02812 const vdc5_channel_t ch, 02813 const vdc5_graphics_type_t graphics_id, 02814 const vdc5_gr_flm_sel_t gr_flm_sel, 02815 const vdc5_onoff_t gr_enlarge) 02816 { 02817 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 02818 const vdc5_regaddr_img_synthesizer_t * img_synthesizer_vin; 02819 vdc5_onoff_t cascade; 02820 vdc5_resource_state_t rsrc_state; 02821 vdc5_onoff_t und_sel; 02822 uint32_t reg_data; 02823 02824 /* Cascade */ 02825 cascade = SetCascade(ch, graphics_id, gr_flm_sel, gr_enlarge); 02826 02827 /* Display area for VIN */ 02828 rsrc_state = VDC5_ShrdPrmGetLayerResource(ch, VDC5_LAYER_ID_0_RD ); 02829 if ((cascade == VDC5_OFF ) && 02830 ((rsrc_state != VDC5_RESOURCE_ST_INVALID ) || (graphics_id == VDC5_GR_TYPE_GR0 ))) { 02831 /* Cascade connection OFF and graphics 0 is used */ 02832 img_synthesizer_vin = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_VIN ]; 02833 02834 und_sel = VDC5_ShrdPrmGetUndSel(ch); 02835 if (und_sel == VDC5_OFF ) { 02836 /* Graphics 1 is allocated to the upper-layer. */ 02837 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_BIT2; 02838 02839 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 02840 } else { 02841 /* Graphics 0 is allocated to the upper-layer. */ 02842 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 02843 02844 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ]; 02845 } 02846 /* Graphics display mode in VIN */ 02847 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 02848 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_DISPSEL_BLEND ; 02849 /* Copy the graphics display area in the upper-layer to the VIN display area. */ 02850 reg_data = *(img_synthesizer->gr_ab2); 02851 *(img_synthesizer_vin->gr_ab2) = reg_data; 02852 reg_data = *(img_synthesizer->gr_ab3); 02853 *(img_synthesizer_vin->gr_ab3) = reg_data; 02854 /* The valid image area for alpha blending in a rectangular area */ 02855 reg_data = *(img_synthesizer_vin->gr_ab2); 02856 *(img_synthesizer_vin->gr_ab4) = reg_data; 02857 reg_data = *(img_synthesizer_vin->gr_ab3); 02858 *(img_synthesizer_vin->gr_ab5) = reg_data; 02859 02860 /* Graphics register update control register (GR_VIN_UPDATE) 02861 b8 GR_VIN_UPDATE 02862 b4 GR_VIN_P_VEN */ 02863 *(img_synthesizer_vin->gr_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 02864 } 02865 } /* End of function SetupGraphicsVIN() */ 02866 02867 /**************************************************************************//** 02868 * @brief Changes VIN synthesizer settings 02869 * @param[in] ch : Channel 02870 * @param[in] graphics_id : Graphics type ID 02871 * @param[in] gr_flm_sel : Frame buffer address setting signal 02872 * @param[in] gr_enlarge : Graphics enlargement on/off 02873 * @retval None 02874 ******************************************************************************/ 02875 static void ChangeGraphicsVIN ( 02876 const vdc5_channel_t ch, 02877 const vdc5_graphics_type_t graphics_id, 02878 const vdc5_gr_flm_sel_t gr_flm_sel, 02879 const vdc5_onoff_t gr_enlarge) 02880 { 02881 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 02882 const vdc5_regaddr_img_synthesizer_t * img_synthesizer_vin; 02883 vdc5_onoff_t cascade; 02884 vdc5_onoff_t cascade_prev; 02885 vdc5_resource_state_t rsrc_state; 02886 vdc5_onoff_t und_sel; 02887 uint32_t reg_data; 02888 02889 /* Cascade */ 02890 cascade_prev = VDC5_ShrdPrmGetCascade(ch); 02891 cascade = SetCascade(ch, graphics_id, gr_flm_sel, gr_enlarge); 02892 02893 /* Display area for VIN */ 02894 rsrc_state = VDC5_ShrdPrmGetLayerResource(ch, VDC5_LAYER_ID_0_RD ); 02895 if (rsrc_state != VDC5_RESOURCE_ST_INVALID ) { 02896 /* Graphics 0 is used */ 02897 img_synthesizer_vin = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_VIN ]; 02898 if (cascade == VDC5_OFF ) { 02899 /* Cascade connection is OFF */ 02900 und_sel = VDC5_ShrdPrmGetUndSel(ch); 02901 if (und_sel == VDC5_OFF ) { 02902 /* Graphics 1 is allocated to the upper-layer. */ 02903 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_BIT2; 02904 02905 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 02906 } else { 02907 /* Graphics 0 is allocated to the upper-layer. */ 02908 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 02909 02910 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ]; 02911 } 02912 /* Graphics display mode in VIN */ 02913 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 02914 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_DISPSEL_BLEND ; 02915 /* Copy the graphics display area in the upper-layer to the VIN display area. */ 02916 reg_data = *(img_synthesizer->gr_ab2); 02917 *(img_synthesizer_vin->gr_ab2) = reg_data; 02918 reg_data = *(img_synthesizer->gr_ab3); 02919 *(img_synthesizer_vin->gr_ab3) = reg_data; 02920 } else { 02921 /* Cascade connection is ON */ 02922 if (cascade_prev == VDC5_OFF ) { 02923 /* Cascade connection was turned on in this function. */ 02924 /* Selection of lower-layer plane in scaler is set to 1. */ 02925 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 02926 /* Graphics display mode in VIN */ 02927 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 02928 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 02929 } 02930 } 02931 /* Graphics register update control register (GR_VIN_UPDATE) 02932 b8 GR_VIN_UPDATE 02933 b4 GR_VIN_P_VEN */ 02934 *(img_synthesizer_vin->gr_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 02935 } 02936 } /* End of function ChangeGraphicsVIN() */ 02937 02938 /**************************************************************************//** 02939 * @brief Sets color matrix in the image quality improver 02940 * @param[in] ch : Channel 02941 * @param[in] graphics_id : Graphics type ID 02942 * @param[in] color_space : Color space (GBR or YCbCr) 02943 * @retval None 02944 ******************************************************************************/ 02945 static void SetImgQaImproverColorMtx ( 02946 const vdc5_channel_t ch, 02947 const vdc5_graphics_type_t graphics_id, 02948 const vdc5_color_space_t color_space) 02949 { 02950 vdc5_colormtx_module_t module_tmp; 02951 vdc5_colormtx_mode_t mtx_mode_tmp; 02952 const uint16_t * offset_tmp; 02953 const uint16_t * gain_tmp; 02954 02955 /* Color matrix operating mode */ 02956 if (color_space == VDC5_COLOR_SPACE_GBR ) { 02957 mtx_mode_tmp = VDC5_COLORMTX_GBR_GBR ; 02958 } else { 02959 mtx_mode_tmp = VDC5_COLORMTX_YCBCR_GBR ; 02960 } 02961 /* Color matrix module */ 02962 module_tmp = (graphics_id == VDC5_GR_TYPE_GR0 ) ? VDC5_COLORMTX_ADJ_0 : VDC5_COLORMTX_ADJ_1 ; 02963 /* Color matrix offset (DC) adjustment (YG, B, and R) */ 02964 offset_tmp = colormtx_offset_adj ; 02965 /* Color matrix signal gain adjustment (GG, GB, GR, BG, BB, BR, RG, RB, and RR) */ 02966 gain_tmp = colormtx_gain_adj[mtx_mode_tmp]; 02967 02968 SetColorMatrix(mtx_mode_tmp, offset_tmp, gain_tmp, &vdc5_regaddr_color_matrix[ch][module_tmp]); 02969 02970 } /* End of function SetImgQaImproverColorMtx() */ 02971 02972 /**************************************************************************//** 02973 * @brief Activates/deactivates frame buffer writing for scaler 0 02974 * @param[in] ch : Channel 02975 * @param[in] ability : Ability to enable read/write access to the memory 02976 * @retval None 02977 ******************************************************************************/ 02978 static void SetRwProcAbility_Write_0 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 02979 { 02980 const vdc5_regaddr_scaler_t * scaler; 02981 volatile uint32_t dummy_read; 02982 uint32_t reg_data; 02983 02984 /* SC0/GR0 writing */ 02985 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ]; 02986 02987 if (ability == VDC5_OFF ) { 02988 /* SC0 frame buffer writing is disabled */ 02989 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT0; 02990 02991 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_0_WR ); 02992 } else { 02993 /* SC0 frame buffer writing is enabled */ 02994 *(scaler->scl1_wr5) |= (uint32_t)VDC5_REG_BIT0; 02995 /* Reset and reset cancellation of the pointer buffer with dummy read */ 02996 *(scaler->scl1_pbuf_cnt) |= (uint32_t)VDC5_REG_BIT16; 02997 reg_data = *(scaler->scl1_pbuf_cnt); 02998 dummy_read = reg_data; 02999 *(scaler->scl1_pbuf_cnt) &= (uint32_t)~VDC5_REG_BIT16; 03000 reg_data = *(scaler->scl1_pbuf_cnt); 03001 dummy_read = reg_data; 03002 03003 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_0_WR ); 03004 } 03005 } /* End of function SetRwProcAbility_Write_0() */ 03006 03007 /**************************************************************************//** 03008 * @brief Activates/deactivates frame buffer writing for scaler 1 03009 * @param[in] ch : Channel 03010 * @param[in] ability : Ability to enable read/write access to the memory 03011 * @retval None 03012 ******************************************************************************/ 03013 static void SetRwProcAbility_Write_1 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03014 { 03015 const vdc5_regaddr_scaler_t * scaler; 03016 volatile uint32_t dummy_read; 03017 uint32_t reg_data; 03018 03019 /* SC1/GR1 writing */ 03020 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ]; 03021 03022 if (ability == VDC5_OFF ) { 03023 /* SC1 frame buffer writing is disabled */ 03024 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT0; 03025 03026 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_1_WR ); 03027 } else { 03028 /* SC1 frame buffer writing is enabled */ 03029 *(scaler->scl1_wr5) |= (uint32_t)VDC5_REG_BIT0; 03030 /* Reset and reset cancellation of the pointer buffer with dummy read */ 03031 *(scaler->scl1_pbuf_cnt) |= (uint32_t)VDC5_REG_BIT16; 03032 reg_data = *(scaler->scl1_pbuf_cnt); 03033 dummy_read = reg_data; 03034 *(scaler->scl1_pbuf_cnt) &= (uint32_t)~VDC5_REG_BIT16; 03035 reg_data = *(scaler->scl1_pbuf_cnt); 03036 dummy_read = reg_data; 03037 03038 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_1_WR ); 03039 } 03040 } /* End of function SetRwProcAbility_Write_1() */ 03041 03042 /**************************************************************************//** 03043 * @brief Activates/deactivates frame buffer writing for OIR 03044 * @param[in] ch : Channel 03045 * @param[in] ability : Ability to enable read/write access to the memory 03046 * @retval None 03047 ******************************************************************************/ 03048 static void SetRwProcAbility_Write_OIR (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03049 { 03050 const vdc5_regaddr_scaler_t * scaler; 03051 03052 /* OIR writing */ 03053 scaler = &vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ]; 03054 03055 if (ability == VDC5_OFF ) { 03056 /* OIR frame buffer writing is disabled */ 03057 *(scaler->scl1_wr5) &= (uint32_t)~VDC5_REG_BIT0; 03058 03059 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_OIR_WR ); 03060 } else { 03061 /* OIR frame buffer writing is enabled */ 03062 *(scaler->scl1_wr5) |= (uint32_t)VDC5_REG_BIT0; 03063 03064 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_OIR_WR ); 03065 } 03066 } /* End of function SetRwProcAbility_Write_OIR() */ 03067 03068 /**************************************************************************//** 03069 * @brief Activates/deactivates frame buffer reading for graphics 0 03070 * @param[in] ch : Channel 03071 * @param[in] ability : Ability to enable read/write access to the memory 03072 * @retval None 03073 ******************************************************************************/ 03074 static void SetRwProcAbility_Read_0 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03075 { 03076 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03077 03078 /* SC0/GR0 reading */ 03079 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ]; 03080 03081 if (ability == VDC5_OFF ) { 03082 /* GR0 frame buffer reading is disabled */ 03083 *(img_synthesizer->gr_flm_rd) &= (uint32_t)~VDC5_REG_BIT0; 03084 /* Graphics display mode */ 03085 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03086 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_BACK ; 03087 03088 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_0_RD ); 03089 } else { 03090 /* GR0 frame buffer reading is enabled */ 03091 *(img_synthesizer->gr_flm_rd) |= (uint32_t)VDC5_REG_BIT0; 03092 03093 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_0_RD ); 03094 } 03095 } /* End of function SetRwProcAbility_Read_0() */ 03096 03097 /**************************************************************************//** 03098 * @brief Activates/deactivates frame buffer reading for graphics 1 03099 * @param[in] ch : Channel 03100 * @param[in] ability : Ability to enable read/write access to the memory 03101 * @retval None 03102 ******************************************************************************/ 03103 static void SetRwProcAbility_Read_1 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03104 { 03105 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03106 03107 /* SC1/GR1 reading */ 03108 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 03109 03110 if (ability == VDC5_OFF ) { 03111 /* GR1 frame buffer reading is disabled */ 03112 *(img_synthesizer->gr_flm_rd) &= (uint32_t)~VDC5_REG_BIT0; 03113 /* Graphics display mode */ 03114 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03115 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 03116 03117 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_1_RD ); 03118 } else { 03119 /* GR1 frame buffer reading is enabled */ 03120 *(img_synthesizer->gr_flm_rd) |= (uint32_t)VDC5_REG_BIT0; 03121 03122 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_1_RD ); 03123 } 03124 } /* End of function SetRwProcAbility_Read_1() */ 03125 03126 /**************************************************************************//** 03127 * @brief Activates/deactivates frame buffer reading for graphics 2 03128 * @param[in] ch : Channel 03129 * @param[in] ability : Ability to enable read/write access to the memory 03130 * @retval None 03131 ******************************************************************************/ 03132 static void SetRwProcAbility_Read_2 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03133 { 03134 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03135 03136 /* GR2 reading */ 03137 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR2 ]; 03138 03139 if (ability == VDC5_OFF ) { 03140 /* GR2 frame buffer reading is disabled */ 03141 *(img_synthesizer->gr_flm_rd) &= (uint32_t)~VDC5_REG_BIT0; 03142 /* Graphics display mode */ 03143 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03144 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 03145 03146 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_2_RD ); 03147 } else { 03148 /* GR2 frame buffer reading is enabled */ 03149 *(img_synthesizer->gr_flm_rd) |= (uint32_t)VDC5_REG_BIT0; 03150 03151 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_2_RD ); 03152 } 03153 } /* End of function SetRwProcAbility_Read_2() */ 03154 03155 /**************************************************************************//** 03156 * @brief Activates/deactivates frame buffer reading for graphics 3 03157 * @param[in] ch : Channel 03158 * @param[in] ability : Ability to enable read/write access to the memory 03159 * @retval None 03160 ******************************************************************************/ 03161 static void SetRwProcAbility_Read_3 (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03162 { 03163 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03164 03165 /* GR3 reading */ 03166 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR3 ]; 03167 03168 if (ability == VDC5_OFF ) { 03169 /* GR3 frame buffer reading is disabled */ 03170 *(img_synthesizer->gr_flm_rd) &= (uint32_t)~VDC5_REG_BIT0; 03171 /* Graphics display mode */ 03172 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03173 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_LOWER ; 03174 03175 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_3_RD ); 03176 } else { 03177 /* GR3 frame buffer reading is enabled */ 03178 *(img_synthesizer->gr_flm_rd) |= (uint32_t)VDC5_REG_BIT0; 03179 03180 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_3_RD ); 03181 } 03182 } /* End of function SetRwProcAbility_Read_3() */ 03183 03184 /**************************************************************************//** 03185 * @brief Activates/deactivates frame buffer reading for OIR 03186 * @param[in] ch : Channel 03187 * @param[in] ability : Ability to enable read/write access to the memory 03188 * @retval None 03189 ******************************************************************************/ 03190 static void SetRwProcAbility_Read_OIR (const vdc5_channel_t ch, const vdc5_onoff_t ability) 03191 { 03192 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03193 03194 /* OIR reading */ 03195 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_OIR ]; 03196 03197 if (ability == VDC5_OFF ) { 03198 /* OIR frame buffer reading is disabled */ 03199 *(img_synthesizer->gr_flm_rd) &= (uint32_t)~VDC5_REG_BIT0; 03200 /* Graphics display mode */ 03201 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03202 *(img_synthesizer->gr_ab1) |= (uint32_t)VDC5_DISPSEL_BACK ; 03203 03204 VDC5_ShrdPrmSetRwProcDisable(ch, VDC5_LAYER_ID_OIR_RD ); 03205 } else { 03206 /* OIR frame buffer reading is enabled */ 03207 *(img_synthesizer->gr_flm_rd) |= (uint32_t)VDC5_REG_BIT0; 03208 03209 VDC5_ShrdPrmSetRwProcEnable(ch, VDC5_LAYER_ID_OIR_RD ); 03210 } 03211 } /* End of function SetRwProcAbility_Read_OIR() */ 03212 03213 /**************************************************************************//** 03214 * @brief Sets register update control register to update the frame buffer 03215 * read and write enable settings. 03216 * @param[in] ch : Channel 03217 * @param[in] layer_id : Layer ID 03218 * @retval None 03219 ******************************************************************************/ 03220 static void SetRegUpdateRwEnable (const vdc5_channel_t ch, const vdc5_layer_id_t layer_id) 03221 { 03222 volatile uint32_t * scl1_update_reg; 03223 volatile uint32_t * gr_update_reg; 03224 03225 switch (layer_id) { 03226 case VDC5_LAYER_ID_0_WR : /* Layer 0, write process */ 03227 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl1_update; 03228 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT20 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 03229 break; 03230 case VDC5_LAYER_ID_1_WR : /* Layer 1, write process */ 03231 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl1_update; 03232 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT20 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 03233 break; 03234 case VDC5_LAYER_ID_OIR_WR : /* Layer OIR, write process */ 03235 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ].scl1_update; 03236 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03237 break; 03238 case VDC5_LAYER_ID_0_RD : /* Layer 0, read process */ 03239 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ].gr_update; 03240 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03241 break; 03242 case VDC5_LAYER_ID_1_RD : /* Layer 1, read process */ 03243 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ].gr_update; 03244 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03245 break; 03246 case VDC5_LAYER_ID_2_RD : /* Layer 2, read process */ 03247 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR2 ].gr_update; 03248 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03249 break; 03250 case VDC5_LAYER_ID_3_RD : /* Layer 3, read process */ 03251 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR3 ].gr_update; 03252 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03253 break; 03254 case VDC5_LAYER_ID_OIR_RD : /* Layer OIR, read process */ 03255 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_OIR ].gr_update; 03256 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03257 break; 03258 03259 case VDC5_LAYER_ID_ALL : /* All */ 03260 /* Register update control register (SCx_SCL1_UPDATE) 03261 b20 SCL1_UPDATE_B 03262 b4 SCL1_VEN_B 03263 b0 SCL1_VEN_A */ 03264 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl1_update; 03265 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT20 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 03266 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl1_update; 03267 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT20 | VDC5_REG_BIT4 | VDC5_REG_BIT0); 03268 /* Register update control register (OIR_SCL1_UPDATE) 03269 b4 SCL1_VEN_B 03270 b0 SCL1_VEN_A */ 03271 scl1_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_OIR ].scl1_update; 03272 *scl1_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03273 /* Graphics register update control register (GRx_UPDATE) 03274 b4 GRx_P_VEN 03275 b0 GRx_IBUS_VEN */ 03276 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ].gr_update; 03277 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03278 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ].gr_update; 03279 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03280 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR2 ].gr_update; 03281 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03282 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR3 ].gr_update; 03283 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03284 gr_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_OIR ].gr_update; 03285 *gr_update_reg |= (uint32_t)(VDC5_REG_BIT4|VDC5_REG_BIT0); 03286 break; 03287 03288 default: 03289 /* DO NOTHING */ 03290 break; 03291 } 03292 } /* End of function SetRegUpdateRwEnable() */ 03293 03294 /**************************************************************************//** 03295 * @brief Sets graphics display mode 03296 * @param[in] ch : Channel 03297 * @param[in] layer_id : Layer ID 03298 * @param[in] gr_disp_sel : Graphics display mode 03299 * @retval None 03300 ******************************************************************************/ 03301 static void SetGraphicsDisplayMode ( 03302 const vdc5_channel_t ch, 03303 const vdc5_layer_id_t layer_id, 03304 const vdc5_gr_disp_sel_t * const gr_disp_sel) 03305 { 03306 uint32_t graphics_id; 03307 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03308 03309 if (layer_id == VDC5_LAYER_ID_ALL ) { 03310 for (graphics_id = 0; graphics_id < VDC5_GR_TYPE_NUM ; graphics_id++) { 03311 if ((graphics_id != VDC5_GR_TYPE_VIN ) && (gr_disp_sel[graphics_id] != VDC5_DISPSEL_IGNORED )) { 03312 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 03313 03314 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03315 *(img_synthesizer->gr_ab1) |= (uint32_t)gr_disp_sel[graphics_id]; 03316 /* Graphics register update control register (GRx_UPDATE) 03317 b4 GRx_P_VEN */ 03318 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 03319 } 03320 } 03321 } else if ((layer_id >= VDC5_LAYER_ID_0_RD ) && (layer_id <= VDC5_LAYER_ID_OIR_RD )) { 03322 if (*gr_disp_sel != VDC5_DISPSEL_IGNORED ) { 03323 graphics_id = (uint32_t)(layer_id - VDC5_SC_TYPE_NUM ); 03324 03325 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][graphics_id]; 03326 03327 *(img_synthesizer->gr_ab1) &= (uint32_t)~VDC5_REG_MASK_0X00000003; 03328 *(img_synthesizer->gr_ab1) |= (uint32_t)*gr_disp_sel; 03329 /* Graphics register update control register (GRx_UPDATE) 03330 b4 GRx_P_VEN */ 03331 *(img_synthesizer->gr_update) |= (uint32_t)VDC5_REG_BIT4; 03332 } 03333 } else { 03334 /* Do nothing */ 03335 } 03336 } /* End of function SetGraphicsDisplayMode() */ 03337 03338 /**************************************************************************//** 03339 * @brief Sets color matrix 03340 * @param[in] mtx_mode : Operating mode 03341 * @param[in] offset : Offset (DC) adjustment of Y/G, B, and R signal 03342 * @param[in] gain : GG, GB, GR, BG, BB, BR, RG, RB, and RR signal gain adjustment 03343 * @param[in] color_matrix : Color matrix registers 03344 * @retval None 03345 ******************************************************************************/ 03346 static void SetColorMatrix ( 03347 const vdc5_colormtx_mode_t mtx_mode, 03348 const uint16_t * const offset, 03349 const uint16_t * const gain, 03350 const vdc5_regaddr_color_matrix_t * const color_matrix) 03351 { 03352 /* Operating mode */ 03353 *(color_matrix->mtx_mode) = (uint32_t)mtx_mode; 03354 03355 /* Offset (DC) adjustment of Y/G, B, and R signal and 03356 GG, GB, GR, BG, BB, BR, RG, RB, and RR signal gain adjustment */ 03357 *(color_matrix->mtx_yg_adj0) = (uint32_t)offset[VDC5_COLORMTX_OFFST_YG ] << VDC5_REG_SHIFT_16; 03358 *(color_matrix->mtx_yg_adj0) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_GG ]; 03359 *(color_matrix->mtx_yg_adj1) = (uint32_t)gain[VDC5_COLORMTX_GAIN_GB ] << VDC5_REG_SHIFT_16; 03360 *(color_matrix->mtx_yg_adj1) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_GR ]; 03361 *(color_matrix->mtx_cbb_adj0) = (uint32_t)offset[VDC5_COLORMTX_OFFST_B ] << VDC5_REG_SHIFT_16; 03362 *(color_matrix->mtx_cbb_adj0) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_BG ]; 03363 *(color_matrix->mtx_cbb_adj1) = (uint32_t)gain[VDC5_COLORMTX_GAIN_BB ] << VDC5_REG_SHIFT_16; 03364 *(color_matrix->mtx_cbb_adj1) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_BR ]; 03365 *(color_matrix->mtx_crr_adj0) = (uint32_t)offset[VDC5_COLORMTX_OFFST_R ] << VDC5_REG_SHIFT_16; 03366 *(color_matrix->mtx_crr_adj0) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_RG ]; 03367 *(color_matrix->mtx_crr_adj1) = (uint32_t)gain[VDC5_COLORMTX_GAIN_RB ] << VDC5_REG_SHIFT_16; 03368 *(color_matrix->mtx_crr_adj1) |= (uint32_t)gain[VDC5_COLORMTX_GAIN_RR ]; 03369 03370 /* Register update control register (IMGCNT_UPDATE/ADJx_UPDATE) 03371 b0 IMGCNT_VEN/ADJx_VEN */ 03372 *(color_matrix->mtx_update) |= (uint32_t)VDC5_REG_BIT0; 03373 03374 } /* End of function SetColorMatrix() */ 03375 03376 /**************************************************************************//** 03377 * @brief Sets sharpness 03378 * @param[in] shp_h_on : Sharpness ON/OFF setting 03379 * @param[in] sharp_param : Sharpness ON/OFF setting 03380 * @param[in] img_qlty_imp : Image quality improver registers 03381 * @retval None 03382 ******************************************************************************/ 03383 static void SetImageEnhancementSharpness ( 03384 const vdc5_onoff_t shp_h_on, 03385 const vdc5_enhance_sharp_t * const sharp_param, 03386 const vdc5_regaddr_img_qlty_imp_t * const img_qlty_imp) 03387 { 03388 const vdc5_sharpness_ctrl_t * sharpness_ctrl; 03389 03390 if (sharp_param != NULL) { 03391 /* H1, adjacent pixel used as reference */ 03392 sharpness_ctrl = &sharp_param->hrz_sharp [VDC5_IMGENH_SHARP_H1 ]; 03393 /* Active sharpness range */ 03394 *(img_qlty_imp->adj_enh_shp1) &= (uint32_t)~VDC5_REG_MASK_0X0000003F; 03395 *(img_qlty_imp->adj_enh_shp1) |= (uint32_t)sharpness_ctrl->shp_core ; 03396 /* Sharpness correction value clipping and sharpness edge amplitude value gain */ 03397 *(img_qlty_imp->adj_enh_shp2) = (uint32_t)sharpness_ctrl->shp_clip_o << VDC5_REG_SHIFT_24; 03398 *(img_qlty_imp->adj_enh_shp2) |= (uint32_t)sharpness_ctrl->shp_clip_u << VDC5_REG_SHIFT_16; 03399 *(img_qlty_imp->adj_enh_shp2) |= (uint32_t)sharpness_ctrl->shp_gain_o << VDC5_REG_SHIFT_8; 03400 *(img_qlty_imp->adj_enh_shp2) |= (uint32_t)sharpness_ctrl->shp_gain_u ; 03401 03402 /* H2, second adjacent pixel used as reference */ 03403 sharpness_ctrl = &sharp_param->hrz_sharp [VDC5_IMGENH_SHARP_H2 ]; 03404 /* LPF selection for folding prevention before H2 edge detection */ 03405 if (sharp_param->shp_h2_lpf_sel == VDC5_OFF ) { 03406 *(img_qlty_imp->adj_enh_shp3) = (uint32_t)0x00000000u; 03407 } else { 03408 *(img_qlty_imp->adj_enh_shp3) = (uint32_t)VDC5_REG_BIT16; 03409 } 03410 /* Active sharpness range */ 03411 *(img_qlty_imp->adj_enh_shp3) |= (uint32_t)sharpness_ctrl->shp_core ; 03412 /* Sharpness correction value clipping and sharpness edge amplitude value gain */ 03413 *(img_qlty_imp->adj_enh_shp4) = (uint32_t)sharpness_ctrl->shp_clip_o << VDC5_REG_SHIFT_24; 03414 *(img_qlty_imp->adj_enh_shp4) |= (uint32_t)sharpness_ctrl->shp_clip_u << VDC5_REG_SHIFT_16; 03415 *(img_qlty_imp->adj_enh_shp4) |= (uint32_t)sharpness_ctrl->shp_gain_o << VDC5_REG_SHIFT_8; 03416 *(img_qlty_imp->adj_enh_shp4) |= (uint32_t)sharpness_ctrl->shp_gain_u ; 03417 03418 /* H3, third adjacent pixel used as reference */ 03419 sharpness_ctrl = &sharp_param->hrz_sharp [VDC5_IMGENH_SHARP_H3 ]; 03420 /* Active sharpness range */ 03421 *(img_qlty_imp->adj_enh_shp5) = (uint32_t)sharpness_ctrl->shp_core ; 03422 /* Sharpness correction value clipping and sharpness edge amplitude value gain */ 03423 *(img_qlty_imp->adj_enh_shp6) = (uint32_t)sharpness_ctrl->shp_clip_o << VDC5_REG_SHIFT_24; 03424 *(img_qlty_imp->adj_enh_shp6) |= (uint32_t)sharpness_ctrl->shp_clip_u << VDC5_REG_SHIFT_16; 03425 *(img_qlty_imp->adj_enh_shp6) |= (uint32_t)sharpness_ctrl->shp_gain_o << VDC5_REG_SHIFT_8; 03426 *(img_qlty_imp->adj_enh_shp6) |= (uint32_t)sharpness_ctrl->shp_gain_u ; 03427 } 03428 03429 if (shp_h_on == VDC5_OFF ) { 03430 *(img_qlty_imp->adj_enh_shp1) &= (uint32_t)~VDC5_REG_BIT16; 03431 } else { 03432 *(img_qlty_imp->adj_enh_shp1) |= (uint32_t)VDC5_REG_BIT16; 03433 } 03434 } /* End of function SetImageEnhancementSharpness() */ 03435 03436 /**************************************************************************//** 03437 * @brief Sets LTI 03438 * @param[in] lti_h_on : LTI ON/OFF setting 03439 * @param[in] lti_param : LTI setup parameter 03440 * @param[in] img_qlty_imp : Image quality improver registers 03441 * @retval None 03442 ******************************************************************************/ 03443 static void SetImageEnhancementLti ( 03444 const vdc5_onoff_t lti_h_on, 03445 const vdc5_enhance_lti_t * const lti_param, 03446 const vdc5_regaddr_img_qlty_imp_t * const img_qlty_imp) 03447 { 03448 const vdc5_lti_ctrl_t * lti_ctrl; 03449 03450 if (lti_param != NULL) { 03451 /* H2, second adjacent pixel used as reference */ 03452 lti_ctrl = <i_param->lti [VDC5_IMGENH_LTI1 ]; 03453 /* LPF selection for folding prevention before H2 edge detection */ 03454 if (lti_param->lti_h2_lpf_sel == VDC5_OFF ) { 03455 *(img_qlty_imp->adj_enh_lti1) = (uint32_t)0x00000000u; 03456 } else { 03457 *(img_qlty_imp->adj_enh_lti1) = (uint32_t)VDC5_REG_BIT24; 03458 } 03459 /* Median filter LTI correction threshold */ 03460 *(img_qlty_imp->adj_enh_lti1) |= (uint32_t)((uint32_t)lti_ctrl->lti_inc_zero << VDC5_REG_SHIFT_16); 03461 /* LTI edge amplitude value gain */ 03462 *(img_qlty_imp->adj_enh_lti1) |= (uint32_t)((uint32_t)lti_ctrl->lti_gain << VDC5_REG_SHIFT_8); 03463 /* LTI coring (maximum core value of 255) */ 03464 *(img_qlty_imp->adj_enh_lti1) |= (uint32_t)lti_ctrl->lti_core ; 03465 03466 /* H4, fourth adjacent pixel used as reference */ 03467 lti_ctrl = <i_param->lti [VDC5_IMGENH_LTI2 ]; 03468 /* Median filter reference pixel select */ 03469 if (lti_param->lti_h4_median_tap_sel == VDC5_LTI_MDFIL_SEL_ADJ2 ) { 03470 *(img_qlty_imp->adj_enh_lti2) = (uint32_t)0x00000000u; 03471 } else { 03472 *(img_qlty_imp->adj_enh_lti2) = (uint32_t)VDC5_REG_BIT24; 03473 } 03474 /* Median filter LTI correction threshold */ 03475 *(img_qlty_imp->adj_enh_lti2) |= (uint32_t)((uint32_t)lti_ctrl->lti_inc_zero << VDC5_REG_SHIFT_16); 03476 /* LTI edge amplitude value gain */ 03477 *(img_qlty_imp->adj_enh_lti2) |= (uint32_t)((uint32_t)lti_ctrl->lti_gain << VDC5_REG_SHIFT_8); 03478 /* LTI coring (maximum core value of 255) */ 03479 *(img_qlty_imp->adj_enh_lti2) |= (uint32_t)lti_ctrl->lti_core ; 03480 } 03481 03482 if (lti_h_on == VDC5_OFF ) { 03483 *(img_qlty_imp->adj_enh_lti1) &= (uint32_t)~VDC5_REG_BIT31; 03484 } else { 03485 *(img_qlty_imp->adj_enh_lti1) |= (uint32_t)VDC5_REG_BIT31; 03486 } 03487 } /* End of function SetImageEnhancementLti() */ 03488 03489 /**************************************************************************//** 03490 * @brief Sets alpha blending area of a rectangle 03491 * @param[in] pd_disp_rect : Rectangular area for alpha blending 03492 * @param[in] img_synthesizer : Image synthesizer registers 03493 * @retval None 03494 ******************************************************************************/ 03495 static void SetAlphaRectArea ( 03496 const vdc5_pd_disp_rect_t * const pd_disp_rect, 03497 const vdc5_regaddr_img_synthesizer_t * const img_synthesizer) 03498 { 03499 uint32_t v_start; 03500 uint32_t h_start; 03501 03502 if (pd_disp_rect != NULL) { 03503 v_start = *(img_synthesizer->gr_ab2) >> VDC5_REG_SHIFT_16; 03504 h_start = *(img_synthesizer->gr_ab3) >> VDC5_REG_SHIFT_16; 03505 03506 v_start += (uint32_t)pd_disp_rect->vs_rel ; 03507 h_start += (uint32_t)pd_disp_rect->hs_rel ; 03508 /* If the start position exceeds the maximum bit width, the value should be saturated. */ 03509 if ((v_start & (uint32_t)~VDC5_REG_MASK_0X000007FF) != 0u) { 03510 v_start = (uint32_t)VDC5_REG_MASK_0X000007FF; 03511 } 03512 if ((h_start & (uint32_t)~VDC5_REG_MASK_0X000007FF) != 0u) { 03513 h_start = (uint32_t)VDC5_REG_MASK_0X000007FF; 03514 } 03515 03516 *(img_synthesizer->gr_ab4) = (v_start << VDC5_REG_SHIFT_16) | (uint32_t)pd_disp_rect->vw_rel ; 03517 *(img_synthesizer->gr_ab5) = (h_start << VDC5_REG_SHIFT_16) | (uint32_t)pd_disp_rect->hw_rel ; 03518 } 03519 } /* End of function SetAlphaRectArea() */ 03520 03521 /**************************************************************************//** 03522 * @brief Sets upper-layer and lower-layer plane in scaler 03523 * @param[in] ch : Channel 03524 * @param[in] und_sel : Selection of lower-layer plane in scaler 03525 * @retval None 03526 *****************************************************************************/ 03527 static void SetUndSel (const vdc5_channel_t ch, const vdc5_onoff_t und_sel) 03528 { 03529 const vdc5_regaddr_img_synthesizer_t * img_synthesizer; 03530 const vdc5_regaddr_img_synthesizer_t * img_synthesizer_vin; 03531 vdc5_onoff_t current_und_sel; 03532 vdc5_onoff_t cascade; 03533 vdc5_resource_state_t rsrc_state; 03534 uint32_t reg_data; 03535 03536 current_und_sel = VDC5_ShrdPrmGetUndSel(ch); 03537 if (und_sel != current_und_sel) { 03538 VDC5_ShrdPrmSetUndSel(ch, und_sel); 03539 03540 cascade = VDC5_ShrdPrmGetCascade(ch); 03541 rsrc_state = VDC5_ShrdPrmGetLayerResource(ch, VDC5_LAYER_ID_0_RD ); 03542 if ((cascade == VDC5_OFF ) && (rsrc_state != VDC5_RESOURCE_ST_INVALID )) { 03543 /* Cascade connection OFF and graphics 0 is used */ 03544 img_synthesizer_vin = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_VIN ]; 03545 if (und_sel == VDC5_OFF ) { 03546 /* Graphics 1 is allocated to the upper-layer. */ 03547 *(img_synthesizer_vin->gr_ab1) &= (uint32_t)~VDC5_REG_BIT2; 03548 03549 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR1 ]; 03550 } else { 03551 /* Graphics 0 is allocated to the upper-layer. */ 03552 *(img_synthesizer_vin->gr_ab1) |= (uint32_t)VDC5_REG_BIT2; 03553 03554 img_synthesizer = &vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR0 ]; 03555 } 03556 /* Copy the graphics display area in the upper-layer to the VIN display area. */ 03557 reg_data = *(img_synthesizer->gr_ab2); 03558 *(img_synthesizer_vin->gr_ab2) = reg_data; 03559 reg_data = *(img_synthesizer->gr_ab3); 03560 *(img_synthesizer_vin->gr_ab3) = reg_data; 03561 03562 /* Graphics register update control register (GR_VIN_UPDATE) 03563 b8 GR_VIN_UPDATE 03564 b4 GR_VIN_P_VEN */ 03565 *(img_synthesizer_vin->gr_update) |= (uint32_t)(VDC5_REG_BIT8|VDC5_REG_BIT4); 03566 } 03567 } 03568 } /* End of function SetUndSel() */ 03569 03570 /**************************************************************************//** 03571 * @brief Converts the color format from gr_format format into 24-bit RGB888 format 03572 * @param[in] gr_format : Color format 03573 * @param[in] input_color : Color data 03574 * @retval RGB888 color data 03575 ******************************************************************************/ 03576 static uint32_t ColorConversion (const vdc5_gr_format_t gr_format, const uint32_t input_color) 03577 { 03578 uint32_t red; 03579 uint32_t green; 03580 uint32_t blue; 03581 uint32_t conv_color; 03582 03583 conv_color = 0; 03584 switch (gr_format) { 03585 case VDC5_GR_FORMAT_RGB565 : 03586 red = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_RGB565_R_MASK) >> VDC5_REG_SHIFT_11)); 03587 green = ColorConversionFrom6to8((uint32_t)((input_color & VDC5_REG_RGB565_G_MASK) >> VDC5_REG_SHIFT_5)); 03588 blue = ColorConversionFrom5to8((uint32_t)(input_color & VDC5_REG_RGB565_B_MASK)); 03589 conv_color = (green << VDC5_REG_SHIFT_16) | (blue << VDC5_REG_SHIFT_8) | red; 03590 break; 03591 case VDC5_GR_FORMAT_RGB888 : 03592 conv_color = ColorConversionIntoAgbr(input_color); 03593 break; 03594 case VDC5_GR_FORMAT_ARGB1555 : 03595 red = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_ARGB1555_R_MASK) >> VDC5_REG_SHIFT_10)); 03596 green = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_ARGB1555_G_MASK) >> VDC5_REG_SHIFT_5)); 03597 blue = ColorConversionFrom5to8((uint32_t)(input_color & VDC5_REG_ARGB1555_B_MASK)); 03598 conv_color = (green << VDC5_REG_SHIFT_16) | (blue << VDC5_REG_SHIFT_8) | red; 03599 break; 03600 case VDC5_GR_FORMAT_ARGB4444 : 03601 red = ColorConversionFrom4to8((uint32_t)((input_color & VDC5_REG_ARGB4444_R_MASK) >> VDC5_REG_SHIFT_8)); 03602 green = ColorConversionFrom4to8((uint32_t)((input_color & VDC5_REG_ARGB4444_G_MASK) >> VDC5_REG_SHIFT_4)); 03603 blue = ColorConversionFrom4to8((uint32_t)(input_color & VDC5_REG_ARGB4444_B_MASK)); 03604 conv_color = (green << VDC5_REG_SHIFT_16) | (blue << VDC5_REG_SHIFT_8) | red; 03605 break; 03606 case VDC5_GR_FORMAT_ARGB8888 : 03607 conv_color = ColorConversionIntoAgbr((uint32_t)(input_color & VDC5_REG_RGB_24BIT_MASK)); 03608 break; 03609 case VDC5_GR_FORMAT_RGBA5551 : 03610 red = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_RGBA5551_R_MASK) >> VDC5_REG_SHIFT_11)); 03611 green = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_RGBA5551_G_MASK) >> VDC5_REG_SHIFT_6)); 03612 blue = ColorConversionFrom5to8((uint32_t)((input_color & VDC5_REG_RGBA5551_B_MASK) >> VDC5_REG_SHIFT_1)); 03613 conv_color = (green << VDC5_REG_SHIFT_16) | (blue << VDC5_REG_SHIFT_8) | red; 03614 break; 03615 case VDC5_GR_FORMAT_RGBA8888 : 03616 conv_color = ColorConversionIntoAgbr((uint32_t)((input_color >> VDC5_REG_SHIFT_8) & VDC5_REG_RGB_24BIT_MASK)); 03617 break; 03618 default: 03619 /* DO NOTHING */ 03620 break; 03621 } 03622 return conv_color; 03623 } /* End of function ColorConversion() */ 03624 03625 /**************************************************************************//** 03626 * @brief Converts the color data from 4 bits value into 8 bits value 03627 * @param[in] color_value : 4-bit color data 03628 * @retval 8-bit color data 03629 ******************************************************************************/ 03630 static uint32_t ColorConversionFrom4to8 (const uint32_t color_value) 03631 { 03632 uint32_t color; 03633 03634 color = color_value & (uint32_t)VDC5_REG_COLOR_4BIT_MASK; 03635 color *= (uint32_t)VDC5_REG_EXTENSION_VALUE_17; 03636 03637 return color; 03638 } /* End of function ColorConversionFrom4to8() */ 03639 03640 /**************************************************************************//** 03641 * @brief Converts the color data from 5 bits value into 8 bits value 03642 * @param[in] color_value : 5-bit color data 03643 * @retval 8-bit color data 03644 ******************************************************************************/ 03645 static uint32_t ColorConversionFrom5to8 (const uint32_t color_value) 03646 { 03647 uint32_t color; 03648 03649 color = color_value & (uint32_t)VDC5_REG_COLOR_5BIT_MASK; 03650 color *= (uint32_t)(VDC5_REG_EXTENSION_VALUE_263 * VDC5_REG_ROUND_OFF_VALUE_2); 03651 color /= (uint32_t)VDC5_REG_EXTENSION_VALUE_32; 03652 /* Round off */ 03653 color += (uint32_t)VDC5_REG_ROUND_OFF_VALUE_1; 03654 color /= (uint32_t)VDC5_REG_ROUND_OFF_VALUE_2; 03655 03656 return color; 03657 } /* End of function ColorConversionFrom5to8() */ 03658 03659 /**************************************************************************//** 03660 * @brief Converts the color data from 6 bits value into 8 bits value 03661 * @param[in] color_value : 6-bit color data 03662 * @retval 8-bit color data 03663 ******************************************************************************/ 03664 static uint32_t ColorConversionFrom6to8 (const uint32_t color_value) 03665 { 03666 uint32_t color; 03667 03668 color = color_value & (uint32_t)VDC5_REG_COLOR_6BIT_MASK; 03669 color *= (uint32_t)(VDC5_REG_EXTENSION_VALUE_259 * VDC5_REG_ROUND_OFF_VALUE_2); 03670 color /= (uint32_t)VDC5_REG_EXTENSION_VALUE_64; 03671 /* Round off */ 03672 color += (uint32_t)VDC5_REG_ROUND_OFF_VALUE_1; 03673 color /= (uint32_t)VDC5_REG_ROUND_OFF_VALUE_2; 03674 03675 return color; 03676 } /* End of function ColorConversionFrom6to8() */ 03677 03678 /**************************************************************************//** 03679 * @brief Converts the color data from ARGB8888 format into AGBR8888 format 03680 * @param[in] color_value : ARGB8888 color data 03681 * @retval AGBR8888 color data 03682 ******************************************************************************/ 03683 static uint32_t ColorConversionIntoAgbr (const uint32_t color_value) 03684 { 03685 uint32_t color_bgr; 03686 uint32_t color_dat; 03687 03688 /* Green and blue */ 03689 color_bgr = (uint32_t)(color_value << VDC5_REG_SHIFT_8); 03690 color_bgr &= (uint32_t)VDC5_REG_ARGB8888_GB_MASK; 03691 /* Alpha */ 03692 color_dat = (uint32_t)(color_value & VDC5_REG_ALPHA_8BIT); 03693 color_bgr |= color_dat; 03694 /* Red */ 03695 color_dat = (uint32_t)((color_value >> VDC5_REG_SHIFT_16) & VDC5_REG_COLOR_8BIT_MASK); 03696 color_bgr |= color_dat; 03697 03698 return color_bgr; 03699 } /* End of function ColorConversionIntoAgbr() */ 03700 03701 /**************************************************************************//** 03702 * @brief Sets color lookup table (CLUT) 03703 * @param[in] param : CLUT parameter 03704 * @param[out] regaddr_clut : CLUT register top address 03705 * @retval None 03706 ******************************************************************************/ 03707 static void Set_Clut (const vdc5_clut_t * const param, volatile uint32_t * regaddr_clut) 03708 { 03709 uint32_t data_len; 03710 const uint32_t * clut_tmp; 03711 03712 clut_tmp = param->clut ; 03713 for (data_len = 0; data_len < param->color_num ; data_len++) { 03714 *regaddr_clut = *clut_tmp; 03715 regaddr_clut++; 03716 clut_tmp++; 03717 } 03718 } /* End of function Set_Clut() */ 03719 03720 /**************************************************************************//** 03721 * @brief Sets start threshold for gamma correction 03722 * @param[in] gam_th : Start threshold of area 1 to 31 03723 * @param[out] gam_area : Gamma correction registers for start threshold 03724 * @retval None 03725 ******************************************************************************/ 03726 static void Set_StartThreshold_Gamma (const uint8_t * gam_th, volatile uint32_t * const * const gam_area) 03727 { 03728 uint32_t reg_index; 03729 03730 if (gam_th != NULL) { 03731 *(gam_area[0]) = (uint32_t)*gam_th << VDC5_REG_SHIFT_16; 03732 gam_th++; 03733 *(gam_area[0]) |= (uint32_t)*gam_th << VDC5_REG_SHIFT_8; 03734 gam_th++; 03735 *(gam_area[0]) |= (uint32_t)*gam_th; 03736 gam_th++; 03737 03738 for (reg_index = 1; reg_index < VDC5_GAM_AREA_REG_NUM; reg_index++) { 03739 *(gam_area[reg_index]) = (uint32_t)*gam_th << VDC5_REG_SHIFT_24; 03740 gam_th++; 03741 *(gam_area[reg_index]) |= (uint32_t)*gam_th << VDC5_REG_SHIFT_16; 03742 gam_th++; 03743 *(gam_area[reg_index]) |= (uint32_t)*gam_th << VDC5_REG_SHIFT_8; 03744 gam_th++; 03745 *(gam_area[reg_index]) |= (uint32_t)*gam_th; 03746 gam_th++; 03747 } 03748 } 03749 } /* End of function Set_StartThreshold_Gamma() */ 03750 03751 /**************************************************************************//** 03752 * @brief Sets gain adjustment for gamma correction 03753 * @param[in] gam_gain : Gain adjustment of area 0 to 31 03754 * @param[out] gam_lut : Gamma correction registers for gain adjustment 03755 * @retval None 03756 ******************************************************************************/ 03757 static void Set_GainAdjustment_Gamma (const uint16_t * gam_gain, volatile uint32_t * const * const gam_lut) 03758 { 03759 uint32_t reg_index; 03760 03761 if (gam_gain != NULL) { 03762 for (reg_index = 0; reg_index < VDC5_GAM_LUT_REG_NUM; reg_index++) { 03763 *(gam_lut[reg_index]) = (uint32_t)*gam_gain << VDC5_REG_SHIFT_16; 03764 gam_gain++; 03765 *(gam_lut[reg_index]) |= (uint32_t)*gam_gain; 03766 gam_gain++; 03767 } 03768 } 03769 } /* End of function Set_GainAdjustment_Gamma() */ 03770 03771 /**************************************************************************//** 03772 * @brief Waits for 200 usec 03773 * @param[in] void 03774 * @retval None 03775 ******************************************************************************/ 03776 static void Wait_200_usec (void) 03777 { 03778 volatile uint32_t counter; 03779 03780 for (counter = 0; counter < (uint32_t)VDC5_LVDS_PLL_WAIT_200USEC; counter++) { 03781 /* Wait for 200 usec. */ 03782 } 03783 } /* End of function Wait_200_usec() */ 03784
Generated on Tue Jul 12 2022 15:08:46 by
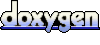