Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdc5_interrupt.c
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdc5_interrupt.c 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDC5 driver interrupt related processing 00029 ******************************************************************************/ 00030 00031 /****************************************************************************** 00032 Includes <System Includes> , "Project Includes" 00033 ******************************************************************************/ 00034 #include "r_vdc5.h" 00035 #include "r_vdc5_user.h" 00036 #include "r_vdc5_register.h" 00037 00038 00039 /****************************************************************************** 00040 Macro definitions 00041 ******************************************************************************/ 00042 #define UNUSED_PARAM(param) (void)(param) 00043 00044 #define VDC5_INT_BIT_S0_VI_VSYNC ((uint32_t)0x00000001u) 00045 #define VDC5_INT_BIT_S0_LO_VSYNC ((uint32_t)0x00000010u) 00046 #define VDC5_INT_BIT_S0_VSYNCERR ((uint32_t)0x00000100u) 00047 #define VDC5_INT_BIT_VLINE ((uint32_t)0x00001000u) 00048 #define VDC5_INT_BIT_S0_VFIELD ((uint32_t)0x00010000u) 00049 #define VDC5_INT_BIT_IV1_VBUFERR ((uint32_t)0x00100000u) 00050 #define VDC5_INT_BIT_IV3_VBUFERR ((uint32_t)0x01000000u) 00051 #define VDC5_INT_BIT_IV5_VBUFERR ((uint32_t)0x10000000u) 00052 #define VDC5_INT_BIT_IV6_VBUFERR ((uint32_t)0x00000001u) 00053 #define VDC5_INT_BIT_S0_WLINE ((uint32_t)0x00000010u) 00054 #define VDC5_INT_BIT_S1_VI_VSYNC ((uint32_t)0x00000100u) 00055 #define VDC5_INT_BIT_S1_LO_VSYNC ((uint32_t)0x00001000u) 00056 #define VDC5_INT_BIT_S1_VSYNCERR ((uint32_t)0x00010000u) 00057 #define VDC5_INT_BIT_S1_VFIELD ((uint32_t)0x00100000u) 00058 #define VDC5_INT_BIT_IV2_VBUFERR ((uint32_t)0x01000000u) 00059 #define VDC5_INT_BIT_IV4_VBUFERR ((uint32_t)0x10000000u) 00060 #define VDC5_INT_BIT_S1_WLINE ((uint32_t)0x00000001u) 00061 #define VDC5_INT_BIT_OIR_VI_VSYNC ((uint32_t)0x00000010u) 00062 #define VDC5_INT_BIT_OIR_LO_VSYNC ((uint32_t)0x00000100u) 00063 #define VDC5_INT_BIT_OIR_VLINE ((uint32_t)0x00001000u) 00064 #define VDC5_INT_BIT_OIR_VFIELD ((uint32_t)0x00010000u) 00065 #define VDC5_INT_BIT_IV7_VBUFERR ((uint32_t)0x00100000u) 00066 #define VDC5_INT_BIT_IV8_VBUFERR ((uint32_t)0x01000000u) 00067 00068 #define VDC5_GR_UPDATE_P_VEN_BIT ((uint32_t)0x00000010u) 00069 #define VDC5_SCL0_UPDATE_VEN_A_BIT ((uint32_t)0x00000001u) 00070 00071 /* Valid bit range */ 00072 #define VDC5_INT_RANGE_0X000007FF (0x000007FFu) 00073 00074 /****************************************************************************** 00075 Typedef definitions 00076 ******************************************************************************/ 00077 00078 /****************************************************************************** 00079 Private global variables and functions 00080 ******************************************************************************/ 00081 static void VDC5_Ch0_s0_vi_vsync_ISR(const uint32_t int_sense); 00082 static void VDC5_Ch0_s0_lo_vsync_ISR(const uint32_t int_sense); 00083 static void VDC5_Ch0_s0_vsyncerr_ISR(const uint32_t int_sense); 00084 static void VDC5_Ch0_vline_ISR(const uint32_t int_sense); 00085 static void VDC5_Ch0_s0_vfield_ISR(const uint32_t int_sense); 00086 static void VDC5_Ch0_iv1_vbuferr_ISR(const uint32_t int_sense); 00087 static void VDC5_Ch0_iv3_vbuferr_ISR(const uint32_t int_sense); 00088 static void VDC5_Ch0_iv5_vbuferr_ISR(const uint32_t int_sense); 00089 static void VDC5_Ch0_iv6_vbuferr_ISR(const uint32_t int_sense); 00090 static void VDC5_Ch0_s0_wline_ISR(const uint32_t int_sense); 00091 static void VDC5_Ch0_s1_vi_vsync_ISR(const uint32_t int_sense); 00092 static void VDC5_Ch0_s1_lo_vsync_ISR(const uint32_t int_sense); 00093 static void VDC5_Ch0_s1_vsyncerr_ISR(const uint32_t int_sense); 00094 static void VDC5_Ch0_s1_vfield_ISR(const uint32_t int_sense); 00095 static void VDC5_Ch0_iv2_vbuferr_ISR(const uint32_t int_sense); 00096 static void VDC5_Ch0_iv4_vbuferr_ISR(const uint32_t int_sense); 00097 static void VDC5_Ch0_s1_wline_ISR(const uint32_t int_sense); 00098 static void VDC5_Ch0_oir_vi_vsync_ISR(const uint32_t int_sense); 00099 static void VDC5_Ch0_oir_lo_vsync_ISR(const uint32_t int_sense); 00100 static void VDC5_Ch0_oir_vline_ISR(const uint32_t int_sense); 00101 static void VDC5_Ch0_oir_vfield_ISR(const uint32_t int_sense); 00102 static void VDC5_Ch0_iv7_vbuferr_ISR(const uint32_t int_sense); 00103 static void VDC5_Ch0_iv8_vbuferr_ISR(const uint32_t int_sense); 00104 00105 static void VDC5_Ch1_s0_vi_vsync_ISR(const uint32_t int_sense); 00106 static void VDC5_Ch1_s0_lo_vsync_ISR(const uint32_t int_sense); 00107 static void VDC5_Ch1_s0_vsyncerr_ISR(const uint32_t int_sense); 00108 static void VDC5_Ch1_vline_ISR(const uint32_t int_sense); 00109 static void VDC5_Ch1_s0_vfield_ISR(const uint32_t int_sense); 00110 static void VDC5_Ch1_iv1_vbuferr_ISR(const uint32_t int_sense); 00111 static void VDC5_Ch1_iv3_vbuferr_ISR(const uint32_t int_sense); 00112 static void VDC5_Ch1_iv5_vbuferr_ISR(const uint32_t int_sense); 00113 static void VDC5_Ch1_iv6_vbuferr_ISR(const uint32_t int_sense); 00114 static void VDC5_Ch1_s0_wline_ISR(const uint32_t int_sense); 00115 static void VDC5_Ch1_s1_vi_vsync_ISR(const uint32_t int_sense); 00116 static void VDC5_Ch1_s1_lo_vsync_ISR(const uint32_t int_sense); 00117 static void VDC5_Ch1_s1_vsyncerr_ISR(const uint32_t int_sense); 00118 static void VDC5_Ch1_s1_vfield_ISR(const uint32_t int_sense); 00119 static void VDC5_Ch1_iv2_vbuferr_ISR(const uint32_t int_sense); 00120 static void VDC5_Ch1_iv4_vbuferr_ISR(const uint32_t int_sense); 00121 static void VDC5_Ch1_s1_wline_ISR(const uint32_t int_sense); 00122 static void VDC5_Ch1_oir_vi_vsync_ISR(const uint32_t int_sense); 00123 static void VDC5_Ch1_oir_lo_vsync_ISR(const uint32_t int_sense); 00124 static void VDC5_Ch1_oir_vline_ISR(const uint32_t int_sense); 00125 static void VDC5_Ch1_oir_vfield_ISR(const uint32_t int_sense); 00126 static void VDC5_Ch1_iv7_vbuferr_ISR(const uint32_t int_sense); 00127 static void VDC5_Ch1_iv8_vbuferr_ISR(const uint32_t int_sense); 00128 00129 /*! List of the callback function pointers */ 00130 static void (*vdc5_int_callback [VDC5_CHANNEL_NUM ][VDC5_INT_TYPE_NUM ])(vdc5_int_type_t int_type); 00131 00132 00133 /**************************************************************************//** 00134 * @brief Interrupt service routine acquisition processing 00135 * 00136 * Description:<br> 00137 * This function returns the function pointer to the specified interrupt service routine. 00138 * @param[in] ch : Channel 00139 * @param[in] type : VDC5 interrupt type 00140 * @retval VDC5 Interrupt service routine 00141 *****************************************************************************/ 00142 void (*R_VDC5_GetISR (const vdc5_channel_t ch, const vdc5_int_type_t type))(const uint32_t int_sense) 00143 { 00144 static void (* const isr_table[VDC5_CHANNEL_NUM ][VDC5_INT_TYPE_NUM ])(const uint32_t int_sense) = { 00145 { 00146 &VDC5_Ch0_s0_vi_vsync_ISR, 00147 &VDC5_Ch0_s0_lo_vsync_ISR, 00148 &VDC5_Ch0_s0_vsyncerr_ISR, 00149 &VDC5_Ch0_vline_ISR, 00150 &VDC5_Ch0_s0_vfield_ISR, 00151 &VDC5_Ch0_iv1_vbuferr_ISR, 00152 &VDC5_Ch0_iv3_vbuferr_ISR, 00153 &VDC5_Ch0_iv5_vbuferr_ISR, 00154 &VDC5_Ch0_iv6_vbuferr_ISR, 00155 &VDC5_Ch0_s0_wline_ISR, 00156 &VDC5_Ch0_s1_vi_vsync_ISR, 00157 &VDC5_Ch0_s1_lo_vsync_ISR, 00158 &VDC5_Ch0_s1_vsyncerr_ISR, 00159 &VDC5_Ch0_s1_vfield_ISR, 00160 &VDC5_Ch0_iv2_vbuferr_ISR, 00161 &VDC5_Ch0_iv4_vbuferr_ISR, 00162 &VDC5_Ch0_s1_wline_ISR, 00163 &VDC5_Ch0_oir_vi_vsync_ISR, 00164 &VDC5_Ch0_oir_lo_vsync_ISR, 00165 &VDC5_Ch0_oir_vline_ISR, 00166 &VDC5_Ch0_oir_vfield_ISR, 00167 &VDC5_Ch0_iv7_vbuferr_ISR, 00168 &VDC5_Ch0_iv8_vbuferr_ISR 00169 }, 00170 { 00171 &VDC5_Ch1_s0_vi_vsync_ISR, 00172 &VDC5_Ch1_s0_lo_vsync_ISR, 00173 &VDC5_Ch1_s0_vsyncerr_ISR, 00174 &VDC5_Ch1_vline_ISR, 00175 &VDC5_Ch1_s0_vfield_ISR, 00176 &VDC5_Ch1_iv1_vbuferr_ISR, 00177 &VDC5_Ch1_iv3_vbuferr_ISR, 00178 &VDC5_Ch1_iv5_vbuferr_ISR, 00179 &VDC5_Ch1_iv6_vbuferr_ISR, 00180 &VDC5_Ch1_s0_wline_ISR, 00181 &VDC5_Ch1_s1_vi_vsync_ISR, 00182 &VDC5_Ch1_s1_lo_vsync_ISR, 00183 &VDC5_Ch1_s1_vsyncerr_ISR, 00184 &VDC5_Ch1_s1_vfield_ISR, 00185 &VDC5_Ch1_iv2_vbuferr_ISR, 00186 &VDC5_Ch1_iv4_vbuferr_ISR, 00187 &VDC5_Ch1_s1_wline_ISR, 00188 &VDC5_Ch1_oir_vi_vsync_ISR, 00189 &VDC5_Ch1_oir_lo_vsync_ISR, 00190 &VDC5_Ch1_oir_vline_ISR, 00191 &VDC5_Ch1_oir_vfield_ISR, 00192 &VDC5_Ch1_iv7_vbuferr_ISR, 00193 &VDC5_Ch1_iv8_vbuferr_ISR 00194 } 00195 }; 00196 void (* isr_function)(const uint32_t int_sense); 00197 00198 isr_function = isr_table[ch][type]; 00199 00200 #ifdef R_VDC5_CHECK_PARAMETERS 00201 /* Channel and interrupt type */ 00202 if ((ch >= VDC5_CHANNEL_NUM ) || (type >= VDC5_INT_TYPE_NUM )) { 00203 isr_function = 0; 00204 } 00205 #endif /* R_VDC5_CHECK_PARAMETERS */ 00206 00207 return isr_function; 00208 } /* End of function R_VDC5_GetISR() */ 00209 00210 /**************************************************************************//** 00211 * @brief Disables all VDC5 interrupts 00212 * @param[in] ch : Channel 00213 * @retval None 00214 *****************************************************************************/ 00215 void VDC5_Int_Disable (const vdc5_channel_t ch) 00216 { 00217 const vdc5_regaddr_system_ctrl_t * system_ctrl; 00218 int32_t int_type; 00219 00220 system_ctrl = &vdc5_regaddr_system_ctrl[ch]; 00221 00222 for (int_type = 0; int_type < VDC5_INT_TYPE_NUM ; int_type++) { 00223 vdc5_int_callback [ch][int_type] = 0; 00224 } 00225 /* Interrupt output off */ 00226 *(system_ctrl->syscnt_int4) = 0u; 00227 *(system_ctrl->syscnt_int5) = 0u; 00228 *(system_ctrl->syscnt_int6) = 0u; 00229 00230 } /* End of function VDC5_Int_Disable() */ 00231 00232 /**************************************************************************//** 00233 * @brief Enables/disables the specified VDC5 interrupt 00234 * @param[in] ch : Channel 00235 * @param[in] param : Interrupt callback setup parameter 00236 * @retval None 00237 *****************************************************************************/ 00238 void VDC5_Int_SetInterrupt (const vdc5_channel_t ch, const vdc5_int_t * const param) 00239 { 00240 volatile uint32_t * int_clhd_reg; 00241 volatile uint32_t * int_onoff_reg; 00242 volatile uint32_t * linenum_reg; 00243 volatile uint16_t * linenum16_reg; 00244 volatile uint32_t * linenum_update_reg; 00245 uint32_t mask_bit; 00246 uint32_t reg_data; 00247 static const uint32_t interrupt_bit_table[VDC5_INT_TYPE_NUM ] = { 00248 VDC5_INT_BIT_S0_VI_VSYNC, 00249 VDC5_INT_BIT_S0_LO_VSYNC, 00250 VDC5_INT_BIT_S0_VSYNCERR, 00251 VDC5_INT_BIT_VLINE, 00252 VDC5_INT_BIT_S0_VFIELD, 00253 VDC5_INT_BIT_IV1_VBUFERR, 00254 VDC5_INT_BIT_IV3_VBUFERR, 00255 VDC5_INT_BIT_IV5_VBUFERR, 00256 VDC5_INT_BIT_IV6_VBUFERR, 00257 VDC5_INT_BIT_S0_WLINE, 00258 VDC5_INT_BIT_S1_VI_VSYNC, 00259 VDC5_INT_BIT_S1_LO_VSYNC, 00260 VDC5_INT_BIT_S1_VSYNCERR, 00261 VDC5_INT_BIT_S1_VFIELD, 00262 VDC5_INT_BIT_IV2_VBUFERR, 00263 VDC5_INT_BIT_IV4_VBUFERR, 00264 VDC5_INT_BIT_S1_WLINE, 00265 VDC5_INT_BIT_OIR_VI_VSYNC, 00266 VDC5_INT_BIT_OIR_LO_VSYNC, 00267 VDC5_INT_BIT_OIR_VLINE, 00268 VDC5_INT_BIT_OIR_VFIELD, 00269 VDC5_INT_BIT_IV7_VBUFERR, 00270 VDC5_INT_BIT_IV8_VBUFERR 00271 }; 00272 00273 if (param->type < VDC5_INT_TYPE_IV6_VBUFERR ) { 00274 /* INT0: VDC5_INT_TYPE_S0_VI_VSYNC ~ INT7: VDC5_INT_TYPE_IV5_VBUFERR */ 00275 int_clhd_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int1; 00276 int_onoff_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int4; 00277 } else if (param->type < VDC5_INT_TYPE_S1_WLINE ) { 00278 /* INT8: VDC5_INT_TYPE_IV6_VBUFERR ~ INT15: VDC5_INT_TYPE_IV4_VBUFERR */ 00279 int_clhd_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int2; 00280 int_onoff_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int5; 00281 } else { 00282 /* INT16: VDC5_INT_TYPE_S1_WLINE ~ INT22: VDC5_INT_TYPE_IV8_VBUFERR */ 00283 int_clhd_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int3; 00284 int_onoff_reg = vdc5_regaddr_system_ctrl[ch].syscnt_int6; 00285 } 00286 mask_bit = interrupt_bit_table[param->type ]; 00287 00288 /* Interrupt callback function pointer */ 00289 vdc5_int_callback [ch][param->type ] = param->callback ; 00290 00291 if (param->callback != 0) { 00292 /* ON */ 00293 if ((*int_onoff_reg & mask_bit) == 0u) { 00294 /* OFF to ON */ 00295 *int_onoff_reg |= mask_bit; 00296 reg_data = *int_onoff_reg; 00297 *int_clhd_reg = reg_data; 00298 } 00299 00300 if (param->type == VDC5_INT_TYPE_VLINE ) { 00301 /* Specified line signal for panel output in graphics 3 */ 00302 linenum_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR3 ].gr_clut; 00303 linenum_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_GR3 ].gr_update; 00304 *linenum_reg &= (uint32_t)~VDC5_INT_RANGE_0X000007FF; 00305 *linenum_reg |= (uint32_t)param->line_num ; 00306 *linenum_update_reg |= VDC5_GR_UPDATE_P_VEN_BIT; 00307 } else if (param->type == VDC5_INT_TYPE_S0_WLINE ) { 00308 /* Write specification line signal input to scaling-down control block in scaler 0 */ 00309 linenum16_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl0_int; 00310 linenum_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC0 ].scl0_update; 00311 *linenum16_reg = param->line_num ; 00312 *linenum_update_reg |= VDC5_SCL0_UPDATE_VEN_A_BIT; 00313 } else if (param->type == VDC5_INT_TYPE_S1_WLINE ) { 00314 /* Write specification line signal input to scaling-down control block in scaler 1 */ 00315 linenum16_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl0_int; 00316 linenum_update_reg = vdc5_regaddr_scaler[ch][VDC5_SC_TYPE_SC1 ].scl0_update; 00317 *linenum16_reg = param->line_num ; 00318 *linenum_update_reg |= VDC5_SCL0_UPDATE_VEN_A_BIT; 00319 } else if (param->type == VDC5_INT_TYPE_OIR_VLINE ) { 00320 /* Specified line signal for panel output in output image generator */ 00321 linenum_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_OIR ].gr_clut; 00322 linenum_update_reg = vdc5_regaddr_img_synthesizer[ch][VDC5_GR_TYPE_OIR ].gr_update; 00323 *linenum_reg &= (uint32_t)~VDC5_INT_RANGE_0X000007FF; 00324 *linenum_reg |= (uint32_t)param->line_num ; 00325 *linenum_update_reg |= VDC5_GR_UPDATE_P_VEN_BIT; 00326 } else { 00327 /* Do nothing */ 00328 } 00329 } else { 00330 /* OFF */ 00331 *int_onoff_reg &= (uint32_t)~mask_bit; 00332 reg_data = *int_onoff_reg; 00333 *int_clhd_reg = reg_data; 00334 } 00335 00336 } /* End of function VDC5_Int_Disable() */ 00337 00338 /**************************************************************************//** 00339 * @brief VDC5 S0_VI_VSYNC interrupt service routine 00340 * @param[in] int_sense 00341 * @retval None 00342 *****************************************************************************/ 00343 static void VDC5_Ch0_s0_vi_vsync_ISR (const uint32_t int_sense) 00344 { 00345 uint32_t IntState; 00346 volatile uint32_t * int_clhd_reg; 00347 volatile uint32_t * int_onoff_reg; 00348 00349 UNUSED_PARAM(int_sense); 00350 00351 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00352 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00353 IntState = *int_onoff_reg; 00354 00355 if (((IntState & VDC5_INT_BIT_S0_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VI_VSYNC) != 0u)) { 00356 /* Clear */ 00357 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VI_VSYNC; 00358 00359 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VI_VSYNC ] != 0) { 00360 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VI_VSYNC ](VDC5_INT_TYPE_S0_VI_VSYNC ); 00361 } 00362 /* Set */ 00363 *int_clhd_reg = IntState; 00364 } 00365 } /* End of function VDC5_Ch0_s0_vi_vsync_ISR() */ 00366 00367 /**************************************************************************//** 00368 * @brief VDC5 S0_LO_VSYNC interrupt service routine 00369 * @param[in] int_sense 00370 * @retval None 00371 *****************************************************************************/ 00372 static void VDC5_Ch0_s0_lo_vsync_ISR (const uint32_t int_sense) 00373 { 00374 uint32_t IntState; 00375 volatile uint32_t * int_clhd_reg; 00376 volatile uint32_t * int_onoff_reg; 00377 00378 UNUSED_PARAM(int_sense); 00379 00380 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00381 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00382 IntState = *int_onoff_reg; 00383 00384 if (((IntState & VDC5_INT_BIT_S0_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_LO_VSYNC) != 0u)) { 00385 /* Clear */ 00386 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_LO_VSYNC; 00387 00388 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_LO_VSYNC ] != 0) { 00389 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_LO_VSYNC ](VDC5_INT_TYPE_S0_LO_VSYNC ); 00390 } 00391 /* Set */ 00392 *int_clhd_reg = IntState; 00393 } 00394 } /* End of function VDC5_Ch0_s0_lo_vsync_ISR() */ 00395 00396 /**************************************************************************//** 00397 * @brief VDC5 S0_VSYNCERR interrupt service routine 00398 * @param[in] int_sense 00399 * @retval None 00400 *****************************************************************************/ 00401 static void VDC5_Ch0_s0_vsyncerr_ISR (const uint32_t int_sense) 00402 { 00403 uint32_t IntState; 00404 volatile uint32_t * int_clhd_reg; 00405 volatile uint32_t * int_onoff_reg; 00406 00407 UNUSED_PARAM(int_sense); 00408 00409 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00410 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00411 IntState = *int_onoff_reg; 00412 00413 if (((IntState & VDC5_INT_BIT_S0_VSYNCERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VSYNCERR) != 0u)) { 00414 /* Clear */ 00415 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VSYNCERR; 00416 00417 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VSYNCERR ] != 0) { 00418 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VSYNCERR ](VDC5_INT_TYPE_S0_VSYNCERR ); 00419 } 00420 /* Set */ 00421 *int_clhd_reg = IntState; 00422 } 00423 } /* End of function VDC5_Ch0_s0_vsyncerr_ISR() */ 00424 00425 /**************************************************************************//** 00426 * @brief VDC5 VLINE interrupt service routine 00427 * @param[in] int_sense 00428 * @retval None 00429 *****************************************************************************/ 00430 static void VDC5_Ch0_vline_ISR (const uint32_t int_sense) 00431 { 00432 uint32_t IntState; 00433 volatile uint32_t * int_clhd_reg; 00434 volatile uint32_t * int_onoff_reg; 00435 00436 UNUSED_PARAM(int_sense); 00437 00438 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00439 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00440 IntState = *int_onoff_reg; 00441 00442 if (((IntState & VDC5_INT_BIT_VLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_VLINE) != 0u)) { 00443 /* Clear */ 00444 *int_clhd_reg = IntState & ~VDC5_INT_BIT_VLINE; 00445 00446 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_VLINE ] != 0) { 00447 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_VLINE ](VDC5_INT_TYPE_VLINE ); 00448 } 00449 /* Set */ 00450 *int_clhd_reg = IntState; 00451 } 00452 } /* End of function VDC5_Ch0_vline_ISR() */ 00453 00454 /**************************************************************************//** 00455 * @brief VDC5 S0_VFIELD interrupt service routine 00456 * @param[in] int_sense 00457 * @retval None 00458 *****************************************************************************/ 00459 static void VDC5_Ch0_s0_vfield_ISR (const uint32_t int_sense) 00460 { 00461 uint32_t IntState; 00462 volatile uint32_t * int_clhd_reg; 00463 volatile uint32_t * int_onoff_reg; 00464 00465 UNUSED_PARAM(int_sense); 00466 00467 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00468 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00469 IntState = *int_onoff_reg; 00470 00471 if (((IntState & VDC5_INT_BIT_S0_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VFIELD) != 0u)) { 00472 /* Clear */ 00473 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VFIELD; 00474 00475 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VFIELD ] != 0) { 00476 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_VFIELD ](VDC5_INT_TYPE_S0_VFIELD ); 00477 } 00478 /* Set */ 00479 *int_clhd_reg = IntState; 00480 } 00481 } /* End of function VDC5_Ch0_s0_vfield_ISR() */ 00482 00483 /**************************************************************************//** 00484 * @brief VDC5 IV1_VBUFERR interrupt service routine 00485 * @param[in] int_sense 00486 * @retval None 00487 *****************************************************************************/ 00488 static void VDC5_Ch0_iv1_vbuferr_ISR (const uint32_t int_sense) 00489 { 00490 uint32_t IntState; 00491 volatile uint32_t * int_clhd_reg; 00492 volatile uint32_t * int_onoff_reg; 00493 00494 UNUSED_PARAM(int_sense); 00495 00496 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00497 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00498 IntState = *int_onoff_reg; 00499 00500 if (((IntState & VDC5_INT_BIT_IV1_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV1_VBUFERR) != 0u)) { 00501 /* Clear */ 00502 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV1_VBUFERR; 00503 00504 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV1_VBUFERR ] != 0) { 00505 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV1_VBUFERR ](VDC5_INT_TYPE_IV1_VBUFERR ); 00506 } 00507 /* Set */ 00508 *int_clhd_reg = IntState; 00509 } 00510 } /* End of function VDC5_Ch0_iv1_vbuferr_ISR() */ 00511 00512 /**************************************************************************//** 00513 * @brief VDC5 IV3_VBUFERR interrupt service routine 00514 * @param[in] int_sense 00515 * @retval None 00516 *****************************************************************************/ 00517 static void VDC5_Ch0_iv3_vbuferr_ISR (const uint32_t int_sense) 00518 { 00519 uint32_t IntState; 00520 volatile uint32_t * int_clhd_reg; 00521 volatile uint32_t * int_onoff_reg; 00522 00523 UNUSED_PARAM(int_sense); 00524 00525 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00526 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00527 IntState = *int_onoff_reg; 00528 00529 if (((IntState & VDC5_INT_BIT_IV3_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV3_VBUFERR) != 0u)) { 00530 /* Clear */ 00531 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV3_VBUFERR; 00532 00533 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV3_VBUFERR ] != 0) { 00534 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV3_VBUFERR ](VDC5_INT_TYPE_IV3_VBUFERR ); 00535 } 00536 /* Set */ 00537 *int_clhd_reg = IntState; 00538 } 00539 } /* End of function VDC5_Ch0_iv3_vbuferr_ISR() */ 00540 00541 /**************************************************************************//** 00542 * @brief VDC5 IV5_VBUFERR interrupt service routine 00543 * @param[in] int_sense 00544 * @retval None 00545 *****************************************************************************/ 00546 static void VDC5_Ch0_iv5_vbuferr_ISR (const uint32_t int_sense) 00547 { 00548 uint32_t IntState; 00549 volatile uint32_t * int_clhd_reg; 00550 volatile uint32_t * int_onoff_reg; 00551 00552 UNUSED_PARAM(int_sense); 00553 00554 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int1; 00555 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int4; 00556 IntState = *int_onoff_reg; 00557 00558 if (((IntState & VDC5_INT_BIT_IV5_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV5_VBUFERR) != 0u)) { 00559 /* Clear */ 00560 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV5_VBUFERR; 00561 00562 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV5_VBUFERR ] != 0) { 00563 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV5_VBUFERR ](VDC5_INT_TYPE_IV5_VBUFERR ); 00564 } 00565 /* Set */ 00566 *int_clhd_reg = IntState; 00567 } 00568 } /* End of function VDC5_Ch0_iv5_vbuferr_ISR() */ 00569 00570 /**************************************************************************//** 00571 * @brief VDC5 IV6_VBUFERR interrupt service routine 00572 * @param[in] int_sense 00573 * @retval None 00574 *****************************************************************************/ 00575 static void VDC5_Ch0_iv6_vbuferr_ISR (const uint32_t int_sense) 00576 { 00577 uint32_t IntState; 00578 volatile uint32_t * int_clhd_reg; 00579 volatile uint32_t * int_onoff_reg; 00580 00581 UNUSED_PARAM(int_sense); 00582 00583 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00584 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00585 IntState = *int_onoff_reg; 00586 00587 if (((IntState & VDC5_INT_BIT_IV6_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV6_VBUFERR) != 0u)) { 00588 /* Clear */ 00589 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV6_VBUFERR; 00590 00591 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV6_VBUFERR ] != 0) { 00592 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV6_VBUFERR ](VDC5_INT_TYPE_IV6_VBUFERR ); 00593 } 00594 /* Set */ 00595 *int_clhd_reg = IntState; 00596 } 00597 } /* End of function VDC5_Ch0_iv6_vbuferr_ISR() */ 00598 00599 /**************************************************************************//** 00600 * @brief VDC5 S0_WLINE interrupt service routine 00601 * @param[in] int_sense 00602 * @retval None 00603 *****************************************************************************/ 00604 static void VDC5_Ch0_s0_wline_ISR (const uint32_t int_sense) 00605 { 00606 uint32_t IntState; 00607 volatile uint32_t * int_clhd_reg; 00608 volatile uint32_t * int_onoff_reg; 00609 00610 UNUSED_PARAM(int_sense); 00611 00612 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00613 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00614 IntState = *int_onoff_reg; 00615 00616 if (((IntState & VDC5_INT_BIT_S0_WLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_WLINE) != 0u)) { 00617 /* Clear */ 00618 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_WLINE; 00619 00620 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_WLINE ] != 0) { 00621 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S0_WLINE ](VDC5_INT_TYPE_S0_WLINE ); 00622 } 00623 /* Set */ 00624 *int_clhd_reg = IntState; 00625 } 00626 } /* End of function VDC5_Ch0_s0_wline_ISR() */ 00627 00628 /**************************************************************************//** 00629 * @brief VDC5 S1_VI_VSYNC interrupt service routine 00630 * @param[in] int_sense 00631 * @retval None 00632 *****************************************************************************/ 00633 static void VDC5_Ch0_s1_vi_vsync_ISR (const uint32_t int_sense) 00634 { 00635 uint32_t IntState; 00636 volatile uint32_t * int_clhd_reg; 00637 volatile uint32_t * int_onoff_reg; 00638 00639 UNUSED_PARAM(int_sense); 00640 00641 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00642 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00643 IntState = *int_onoff_reg; 00644 00645 if (((IntState & VDC5_INT_BIT_S1_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VI_VSYNC) != 0u)) { 00646 /* Clear */ 00647 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VI_VSYNC; 00648 00649 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VI_VSYNC ] != 0) { 00650 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VI_VSYNC ](VDC5_INT_TYPE_S1_VI_VSYNC ); 00651 } 00652 /* Set */ 00653 *int_clhd_reg = IntState; 00654 } 00655 } /* End of function VDC5_Ch0_s1_vi_vsync_ISR() */ 00656 00657 /**************************************************************************//** 00658 * @brief VDC5 S1_LO_VSYNC interrupt service routine 00659 * @param[in] int_sense 00660 * @retval None 00661 *****************************************************************************/ 00662 static void VDC5_Ch0_s1_lo_vsync_ISR (const uint32_t int_sense) 00663 { 00664 uint32_t IntState; 00665 volatile uint32_t * int_clhd_reg; 00666 volatile uint32_t * int_onoff_reg; 00667 00668 UNUSED_PARAM(int_sense); 00669 00670 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00671 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00672 IntState = *int_onoff_reg; 00673 00674 if (((IntState & VDC5_INT_BIT_S1_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_LO_VSYNC) != 0u)) { 00675 /* Clear */ 00676 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_LO_VSYNC; 00677 00678 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_LO_VSYNC ] != 0) { 00679 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_LO_VSYNC ](VDC5_INT_TYPE_S1_LO_VSYNC ); 00680 } 00681 /* Set */ 00682 *int_clhd_reg = IntState; 00683 } 00684 } /* End of function VDC5_Ch0_s1_lo_vsync_ISR() */ 00685 00686 /**************************************************************************//** 00687 * @brief VDC5 S1_VSYNCERR interrupt service routine 00688 * @param[in] int_sense 00689 * @retval None 00690 *****************************************************************************/ 00691 static void VDC5_Ch0_s1_vsyncerr_ISR (const uint32_t int_sense) 00692 { 00693 uint32_t IntState; 00694 volatile uint32_t * int_clhd_reg; 00695 volatile uint32_t * int_onoff_reg; 00696 00697 UNUSED_PARAM(int_sense); 00698 00699 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00700 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00701 IntState = *int_onoff_reg; 00702 00703 if (((IntState & VDC5_INT_BIT_S1_VSYNCERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VSYNCERR) != 0u)) { 00704 /* Clear */ 00705 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VSYNCERR; 00706 00707 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VSYNCERR ] != 0) { 00708 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VSYNCERR ](VDC5_INT_TYPE_S1_VSYNCERR ); 00709 } 00710 /* Set */ 00711 *int_clhd_reg = IntState; 00712 } 00713 } /* End of function VDC5_Ch0_s1_vsyncerr_ISR() */ 00714 00715 /**************************************************************************//** 00716 * @brief VDC5 S1_VFIELD interrupt service routine 00717 * @param[in] int_sense 00718 * @retval None 00719 *****************************************************************************/ 00720 static void VDC5_Ch0_s1_vfield_ISR (const uint32_t int_sense) 00721 { 00722 uint32_t IntState; 00723 volatile uint32_t * int_clhd_reg; 00724 volatile uint32_t * int_onoff_reg; 00725 00726 UNUSED_PARAM(int_sense); 00727 00728 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00729 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00730 IntState = *int_onoff_reg; 00731 00732 if (((IntState & VDC5_INT_BIT_S1_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VFIELD) != 0u)) { 00733 /* Clear */ 00734 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VFIELD; 00735 00736 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VFIELD ] != 0) { 00737 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_VFIELD ](VDC5_INT_TYPE_S1_VFIELD ); 00738 } 00739 /* Set */ 00740 *int_clhd_reg = IntState; 00741 } 00742 } /* End of function VDC5_Ch0_s1_vfield_ISR() */ 00743 00744 /**************************************************************************//** 00745 * @brief VDC5 IV2_VBUFERR interrupt service routine 00746 * @param[in] int_sense 00747 * @retval None 00748 *****************************************************************************/ 00749 static void VDC5_Ch0_iv2_vbuferr_ISR (const uint32_t int_sense) 00750 { 00751 uint32_t IntState; 00752 volatile uint32_t * int_clhd_reg; 00753 volatile uint32_t * int_onoff_reg; 00754 00755 UNUSED_PARAM(int_sense); 00756 00757 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00758 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00759 IntState = *int_onoff_reg; 00760 00761 if (((IntState & VDC5_INT_BIT_IV2_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV2_VBUFERR) != 0u)) { 00762 /* Clear */ 00763 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV2_VBUFERR; 00764 00765 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV2_VBUFERR ] != 0) { 00766 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV2_VBUFERR ](VDC5_INT_TYPE_IV2_VBUFERR ); 00767 } 00768 /* Set */ 00769 *int_clhd_reg = IntState; 00770 } 00771 } /* End of function VDC5_Ch0_iv2_vbuferr_ISR() */ 00772 00773 /**************************************************************************//** 00774 * @brief VDC5 IV4_VBUFERR interrupt service routine 00775 * @param[in] int_sense 00776 * @retval None 00777 *****************************************************************************/ 00778 static void VDC5_Ch0_iv4_vbuferr_ISR (const uint32_t int_sense) 00779 { 00780 uint32_t IntState; 00781 volatile uint32_t * int_clhd_reg; 00782 volatile uint32_t * int_onoff_reg; 00783 00784 UNUSED_PARAM(int_sense); 00785 00786 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int2; 00787 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int5; 00788 IntState = *int_onoff_reg; 00789 00790 if (((IntState & VDC5_INT_BIT_IV4_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV4_VBUFERR) != 0u)) { 00791 /* Clear */ 00792 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV4_VBUFERR; 00793 00794 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV4_VBUFERR ] != 0) { 00795 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV4_VBUFERR ](VDC5_INT_TYPE_IV4_VBUFERR ); 00796 } 00797 /* Set */ 00798 *int_clhd_reg = IntState; 00799 } 00800 } /* End of function VDC5_Ch0_iv4_vbuferr_ISR() */ 00801 00802 /**************************************************************************//** 00803 * @brief VDC5 S1_WLINE interrupt service routine 00804 * @param[in] int_sense 00805 * @retval None 00806 *****************************************************************************/ 00807 static void VDC5_Ch0_s1_wline_ISR (const uint32_t int_sense) 00808 { 00809 uint32_t IntState; 00810 volatile uint32_t * int_clhd_reg; 00811 volatile uint32_t * int_onoff_reg; 00812 00813 UNUSED_PARAM(int_sense); 00814 00815 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00816 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00817 IntState = *int_onoff_reg; 00818 00819 if (((IntState & VDC5_INT_BIT_S1_WLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_WLINE) != 0u)) { 00820 /* Clear */ 00821 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_WLINE; 00822 00823 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_WLINE ] != 0) { 00824 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_S1_WLINE ](VDC5_INT_TYPE_S1_WLINE ); 00825 } 00826 /* Set */ 00827 *int_clhd_reg = IntState; 00828 } 00829 } /* End of function VDC5_Ch0_s1_wline_ISR() */ 00830 00831 /**************************************************************************//** 00832 * @brief VDC5 OIR_VI_VSYNC interrupt service routine 00833 * @param[in] int_sense 00834 * @retval None 00835 *****************************************************************************/ 00836 static void VDC5_Ch0_oir_vi_vsync_ISR (const uint32_t int_sense) 00837 { 00838 uint32_t IntState; 00839 volatile uint32_t * int_clhd_reg; 00840 volatile uint32_t * int_onoff_reg; 00841 00842 UNUSED_PARAM(int_sense); 00843 00844 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00845 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00846 IntState = *int_onoff_reg; 00847 00848 if (((IntState & VDC5_INT_BIT_OIR_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VI_VSYNC) != 0u)) { 00849 /* Clear */ 00850 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VI_VSYNC; 00851 00852 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VI_VSYNC ] != 0) { 00853 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VI_VSYNC ](VDC5_INT_TYPE_OIR_VI_VSYNC ); 00854 } 00855 /* Set */ 00856 *int_clhd_reg = IntState; 00857 } 00858 } /* End of function VDC5_Ch0_oir_vi_vsync_ISR() */ 00859 00860 /**************************************************************************//** 00861 * @brief VDC5 OIR_LO_VSYNC interrupt service routine 00862 * @param[in] int_sense 00863 * @retval None 00864 *****************************************************************************/ 00865 static void VDC5_Ch0_oir_lo_vsync_ISR (const uint32_t int_sense) 00866 { 00867 uint32_t IntState; 00868 volatile uint32_t * int_clhd_reg; 00869 volatile uint32_t * int_onoff_reg; 00870 00871 UNUSED_PARAM(int_sense); 00872 00873 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00874 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00875 IntState = *int_onoff_reg; 00876 00877 if (((IntState & VDC5_INT_BIT_OIR_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_LO_VSYNC) != 0u)) { 00878 /* Clear */ 00879 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_LO_VSYNC; 00880 00881 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_LO_VSYNC ] != 0) { 00882 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_LO_VSYNC ](VDC5_INT_TYPE_OIR_LO_VSYNC ); 00883 } 00884 /* Set */ 00885 *int_clhd_reg = IntState; 00886 } 00887 } /* End of function VDC5_Ch0_oir_lo_vsync_ISR() */ 00888 00889 /**************************************************************************//** 00890 * @brief VDC5 OIR_VLINE interrupt service routine 00891 * @param[in] int_sense 00892 * @retval None 00893 *****************************************************************************/ 00894 static void VDC5_Ch0_oir_vline_ISR (const uint32_t int_sense) 00895 { 00896 uint32_t IntState; 00897 volatile uint32_t * int_clhd_reg; 00898 volatile uint32_t * int_onoff_reg; 00899 00900 UNUSED_PARAM(int_sense); 00901 00902 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00903 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00904 IntState = *int_onoff_reg; 00905 00906 if (((IntState & VDC5_INT_BIT_OIR_VLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VLINE) != 0u)) { 00907 /* Clear */ 00908 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VLINE; 00909 00910 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VLINE ] != 0) { 00911 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VLINE ](VDC5_INT_TYPE_OIR_VLINE ); 00912 } 00913 /* Set */ 00914 *int_clhd_reg = IntState; 00915 } 00916 } /* End of function VDC5_Ch0_oir_vline_ISR() */ 00917 00918 /**************************************************************************//** 00919 * @brief VDC5 OIR_VFIELD interrupt service routine 00920 * @param[in] int_sense 00921 * @retval None 00922 *****************************************************************************/ 00923 static void VDC5_Ch0_oir_vfield_ISR (const uint32_t int_sense) 00924 { 00925 uint32_t IntState; 00926 volatile uint32_t * int_clhd_reg; 00927 volatile uint32_t * int_onoff_reg; 00928 00929 UNUSED_PARAM(int_sense); 00930 00931 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00932 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00933 IntState = *int_onoff_reg; 00934 00935 if (((IntState & VDC5_INT_BIT_OIR_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VFIELD) != 0u)) { 00936 /* Clear */ 00937 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VFIELD; 00938 00939 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VFIELD ] != 0) { 00940 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_OIR_VFIELD ](VDC5_INT_TYPE_OIR_VFIELD ); 00941 } 00942 /* Set */ 00943 *int_clhd_reg = IntState; 00944 } 00945 } /* End of function VDC5_Ch0_oir_vfield_ISR() */ 00946 00947 /**************************************************************************//** 00948 * @brief VDC5 IV7_VBUFERR interrupt service routine 00949 * @param[in] int_sense 00950 * @retval None 00951 *****************************************************************************/ 00952 static void VDC5_Ch0_iv7_vbuferr_ISR (const uint32_t int_sense) 00953 { 00954 uint32_t IntState; 00955 volatile uint32_t * int_clhd_reg; 00956 volatile uint32_t * int_onoff_reg; 00957 00958 UNUSED_PARAM(int_sense); 00959 00960 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00961 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00962 IntState = *int_onoff_reg; 00963 00964 if (((IntState & VDC5_INT_BIT_IV7_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV7_VBUFERR) != 0u)) { 00965 /* Clear */ 00966 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV7_VBUFERR; 00967 00968 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV7_VBUFERR ] != 0) { 00969 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV7_VBUFERR ](VDC5_INT_TYPE_IV7_VBUFERR ); 00970 } 00971 /* Set */ 00972 *int_clhd_reg = IntState; 00973 } 00974 } /* End of function VDC5_Ch0_iv7_vbuferr_ISR() */ 00975 00976 /**************************************************************************//** 00977 * @brief VDC5 IV8_VBUFERR interrupt service routine 00978 * @param[in] int_sense 00979 * @retval None 00980 *****************************************************************************/ 00981 static void VDC5_Ch0_iv8_vbuferr_ISR (const uint32_t int_sense) 00982 { 00983 uint32_t IntState; 00984 volatile uint32_t * int_clhd_reg; 00985 volatile uint32_t * int_onoff_reg; 00986 00987 UNUSED_PARAM(int_sense); 00988 00989 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int3; 00990 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_0 ].syscnt_int6; 00991 IntState = *int_onoff_reg; 00992 00993 if (((IntState & VDC5_INT_BIT_IV8_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV8_VBUFERR) != 0u)) { 00994 /* Clear */ 00995 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV8_VBUFERR; 00996 00997 if (vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV8_VBUFERR ] != 0) { 00998 vdc5_int_callback [VDC5_CHANNEL_0 ][VDC5_INT_TYPE_IV8_VBUFERR ](VDC5_INT_TYPE_IV8_VBUFERR ); 00999 } 01000 /* Set */ 01001 *int_clhd_reg = IntState; 01002 } 01003 } /* End of function VDC5_Ch0_iv8_vbuferr_ISR() */ 01004 01005 /**************************************************************************//** 01006 * @brief VDC5 S0_VI_VSYNC interrupt service routine 01007 * @param[in] int_sense 01008 * @retval None 01009 *****************************************************************************/ 01010 static void VDC5_Ch1_s0_vi_vsync_ISR (const uint32_t int_sense) 01011 { 01012 uint32_t IntState; 01013 volatile uint32_t * int_clhd_reg; 01014 volatile uint32_t * int_onoff_reg; 01015 01016 UNUSED_PARAM(int_sense); 01017 01018 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01019 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01020 IntState = *int_onoff_reg; 01021 01022 if (((IntState & VDC5_INT_BIT_S0_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VI_VSYNC) != 0u)) { 01023 /* Clear */ 01024 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VI_VSYNC; 01025 01026 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VI_VSYNC ] != 0) { 01027 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VI_VSYNC ](VDC5_INT_TYPE_S0_VI_VSYNC ); 01028 } 01029 /* Set */ 01030 *int_clhd_reg = IntState; 01031 } 01032 } /* End of function VDC5_Ch1_s0_vi_vsync_ISR() */ 01033 01034 /**************************************************************************//** 01035 * @brief VDC5 S0_LO_VSYNC interrupt service routine 01036 * @param[in] int_sense 01037 * @retval None 01038 *****************************************************************************/ 01039 static void VDC5_Ch1_s0_lo_vsync_ISR (const uint32_t int_sense) 01040 { 01041 uint32_t IntState; 01042 volatile uint32_t * int_clhd_reg; 01043 volatile uint32_t * int_onoff_reg; 01044 01045 UNUSED_PARAM(int_sense); 01046 01047 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01048 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01049 IntState = *int_onoff_reg; 01050 01051 if (((IntState & VDC5_INT_BIT_S0_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_LO_VSYNC) != 0u)) { 01052 /* Clear */ 01053 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_LO_VSYNC; 01054 01055 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_LO_VSYNC ] != 0) { 01056 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_LO_VSYNC ](VDC5_INT_TYPE_S0_LO_VSYNC ); 01057 } 01058 /* Set */ 01059 *int_clhd_reg = IntState; 01060 } 01061 } /* End of function VDC5_Ch1_s0_lo_vsync_ISR() */ 01062 01063 /**************************************************************************//** 01064 * @brief VDC5 S0_VSYNCERR interrupt service routine 01065 * @param[in] int_sense 01066 * @retval None 01067 *****************************************************************************/ 01068 static void VDC5_Ch1_s0_vsyncerr_ISR (const uint32_t int_sense) 01069 { 01070 uint32_t IntState; 01071 volatile uint32_t * int_clhd_reg; 01072 volatile uint32_t * int_onoff_reg; 01073 01074 UNUSED_PARAM(int_sense); 01075 01076 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01077 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01078 IntState = *int_onoff_reg; 01079 01080 if (((IntState & VDC5_INT_BIT_S0_VSYNCERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VSYNCERR) != 0u)) { 01081 /* Clear */ 01082 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VSYNCERR; 01083 01084 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VSYNCERR ] != 0) { 01085 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VSYNCERR ](VDC5_INT_TYPE_S0_VSYNCERR ); 01086 } 01087 /* Set */ 01088 *int_clhd_reg = IntState; 01089 } 01090 } /* End of function VDC5_Ch1_s0_vsyncerr_ISR() */ 01091 01092 /**************************************************************************//** 01093 * @brief VDC5 VLINE interrupt service routine 01094 * @param[in] int_sense 01095 * @retval None 01096 *****************************************************************************/ 01097 static void VDC5_Ch1_vline_ISR (const uint32_t int_sense) 01098 { 01099 uint32_t IntState; 01100 volatile uint32_t * int_clhd_reg; 01101 volatile uint32_t * int_onoff_reg; 01102 01103 UNUSED_PARAM(int_sense); 01104 01105 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01106 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01107 IntState = *int_onoff_reg; 01108 01109 if (((IntState & VDC5_INT_BIT_VLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_VLINE) != 0u)) { 01110 /* Clear */ 01111 *int_clhd_reg = IntState & ~VDC5_INT_BIT_VLINE; 01112 01113 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_VLINE ] != 0) { 01114 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_VLINE ](VDC5_INT_TYPE_VLINE ); 01115 } 01116 /* Set */ 01117 *int_clhd_reg = IntState; 01118 } 01119 } /* End of function VDC5_Ch1_vline_ISR() */ 01120 01121 /**************************************************************************//** 01122 * @brief VDC5 S0_VFIELD interrupt service routine 01123 * @param[in] int_sense 01124 * @retval None 01125 *****************************************************************************/ 01126 static void VDC5_Ch1_s0_vfield_ISR (const uint32_t int_sense) 01127 { 01128 uint32_t IntState; 01129 volatile uint32_t * int_clhd_reg; 01130 volatile uint32_t * int_onoff_reg; 01131 01132 UNUSED_PARAM(int_sense); 01133 01134 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01135 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01136 IntState = *int_onoff_reg; 01137 01138 if (((IntState & VDC5_INT_BIT_S0_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_VFIELD) != 0u)) { 01139 /* Clear */ 01140 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_VFIELD; 01141 01142 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VFIELD ] != 0) { 01143 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_VFIELD ](VDC5_INT_TYPE_S0_VFIELD ); 01144 } 01145 /* Set */ 01146 *int_clhd_reg = IntState; 01147 } 01148 } /* End of function VDC5_Ch1_s0_vfield_ISR() */ 01149 01150 /**************************************************************************//** 01151 * @brief VDC5 IV1_VBUFERR interrupt service routine 01152 * @param[in] int_sense 01153 * @retval None 01154 *****************************************************************************/ 01155 static void VDC5_Ch1_iv1_vbuferr_ISR (const uint32_t int_sense) 01156 { 01157 uint32_t IntState; 01158 volatile uint32_t * int_clhd_reg; 01159 volatile uint32_t * int_onoff_reg; 01160 01161 UNUSED_PARAM(int_sense); 01162 01163 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01164 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01165 IntState = *int_onoff_reg; 01166 01167 if (((IntState & VDC5_INT_BIT_IV1_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV1_VBUFERR) != 0u)) { 01168 /* Clear */ 01169 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV1_VBUFERR; 01170 01171 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV1_VBUFERR ] != 0) { 01172 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV1_VBUFERR ](VDC5_INT_TYPE_IV1_VBUFERR ); 01173 } 01174 /* Set */ 01175 *int_clhd_reg = IntState; 01176 } 01177 } /* End of function VDC5_Ch1_iv1_vbuferr_ISR() */ 01178 01179 /**************************************************************************//** 01180 * @brief VDC5 IV3_VBUFERR interrupt service routine 01181 * @param[in] int_sense 01182 * @retval None 01183 *****************************************************************************/ 01184 static void VDC5_Ch1_iv3_vbuferr_ISR (const uint32_t int_sense) 01185 { 01186 uint32_t IntState; 01187 volatile uint32_t * int_clhd_reg; 01188 volatile uint32_t * int_onoff_reg; 01189 01190 UNUSED_PARAM(int_sense); 01191 01192 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01193 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01194 IntState = *int_onoff_reg; 01195 01196 if (((IntState & VDC5_INT_BIT_IV3_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV3_VBUFERR) != 0u)) { 01197 /* Clear */ 01198 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV3_VBUFERR; 01199 01200 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV3_VBUFERR ] != 0) { 01201 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV3_VBUFERR ](VDC5_INT_TYPE_IV3_VBUFERR ); 01202 } 01203 /* Set */ 01204 *int_clhd_reg = IntState; 01205 } 01206 } /* End of function VDC5_Ch1_iv3_vbuferr_ISR() */ 01207 01208 /**************************************************************************//** 01209 * @brief VDC5 IV5_VBUFERR interrupt service routine 01210 * @param[in] int_sense 01211 * @retval None 01212 *****************************************************************************/ 01213 static void VDC5_Ch1_iv5_vbuferr_ISR (const uint32_t int_sense) 01214 { 01215 uint32_t IntState; 01216 volatile uint32_t * int_clhd_reg; 01217 volatile uint32_t * int_onoff_reg; 01218 01219 UNUSED_PARAM(int_sense); 01220 01221 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int1; 01222 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int4; 01223 IntState = *int_onoff_reg; 01224 01225 if (((IntState & VDC5_INT_BIT_IV5_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV5_VBUFERR) != 0u)) { 01226 /* Clear */ 01227 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV5_VBUFERR; 01228 01229 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV5_VBUFERR ] != 0) { 01230 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV5_VBUFERR ](VDC5_INT_TYPE_IV5_VBUFERR ); 01231 } 01232 /* Set */ 01233 *int_clhd_reg = IntState; 01234 } 01235 } /* End of function VDC5_Ch1_iv5_vbuferr_ISR() */ 01236 01237 /**************************************************************************//** 01238 * @brief VDC5 IV6_VBUFERR interrupt service routine 01239 * @param[in] int_sense 01240 * @retval None 01241 *****************************************************************************/ 01242 static void VDC5_Ch1_iv6_vbuferr_ISR (const uint32_t int_sense) 01243 { 01244 uint32_t IntState; 01245 volatile uint32_t * int_clhd_reg; 01246 volatile uint32_t * int_onoff_reg; 01247 01248 UNUSED_PARAM(int_sense); 01249 01250 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01251 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01252 IntState = *int_onoff_reg; 01253 01254 if (((IntState & VDC5_INT_BIT_IV6_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV6_VBUFERR) != 0u)) { 01255 /* Clear */ 01256 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV6_VBUFERR; 01257 01258 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV6_VBUFERR ] != 0) { 01259 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV6_VBUFERR ](VDC5_INT_TYPE_IV6_VBUFERR ); 01260 } 01261 /* Set */ 01262 *int_clhd_reg = IntState; 01263 } 01264 } /* End of function VDC5_Ch1_iv6_vbuferr_ISR() */ 01265 01266 /**************************************************************************//** 01267 * @brief VDC5 S0_WLINE interrupt service routine 01268 * @param[in] int_sense 01269 * @retval None 01270 *****************************************************************************/ 01271 static void VDC5_Ch1_s0_wline_ISR (const uint32_t int_sense) 01272 { 01273 uint32_t IntState; 01274 volatile uint32_t * int_clhd_reg; 01275 volatile uint32_t * int_onoff_reg; 01276 01277 UNUSED_PARAM(int_sense); 01278 01279 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01280 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01281 IntState = *int_onoff_reg; 01282 01283 if (((IntState & VDC5_INT_BIT_S0_WLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S0_WLINE) != 0u)) { 01284 /* Clear */ 01285 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S0_WLINE; 01286 01287 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_WLINE ] != 0) { 01288 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S0_WLINE ](VDC5_INT_TYPE_S0_WLINE ); 01289 } 01290 /* Set */ 01291 *int_clhd_reg = IntState; 01292 } 01293 } /* End of function VDC5_Ch1_s0_wline_ISR() */ 01294 01295 /**************************************************************************//** 01296 * @brief VDC5 S1_VI_VSYNC interrupt service routine 01297 * @param[in] int_sense 01298 * @retval None 01299 *****************************************************************************/ 01300 static void VDC5_Ch1_s1_vi_vsync_ISR (const uint32_t int_sense) 01301 { 01302 uint32_t IntState; 01303 volatile uint32_t * int_clhd_reg; 01304 volatile uint32_t * int_onoff_reg; 01305 01306 UNUSED_PARAM(int_sense); 01307 01308 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01309 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01310 IntState = *int_onoff_reg; 01311 01312 if (((IntState & VDC5_INT_BIT_S1_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VI_VSYNC) != 0u)) { 01313 /* Clear */ 01314 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VI_VSYNC; 01315 01316 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VI_VSYNC ] != 0) { 01317 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VI_VSYNC ](VDC5_INT_TYPE_S1_VI_VSYNC ); 01318 } 01319 /* Set */ 01320 *int_clhd_reg = IntState; 01321 } 01322 } /* End of function VDC5_Ch1_s1_vi_vsync_ISR() */ 01323 01324 /**************************************************************************//** 01325 * @brief VDC5 S1_LO_VSYNC interrupt service routine 01326 * @param[in] int_sense 01327 * @retval None 01328 *****************************************************************************/ 01329 static void VDC5_Ch1_s1_lo_vsync_ISR (const uint32_t int_sense) 01330 { 01331 uint32_t IntState; 01332 volatile uint32_t * int_clhd_reg; 01333 volatile uint32_t * int_onoff_reg; 01334 01335 UNUSED_PARAM(int_sense); 01336 01337 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01338 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01339 IntState = *int_onoff_reg; 01340 01341 if (((IntState & VDC5_INT_BIT_S1_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_LO_VSYNC) != 0u)) { 01342 /* Clear */ 01343 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_LO_VSYNC; 01344 01345 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_LO_VSYNC ] != 0) { 01346 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_LO_VSYNC ](VDC5_INT_TYPE_S1_LO_VSYNC ); 01347 } 01348 /* Set */ 01349 *int_clhd_reg = IntState; 01350 } 01351 } /* End of function VDC5_Ch1_s1_lo_vsync_ISR() */ 01352 01353 /**************************************************************************//** 01354 * @brief VDC5 S1_VSYNCERR interrupt service routine 01355 * @param[in] int_sense 01356 * @retval None 01357 *****************************************************************************/ 01358 static void VDC5_Ch1_s1_vsyncerr_ISR (const uint32_t int_sense) 01359 { 01360 uint32_t IntState; 01361 volatile uint32_t * int_clhd_reg; 01362 volatile uint32_t * int_onoff_reg; 01363 01364 UNUSED_PARAM(int_sense); 01365 01366 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01367 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01368 IntState = *int_onoff_reg; 01369 01370 if (((IntState & VDC5_INT_BIT_S1_VSYNCERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VSYNCERR) != 0u)) { 01371 /* Clear */ 01372 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VSYNCERR; 01373 01374 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VSYNCERR ] != 0) { 01375 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VSYNCERR ](VDC5_INT_TYPE_S1_VSYNCERR ); 01376 } 01377 /* Set */ 01378 *int_clhd_reg = IntState; 01379 } 01380 } /* End of function VDC5_Ch1_s1_vsyncerr_ISR() */ 01381 01382 /**************************************************************************//** 01383 * @brief VDC5 S1_VFIELD interrupt service routine 01384 * @param[in] int_sense 01385 * @retval None 01386 *****************************************************************************/ 01387 static void VDC5_Ch1_s1_vfield_ISR (const uint32_t int_sense) 01388 { 01389 uint32_t IntState; 01390 volatile uint32_t * int_clhd_reg; 01391 volatile uint32_t * int_onoff_reg; 01392 01393 UNUSED_PARAM(int_sense); 01394 01395 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01396 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01397 IntState = *int_onoff_reg; 01398 01399 if (((IntState & VDC5_INT_BIT_S1_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_VFIELD) != 0u)) { 01400 /* Clear */ 01401 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_VFIELD; 01402 01403 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VFIELD ] != 0) { 01404 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_VFIELD ](VDC5_INT_TYPE_S1_VFIELD ); 01405 } 01406 /* Set */ 01407 *int_clhd_reg = IntState; 01408 } 01409 } /* End of function VDC5_Ch1_s1_vfield_ISR() */ 01410 01411 /**************************************************************************//** 01412 * @brief VDC5 IV2_VBUFERR interrupt service routine 01413 * @param[in] int_sense 01414 * @retval None 01415 *****************************************************************************/ 01416 static void VDC5_Ch1_iv2_vbuferr_ISR (const uint32_t int_sense) 01417 { 01418 uint32_t IntState; 01419 volatile uint32_t * int_clhd_reg; 01420 volatile uint32_t * int_onoff_reg; 01421 01422 UNUSED_PARAM(int_sense); 01423 01424 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01425 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01426 IntState = *int_onoff_reg; 01427 01428 if (((IntState & VDC5_INT_BIT_IV2_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV2_VBUFERR) != 0u)) { 01429 /* Clear */ 01430 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV2_VBUFERR; 01431 01432 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV2_VBUFERR ] != 0) { 01433 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV2_VBUFERR ](VDC5_INT_TYPE_IV2_VBUFERR ); 01434 } 01435 /* Set */ 01436 *int_clhd_reg = IntState; 01437 } 01438 } /* End of function VDC5_Ch1_iv2_vbuferr_ISR() */ 01439 01440 /**************************************************************************//** 01441 * @brief VDC5 IV4_VBUFERR interrupt service routine 01442 * @param[in] int_sense 01443 * @retval None 01444 *****************************************************************************/ 01445 static void VDC5_Ch1_iv4_vbuferr_ISR (const uint32_t int_sense) 01446 { 01447 uint32_t IntState; 01448 volatile uint32_t * int_clhd_reg; 01449 volatile uint32_t * int_onoff_reg; 01450 01451 UNUSED_PARAM(int_sense); 01452 01453 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int2; 01454 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int5; 01455 IntState = *int_onoff_reg; 01456 01457 if (((IntState & VDC5_INT_BIT_IV4_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV4_VBUFERR) != 0u)) { 01458 /* Clear */ 01459 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV4_VBUFERR; 01460 01461 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV4_VBUFERR ] != 0) { 01462 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV4_VBUFERR ](VDC5_INT_TYPE_IV4_VBUFERR ); 01463 } 01464 /* Set */ 01465 *int_clhd_reg = IntState; 01466 } 01467 } /* End of function VDC5_Ch1_iv4_vbuferr_ISR() */ 01468 01469 /**************************************************************************//** 01470 * @brief VDC5 S1_WLINE interrupt service routine 01471 * @param[in] int_sense 01472 * @retval None 01473 *****************************************************************************/ 01474 static void VDC5_Ch1_s1_wline_ISR (const uint32_t int_sense) 01475 { 01476 uint32_t IntState; 01477 volatile uint32_t * int_clhd_reg; 01478 volatile uint32_t * int_onoff_reg; 01479 01480 UNUSED_PARAM(int_sense); 01481 01482 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01483 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01484 IntState = *int_onoff_reg; 01485 01486 if (((IntState & VDC5_INT_BIT_S1_WLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_S1_WLINE) != 0u)) { 01487 /* Clear */ 01488 *int_clhd_reg = IntState & ~VDC5_INT_BIT_S1_WLINE; 01489 01490 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_WLINE ] != 0) { 01491 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_S1_WLINE ](VDC5_INT_TYPE_S1_WLINE ); 01492 } 01493 /* Set */ 01494 *int_clhd_reg = IntState; 01495 } 01496 } /* End of function VDC5_Ch1_s1_wline_ISR() */ 01497 01498 /**************************************************************************//** 01499 * @brief VDC5 OIR_VI_VSYNC interrupt service routine 01500 * @param[in] int_sense 01501 * @retval None 01502 *****************************************************************************/ 01503 static void VDC5_Ch1_oir_vi_vsync_ISR (const uint32_t int_sense) 01504 { 01505 uint32_t IntState; 01506 volatile uint32_t * int_clhd_reg; 01507 volatile uint32_t * int_onoff_reg; 01508 01509 UNUSED_PARAM(int_sense); 01510 01511 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01512 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01513 IntState = *int_onoff_reg; 01514 01515 if (((IntState & VDC5_INT_BIT_OIR_VI_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VI_VSYNC) != 0u)) { 01516 /* Clear */ 01517 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VI_VSYNC; 01518 01519 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VI_VSYNC ] != 0) { 01520 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VI_VSYNC ](VDC5_INT_TYPE_OIR_VI_VSYNC ); 01521 } 01522 /* Set */ 01523 *int_clhd_reg = IntState; 01524 } 01525 } /* End of function VDC5_Ch1_oir_vi_vsync_ISR() */ 01526 01527 /**************************************************************************//** 01528 * @brief VDC5 OIR_LO_VSYNC interrupt service routine 01529 * @param[in] int_sense 01530 * @retval None 01531 *****************************************************************************/ 01532 static void VDC5_Ch1_oir_lo_vsync_ISR (const uint32_t int_sense) 01533 { 01534 uint32_t IntState; 01535 volatile uint32_t * int_clhd_reg; 01536 volatile uint32_t * int_onoff_reg; 01537 01538 UNUSED_PARAM(int_sense); 01539 01540 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01541 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01542 IntState = *int_onoff_reg; 01543 01544 if (((IntState & VDC5_INT_BIT_OIR_LO_VSYNC) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_LO_VSYNC) != 0u)) { 01545 /* Clear */ 01546 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_LO_VSYNC; 01547 01548 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_LO_VSYNC ] != 0) { 01549 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_LO_VSYNC ](VDC5_INT_TYPE_OIR_LO_VSYNC ); 01550 } 01551 /* Set */ 01552 *int_clhd_reg = IntState; 01553 } 01554 } /* End of function VDC5_Ch1_oir_lo_vsync_ISR() */ 01555 01556 /**************************************************************************//** 01557 * @brief VDC5 OIR_VLINE interrupt service routine 01558 * @param[in] int_sense 01559 * @retval None 01560 *****************************************************************************/ 01561 static void VDC5_Ch1_oir_vline_ISR (const uint32_t int_sense) 01562 { 01563 uint32_t IntState; 01564 volatile uint32_t * int_clhd_reg; 01565 volatile uint32_t * int_onoff_reg; 01566 01567 UNUSED_PARAM(int_sense); 01568 01569 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01570 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01571 IntState = *int_onoff_reg; 01572 01573 if (((IntState & VDC5_INT_BIT_OIR_VLINE) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VLINE) != 0u)) { 01574 /* Clear */ 01575 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VLINE; 01576 01577 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VLINE ] != 0) { 01578 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VLINE ](VDC5_INT_TYPE_OIR_VLINE ); 01579 } 01580 /* Set */ 01581 *int_clhd_reg = IntState; 01582 } 01583 } /* End of function VDC5_Ch1_oir_vline_ISR() */ 01584 01585 /**************************************************************************//** 01586 * @brief VDC5 OIR_VFIELD interrupt service routine 01587 * @param[in] int_sense 01588 * @retval None 01589 *****************************************************************************/ 01590 static void VDC5_Ch1_oir_vfield_ISR (const uint32_t int_sense) 01591 { 01592 uint32_t IntState; 01593 volatile uint32_t * int_clhd_reg; 01594 volatile uint32_t * int_onoff_reg; 01595 01596 UNUSED_PARAM(int_sense); 01597 01598 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01599 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01600 IntState = *int_onoff_reg; 01601 01602 if (((IntState & VDC5_INT_BIT_OIR_VFIELD) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_OIR_VFIELD) != 0u)) { 01603 /* Clear */ 01604 *int_clhd_reg = IntState & ~VDC5_INT_BIT_OIR_VFIELD; 01605 01606 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VFIELD ] != 0) { 01607 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_OIR_VFIELD ](VDC5_INT_TYPE_OIR_VFIELD ); 01608 } 01609 /* Set */ 01610 *int_clhd_reg = IntState; 01611 } 01612 } /* End of function VDC5_Ch1_oir_vfield_ISR() */ 01613 01614 /**************************************************************************//** 01615 * @brief VDC5 IV7_VBUFERR interrupt service routine 01616 * @param[in] int_sense 01617 * @retval None 01618 *****************************************************************************/ 01619 static void VDC5_Ch1_iv7_vbuferr_ISR (const uint32_t int_sense) 01620 { 01621 uint32_t IntState; 01622 volatile uint32_t * int_clhd_reg; 01623 volatile uint32_t * int_onoff_reg; 01624 01625 UNUSED_PARAM(int_sense); 01626 01627 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01628 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01629 IntState = *int_onoff_reg; 01630 01631 if (((IntState & VDC5_INT_BIT_IV7_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV7_VBUFERR) != 0u)) { 01632 /* Clear */ 01633 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV7_VBUFERR; 01634 01635 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV7_VBUFERR ] != 0) { 01636 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV7_VBUFERR ](VDC5_INT_TYPE_IV7_VBUFERR ); 01637 } 01638 /* Set */ 01639 *int_clhd_reg = IntState; 01640 } 01641 } /* End of function VDC5_Ch1_iv7_vbuferr_ISR() */ 01642 01643 /**************************************************************************//** 01644 * @brief VDC5 IV8_VBUFERR interrupt service routine 01645 * @param[in] int_sense 01646 * @retval None 01647 *****************************************************************************/ 01648 static void VDC5_Ch1_iv8_vbuferr_ISR (const uint32_t int_sense) 01649 { 01650 uint32_t IntState; 01651 volatile uint32_t * int_clhd_reg; 01652 volatile uint32_t * int_onoff_reg; 01653 01654 UNUSED_PARAM(int_sense); 01655 01656 int_clhd_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int3; 01657 int_onoff_reg = vdc5_regaddr_system_ctrl[VDC5_CHANNEL_1 ].syscnt_int6; 01658 IntState = *int_onoff_reg; 01659 01660 if (((IntState & VDC5_INT_BIT_IV8_VBUFERR) != 0u) && ((*int_clhd_reg & VDC5_INT_BIT_IV8_VBUFERR) != 0u)) { 01661 /* Clear */ 01662 *int_clhd_reg = IntState & ~VDC5_INT_BIT_IV8_VBUFERR; 01663 01664 if (vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV8_VBUFERR ] != 0) { 01665 vdc5_int_callback [VDC5_CHANNEL_1 ][VDC5_INT_TYPE_IV8_VBUFERR ](VDC5_INT_TYPE_IV8_VBUFERR ); 01666 } 01667 /* Set */ 01668 *int_clhd_reg = IntState; 01669 } 01670 } /* End of function VDC5_Ch1_iv8_vbuferr_ISR() */ 01671
Generated on Tue Jul 12 2022 15:08:46 by
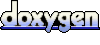