
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
PeInterface.h
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #if !defined( _INTERFACE_H_ ) 00018 #define _INTERFACE_H_ 00019 #include "STBPeTypedef.h" 00020 #include "STBPeValidValue.h" 00021 00022 00023 #ifdef __cplusplus 00024 extern "C" { 00025 #endif 00026 00027 ////////////////////////////////////////////////////////////////////////////////// 00028 ////////////////////////////////////////////////////////////////////////////////// 00029 /////////// Define ////////////// 00030 ////////////////////////////////////////////////////////////////////////////////// 00031 ////////////////////////////////////////////////////////////////////////////////// 00032 00033 00034 #define STB_PE_BACK_MAX 20 /* refer to past "STB_BACK_MAX" frames of results */ 00035 #define STB_PE_EX_MAX 5 //A type of Facial expression 00036 00037 #define STB_PE_TRA_CNT_MAX 35 00038 00039 #define STB_PE_DIR_MIN_UD_INI -15 00040 #define STB_PE_DIR_MIN_UD_MIN -90 00041 #define STB_PE_DIR_MIN_UD_MAX 90 00042 00043 #define STB_PE_DIR_MAX_UD_INI 20 00044 #define STB_PE_DIR_MAX_UD_MIN -90 00045 #define STB_PE_DIR_MAX_UD_MAX 90 00046 00047 #define STB_PE_DIR_MIN_LR_INI -30 00048 #define STB_PE_DIR_MIN_LR_MIN -90 00049 #define STB_PE_DIR_MIN_LR_MAX 90 00050 00051 #define STB_PE_DIR_MAX_LR_INI 30 00052 #define STB_PE_DIR_MAX_LR_MIN -90 00053 #define STB_PE_DIR_MAX_LR_MAX 90 00054 00055 00056 #define STB_PE_FRAME_CNT_INI 5 00057 #define STB_PE_FRAME_CNT_MIN 1 00058 #define STB_PE_FRAME_CNT_MAX 20 00059 00060 #define STB_PE_DIR_THR_INI 300 00061 #define STB_PE_DIR_THR_MIN 0 00062 #define STB_PE_DIR_THR_MAX 1000 00063 00064 ////////////////////////////////////////////////////////////////////////////////// 00065 ////////////////////////////////////////////////////////////////////////////////// 00066 /////////// Struct ////////////// 00067 ////////////////////////////////////////////////////////////////////////////////// 00068 ////////////////////////////////////////////////////////////////////////////////// 00069 typedef struct tagPEHANDLE { 00070 00071 STB_INT8 *pePtr ; 00072 /* param */ 00073 STB_INT32 peCntMax ;//Maximum number of tracking people 00074 STB_INT32 peFaceDirUDMin ;//The face on top/down allowable range min. 00075 STB_INT32 peFaceDirUDMax ;//The face on top/down allowable range max. 00076 STB_INT32 peFaceDirLRMin ;//The face on left /right side allowable range min. 00077 STB_INT32 peFaceDirLRMax ;//The face on left /right side allowable range max. 00078 STB_INT32 peFaceDirThr ;//If the confidence of Face direction estimation doesn't exceed the reference value, the recognition result isn't trusted. 00079 STB_INT32 peFrameCount ; 00080 00081 /* PE_Face */ 00082 STB_PE_DET peDet ;//Present data before the stabilization(input). 00083 STB_PE_DET *peDetRec ;//past data before the stabilization 00084 STB_PE_RES peRes ;//present data after the stabilization(output) 00085 STBExecFlg *execFlg ; 00086 00087 } *PEHANDLE; 00088 00089 ////////////////////////////////////////////////////////////////////////////////// 00090 ////////////////////////////////////////////////////////////////////////////////// 00091 /////////// Func ////////////// 00092 ////////////////////////////////////////////////////////////////////////////////// 00093 ////////////////////////////////////////////////////////////////////////////////// 00094 PEHANDLE PeCreateHandle ( const STBExecFlg* execFlg ,const STB_INT32 nTraCntMax); 00095 STB_INT32 PeDeleteHandle ( PEHANDLE handle); 00096 STB_INT32 PeSetDetect ( PEHANDLE handle,const STB_PE_DET *stbPeDet); 00097 STB_INT32 PeExecute ( PEHANDLE handle); 00098 STB_INT32 PeGetResult ( PEHANDLE handle , STB_PE_RES* peResult); 00099 STB_INT32 PeSetFaceDirMinMax ( PEHANDLE handle , STB_INT32 nMinUDAngle , STB_INT32 nMaxUDAngle ,STB_INT32 nMinLRAngle , STB_INT32 nMaxLRAngle ); 00100 STB_INT32 PeGetFaceDirMinMax ( PEHANDLE handle , STB_INT32 *pnMinUDAngle, STB_INT32 *pnMaxUDAngle ,STB_INT32 *pnMinLRAngle, STB_INT32 *pnMaxLRAngle ); 00101 STB_INT32 PeClear ( PEHANDLE handle ); 00102 STB_INT32 PeSetFaceDirThreshold ( PEHANDLE handle , STB_INT32 threshold ); 00103 STB_INT32 PeGetFaceDirThreshold ( PEHANDLE handle , STB_INT32* threshold ); 00104 STB_INT32 PeSetFrameCount ( PEHANDLE handle , STB_INT32 nFrameCount ); 00105 STB_INT32 PeGetFrameCount ( PEHANDLE handle , STB_INT32* nFrameCount ); 00106 00107 #ifdef __cplusplus 00108 } 00109 #endif 00110 00111 #endif
Generated on Fri Jul 15 2022 01:15:47 by
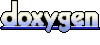