
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
STBTypedef.h
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #ifndef __STB_TYPEDEF_H__ 00018 #define __STB_TYPEDEF_H__ 00019 00020 00021 #ifndef VOID 00022 #define VOID void 00023 #endif /* VOID */ 00024 00025 typedef signed char STB_INT8 ; /* 8-bit signed integer */ 00026 typedef unsigned char STB_UINT8 ; /* 8-bit unsigned integer */ 00027 typedef signed short STB_INT16 ; /* 16-bit signed integer */ 00028 typedef unsigned short STB_UINT16 ; /* 16-bit unsigned integer */ 00029 typedef int STB_INT32 ; /* 32 bit signed integer */ 00030 typedef unsigned int STB_UINT32 ; /* 32 bit unsigned integer */ 00031 typedef float STB_FLOAT32 ; /* 32-bit floating point number */ 00032 typedef double STB_FLOAT64 ; /* 64-bit floating point number */ 00033 00034 00035 00036 /****************************************/ 00037 /* INPUT data strucrure to STBLib. */ 00038 /****************************************/ 00039 00040 typedef enum { 00041 STB_Expression_Neutral, 00042 STB_Expression_Happiness, 00043 STB_Expression_Surprise, 00044 STB_Expression_Anger, 00045 STB_Expression_Sadness, 00046 STB_Expression_Max 00047 } STB_OKAO_EXPRESSION; 00048 00049 typedef struct { 00050 STB_INT32 nX; 00051 STB_INT32 nY; 00052 } STB_POINT; 00053 00054 /* Face direction estimation */ 00055 typedef struct { 00056 STB_INT32 nLR; 00057 STB_INT32 nUD; 00058 STB_INT32 nRoll; 00059 STB_INT32 nConfidence; 00060 } STB_FRAME_RESULT_DIRECTION; 00061 00062 /* Age estimation */ 00063 typedef struct { 00064 STB_INT32 nAge; 00065 STB_INT32 nConfidence; 00066 } STB_FRAME_RESULT_AGE; 00067 00068 /* Gender estimation */ 00069 typedef struct { 00070 STB_INT32 nGender; 00071 STB_INT32 nConfidence; 00072 } STB_FRAME_RESULT_GENDER; 00073 00074 /* Gaze estimation */ 00075 typedef struct { 00076 STB_INT32 nLR; 00077 STB_INT32 nUD; 00078 } STB_FRAME_RESULT_GAZE; 00079 00080 /* Blink estimation */ 00081 typedef struct { 00082 STB_INT32 nLeftEye; 00083 STB_INT32 nRightEye; 00084 } STB_FRAME_RESULT_BLINK; 00085 00086 /* Facial expression estimation */ 00087 typedef struct { 00088 STB_INT32 anScore[STB_Expression_Max]; 00089 STB_INT32 nDegree; 00090 } STB_FRAME_RESULT_EXPRESSION; 00091 00092 /* Face recognition */ 00093 typedef struct { 00094 STB_INT32 nUID; 00095 STB_INT32 nScore; 00096 } STB_FRAME_RESULT_RECOGNITION; 00097 00098 /* One detection result */ 00099 typedef struct { 00100 STB_POINT center; 00101 STB_INT32 nSize; 00102 STB_INT32 nConfidence ; 00103 } STB_FRAME_RESULT_DETECTION; 00104 00105 /* Face detection and post-processing result (1 person) */ 00106 typedef struct { 00107 STB_POINT center; 00108 STB_INT32 nSize; 00109 STB_INT32 nConfidence; 00110 STB_FRAME_RESULT_DIRECTION direction; 00111 STB_FRAME_RESULT_AGE age; 00112 STB_FRAME_RESULT_GENDER gender; 00113 STB_FRAME_RESULT_GAZE gaze; 00114 STB_FRAME_RESULT_BLINK blink; 00115 STB_FRAME_RESULT_EXPRESSION expression; 00116 STB_FRAME_RESULT_RECOGNITION recognition; 00117 } STB_FRAME_RESULT_FACE; 00118 00119 /* One human body detection result */ 00120 typedef struct { 00121 STB_INT32 nCount; 00122 STB_FRAME_RESULT_DETECTION body[35]; 00123 } STB_FRAME_RESULT_BODYS; 00124 00125 /* Face detection and post-processing result (1 frame) */ 00126 typedef struct { 00127 STB_INT32 nCount; 00128 STB_FRAME_RESULT_FACE face[35]; 00129 } STB_FRAME_RESULT_FACES; 00130 00131 /* FRAME result (1 frame) */ 00132 typedef struct { 00133 STB_FRAME_RESULT_BODYS bodys; 00134 STB_FRAME_RESULT_FACES faces; 00135 } STB_FRAME_RESULT; 00136 00137 00138 /****************************************/ 00139 /* OUTPUT data strucrure from STBLib. */ 00140 /****************************************/ 00141 00142 #define STB_CONF_NO_DATA -1 /* No confidence (No data or in the case of stabilization time) */ 00143 00144 typedef enum { 00145 STB_STATUS_NO_DATA = -1, /* No data : No data for the relevant person */ 00146 STB_STATUS_CALCULATING = 0, /* During stabilization : a number of data for relevant people aren't enough(a number of frames that relevant people are taken) */ 00147 STB_STATUS_COMPLETE = 1, /* Stabilization done : the frames which done stabilization */ 00148 STB_STATUS_FIXED = 2, /* Stabilization fixed : already stabilization done, the results is fixed */ 00149 } STB_STATUS; /* Status of stabilization */ 00150 00151 00152 /* Expression */ 00153 typedef enum { 00154 STB_EX_UNKNOWN = -1, 00155 STB_EX_NEUTRAL = 0, 00156 STB_EX_HAPPINESS, 00157 STB_EX_SURPRISE, 00158 STB_EX_ANGER, 00159 STB_EX_SADNESS, 00160 STB_EX_MAX 00161 }STB_EXPRESSION; 00162 00163 /* General purpose stabilization result structure */ 00164 typedef struct { 00165 STB_STATUS status; /* Stabilization status */ 00166 STB_INT32 conf; /* Stabilization confidence */ 00167 STB_INT32 value; 00168 } STB_RES; 00169 00170 /* Result of Gaze estimation */ 00171 typedef struct { 00172 STB_STATUS status; /* Stabilization status */ 00173 STB_INT32 conf; /* Stabilization confidence */ 00174 STB_INT32 UD; 00175 STB_INT32 LR; 00176 } STB_GAZE; 00177 00178 /* Result of Face direction estimation */ 00179 typedef struct { 00180 STB_STATUS status; /* Stabilization status */ 00181 STB_INT32 conf; /* Stabilization confidence */ 00182 STB_INT32 yaw; 00183 STB_INT32 pitch; 00184 STB_INT32 roll; 00185 } STB_DIR; 00186 00187 /* Result of Blink estimation */ 00188 typedef struct { 00189 STB_STATUS status; /* Stabilization status */ 00190 STB_INT32 ratioL; 00191 STB_INT32 ratioR; 00192 } STB_BLINK; 00193 00194 /* Detection position structure */ 00195 typedef struct { 00196 STB_UINT32 x; 00197 STB_UINT32 y; 00198 } STB_POS; 00199 00200 /* Face stabilization result structure */ 00201 typedef struct { 00202 STB_INT32 nDetectID; 00203 STB_INT32 nTrackingID; 00204 STB_POS center; 00205 STB_UINT32 nSize; 00206 STB_INT32 conf; 00207 STB_DIR direction; 00208 STB_RES age; 00209 STB_RES gender; 00210 STB_GAZE gaze; 00211 STB_BLINK blink; 00212 STB_RES expression; 00213 STB_RES recognition; 00214 } STB_FACE; 00215 00216 /* Human body stabilization result structure */ 00217 typedef struct { 00218 STB_INT32 nDetectID; 00219 STB_INT32 nTrackingID; 00220 STB_POS center; 00221 STB_UINT32 nSize; 00222 STB_INT32 conf; 00223 } STB_BODY; 00224 00225 00226 #endif /* __STB_TYPEDEF_H__ */ 00227
Generated on Fri Jul 15 2022 01:15:47 by
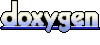