Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
r_vdc5.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file r_vdc5.h 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief VDC5 driver API definitions 00029 ******************************************************************************/ 00030 00031 #ifndef R_VDC5_H 00032 #define R_VDC5_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include "r_vdc5_user.h" 00038 00039 00040 #ifdef __cplusplus 00041 extern "C" 00042 { 00043 #endif /* __cplusplus */ 00044 00045 00046 /****************************************************************************** 00047 Macro definitions 00048 ******************************************************************************/ 00049 #define VDC5_GAM_GAIN_ADJ_NUM (32u) /*!< The number of the gamma correction gain coefficient */ 00050 #define VDC5_GAM_START_TH_NUM (31u) /*!< The number of the gamma correction start threshold */ 00051 00052 00053 /****************************************************************************** 00054 Typedef definitions 00055 ******************************************************************************/ 00056 /*! Error codes of the VDC5 driver */ 00057 typedef enum { 00058 VDC5_OK = 0, /*!< Normal termination */ 00059 VDC5_ERR_PARAM_CHANNEL , /*!< Invalid channel error (parameter error): An illegal channel is specified. */ 00060 VDC5_ERR_PARAM_LAYER_ID , /*!< Invalid layer ID error (parameter error): An illegal layer ID is specified. */ 00061 VDC5_ERR_PARAM_NULL , /*!< NULL specification error (parameter error): 00062 NULL is specified for a required parameter. */ 00063 VDC5_ERR_PARAM_BIT_WIDTH , /*!< Bit width error (parameter error): 00064 A value exceeding the possible bit width is specified. */ 00065 VDC5_ERR_PARAM_UNDEFINED , /*!< Undefined parameter error (parameter error): 00066 A value that is not defined in the specification is specified. */ 00067 VDC5_ERR_PARAM_EXCEED_RANGE ,/*!< Out-of-value-range error (parameter error): 00068 The specified parameter value is beyond the value range defined 00069 in the specification. */ 00070 VDC5_ERR_PARAM_CONDITION , /*!< Unauthorized condition error (parameter error): 00071 A parameter is specified under conditions that are not authorized 00072 by the specification. */ 00073 VDC5_ERR_IF_CONDITION , /*!< Interface condition error (interface error): 00074 An API function is called under unauthorized conditions. */ 00075 VDC5_ERR_RESOURCE_CLK , /*!< Clock resource error (resource error): No panel clock is set up. */ 00076 VDC5_ERR_RESOURCE_VSYNC , /*!< Vsync signal resource error (resource error): No Vsync signal is set up. */ 00077 VDC5_ERR_RESOURCE_INPUT , /*!< Input signal resource error (resource error): No video image input is set up. */ 00078 VDC5_ERR_RESOURCE_OUTPUT , /*!< Output resource error (resource error): No display output is set up. */ 00079 VDC5_ERR_RESOURCE_LVDS_CLK , /*!< LVDS clock resource error (resource error): 00080 An attempt is made to use the LVDS clock without setting it up, 00081 or the LVDS clock is specified when it is already set up. */ 00082 VDC5_ERR_RESOURCE_LAYER , /*!< Layer resource error (resource error): 00083 The specified layer is under unavailable conditions. */ 00084 VDC5_ERR_NUM /*!< The number of error codes */ 00085 } vdc5_error_t ; 00086 00087 /*! VDC5 channels */ 00088 typedef enum { 00089 VDC5_CHANNEL_0 = 0, /*!< Channel 0 */ 00090 VDC5_CHANNEL_1 , /*!< Channel 1 */ 00091 VDC5_CHANNEL_NUM /*!< The number of channels */ 00092 } vdc5_channel_t ; 00093 00094 /*! On/off */ 00095 typedef enum { 00096 VDC5_OFF = 0, /*!< Off */ 00097 VDC5_ON = 1 /*!< On */ 00098 } vdc5_onoff_t ; 00099 /*! Edge of a signal */ 00100 typedef enum { 00101 VDC5_EDGE_RISING = 0, /*!< Rising edge */ 00102 VDC5_EDGE_FALLING = 1 /*!< Falling edge */ 00103 } vdc5_edge_t ; 00104 /*! Polarity of a signal */ 00105 typedef enum { 00106 VDC5_SIG_POL_NOT_INVERTED = 0, /*!< Not inverted */ 00107 VDC5_SIG_POL_INVERTED = 1 /*!< Inverted */ 00108 } vdc5_sig_pol_t ; 00109 00110 /*! Scaling type ID */ 00111 typedef enum { 00112 VDC5_SC_TYPE_SC0 = 0, /*!< Scaler 0 */ 00113 VDC5_SC_TYPE_SC1 , /*!< Scaler 1 */ 00114 VDC5_SC_TYPE_OIR , /*!< Output image generator (OIR) */ 00115 VDC5_SC_TYPE_NUM /*!< The number of scaler types */ 00116 } vdc5_scaling_type_t ; 00117 /*! Graphics type ID */ 00118 typedef enum { 00119 VDC5_GR_TYPE_GR0 = 0, /*!< Graphics 0 */ 00120 VDC5_GR_TYPE_GR1 , /*!< Graphics 1 */ 00121 VDC5_GR_TYPE_GR2 , /*!< Graphics 2 */ 00122 VDC5_GR_TYPE_GR3 , /*!< Graphics 3 */ 00123 VDC5_GR_TYPE_VIN , /*!< VIN synthesizer */ 00124 VDC5_GR_TYPE_OIR , /*!< Output image generator (OIR) */ 00125 VDC5_GR_TYPE_NUM /*!< The number of graphics types */ 00126 } vdc5_graphics_type_t ; 00127 /*! Layer ID */ 00128 typedef enum { 00129 VDC5_LAYER_ID_ALL = -1, /*!< All layers */ 00130 VDC5_LAYER_ID_0_WR = (VDC5_SC_TYPE_SC0 + 0), /*!< Write process for layer 0 */ 00131 VDC5_LAYER_ID_1_WR = (VDC5_SC_TYPE_SC1 + 0), /*!< Write process for layer 1 */ 00132 VDC5_LAYER_ID_OIR_WR = (VDC5_SC_TYPE_OIR + 0), /*!< Write process for layer OIR */ 00133 VDC5_LAYER_ID_0_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_GR0 ), /*!< Read process for layer 0 */ 00134 VDC5_LAYER_ID_1_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_GR1 ), /*!< Read process for layer 1 */ 00135 VDC5_LAYER_ID_2_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_GR2 ), /*!< Read process for layer 2 */ 00136 VDC5_LAYER_ID_3_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_GR3 ), /*!< Read process for layer 3 */ 00137 VDC5_LAYER_ID_VIN_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_VIN ), /*!< VIN synthesizer */ 00138 VDC5_LAYER_ID_OIR_RD = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_OIR ), /*!< Read process for layer OIR */ 00139 VDC5_LAYER_ID_NUM = (VDC5_SC_TYPE_NUM + VDC5_GR_TYPE_NUM ) /*!< The number of layer IDs */ 00140 } vdc5_layer_id_t ; 00141 /*! The horizontal/vertical timing of the VDC5 signals */ 00142 typedef struct { 00143 uint16_t vs ; /*!< Vertical signal start position from the reference signal */ 00144 uint16_t vw ; /*!< Vertical signal width (height) */ 00145 uint16_t hs ; /*!< Horizontal signal start position from the reference signal */ 00146 uint16_t hw ; /*!< Horizontal signal width */ 00147 } vdc5_period_rect_t ; 00148 /*! The relative position within the graphics display area */ 00149 typedef struct { 00150 uint16_t vs_rel ; /*!< Vertical start position */ 00151 uint16_t vw_rel ; /*!< Vertical width (height) */ 00152 uint16_t hs_rel ; /*!< Horizontal start position */ 00153 uint16_t hw_rel ; /*!< Horizontal width */ 00154 } vdc5_pd_disp_rect_t ; 00155 00156 /*********************** For R_VDC5_Initialize ***********************/ 00157 /*! Panel clock select */ 00158 typedef enum { 00159 VDC5_PANEL_ICKSEL_IMG = 0, /*!< Divided video image clock (VIDEO_X1) */ 00160 VDC5_PANEL_ICKSEL_IMG_DV , /*!< Divided video image clock (DV_CLK) */ 00161 VDC5_PANEL_ICKSEL_EXT_0 , /*!< Divided external clock (LCD0_EXTCLK) */ 00162 VDC5_PANEL_ICKSEL_EXT_1 , /*!< Divided external clock (LCD1_EXTCLK) */ 00163 VDC5_PANEL_ICKSEL_PERI , /*!< Divided peripheral clock 1 */ 00164 VDC5_PANEL_ICKSEL_LVDS , /*!< LVDS PLL clock */ 00165 VDC5_PANEL_ICKSEL_LVDS_DIV7 , /*!< LVDS PLL clock divided by 7 */ 00166 VDC5_PANEL_ICKSEL_NUM /*!< The number of panel clock select settings */ 00167 } vdc5_panel_clksel_t ; 00168 /*! Clock frequency division ratio */ 00169 typedef enum { 00170 VDC5_PANEL_CLKDIV_1_1 = 0, /*!< Division Ratio 1/1 */ 00171 VDC5_PANEL_CLKDIV_1_2 , /*!< Division Ratio 1/2 */ 00172 VDC5_PANEL_CLKDIV_1_3 , /*!< Division Ratio 1/3 */ 00173 VDC5_PANEL_CLKDIV_1_4 , /*!< Division Ratio 1/4 */ 00174 VDC5_PANEL_CLKDIV_1_5 , /*!< Division Ratio 1/5 */ 00175 VDC5_PANEL_CLKDIV_1_6 , /*!< Division Ratio 1/6 */ 00176 VDC5_PANEL_CLKDIV_1_7 , /*!< Division Ratio 1/7 */ 00177 VDC5_PANEL_CLKDIV_1_8 , /*!< Division Ratio 1/8 */ 00178 VDC5_PANEL_CLKDIV_1_9 , /*!< Division Ratio 1/9 */ 00179 VDC5_PANEL_CLKDIV_1_12 , /*!< Division Ratio 1/12 */ 00180 VDC5_PANEL_CLKDIV_1_16 , /*!< Division Ratio 1/16 */ 00181 VDC5_PANEL_CLKDIV_1_24 , /*!< Division Ratio 1/24 */ 00182 VDC5_PANEL_CLKDIV_1_32 , /*!< Division Ratio 1/32 */ 00183 VDC5_PANEL_CLKDIV_NUM /*!< The number of division ratio settings */ 00184 } vdc5_panel_clk_dcdr_t ; 00185 /*! The clock input to frequency divider 1 */ 00186 typedef enum { 00187 VDC5_LVDS_INCLK_SEL_IMG = 0, /*!< Video image clock (VIDEO_X1) */ 00188 VDC5_LVDS_INCLK_SEL_DV_0 , /*!< Video image clock (DV0_CLK) */ 00189 VDC5_LVDS_INCLK_SEL_DV_1 , /*!< Video image clock (DV1_CLK) */ 00190 VDC5_LVDS_INCLK_SEL_EXT_0 , /*!< External clock (LCD0_EXTCLK) */ 00191 VDC5_LVDS_INCLK_SEL_EXT_1 , /*!< External clock (LCD1_EXTCLK) */ 00192 VDC5_LVDS_INCLK_SEL_PERI , /*!< Peripheral clock 1 */ 00193 VDC5_LVDS_INCLK_SEL_NUM 00194 } vdc5_lvds_in_clk_sel_t ; 00195 /*! The frequency dividing value (NIDIV or NODIV) */ 00196 typedef enum { 00197 VDC5_LVDS_NDIV_1 = 0, /*!< Div 1 */ 00198 VDC5_LVDS_NDIV_2 , /*!< Div 2 */ 00199 VDC5_LVDS_NDIV_4 , /*!< Div 4 */ 00200 VDC5_LVDS_NDIV_NUM 00201 } vdc5_lvds_ndiv_t ; 00202 /*! The frequency dividing value (NOD) for the output frequency */ 00203 typedef enum { 00204 VDC5_LVDS_PLL_NOD_1 = 0, /*!< Div 1 */ 00205 VDC5_LVDS_PLL_NOD_2 , /*!< Div 2 */ 00206 VDC5_LVDS_PLL_NOD_4 , /*!< Div 4 */ 00207 VDC5_LVDS_PLL_NOD_8 , /*!< Div 8 */ 00208 VDC5_LVDS_PLL_NOD_NUM 00209 } vdc5_lvds_pll_nod_t ; 00210 /*! LVDS parameter */ 00211 typedef struct { 00212 vdc5_lvds_in_clk_sel_t lvds_in_clk_sel ;/*!< The clock input to frequency divider 1 */ 00213 vdc5_lvds_ndiv_t lvds_idiv_set ; /*!< The frequency dividing value (NIDIV) for frequency divider 1 */ 00214 uint16_t lvdspll_tst ; /*!< Internal parameter setting for LVDS PLL */ 00215 vdc5_lvds_ndiv_t lvds_odiv_set ; /*!< The frequency dividing value (NODIV) for frequency divider 2 */ 00216 vdc5_channel_t lvds_vdc_sel ; /*!< A channel in VDC5 whose data is to be output through the LVDS */ 00217 uint16_t lvdspll_fd ; /*!< The frequency dividing value (NFD) for the feedback frequency */ 00218 uint16_t lvdspll_rd ; /*!< The frequency dividing value (NRD) for the input frequency */ 00219 vdc5_lvds_pll_nod_t lvdspll_od ; /*!< The frequency dividing value (NOD) for the output frequency */ 00220 } vdc5_lvds_t ; 00221 /*! Initialization parameter */ 00222 typedef struct { 00223 vdc5_panel_clksel_t panel_icksel ; /*!< Panel clock select */ 00224 vdc5_panel_clk_dcdr_t panel_dcdr ; /*!< Clock frequency division ratio */ 00225 const vdc5_lvds_t * lvds ; /*!< LVDS-related parameter */ 00226 } vdc5_init_t ; 00227 00228 /*********************** For R_VDC5_VideoInput ***********************/ 00229 /*! Input select */ 00230 typedef enum { 00231 VDC5_INPUT_SEL_VDEC = 0, /*!< Video decoder output signals */ 00232 VDC5_INPUT_SEL_EXT = 1 /*!< Signals supplied via the external input pins */ 00233 } vdc5_input_sel_t ; 00234 /*! Sync signal delay adjustment parameter */ 00235 typedef struct { 00236 uint16_t inp_vs_dly_l ; /*!< Number of lines for delaying Vsync signal and field differentiation signal */ 00237 uint16_t inp_fld_dly ; /*!< Field differentiation signal delay amount */ 00238 uint16_t inp_vs_dly ; /*!< Vsync signal delay amount */ 00239 uint16_t inp_hs_dly ; /*!< Hsync signal delay amount */ 00240 } vdc5_sync_delay_t ; 00241 /*! External input format select */ 00242 typedef enum { 00243 VDC5_EXTIN_FORMAT_RGB888 = 0, /*!< RGB888 */ 00244 VDC5_EXTIN_FORMAT_RGB666 , /*!< RGB666 */ 00245 VDC5_EXTIN_FORMAT_RGB565 , /*!< RGB565 */ 00246 VDC5_EXTIN_FORMAT_BT656 , /*!< BT6556 */ 00247 VDC5_EXTIN_FORMAT_BT601 , /*!< BT6501 */ 00248 VDC5_EXTIN_FORMAT_YCBCR422 , /*!< YCbCr422 */ 00249 VDC5_EXTIN_FORMAT_YCBCR444 , /*!< YCbCr444 */ 00250 VDC5_EXTIN_FORMAT_NUM 00251 } vdc5_extin_format_t ; 00252 /*! Reference select for external input BT.656 Hsync signal */ 00253 typedef enum { 00254 VDC5_EXTIN_REF_H_EAV = 0, /*!< EAV */ 00255 VDC5_EXTIN_REF_H_SAV = 1 /*!< SAV */ 00256 } vdc5_extin_ref_hsync_t ; 00257 /*! Number of lines for BT.656 external input */ 00258 typedef enum { 00259 VDC5_EXTIN_LINE_525 = 0, /*!< 525 lines */ 00260 VDC5_EXTIN_LINE_625 = 1 /*!< 625 lines */ 00261 } vdc5_extin_input_line_t ; 00262 /*! Y/Cb/Y/Cr data string start timing */ 00263 typedef enum { 00264 VDC5_EXTIN_H_POS_CBYCRY = 0, /*!< Cb/Y/Cr/Y (BT656/601), Cb/Cr (YCbCr422) */ 00265 VDC5_EXTIN_H_POS_YCRYCB , /*!< Y/Cr/Y/Cb (BT656/601), setting prohibited (YCbCr422) */ 00266 VDC5_EXTIN_H_POS_CRYCBY , /*!< Cr/Y/Cb/Y (BT656/601), setting prohibited (YCbCr422) */ 00267 VDC5_EXTIN_H_POS_YCBYCR , /*!< Y/Cb/Y/Cr (BT656/601), Cr/Cb (YCbCr422) */ 00268 VDC5_EXTIN_H_POS_NUM 00269 } vdc5_extin_h_pos_t ; 00270 /*! External input signal parameter */ 00271 typedef struct { 00272 vdc5_extin_format_t inp_format ; /*!< External input format select */ 00273 vdc5_edge_t inp_pxd_edge ; /*!< Clock edge select for capturing external input video image signals */ 00274 vdc5_edge_t inp_vs_edge ; /*!< Clock edge select for capturing external input Vsync signals */ 00275 vdc5_edge_t inp_hs_edge ; /*!< Clock edge select for capturing external input Hsync signals */ 00276 vdc5_onoff_t inp_endian_on ; /*!< External input bit endian change on/off control */ 00277 vdc5_onoff_t inp_swap_on ; /*!< External input B/R signal swap on/off control */ 00278 vdc5_sig_pol_t inp_vs_inv ; /*!< External input Vsync signal DV_VSYNC inversion control */ 00279 vdc5_sig_pol_t inp_hs_inv ; /*!< External input Hsync signal DV_HSYNC inversion control */ 00280 vdc5_extin_ref_hsync_t inp_h_edge_sel ; /*!< Reference select for external input BT.656 Hsync signal */ 00281 vdc5_extin_input_line_t inp_f525_625 ; /*!< Number of lines for BT.656 external input */ 00282 vdc5_extin_h_pos_t inp_h_pos ; /*!< Y/Cb/Y/Cr data string start timing to Hsync reference */ 00283 } vdc5_ext_in_sig_t ; 00284 /*! Video input setup parameter */ 00285 typedef struct { 00286 vdc5_input_sel_t inp_sel ; /*!< Input select */ 00287 uint16_t inp_fh50 ; /*!< Vsync signal 1/2fH phase timing */ 00288 uint16_t inp_fh25 ; /*!< Vsync signal 1/4fH phase timing */ 00289 const vdc5_sync_delay_t * dly ; /*!< Sync signal delay adjustment parameter */ 00290 const vdc5_ext_in_sig_t * ext_sig ; /*!< External input signal parameter */ 00291 } vdc5_input_t ; 00292 00293 /*********************** For R_VDC5_SyncControl ***********************/ 00294 /*! Horizontal/vertical sync signal output and full-screen enable signal select */ 00295 typedef enum { 00296 VDC5_RES_VS_IN_SEL_SC0 = 0, /*!< Sync signal output and full-screen enable signal from scaler 0 */ 00297 VDC5_RES_VS_IN_SEL_SC1 = 1 /*!< Sync signal output and full-screen enable signal from scaler 1 */ 00298 } vdc5_res_vs_in_sel_t ; 00299 /*! Vsync signal compensation parameter */ 00300 typedef struct { 00301 uint16_t res_vmask ; /*!< Repeated Vsync signal masking period */ 00302 uint16_t res_vlack ; /*!< Missing-Sync compensating pulse output wait time */ 00303 } vdc5_vsync_cpmpe_t ; 00304 /*! Sync signal control parameter */ 00305 typedef struct { 00306 vdc5_onoff_t res_vs_sel ; /*!< Vsync signal output select (free-running Vsync on/off control) */ 00307 vdc5_res_vs_in_sel_t res_vs_in_sel; /*!< Horizontal/vertical sync signal output 00308 and full-screen enable signal select */ 00309 uint16_t res_fv ; /*!< Free-running Vsync period setting */ 00310 uint16_t res_fh ; /*!< Hsync period setting */ 00311 uint16_t res_vsdly ; /*!< Vsync signal delay control */ 00312 vdc5_period_rect_t res_f ; /*!< Full-screen enable signal */ 00313 const vdc5_vsync_cpmpe_t * vsync_cpmpe ; /*!< Vsync signal compensation parameter */ 00314 } vdc5_sync_ctrl_t ; 00315 00316 /*********************** For R_VDC5_DisplayOutput ***********************/ 00317 /*! POLA/POLB signal generation mode select */ 00318 typedef enum { 00319 VDC5_LCD_TCON_POLMD_NORMAL = 0, /*!< Normal mode */ 00320 VDC5_LCD_TCON_POLMD_1X1REV , /*!< 1x1 reverse mode */ 00321 VDC5_LCD_TCON_POLMD_1X2REV , /*!< 1x2 reverse mode */ 00322 VDC5_LCD_TCON_POLMD_2X2REV , /*!< 2x2 reverse mode */ 00323 VDC5_LCD_TCON_POLMD_NUM 00324 } vdc5_lcd_tcon_polmode_t ; 00325 /*! Signal operating reference select */ 00326 typedef enum { 00327 VDC5_LCD_TCON_REFSEL_HSYNC = 0, /*!< Hsync signal reference */ 00328 VDC5_LCD_TCON_REFSEL_OFFSET_H = 1 /*!< Offset Hsync signal reference */ 00329 } vdc5_lcd_tcon_refsel_t ; 00330 /*! LCD TCON output pin select */ 00331 typedef enum { 00332 VDC5_LCD_TCON_PIN_NON = -1, /*!< Nothing output */ 00333 VDC5_LCD_TCON_PIN_0 , /*!< LCD_TCON0 */ 00334 VDC5_LCD_TCON_PIN_1 , /*!< LCD_TCON1 */ 00335 VDC5_LCD_TCON_PIN_2 , /*!< LCD_TCON2 */ 00336 VDC5_LCD_TCON_PIN_3 , /*!< LCD_TCON3 */ 00337 VDC5_LCD_TCON_PIN_4 , /*!< LCD_TCON4 */ 00338 VDC5_LCD_TCON_PIN_5 , /*!< LCD_TCON5 */ 00339 VDC5_LCD_TCON_PIN_6 , /*!< LCD_TCON6 */ 00340 VDC5_LCD_TCON_PIN_NUM 00341 } vdc5_lcd_tcon_pin_t ; 00342 /*! LCD TCON timing signal parameter */ 00343 typedef struct { 00344 uint16_t tcon_hsvs ; /*!< Signal pulse start position */ 00345 uint16_t tcon_hwvw ; /*!< Pulse width */ 00346 vdc5_lcd_tcon_polmode_t tcon_md ; /*!< POLA/POLB signal generation mode select */ 00347 vdc5_lcd_tcon_refsel_t tcon_hs_sel ; /*!< Signal operating reference select */ 00348 vdc5_sig_pol_t tcon_inv ; /*!< Polarity inversion control of signal */ 00349 vdc5_lcd_tcon_pin_t tcon_pin ; /*!< LCD TCON output pin select */ 00350 vdc5_edge_t outcnt_edge ; /*!< Output phase control of signal */ 00351 } vdc5_lcd_tcon_timing_t ; 00352 /*! Timing signals for driving the LCD panel */ 00353 typedef enum { 00354 VDC5_LCD_TCONSIG_STVA_VS = 0, /*!< STVA/VS */ 00355 VDC5_LCD_TCONSIG_STVB_VE , /*!< STVB/VE */ 00356 VDC5_LCD_TCONSIG_STH_SP_HS , /*!< STH/SP/HS */ 00357 VDC5_LCD_TCONSIG_STB_LP_HE , /*!< STB/LP/HE */ 00358 VDC5_LCD_TCONSIG_CPV_GCK , /*!< CPV/GCK */ 00359 VDC5_LCD_TCONSIG_POLA , /*!< POLA */ 00360 VDC5_LCD_TCONSIG_POLB , /*!< POLB */ 00361 VDC5_LCD_TCONSIG_DE , /*!< DE */ 00362 VDC5_LCD_TCONSIG_NUM 00363 } vdc5_lcd_tcon_sigsel_t ; 00364 /*! Output format select */ 00365 typedef enum { 00366 VDC5_LCD_OUTFORMAT_RGB888 = 0, /*!< RGB888 */ 00367 VDC5_LCD_OUTFORMAT_RGB666 , /*!< RGB666 */ 00368 VDC5_LCD_OUTFORMAT_RGB565 , /*!< RGB565 */ 00369 VDC5_LCD_OUTFORMAT_SERIAL_RGB , /*!< Serial RGB */ 00370 VDC5_LCD_OUTFORMAT_NUM 00371 } vdc5_lcd_outformat_t ; 00372 /*! Clock frequency control */ 00373 typedef enum { 00374 VDC5_LCD_PARALLEL_CLKFRQ_1 = 0, /*!< 100% speed (parallel RGB) */ 00375 VDC5_LCD_SERIAL_CLKFRQ_3 , /*!< Triple speed (serial RGB) */ 00376 VDC5_LCD_SERIAL_CLKFRQ_4 , /*!< Quadruple speed (serial RGB) */ 00377 VDC5_LCD_SERIAL_CLKFRQ_NUM 00378 } vdc5_lcd_clkfreqsel_t ; 00379 /*! Scan direction select */ 00380 typedef enum { 00381 VDC5_LCD_SERIAL_SCAN_FORWARD = 0, /*!< Forward scan */ 00382 VDC5_LCD_SERIAL_SCAN_REVERSE = 1 /*!< Reverse scan */ 00383 } vdc5_lcd_scan_t ; 00384 /*! Clock phase adjustment for serial RGB output */ 00385 typedef enum { 00386 VDC5_LCD_SERIAL_CLKPHASE_0 = 0, /*!< 0[clk] */ 00387 VDC5_LCD_SERIAL_CLKPHASE_1 , /*!< 1[clk] */ 00388 VDC5_LCD_SERIAL_CLKPHASE_2 , /*!< 2[clk] */ 00389 VDC5_LCD_SERIAL_CLKPHASE_3 , /*!< 3[clk] */ 00390 VDC5_LCD_SERIAL_CLKPHASE_NUM 00391 } vdc5_lcd_clkphase_t ; 00392 /*! Display output configuration parameter */ 00393 typedef struct { 00394 uint16_t tcon_half ; /*!< 1/2fH timing */ 00395 uint16_t tcon_offset ; /*!< Offset Hsync signal timing */ 00396 const vdc5_lcd_tcon_timing_t * outctrl[VDC5_LCD_TCONSIG_NUM]; /*!< LCD TCON timing signal parameter */ 00397 vdc5_edge_t outcnt_lcd_edge; /*!< Output phase control of LCD_DATA23 00398 to LCD_DATA0 pin */ 00399 vdc5_onoff_t out_endian_on ; /*!< Bit endian change on/off control */ 00400 vdc5_onoff_t out_swap_on ; /*!< B/R signal swap on/off control */ 00401 vdc5_lcd_outformat_t out_format ; /*!< Output format select */ 00402 vdc5_lcd_clkfreqsel_t out_frq_sel ; /*!< Clock frequency control */ 00403 vdc5_lcd_scan_t out_dir_sel ; /*!< Scan direction select */ 00404 vdc5_lcd_clkphase_t out_phase; /*!< Clock phase adjustment 00405 for serial RGB output */ 00406 uint32_t bg_color ; /*!< Background color in 24-bit RGB color format */ 00407 } vdc5_output_t ; 00408 00409 /*********************** For R_VDC5_CallbackISR ***********************/ 00410 /*! VDC5 interrupt type */ 00411 typedef enum { 00412 VDC5_INT_TYPE_S0_VI_VSYNC = 0, /*!< Vsync signal input to scaler 0 */ 00413 VDC5_INT_TYPE_S0_LO_VSYNC , /*!< Vsync signal output from scaler 0 */ 00414 VDC5_INT_TYPE_S0_VSYNCERR , /*!< Missing Vsync signal for scaler 0 */ 00415 VDC5_INT_TYPE_VLINE , /*!< Specified line signal for panel output in graphics 3 */ 00416 VDC5_INT_TYPE_S0_VFIELD , /*!< Field end signal for recording function in scaler 0 */ 00417 VDC5_INT_TYPE_IV1_VBUFERR , /*!< Frame buffer write overflow signal for scaler 0 */ 00418 VDC5_INT_TYPE_IV3_VBUFERR , /*!< Frame buffer read underflow signal for graphics 0 */ 00419 VDC5_INT_TYPE_IV5_VBUFERR , /*!< Frame buffer read underflow signal for graphics 2 */ 00420 VDC5_INT_TYPE_IV6_VBUFERR , /*!< Frame buffer read underflow signal for graphics 3 */ 00421 VDC5_INT_TYPE_S0_WLINE , /*!< Write specification line signal input to scaling-down control block 00422 in scaler 0 */ 00423 VDC5_INT_TYPE_S1_VI_VSYNC , /*!< Vsync signal input to scaler 1 */ 00424 VDC5_INT_TYPE_S1_LO_VSYNC , /*!< Vsync signal output from scaler 1 */ 00425 VDC5_INT_TYPE_S1_VSYNCERR , /*!< Missing Vsync signal for scaler 1 */ 00426 VDC5_INT_TYPE_S1_VFIELD , /*!< Field end signal for recording function in scaler 1 */ 00427 VDC5_INT_TYPE_IV2_VBUFERR , /*!< Frame buffer write overflow signal for scaler 1 */ 00428 VDC5_INT_TYPE_IV4_VBUFERR , /*!< Frame buffer read underflow signal for graphics 1 */ 00429 VDC5_INT_TYPE_S1_WLINE , /*!< Write specification line signal input to scaling-down control block 00430 in scaler 1 */ 00431 VDC5_INT_TYPE_OIR_VI_VSYNC , /*!< Vsync signal input to output image generator */ 00432 VDC5_INT_TYPE_OIR_LO_VSYNC , /*!< Vsync signal output from output image generator */ 00433 VDC5_INT_TYPE_OIR_VLINE , /*!< Specified line signal for panel output in output image generator */ 00434 VDC5_INT_TYPE_OIR_VFIELD , /*!< Field end signal for recording function in output image generator */ 00435 VDC5_INT_TYPE_IV7_VBUFERR , /*!< Frame buffer write overflow signal for output image generator */ 00436 VDC5_INT_TYPE_IV8_VBUFERR , /*!< Frame buffer read underflow signal for graphics (OIR) */ 00437 VDC5_INT_TYPE_NUM /*!< The number of VDC5 interrupt types */ 00438 } vdc5_int_type_t ; 00439 /*! Interrupt callback setup parameter */ 00440 typedef struct { 00441 vdc5_int_type_t type ; /*!< VDC5 interrupt type */ 00442 void (* callback)(vdc5_int_type_t ); /*!< Interrupt callback function pointer */ 00443 uint16_t line_num ; /*!< Line interrupt set */ 00444 } vdc5_int_t ; 00445 00446 /*********************** For R_VDC5_WriteDataControl ***********************/ 00447 /*! Frame buffer writing mode for image processing */ 00448 typedef enum { 00449 VDC5_WR_MD_NORMAL = 0, /*!< Normal */ 00450 VDC5_WR_MD_MIRROR , /*!< Horizontal mirroring */ 00451 VDC5_WR_MD_ROT_90DEG , /*!< 90 degree rotation */ 00452 VDC5_WR_MD_ROT_180DEG , /*!< 180 degree rotation */ 00453 VDC5_WR_MD_ROT_270DEG , /*!< 270 degree rotation */ 00454 VDC5_WR_MD_NUM 00455 } vdc5_wr_md_t ; 00456 /*! Scaling-down and rotation parameter */ 00457 typedef struct { 00458 vdc5_period_rect_t res ; /*!< Image area to be captured */ 00459 vdc5_onoff_t res_pfil_sel ; /*!< Prefilter mode select for brightness signals (on/off) */ 00460 uint16_t res_out_vw; /*!< Number of valid lines in vertical direction 00461 output by scaling-down control block */ 00462 uint16_t res_out_hw; /*!< Number of valid horizontal pixels 00463 output by scaling-down control block */ 00464 vdc5_onoff_t adj_sel ; /*!< Handling for lack of last-input line (on/off) */ 00465 vdc5_wr_md_t res_ds_wr_md ; /*!< Frame buffer writing mode for image processing */ 00466 } vdc5_scalingdown_rot_t ; 00467 /*! Frame buffer swap setting */ 00468 typedef enum { 00469 VDC5_WR_RD_WRSWA_NON = 0, /*!< Not swapped: 1-2-3-4-5-6-7-8 */ 00470 VDC5_WR_RD_WRSWA_8BIT , /*!< Swapped in 8-bit units: 2-1-4-3-6-5-8-7 */ 00471 VDC5_WR_RD_WRSWA_16BIT , /*!< Swapped in 16-bit units: 3-4-1-2-7-8-5-6 */ 00472 VDC5_WR_RD_WRSWA_16_8BIT , /*!< Swapped in 16-bit units + 8-bit units: 4-3-2-1-8-7-6-5 */ 00473 VDC5_WR_RD_WRSWA_32BIT , /*!< Swapped in 32-bit units: 5-6-7-8-1-2-3-4 */ 00474 VDC5_WR_RD_WRSWA_32_8BIT , /*!< Swapped in 32-bit units + 8-bit units: 6-5-8-7-2-1-4-3 */ 00475 VDC5_WR_RD_WRSWA_32_16BIT , /*!< Swapped in 32-bit units + 16-bit units: 7-8-5-6-3-4-1-2 */ 00476 VDC5_WR_RD_WRSWA_32_16_8BIT , /*!< Swapped in 32-bit units + 16-bit units + 8-bit units: 8-7-6-5-4-3-2-1 */ 00477 VDC5_WR_RD_WRSWA_NUM 00478 } vdc5_wr_rd_swa_t ; 00479 /*! Frame buffer video-signal writing format */ 00480 typedef enum { 00481 VDC5_RES_MD_YCBCR422 = 0, /*!< YCbCr422 */ 00482 VDC5_RES_MD_RGB565 , /*!< RGB565 */ 00483 VDC5_RES_MD_RGB888 , /*!< RGB888 */ 00484 VDC5_RES_MD_YCBCR444 , /*!< YCbCr444 */ 00485 VDC5_RES_MD_NUM 00486 } vdc5_res_md_t ; 00487 /*! Transfer burst length */ 00488 typedef enum { 00489 VDC5_BST_MD_32BYTE = 0, /*!< 32-byte transfer (4 bursts) */ 00490 VDC5_BST_MD_128BYTE /*!< 128-byte transfer (16 bursts) */ 00491 } vdc5_bst_md_t ; 00492 /*! Field operating mode select */ 00493 typedef enum { 00494 VDC5_RES_INTER_PROGRESSIVE = 0, /*!< Progressive */ 00495 VDC5_RES_INTER_INTERLACE = 1 /*!< Interlace */ 00496 } vdc5_res_inter_t ; 00497 /*! Writing rate */ 00498 typedef enum { 00499 VDC5_RES_FS_RATE_PER1 = 0, /* 1/1 an input signal */ 00500 VDC5_RES_FS_RATE_PER2, /* 1/2 an input signal */ 00501 VDC5_RES_FS_RATE_PER4, /* 1/4 an input signal */ 00502 VDC5_RES_FS_RATE_PER8, /* 1/8 an input signal */ 00503 VDC5_RES_FS_RATE_NUM 00504 } vdc5_res_fs_rate_t ; 00505 /*! Write field select */ 00506 typedef enum { 00507 VDC5_RES_FLD_SEL_TOP = 0, /*!< Top field */ 00508 VDC5_RES_FLD_SEL_BOTTOM = 1 /*!< Bottom field */ 00509 } vdc5_res_fld_sel_t ; 00510 /*! Data write control parameter */ 00511 typedef struct { 00512 vdc5_scalingdown_rot_t scalingdown_rot ;/*!< Scaling-down and rotation parameter */ 00513 vdc5_wr_rd_swa_t res_wrswa ; /*!< Swap setting in frame buffer writing */ 00514 vdc5_res_md_t res_md ; /*!< Frame buffer video-signal writing format */ 00515 vdc5_bst_md_t res_bst_md ; /*!< Transfer burst length for frame buffer writing */ 00516 vdc5_res_inter_t res_inter ; /*!< Field operating mode select */ 00517 vdc5_res_fs_rate_t res_fs_rate ; /*!< Writing rate */ 00518 vdc5_res_fld_sel_t res_fld_sel ; /*!< Write field select */ 00519 vdc5_onoff_t res_dth_on ; /*!< Dither correction on/off */ 00520 void * base ; /*!< Frame buffer base address */ 00521 uint32_t ln_off ; /*!< Frame buffer line offset address [byte] */ 00522 uint32_t flm_num ; /*!< Number of frames of buffer to be written to (res_flm_num + 1) */ 00523 uint32_t flm_off ; /*!< Frame buffer frame offset address [byte] */ 00524 void * btm_base ; /*!< Frame buffer base address for bottom */ 00525 } vdc5_write_t ; 00526 00527 /*********************** For R_VDC5_ChangeWriteProcess ***********************/ 00528 /*! Data write change parameter */ 00529 typedef struct { 00530 vdc5_scalingdown_rot_t scalingdown_rot ;/*!< Scaling-down and rotation parameter */ 00531 } vdc5_write_chg_t ; 00532 00533 /*********************** For R_VDC5_ReadDataControl ***********************/ 00534 /*! Line offset address direction of the frame buffer */ 00535 typedef enum { 00536 VDC5_GR_LN_OFF_DIR_INC = 0, /*!< Increments the address by the line offset address */ 00537 VDC5_GR_LN_OFF_DIR_DEC /*!< Decrements the address by the line offset address */ 00538 } vdc5_gr_ln_off_dir_t ; 00539 /*! Frame buffer address setting signal */ 00540 typedef enum { 00541 VDC5_GR_FLM_SEL_SCALE_DOWN = 0, /*!< Links to scaling-down process */ 00542 VDC5_GR_FLM_SEL_FLM_NUM , /*!< Selects frame 0 (graphics display) */ 00543 VDC5_GR_FLM_SEL_DISTORTION , /*!< Links to distortion correction */ 00544 VDC5_GR_FLM_SEL_POINTER_BUFF , /*!< Links to pointer buffer */ 00545 VDC5_GR_FLM_SEL_NUM 00546 } vdc5_gr_flm_sel_t ; 00547 /*! Size of the frame buffer to be read */ 00548 typedef struct { 00549 uint16_t in_vw ; /*!< Number of lines in a frame */ 00550 uint16_t in_hw ; /*!< Width of the horizontal valid period */ 00551 } vdc5_width_read_fb_t ; 00552 /*! Format of the frame buffer read signal */ 00553 typedef enum { 00554 VDC5_GR_FORMAT_RGB565 = 0, /*!< RGB565 */ 00555 VDC5_GR_FORMAT_RGB888 , /*!< RGB888 */ 00556 VDC5_GR_FORMAT_ARGB1555 , /*!< ARGB1555 */ 00557 VDC5_GR_FORMAT_ARGB4444 , /*!< ARGB4444 */ 00558 VDC5_GR_FORMAT_ARGB8888 , /*!< ARGB8888 */ 00559 VDC5_GR_FORMAT_CLUT8 , /*!< CLUT8 */ 00560 VDC5_GR_FORMAT_CLUT4 , /*!< CLUT4 */ 00561 VDC5_GR_FORMAT_CLUT1 , /*!< CLUT1 */ 00562 VDC5_GR_FORMAT_YCBCR422 , /*!< YCbCr422: This setting is prohibited for the graphics 2 and 3 */ 00563 VDC5_GR_FORMAT_YCBCR444 , /*!< YCbCr444: This setting is prohibited for the graphics 2 and 3 */ 00564 VDC5_GR_FORMAT_RGBA5551 , /*!< RGBA5551 */ 00565 VDC5_GR_FORMAT_RGBA8888 , /*!< RGBA8888 */ 00566 VDC5_GR_FORMAT_NUM /*!< The number of signal formats */ 00567 } vdc5_gr_format_t ; 00568 /*! Swapping of data read from buffer in the YCbCr422 format */ 00569 typedef enum { 00570 VDC5_GR_YCCSWAP_CBY0CRY1 = 0, 00571 VDC5_GR_YCCSWAP_Y0CBY1CR, 00572 VDC5_GR_YCCSWAP_CRY0CBY1, 00573 VDC5_GR_YCCSWAP_Y0CRY1CB, 00574 VDC5_GR_YCCSWAP_Y1CRY0CB, 00575 VDC5_GR_YCCSWAP_CRY1CBY0, 00576 VDC5_GR_YCCSWAP_Y1CBY0CR, 00577 VDC5_GR_YCCSWAP_CBY1CRY0, 00578 VDC5_GR_YCCSWAP_NUM 00579 } vdc5_gr_ycc_swap_t ; 00580 /*! Data read control parameter */ 00581 typedef struct { 00582 vdc5_gr_ln_off_dir_t gr_ln_off_dir ; /*!< Line offset address direction of the frame buffer */ 00583 vdc5_gr_flm_sel_t gr_flm_sel ; /*!< Frame buffer address setting signal */ 00584 vdc5_onoff_t gr_imr_flm_inv ; /*!< Frame buffer number for distortion correction */ 00585 vdc5_bst_md_t gr_bst_md ; /*!< Frame buffer burst transfer mode */ 00586 void * gr_base ; /*!< Frame buffer base address */ 00587 uint32_t gr_ln_off ; /*!< Frame buffer line offset address */ 00588 const vdc5_width_read_fb_t * width_read_fb ; /*!< Size of the frame buffer to be read */ 00589 vdc5_onoff_t adj_sel ; /*!< Folding handling (on/off) */ 00590 vdc5_gr_format_t gr_format ; /*!< Format of the frame buffer read signal */ 00591 vdc5_gr_ycc_swap_t gr_ycc_swap; /*!< Swapping of data read from buffer 00592 in the YCbCr422 format */ 00593 vdc5_wr_rd_swa_t gr_rdswa ; /*!< Swap setting in frame buffer reading */ 00594 vdc5_period_rect_t gr_grc ; /*!< Graphics display area */ 00595 } vdc5_read_t ; 00596 00597 /******************************* For R_VDC5_ChangeReadProcess *******************************/ 00598 /*! The type of graphics display modes */ 00599 typedef enum { 00600 VDC5_DISPSEL_IGNORED = -1, /*!< Ignored */ 00601 VDC5_DISPSEL_BACK = 0, /*!< Background color display */ 00602 VDC5_DISPSEL_LOWER = 1, /*!< Lower-layer graphics display */ 00603 VDC5_DISPSEL_CURRENT = 2, /*!< Current graphics display */ 00604 VDC5_DISPSEL_BLEND = 3, /*!< Blended display of lower-layer graphics and current graphics */ 00605 VDC5_DISPSEL_NUM = 4 /*!< The number of graphics display modes */ 00606 } vdc5_gr_disp_sel_t ; 00607 /*! Data read change parameter */ 00608 typedef struct { 00609 void * gr_base ; /*!< Frame buffer base address */ 00610 const vdc5_width_read_fb_t * width_read_fb ; /*!< Size of the frame buffer to be read */ 00611 const vdc5_period_rect_t * gr_grc ; /*!< Graphics display area */ 00612 const vdc5_gr_disp_sel_t * gr_disp_sel ; /*!< Graphics display mode */ 00613 } vdc5_read_chg_t ; 00614 00615 /******************************* For R_VDC5_StartProcess *******************************/ 00616 /*! Data write/read start parameter */ 00617 typedef struct { 00618 const vdc5_gr_disp_sel_t * gr_disp_sel ; /*!< Graphics display mode */ 00619 } vdc5_start_t ; 00620 00621 /******************************* For R_VDC5_VideoNoiseReduction *******************************/ 00622 /*! TAP select */ 00623 typedef enum { 00624 VDC5_NR_TAPSEL_1 = 0, /*!< Adjacent pixel */ 00625 VDC5_NR_TAPSEL_2 , /*!< 2 adjacent pixels */ 00626 VDC5_NR_TAPSEL_3 , /*!< 3 adjacent pixels */ 00627 VDC5_NR_TAPSEL_4 , /*!< 4 adjacent pixels */ 00628 VDC5_NR_TAPSEL_NUM 00629 } vdc5_nr_tap_t ; 00630 /*! Noise reduction gain adjustment */ 00631 typedef enum { 00632 VDC5_NR_GAIN_1_2 = 0, /*!< 1/2 */ 00633 VDC5_NR_GAIN_1_4 , /*!< 1/4 */ 00634 VDC5_NR_GAIN_1_8 , /*!< 1/8 */ 00635 VDC5_NR_GAIN_1_16 , /*!< 1/16 */ 00636 VDC5_NR_GAIN_NUM 00637 } vdc5_nr_gain_t ; 00638 /*! Noise reduction parameter */ 00639 typedef struct { 00640 vdc5_nr_tap_t nr1d_tap ; /*!< TAP select */ 00641 uint32_t nr1d_th ; /*!< Maximum value of coring (absolute value) */ 00642 vdc5_nr_gain_t nr1d_gain ; /*!< Noise reduction gain adjustment */ 00643 } vdc5_nr_param_t ; 00644 /*! Noise reduction setup parameter */ 00645 typedef struct { 00646 vdc5_nr_param_t y ; /*!< Y/G signal noise reduction parameter */ 00647 vdc5_nr_param_t cb ; /*!< Cb/B signal noise reduction parameter */ 00648 vdc5_nr_param_t cr ; /*!< Cr/R signal noise reduction parameter */ 00649 } vdc5_noise_reduction_t ; 00650 00651 /******************************* For R_VDC5_ImageColorMatrix *******************************/ 00652 /*! Color matrix module */ 00653 typedef enum { 00654 VDC5_COLORMTX_IMGCNT = 0, /*!< Input Controller (input video signal) */ 00655 VDC5_COLORMTX_ADJ_0 , /*!< Image quality improver 0 (scaler 0 output) */ 00656 VDC5_COLORMTX_ADJ_1 , /*!< Image quality improver 1 (scaler 1 output) */ 00657 VDC5_COLORMTX_NUM 00658 } vdc5_colormtx_module_t ; 00659 /*! Operating mode */ 00660 typedef enum { 00661 VDC5_COLORMTX_GBR_GBR = 0, /*!< GBR to GBR */ 00662 VDC5_COLORMTX_GBR_YCBCR , /*!< GBR to YCbCr */ 00663 VDC5_COLORMTX_YCBCR_GBR , /*!< YCbCr to GBR */ 00664 VDC5_COLORMTX_YCBCR_YCBCR , /*!< YCbCr to YCbCr */ 00665 VDC5_COLORMTX_MODE_NUM /*!< The number of operating modes */ 00666 } vdc5_colormtx_mode_t ; 00667 /*! Color matrix offset (DC) adjustment */ 00668 typedef enum { 00669 VDC5_COLORMTX_OFFST_YG = 0, /*!< YG */ 00670 VDC5_COLORMTX_OFFST_B , /*!< B */ 00671 VDC5_COLORMTX_OFFST_R , /*!< R */ 00672 VDC5_COLORMTX_OFFST_NUM /*!< The number of the color matrix DC offset values */ 00673 } vdc5_colormtx_offset_t ; 00674 /*! Color matrix signal gain adjustment */ 00675 typedef enum { 00676 VDC5_COLORMTX_GAIN_GG = 0, /*!< GG */ 00677 VDC5_COLORMTX_GAIN_GB , /*!< GB */ 00678 VDC5_COLORMTX_GAIN_GR , /*!< GR */ 00679 VDC5_COLORMTX_GAIN_BG , /*!< BG */ 00680 VDC5_COLORMTX_GAIN_BB , /*!< BB */ 00681 VDC5_COLORMTX_GAIN_BR , /*!< BR */ 00682 VDC5_COLORMTX_GAIN_RG , /*!< RG */ 00683 VDC5_COLORMTX_GAIN_RB , /*!< RB */ 00684 VDC5_COLORMTX_GAIN_RR , /*!< RR */ 00685 VDC5_COLORMTX_GAIN_NUM /*!< The number of the color matrix gain values */ 00686 } vdc5_colormtx_gain_t ; 00687 /*! Color matrix setup parameter */ 00688 typedef struct { 00689 vdc5_colormtx_module_t module ; /*!< Color matrix module */ 00690 vdc5_colormtx_mode_t mtx_mode ; /*!< Operating mode */ 00691 uint16_t offset[VDC5_COLORMTX_OFFST_NUM ];/*!< Offset (DC) adjustment of Y/G, B, and R signal */ 00692 uint16_t gain[VDC5_COLORMTX_GAIN_NUM ]; /*!< GG, GB, GR, BG, BB, BR, RG, RB, and RR signal 00693 gain adjustment */ 00694 } vdc5_color_matrix_t ; 00695 00696 /******************************* For R_VDC5_ImageEnhancement *******************************/ 00697 /*! Image quality improver ID */ 00698 typedef enum { 00699 VDC5_IMG_IMPRV_0 = 0, /*!< Image quality improver 0 */ 00700 VDC5_IMG_IMPRV_1 , /*!< Image quality improver 1 */ 00701 VDC5_IMG_IMPRV_NUM /*!< The number of image quality improvers */ 00702 } vdc5_imgimprv_id_t ; 00703 00704 /*! Sharpness band */ 00705 typedef enum { 00706 VDC5_IMGENH_SHARP_H1 = 0, /*!< H1: Adjacent pixel used as reference */ 00707 VDC5_IMGENH_SHARP_H2 , /*!< H2: Second adjacent pixel used as reference */ 00708 VDC5_IMGENH_SHARP_H3 , /*!< H3: Third adjacent pixel used as reference */ 00709 VDC5_IMGENH_SHARP_NUM /*!< The number of horizontal sharpness bands */ 00710 } vdc5_img_enh_sh_t ; 00711 /*! Sharpness control parameter */ 00712 typedef struct { 00713 uint8_t shp_clip_o ; /*!< Sharpness correction value clipping (on the overshoot side) */ 00714 uint8_t shp_clip_u ; /*!< Sharpness correction value clipping (on the undershoot side) */ 00715 uint8_t shp_gain_o ; /*!< Sharpness edge amplitude value gain (on the overshoot side) */ 00716 uint8_t shp_gain_u ; /*!< Sharpness edge amplitude value gain (on the undershoot side) */ 00717 uint8_t shp_core ; /*!< Active sharpness range */ 00718 } vdc5_sharpness_ctrl_t ; 00719 /*! Sharpness setup parameter */ 00720 typedef struct { 00721 vdc5_onoff_t shp_h2_lpf_sel; /*!< LPF selection for folding prevention 00722 before H2 edge detection */ 00723 vdc5_sharpness_ctrl_t hrz_sharp[VDC5_IMGENH_SHARP_NUM ]; /*!< Sharpness control parameter (H1, H2, and H3) */ 00724 } vdc5_enhance_sharp_t ; 00725 /*! LTI band */ 00726 typedef enum { 00727 VDC5_IMGENH_LTI1 = 0, /*!< H2: Second adjacent pixel used as reference */ 00728 VDC5_IMGENH_LTI2 , /*!< H4: Fourth adjacent pixel used as reference */ 00729 VDC5_IMGENH_LTI_NUM /*!< The number of horizontal LTI bands */ 00730 } vdc5_img_enh_lti_t ; 00731 /*! Median filter reference pixel select */ 00732 typedef enum { 00733 VDC5_LTI_MDFIL_SEL_ADJ2 = 0, /*!< Second adjacent pixel selected as reference */ 00734 VDC5_LTI_MDFIL_SEL_ADJ1 /*!< Adjacent pixel selected as reference */ 00735 } vdc5_lti_mdfil_sel_t ; 00736 /*! LTI control parameter */ 00737 typedef struct { 00738 uint8_t lti_inc_zero ; /*!< Median filter LTI correction threshold */ 00739 uint8_t lti_gain ; /*!< LTI edge amplitude value gain */ 00740 uint8_t lti_core ; /*!< LTI coring (maximum core value of 255) */ 00741 } vdc5_lti_ctrl_t ; 00742 /*! Luminance Transient Improvement setup parameter */ 00743 typedef struct { 00744 vdc5_onoff_t lti_h2_lpf_sel; /*!< LPF selection for folding prevention 00745 before H2 edge detection */ 00746 vdc5_lti_mdfil_sel_t lti_h4_median_tap_sel ; /*!< Median filter reference pixel select */ 00747 vdc5_lti_ctrl_t lti[VDC5_IMGENH_LTI_NUM ]; /*!< LTI control parameter (H2 and H4) */ 00748 } vdc5_enhance_lti_t ; 00749 00750 /******************************* For R_VDC5_ImageBlackStretch *******************************/ 00751 /*! Black stretch setup parameter */ 00752 typedef struct { 00753 uint16_t bkstr_st ; /*!< Black stretch start point */ 00754 uint16_t bkstr_d ; /*!< Black stretch depth */ 00755 uint16_t bkstr_t1 ; /*!< Black stretch time constant (T1) */ 00756 uint16_t bkstr_t2 ; /*!< Black stretch time constant (T2) */ 00757 } vdc5_black_t ; 00758 00759 /******************************* For R_VDC5_AlphaBlending *******************************/ 00760 /*! Alpha signal of the ARGB1555/ARGB5551 format */ 00761 typedef struct { 00762 uint8_t gr_a0 ; /*!< Alpha signal when alpha is set to '0' */ 00763 uint8_t gr_a1 ; /*!< Alpha signal when alpha is set to '1' */ 00764 } vdc5_alpha_argb1555_t ; 00765 /*! Alpha blending in one-pixel units */ 00766 typedef struct { 00767 vdc5_onoff_t gr_acalc_md; /*!< Premultiplication processing at alpha blending 00768 in one-pixel units (on/off) */ 00769 } vdc5_alpha_pixel_t ; 00770 /*! Alpha blending setup parameter */ 00771 typedef struct { 00772 const vdc5_alpha_argb1555_t * alpha_1bit ; /*!< Alpha signal of the ARGB1555/ARGB5551 format */ 00773 const vdc5_alpha_pixel_t * alpha_pixel ;/*!< Premultiplication processing at alpha blending in one-pixel */ 00774 } vdc5_alpha_blending_t ; 00775 00776 /******************************* For R_VDC5_AlphaBlendingRect *******************************/ 00777 /*! Parameter for alpha blending in a rectangular area */ 00778 typedef struct { 00779 int16_t gr_arc_coef; /*!< Alpha coefficient for alpha blending in a rectangular area 00780 (-255 to 255) */ 00781 uint8_t gr_arc_rate ; /*!< Frame rate for alpha blending in a rectangular area (gr_arc_rate + 1) */ 00782 uint8_t gr_arc_def ; /*!< Initial alpha value for alpha blending in a rectangular area */ 00783 vdc5_onoff_t gr_arc_mul; /*!< Multiplication processing with current alpha at alpha blending 00784 in a rectangular area (on/off) */ 00785 } vdc5_alpha_rect_t ; 00786 /*! Selection of lower-layer plane in scaler */ 00787 typedef struct { 00788 vdc5_onoff_t gr_vin_scl_und_sel; /*!< Selection of lower-layer plane in scaler 00789 - VDC5_OFF: Selects graphics 0 as lower-layer graphics 00790 and graphics 1 as current graphics 00791 - VDC5_ON: Selects graphics 1 as lower-layer graphics 00792 and graphics 0 as current graphics */ 00793 } vdc5_scl_und_sel_t ; 00794 /*! Setup parameter for alpha blending in a rectangular area */ 00795 typedef struct { 00796 const vdc5_pd_disp_rect_t * gr_arc ; /*!< Rectangular area subjected to alpha blending */ 00797 const vdc5_alpha_rect_t * alpha_rect ; /*!< Parameter for alpha blending in a rectangular area */ 00798 const vdc5_scl_und_sel_t * scl_und_sel ;/*!< Selection of lower-layer plane in scaler */ 00799 } vdc5_alpha_blending_rect_t ; 00800 00801 /******************************* For R_VDC5_Chromakey *******************************/ 00802 /*! Chroma-key setup parameter */ 00803 typedef struct { 00804 uint32_t ck_color ; /*!< RGB/CLUT signal for RGB/CLUT-index chroma-key processing */ 00805 uint32_t rep_color ; /*!< Replaced ARGB signal after RGB/CLUT-index chroma-key processing */ 00806 uint8_t rep_alpha ; /*!< Replaced alpha signal after RGB-index chroma-key processing (in 8 bits) */ 00807 } vdc5_chromakey_t ; 00808 00809 /******************************* For R_VDC5_CLUT *******************************/ 00810 /*! CLUT setup parameter */ 00811 typedef struct { 00812 uint32_t color_num ; /*!< The number of colors in CLUT */ 00813 const uint32_t * clut ; /*!< Address of the area storing the CLUT data (in ARGB8888 format) */ 00814 } vdc5_clut_t ; 00815 00816 /******************************* For R_VDC5_DisplayCalibration *******************************/ 00817 /*! Correction circuit sequence control */ 00818 typedef enum { 00819 VDC5_CALIBR_ROUTE_BCG = 0, /*!< Brightness -> contrast -> gamma correction */ 00820 VDC5_CALIBR_ROUTE_GBC /*!< Gamma correction -> brightness -> contrast */ 00821 } vdc5_calibr_route_t ; 00822 /*! Brightness (DC) adjustment parameter */ 00823 typedef struct { 00824 uint16_t pbrt_g ; /*!< Brightness (DC) adjustment of G signal */ 00825 uint16_t pbrt_b ; /*!< Brightness (DC) adjustment of B signal */ 00826 uint16_t pbrt_r ; /*!< Brightness (DC) adjustment of R signal */ 00827 } vdc5_calibr_bright_t ; 00828 /*! Contrast (gain) adjustment parameter */ 00829 typedef struct { 00830 uint8_t cont_g ; /*!< Contrast (gain) adjustment of G signal */ 00831 uint8_t cont_b ; /*!< Contrast (gain) adjustment of B signal */ 00832 uint8_t cont_r ; /*!< Contrast (gain) adjustment of R signal */ 00833 } vdc5_calibr_contrast_t ; 00834 /*! Panel dither operation mode */ 00835 typedef enum { 00836 VDC5_PDTH_MD_TRU = 0, /*!< Truncate */ 00837 VDC5_PDTH_MD_RDOF , /*!< Round-off */ 00838 VDC5_PDTH_MD_2X2 , /*!< 2 x 2 pattern dither */ 00839 VDC5_PDTH_MD_RAND , /*!< Random pattern dither */ 00840 VDC5_PDTH_MD_NUM 00841 } vdc5_panel_dither_md_t ; 00842 /*! Panel dithering parameter */ 00843 typedef struct { 00844 vdc5_panel_dither_md_t pdth_sel ; /*!< Panel dither operation mode */ 00845 uint8_t pdth_pa ; /*!< Pattern value (A) of 2x2 pattern dither */ 00846 uint8_t pdth_pb ; /*!< Pattern value (B) of 2x2 pattern dither */ 00847 uint8_t pdth_pc ; /*!< Pattern value (C) of 2x2 pattern dither */ 00848 uint8_t pdth_pd ; /*!< Pattern value (D) of 2x2 pattern dither */ 00849 } vdc5_calibr_dither_t ; 00850 /*! Display calibration parameter */ 00851 typedef struct { 00852 vdc5_calibr_route_t route ; /*!< Correction circuit sequence control */ 00853 const vdc5_calibr_bright_t * bright ; /*!< Brightness (DC) adjustment parameter */ 00854 const vdc5_calibr_contrast_t * contrast ; /*!< Contrast (gain) adjustment parameter */ 00855 const vdc5_calibr_dither_t * panel_dither ; /*!< Panel dithering parameter */ 00856 } vdc5_disp_calibration_t ; 00857 00858 /******************************* For R_VDC5_GammaCorrection *******************************/ 00859 /*! Gamma correction setup parameter */ 00860 typedef struct { 00861 const uint16_t * gam_g_gain ; /*!< Gain adjustment of area 0 to 31 of G signal */ 00862 const uint8_t * gam_g_th ; /*!< Start threshold of area 1 to 31 of G signal */ 00863 const uint16_t * gam_b_gain ; /*!< Gain adjustment of area 0 to 31 of B signal */ 00864 const uint8_t * gam_b_th ; /*!< Start threshold of area 1 to 31 of B signal */ 00865 const uint16_t * gam_r_gain ; /*!< Gain adjustment of area 0 to 31 of R signal */ 00866 const uint8_t * gam_r_th ; /*!< Start threshold of area 1 to 31 of R signal */ 00867 } vdc5_gamma_correction_t ; 00868 00869 00870 /****************************************************************************** 00871 Exported global variables 00872 ******************************************************************************/ 00873 00874 /****************************************************************************** 00875 Exported global functions (to be accessed by other files) 00876 ******************************************************************************/ 00877 vdc5_error_t R_VDC5_Initialize( 00878 const vdc5_channel_t ch, 00879 const vdc5_init_t * const param, 00880 void (* const init_func)(uint32_t), 00881 const uint32_t user_num); 00882 vdc5_error_t R_VDC5_Terminate(const vdc5_channel_t ch, void (* const quit_func)(uint32_t), const uint32_t user_num); 00883 vdc5_error_t R_VDC5_VideoInput(const vdc5_channel_t ch, const vdc5_input_t * const param); 00884 vdc5_error_t R_VDC5_SyncControl(const vdc5_channel_t ch, const vdc5_sync_ctrl_t * const param); 00885 vdc5_error_t R_VDC5_DisplayOutput(const vdc5_channel_t ch, const vdc5_output_t * const param); 00886 vdc5_error_t R_VDC5_CallbackISR(const vdc5_channel_t ch, const vdc5_int_t * const param); 00887 vdc5_error_t R_VDC5_WriteDataControl( 00888 const vdc5_channel_t ch, 00889 const vdc5_layer_id_t layer_id, 00890 const vdc5_write_t * const param); 00891 vdc5_error_t R_VDC5_ChangeWriteProcess( 00892 const vdc5_channel_t ch, 00893 const vdc5_layer_id_t layer_id, 00894 const vdc5_write_chg_t * const param); 00895 vdc5_error_t R_VDC5_ReadDataControl( 00896 const vdc5_channel_t ch, 00897 const vdc5_layer_id_t layer_id, 00898 const vdc5_read_t * const param); 00899 vdc5_error_t R_VDC5_ChangeReadProcess( 00900 const vdc5_channel_t ch, 00901 const vdc5_layer_id_t layer_id, 00902 const vdc5_read_chg_t * const param); 00903 vdc5_error_t R_VDC5_StartProcess( 00904 const vdc5_channel_t ch, 00905 const vdc5_layer_id_t layer_id, 00906 const vdc5_start_t * const param); 00907 vdc5_error_t R_VDC5_StopProcess(const vdc5_channel_t ch, const vdc5_layer_id_t layer_id); 00908 vdc5_error_t R_VDC5_ReleaseDataControl(const vdc5_channel_t ch, const vdc5_layer_id_t layer_id); 00909 vdc5_error_t R_VDC5_VideoNoiseReduction( 00910 const vdc5_channel_t ch, 00911 const vdc5_onoff_t nr1d_on, 00912 const vdc5_noise_reduction_t * const param); 00913 vdc5_error_t R_VDC5_ImageColorMatrix(const vdc5_channel_t ch, const vdc5_color_matrix_t * const param); 00914 vdc5_error_t R_VDC5_ImageEnhancement( 00915 const vdc5_channel_t ch, 00916 const vdc5_imgimprv_id_t imgimprv_id, 00917 const vdc5_onoff_t shp_h_on, 00918 const vdc5_enhance_sharp_t * const sharp_param, 00919 const vdc5_onoff_t lti_h_on, 00920 const vdc5_enhance_lti_t * const lti_param, 00921 const vdc5_period_rect_t * const enh_area); 00922 vdc5_error_t R_VDC5_ImageBlackStretch( 00923 const vdc5_channel_t ch, 00924 const vdc5_imgimprv_id_t imgimprv_id, 00925 const vdc5_onoff_t bkstr_on, 00926 const vdc5_black_t * const param); 00927 vdc5_error_t R_VDC5_AlphaBlending( 00928 const vdc5_channel_t ch, 00929 const vdc5_layer_id_t layer_id, 00930 const vdc5_alpha_blending_t * const param); 00931 vdc5_error_t R_VDC5_AlphaBlendingRect( 00932 const vdc5_channel_t ch, 00933 const vdc5_layer_id_t layer_id, 00934 const vdc5_onoff_t gr_arc_on, 00935 const vdc5_alpha_blending_rect_t * const param); 00936 vdc5_error_t R_VDC5_Chromakey( 00937 const vdc5_channel_t ch, 00938 const vdc5_layer_id_t layer_id, 00939 const vdc5_onoff_t gr_ck_on, 00940 const vdc5_chromakey_t * const param); 00941 vdc5_error_t R_VDC5_CLUT(const vdc5_channel_t ch, const vdc5_layer_id_t layer_id, const vdc5_clut_t * const param); 00942 vdc5_error_t R_VDC5_DisplayCalibration(const vdc5_channel_t ch, const vdc5_disp_calibration_t * const param); 00943 vdc5_error_t R_VDC5_GammaCorrection( 00944 const vdc5_channel_t ch, 00945 const vdc5_onoff_t gam_on, 00946 const vdc5_gamma_correction_t * const param); 00947 00948 void (*R_VDC5_GetISR(const vdc5_channel_t ch, const vdc5_int_type_t type))(const uint32_t int_sense); 00949 00950 00951 #ifdef __cplusplus 00952 } 00953 #endif /* __cplusplus */ 00954 00955 00956 #endif /* R_VDC5_H */ 00957
Generated on Tue Jul 12 2022 15:08:46 by
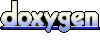