
The "GR-PEACH_Audio_Playback_7InchLCD_Sample" is a sample code that can provides high-resolution audio playback of FLAC format files. It also allows the user to audio-playback control functions such as play, pause, and stop by manipulating key switches.
Dependencies: GR-PEACH_video R_BSP TLV320_RBSP USBHost_custom
Fork of GR-PEACH_Audio_Playback_Sample by
disp_tft_scrn.cpp
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #include "disp_graphics.h" 00025 #include "disp_tft.h" 00026 #include "disp_tft_scrn.h" 00027 #include "img_tbl.h" 00028 #include "system.h" 00029 00030 /*--- Macro definition ---*/ 00031 /*-- The making phase of the display image --*/ 00032 #define SCRN_PHASE_INIT1 (0) /* 1st phase of the initialization process. */ 00033 #define SCRN_PHASE_INIT2 (1) /* 2nd phase of the initialization process. */ 00034 #define SCRN_PHASE_INIT3 (2) /* 3rd phase of the initialization process. */ 00035 #define SCRN_PHASE_INIT4 (3) /* 4th phase of the initialization process. */ 00036 #define SCRN_PHASE_INIT5 (4) /* 5th phase of the initialization process. */ 00037 #define SCRN_PHASE_INIT6 (5) /* 6th phase of the initialization process. */ 00038 #define SCRN_PHASE_INIT7 (6) /* 7th phase of the initialization process. */ 00039 #define SCRN_PHASE_INIT8 (7) /* 8th phase of the initialization process. */ 00040 #define SCRN_PHASE_INIT9 (8) /* 9th phase of the initialization process. */ 00041 #define SCRN_PHASE_INIT10 (9) /* 10th phase of the initialization process. */ 00042 #define SCRN_PHASE_INIT11 (10) /* 11th phase of the initialization process. */ 00043 #define SCRN_PHASE_INIT12 (11) /* 12th phase of the initialization process. */ 00044 #define SCRN_PHASE_INIT13 (12) /* 13th phase of the initialization process. */ 00045 #define SCRN_PHASE_INIT14 (13) /* 14th phase of the initialization process. */ 00046 #define SCRN_PHASE_INIT15 (14) /* 15th phase of the initialization process. */ 00047 #define SCRN_PHASE_INIT16 (15) /* 16th phase of the initialization process. */ 00048 #define SCRN_PHASE_INIT17 (16) /* 17th phase of the initialization process. */ 00049 #define SCRN_PHASE_INIT18 (17) /* 18th phase of the initialization process. */ 00050 #define SCRN_PHASE_INIT19 (18) /* 19th phase of the initialization process. */ 00051 #define SCRN_PHASE_INIT20 (19) /* 20th phase of the initialization process. */ 00052 #define SCRN_PHASE_INIT21 (20) /* 21th phase of the initialization process. */ 00053 #define SCRN_PHASE_INIT22 (21) /* 22th phase of the initialization process. */ 00054 #define SCRN_PHASE_INIT23 (22) /* 23th phase of the initialization process. */ 00055 #define SCRN_PHASE_INIT24 (23) /* 24th phase of the initialization process. */ 00056 #define SCRN_PHASE_NORM1 (24) /* 1st phase of the normal process. */ 00057 #define SCRN_PHASE_NORM2 (25) /* 2nd phase of the normal process. */ 00058 #define SCRN_PHASE_NORM3 (26) /* 3rd phase of the normal process. */ 00059 #define SCRN_PHASE_NORM4 (27) /* 4th phase of the normal process. */ 00060 #define SCRN_PHASE_NORM5 (28) /* 5th phase of the normal process. */ 00061 #define SCRN_PHASE_NORM6 (29) /* 6th phase of the normal process. */ 00062 00063 /*-- Display position of the picture --*/ 00064 /* DSP_IMG_ID_BACK1 */ 00065 #define XPOS_BACK1 ( 0) 00066 #define YPOS_BACK1 ( 0) 00067 00068 /* DSP_IMG_ID_BACK2 */ 00069 #define XPOS_BACK2 (XPOS_BACK1) 00070 #define YPOS_BACK2 (YPOS_BACK1 + (int32_t)DSP_IMG_HS_BACK1) 00071 00072 /* DSP_IMG_ID_BACK3 */ 00073 #define XPOS_BACK3 (XPOS_BACK2) 00074 #define YPOS_BACK3 (YPOS_BACK2 + (int32_t)DSP_IMG_HS_BACK2) 00075 00076 /* DSP_IMG_ID_BACK4 */ 00077 #define XPOS_BACK4 (XPOS_BACK3) 00078 #define YPOS_BACK4 (YPOS_BACK3 + (int32_t)DSP_IMG_HS_BACK3) 00079 00080 /* DSP_IMG_ID_BACK5 */ 00081 #define XPOS_BACK5 (XPOS_BACK4) 00082 #define YPOS_BACK5 (YPOS_BACK4 + (int32_t)DSP_IMG_HS_BACK4) 00083 00084 /* DSP_IMG_ID_BACK6 */ 00085 #define XPOS_BACK6 (XPOS_BACK5) 00086 #define YPOS_BACK6 (YPOS_BACK5 + (int32_t)DSP_IMG_HS_BACK5) 00087 00088 /* DSP_IMG_ID_AUDIO */ 00089 #define XPOS_AUDIO ( 20) 00090 #define YPOS_AUDIO ( 25) 00091 00092 /* DSP_IMG_ID_RENESAS */ 00093 #define XPOS_RENESAS (260) 00094 #define YPOS_RENESAS ( 25) 00095 00096 /* DSP_IMG_ID_AUDIO_BUTTON1 */ 00097 #define XPOS_BUTTON1 (230) 00098 #define YPOS_BUTTON1 (410) 00099 00100 /* DSP_IMG_ID_AUDIO_BUTTON2 */ 00101 #define XPOS_BUTTON2 (370) 00102 #define YPOS_BUTTON2 (YPOS_BUTTON1) 00103 00104 /* DSP_IMG_ID_AUDIO_BUTTON3 */ 00105 #define XPOS_BUTTON3 (510) 00106 #define YPOS_BUTTON3 (YPOS_BUTTON1) 00107 00108 /* DSP_IMG_ID_AUDIO_BUTTON4 */ 00109 #define XPOS_BUTTON4 (650) 00110 #define YPOS_BUTTON4 (YPOS_BUTTON1) 00111 00112 /* DSP_IMG_ID_AUDIO_BAR */ 00113 #define XPOS_AUDIO_BAR (240) 00114 #define YPOS_AUDIO_BAR (385) 00115 00116 /* DSP_IMG_ID_AUDIO_WINDOW1 */ 00117 #define XPOS_AUDIO_WINDOW1 (0) 00118 #define YPOS_AUDIO_WINDOW1 (105) 00119 00120 /* DSP_IMG_ID_AUDIO_WINDOW2 */ 00121 #define XPOS_AUDIO_WINDOW2 (XPOS_AUDIO_WINDOW1) 00122 #define YPOS_AUDIO_WINDOW2 (YPOS_AUDIO_WINDOW1 + (int32_t)DSP_IMG_HS_AUDIO_WINDOW1) 00123 00124 /* DSP_IMG_ID_AUDIO_WINDOW3 */ 00125 #define XPOS_AUDIO_WINDOW3 (XPOS_AUDIO_WINDOW2) 00126 #define YPOS_AUDIO_WINDOW3 (YPOS_AUDIO_WINDOW2 + (int32_t)DSP_IMG_HS_AUDIO_WINDOW2) 00127 00128 /* DSP_IMG_ID_AUDIO_WINDOW4 */ 00129 #define XPOS_AUDIO_WINDOW4 (XPOS_AUDIO_WINDOW3) 00130 #define YPOS_AUDIO_WINDOW4 (YPOS_AUDIO_WINDOW3 + (int32_t)DSP_IMG_HS_AUDIO_WINDOW3) 00131 00132 /* DSP_IMG_ID_MODE */ 00133 #define XPOS_MODE (30) 00134 #define YPOS_MODE (380) 00135 00136 /* DSP_IMG_ID_TV */ 00137 #define XPOS_TV (60) 00138 #define YPOS_TV (400) 00139 00140 /* Mode Number */ 00141 #define XPOS_MODE_NUMBER (90) 00142 #define YPOS_MODE_NUMBER (410) 00143 #define MSG_FORM_MODE "%ld" 00144 00145 /* DSP_IMG_ID_BAR_LEFT */ 00146 #define OFFSET_BAR_FROM_AUDIO_BAR (2) /* offset level from audio bar */ 00147 00148 #define XPOS_BAR_LEFT (XPOS_AUDIO_BAR + OFFSET_BAR_FROM_AUDIO_BAR) 00149 #define YPOS_BAR_LEFT (YPOS_AUDIO_BAR + OFFSET_BAR_FROM_AUDIO_BAR) 00150 00151 /* DSP_IMG_ID_BAR_MID */ 00152 #define XPOS_BAR_MID (int32_t)((uint32_t)XPOS_BAR_LEFT + DSP_IMG_WS_BAR_LEFT) 00153 #define YPOS_BAR_MID (YPOS_BAR_LEFT) 00154 00155 /* DSP_IMG_ID_BAR_RIGHT */ 00156 #define XPOS_BAR_RIGHT (XPOS_BAR_MID) 00157 #define YPOS_BAR_RIGHT (YPOS_BAR_MID) 00158 00159 /* The range of the bar movement on the audio-bar. */ 00160 #define MOV_RANGE_BAR \ 00161 ((DSP_IMG_WS_AUDIO_BAR - (DSP_IMG_WS_BAR_LEFT + DSP_IMG_WS_BAR_RIGHT)) - \ 00162 (OFFSET_BAR_FROM_AUDIO_BAR + OFFSET_BAR_FROM_AUDIO_BAR)) 00163 00164 /* Display position of text in the case of display mode 1. */ 00165 /* File Number */ 00166 #define M1_XPOS_FILE_NUM (15) 00167 #define M1_YPOS_FILE_NUM (130) 00168 #define M1_MSG_FORM_FILE_NUM "T%03ld" 00169 00170 /* Playback Time */ 00171 #define M1_XPOS_PLAY_TIME (165) 00172 #define M1_YPOS_PLAY_TIME (M1_YPOS_FILE_NUM) 00173 #define M1_MSG_FORM_PLAY_TIME "%3ld:%02ld:%02ld" 00174 00175 /* Total playback Time */ 00176 #define M1_XPOS_TOTAL_TIME (435) 00177 #define M1_YPOS_TOTAL_TIME (M1_YPOS_FILE_NUM) 00178 #define M1_MSG_FORM_TOTAL_TIME " / %3ld:%02ld:%02ld" 00179 00180 /* EIGHTH NOTE */ 00181 #define M1_XPOS_EIGHTH_NOTE (15) 00182 #define M1_YPOS_EIGHTH_NOTE (210) 00183 00184 /* FILE NAME */ 00185 #define M1_XPOS_FILE_NAME (45) 00186 #define M1_YPOS_FILE_NAME (M1_YPOS_EIGHTH_NOTE) 00187 00188 /* PLAY INFO */ 00189 #define M1_XPOS_PLAY_INFO (15) 00190 #define M1_YPOS_PLAY_INFO (290) 00191 #define M1_MSG_FORM_PLAY_INFO "FLAC %5ld Hz, %s" 00192 00193 /* Channel number */ 00194 #define M1_STR_CHANNEL_MONO "Mono" 00195 #define M1_STR_CHANNEL_STEREO "Stereo" 00196 00197 /* Display position of text in the case of display mode 2. */ 00198 /* File Number */ 00199 #define M2_XPOS_FILE_NUM (150) 00200 #define M2_YPOS_FILE_NUM (320) 00201 #define M2_MSG_FORM_FILE_NUM "T%03ld" 00202 00203 /* Playback Time */ 00204 #define M2_XPOS_PLAY_TIME (450) 00205 #define M2_YPOS_PLAY_TIME (M2_YPOS_FILE_NUM) 00206 #define M2_MSG_FORM_PLAY_TIME "%3ld:%02ld:%02ld" 00207 00208 /* L-ch */ 00209 #define M2_XPOS_TEXT_LCH (150) 00210 #define M2_YPOS_TEXT_LCH (130) 00211 #define M2_STR_TEXT_LCH "L-ch" 00212 #define M2_XPOS_AUDIO_LCH (0) 00213 #define M2_YPOS_AUDIO_LCH (238) 00214 #define M2_XSIZE_AUDIO_LCH (1) 00215 #define M2_COL_AUDIO_LCH (0xFF00FF00u) 00216 00217 /* R-ch */ 00218 #define M2_XPOS_TEXT_RCH (530) 00219 #define M2_YPOS_TEXT_RCH (M2_YPOS_TEXT_LCH) 00220 #define M2_STR_TEXT_RCH "R-ch" 00221 #define M2_XPOS_AUDIO_RCH (401) 00222 #define M2_YPOS_AUDIO_RCH (M2_YPOS_AUDIO_LCH) 00223 #define M2_XSIZE_AUDIO_RCH (M2_XSIZE_AUDIO_LCH) 00224 #define M2_COL_AUDIO_RCH (0xFF0000FFu) 00225 00226 /* Center of the vertical line */ 00227 #define M2_XPOS_VLINE (399) 00228 #define M2_YPOS_VLINE (110) 00229 #define M2_XSIZE_VLINE (2) 00230 #define M2_YSIZE_VLINE (256) 00231 #define M2_COL_VLINE (DSP_IMG_FONT_FG_COL) 00232 00233 /* Center of the horizontal line */ 00234 #define M2_XPOS_HLINE (M2_XPOS_AUDIO_LCH) 00235 #define M2_YPOS_HLINE (M2_YPOS_AUDIO_LCH) 00236 #define M2_XSIZE_HLINE (800) 00237 #define M2_YSIZE_HLINE (1) 00238 #define M2_COL_HLINE (0xFFF02020u) 00239 00240 /* Display position of text in the case of display mode 3. */ 00241 /* File Number */ 00242 #define M3_XPOS_FILE_NUM (15) 00243 #define M3_YPOS_FILE_NUM (130) 00244 #define M3_MSG_FORM_FILE_NUM "T%03ld" 00245 00246 /* Playback Time */ 00247 #define M3_XPOS_PLAY_TIME (0) 00248 #define M3_YPOS_PLAY_TIME (335) 00249 #define M3_MSG_FORM_PLAY_TIME "%3ld:%02ld:%02ld" 00250 00251 /* L-ch + R-ch */ 00252 #define M3_XPOS_AUDIO (0) 00253 #define M3_YPOS_AUDIO (238) 00254 #define M3_XSIZE_AUDIO (1) 00255 #define M3_COL_AUDIO (0xFF00FF00u) 00256 00257 /* Center of the vertical line */ 00258 #define M3_XPOS_VLINE (M3_XPOS_PLAY_TIME) 00259 #define M3_YPOS_VLINE (110) 00260 #define M3_XSIZE_VLINE (2) 00261 #define M3_YSIZE_VLINE (256) 00262 #define M3_COL_VLINE (DSP_IMG_FONT_FG_COL) 00263 00264 /* Center of the horizontal line */ 00265 #define M3_XPOS_HLINE (M3_XPOS_AUDIO) 00266 #define M3_YPOS_HLINE (M3_YPOS_AUDIO) 00267 #define M3_XSIZE_HLINE (800) 00268 #define M3_YSIZE_HLINE (1) 00269 #define M3_COL_HLINE (0xFFF02020u) 00270 00271 /* Interval in the seconds between the vertical line. */ 00272 #define M3_INTERVAL_TIME_SEC (5u) 00273 #define M3_INTERVAL_VLINE (M3_INTERVAL_TIME_SEC * DSP_TFT_M3_AUDIO_SAMPLE_PER_SEC) 00274 /* Maximum number of the drawing process of the vertical line. */ 00275 #define M3_MAX_DRAW_NUM_VLINE ((DSP_TFT_WIDTH / M3_INTERVAL_VLINE) + 1u) 00276 00277 /* Fixed character string. */ 00278 /* The maximum length of the file name in case of the display. */ 00279 #define FILE_NAME_MAX_LEN (25) 00280 00281 /* The mark of eighth note */ 00282 #define STR_EIGHTH_NOTE "\x1" 00283 00284 /* The number of key picture */ 00285 #define KEY_PIC_NUM (5) 00286 00287 /* Converts the playback time. */ 00288 #define HOUR_TO_SEC (3600u) /* Coefficient for conversion from hour to seconds. */ 00289 #define MIN_TO_SEC (60u) /* Coefficient for conversion from minute to seconds. */ 00290 00291 static uint32_t get_time_hour(const uint32_t play_time); 00292 static uint32_t get_time_min(const uint32_t play_time); 00293 static uint32_t get_time_sec(const uint32_t play_time); 00294 static uint32_t make_scrn_mode(const dsp_com_ctrl_t * const p_com, 00295 const dsp_tft_ctrl_t * const p_tft); 00296 static uint32_t make_scrn_mode1(const dsp_com_ctrl_t * const p_com, 00297 const dsp_tft_ctrl_t * const p_tft); 00298 static uint32_t make_scrn_mode2(const dsp_com_ctrl_t * const p_com, 00299 const dsp_tft_ctrl_t * const p_tft); 00300 static uint32_t make_scrn_mode3(const dsp_com_ctrl_t * const p_com, 00301 const dsp_tft_ctrl_t * const p_tft); 00302 static void draw_audio_wave(const dsp_tftlayer_t * const p_info, const int32_t start_x, 00303 const int32_t start_y, const int32_t size_x, const int16_t audio_value, const uint32_t col_data); 00304 static bool is_playbar_output(const SYS_PlayStat stat); 00305 00306 void dsp_init_tft_scrn(dsp_tft_ctrl_t * const p_tft) 00307 { 00308 if (p_tft != NULL) { 00309 p_tft->disp_phase_no = SCRN_PHASE_INIT1; 00310 dsp_clear_tft_audio_data(p_tft); 00311 } 00312 } 00313 00314 uint32_t dsp_make_tft_scrn(const dsp_com_ctrl_t * const p_com, dsp_tft_ctrl_t * const p_tft) 00315 { 00316 uint32_t ret = DSP_TFT_LAYER_NON; 00317 int32_t img_id; 00318 uint32_t cnt_x; 00319 uint32_t offset_x; 00320 char_t str[DSP_DISP_STR_MAX_LEN]; 00321 00322 if ((p_com != NULL) && (p_tft != NULL)) { 00323 switch (p_tft->disp_phase_no) { 00324 case SCRN_PHASE_INIT1: 00325 case SCRN_PHASE_INIT13: 00326 /* Outputs the picture. */ 00327 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK1, 00328 YPOS_BACK1, DSP_IMG_ID_BACK1, DSP_IMG_FORM_AUTOSEL); 00329 break; 00330 case SCRN_PHASE_INIT2: 00331 case SCRN_PHASE_INIT14: 00332 /* Outputs the picture. */ 00333 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK2, 00334 YPOS_BACK2, DSP_IMG_ID_BACK2, DSP_IMG_FORM_AUTOSEL); 00335 break; 00336 case SCRN_PHASE_INIT3: 00337 case SCRN_PHASE_INIT15: 00338 /* Outputs the picture. */ 00339 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK3, 00340 YPOS_BACK3, DSP_IMG_ID_BACK3, DSP_IMG_FORM_AUTOSEL); 00341 break; 00342 case SCRN_PHASE_INIT4: 00343 case SCRN_PHASE_INIT16: 00344 /* Outputs the picture. */ 00345 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK4, 00346 YPOS_BACK4, DSP_IMG_ID_BACK4, DSP_IMG_FORM_AUTOSEL); 00347 break; 00348 case SCRN_PHASE_INIT5: 00349 case SCRN_PHASE_INIT17: 00350 /* Outputs the picture. */ 00351 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK5, 00352 YPOS_BACK5, DSP_IMG_ID_BACK5, DSP_IMG_FORM_AUTOSEL); 00353 break; 00354 case SCRN_PHASE_INIT6: 00355 case SCRN_PHASE_INIT18: 00356 /* Outputs the picture. */ 00357 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_BACK6, 00358 YPOS_BACK6, DSP_IMG_ID_BACK6, DSP_IMG_FORM_AUTOSEL); 00359 break; 00360 case SCRN_PHASE_INIT7: 00361 case SCRN_PHASE_INIT19: 00362 /* Outputs the picture. */ 00363 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO_WINDOW1, 00364 YPOS_AUDIO_WINDOW1, DSP_IMG_ID_AUDIO_WINDOW1, DSP_IMG_FORM_AUTOSEL); 00365 break; 00366 case SCRN_PHASE_INIT8: 00367 case SCRN_PHASE_INIT20: 00368 /* Outputs the picture. */ 00369 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO_WINDOW2, 00370 YPOS_AUDIO_WINDOW2, DSP_IMG_ID_AUDIO_WINDOW2, DSP_IMG_FORM_AUTOSEL); 00371 break; 00372 case SCRN_PHASE_INIT9: 00373 case SCRN_PHASE_INIT21: 00374 /* Outputs the picture. */ 00375 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO_WINDOW3, 00376 YPOS_AUDIO_WINDOW3, DSP_IMG_ID_AUDIO_WINDOW3, DSP_IMG_FORM_AUTOSEL); 00377 break; 00378 case SCRN_PHASE_INIT10: 00379 case SCRN_PHASE_INIT22: 00380 /* Outputs the picture. */ 00381 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO_WINDOW4, 00382 YPOS_AUDIO_WINDOW4, DSP_IMG_ID_AUDIO_WINDOW4, DSP_IMG_FORM_AUTOSEL); 00383 break; 00384 case SCRN_PHASE_INIT11: 00385 case SCRN_PHASE_INIT23: 00386 /* Outputs the picture. */ 00387 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO_BAR, 00388 YPOS_AUDIO_BAR, DSP_IMG_ID_AUDIO_BAR, DSP_IMG_FORM_AUTOSEL); 00389 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_MODE, 00390 YPOS_MODE, DSP_IMG_ID_MODE, DSP_IMG_FORM_AUTOSEL); 00391 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_TV, 00392 YPOS_TV, DSP_IMG_ID_TV, DSP_IMG_FORM_AUTOSEL); 00393 00394 dsp_clear_all(&p_tft->tft_info[DSP_TFT_LAYER_1]); 00395 ret = DSP_TFT_LAYER_1; 00396 break; 00397 case SCRN_PHASE_INIT12: 00398 case SCRN_PHASE_INIT24: 00399 /* Outputs the picture. */ 00400 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_AUDIO, 00401 YPOS_AUDIO, DSP_IMG_ID_AUDIO, DSP_IMG_FORM_AUTOSEL); 00402 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_0], XPOS_RENESAS, 00403 YPOS_RENESAS, DSP_IMG_ID_RENESAS, DSP_IMG_FORM_AUTOSEL); 00404 ret = DSP_TFT_LAYER_0; 00405 break; 00406 case SCRN_PHASE_NORM1: 00407 /* Outputs the picture. */ 00408 /* Prev key */ 00409 if (p_tft->key_code == SYS_KEYCODE_PREV) { 00410 img_id = DSP_IMG_ID_AUDIO_BUTTON1D; 00411 } else { 00412 img_id = DSP_IMG_ID_AUDIO_BUTTON1; 00413 } 00414 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BUTTON1, 00415 YPOS_BUTTON1, img_id, DSP_IMG_FORM_ARGB8888_NO_BLEND); 00416 break; 00417 case SCRN_PHASE_NORM2: 00418 /* Outputs the picture. */ 00419 /* Play/Pause key */ 00420 if (p_tft->key_code == SYS_KEYCODE_PLAYPAUSE) { 00421 img_id = DSP_IMG_ID_AUDIO_BUTTON2D; 00422 } else { 00423 img_id = DSP_IMG_ID_AUDIO_BUTTON2; 00424 } 00425 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BUTTON2, 00426 YPOS_BUTTON2, img_id, DSP_IMG_FORM_ARGB8888_NO_BLEND); 00427 break; 00428 case SCRN_PHASE_NORM3: 00429 /* Outputs the picture. */ 00430 /* Next key */ 00431 if (p_tft->key_code == SYS_KEYCODE_NEXT) { 00432 img_id = DSP_IMG_ID_AUDIO_BUTTON3D; 00433 } else { 00434 img_id = DSP_IMG_ID_AUDIO_BUTTON3; 00435 } 00436 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BUTTON3, 00437 YPOS_BUTTON3, img_id, DSP_IMG_FORM_ARGB8888_NO_BLEND); 00438 break; 00439 case SCRN_PHASE_NORM4: 00440 /* Outputs the picture. */ 00441 /* Repeat key */ 00442 if (p_tft->key_code == SYS_KEYCODE_REPEAT) { 00443 img_id = DSP_IMG_ID_AUDIO_BUTTON4D; 00444 } else { 00445 img_id = DSP_IMG_ID_AUDIO_BUTTON4; 00446 } 00447 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BUTTON4, 00448 YPOS_BUTTON4, img_id, DSP_IMG_FORM_ARGB8888_NO_BLEND); 00449 break; 00450 case SCRN_PHASE_NORM5: 00451 /* Clears the img_audio_window picture area. */ 00452 dsp_clear_area(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_AUDIO_WINDOW1, 00453 YPOS_AUDIO_WINDOW1, DSP_IMG_WS_AUDIO_WINDOW, DSP_IMG_HS_AUDIO_WINDOW); 00454 /* Outputs the picture of the play bar. */ 00455 if (is_playbar_output(p_com->play_stat) == true) { 00456 /* The bar indicates the position of current playback time */ 00457 /* in total playback time. */ 00458 if (p_com->total_time != 0u) { 00459 offset_x = (MOV_RANGE_BAR * p_com->play_time) / p_com->total_time; 00460 } else { 00461 offset_x = 0u; 00462 } 00463 /* LEFT */ 00464 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BAR_LEFT, 00465 YPOS_BAR_LEFT, DSP_IMG_ID_BAR_LEFT, DSP_IMG_FORM_AUTOSEL); 00466 /* MID */ 00467 for (cnt_x = 0; cnt_x < offset_x; cnt_x += DSP_IMG_WS_BAR_MID) { 00468 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BAR_MID + (int32_t)cnt_x, 00469 YPOS_BAR_MID, DSP_IMG_ID_BAR_MID, DSP_IMG_FORM_AUTOSEL); 00470 } 00471 /* RIGHT */ 00472 dsp_draw_picture(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_BAR_RIGHT + (int32_t)offset_x, 00473 YPOS_BAR_RIGHT, DSP_IMG_ID_BAR_RIGHT, DSP_IMG_FORM_AUTOSEL); 00474 } 00475 00476 ret = make_scrn_mode(p_com, p_tft); 00477 break; 00478 case SCRN_PHASE_NORM6: 00479 /* Clears the img_tv picture area. */ 00480 dsp_clear_area(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_TV, 00481 YPOS_TV, DSP_IMG_WS_TV, DSP_IMG_HS_TV); 00482 /* Outputs a display mode number. */ 00483 (void) sprintf(str, MSG_FORM_MODE, p_com->disp_mode); 00484 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], XPOS_MODE_NUMBER, YPOS_MODE_NUMBER, str); 00485 00486 ret = make_scrn_mode(p_com, p_tft); 00487 break; 00488 default: 00489 ret = DSP_TFT_LAYER_NON; 00490 break; 00491 } 00492 00493 if (p_tft->disp_phase_no < SCRN_PHASE_NORM6) { 00494 p_tft->disp_phase_no++; 00495 } else { 00496 p_tft->disp_phase_no = SCRN_PHASE_NORM1; 00497 } 00498 } 00499 return ret; 00500 } 00501 00502 SYS_KeyCode dsp_convert_key_tft_scrn(const uint32_t pos_x, const uint32_t pos_y) 00503 { 00504 static const dsp_cnv_key_t cnv_tbl[KEY_PIC_NUM] = { 00505 { /* Prev key */ 00506 XPOS_BUTTON1, /* X position */ 00507 YPOS_BUTTON1, /* Y position */ 00508 DSP_IMG_WS_AUDIO_BUTTON1, /* Width size */ 00509 DSP_IMG_HS_AUDIO_BUTTON1, /* Height size */ 00510 SYS_KEYCODE_PREV /* Key code */ 00511 }, 00512 { /* Play/Pause key */ 00513 XPOS_BUTTON2, /* X position */ 00514 YPOS_BUTTON2, /* Y position */ 00515 DSP_IMG_WS_AUDIO_BUTTON2, /* Width size */ 00516 DSP_IMG_HS_AUDIO_BUTTON2, /* Height size */ 00517 SYS_KEYCODE_PLAYPAUSE /* Key code */ 00518 }, 00519 { /* Next key */ 00520 XPOS_BUTTON3, /* X position */ 00521 YPOS_BUTTON3, /* Y position */ 00522 DSP_IMG_WS_AUDIO_BUTTON3, /* Width size */ 00523 DSP_IMG_HS_AUDIO_BUTTON3, /* Height size */ 00524 SYS_KEYCODE_NEXT /* Key code */ 00525 }, 00526 { /* Repeat key */ 00527 XPOS_BUTTON4, /* X position */ 00528 YPOS_BUTTON4, /* Y position */ 00529 DSP_IMG_WS_AUDIO_BUTTON4, /* Width size */ 00530 DSP_IMG_HS_AUDIO_BUTTON4, /* Height size */ 00531 SYS_KEYCODE_REPEAT /* Key code */ 00532 }, 00533 { /* Mode key */ 00534 XPOS_MODE, /* X position */ 00535 YPOS_MODE, /* Y position */ 00536 DSP_IMG_WS_MODE, /* Width size */ 00537 DSP_IMG_HS_MODE, /* Height size */ 00538 SYS_KEYCODE_MODE /* Key code */ 00539 } 00540 }; 00541 int32_t i; 00542 SYS_KeyCode ret; 00543 00544 ret = SYS_KEYCODE_NON; 00545 for (i = 0; (i < KEY_PIC_NUM) && (ret == SYS_KEYCODE_NON); i++) { 00546 /* Is X position within the picture of key? */ 00547 if ((pos_x >= cnv_tbl[i].pic_pos_x) && 00548 (pos_x <= (cnv_tbl[i].pic_pos_x + cnv_tbl[i].pic_siz_x))) { 00549 /* Is Y position within the picture of key? */ 00550 if ((pos_y >= cnv_tbl[i].pic_pos_y) && 00551 (pos_y <= (cnv_tbl[i].pic_pos_y + cnv_tbl[i].pic_siz_y))) { 00552 /* Decides the key code. */ 00553 ret = cnv_tbl[i].key_code; 00554 } 00555 } 00556 } 00557 return ret; 00558 } 00559 00560 /** Creates the display image of playback screen. 00561 * 00562 * @param p_com Pointer to common data in all module. 00563 * @param p_tft Pointer to management data of TFT module. 00564 * 00565 * @returns 00566 * Returns the number of the display layer to update. 00567 */ 00568 static uint32_t make_scrn_mode(const dsp_com_ctrl_t * const p_com, 00569 const dsp_tft_ctrl_t * const p_tft) 00570 { 00571 uint32_t ret = DSP_TFT_LAYER_NON; 00572 00573 if ((p_com != NULL) && (p_tft != NULL)) { 00574 switch (p_com->disp_mode) { 00575 case DSP_DISPMODE_1: 00576 ret = make_scrn_mode1(p_com, p_tft); 00577 break; 00578 case DSP_DISPMODE_2: 00579 ret = make_scrn_mode2(p_com, p_tft); 00580 break; 00581 case DSP_DISPMODE_3: 00582 ret = make_scrn_mode3(p_com, p_tft); 00583 break; 00584 default: 00585 ret = DSP_TFT_LAYER_NON; 00586 break; 00587 } 00588 } 00589 return ret; 00590 } 00591 00592 /** Creates the display image of TFT display mode 1. 00593 * 00594 * @param p_com Pointer to common data in all module. 00595 * @param p_tft Pointer to management data of TFT module. 00596 * 00597 * @returns 00598 * Returns the number of the display layer to update. 00599 */ 00600 static uint32_t make_scrn_mode1(const dsp_com_ctrl_t * const p_com, 00601 const dsp_tft_ctrl_t * const p_tft) 00602 { 00603 uint32_t ret = DSP_TFT_LAYER_NON; 00604 uint32_t time_h; 00605 uint32_t time_m; 00606 uint32_t time_s; 00607 char_t str[DSP_DISP_STR_MAX_LEN]; 00608 const char_t *p_str; 00609 00610 if ((p_com != NULL) && (p_tft != NULL)) { 00611 switch (p_tft->disp_phase_no) { 00612 case SCRN_PHASE_NORM5: 00613 /* Outputs the mark of eighth note. */ 00614 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00615 M1_XPOS_EIGHTH_NOTE, M1_YPOS_EIGHTH_NOTE, STR_EIGHTH_NOTE); 00616 00617 /* Outputs a file name in less than 25 characters. */ 00618 (void) strncpy(str, p_com->file_name, FILE_NAME_MAX_LEN); 00619 str[FILE_NAME_MAX_LEN] = '\0'; 00620 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00621 M1_XPOS_FILE_NAME, M1_YPOS_FILE_NAME, str); 00622 break; 00623 case SCRN_PHASE_NORM6: 00624 /* Outputs the file number. */ 00625 (void) sprintf(str, M1_MSG_FORM_FILE_NUM, p_com->track_id); 00626 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00627 M1_XPOS_FILE_NUM, M1_YPOS_FILE_NUM, str); 00628 00629 /* Outputs the playback time. */ 00630 time_h = get_time_hour(p_com->play_time); 00631 time_m = get_time_min(p_com->play_time); 00632 time_s = get_time_sec(p_com->play_time); 00633 (void) sprintf(str, M1_MSG_FORM_PLAY_TIME, time_h, time_m, time_s); 00634 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00635 M1_XPOS_PLAY_TIME, M1_YPOS_PLAY_TIME, str); 00636 00637 /* Outputs the total playback time. */ 00638 time_h = get_time_hour(p_com->total_time); 00639 time_m = get_time_min(p_com->total_time); 00640 time_s = get_time_sec(p_com->total_time); 00641 (void) sprintf(str, M1_MSG_FORM_TOTAL_TIME, time_h, time_m, time_s); 00642 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00643 M1_XPOS_TOTAL_TIME, M1_YPOS_TOTAL_TIME, str); 00644 00645 /* Outputs the playback information. */ 00646 if ((p_com->channel > 0u) && (p_com->samp_freq > 0u)) { 00647 if (p_com->channel == 1u) { 00648 p_str = M1_STR_CHANNEL_MONO; 00649 } else { 00650 p_str = M1_STR_CHANNEL_STEREO; 00651 } 00652 (void) sprintf(str, M1_MSG_FORM_PLAY_INFO, p_com->samp_freq, p_str); 00653 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00654 M1_XPOS_PLAY_INFO, M1_YPOS_PLAY_INFO, str); 00655 } 00656 ret = DSP_TFT_LAYER_1; 00657 break; 00658 default: 00659 ret = DSP_TFT_LAYER_NON; 00660 break; 00661 } 00662 } 00663 return ret; 00664 } 00665 00666 /** Creates the display image of TFT display mode 2. 00667 * 00668 * @param p_com Pointer to common data in all module. 00669 * @param p_tft Pointer to management data of TFT module. 00670 * 00671 * @returns 00672 * Returns the number of the display layer to update. 00673 */ 00674 static uint32_t make_scrn_mode2(const dsp_com_ctrl_t * const p_com, 00675 const dsp_tft_ctrl_t * const p_tft) 00676 { 00677 uint32_t ret = DSP_TFT_LAYER_NON; 00678 uint32_t time_h; 00679 uint32_t time_m; 00680 uint32_t time_s; 00681 int32_t xpos_offset; 00682 int32_t buf_id; 00683 char_t str[DSP_DISP_STR_MAX_LEN]; 00684 const dsp_audio_t *p_aud; 00685 00686 if ((p_com != NULL) && (p_tft != NULL)) { 00687 switch (p_tft->disp_phase_no) { 00688 case SCRN_PHASE_NORM5: 00689 /* Outputs the audio wave. */ 00690 p_aud = &p_tft->audio_data; 00691 buf_id = 0; 00692 while (buf_id < (int32_t)p_aud->m2_buf_cnt) { 00693 xpos_offset = buf_id; 00694 /* L-ch */ 00695 draw_audio_wave(&p_tft->tft_info[DSP_TFT_LAYER_1], M2_XPOS_AUDIO_LCH + xpos_offset, 00696 M2_YPOS_AUDIO_LCH, M2_XSIZE_AUDIO_LCH, p_aud->m2_buf[buf_id], M2_COL_AUDIO_LCH); 00697 buf_id++; 00698 /* R-ch */ 00699 draw_audio_wave(&p_tft->tft_info[DSP_TFT_LAYER_1], M2_XPOS_AUDIO_RCH + xpos_offset, 00700 M2_YPOS_AUDIO_RCH, M2_XSIZE_AUDIO_RCH, p_aud->m2_buf[buf_id], M2_COL_AUDIO_RCH); 00701 buf_id++; 00702 } 00703 /* Outputs the center line. */ 00704 dsp_fill_rect(&p_tft->tft_info[DSP_TFT_LAYER_1], M2_XPOS_VLINE, 00705 M2_YPOS_VLINE, M2_XSIZE_VLINE, M2_YSIZE_VLINE, M2_COL_VLINE); 00706 dsp_fill_rect(&p_tft->tft_info[DSP_TFT_LAYER_1], M2_XPOS_HLINE, 00707 M2_YPOS_HLINE, M2_XSIZE_HLINE, M2_YSIZE_HLINE, M2_COL_HLINE); 00708 break; 00709 case SCRN_PHASE_NORM6: 00710 /* Outputs the text of the channel. */ 00711 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00712 M2_XPOS_TEXT_LCH, M2_YPOS_TEXT_LCH, M2_STR_TEXT_LCH); 00713 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00714 M2_XPOS_TEXT_RCH, M2_YPOS_TEXT_RCH, M2_STR_TEXT_RCH); 00715 00716 /* Outputs the file number. */ 00717 (void) sprintf(str, M2_MSG_FORM_FILE_NUM, p_com->track_id); 00718 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00719 M2_XPOS_FILE_NUM, M2_YPOS_FILE_NUM, str); 00720 00721 /* Outputs the playback time. */ 00722 time_h = get_time_hour(p_com->play_time); 00723 time_m = get_time_min(p_com->play_time); 00724 time_s = get_time_sec(p_com->play_time); 00725 (void) sprintf(str, M2_MSG_FORM_PLAY_TIME, time_h, time_m, time_s); 00726 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00727 M2_XPOS_PLAY_TIME, M2_YPOS_PLAY_TIME, str); 00728 ret = DSP_TFT_LAYER_1; 00729 break; 00730 default: 00731 ret = DSP_TFT_LAYER_NON; 00732 break; 00733 } 00734 } 00735 return ret; 00736 } 00737 00738 /** Creates the display image of TFT display mode 3. 00739 * 00740 * @param p_com Pointer to common data in all module. 00741 * @param p_tft Pointer to management data of TFT module. 00742 * 00743 * @returns 00744 * Returns the number of the display layer to update. 00745 */ 00746 static uint32_t make_scrn_mode3(const dsp_com_ctrl_t * const p_com, 00747 const dsp_tft_ctrl_t * const p_tft) 00748 { 00749 uint32_t ret = DSP_TFT_LAYER_NON; 00750 uint32_t i; 00751 uint32_t time_h; 00752 uint32_t time_m; 00753 uint32_t time_s; 00754 uint32_t xpos_time; 00755 int32_t xpos_offset; 00756 int32_t mod; 00757 char_t str[DSP_DISP_STR_MAX_LEN]; 00758 const dsp_audio_t *p_aud; 00759 00760 if ((p_com != NULL) && (p_tft != NULL)) { 00761 switch (p_tft->disp_phase_no) { 00762 case SCRN_PHASE_NORM5: 00763 p_aud = &p_tft->audio_data; 00764 for (i = 0u; i < p_aud->m3_buf_cnt; i++) { 00765 draw_audio_wave(&p_tft->tft_info[DSP_TFT_LAYER_1], M3_XPOS_AUDIO + (int32_t) i, 00766 M3_YPOS_AUDIO, M3_XSIZE_AUDIO, p_aud->m3_buf[i], M3_COL_AUDIO); 00767 } 00768 /* Calculates the display position. */ 00769 if (p_aud->m3_sample_cnt < DSP_TFT_M3_AUDIO_BUF_SIZE) { 00770 xpos_offset = 0; 00771 xpos_time = 0u; 00772 } else { 00773 mod = (int32_t) (p_aud->m3_sample_cnt % M3_INTERVAL_VLINE); 00774 xpos_offset = -mod; 00775 /* Calculates the current time. */ 00776 time_s = (p_aud->m3_sample_cnt - DSP_TFT_M3_AUDIO_BUF_SIZE) - (uint32_t) mod; 00777 xpos_time = time_s / DSP_TFT_M3_AUDIO_SAMPLE_PER_SEC; 00778 } 00779 for (i = 0u; i < M3_MAX_DRAW_NUM_VLINE; i++) { 00780 /* Outputs the vertical line. */ 00781 dsp_fill_rect(&p_tft->tft_info[DSP_TFT_LAYER_1], M3_XPOS_VLINE + xpos_offset, 00782 M3_YPOS_VLINE, M3_XSIZE_VLINE, M3_YSIZE_VLINE, M3_COL_VLINE); 00783 /* Outputs the playback time. */ 00784 time_h = get_time_hour(xpos_time); 00785 time_m = get_time_min(xpos_time); 00786 time_s = get_time_sec(xpos_time); 00787 (void) sprintf(str, M3_MSG_FORM_PLAY_TIME, time_h, time_m, time_s); 00788 dsp_draw_text15x17(&p_tft->tft_info[DSP_TFT_LAYER_1], 00789 M3_XPOS_PLAY_TIME + xpos_offset, M3_YPOS_PLAY_TIME, str); 00790 xpos_offset += (int32_t) M3_INTERVAL_VLINE; 00791 xpos_time += M3_INTERVAL_TIME_SEC; 00792 } 00793 break; 00794 case SCRN_PHASE_NORM6: 00795 /* Outputs the horizontal line. */ 00796 dsp_fill_rect(&p_tft->tft_info[DSP_TFT_LAYER_1], M3_XPOS_HLINE, 00797 M3_YPOS_HLINE, M3_XSIZE_HLINE, M3_YSIZE_HLINE, M3_COL_HLINE); 00798 /* Outputs the file number. */ 00799 (void) sprintf(str, M3_MSG_FORM_FILE_NUM, p_com->track_id); 00800 dsp_draw_text30x34(&p_tft->tft_info[DSP_TFT_LAYER_1], 00801 M3_XPOS_FILE_NUM, M3_YPOS_FILE_NUM, str); 00802 ret = DSP_TFT_LAYER_1; 00803 break; 00804 default: 00805 ret = DSP_TFT_LAYER_NON; 00806 break; 00807 } 00808 } 00809 00810 return ret; 00811 } 00812 00813 /** Draws the audio wave to VRAM. 00814 * 00815 * @param p_info Pointer to VRAM structure 00816 * @param start_x Display position X of the picture 00817 * @param start_y Display position Y of the picture 00818 * @param size_x The width of the audio wave 00819 * @param audio_value The value of the audio wave 00820 * @param col_data Color data of ARGB8888 format 00821 */ 00822 static void draw_audio_wave(const dsp_tftlayer_t * const p_info, const int32_t start_x, 00823 const int32_t start_y, const int32_t size_x, const int16_t audio_value, const uint32_t col_data) 00824 { 00825 int32_t pos_y; 00826 int32_t draw_size; 00827 00828 if (p_info != NULL) { 00829 if (audio_value >= 0) { 00830 pos_y = start_y - audio_value; 00831 draw_size = audio_value; 00832 } else { 00833 pos_y = start_y; 00834 draw_size = -audio_value; 00835 } 00836 dsp_fill_rect(p_info, start_x, pos_y, size_x, draw_size, col_data); 00837 } 00838 } 00839 00840 /** Gets the playback time. (Hours) 00841 * 00842 * @param play_time Playback time 00843 * 00844 * @returns 00845 * Hours 00846 */ 00847 static uint32_t get_time_hour(const uint32_t play_time) 00848 { 00849 return (play_time / HOUR_TO_SEC); 00850 } 00851 00852 /** Gets the playback time. (Minutes) 00853 * 00854 * @param play_time Playback time 00855 * 00856 * @returns 00857 * Minutes 00858 */ 00859 static uint32_t get_time_min(const uint32_t play_time) 00860 { 00861 return ((play_time % HOUR_TO_SEC) / MIN_TO_SEC); 00862 } 00863 00864 /** Gets the playback time. (Seconds) 00865 * 00866 * @param play_time Playback time 00867 * 00868 * @returns 00869 * Seconds 00870 */ 00871 static uint32_t get_time_sec(const uint32_t play_time) 00872 { 00873 return (play_time % MIN_TO_SEC); 00874 } 00875 00876 /** Checks the output status of play bar. 00877 * 00878 * @param stat Playback status 00879 * 00880 * @returns 00881 * true = output the play bar, false = don't output the play bar 00882 */ 00883 static bool is_playbar_output(const SYS_PlayStat stat) 00884 { 00885 bool ret; 00886 00887 switch (stat) { 00888 case SYS_PLAYSTAT_PLAY: 00889 case SYS_PLAYSTAT_PAUSE: 00890 ret = true; 00891 break; 00892 case SYS_PLAYSTAT_STOP: 00893 default: 00894 ret = false; 00895 break; 00896 } 00897 return ret; 00898 }
Generated on Tue Jul 12 2022 19:32:28 by
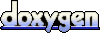