
The "GR-PEACH_Audio_Playback_7InchLCD_Sample" is a sample code that can provides high-resolution audio playback of FLAC format files. It also allows the user to audio-playback control functions such as play, pause, and stop by manipulating key switches.
Dependencies: GR-PEACH_video R_BSP TLV320_RBSP USBHost_custom
Fork of GR-PEACH_Audio_Playback_Sample by
dec_flac.h
00001 /******************************************************************************* 00002 * DISCLAIMER 00003 * This software is supplied by Renesas Electronics Corporation and is only 00004 * intended for use with Renesas products. No other uses are authorized. This 00005 * software is owned by Renesas Electronics Corporation and is protected under 00006 * all applicable laws, including copyright laws. 00007 * THIS SOFTWARE IS PROVIDED "AS IS" AND RENESAS MAKES NO WARRANTIES REGARDING 00008 * THIS SOFTWARE, WHETHER EXPRESS, IMPLIED OR STATUTORY, INCLUDING BUT NOT 00009 * LIMITED TO WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE 00010 * AND NON-INFRINGEMENT. ALL SUCH WARRANTIES ARE EXPRESSLY DISCLAIMED. 00011 * TO THE MAXIMUM EXTENT PERMITTED NOT PROHIBITED BY LAW, NEITHER RENESAS 00012 * ELECTRONICS CORPORATION NOR ANY OF ITS AFFILIATED COMPANIES SHALL BE LIABLE 00013 * FOR ANY DIRECT, INDIRECT, SPECIAL, INCIDENTAL OR CONSEQUENTIAL DAMAGES FOR 00014 * ANY REASON RELATED TO THIS SOFTWARE, EVEN IF RENESAS OR ITS AFFILIATES HAVE 00015 * BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGES. 00016 * Renesas reserves the right, without notice, to make changes to this software 00017 * and to discontinue the availability of this software. By using this software, 00018 * you agree to the additional terms and conditions found by accessing the 00019 * following link: 00020 * http://www.renesas.com/disclaimer* 00021 * Copyright (C) 2015 Renesas Electronics Corporation. All rights reserved. 00022 *******************************************************************************/ 00023 00024 #ifndef DEC_FLAC_H 00025 #define DEC_FLAC_H 00026 00027 #include "r_typedefs.h" 00028 #include "stream_decoder.h" 00029 00030 /*--- User defined types ---*/ 00031 typedef struct { 00032 FLAC__StreamDecoder *p_decoder; /* Handle of flac decoder */ 00033 FILE *p_file_handle; /* Handle of flac file */ 00034 uint64_t decoded_sample; /* Number of a decoded sample */ 00035 uint64_t total_sample; /* Total number of sample */ 00036 uint32_t sample_rate; /* Sample rate in Hz */ 00037 uint32_t channel_num; /* Number of channels */ 00038 uint32_t bits_per_sample; /* bit per sample */ 00039 int32_t *p_pcm_buf; /* Pointer of PCM buffer */ 00040 uint32_t pcm_buf_num; /* Size of PCM buffer */ 00041 uint32_t pcm_buf_used_cnt; /* Counter of used elements in PCM buffer */ 00042 } flac_ctrl_t; 00043 00044 /** Sets the PCM buffer to store decoded data 00045 * 00046 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00047 * @param p_buf_addr Pointer to PCM buffer to store decoded data. 00048 * @param buf_num Elements number of PCM buffer array. 00049 * 00050 * @returns 00051 * Results of process. true is success. false is failure. 00052 */ 00053 bool flac_set_pcm_buf(flac_ctrl_t * const p_flac_ctrl, 00054 int32_t * const p_buf_addr, const uint32_t buf_num); 00055 00056 /** Gets elements number of the decoded data 00057 * 00058 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00059 * 00060 * @returns 00061 * Elements number of the decoded data. 00062 */ 00063 uint32_t flac_get_pcm_cnt(const flac_ctrl_t * const p_flac_ctrl); 00064 00065 /** Gets the playback time 00066 * 00067 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00068 * 00069 * @returns 00070 * Playback time (second). 00071 */ 00072 uint32_t flac_get_play_time(const flac_ctrl_t * const p_flac_ctrl); 00073 00074 /** Gets the total playback time 00075 * 00076 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00077 * 00078 * @returns 00079 * Total playback time (second). 00080 */ 00081 uint32_t flac_get_total_time(const flac_ctrl_t * const p_flac_ctrl); 00082 00083 /** Open the FLAC decoder 00084 * 00085 * @param p_handle Pointer to the handle of FLAC file. 00086 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00087 * 00088 * @returns 00089 * Results of process. true is success. false is failure. 00090 */ 00091 bool flac_open(FILE * const p_handle, flac_ctrl_t * const p_flac_ctrl); 00092 00093 /** Decode some audio frames. 00094 * 00095 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00096 * 00097 * @returns 00098 * Results of process. true is success. false is failure. 00099 */ 00100 bool flac_decode(const flac_ctrl_t * const p_flac_ctrl); 00101 00102 /** Close the FLAC decoder 00103 * 00104 * @param p_flac_ctrl Pointer to the control data of FLAC module. 00105 */ 00106 void flac_close(flac_ctrl_t * const p_flac_ctrl); 00107 00108 #endif /* DEC_FLAC_H */
Generated on Tue Jul 12 2022 19:32:28 by
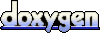