
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
STBFrValidValue.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #include "STBFrValidValue.h" 00018 00019 /*Value range check*/ 00020 #define IS_OUT_RANGE( val , min , max ) ( ( (val) < (min) ) || ( (max) < (val) ) ) 00021 #define IS_OUT_VALUE( val , min , max , accept ) ( IS_OUT_RANGE( val , min , max ) && ( (val) != (accept) ) ) 00022 #define IS_OUT_FR_UID( val , min , max , acceptA , acceptB , acceptC ) ( IS_OUT_RANGE( val , min , max ) && ( (val) != (acceptA) ) && ( (val) != (acceptB) ) && ( (val) != (acceptC) ) ) 00023 #define IS_OUT_FR_SCORE( val , min , max , acceptA , acceptB ) ( IS_OUT_RANGE( val , min , max ) && ( (val) != (acceptA) ) && ( (val) != (acceptB) ) ) 00024 00025 /*------------------------------------------------------------------------------------------------------------------*/ 00026 /* STB_IsValidValue */ 00027 /*------------------------------------------------------------------------------------------------------------------*/ 00028 STB_INT32 STB_FrIsValidValue(const STB_FR_DET *input) 00029 { 00030 STB_INT32 i ; 00031 00032 for( i = 0 ; i < input->num ; i++) 00033 { 00034 00035 if( IS_OUT_VALUE( input->fcDet[i].dirDetYaw , STB_FACE_DIR_LR_MIN , STB_FACE_DIR_LR_MAX , STB_ERR_DIR_CANNOT ) ){ return STB_FALSE;} 00036 if( IS_OUT_VALUE( input->fcDet[i].dirDetPitch , STB_FACE_DIR_UD_MIN , STB_FACE_DIR_UD_MAX , STB_ERR_DIR_CANNOT ) ){ return STB_FALSE;} 00037 if( IS_OUT_VALUE( input->fcDet[i].dirDetRoll , STB_FACE_DIR_ROLL_MIN , STB_FACE_DIR_ROLL_MAX , STB_ERR_DIR_CANNOT ) ){ return STB_FALSE;} 00038 if( IS_OUT_VALUE( input->fcDet[i].dirDetConf , STB_FACE_DIR_CONF_MIN , STB_FACE_DIR_CONF_MAX , STB_ERR_PE_CANNOT ) ){ return STB_FALSE;} 00039 00040 } 00041 00042 for( i = 0 ; i < input->num ; i++) 00043 { 00044 if( IS_OUT_FR_UID( input->fcDet[i].frDetID , STB_FACE_FR_UID_MIN , STB_FACE_FR_UID_MAX ,STB_ERR_FR_CANNOT ,STB_ERR_FR_NOID ,STB_ERR_FR_NOALBUM ) ){ return STB_FALSE;} 00045 if( IS_OUT_FR_SCORE( input->fcDet[i].frDetConf , STB_FACE_FR_SCORE_MIN , STB_FACE_FR_SCORE_MAX ,STB_ERR_FR_CANNOT ,STB_ERR_FR_NOALBUM) ){ return STB_FALSE;} 00046 } 00047 00048 00049 00050 return STB_TRUE; 00051 }
Generated on Fri Jul 15 2022 01:15:47 by
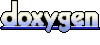