
Sample to operate omron HVC-P2 on GR-PEACH.
Dependencies: AsciiFont
HVCApi.c
00001 /*---------------------------------------------------------------------------*/ 00002 /* Copyright(C) 2017 OMRON Corporation */ 00003 /* */ 00004 /* Licensed under the Apache License, Version 2.0 (the "License"); */ 00005 /* you may not use this file except in compliance with the License. */ 00006 /* You may obtain a copy of the License at */ 00007 /* */ 00008 /* http://www.apache.org/licenses/LICENSE-2.0 */ 00009 /* */ 00010 /* Unless required by applicable law or agreed to in writing, software */ 00011 /* distributed under the License is distributed on an "AS IS" BASIS, */ 00012 /* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. */ 00013 /* See the License for the specific language governing permissions and */ 00014 /* limitations under the License. */ 00015 /*---------------------------------------------------------------------------*/ 00016 00017 /* 00018 HVC Sample API 00019 */ 00020 00021 #include <stdlib.h> 00022 #include "HVCApi.h" 00023 #include "HVCExtraUartFunc.h" 00024 00025 /*----------------------------------------------------------------------------*/ 00026 /* Command number */ 00027 /*----------------------------------------------------------------------------*/ 00028 #define HVC_COM_GET_VERSION (UINT8)0x00 00029 #define HVC_COM_SET_CAMERA_ANGLE (UINT8)0x01 00030 #define HVC_COM_GET_CAMERA_ANGLE (UINT8)0x02 00031 #define HVC_COM_EXECUTE (UINT8)0x03 00032 #define HVC_COM_EXECUTEEX (UINT8)0x04 00033 #define HVC_COM_SET_THRESHOLD (UINT8)0x05 00034 #define HVC_COM_GET_THRESHOLD (UINT8)0x06 00035 #define HVC_COM_SET_SIZE_RANGE (UINT8)0x07 00036 #define HVC_COM_GET_SIZE_RANGE (UINT8)0x08 00037 #define HVC_COM_SET_DETECTION_ANGLE (UINT8)0x09 00038 #define HVC_COM_GET_DETECTION_ANGLE (UINT8)0x0A 00039 #define HVC_COM_SET_BAUDRATE (UINT8)0x0E 00040 #define HVC_COM_REGISTRATION (UINT8)0x10 00041 #define HVC_COM_DELETE_DATA (UINT8)0x11 00042 #define HVC_COM_DELETE_USER (UINT8)0x12 00043 #define HVC_COM_DELETE_ALL (UINT8)0x13 00044 #define HVC_COM_GET_PERSON_DATA (UINT8)0x15 00045 #define HVC_COM_SAVE_ALBUM (UINT8)0x20 00046 #define HVC_COM_LOAD_ALBUM (UINT8)0x21 00047 #define HVC_COM_WRITE_ALBUM (UINT8)0x22 00048 00049 /*----------------------------------------------------------------------------*/ 00050 /* Header for send signal data */ 00051 typedef enum { 00052 SEND_HEAD_SYNCBYTE = 0, 00053 SEND_HEAD_COMMANDNO, 00054 SEND_HEAD_DATALENGTHLSB, 00055 SEND_HEAD_DATALENGTHMSB, 00056 SEND_HEAD_NUM 00057 }SEND_HEADER; 00058 /*----------------------------------------------------------------------------*/ 00059 /* Header for receive signal data */ 00060 typedef enum { 00061 RECEIVE_HEAD_SYNCBYTE = 0, 00062 RECEIVE_HEAD_STATUS, 00063 RECEIVE_HEAD_DATALENLL, 00064 RECEIVE_HEAD_DATALENLM, 00065 RECEIVE_HEAD_DATALENML, 00066 RECEIVE_HEAD_DATALENMM, 00067 RECEIVE_HEAD_NUM 00068 }RECEIVE_HEADER; 00069 00070 /*----------------------------------------------------------------------------*/ 00071 /* Send command signal */ 00072 /* param : UINT8 inCommandNo command number */ 00073 /* : INT32 inDataSize sending signal data size */ 00074 /* : UINT8 *inData sending signal data */ 00075 /* return : INT32 execution result error code */ 00076 /* : 0...normal */ 00077 /* : -10...timeout error */ 00078 /*----------------------------------------------------------------------------*/ 00079 static INT32 HVC_SendCommand(UINT8 inCommandNo, INT32 inDataSize, UINT8 *inData) 00080 { 00081 INT32 i; 00082 INT32 ret = 0; 00083 UINT8 sendData[32]; 00084 00085 /* Create header */ 00086 sendData[SEND_HEAD_SYNCBYTE] = (UINT8)0xFE; 00087 sendData[SEND_HEAD_COMMANDNO] = (UINT8)inCommandNo; 00088 sendData[SEND_HEAD_DATALENGTHLSB] = (UINT8)(inDataSize&0xff); 00089 sendData[SEND_HEAD_DATALENGTHMSB] = (UINT8)((inDataSize>>8)&0xff); 00090 00091 for(i = 0; i < inDataSize; i++){ 00092 sendData[SEND_HEAD_NUM + i] = inData[i]; 00093 } 00094 00095 /* Send command signal */ 00096 ret = UART_SendData(SEND_HEAD_NUM+inDataSize, sendData); 00097 if(ret != SEND_HEAD_NUM+inDataSize){ 00098 return HVC_ERROR_SEND_DATA; 00099 } 00100 return 0; 00101 } 00102 00103 /*----------------------------------------------------------------------------*/ 00104 /* Send command signal of LoadAlbum */ 00105 /* param : UINT8 inCommandNo command number */ 00106 /* : INT32 inDataSize sending signal data size */ 00107 /* : UINT8 *inData sending signal data */ 00108 /* return : INT32 execution result error code */ 00109 /* : 0...normal */ 00110 /* : -10...timeout error */ 00111 /*----------------------------------------------------------------------------*/ 00112 static INT32 HVC_SendCommandOfLoadAlbum(UINT8 inCommandNo, INT32 inDataSize, UINT8 *inData) 00113 { 00114 INT32 i; 00115 INT32 ret = 0; 00116 UINT8 *pSendData = NULL; 00117 00118 pSendData = (UINT8*)malloc(SEND_HEAD_NUM + 4 + inDataSize); 00119 00120 /* Create header */ 00121 pSendData[SEND_HEAD_SYNCBYTE] = (UINT8)0xFE; 00122 pSendData[SEND_HEAD_COMMANDNO] = (UINT8)inCommandNo; 00123 pSendData[SEND_HEAD_DATALENGTHLSB] = (UINT8)4; 00124 pSendData[SEND_HEAD_DATALENGTHMSB] = (UINT8)0; 00125 00126 pSendData[SEND_HEAD_NUM + 0] = (UINT8)(inDataSize & 0x000000ff); 00127 pSendData[SEND_HEAD_NUM + 1] = (UINT8)((inDataSize >> 8) & 0x000000ff); 00128 pSendData[SEND_HEAD_NUM + 2] = (UINT8)((inDataSize >> 16) & 0x000000ff); 00129 pSendData[SEND_HEAD_NUM + 3] = (UINT8)((inDataSize >> 24) & 0x000000ff); 00130 00131 for(i = 0; i < inDataSize; i++){ 00132 pSendData[SEND_HEAD_NUM + 4 + i] = inData[i]; 00133 } 00134 00135 /* Send command signal */ 00136 ret = UART_SendData(SEND_HEAD_NUM+4+inDataSize, pSendData); 00137 if(ret != SEND_HEAD_NUM + 4 + inDataSize){ 00138 ret = HVC_ERROR_SEND_DATA; 00139 } 00140 else{ 00141 ret = 0; 00142 } 00143 free(pSendData); 00144 00145 return ret; 00146 } 00147 00148 /*----------------------------------------------------------------------------*/ 00149 /* Receive header */ 00150 /* param : INT32 inTimeOutTime timeout time */ 00151 /* : INT32 *outDataSize receive signal data length */ 00152 /* : UINT8 *outStatus status */ 00153 /* return : INT32 execution result error code */ 00154 /* : 0...normal */ 00155 /* : -20...timeout error */ 00156 /* : -21...invalid header error */ 00157 /*----------------------------------------------------------------------------*/ 00158 static INT32 HVC_ReceiveHeader(INT32 inTimeOutTime, INT32 *outDataSize, UINT8 *outStatus) 00159 { 00160 INT32 ret = 0; 00161 UINT8 headerData[32]; 00162 00163 /* Get header part */ 00164 ret = UART_ReceiveData(inTimeOutTime, RECEIVE_HEAD_NUM, headerData); 00165 if(ret != RECEIVE_HEAD_NUM){ 00166 return HVC_ERROR_HEADER_TIMEOUT; 00167 } 00168 else if((UINT8)0xFE != headerData[RECEIVE_HEAD_SYNCBYTE]){ 00169 /* Different value indicates an invalid result */ 00170 return HVC_ERROR_HEADER_INVALID; 00171 } 00172 00173 /* Get data length */ 00174 *outDataSize = headerData[RECEIVE_HEAD_DATALENLL] + 00175 (headerData[RECEIVE_HEAD_DATALENLM]<<8) + 00176 (headerData[RECEIVE_HEAD_DATALENML]<<16) + 00177 (headerData[RECEIVE_HEAD_DATALENMM]<<24); 00178 00179 /* Get command execution result */ 00180 *outStatus = headerData[RECEIVE_HEAD_STATUS]; 00181 return 0; 00182 } 00183 00184 /*----------------------------------------------------------------------------*/ 00185 /* Receive data */ 00186 /* param : INT32 inTimeOutTime timeout time */ 00187 /* : INT32 inDataSize receive signal data size */ 00188 /* : UINT8 *outResult receive signal data */ 00189 /* return : INT32 execution result error code */ 00190 /* : 0...normal */ 00191 /* : -20...timeout error */ 00192 /*----------------------------------------------------------------------------*/ 00193 static INT32 HVC_ReceiveData(INT32 inTimeOutTime, INT32 inDataSize, UINT8 *outResult) 00194 { 00195 INT32 ret = 0; 00196 00197 if ( inDataSize <= 0 ) return 0; 00198 00199 /* Receive data */ 00200 ret = UART_ReceiveData(inTimeOutTime, inDataSize, outResult); 00201 if(ret != inDataSize){ 00202 return HVC_ERROR_DATA_TIMEOUT; 00203 } 00204 return 0; 00205 } 00206 00207 /*----------------------------------------------------------------------------*/ 00208 /* HVC_GetVersion */ 00209 /* param : INT32 inTimeOutTime timeout time (ms) */ 00210 /* : HVC_VERSION *outVersion version data */ 00211 /* : UINT8 *outStatus response code */ 00212 /* return : INT32 execution result error code */ 00213 /* : 0...normal */ 00214 /* : -1...parameter error */ 00215 /* : other...signal error */ 00216 /*----------------------------------------------------------------------------*/ 00217 INT32 HVC_GetVersion(INT32 inTimeOutTime, HVC_VERSION *outVersion, UINT8 *outStatus) 00218 { 00219 INT32 ret = 0; 00220 INT32 size = 0; 00221 00222 if((NULL == outVersion) || (NULL == outStatus)){ 00223 return HVC_ERROR_PARAMETER; 00224 } 00225 00226 /* Send GetVersion command signal */ 00227 ret = HVC_SendCommand(HVC_COM_GET_VERSION, 0, NULL); 00228 if ( ret != 0 ) return ret; 00229 00230 /* Receive header */ 00231 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00232 if ( ret != 0 ) return ret; 00233 00234 if ( size > (INT32)sizeof(HVC_VERSION) ) { 00235 size = sizeof(HVC_VERSION); 00236 } 00237 00238 /* Receive data */ 00239 return HVC_ReceiveData(inTimeOutTime, size, (UINT8*)outVersion); 00240 } 00241 00242 /*----------------------------------------------------------------------------*/ 00243 /* HVC_SetCameraAngle */ 00244 /* param : INT32 inTimeOutTime timeout time (ms) */ 00245 /* : INT32 inAngleNo camera angle number */ 00246 /* : UINT8 *outStatus response code */ 00247 /* return : INT32 execution result error code */ 00248 /* : 0...normal */ 00249 /* : -1...parameter error */ 00250 /* : other...signal error */ 00251 /*----------------------------------------------------------------------------*/ 00252 INT32 HVC_SetCameraAngle(INT32 inTimeOutTime, INT32 inAngleNo, UINT8 *outStatus) 00253 { 00254 INT32 ret = 0; 00255 INT32 size = 0; 00256 UINT8 sendData[32]; 00257 00258 if(NULL == outStatus){ 00259 return HVC_ERROR_PARAMETER; 00260 } 00261 00262 sendData[0] = (UINT8)(inAngleNo&0xff); 00263 /* Send SetCameraAngle command signal */ 00264 ret = HVC_SendCommand(HVC_COM_SET_CAMERA_ANGLE, sizeof(UINT8), sendData); 00265 if ( ret != 0 ) return ret; 00266 00267 /* Receive header */ 00268 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00269 if ( ret != 0 ) return ret; 00270 return 0; 00271 } 00272 00273 /*----------------------------------------------------------------------------*/ 00274 /* HVC_GetCameraAngle */ 00275 /* param : INT32 inTimeOutTime timeout time (ms) */ 00276 /* : INT32 *outAngleNo camera angle number */ 00277 /* : UINT8 *outStatus response code */ 00278 /* return : INT32 execution result error code */ 00279 /* : 0...normal */ 00280 /* : -1...parameter error */ 00281 /* : other...signal error */ 00282 /*----------------------------------------------------------------------------*/ 00283 INT32 HVC_GetCameraAngle(INT32 inTimeOutTime, INT32 *outAngleNo, UINT8 *outStatus) 00284 { 00285 INT32 ret = 0; 00286 INT32 size = 0; 00287 UINT8 recvData[32]; 00288 00289 if((NULL == outAngleNo) || (NULL == outStatus)){ 00290 return HVC_ERROR_PARAMETER; 00291 } 00292 00293 /* Send GetCameraAngle command signal */ 00294 ret = HVC_SendCommand(HVC_COM_GET_CAMERA_ANGLE, 0, NULL); 00295 if ( ret != 0 ) return ret; 00296 00297 /* Receive header */ 00298 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00299 if ( ret != 0 ) return ret; 00300 00301 if ( size > (INT32)sizeof(UINT8) ) { 00302 size = sizeof(UINT8); 00303 } 00304 00305 /* Receive data */ 00306 ret = HVC_ReceiveData(inTimeOutTime, size, recvData); 00307 *outAngleNo = recvData[0]; 00308 return ret; 00309 } 00310 00311 /*----------------------------------------------------------------------------*/ 00312 /* HVC_Execute */ 00313 /* param : INT32 inTimeOutTime timeout time (ms) */ 00314 /* : INT32 inExec executable function */ 00315 /* : INT32 inImage image info */ 00316 /* : HVC_RESULT *outHVCResult result data */ 00317 /* : UINT8 *outStatus response code */ 00318 /* return : INT32 execution result error code */ 00319 /* : 0...normal */ 00320 /* : -1...parameter error */ 00321 /* : other...signal error */ 00322 /*----------------------------------------------------------------------------*/ 00323 INT32 HVC_Execute(INT32 inTimeOutTime, INT32 inExec, INT32 inImage, HVC_RESULT *outHVCResult, UINT8 *outStatus) 00324 { 00325 int i; 00326 INT32 ret = 0; 00327 INT32 size = 0; 00328 UINT8 sendData[32]; 00329 UINT8 recvData[32]; 00330 00331 if((NULL == outHVCResult) || (NULL == outStatus)){ 00332 return HVC_ERROR_PARAMETER; 00333 } 00334 00335 /* Send Execute command signal */ 00336 sendData[0] = (UINT8)(inExec&0xff); 00337 sendData[1] = (UINT8)((inExec>>8)&0xff); 00338 sendData[2] = (UINT8)(inImage&0xff); 00339 ret = HVC_SendCommand(HVC_COM_EXECUTE, sizeof(UINT8)*3, sendData); 00340 if ( ret != 0 ) return ret; 00341 00342 /* Receive header */ 00343 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00344 if ( ret != 0 ) return ret; 00345 00346 /* Receive result data */ 00347 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00348 outHVCResult->executedFunc = inExec; 00349 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00350 outHVCResult->bdResult.num = recvData[0]; 00351 outHVCResult->hdResult.num = recvData[1]; 00352 outHVCResult->fdResult.num = recvData[2]; 00353 if ( ret != 0 ) return ret; 00354 size -= sizeof(UINT8)*4; 00355 } 00356 00357 /* Get Human Body Detection result */ 00358 for(i = 0; i < outHVCResult->bdResult.num; i++){ 00359 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00360 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00361 outHVCResult->bdResult.bdResult[i].posX = (short)(recvData[0] + (recvData[1]<<8)); 00362 outHVCResult->bdResult.bdResult[i].posY = (short)(recvData[2] + (recvData[3]<<8)); 00363 outHVCResult->bdResult.bdResult[i].size = (short)(recvData[4] + (recvData[5]<<8)); 00364 outHVCResult->bdResult.bdResult[i].confidence = (short)(recvData[6] + (recvData[7]<<8)); 00365 if ( ret != 0 ) return ret; 00366 size -= sizeof(UINT8)*8; 00367 } 00368 } 00369 00370 /* Get Hand Detection result */ 00371 for(i = 0; i < outHVCResult->hdResult.num; i++){ 00372 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00373 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00374 outHVCResult->hdResult.hdResult[i].posX = (short)(recvData[0] + (recvData[1]<<8)); 00375 outHVCResult->hdResult.hdResult[i].posY = (short)(recvData[2] + (recvData[3]<<8)); 00376 outHVCResult->hdResult.hdResult[i].size = (short)(recvData[4] + (recvData[5]<<8)); 00377 outHVCResult->hdResult.hdResult[i].confidence = (short)(recvData[6] + (recvData[7]<<8)); 00378 if ( ret != 0 ) return ret; 00379 size -= sizeof(UINT8)*8; 00380 } 00381 } 00382 00383 /* Face-related results */ 00384 for(i = 0; i < outHVCResult->fdResult.num; i++){ 00385 /* Face Detection result */ 00386 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_DETECTION)){ 00387 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00388 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00389 outHVCResult->fdResult.fcResult[i].dtResult.posX = (short)(recvData[0] + (recvData[1]<<8)); 00390 outHVCResult->fdResult.fcResult[i].dtResult.posY = (short)(recvData[2] + (recvData[3]<<8)); 00391 outHVCResult->fdResult.fcResult[i].dtResult.size = (short)(recvData[4] + (recvData[5]<<8)); 00392 outHVCResult->fdResult.fcResult[i].dtResult.confidence = (short)(recvData[6] + (recvData[7]<<8)); 00393 if ( ret != 0 ) return ret; 00394 size -= sizeof(UINT8)*8; 00395 } 00396 } 00397 00398 /* Face direction */ 00399 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_DIRECTION)){ 00400 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00401 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00402 outHVCResult->fdResult.fcResult[i].dirResult.yaw = (short)(recvData[0] + (recvData[1]<<8)); 00403 outHVCResult->fdResult.fcResult[i].dirResult.pitch = (short)(recvData[2] + (recvData[3]<<8)); 00404 outHVCResult->fdResult.fcResult[i].dirResult.roll = (short)(recvData[4] + (recvData[5]<<8)); 00405 outHVCResult->fdResult.fcResult[i].dirResult.confidence = (short)(recvData[6] + (recvData[7]<<8)); 00406 if ( ret != 0 ) return ret; 00407 size -= sizeof(UINT8)*8; 00408 } 00409 } 00410 00411 /* Age */ 00412 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_AGE_ESTIMATION)){ 00413 if ( size >= (INT32)sizeof(UINT8)*3 ) { 00414 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*3, recvData); 00415 #if(1) // Mbed 00416 outHVCResult->fdResult.fcResult[i].ageResult.age = (signed char)(recvData[0]); 00417 #else 00418 outHVCResult->fdResult.fcResult[i].ageResult.age = (char)(recvData[0]); 00419 #endif 00420 outHVCResult->fdResult.fcResult[i].ageResult.confidence = (short)(recvData[1] + (recvData[2]<<8)); 00421 if ( ret != 0 ) return ret; 00422 size -= sizeof(UINT8)*3; 00423 } 00424 } 00425 00426 /* Gender */ 00427 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_GENDER_ESTIMATION)){ 00428 if ( size >= (INT32)sizeof(UINT8)*3 ) { 00429 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*3, recvData); 00430 #if(1) // Mbed 00431 outHVCResult->fdResult.fcResult[i].genderResult.gender = (signed char)(recvData[0]); 00432 #else 00433 outHVCResult->fdResult.fcResult[i].genderResult.gender = (char)(recvData[0]); 00434 #endif 00435 outHVCResult->fdResult.fcResult[i].genderResult.confidence = (short)(recvData[1] + (recvData[2]<<8)); 00436 if ( ret != 0 ) return ret; 00437 size -= sizeof(UINT8)*3; 00438 } 00439 } 00440 00441 /* Gaze */ 00442 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_GAZE_ESTIMATION)){ 00443 if ( size >= (INT32)sizeof(UINT8)*2 ) { 00444 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*2, recvData); 00445 #if(1) // Mbed 00446 outHVCResult->fdResult.fcResult[i].gazeResult.gazeLR = (signed char)(recvData[0]); 00447 outHVCResult->fdResult.fcResult[i].gazeResult.gazeUD = (signed char)(recvData[1]); 00448 #else 00449 outHVCResult->fdResult.fcResult[i].gazeResult.gazeLR = (char)(recvData[0]); 00450 outHVCResult->fdResult.fcResult[i].gazeResult.gazeUD = (char)(recvData[1]); 00451 #endif 00452 if ( ret != 0 ) return ret; 00453 size -= sizeof(UINT8)*2; 00454 } 00455 } 00456 00457 /* Blink */ 00458 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_BLINK_ESTIMATION)){ 00459 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00460 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00461 outHVCResult->fdResult.fcResult[i].blinkResult.ratioL = (short)(recvData[0] + (recvData[1]<<8)); 00462 outHVCResult->fdResult.fcResult[i].blinkResult.ratioR = (short)(recvData[2] + (recvData[3]<<8)); 00463 if ( ret != 0 ) return ret; 00464 size -= sizeof(UINT8)*4; 00465 } 00466 } 00467 00468 /* Expression */ 00469 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_EXPRESSION_ESTIMATION)){ 00470 if ( size >= (INT32)sizeof(UINT8)*3 ) { 00471 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*3, recvData); 00472 #if(1) // Mbed 00473 outHVCResult->fdResult.fcResult[i].expressionResult.topExpression = (signed char)(recvData[0]); 00474 outHVCResult->fdResult.fcResult[i].expressionResult.topScore = (signed char)(recvData[1]); 00475 outHVCResult->fdResult.fcResult[i].expressionResult.degree = (signed char)(recvData[2]); 00476 #else 00477 outHVCResult->fdResult.fcResult[i].expressionResult.topExpression = (char)(recvData[0]); 00478 outHVCResult->fdResult.fcResult[i].expressionResult.topScore = (char)(recvData[1]); 00479 outHVCResult->fdResult.fcResult[i].expressionResult.degree = (char)(recvData[2]); 00480 #endif 00481 if ( ret != 0 ) return ret; 00482 size -= sizeof(UINT8)*3; 00483 } 00484 } 00485 00486 /* Face Recognition */ 00487 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_RECOGNITION)){ 00488 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00489 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00490 outHVCResult->fdResult.fcResult[i].recognitionResult.uid = (short)(recvData[0] + (recvData[1]<<8)); 00491 outHVCResult->fdResult.fcResult[i].recognitionResult.confidence = (short)(recvData[2] + (recvData[3]<<8)); 00492 if ( ret != 0 ) return ret; 00493 size -= sizeof(UINT8)*4; 00494 } 00495 } 00496 } 00497 00498 if(HVC_EXECUTE_IMAGE_NONE != inImage){ 00499 /* Image data */ 00500 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00501 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00502 outHVCResult->image.width = (short)(recvData[0] + (recvData[1]<<8)); 00503 outHVCResult->image.height = (short)(recvData[2] + (recvData[3]<<8)); 00504 if ( ret != 0 ) return ret; 00505 size -= sizeof(UINT8)*4; 00506 } 00507 00508 if ( size >= (INT32)sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height ) { 00509 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height, outHVCResult->image.image); 00510 if ( ret != 0 ) return ret; 00511 size -= sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height; 00512 } 00513 } 00514 return 0; 00515 } 00516 00517 /*----------------------------------------------------------------------------*/ 00518 /* HVC_ExecuteEx */ 00519 /* param : INT32 inTimeOutTime timeout time (ms) */ 00520 /* : INT32 inExec executable function */ 00521 /* : INT32 inImage image info */ 00522 /* : HVC_RESULT *outHVCResult result data */ 00523 /* : UINT8 *outStatus response code */ 00524 /* return : INT32 execution result error code */ 00525 /* : 0...normal */ 00526 /* : -1...parameter error */ 00527 /* : other...signal error */ 00528 /*----------------------------------------------------------------------------*/ 00529 INT32 HVC_ExecuteEx(INT32 inTimeOutTime, INT32 inExec, INT32 inImage, HVC_RESULT *outHVCResult, UINT8 *outStatus) 00530 { 00531 int i, j; 00532 INT32 ret = 0; 00533 INT32 size = 0; 00534 UINT8 sendData[32]; 00535 UINT8 recvData[32]; 00536 00537 if((NULL == outHVCResult) || (NULL == outStatus)){ 00538 return HVC_ERROR_PARAMETER; 00539 } 00540 00541 /* Send Execute command signal */ 00542 sendData[0] = (UINT8)(inExec&0xff); 00543 sendData[1] = (UINT8)((inExec>>8)&0xff); 00544 sendData[2] = (UINT8)(inImage&0xff); 00545 ret = HVC_SendCommand(HVC_COM_EXECUTEEX, sizeof(UINT8)*3, sendData); 00546 if ( ret != 0 ) return ret; 00547 00548 /* Receive header */ 00549 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00550 if ( ret != 0 ) return ret; 00551 00552 /* Receive result data */ 00553 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00554 outHVCResult->executedFunc = inExec; 00555 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00556 outHVCResult->bdResult.num = recvData[0]; 00557 outHVCResult->hdResult.num = recvData[1]; 00558 outHVCResult->fdResult.num = recvData[2]; 00559 if ( ret != 0 ) return ret; 00560 size -= sizeof(UINT8)*4; 00561 } 00562 00563 /* Get Human Body Detection result */ 00564 for(i = 0; i < outHVCResult->bdResult.num; i++){ 00565 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00566 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00567 outHVCResult->bdResult.bdResult[i].posX = (short)(recvData[0] + (recvData[1]<<8)); 00568 outHVCResult->bdResult.bdResult[i].posY = (short)(recvData[2] + (recvData[3]<<8)); 00569 outHVCResult->bdResult.bdResult[i].size = (short)(recvData[4] + (recvData[5]<<8)); 00570 outHVCResult->bdResult.bdResult[i].confidence = (short)(recvData[6] + (recvData[7]<<8)); 00571 if ( ret != 0 ) return ret; 00572 size -= sizeof(UINT8)*8; 00573 } 00574 } 00575 00576 /* Get Hand Detection result */ 00577 for(i = 0; i < outHVCResult->hdResult.num; i++){ 00578 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00579 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00580 outHVCResult->hdResult.hdResult[i].posX = (short)(recvData[0] + (recvData[1]<<8)); 00581 outHVCResult->hdResult.hdResult[i].posY = (short)(recvData[2] + (recvData[3]<<8)); 00582 outHVCResult->hdResult.hdResult[i].size = (short)(recvData[4] + (recvData[5]<<8)); 00583 outHVCResult->hdResult.hdResult[i].confidence = (short)(recvData[6] + (recvData[7]<<8)); 00584 if ( ret != 0 ) return ret; 00585 size -= sizeof(UINT8)*8; 00586 } 00587 } 00588 00589 /* Face-related results */ 00590 for(i = 0; i < outHVCResult->fdResult.num; i++){ 00591 /* Face Detection result */ 00592 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_DETECTION)){ 00593 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00594 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00595 outHVCResult->fdResult.fcResult[i].dtResult.posX = (short)(recvData[0] + (recvData[1]<<8)); 00596 outHVCResult->fdResult.fcResult[i].dtResult.posY = (short)(recvData[2] + (recvData[3]<<8)); 00597 outHVCResult->fdResult.fcResult[i].dtResult.size = (short)(recvData[4] + (recvData[5]<<8)); 00598 outHVCResult->fdResult.fcResult[i].dtResult.confidence = (short)(recvData[6] + (recvData[7]<<8)); 00599 if ( ret != 0 ) return ret; 00600 size -= sizeof(UINT8)*8; 00601 } 00602 } 00603 00604 /* Face direction */ 00605 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_DIRECTION)){ 00606 if ( size >= (INT32)sizeof(UINT8)*8 ) { 00607 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*8, recvData); 00608 outHVCResult->fdResult.fcResult[i].dirResult.yaw = (short)(recvData[0] + (recvData[1]<<8)); 00609 outHVCResult->fdResult.fcResult[i].dirResult.pitch = (short)(recvData[2] + (recvData[3]<<8)); 00610 outHVCResult->fdResult.fcResult[i].dirResult.roll = (short)(recvData[4] + (recvData[5]<<8)); 00611 outHVCResult->fdResult.fcResult[i].dirResult.confidence = (short)(recvData[6] + (recvData[7]<<8)); 00612 if ( ret != 0 ) return ret; 00613 size -= sizeof(UINT8)*8; 00614 } 00615 } 00616 00617 /* Age */ 00618 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_AGE_ESTIMATION)){ 00619 if ( size >= (INT32)sizeof(UINT8)*3 ) { 00620 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*3, recvData); 00621 #if(1) // Mbed 00622 outHVCResult->fdResult.fcResult[i].ageResult.age = (signed char)(recvData[0]); 00623 #else 00624 outHVCResult->fdResult.fcResult[i].ageResult.age = (char)(recvData[0]); 00625 #endif 00626 outHVCResult->fdResult.fcResult[i].ageResult.confidence = (short)(recvData[1] + (recvData[2]<<8)); 00627 if ( ret != 0 ) return ret; 00628 size -= sizeof(UINT8)*3; 00629 } 00630 } 00631 00632 /* Gender */ 00633 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_GENDER_ESTIMATION)){ 00634 if ( size >= (INT32)sizeof(UINT8)*3 ) { 00635 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*3, recvData); 00636 #if(1) // Mbed 00637 outHVCResult->fdResult.fcResult[i].genderResult.gender = (signed char)(recvData[0]); 00638 #else 00639 outHVCResult->fdResult.fcResult[i].genderResult.gender = (char)(recvData[0]); 00640 #endif 00641 outHVCResult->fdResult.fcResult[i].genderResult.confidence = (short)(recvData[1] + (recvData[2]<<8)); 00642 if ( ret != 0 ) return ret; 00643 size -= sizeof(UINT8)*3; 00644 } 00645 } 00646 00647 /* Gaze */ 00648 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_GAZE_ESTIMATION)){ 00649 if ( size >= (INT32)sizeof(UINT8)*2 ) { 00650 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*2, recvData); 00651 #if(1) // Mbed 00652 outHVCResult->fdResult.fcResult[i].gazeResult.gazeLR = (signed char)(recvData[0]); 00653 outHVCResult->fdResult.fcResult[i].gazeResult.gazeUD = (signed char)(recvData[1]); 00654 #else 00655 outHVCResult->fdResult.fcResult[i].gazeResult.gazeLR = (char)(recvData[0]); 00656 outHVCResult->fdResult.fcResult[i].gazeResult.gazeUD = (char)(recvData[1]); 00657 #endif 00658 if ( ret != 0 ) return ret; 00659 size -= sizeof(UINT8)*2; 00660 } 00661 } 00662 00663 /* Blink */ 00664 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_BLINK_ESTIMATION)){ 00665 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00666 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00667 outHVCResult->fdResult.fcResult[i].blinkResult.ratioL = (short)(recvData[0] + (recvData[1]<<8)); 00668 outHVCResult->fdResult.fcResult[i].blinkResult.ratioR = (short)(recvData[2] + (recvData[3]<<8)); 00669 if ( ret != 0 ) return ret; 00670 size -= sizeof(UINT8)*4; 00671 } 00672 } 00673 00674 /* Expression */ 00675 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_EXPRESSION_ESTIMATION)){ 00676 if ( size >= (INT32)sizeof(UINT8)*6 ) { 00677 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*6, recvData); 00678 outHVCResult->fdResult.fcResult[i].expressionResult.topExpression = -128; 00679 outHVCResult->fdResult.fcResult[i].expressionResult.topScore = -128; 00680 for(j = 0; j < 5; j++){ 00681 #if(1) // Mbed 00682 outHVCResult->fdResult.fcResult[i].expressionResult.score[j] = (signed char)(recvData[j]); 00683 #else 00684 outHVCResult->fdResult.fcResult[i].expressionResult.score[j] = (char)(recvData[j]); 00685 #endif 00686 if(outHVCResult->fdResult.fcResult[i].expressionResult.topScore < outHVCResult->fdResult.fcResult[i].expressionResult.score[j]){ 00687 outHVCResult->fdResult.fcResult[i].expressionResult.topScore = outHVCResult->fdResult.fcResult[i].expressionResult.score[j]; 00688 outHVCResult->fdResult.fcResult[i].expressionResult.topExpression = j + 1; 00689 } 00690 } 00691 #if(1) // Mbed 00692 outHVCResult->fdResult.fcResult[i].expressionResult.degree = (signed char)(recvData[5]); 00693 #else 00694 outHVCResult->fdResult.fcResult[i].expressionResult.degree = (char)(recvData[5]); 00695 #endif 00696 if ( ret != 0 ) return ret; 00697 size -= sizeof(UINT8)*6; 00698 } 00699 } 00700 00701 /* Face Recognition */ 00702 if(0 != (outHVCResult->executedFunc & HVC_ACTIV_FACE_RECOGNITION)){ 00703 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00704 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00705 outHVCResult->fdResult.fcResult[i].recognitionResult.uid = (short)(recvData[0] + (recvData[1]<<8)); 00706 outHVCResult->fdResult.fcResult[i].recognitionResult.confidence = (short)(recvData[2] + (recvData[3]<<8)); 00707 if ( ret != 0 ) return ret; 00708 size -= sizeof(UINT8)*4; 00709 } 00710 } 00711 } 00712 00713 if(HVC_EXECUTE_IMAGE_NONE != inImage){ 00714 /* Image data */ 00715 if ( size >= (INT32)sizeof(UINT8)*4 ) { 00716 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 00717 outHVCResult->image.width = (short)(recvData[0] + (recvData[1]<<8)); 00718 outHVCResult->image.height = (short)(recvData[2] + (recvData[3]<<8)); 00719 if ( ret != 0 ) return ret; 00720 size -= sizeof(UINT8)*4; 00721 } 00722 00723 if ( size >= (INT32)sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height ) { 00724 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height, outHVCResult->image.image); 00725 if ( ret != 0 ) return ret; 00726 size -= sizeof(UINT8)*outHVCResult->image.width*outHVCResult->image.height; 00727 } 00728 } 00729 return 0; 00730 } 00731 00732 /*----------------------------------------------------------------------------*/ 00733 /* HVC_SetThreshold */ 00734 /* param : INT32 inTimeOutTime timeout time (ms) */ 00735 /* : HVC_THRESHOLD *inThreshold threshold values */ 00736 /* : UINT8 *outStatus response code */ 00737 /* return : INT32 execution result error code */ 00738 /* : 0...normal */ 00739 /* : -1...parameter error */ 00740 /* : other...signal error */ 00741 /*----------------------------------------------------------------------------*/ 00742 INT32 HVC_SetThreshold(INT32 inTimeOutTime, HVC_THRESHOLD *inThreshold, UINT8 *outStatus) 00743 { 00744 INT32 ret = 0; 00745 INT32 size = 0; 00746 UINT8 sendData[32]; 00747 00748 if((NULL == inThreshold) || (NULL == outStatus)){ 00749 return HVC_ERROR_PARAMETER; 00750 } 00751 00752 sendData[0] = (UINT8)(inThreshold->bdThreshold&0xff); 00753 sendData[1] = (UINT8)((inThreshold->bdThreshold>>8)&0xff); 00754 sendData[2] = (UINT8)(inThreshold->hdThreshold&0xff); 00755 sendData[3] = (UINT8)((inThreshold->hdThreshold>>8)&0xff); 00756 sendData[4] = (UINT8)(inThreshold->dtThreshold&0xff); 00757 sendData[5] = (UINT8)((inThreshold->dtThreshold>>8)&0xff); 00758 sendData[6] = (UINT8)(inThreshold->rsThreshold&0xff); 00759 sendData[7] = (UINT8)((inThreshold->rsThreshold>>8)&0xff); 00760 /* Send SetThreshold command signal */ 00761 ret = HVC_SendCommand(HVC_COM_SET_THRESHOLD, sizeof(UINT8)*8, sendData); 00762 if ( ret != 0 ) return ret; 00763 00764 /* Receive header */ 00765 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00766 if ( ret != 0 ) return ret; 00767 return 0; 00768 } 00769 00770 /*----------------------------------------------------------------------------*/ 00771 /* HVC_GetThreshold */ 00772 /* param : INT32 inTimeOutTime timeout time (ms) */ 00773 /* : HVC_THRESHOLD *outThreshold threshold values */ 00774 /* : UINT8 *outStatus response code */ 00775 /* return : INT32 execution result error code */ 00776 /* : 0...normal */ 00777 /* : -1...parameter error */ 00778 /* : other...signal error */ 00779 /*----------------------------------------------------------------------------*/ 00780 INT32 HVC_GetThreshold(INT32 inTimeOutTime, HVC_THRESHOLD *outThreshold, UINT8 *outStatus) 00781 { 00782 INT32 ret = 0; 00783 INT32 size = 0; 00784 UINT8 recvData[32]; 00785 00786 if((NULL == outThreshold) || (NULL == outStatus)){ 00787 return HVC_ERROR_PARAMETER; 00788 } 00789 00790 /* Send GetThreshold command signal */ 00791 ret = HVC_SendCommand(HVC_COM_GET_THRESHOLD, 0, NULL); 00792 if ( ret != 0 ) return ret; 00793 00794 /* Receive header */ 00795 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00796 if ( ret != 0 ) return ret; 00797 00798 if ( size > (INT32)sizeof(UINT8)*8 ) { 00799 size = sizeof(UINT8)*8; 00800 } 00801 00802 /* Receive data */ 00803 ret = HVC_ReceiveData(inTimeOutTime, size, recvData); 00804 outThreshold->bdThreshold = recvData[0] + (recvData[1]<<8); 00805 outThreshold->hdThreshold = recvData[2] + (recvData[3]<<8); 00806 outThreshold->dtThreshold = recvData[4] + (recvData[5]<<8); 00807 outThreshold->rsThreshold = recvData[6] + (recvData[7]<<8); 00808 return ret; 00809 } 00810 00811 /*----------------------------------------------------------------------------*/ 00812 /* HVC_SetSizeRange */ 00813 /* param : INT32 inTimeOutTime timeout time (ms) */ 00814 /* : HVC_SIZERANGE *inSizeRange detection sizes */ 00815 /* : UINT8 *outStatus response code */ 00816 /* return : INT32 execution result error code */ 00817 /* : 0...normal */ 00818 /* : -1...parameter error */ 00819 /* : other...signal error */ 00820 /*----------------------------------------------------------------------------*/ 00821 INT32 HVC_SetSizeRange(INT32 inTimeOutTime, HVC_SIZERANGE *inSizeRange, UINT8 *outStatus) 00822 { 00823 INT32 ret = 0; 00824 INT32 size = 0; 00825 UINT8 sendData[32]; 00826 00827 if((NULL == inSizeRange) || (NULL == outStatus)){ 00828 return HVC_ERROR_PARAMETER; 00829 } 00830 00831 sendData[0] = (UINT8)(inSizeRange->bdMinSize&0xff); 00832 sendData[1] = (UINT8)((inSizeRange->bdMinSize>>8)&0xff); 00833 sendData[2] = (UINT8)(inSizeRange->bdMaxSize&0xff); 00834 sendData[3] = (UINT8)((inSizeRange->bdMaxSize>>8)&0xff); 00835 sendData[4] = (UINT8)(inSizeRange->hdMinSize&0xff); 00836 sendData[5] = (UINT8)((inSizeRange->hdMinSize>>8)&0xff); 00837 sendData[6] = (UINT8)(inSizeRange->hdMaxSize&0xff); 00838 sendData[7] = (UINT8)((inSizeRange->hdMaxSize>>8)&0xff); 00839 sendData[8] = (UINT8)(inSizeRange->dtMinSize&0xff); 00840 sendData[9] = (UINT8)((inSizeRange->dtMinSize>>8)&0xff); 00841 sendData[10] = (UINT8)(inSizeRange->dtMaxSize&0xff); 00842 sendData[11] = (UINT8)((inSizeRange->dtMaxSize>>8)&0xff); 00843 /* Send SetSizeRange command signal */ 00844 ret = HVC_SendCommand(HVC_COM_SET_SIZE_RANGE, sizeof(UINT8)*12, sendData); 00845 if ( ret != 0 ) return ret; 00846 00847 /* Receive header */ 00848 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00849 if ( ret != 0 ) return ret; 00850 return 0; 00851 } 00852 00853 /*----------------------------------------------------------------------------*/ 00854 /* HVC_GetSizeRange */ 00855 /* param : INT32 inTimeOutTime timeout time (ms) */ 00856 /* : HVC_SIZERANGE *outSizeRange detection sizes */ 00857 /* : UINT8 *outStatus response code */ 00858 /* return : INT32 execution result error code */ 00859 /* : 0...normal */ 00860 /* : -1...parameter error */ 00861 /* : other...signal error */ 00862 /*----------------------------------------------------------------------------*/ 00863 INT32 HVC_GetSizeRange(INT32 inTimeOutTime, HVC_SIZERANGE *outSizeRange, UINT8 *outStatus) 00864 { 00865 INT32 ret = 0; 00866 INT32 size = 0; 00867 UINT8 recvData[32]; 00868 00869 if((NULL == outSizeRange) || (NULL == outStatus)){ 00870 return HVC_ERROR_PARAMETER; 00871 } 00872 00873 /* Send GetSizeRange command signal */ 00874 ret = HVC_SendCommand(HVC_COM_GET_SIZE_RANGE, 0, NULL); 00875 if ( ret != 0 ) return ret; 00876 00877 /* Receive header */ 00878 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00879 if ( ret != 0 ) return ret; 00880 00881 if ( size > (INT32)sizeof(UINT8)*12 ) { 00882 size = sizeof(UINT8)*12; 00883 } 00884 00885 /* Receive data */ 00886 ret = HVC_ReceiveData(inTimeOutTime, size, recvData); 00887 outSizeRange->bdMinSize = recvData[0] + (recvData[1]<<8); 00888 outSizeRange->bdMaxSize = recvData[2] + (recvData[3]<<8); 00889 outSizeRange->hdMinSize = recvData[4] + (recvData[5]<<8); 00890 outSizeRange->hdMaxSize = recvData[6] + (recvData[7]<<8); 00891 outSizeRange->dtMinSize = recvData[8] + (recvData[9]<<8); 00892 outSizeRange->dtMaxSize = recvData[10] + (recvData[11]<<8); 00893 return ret; 00894 } 00895 00896 /*----------------------------------------------------------------------------*/ 00897 /* HVC_SetFaceDetectionAngle */ 00898 /* param : INT32 inTimeOutTime timeout time (ms) */ 00899 /* : INT32 inPose Yaw angle range */ 00900 /* : INT32 inAngle Roll angle range */ 00901 /* : UINT8 *outStatus response code */ 00902 /* return : INT32 execution result error code */ 00903 /* : 0...normal */ 00904 /* : -1...parameter error */ 00905 /* : other...signal error */ 00906 /*----------------------------------------------------------------------------*/ 00907 INT32 HVC_SetFaceDetectionAngle(INT32 inTimeOutTime, INT32 inPose, INT32 inAngle, UINT8 *outStatus) 00908 { 00909 INT32 ret = 0; 00910 INT32 size = 0; 00911 UINT8 sendData[32]; 00912 00913 if(NULL == outStatus){ 00914 return HVC_ERROR_PARAMETER; 00915 } 00916 00917 sendData[0] = (UINT8)(inPose&0xff); 00918 sendData[1] = (UINT8)(inAngle&0xff); 00919 /* Send SetFaceDetectionAngle command signal */ 00920 ret = HVC_SendCommand(HVC_COM_SET_DETECTION_ANGLE, sizeof(UINT8)*2, sendData); 00921 if ( ret != 0 ) return ret; 00922 00923 /* Receive header */ 00924 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00925 if ( ret != 0 ) return ret; 00926 return 0; 00927 } 00928 00929 /*----------------------------------------------------------------------------*/ 00930 /* HVC_GetFaceDetectionAngle */ 00931 /* param : INT32 inTimeOutTime timeout time (ms) */ 00932 /* : INT32 *outPose Yaw angle range */ 00933 /* : INT32 *outAngle Roll angle range */ 00934 /* : UINT8 *outStatus response code */ 00935 /* return : INT32 execution result error code */ 00936 /* : 0...normal */ 00937 /* : -1...parameter error */ 00938 /* : other...signal error */ 00939 /*----------------------------------------------------------------------------*/ 00940 INT32 HVC_GetFaceDetectionAngle(INT32 inTimeOutTime, INT32 *outPose, INT32 *outAngle, UINT8 *outStatus) 00941 { 00942 INT32 ret = 0; 00943 INT32 size = 0; 00944 UINT8 recvData[32]; 00945 00946 if((NULL == outPose) || (NULL == outAngle) || (NULL == outStatus)){ 00947 return HVC_ERROR_PARAMETER; 00948 } 00949 00950 /* Send GetFaceDetectionAngle signal command */ 00951 ret = HVC_SendCommand(HVC_COM_GET_DETECTION_ANGLE, 0, NULL); 00952 if ( ret != 0 ) return ret; 00953 00954 /* Receive header */ 00955 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00956 if ( ret != 0 ) return ret; 00957 00958 if ( size > (INT32)sizeof(UINT8)*2 ) { 00959 size = sizeof(UINT8)*2; 00960 } 00961 00962 /* Receive data */ 00963 ret = HVC_ReceiveData(inTimeOutTime, size, recvData); 00964 *outPose = recvData[0]; 00965 *outAngle = recvData[1]; 00966 return ret; 00967 } 00968 00969 /*----------------------------------------------------------------------------*/ 00970 /* HVC_SetBaudRate */ 00971 /* param : INT32 inTimeOutTime timeout time (ms) */ 00972 /* : INT32 inRate Baudrate */ 00973 /* : UINT8 *outStatus response code */ 00974 /* return : INT32 execution result error code */ 00975 /* : 0...normal */ 00976 /* : -1...parameter error */ 00977 /* : other...signal error */ 00978 /*----------------------------------------------------------------------------*/ 00979 INT32 HVC_SetBaudRate(INT32 inTimeOutTime, INT32 inRate, UINT8 *outStatus) 00980 { 00981 INT32 ret = 0; 00982 INT32 size = 0; 00983 UINT8 sendData[32]; 00984 00985 if(NULL == outStatus){ 00986 return HVC_ERROR_PARAMETER; 00987 } 00988 00989 sendData[0] = (UINT8)(inRate&0xff); 00990 /* Send SetBaudRate command signal */ 00991 ret = HVC_SendCommand(HVC_COM_SET_BAUDRATE, sizeof(UINT8), sendData); 00992 if ( ret != 0 ) return ret; 00993 00994 /* Receive header */ 00995 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 00996 if ( ret != 0 ) return ret; 00997 return 0; 00998 } 00999 01000 /*----------------------------------------------------------------------------*/ 01001 /* HVC_Registration */ 01002 /* param : INT32 inTimeOutTime timeout time (ms) */ 01003 /* : INT32 inUserID User ID (0-499) */ 01004 /* : INT32 inDataID Data ID (0-9) */ 01005 /* : HVC_IMAGE *outImage image info */ 01006 /* : UINT8 *outStatus response code */ 01007 /* return : INT32 execution result error code */ 01008 /* : 0...normal */ 01009 /* : -1...parameter error */ 01010 /* : other...signal error */ 01011 /*----------------------------------------------------------------------------*/ 01012 INT32 HVC_Registration(INT32 inTimeOutTime, INT32 inUserID, INT32 inDataID, HVC_IMAGE *outImage, UINT8 *outStatus) 01013 { 01014 INT32 ret = 0; 01015 INT32 size = 0; 01016 UINT8 sendData[32]; 01017 UINT8 recvData[32]; 01018 01019 if((NULL == outImage) || (NULL == outStatus)){ 01020 return HVC_ERROR_PARAMETER; 01021 } 01022 01023 /* Send Registration signal command */ 01024 sendData[0] = (UINT8)(inUserID&0xff); 01025 sendData[1] = (UINT8)((inUserID>>8)&0xff); 01026 sendData[2] = (UINT8)(inDataID&0xff); 01027 ret = HVC_SendCommand(HVC_COM_REGISTRATION, sizeof(UINT8)*3, sendData); 01028 if ( ret != 0 ) return ret; 01029 01030 /* Receive header */ 01031 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01032 if ( ret != 0 ) return ret; 01033 01034 /* Receive data */ 01035 if ( size >= (INT32)sizeof(UINT8)*4 ) { 01036 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, recvData); 01037 outImage->width = recvData[0] + (recvData[1]<<8); 01038 outImage->height = recvData[2] + (recvData[3]<<8); 01039 if ( ret != 0 ) return ret; 01040 size -= sizeof(UINT8)*4; 01041 } 01042 01043 /* Image data */ 01044 if ( size >= (INT32)sizeof(UINT8)*64*64 ) { 01045 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*64*64, outImage->image); 01046 if ( ret != 0 ) return ret; 01047 size -= sizeof(UINT8)*64*64; 01048 } 01049 return 0; 01050 } 01051 01052 /*----------------------------------------------------------------------------*/ 01053 /* HVC_DeleteData */ 01054 /* param : INT32 inTimeOutTime timeout time (ms) */ 01055 /* : INT32 inUserID User ID (0-499) */ 01056 /* : INT32 inDataID Data ID (0-9) */ 01057 /* : UINT8 *outStatus response code */ 01058 /* return : INT32 execution result error code */ 01059 /* : 0...normal */ 01060 /* : -1...parameter error */ 01061 /* : other...signal error */ 01062 /*----------------------------------------------------------------------------*/ 01063 INT32 HVC_DeleteData(INT32 inTimeOutTime, INT32 inUserID, INT32 inDataID, UINT8 *outStatus) 01064 { 01065 INT32 ret = 0; 01066 INT32 size = 0; 01067 UINT8 sendData[32]; 01068 01069 if(NULL == outStatus){ 01070 return HVC_ERROR_PARAMETER; 01071 } 01072 01073 /* Send Delete Data signal command */ 01074 sendData[0] = (UINT8)(inUserID&0xff); 01075 sendData[1] = (UINT8)((inUserID>>8)&0xff); 01076 sendData[2] = (UINT8)(inDataID&0xff); 01077 ret = HVC_SendCommand(HVC_COM_DELETE_DATA, sizeof(UINT8)*3, sendData); 01078 if ( ret != 0 ) return ret; 01079 01080 /* Receive header */ 01081 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01082 if ( ret != 0 ) return ret; 01083 return 0; 01084 } 01085 01086 /*----------------------------------------------------------------------------*/ 01087 /* HVC_DeleteUser */ 01088 /* param : INT32 inTimeOutTime timeout time (ms) */ 01089 /* : INT32 inUserID User ID (0-499) */ 01090 /* : UINT8 *outStatus response code */ 01091 /* return : INT32 execution result error code */ 01092 /* : 0...normal */ 01093 /* : -1...parameter error */ 01094 /* : other...signal error */ 01095 /*----------------------------------------------------------------------------*/ 01096 INT32 HVC_DeleteUser(INT32 inTimeOutTime, INT32 inUserID, UINT8 *outStatus) 01097 { 01098 INT32 ret = 0; 01099 INT32 size = 0; 01100 UINT8 sendData[32]; 01101 01102 if(NULL == outStatus){ 01103 return HVC_ERROR_PARAMETER; 01104 } 01105 01106 /* Send Delete User signal command */ 01107 sendData[0] = (UINT8)(inUserID&0xff); 01108 sendData[1] = (UINT8)((inUserID>>8)&0xff); 01109 ret = HVC_SendCommand(HVC_COM_DELETE_USER, sizeof(UINT8)*2, sendData); 01110 if ( ret != 0 ) return ret; 01111 01112 /* Receive header */ 01113 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01114 if ( ret != 0 ) return ret; 01115 return 0; 01116 } 01117 01118 /*----------------------------------------------------------------------------*/ 01119 /* HVC_DeleteAll */ 01120 /* param : INT32 inTimeOutTime timeout time (ms) */ 01121 /* : UINT8 *outStatus response code */ 01122 /* return : INT32 execution result error code */ 01123 /* : 0...normal */ 01124 /* : -1...parameter error */ 01125 /* : other...signal error */ 01126 /*----------------------------------------------------------------------------*/ 01127 INT32 HVC_DeleteAll(INT32 inTimeOutTime, UINT8 *outStatus) 01128 { 01129 INT32 ret = 0; 01130 INT32 size = 0; 01131 01132 if(NULL == outStatus){ 01133 return HVC_ERROR_PARAMETER; 01134 } 01135 01136 /* Send Delete All signal command */ 01137 ret = HVC_SendCommand(HVC_COM_DELETE_ALL, 0, NULL); 01138 if ( ret != 0 ) return ret; 01139 01140 /* Receive header */ 01141 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01142 if ( ret != 0 ) return ret; 01143 return 0; 01144 } 01145 01146 /*----------------------------------------------------------------------------*/ 01147 /* HVC_GetUserData */ 01148 /* param : INT32 inTimeOutTime timeout time (ms) */ 01149 /* : INT32 inUserID User ID (0-499) */ 01150 /* : INT32 *outDataNo Registration Info */ 01151 /* : UINT8 *outStatus response code */ 01152 /* return : INT32 execution result error code */ 01153 /* : 0...normal */ 01154 /* : -1...parameter error */ 01155 /* : other...signal error */ 01156 /*----------------------------------------------------------------------------*/ 01157 INT32 HVC_GetUserData(INT32 inTimeOutTime, INT32 inUserID, INT32 *outDataNo, UINT8 *outStatus) 01158 { 01159 INT32 ret = 0; 01160 INT32 size = 0; 01161 UINT8 sendData[8]; 01162 UINT8 recvData[8]; 01163 01164 if((NULL == outDataNo) || (NULL == outStatus)){ 01165 return HVC_ERROR_PARAMETER; 01166 } 01167 01168 /* Send Get Registration Info signal command */ 01169 sendData[0] = (UINT8)(inUserID&0xff); 01170 sendData[1] = (UINT8)((inUserID>>8)&0xff); 01171 ret = HVC_SendCommand(HVC_COM_GET_PERSON_DATA, sizeof(UINT8)*2, sendData); 01172 if ( ret != 0 ) return ret; 01173 01174 /* Receive header */ 01175 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01176 if ( ret != 0 ) return ret; 01177 01178 if ( size > (INT32)sizeof(UINT8)*2 ) { 01179 size = sizeof(UINT8)*2; 01180 } 01181 01182 /* Receive data */ 01183 ret = HVC_ReceiveData(inTimeOutTime, size, recvData); 01184 *outDataNo = recvData[0] + (recvData[1]<<8); 01185 return ret; 01186 } 01187 01188 /*----------------------------------------------------------------------------*/ 01189 /* HVC_SaveAlbum */ 01190 /* param : INT32 inTimeOutTime timeout time (ms) */ 01191 /* : UINT8 *outAlbumData Album data */ 01192 /* : INT32 *outAlbumDataSize Album data size */ 01193 /* : UINT8 *outStatus response code */ 01194 /* return : INT32 execution result error code */ 01195 /* : 0...normal */ 01196 /* : -1...parameter error */ 01197 /* : other...signal error */ 01198 /*----------------------------------------------------------------------------*/ 01199 INT32 HVC_SaveAlbum(INT32 inTimeOutTime, UINT8 *outAlbumData, INT32 *outAlbumDataSize, UINT8 *outStatus) 01200 { 01201 INT32 ret = 0; 01202 INT32 size = 0; 01203 01204 UINT8 *tmpAlbumData = NULL;; 01205 01206 if((NULL == outAlbumData) || (NULL == outAlbumDataSize) || (NULL == outStatus)){ 01207 return HVC_ERROR_PARAMETER; 01208 } 01209 01210 /* Send Save Album signal command */ 01211 ret = HVC_SendCommand(HVC_COM_SAVE_ALBUM, 0, NULL); 01212 if ( ret != 0 ) return ret; 01213 01214 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01215 if ( ret != 0 ) return ret; 01216 01217 if ( size >= (INT32)sizeof(UINT8)*8 + HVC_ALBUM_SIZE_MIN ) { 01218 *outAlbumDataSize = size; 01219 tmpAlbumData = outAlbumData; 01220 01221 do{ 01222 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, tmpAlbumData); 01223 if ( ret != 0 ) return ret; 01224 tmpAlbumData += sizeof(UINT8)*4; 01225 01226 ret = HVC_ReceiveData(inTimeOutTime, sizeof(UINT8)*4, tmpAlbumData); 01227 if ( ret != 0 ) return ret; 01228 tmpAlbumData += sizeof(UINT8)*4; 01229 01230 ret = HVC_ReceiveData(inTimeOutTime, size - sizeof(UINT8)*8, tmpAlbumData); 01231 if ( ret != 0 ) return ret; 01232 }while(0); 01233 } 01234 return ret; 01235 } 01236 01237 01238 /*----------------------------------------------------------------------------*/ 01239 /* HVC_LoadAlbum */ 01240 /* param : INT32 inTimeOutTime timeout time (ms) */ 01241 /* : UINT8 *inAlbumData Album data */ 01242 /* : INT32 inAlbumDataSize Album data size */ 01243 /* : UINT8 *outStatus response code */ 01244 /* return : INT32 execution result error code */ 01245 /* : 0...normal */ 01246 /* : -1...parameter error */ 01247 /* : other...signal error */ 01248 /*----------------------------------------------------------------------------*/ 01249 INT32 HVC_LoadAlbum(INT32 inTimeOutTime, UINT8 *inAlbumData, INT32 inAlbumDataSize, UINT8 *outStatus) 01250 { 01251 INT32 ret = 0; 01252 INT32 size = 0; 01253 01254 if((NULL == inAlbumData) || (NULL == outStatus)){ 01255 return HVC_ERROR_PARAMETER; 01256 } 01257 01258 /* Send Save Album signal command */ 01259 ret = HVC_SendCommandOfLoadAlbum(HVC_COM_LOAD_ALBUM, inAlbumDataSize, inAlbumData); 01260 if ( ret != 0 ) return ret; 01261 01262 /* Receive header */ 01263 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01264 if ( ret != 0 ) return ret; 01265 01266 return ret; 01267 } 01268 01269 /*----------------------------------------------------------------------------*/ 01270 /* HVC_WriteAlbum */ 01271 /* param : INT32 inTimeOutTime timeout time (ms) */ 01272 /* : UINT8 *outStatus response code */ 01273 /* return : INT32 execution result error code */ 01274 /* : 0...normal */ 01275 /* : -1...parameter error */ 01276 /* : other...signal error */ 01277 /*----------------------------------------------------------------------------*/ 01278 INT32 HVC_WriteAlbum(INT32 inTimeOutTime, UINT8 *outStatus) 01279 { 01280 INT32 ret = 0; 01281 INT32 size = 0; 01282 01283 if(NULL == outStatus){ 01284 return HVC_ERROR_PARAMETER; 01285 } 01286 01287 /* Send Write Album signal command */ 01288 ret = HVC_SendCommand(HVC_COM_WRITE_ALBUM, 0, NULL); 01289 if ( ret != 0 ) return ret; 01290 01291 /* Receive header */ 01292 ret = HVC_ReceiveHeader(inTimeOutTime, &size, outStatus); 01293 if ( ret != 0 ) return ret; 01294 01295 return ret; 01296 } 01297
Generated on Fri Jul 15 2022 01:15:47 by
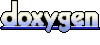