Video library for GR-PEACH
Dependents: Trace_Program2 GR-PEACH_Camera_in_barcode GR-PEACH_LCD_sample GR-PEACH_LCD_4_3inch_sample ... more
lcd_analog_rgb.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer 00021 * Copyright (C) 2012 - 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 /**************************************************************************//** 00024 * @file lcd_analog_rgb.h 00025 * @version 1.00 00026 * $Rev: 199 $ 00027 * $Date:: 2014-05-23 16:33:52 +0900#$ 00028 * @brief LCD panel definition header 00029 ******************************************************************************/ 00030 00031 #ifndef LCD_ANALOG_RGB_H 00032 #define LCD_ANALOG_RGB_H 00033 00034 /****************************************************************************** 00035 Includes <System Includes> , "Project Includes" 00036 ******************************************************************************/ 00037 #include <stdlib.h> 00038 00039 #include "r_typedefs.h" 00040 00041 #include "r_vdc5.h" 00042 00043 00044 /****************************************************************************** 00045 Macro definitions 00046 ******************************************************************************/ 00047 /* SVGA signal 800x600 00048 Pixel clock frequency: 40.0 MHz 00049 Refresh rate: 60.3 Hz 00050 Polarity of horizontal sync pulse: Positive 00051 Polarity of vertical sync pulse: Positive 00052 */ 00053 #define LCD_SVGA_H_VISIBLE_AREA (800u) /* Horizontal visible area [pixel] */ 00054 #define LCD_SVGA_H_FRONT_PORCH (40u) /* Horizontal front porch [pixel] */ 00055 #define LCD_SVGA_H_SYNC_WIDTH (128u) /* Horizontal sync pulse width [pixel] */ 00056 #define LCD_SVGA_H_BACK_PORCH (88u) /* Horizontal back porch [pixel] */ 00057 /* Horizontal total (one line) [pixel] */ 00058 #define LCD_SVGA_H_TOTAL (LCD_SVGA_H_VISIBLE_AREA +\ 00059 LCD_SVGA_H_FRONT_PORCH +\ 00060 LCD_SVGA_H_SYNC_WIDTH +\ 00061 LCD_SVGA_H_BACK_PORCH) 00062 #define LCD_SVGA_H_POLARITY (VDC5_SIG_POL_NOT_INVERTED) /* Polarity of horizontal sync pulse */ 00063 00064 #define LCD_SVGA_V_VISIBLE_AREA (600u) /* Vertical visible area [line] */ 00065 #define LCD_SVGA_V_FRONT_PORCH (1u) /* Vertical front porch [line] */ 00066 #define LCD_SVGA_V_SYNC_WIDTH (4u) /* Vertical sync pulse width [line] */ 00067 #define LCD_SVGA_V_BACK_PORCH (23u) /* Vertical back porch [line] */ 00068 /* Vertical total (one frame) [line] */ 00069 #define LCD_SVGA_V_TOTAL (LCD_SVGA_V_VISIBLE_AREA +\ 00070 LCD_SVGA_V_FRONT_PORCH +\ 00071 LCD_SVGA_V_SYNC_WIDTH +\ 00072 LCD_SVGA_V_BACK_PORCH) 00073 #define LCD_SVGA_V_POLARITY (VDC5_SIG_POL_NOT_INVERTED) /* Polarity of vertical sync pulse */ 00074 00075 00076 /* XGA signal 1024x768 00077 Pixel clock frequency: 65.0 MHz 00078 Refresh rate: 60.0 Hz 00079 Polarity of horizontal sync pulse: Negative 00080 Polarity of vertical sync pulse: Negative 00081 */ 00082 #define LCD_XGA_H_VISIBLE_AREA (1024u) /* Horizontal visible area [pixel] */ 00083 #define LCD_XGA_H_FRONT_PORCH (24u) /* Horizontal front porch [pixel] */ 00084 #define LCD_XGA_H_SYNC_WIDTH (136u) /* Horizontal sync pulse width [pixel] */ 00085 #define LCD_XGA_H_BACK_PORCH (160u) /* Horizontal back porch [pixel] */ 00086 /* Horizontal total (one line) [pixel] */ 00087 #define LCD_XGA_H_TOTAL (LCD_XGA_H_VISIBLE_AREA +\ 00088 LCD_XGA_H_FRONT_PORCH +\ 00089 LCD_XGA_H_SYNC_WIDTH +\ 00090 LCD_XGA_H_BACK_PORCH) 00091 #define LCD_XGA_H_POLARITY (VDC5_SIG_POL_INVERTED) /* Polarity of horizontal sync pulse */ 00092 00093 #define LCD_XGA_V_VISIBLE_AREA (768u) /* Vertical visible area [line] */ 00094 #define LCD_XGA_V_FRONT_PORCH (3u) /* Vertical front porch [line] */ 00095 #define LCD_XGA_V_SYNC_WIDTH (6u) /* Vertical sync pulse width [line] */ 00096 #define LCD_XGA_V_BACK_PORCH (29u) /* Vertical back porch [line] */ 00097 /* Vertical total (one frame) [line] */ 00098 #define LCD_XGA_V_TOTAL (LCD_XGA_V_VISIBLE_AREA +\ 00099 LCD_XGA_V_FRONT_PORCH +\ 00100 LCD_XGA_V_SYNC_WIDTH +\ 00101 LCD_XGA_V_BACK_PORCH) 00102 #define LCD_XGA_V_POLARITY (VDC5_SIG_POL_INVERTED) /* Polarity of vertical sync pulse */ 00103 00104 00105 /* VGA signal 640x480 00106 Pixel clock frequency: 25.175 MHz 00107 Refresh rate: 59.94 Hz 00108 Polarity of horizontal sync pulse: Negative 00109 Polarity of vertical sync pulse: Negative 00110 */ 00111 #define LCD_VGA_H_VISIBLE_AREA (640u) /* Horizontal visible area [pixel] */ 00112 #define LCD_VGA_H_FRONT_PORCH (16u) /* Horizontal front porch [pixel] */ 00113 #define LCD_VGA_H_SYNC_WIDTH (96u) /* Horizontal sync pulse width [pixel] */ 00114 #define LCD_VGA_H_BACK_PORCH (48u) /* Horizontal back porch [pixel] */ 00115 /* Horizontal total (one line) [pixel] */ 00116 #define LCD_VGA_H_TOTAL (LCD_VGA_H_VISIBLE_AREA +\ 00117 LCD_VGA_H_FRONT_PORCH +\ 00118 LCD_VGA_H_SYNC_WIDTH +\ 00119 LCD_VGA_H_BACK_PORCH) 00120 #define LCD_VGA_H_POLARITY (VDC5_SIG_POL_INVERTED) /* Polarity of horizontal sync pulse */ 00121 00122 #define LCD_VGA_V_VISIBLE_AREA (480u) /* Vertical visible area [line] */ 00123 #define LCD_VGA_V_FRONT_PORCH (10u) /* Vertical front porch [line] */ 00124 #define LCD_VGA_V_SYNC_WIDTH (2u) /* Vertical sync pulse width [line] */ 00125 #define LCD_VGA_V_BACK_PORCH (33u) /* Vertical back porch [line] */ 00126 /* Vertical total (one frame) [line] */ 00127 #define LCD_VGA_V_TOTAL (LCD_VGA_V_VISIBLE_AREA +\ 00128 LCD_VGA_V_FRONT_PORCH +\ 00129 LCD_VGA_V_SYNC_WIDTH +\ 00130 LCD_VGA_V_BACK_PORCH) 00131 #define LCD_VGA_V_POLARITY (VDC5_SIG_POL_INVERTED) /* Polarity of vertical sync pulse */ 00132 00133 00134 #endif /* LCD_ANALOG_RGB_H */
Generated on Tue Jul 12 2022 15:08:46 by
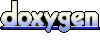