
The "GR-PEACH_Audio_Playback_7InchLCD_Sample" is a sample code that can provides high-resolution audio playback of FLAC format files. It also allows the user to audio-playback control functions such as play, pause, and stop by manipulating key switches.
Dependencies: GR-PEACH_video R_BSP TLV320_RBSP USBHost_custom
Fork of GR-PEACH_Audio_Playback_Sample by
lpc.h
00001 /* libFLAC - Free Lossless Audio Codec library 00002 * Copyright (C) 2000-2009 Josh Coalson 00003 * Copyright (C) 2011-2014 Xiph.Org Foundation 00004 * 00005 * Redistribution and use in source and binary forms, with or without 00006 * modification, are permitted provided that the following conditions 00007 * are met: 00008 * 00009 * - Redistributions of source code must retain the above copyright 00010 * notice, this list of conditions and the following disclaimer. 00011 * 00012 * - Redistributions in binary form must reproduce the above copyright 00013 * notice, this list of conditions and the following disclaimer in the 00014 * documentation and/or other materials provided with the distribution. 00015 * 00016 * - Neither the name of the Xiph.org Foundation nor the names of its 00017 * contributors may be used to endorse or promote products derived from 00018 * this software without specific prior written permission. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00023 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE FOUNDATION OR 00024 * CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00025 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00026 * PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 00027 * PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00028 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00029 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS 00030 * SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 */ 00032 00033 #ifndef FLAC__PRIVATE__LPC_H 00034 #define FLAC__PRIVATE__LPC_H 00035 00036 #ifdef HAVE_CONFIG_H 00037 #include <config.h> 00038 #endif 00039 00040 #include "private/cpu.h" 00041 #include "private/float.h" 00042 #include "FLAC/format.h" 00043 00044 #ifndef FLAC__INTEGER_ONLY_LIBRARY 00045 00046 /* 00047 * FLAC__lpc_window_data() 00048 * -------------------------------------------------------------------- 00049 * Applies the given window to the data. 00050 * OPT: asm implementation 00051 * 00052 * IN in[0,data_len-1] 00053 * IN window[0,data_len-1] 00054 * OUT out[0,lag-1] 00055 * IN data_len 00056 */ 00057 void FLAC__lpc_window_data(const FLAC__int32 in[], const FLAC__real window[], FLAC__real out[], unsigned data_len); 00058 00059 /* 00060 * FLAC__lpc_compute_autocorrelation() 00061 * -------------------------------------------------------------------- 00062 * Compute the autocorrelation for lags between 0 and lag-1. 00063 * Assumes data[] outside of [0,data_len-1] == 0. 00064 * Asserts that lag > 0. 00065 * 00066 * IN data[0,data_len-1] 00067 * IN data_len 00068 * IN 0 < lag <= data_len 00069 * OUT autoc[0,lag-1] 00070 */ 00071 void FLAC__lpc_compute_autocorrelation(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00072 #ifndef FLAC__NO_ASM 00073 # ifdef FLAC__CPU_IA32 00074 # ifdef FLAC__HAS_NASM 00075 void FLAC__lpc_compute_autocorrelation_asm_ia32(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00076 void FLAC__lpc_compute_autocorrelation_asm_ia32_sse_lag_4(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00077 void FLAC__lpc_compute_autocorrelation_asm_ia32_sse_lag_8(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00078 void FLAC__lpc_compute_autocorrelation_asm_ia32_sse_lag_12(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00079 void FLAC__lpc_compute_autocorrelation_asm_ia32_sse_lag_16(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00080 # endif 00081 # endif 00082 # if (defined FLAC__CPU_IA32 || defined FLAC__CPU_X86_64) && defined FLAC__HAS_X86INTRIN 00083 # ifdef FLAC__SSE_SUPPORTED 00084 void FLAC__lpc_compute_autocorrelation_intrin_sse_lag_4(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00085 void FLAC__lpc_compute_autocorrelation_intrin_sse_lag_8(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00086 void FLAC__lpc_compute_autocorrelation_intrin_sse_lag_12(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00087 void FLAC__lpc_compute_autocorrelation_intrin_sse_lag_16(const FLAC__real data[], unsigned data_len, unsigned lag, FLAC__real autoc[]); 00088 # endif 00089 # endif 00090 #endif 00091 00092 /* 00093 * FLAC__lpc_compute_lp_coefficients() 00094 * -------------------------------------------------------------------- 00095 * Computes LP coefficients for orders 1..max_order. 00096 * Do not call if autoc[0] == 0.0. This means the signal is zero 00097 * and there is no point in calculating a predictor. 00098 * 00099 * IN autoc[0,max_order] autocorrelation values 00100 * IN 0 < max_order <= FLAC__MAX_LPC_ORDER max LP order to compute 00101 * OUT lp_coeff[0,max_order-1][0,max_order-1] LP coefficients for each order 00102 * *** IMPORTANT: 00103 * *** lp_coeff[0,max_order-1][max_order,FLAC__MAX_LPC_ORDER-1] are untouched 00104 * OUT error[0,max_order-1] error for each order (more 00105 * specifically, the variance of 00106 * the error signal times # of 00107 * samples in the signal) 00108 * 00109 * Example: if max_order is 9, the LP coefficients for order 9 will be 00110 * in lp_coeff[8][0,8], the LP coefficients for order 8 will be 00111 * in lp_coeff[7][0,7], etc. 00112 */ 00113 void FLAC__lpc_compute_lp_coefficients(const FLAC__real autoc[], unsigned *max_order, FLAC__real lp_coeff[][FLAC__MAX_LPC_ORDER], FLAC__double error[]); 00114 00115 /* 00116 * FLAC__lpc_quantize_coefficients() 00117 * -------------------------------------------------------------------- 00118 * Quantizes the LP coefficients. NOTE: precision + bits_per_sample 00119 * must be less than 32 (sizeof(FLAC__int32)*8). 00120 * 00121 * IN lp_coeff[0,order-1] LP coefficients 00122 * IN order LP order 00123 * IN FLAC__MIN_QLP_COEFF_PRECISION < precision 00124 * desired precision (in bits, including sign 00125 * bit) of largest coefficient 00126 * OUT qlp_coeff[0,order-1] quantized coefficients 00127 * OUT shift # of bits to shift right to get approximated 00128 * LP coefficients. NOTE: could be negative. 00129 * RETURN 0 => quantization OK 00130 * 1 => coefficients require too much shifting for *shift to 00131 * fit in the LPC subframe header. 'shift' is unset. 00132 * 2 => coefficients are all zero, which is bad. 'shift' is 00133 * unset. 00134 */ 00135 int FLAC__lpc_quantize_coefficients(const FLAC__real lp_coeff[], unsigned order, unsigned precision, FLAC__int32 qlp_coeff[], int *shift); 00136 00137 /* 00138 * FLAC__lpc_compute_residual_from_qlp_coefficients() 00139 * -------------------------------------------------------------------- 00140 * Compute the residual signal obtained from sutracting the predicted 00141 * signal from the original. 00142 * 00143 * IN data[-order,data_len-1] original signal (NOTE THE INDICES!) 00144 * IN data_len length of original signal 00145 * IN qlp_coeff[0,order-1] quantized LP coefficients 00146 * IN order > 0 LP order 00147 * IN lp_quantization quantization of LP coefficients in bits 00148 * OUT residual[0,data_len-1] residual signal 00149 */ 00150 void FLAC__lpc_compute_residual_from_qlp_coefficients(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00151 void FLAC__lpc_compute_residual_from_qlp_coefficients_wide(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00152 #ifndef FLAC__NO_ASM 00153 # ifdef FLAC__CPU_IA32 00154 # ifdef FLAC__HAS_NASM 00155 void FLAC__lpc_compute_residual_from_qlp_coefficients_asm_ia32(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00156 void FLAC__lpc_compute_residual_from_qlp_coefficients_asm_ia32_mmx(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00157 void FLAC__lpc_compute_residual_from_qlp_coefficients_wide_asm_ia32(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00158 # endif 00159 # endif 00160 # if (defined FLAC__CPU_IA32 || defined FLAC__CPU_X86_64) && defined FLAC__HAS_X86INTRIN 00161 # ifdef FLAC__SSE2_SUPPORTED 00162 void FLAC__lpc_compute_residual_from_qlp_coefficients_16_intrin_sse2(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00163 void FLAC__lpc_compute_residual_from_qlp_coefficients_intrin_sse2(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00164 # endif 00165 # ifdef FLAC__SSE4_1_SUPPORTED 00166 void FLAC__lpc_compute_residual_from_qlp_coefficients_intrin_sse41(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00167 void FLAC__lpc_compute_residual_from_qlp_coefficients_wide_intrin_sse41(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00168 # endif 00169 # ifdef FLAC__AVX2_SUPPORTED 00170 void FLAC__lpc_compute_residual_from_qlp_coefficients_16_intrin_avx2(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00171 void FLAC__lpc_compute_residual_from_qlp_coefficients_intrin_avx2(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00172 void FLAC__lpc_compute_residual_from_qlp_coefficients_wide_intrin_avx2(const FLAC__int32 *data, unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 residual[]); 00173 # endif 00174 # endif 00175 #endif 00176 00177 #endif /* !defined FLAC__INTEGER_ONLY_LIBRARY */ 00178 00179 /* 00180 * FLAC__lpc_restore_signal() 00181 * -------------------------------------------------------------------- 00182 * Restore the original signal by summing the residual and the 00183 * predictor. 00184 * 00185 * IN residual[0,data_len-1] residual signal 00186 * IN data_len length of original signal 00187 * IN qlp_coeff[0,order-1] quantized LP coefficients 00188 * IN order > 0 LP order 00189 * IN lp_quantization quantization of LP coefficients in bits 00190 * *** IMPORTANT: the caller must pass in the historical samples: 00191 * IN data[-order,-1] previously-reconstructed historical samples 00192 * OUT data[0,data_len-1] original signal 00193 */ 00194 void FLAC__lpc_restore_signal(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00195 void FLAC__lpc_restore_signal_wide(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00196 #ifndef FLAC__NO_ASM 00197 # ifdef FLAC__CPU_IA32 00198 # ifdef FLAC__HAS_NASM 00199 void FLAC__lpc_restore_signal_asm_ia32(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00200 void FLAC__lpc_restore_signal_asm_ia32_mmx(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00201 void FLAC__lpc_restore_signal_wide_asm_ia32(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00202 # endif /* FLAC__HAS_NASM */ 00203 # endif /* FLAC__CPU_IA32 */ 00204 # if (defined FLAC__CPU_IA32 || defined FLAC__CPU_X86_64) && defined FLAC__HAS_X86INTRIN 00205 # ifdef FLAC__SSE2_SUPPORTED 00206 void FLAC__lpc_restore_signal_16_intrin_sse2(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00207 # endif 00208 # ifdef FLAC__SSE4_1_SUPPORTED 00209 void FLAC__lpc_restore_signal_wide_intrin_sse41(const FLAC__int32 residual[], unsigned data_len, const FLAC__int32 qlp_coeff[], unsigned order, int lp_quantization, FLAC__int32 data[]); 00210 # endif 00211 # endif 00212 #endif /* FLAC__NO_ASM */ 00213 00214 #ifndef FLAC__INTEGER_ONLY_LIBRARY 00215 00216 /* 00217 * FLAC__lpc_compute_expected_bits_per_residual_sample() 00218 * -------------------------------------------------------------------- 00219 * Compute the expected number of bits per residual signal sample 00220 * based on the LP error (which is related to the residual variance). 00221 * 00222 * IN lpc_error >= 0.0 error returned from calculating LP coefficients 00223 * IN total_samples > 0 # of samples in residual signal 00224 * RETURN expected bits per sample 00225 */ 00226 FLAC__double FLAC__lpc_compute_expected_bits_per_residual_sample(FLAC__double lpc_error, unsigned total_samples); 00227 FLAC__double FLAC__lpc_compute_expected_bits_per_residual_sample_with_error_scale(FLAC__double lpc_error, FLAC__double error_scale); 00228 00229 /* 00230 * FLAC__lpc_compute_best_order() 00231 * -------------------------------------------------------------------- 00232 * Compute the best order from the array of signal errors returned 00233 * during coefficient computation. 00234 * 00235 * IN lpc_error[0,max_order-1] >= 0.0 error returned from calculating LP coefficients 00236 * IN max_order > 0 max LP order 00237 * IN total_samples > 0 # of samples in residual signal 00238 * IN overhead_bits_per_order # of bits overhead for each increased LP order 00239 * (includes warmup sample size and quantized LP coefficient) 00240 * RETURN [1,max_order] best order 00241 */ 00242 unsigned FLAC__lpc_compute_best_order(const FLAC__double lpc_error[], unsigned max_order, unsigned total_samples, unsigned overhead_bits_per_order); 00243 00244 #endif /* !defined FLAC__INTEGER_ONLY_LIBRARY */ 00245 00246 #endif
Generated on Tue Jul 12 2022 19:32:30 by
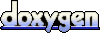