
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
Interface.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 #include "Interface.h" 00018 #include "STBValidValue.h" 00019 #include "STBCommonDef.h" 00020 #include "STBTracking.h" 00021 #include "STBFaceInfo.h" 00022 #include "STBMakeResult.h" 00023 00024 00025 /*Value range check*/ 00026 #define ISVALID_RANGE( val , min , max ) ( ( (min) <= (val) ) && ( (val) <= (max) ) ) 00027 00028 00029 /*------------------------------------------------------------------------------------------------------------------*/ 00030 /*IsValidValue : error check*/ 00031 /*------------------------------------------------------------------------------------------------------------------*/ 00032 static STB_INT32 IsValidValue( 00033 const STB_INT32 nValue , 00034 const STB_INT32 nLimitMin , 00035 const STB_INT32 nLimitMax ) 00036 { 00037 STB_INT32 nRet; 00038 for( nRet = STB_ERR_INVALIDPARAM; nRet != STB_NORMAL; nRet = STB_NORMAL ) 00039 { 00040 if( ! ISVALID_RANGE( nValue , nLimitMin , nLimitMax ) ) 00041 { 00042 break; 00043 } 00044 } 00045 return nRet; 00046 } 00047 /*------------------------------------------------------------------------------------------------------------------*/ 00048 /* IsValidPointer */ 00049 /*------------------------------------------------------------------------------------------------------------------*/ 00050 static STB_INT32 IsValidPointer( const VOID* pPointer ) 00051 { 00052 STB_INT32 nRet; 00053 for( nRet = STB_ERR_INVALIDPARAM; nRet != STB_NORMAL; nRet = STB_NORMAL ) 00054 { 00055 if( NULL == pPointer ){ break; } 00056 } 00057 return nRet; 00058 } 00059 00060 /*------------------------------------------------------------------------------------------------------------------*/ 00061 /* GetVersion */ 00062 /* Interface between SDK layer and functional layer */ 00063 /* Responsible for error check and function call */ 00064 /*------------------------------------------------------------------------------------------------------------------*/ 00065 STB_INT32 GetVersion( STB_INT8* pnMajorVersion , STB_INT8* pnMinorVersion ){ 00066 STB_INT32 nRet; 00067 00068 for( nRet = STB_ERR_INVALIDPARAM; nRet != STB_NORMAL; nRet = STB_NORMAL ) 00069 { 00070 nRet = IsValidPointer( pnMajorVersion ); 00071 if( STB_NORMAL != nRet ) 00072 { 00073 break; 00074 } 00075 nRet = IsValidPointer( pnMinorVersion ); 00076 if( STB_NORMAL != nRet ) 00077 { 00078 break; 00079 } 00080 *pnMajorVersion = VERSION_MAJOR; 00081 *pnMinorVersion = VERSION_MINOR; 00082 } 00083 00084 return nRet; 00085 } 00086 /*------------------------------------------------------------------------------------------------------------------*/ 00087 /* CalcStbSize */ 00088 /*------------------------------------------------------------------------------------------------------------------*/ 00089 STB_UINT32 CalcStbSize ( STBExecFlg *execFlg , STB_UINT32 nTraCntMax) 00090 { 00091 STB_UINT32 retVal ; 00092 00093 retVal = 0 ; 00094 00095 retVal += 100 ;///Margin : alignment 00096 00097 00098 00099 00100 retVal += sizeof( STB_TR_DET );//wSrcTr 00101 if( execFlg->bodyTr == STB_TRUE ) 00102 { 00103 retVal += sizeof( TraObj ) * nTraCntMax ; // trBody 00104 retVal += sizeof( ROI_DET ) * nTraCntMax ; // wSrcTr->bdDet 00105 retVal += sizeof( STB_TR_RES_BODYS ) ; // wDstTrBody 00106 retVal += sizeof( STB_TR_RES ) * nTraCntMax ; // wDstTrBody->body 00107 } 00108 if( execFlg->faceTr == STB_TRUE ) 00109 { 00110 retVal += sizeof( TraObj ) * nTraCntMax ; // trFace 00111 retVal += sizeof( ROI_DET ) * nTraCntMax ; // wSrcTr->fcDet 00112 retVal += sizeof( STB_TR_RES_FACES ) ; // wDstTrFace 00113 retVal += sizeof( STB_TR_RES ) * nTraCntMax ; // wDstTrFace->face 00114 } 00115 if( execFlg->gen == STB_TRUE 00116 || execFlg->age == STB_TRUE 00117 || execFlg->fr == STB_TRUE 00118 || execFlg->exp == STB_TRUE 00119 || execFlg->dir == STB_TRUE 00120 || execFlg->gaz == STB_TRUE 00121 || execFlg->bli == STB_TRUE 00122 ) 00123 { 00124 retVal += sizeof( FaceObj ) * nTraCntMax ; // infoFace 00125 } 00126 00127 00128 if( execFlg->gen == STB_TRUE 00129 || execFlg->age == STB_TRUE 00130 //|| execFlg->fr == STB_TRUE 00131 || execFlg->exp == STB_TRUE 00132 || execFlg->dir == STB_TRUE 00133 || execFlg->gaz == STB_TRUE 00134 || execFlg->bli == STB_TRUE 00135 ) 00136 { 00137 retVal += sizeof( STB_PE_DET ) ; // wSrcPe 00138 retVal += sizeof( FACE_DET ) * nTraCntMax ; // wSrcPe->fcDet 00139 retVal += sizeof( STB_PE_RES ) ; // wDstPe 00140 retVal += sizeof( STB_PE_FACE ) * nTraCntMax ; // wDstPe->peFace 00141 00142 } 00143 00144 if( execFlg->fr == STB_TRUE ) 00145 { 00146 retVal += sizeof( STB_FR_DET ) ; // wSrcFr 00147 retVal += sizeof( FR_DET ) * nTraCntMax ; // wSrcFr->fcDet 00148 retVal += sizeof( STB_FR_RES ) ; // wDstFr 00149 retVal += sizeof( FR_RES ) * nTraCntMax ; // wDstFr->frFace 00150 } 00151 00152 00153 return retVal; 00154 } 00155 /*------------------------------------------------------------------------------------------------------------------*/ 00156 /* ShareStbSize */ 00157 /*------------------------------------------------------------------------------------------------------------------*/ 00158 void ShareStbSize ( STBHANDLE handle , STB_INT8 *stbPtr ) 00159 { 00160 STB_UINT32 nTraCntMax; 00161 00162 nTraCntMax = handle->nTraCntMax; 00163 00164 00165 handle->wSrcTr = (STB_TR_DET*)stbPtr; stbPtr += ( sizeof( STB_TR_DET ) ); 00166 if( handle->execFlg->bodyTr == STB_TRUE ) 00167 { 00168 handle->trBody = ( TraObj* ) stbPtr; stbPtr += ( sizeof( TraObj ) * nTraCntMax ); 00169 handle->wSrcTr->bdDet = ( ROI_DET* ) stbPtr; stbPtr += ( sizeof( ROI_DET ) * nTraCntMax ); 00170 handle->wDstTrBody = ( STB_TR_RES_BODYS* ) stbPtr; stbPtr += ( sizeof( STB_TR_RES_BODYS )); 00171 handle->wDstTrBody->body = ( STB_TR_RES* ) stbPtr; stbPtr += ( sizeof( STB_TR_RES ) * nTraCntMax ); 00172 } 00173 if( handle->execFlg->faceTr == STB_TRUE ) 00174 { 00175 handle->trFace = ( TraObj* ) stbPtr; stbPtr += ( sizeof( TraObj ) * nTraCntMax ); 00176 handle->wSrcTr->fcDet = ( ROI_DET* ) stbPtr; stbPtr += ( sizeof( ROI_DET ) * nTraCntMax ); 00177 handle->wDstTrFace = ( STB_TR_RES_FACES* ) stbPtr; stbPtr += ( sizeof( STB_TR_RES_FACES )); 00178 handle->wDstTrFace->face = ( STB_TR_RES* ) stbPtr; stbPtr += ( sizeof( STB_TR_RES ) * nTraCntMax ); 00179 } 00180 00181 if( handle->execFlg->gen == STB_TRUE 00182 || handle->execFlg->age == STB_TRUE 00183 || handle->execFlg->fr == STB_TRUE 00184 || handle->execFlg->exp == STB_TRUE 00185 || handle->execFlg->dir == STB_TRUE 00186 || handle->execFlg->gaz == STB_TRUE 00187 || handle->execFlg->bli == STB_TRUE 00188 ) 00189 { 00190 handle->infoFace = ( FaceObj* ) stbPtr; stbPtr += ( sizeof( FaceObj ) * nTraCntMax ); 00191 } 00192 00193 if( handle->execFlg->gen == STB_TRUE 00194 || handle->execFlg->age == STB_TRUE 00195 //||| handle->execFlg->fr == STB_TRUE 00196 || handle->execFlg->exp == STB_TRUE 00197 || handle->execFlg->dir == STB_TRUE 00198 || handle->execFlg->gaz == STB_TRUE 00199 || handle->execFlg->bli == STB_TRUE 00200 ) 00201 { 00202 handle->wSrcPe = ( STB_PE_DET* ) stbPtr; stbPtr += ( sizeof( STB_PE_DET ) ); 00203 handle->wSrcPe->fcDet = ( FACE_DET* ) stbPtr; stbPtr += ( sizeof( FACE_DET ) * nTraCntMax ); 00204 handle->wDstPe = ( STB_PE_RES* ) stbPtr; stbPtr += ( sizeof( STB_PE_RES ) ); 00205 handle->wDstPe->peFace = ( STB_PE_FACE*) stbPtr; stbPtr += ( sizeof( STB_PE_FACE) * nTraCntMax ); 00206 } 00207 00208 if( handle->execFlg->fr == STB_TRUE ) 00209 { 00210 handle->wSrcFr = ( STB_FR_DET* ) stbPtr; stbPtr += ( sizeof( STB_FR_DET ) ); 00211 handle->wSrcFr->fcDet = ( FR_DET* ) stbPtr; stbPtr += ( sizeof( FR_DET ) * nTraCntMax ); 00212 handle->wDstFr = ( STB_FR_RES* ) stbPtr; stbPtr += ( sizeof( STB_FR_RES ) ); 00213 handle->wDstFr->frFace = ( FR_RES* ) stbPtr; stbPtr += ( sizeof( FR_RES ) * nTraCntMax ); 00214 } 00215 00216 00217 } 00218 /*------------------------------------------------------------------------------------------------------------------*/ 00219 /*Create handle*/ 00220 /*------------------------------------------------------------------------------------------------------------------*/ 00221 STBHANDLE CreateHandle ( STB_UINT32 stbExecFlg ) 00222 { 00223 00224 STBHANDLE handle; 00225 STB_UINT32 tmpVal; 00226 STB_UINT32 tmpFLG; 00227 00228 00229 00230 /*do STB handle's malloc here*/ 00231 handle = NULL ; 00232 handle = ( STBHANDLE )malloc( sizeof( *handle ) ); 00233 if( handle == NULL ) 00234 { 00235 return NULL;/* FAIL : Create STB handle */ 00236 } 00237 00238 /* ExecFlg */ 00239 handle->execFlg = ( STBExecFlg* )malloc( sizeof( STBExecFlg ) ); 00240 if( handle->execFlg == NULL ) 00241 { 00242 free ( handle ) ;/*Free of Malloc things at the present time*/ 00243 return NULL ;/* FAIL : Create STB handle ExecFlg */ 00244 } 00245 if( ( stbExecFlg & STB_FUNC_BD )== STB_FUNC_BD ){ handle->execFlg->bodyTr = STB_TRUE ;}else{ handle->execFlg->bodyTr = STB_FALSE ;} 00246 if( ( stbExecFlg & STB_FUNC_DT )== STB_FUNC_DT ){ handle->execFlg->faceTr = STB_TRUE ;}else{ handle->execFlg->faceTr = STB_FALSE ;} 00247 if( ( stbExecFlg & STB_FUNC_PT )== STB_FUNC_PT ){ handle->execFlg->dir = STB_TRUE ;}else{ handle->execFlg->dir = STB_FALSE ;} 00248 if( ( stbExecFlg & STB_FUNC_AG )== STB_FUNC_AG ){ handle->execFlg->age = STB_TRUE ;}else{ handle->execFlg->age = STB_FALSE ;} 00249 if( ( stbExecFlg & STB_FUNC_GN )== STB_FUNC_GN ){ handle->execFlg->gen = STB_TRUE ;}else{ handle->execFlg->gen = STB_FALSE ;} 00250 if( ( stbExecFlg & STB_FUNC_GZ )== STB_FUNC_GZ ){ handle->execFlg->gaz = STB_TRUE ;}else{ handle->execFlg->gaz = STB_FALSE ;} 00251 if( ( stbExecFlg & STB_FUNC_BL )== STB_FUNC_BL ){ handle->execFlg->bli = STB_TRUE ;}else{ handle->execFlg->bli = STB_FALSE ;} 00252 if( ( stbExecFlg & STB_FUNC_EX )== STB_FUNC_EX ){ handle->execFlg->exp = STB_TRUE ;}else{ handle->execFlg->exp = STB_FALSE ;} 00253 if( ( stbExecFlg & STB_FUNC_FR )== STB_FUNC_FR ){ handle->execFlg->fr = STB_TRUE ;}else{ handle->execFlg->fr = STB_FALSE ;} 00254 handle->execFlg->pet = STB_FALSE ; 00255 handle->execFlg->hand = STB_FALSE ; 00256 00257 00258 if( handle->execFlg->faceTr == STB_FALSE ) 00259 { 00260 if( handle->execFlg->gen == STB_TRUE 00261 || handle->execFlg->age == STB_TRUE 00262 || handle->execFlg->fr == STB_TRUE 00263 || handle->execFlg->exp == STB_TRUE 00264 || handle->execFlg->dir == STB_TRUE 00265 || handle->execFlg->gaz == STB_TRUE 00266 || handle->execFlg->bli == STB_TRUE 00267 ) 00268 { 00269 free ( handle->execFlg );/*Free of Malloc things at the present time*/ 00270 free ( handle );/*Free of Malloc things at the present time*/ 00271 return NULL ;/*Invalid input parameter stbExecFlg*/ 00272 } 00273 } 00274 00275 00276 /*Setting the initial value here.*/ 00277 handle->nTraCntBody = 0; 00278 handle->nTraCntFace = 0; 00279 handle->nDetCntMax = DETECT_CNT_MAX ;/*A maximum number of detected(input) people*/ 00280 handle->nTraCntMax = TRACK_CNT_MAX ;/*A maximum number of tracking(output) people*/ 00281 handle->nExecuted = STB_FALSE ; 00282 handle->nInitialized= STB_FALSE ; 00283 handle->nDetCntBody = 0; 00284 handle->nDetCntFace = 0; 00285 handle->trFace = NULL; 00286 handle->trBody = NULL; 00287 handle->infoFace = NULL; 00288 handle->wSrcTr = NULL; 00289 handle->wDstTrFace = NULL; 00290 handle->wDstTrBody = NULL; 00291 handle->wSrcPe = NULL; 00292 handle->wDstPe = NULL; 00293 handle->wSrcFr = NULL; 00294 handle->wDstFr = NULL; 00295 00296 00297 /* Do Malloc to things that need Malloc in STB handle */ 00298 handle->stbPtr = NULL ; 00299 handle->hTrHandle = NULL ; 00300 handle->hPeHandle = NULL ; 00301 handle->hFrHandle = NULL ; 00302 tmpVal = CalcStbSize ( handle->execFlg ,handle->nTraCntMax ); /*calculate necessary amount in the STB handle*/ 00303 handle->stbPtr = ( STB_INT8 * )malloc( tmpVal ) ; /*keep necessary amount in the STB handle*/ 00304 if( handle->stbPtr == NULL ) 00305 { 00306 free ( handle->execFlg );/*Free of Malloc things at the present time*/ 00307 free ( handle );/*Free of Malloc things at the present time*/ 00308 return NULL ; 00309 } 00310 ShareStbSize ( handle, handle->stbPtr); /* Malloc-area is allocated to things that need Malloc in STB handle */ 00311 00312 /*Create handles for child functions*/ 00313 tmpFLG = STB_TRUE; 00314 if( handle->execFlg->bodyTr == STB_TRUE 00315 || handle->execFlg->faceTr == STB_TRUE 00316 ) 00317 { 00318 handle->hTrHandle = STB_Tr_CreateHandle( handle->execFlg ,handle->nDetCntMax, handle->nTraCntMax ); 00319 if( handle->hTrHandle == NULL ){ tmpFLG = STB_FALSE; } 00320 } 00321 if( handle->execFlg->gen == STB_TRUE 00322 || handle->execFlg->age == STB_TRUE 00323 //|| handle->execFlg->fr == STB_TRUE 00324 || handle->execFlg->exp == STB_TRUE 00325 || handle->execFlg->dir == STB_TRUE 00326 || handle->execFlg->gaz == STB_TRUE 00327 || handle->execFlg->bli == STB_TRUE 00328 ) 00329 { 00330 handle->hPeHandle = STB_Pe_CreateHandle( handle->execFlg ,handle->nTraCntMax ); 00331 if( handle->hPeHandle == NULL ){ tmpFLG = STB_FALSE; } 00332 } 00333 if( handle->execFlg->fr == STB_TRUE ) 00334 { 00335 handle->hFrHandle = STB_Fr_CreateHandle( handle->nTraCntMax ); 00336 if( handle->hFrHandle == NULL ){ tmpFLG = STB_FALSE; } 00337 } 00338 00339 if( tmpFLG == STB_FALSE ) 00340 { 00341 /*When Malloc failed, Free of Malloc data at the present time*/ 00342 if( handle->hTrHandle != NULL ) { STB_Tr_DeleteHandle ( handle->hTrHandle ) ;} 00343 if( handle->hPeHandle != NULL ) { STB_Pe_DeleteHandle ( handle->hPeHandle ) ;} 00344 if( handle->hFrHandle != NULL ) { STB_Fr_DeleteHandle ( handle->hFrHandle ) ;} 00345 if( handle->stbPtr != NULL ) { free ( handle->stbPtr ) ;}/*Free of Malloc things at the present time*/ 00346 if( handle->execFlg != NULL ) { free ( handle->execFlg ) ;}/*Free of Malloc things at the present time*/ 00347 if( handle != NULL ) { free ( handle ) ;}/*Free of Malloc things at the present time*/ 00348 return NULL; 00349 } 00350 00351 return handle; 00352 } 00353 //------------------------------------------------------------------------------------------------------------------- 00354 // DeleteHandle /*Delete handle*/ 00355 //------------------------------------------------------------------------------------------------------------------- 00356 STB_INT32 DeleteHandle(STBHANDLE handle) 00357 { 00358 00359 STB_INT32 nRet; 00360 00361 /*NULL check*/ 00362 nRet = IsValidPointer(handle); 00363 if(nRet != STB_NORMAL){ 00364 return STB_ERR_NOHANDLE; 00365 } 00366 00367 /*Malloc things here, do free*/ 00368 if( handle->hTrHandle != NULL ) { STB_Tr_DeleteHandle ( handle->hTrHandle ) ;} 00369 if( handle->hPeHandle != NULL ) { STB_Pe_DeleteHandle ( handle->hPeHandle ) ;} 00370 if( handle->hFrHandle != NULL ) { STB_Fr_DeleteHandle ( handle->hFrHandle ) ;} 00371 if( handle->stbPtr != NULL ) { free ( handle->stbPtr ) ;} 00372 if( handle->execFlg != NULL ) { free ( handle->execFlg ) ;} 00373 if( handle != NULL ) { free ( handle ) ;} 00374 00375 00376 00377 return STB_NORMAL; 00378 } 00379 /*------------------------------------------------------------------------------------------------------------------*/ 00380 /* SetFrameResult : Get the result of stbINPUT */ 00381 /*------------------------------------------------------------------------------------------------------------------*/ 00382 STB_INT32 SetFrameResult ( STBHANDLE handle , const STB_FRAME_RESULT *stbINPUTResult ) 00383 { 00384 00385 STB_INT32 nRet; 00386 00387 /*NULL check*/ 00388 nRet = IsValidPointer(handle); 00389 if(nRet != STB_NORMAL) 00390 { 00391 return STB_ERR_NOHANDLE; 00392 } 00393 00394 nRet = IsValidPointer(stbINPUTResult); 00395 if(nRet != STB_NORMAL) 00396 { 00397 return STB_ERR_INVALIDPARAM; 00398 } 00399 00400 00401 /*Input value check*/ 00402 nRet = STB_IsValidValue ( stbINPUTResult ,handle->execFlg ); 00403 if(nRet != STB_TRUE) 00404 { 00405 return STB_ERR_INVALIDPARAM; 00406 } 00407 00408 00409 /*Clear the unexecuted state flag*/ 00410 handle->nExecuted = STB_FALSE; 00411 00412 /*Set the received result to the handle*/ 00413 if( handle->execFlg->bodyTr == STB_TRUE ) 00414 { 00415 handle->nDetCntBody = stbINPUTResult->bodys.nCount; 00416 SetTrackingObjectBody ( &(stbINPUTResult->bodys) ,handle->trBody ); 00417 } 00418 if( handle->execFlg->faceTr == STB_TRUE ) 00419 { 00420 handle->nDetCntFace = stbINPUTResult->faces.nCount; 00421 SetTrackingObjectFace ( &(stbINPUTResult->faces) ,handle->trFace ); 00422 } 00423 00424 /*Set detection result to Face/Property/Recognition data*/ 00425 if( handle->execFlg->gen == STB_TRUE 00426 || handle->execFlg->age == STB_TRUE 00427 || handle->execFlg->fr == STB_TRUE 00428 || handle->execFlg->exp == STB_TRUE 00429 || handle->execFlg->gaz == STB_TRUE 00430 || handle->execFlg->dir == STB_TRUE 00431 || handle->execFlg->bli == STB_TRUE 00432 ) 00433 { 00434 SetFaceObject ( &(stbINPUTResult->faces) ,handle->infoFace ,handle->execFlg , handle->nTraCntMax ); 00435 } 00436 00437 00438 handle->nInitialized = STB_TRUE; 00439 00440 return STB_NORMAL; 00441 } 00442 00443 /*------------------------------------------------------------------------------------------------------------------*/ 00444 /*Execute : Main process execution*/ 00445 /*------------------------------------------------------------------------------------------------------------------*/ 00446 STB_INT32 Execute ( STBHANDLE handle ) 00447 { 00448 STB_INT32 nRet ; 00449 STB_TR_DET *srcTr = handle->wSrcTr ;/*TR : input data*/ 00450 STB_TR_RES_FACES *dstTrFace = handle->wDstTrFace;/*TR : output data*/ 00451 STB_TR_RES_BODYS *dstTrBody = handle->wDstTrBody;/*TR : output data*/ 00452 STB_PE_DET *srcPe = handle->wSrcPe ;/*PR : Input data*/ 00453 STB_PE_RES *dstPe = handle->wDstPe ;/*PE : Output data*/ 00454 STB_FR_DET *srcFr = handle->wSrcFr ;/*FR : Input data*/ 00455 STB_FR_RES *dstFr = handle->wDstFr ;/*FR : Output data*/ 00456 00457 00458 00459 /*NULL check*/ 00460 nRet = IsValidPointer ( handle ); 00461 if( nRet != STB_NORMAL ) 00462 { 00463 return STB_ERR_NOHANDLE; 00464 } 00465 00466 if( handle->nInitialized != STB_TRUE) 00467 { 00468 return STB_ERR_INITIALIZE; 00469 } 00470 handle->nInitialized = STB_FALSE; 00471 handle->nExecuted = STB_FALSE; 00472 00473 /* TR ------------------------------------------------------------------------------------------------*/ 00474 if( handle->execFlg->faceTr == STB_TRUE ) 00475 { 00476 SetSrcTrFace ( handle->nDetCntFace , handle->trFace , srcTr ); /*Creation of tracking input data from handle information*/ 00477 } 00478 if( handle->execFlg->bodyTr == STB_TRUE ) 00479 { 00480 SetSrcTrBody ( handle->nDetCntBody , handle->trBody , srcTr ); /*Creation of tracking input data from handle information*/ 00481 } 00482 nRet = STB_Tr_SetDetect ( handle->hTrHandle , srcTr); /*Frame information settings*/ 00483 if( nRet != STB_NORMAL) { return nRet; } 00484 nRet = STB_Tr_Execute ( handle->hTrHandle ); /*execute tracking*/ 00485 if( nRet != STB_NORMAL) { return nRet; } 00486 nRet = STB_Tr_GetResult ( handle->hTrHandle , dstTrFace , dstTrBody ); /*get the tracking result*/ 00487 if( nRet != STB_NORMAL) { return nRet; } 00488 if( handle->execFlg->faceTr == STB_TRUE ) 00489 { 00490 SetTrackingInfoToFace ( dstTrFace,&(handle->nTraCntFace),handle->trFace);/*copy to handle the tracking result*/ 00491 } 00492 if( handle->execFlg->bodyTr == STB_TRUE ) 00493 { 00494 SetTrackingInfoToBody ( dstTrBody,&(handle->nTraCntBody),handle->trBody);/*copy to handle the tracking result*/ 00495 } 00496 00497 00498 /*Association of face information and tracking ID--------------------------------------------------------------------------------*/ 00499 if( handle->execFlg->gen == STB_TRUE 00500 || handle->execFlg->age == STB_TRUE 00501 || handle->execFlg->fr == STB_TRUE 00502 || handle->execFlg->exp == STB_TRUE 00503 || handle->execFlg->gaz == STB_TRUE 00504 || handle->execFlg->dir == STB_TRUE 00505 || handle->execFlg->bli == STB_TRUE 00506 ) 00507 { 00508 SetTrackingIDToFace ( handle->nTraCntFace ,handle->nDetCntFace, handle->trFace,handle->infoFace , handle->execFlg ); 00509 } 00510 00511 /* Fr ------------------------------------------------------------------------------------------------*/ 00512 if( handle->execFlg->fr == STB_TRUE ) 00513 { 00514 SetFaceToFrInfo ( handle->nTraCntFace,handle->infoFace,srcFr ); /*Creation of recognition input data from handle information*/ 00515 nRet = STB_Fr_SetDetect ( handle->hFrHandle,srcFr ); /*Pass to the recognized stabilization*/ 00516 if(nRet != STB_NORMAL ){ return nRet; } 00517 nRet = STB_Fr_Execute ( handle->hFrHandle ); /* Recognized stabilization execution*/ 00518 if(nRet != STB_NORMAL ){ return nRet; } 00519 nRet = STB_Fr_GetResult ( handle->hFrHandle,dstFr ); /*get the recognized stabilization results*/ 00520 if(nRet != STB_NORMAL ){ return nRet; } 00521 SetFrInfoToFace ( handle->nTraCntFace,dstFr,handle->infoFace ); /*Copy to handle the recognized stabilization results*/ 00522 } 00523 00524 /* Pe ------------------------------------------------------------------------------------------------*/ 00525 if( handle->execFlg->gen == STB_TRUE 00526 || handle->execFlg->age == STB_TRUE 00527 //|| handle->execFlg->fr == STB_TRUE 00528 || handle->execFlg->exp == STB_TRUE 00529 || handle->execFlg->gaz == STB_TRUE 00530 || handle->execFlg->dir == STB_TRUE 00531 || handle->execFlg->bli == STB_TRUE 00532 ) 00533 { 00534 SetFaceToPeInfo ( handle->nTraCntFace,handle->infoFace,srcPe ); /*Creation of property input data from handle information*/ 00535 nRet = STB_Pe_SetDetect ( handle->hPeHandle,srcPe ); /*Pass to property stabilization*/ 00536 if( nRet != STB_NORMAL ){ return nRet; } 00537 nRet = STB_Pe_Execute ( handle->hPeHandle ); /*Property stabilization execution*/ 00538 if( nRet != STB_NORMAL ){ return nRet; } 00539 nRet = STB_Pe_GetResult ( handle->hPeHandle,dstPe ); /*get the property stabilization results*/ 00540 if( nRet != STB_NORMAL ){ return nRet; } 00541 SetPeInfoToFace ( handle->nTraCntFace,dstPe,handle->infoFace , handle->execFlg ); /*Copy to handle the property stabilization results*/ 00542 } 00543 00544 00545 00546 /*Set execution completion flag--------------------------------------------------*/ 00547 handle->nExecuted = STB_TRUE; 00548 00549 return STB_NORMAL; 00550 } 00551 /*------------------------------------------------------------------------------------------------------------------*/ 00552 /* GetFaces : Getting stabilization results of face*/ 00553 /*------------------------------------------------------------------------------------------------------------------*/ 00554 STB_INT32 GetFaces(STBHANDLE handle, STB_UINT32 *face_count, STB_FACE *face ) 00555 { 00556 STB_INT32 nRet , i; 00557 00558 /*NULL check*/ 00559 nRet = IsValidPointer(handle); 00560 if(nRet != STB_NORMAL) 00561 { 00562 return STB_ERR_NOHANDLE; 00563 } 00564 nRet = IsValidPointer(face_count); 00565 if(nRet != STB_NORMAL) 00566 { 00567 return nRet; 00568 } 00569 nRet = IsValidPointer(face); 00570 if(nRet != STB_NORMAL) 00571 { 00572 return nRet; 00573 } 00574 if( handle->nExecuted != STB_TRUE) 00575 { 00576 return STB_ERR_INITIALIZE; 00577 } 00578 00579 /*init*/ 00580 *face_count = 0; 00581 for( i = 0 ; i < handle->nTraCntMax ; i++ ) 00582 { 00583 face[i].nDetectID = -1; 00584 face[i].nTrackingID = -1; 00585 face[i].center.x = 0; 00586 face[i].center.y = 0; 00587 face[i].nSize = 0; 00588 face[i].conf = STB_CONF_NO_DATA ; 00589 face[i].age.conf = STB_CONF_NO_DATA ; 00590 face[i].age.status = STB_STATUS_NO_DATA; 00591 face[i].age.value = -1; 00592 face[i].blink.ratioL = -1; 00593 face[i].blink.ratioR = -1; 00594 face[i].blink.status = STB_STATUS_NO_DATA; 00595 face[i].direction.conf = STB_CONF_NO_DATA ; 00596 face[i].direction.pitch = -1; 00597 face[i].direction.roll = -1; 00598 face[i].direction.status = STB_STATUS_NO_DATA; 00599 face[i].direction.yaw = -1; 00600 face[i].expression.conf = STB_CONF_NO_DATA ; 00601 face[i].expression.status = STB_STATUS_NO_DATA; 00602 face[i].expression.value = -1; 00603 face[i].gaze.conf = STB_CONF_NO_DATA ; 00604 face[i].gaze.LR = -1; 00605 face[i].gaze.status = STB_STATUS_NO_DATA; 00606 face[i].gaze.UD = -1; 00607 face[i].gender.conf = STB_CONF_NO_DATA ; 00608 face[i].gender.status = STB_STATUS_NO_DATA; 00609 face[i].gender.value = -1; 00610 face[i].recognition.conf = STB_CONF_NO_DATA ; 00611 face[i].recognition.status = STB_STATUS_NO_DATA; 00612 face[i].recognition.value = -1; 00613 } 00614 00615 /*Set the result to the structure*/ 00616 if( handle->execFlg->faceTr == STB_TRUE ) 00617 { 00618 *face_count = handle->nTraCntFace; 00619 SetFaceToResult ( handle->nTraCntFace ,handle->trFace ,handle->infoFace ,face , handle->execFlg ); 00620 } 00621 00622 return STB_NORMAL; 00623 } 00624 /*------------------------------------------------------------------------------------------------------------------*/ 00625 /* GetBodies : Getting stabilization results of body */ 00626 /*------------------------------------------------------------------------------------------------------------------*/ 00627 STB_INT32 GetBodies(STBHANDLE handle, STB_UINT32 *body_count, STB_BODY *body) 00628 { 00629 STB_INT32 nRet , i; 00630 00631 /*NULL check*/ 00632 nRet = IsValidPointer(handle); 00633 if(nRet != STB_NORMAL){ 00634 return STB_ERR_NOHANDLE; 00635 } 00636 nRet = IsValidPointer(body_count); 00637 if(nRet != STB_NORMAL){ 00638 return nRet; 00639 } 00640 nRet = IsValidPointer(body); 00641 if(nRet != STB_NORMAL){ 00642 return nRet; 00643 } 00644 if( handle->nExecuted != STB_TRUE){ 00645 return STB_ERR_INITIALIZE; 00646 } 00647 00648 00649 /*init*/ 00650 *body_count = 0; 00651 for( i = 0 ; i < handle->nTraCntMax ; i++ ) 00652 { 00653 body[i].nDetectID = -1; 00654 body[i].nTrackingID = -1; 00655 body[i].center.x = 0; 00656 body[i].center.y = 0; 00657 body[i].nSize = 0; 00658 body[i].conf = STB_CONF_NO_DATA ; 00659 } 00660 00661 /*Set the result to the structure*/ 00662 if( handle->execFlg->bodyTr == STB_TRUE ) 00663 { 00664 *body_count = handle->nTraCntBody; 00665 SetBodyToResult(handle->nTraCntBody,handle->trBody, body); 00666 } 00667 return STB_NORMAL; 00668 } 00669 /*------------------------------------------------------------------------------------------------------------------*/ 00670 /* Clear */ 00671 /*------------------------------------------------------------------------------------------------------------------*/ 00672 STB_INT32 Clear(STBHANDLE handle) 00673 { 00674 STB_INT32 nRet; 00675 00676 /*NULL check*/ 00677 nRet = IsValidPointer(handle); 00678 if(nRet != STB_NORMAL){ 00679 return STB_ERR_NOHANDLE; 00680 } 00681 00682 STB_Tr_Clear( handle->hTrHandle ); 00683 STB_Pe_Clear( handle->hPeHandle ); 00684 if( handle->execFlg->fr == STB_TRUE ) 00685 { 00686 STB_Fr_Clear( handle->hFrHandle ); 00687 } 00688 00689 handle->nInitialized = STB_FALSE; 00690 handle->nExecuted = STB_FALSE; 00691 00692 00693 return STB_NORMAL; 00694 00695 } 00696 /*------------------------------------------------------------------------------------------------------------------*/ 00697 /*Setting function (wrapper for child libraries)*/ 00698 /*------------------------------------------------------------------------------------------------------------------*/ 00699 STB_INT32 SetTrackingRetryCount(STBHANDLE handle, STB_INT32 nMaxRetryCount){ 00700 STB_INT32 nRet; 00701 /*NULL check*/ 00702 nRet = IsValidPointer(handle); 00703 if(nRet != STB_NORMAL){ 00704 return STB_ERR_NOHANDLE; 00705 } 00706 00707 return STB_Tr_SetRetryCount(handle->hTrHandle,nMaxRetryCount); 00708 } 00709 00710 STB_INT32 GetTrackingRetryCount(STBHANDLE handle, STB_INT32 *pnMaxRetryCount){ 00711 STB_INT32 nRet; 00712 /*NULL check*/ 00713 nRet = IsValidPointer(handle); 00714 if(nRet != STB_NORMAL){ 00715 return STB_ERR_NOHANDLE; 00716 } 00717 00718 return STB_Tr_GetRetryCount(handle->hTrHandle,pnMaxRetryCount); 00719 } 00720 00721 STB_INT32 SetTrackingSteadinessParam(STBHANDLE handle, STB_INT32 nPosSteadinessParam, STB_INT32 nSizeSteadinessParam){ 00722 STB_INT32 nRet; 00723 /*NULL check*/ 00724 nRet = IsValidPointer(handle); 00725 if(nRet != STB_NORMAL){ 00726 return STB_ERR_NOHANDLE; 00727 } 00728 00729 return STB_Tr_SetStedinessParam(handle->hTrHandle, nPosSteadinessParam, nSizeSteadinessParam); 00730 } 00731 00732 STB_INT32 GetTrackingSteadinessParam(STBHANDLE handle, STB_INT32 *pnPosSteadinessParam, STB_INT32 *pnSizeSteadinessParam){ 00733 STB_INT32 nRet; 00734 /*NULL check*/ 00735 nRet = IsValidPointer(handle); 00736 if(nRet != STB_NORMAL){ 00737 return STB_ERR_NOHANDLE; 00738 } 00739 00740 return STB_Tr_GetStedinessParam(handle->hTrHandle, pnPosSteadinessParam, pnSizeSteadinessParam); 00741 } 00742 00743 STB_INT32 SetPropertyThreshold(STBHANDLE handle, STB_INT32 nThreshold){ 00744 STB_INT32 nRet; 00745 /*NULL check*/ 00746 nRet = IsValidPointer(handle); 00747 if(nRet != STB_NORMAL){ 00748 return STB_ERR_NOHANDLE; 00749 } 00750 00751 if( handle->execFlg->gen == STB_TRUE 00752 || handle->execFlg->age == STB_TRUE 00753 || handle->execFlg->exp == STB_TRUE 00754 || handle->execFlg->dir == STB_TRUE 00755 || handle->execFlg->gaz == STB_TRUE 00756 || handle->execFlg->bli == STB_TRUE 00757 ) 00758 { 00759 }else 00760 { 00761 return STB_NORMAL; 00762 } 00763 00764 return STB_Pe_SetFaceDirThreshold(handle->hPeHandle, nThreshold); 00765 } 00766 00767 STB_INT32 GetPropertyThreshold(STBHANDLE handle, STB_INT32 *pnThreshold){ 00768 STB_INT32 nRet; 00769 /*NULL check*/ 00770 nRet = IsValidPointer(handle); 00771 if(nRet != STB_NORMAL){ 00772 return STB_ERR_NOHANDLE; 00773 } 00774 if( handle->execFlg->gen == STB_TRUE 00775 || handle->execFlg->age == STB_TRUE 00776 || handle->execFlg->exp == STB_TRUE 00777 || handle->execFlg->dir == STB_TRUE 00778 || handle->execFlg->gaz == STB_TRUE 00779 || handle->execFlg->bli == STB_TRUE 00780 ) 00781 { 00782 }else 00783 { 00784 return STB_NORMAL; 00785 } 00786 return STB_Pe_GetFaceDirThreshold(handle->hPeHandle, pnThreshold); 00787 } 00788 00789 STB_INT32 SetPropertyAngle(STBHANDLE handle,STB_INT32 nMinUDAngle, STB_INT32 nMaxUDAngle, 00790 STB_INT32 nMinLRAngle, STB_INT32 nMaxLRAngle){ 00791 STB_INT32 nRet; 00792 /*NULL check*/ 00793 nRet = IsValidPointer(handle); 00794 if(nRet != STB_NORMAL){ 00795 return STB_ERR_NOHANDLE; 00796 } 00797 if( handle->execFlg->gen == STB_TRUE 00798 || handle->execFlg->age == STB_TRUE 00799 || handle->execFlg->exp == STB_TRUE 00800 || handle->execFlg->dir == STB_TRUE 00801 || handle->execFlg->gaz == STB_TRUE 00802 || handle->execFlg->bli == STB_TRUE 00803 ) 00804 { 00805 }else 00806 { 00807 return STB_NORMAL; 00808 } 00809 return STB_Pe_SetFaceDirMinMax(handle->hPeHandle, nMinUDAngle, nMaxUDAngle, nMinLRAngle, nMaxLRAngle); 00810 } 00811 00812 STB_INT32 GetPropertyAngle(STBHANDLE handle, STB_INT32 *pnMinUDAngle , STB_INT32 *pnMaxUDAngle , 00813 STB_INT32 *pnMinLRAngle , STB_INT32 *pnMaxLRAngle ){ 00814 STB_INT32 nRet; 00815 /*NULL check*/ 00816 nRet = IsValidPointer(handle); 00817 if(nRet != STB_NORMAL){ 00818 return STB_ERR_NOHANDLE; 00819 } 00820 if( handle->execFlg->gen == STB_TRUE 00821 || handle->execFlg->age == STB_TRUE 00822 || handle->execFlg->exp == STB_TRUE 00823 || handle->execFlg->dir == STB_TRUE 00824 || handle->execFlg->gaz == STB_TRUE 00825 || handle->execFlg->bli == STB_TRUE 00826 ) 00827 { 00828 }else 00829 { 00830 return STB_NORMAL; 00831 } 00832 return STB_Pe_GetFaceDirMinMax(handle->hPeHandle, pnMinUDAngle, pnMaxUDAngle, pnMinLRAngle, pnMaxLRAngle); 00833 } 00834 STB_INT32 SetPropertyFrameCount(STBHANDLE handle, STB_INT32 nFrameCount){ 00835 STB_INT32 nRet; 00836 /*NULL check*/ 00837 nRet = IsValidPointer(handle); 00838 if(nRet != STB_NORMAL){ 00839 return STB_ERR_NOHANDLE; 00840 } 00841 if( handle->execFlg->gen == STB_TRUE 00842 || handle->execFlg->age == STB_TRUE 00843 || handle->execFlg->exp == STB_TRUE 00844 || handle->execFlg->dir == STB_TRUE 00845 || handle->execFlg->gaz == STB_TRUE 00846 || handle->execFlg->bli == STB_TRUE 00847 ) 00848 { 00849 }else 00850 { 00851 return STB_NORMAL; 00852 } 00853 return STB_Pe_SetFrameCount(handle->hPeHandle, nFrameCount); 00854 } 00855 STB_INT32 GetPropertyFrameCount(STBHANDLE handle, STB_INT32 *pnFrameCount){ 00856 STB_INT32 nRet; 00857 /*NULL check*/ 00858 nRet = IsValidPointer(handle); 00859 if(nRet != STB_NORMAL){ 00860 return STB_ERR_NOHANDLE; 00861 } 00862 if( handle->execFlg->gen == STB_TRUE 00863 || handle->execFlg->age == STB_TRUE 00864 || handle->execFlg->exp == STB_TRUE 00865 || handle->execFlg->dir == STB_TRUE 00866 || handle->execFlg->gaz == STB_TRUE 00867 || handle->execFlg->bli == STB_TRUE 00868 ) 00869 { 00870 }else 00871 { 00872 return STB_NORMAL; 00873 } 00874 return STB_Pe_GetFrameCount(handle->hPeHandle, pnFrameCount); 00875 } 00876 STB_INT32 SetRecognitionThreshold(STBHANDLE handle, STB_INT32 nThreshold){ 00877 STB_INT32 nRet; 00878 /*NULL check*/ 00879 nRet = IsValidPointer(handle); 00880 if(nRet != STB_NORMAL){ 00881 return STB_ERR_NOHANDLE; 00882 } 00883 if( handle->execFlg->fr == STB_FALSE ) 00884 { 00885 return STB_NORMAL; 00886 } 00887 return STB_Fr_SetFaceDirThreshold(handle->hFrHandle, nThreshold); 00888 } 00889 STB_INT32 GetRecognitionThreshold(STBHANDLE handle, STB_INT32 *pnThreshold){ 00890 STB_INT32 nRet; 00891 /*NULL check*/ 00892 nRet = IsValidPointer(handle); 00893 if(nRet != STB_NORMAL){ 00894 return STB_ERR_NOHANDLE; 00895 } 00896 if( handle->execFlg->fr == STB_FALSE ) 00897 { 00898 return STB_NORMAL; 00899 } 00900 return STB_Fr_GetFaceDirThreshold(handle->hFrHandle, pnThreshold); 00901 } 00902 00903 STB_INT32 SetRecognitionAngle(STBHANDLE handle, STB_INT32 nMinUDAngle, STB_INT32 nMaxUDAngle, 00904 STB_INT32 nMinLRAngle, STB_INT32 nMaxLRAngle){ 00905 STB_INT32 nRet; 00906 /*NULL check*/ 00907 nRet = IsValidPointer(handle); 00908 if(nRet != STB_NORMAL){ 00909 return STB_ERR_NOHANDLE; 00910 } 00911 if( handle->execFlg->fr == STB_FALSE ) 00912 { 00913 return STB_NORMAL; 00914 } 00915 return STB_Fr_SetFaceDirMinMax(handle->hFrHandle, nMinUDAngle, nMaxUDAngle, nMinLRAngle , nMaxLRAngle); 00916 } 00917 00918 STB_INT32 GetRecognitionAngle(STBHANDLE handle, STB_INT32 *pnMinUDAngle , STB_INT32 *pnMaxUDAngle , 00919 STB_INT32 *pnMinLRAngle , STB_INT32 *pnMaxLRAngle){ 00920 STB_INT32 nRet; 00921 /*NULL check*/ 00922 nRet = IsValidPointer(handle); 00923 if(nRet != STB_NORMAL){ 00924 return STB_ERR_NOHANDLE; 00925 } 00926 nRet = IsValidPointer(pnMinUDAngle); 00927 if(nRet != STB_NORMAL){ 00928 return STB_ERR_INVALIDPARAM; 00929 } 00930 nRet = IsValidPointer(pnMaxUDAngle); 00931 if(nRet != STB_NORMAL){ 00932 return STB_ERR_INVALIDPARAM; 00933 } 00934 nRet = IsValidPointer(pnMinLRAngle); 00935 if(nRet != STB_NORMAL){ 00936 return STB_ERR_INVALIDPARAM; 00937 } 00938 nRet = IsValidPointer(pnMaxLRAngle); 00939 if(nRet != STB_NORMAL){ 00940 return STB_ERR_INVALIDPARAM; 00941 } 00942 00943 if( handle->execFlg->fr == STB_FALSE ) 00944 { 00945 return STB_NORMAL; 00946 } 00947 return STB_Fr_GetFaceDirMinMax(handle->hFrHandle, pnMinUDAngle, pnMaxUDAngle, pnMinLRAngle , pnMaxLRAngle); 00948 } 00949 00950 STB_INT32 SetRecognitionFrameCount(STBHANDLE handle, STB_INT32 nFrameCount){ 00951 STB_INT32 nRet; 00952 /*NULL check*/ 00953 nRet = IsValidPointer(handle); 00954 if(nRet != STB_NORMAL){ 00955 return STB_ERR_NOHANDLE; 00956 } 00957 if( handle->execFlg->fr == STB_FALSE ) 00958 { 00959 return STB_NORMAL; 00960 } 00961 return STB_Fr_SetFrameCount(handle->hFrHandle, nFrameCount); 00962 } 00963 STB_INT32 GetRecognitionFrameCount(STBHANDLE handle, STB_INT32 *pnFrameCount){ 00964 STB_INT32 nRet; 00965 /*NULL check*/ 00966 nRet = IsValidPointer(handle); 00967 if(nRet != STB_NORMAL){ 00968 return STB_ERR_NOHANDLE; 00969 } 00970 if( handle->execFlg->fr == STB_FALSE ) 00971 { 00972 return STB_NORMAL; 00973 } 00974 return STB_Fr_GetFrameCount(handle->hFrHandle, pnFrameCount); 00975 } 00976 00977 STB_INT32 SetRecognitionRatio (STBHANDLE handle, STB_INT32 nMinRatio){ 00978 STB_INT32 nRet; 00979 /*NULL check*/ 00980 nRet = IsValidPointer(handle); 00981 if(nRet != STB_NORMAL){ 00982 return STB_ERR_NOHANDLE; 00983 } 00984 if( handle->execFlg->fr == STB_FALSE ) 00985 { 00986 return STB_NORMAL; 00987 } 00988 return STB_Fr_SetMinRatio(handle->hFrHandle, nMinRatio); 00989 } 00990 STB_INT32 GetRecognitionRatio (STBHANDLE handle, STB_INT32 *pnMinRatio){ 00991 STB_INT32 nRet; 00992 /*NULL check*/ 00993 nRet = IsValidPointer(handle); 00994 if(nRet != STB_NORMAL){ 00995 return STB_ERR_NOHANDLE; 00996 } 00997 if( handle->execFlg->fr == STB_FALSE ) 00998 { 00999 return STB_NORMAL; 01000 } 01001 return STB_Fr_GetMinRatio(handle->hFrHandle, pnMinRatio); 01002 }
Generated on Fri Jul 15 2022 01:15:47 by
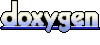