
This sample will play a ".wav" file of the USB root folder. Only RIFF format.
Fork of GR-PEACH_Audio_WAV by
main.cpp
00001 #include "mbed.h" 00002 #include "SdUsbConnect.h" 00003 #include "EasyPlayback.h" 00004 #include "EasyDec_WavCnv2ch.h" 00005 00006 #define FILE_NAME_LEN (64) 00007 #define MOUNT_NAME "storage" 00008 00009 static InterruptIn skip_btn(USER_BUTTON0); 00010 static EasyPlayback AudioPlayer; 00011 00012 static void skip_btn_fall(void) { 00013 AudioPlayer.skip(); 00014 } 00015 00016 int main() { 00017 DIR * d; 00018 struct dirent * p; 00019 char file_path[sizeof("/"MOUNT_NAME"/") + FILE_NAME_LEN]; 00020 SdUsbConnect storage(MOUNT_NAME); 00021 00022 // decoder setting 00023 AudioPlayer.add_decoder<EasyDec_WavCnv2ch>(".wav"); 00024 AudioPlayer.add_decoder<EasyDec_WavCnv2ch>(".WAV"); 00025 00026 // volume control 00027 AudioPlayer.outputVolume(0.5); // Volume control (min:0.0 max:1.0) 00028 00029 // button setting 00030 skip_btn.fall(&skip_btn_fall); 00031 00032 while (1) { 00033 storage.wait_connect(); 00034 // file search 00035 d = opendir("/"MOUNT_NAME"/"); 00036 while ((p = readdir(d)) != NULL) { 00037 size_t len = strlen(p->d_name); 00038 if (len < FILE_NAME_LEN) { 00039 // make file path 00040 sprintf(file_path, "/%s/%s", MOUNT_NAME, p->d_name); 00041 printf("%s\r\n", file_path); 00042 00043 // playback 00044 AudioPlayer.play(file_path); 00045 } 00046 } 00047 closedir(d); 00048 } 00049 }
Generated on Wed Jul 13 2022 23:37:00 by
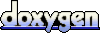