
The program demonstrates how to implement SPI on BLE Nano platform
Dependencies: mbed
spi_master.h
00001 /* 00002 00003 Copyright (c) 2012-2014 RedBearLab 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00006 and associated documentation files (the "Software"), to deal in the Software without restriction, 00007 including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, 00009 subject to the following conditions: 00010 The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. 00011 00012 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, 00013 INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR 00014 PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE 00015 FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00016 ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 00018 */ 00019 00020 #ifndef _SPI_MASTER_H_ 00021 #define _SPI_MASTER_H_ 00022 00023 #include "mbed.h" 00024 00025 #define SPI_FREQUENCY_125K 0x02000000UL 00026 #define SPI_FREQUENCY_250K 0x04000000UL 00027 #define SPI_FREQUENCY_500K 0x08000000UL 00028 #define SPI_FREQUENCY_1M 0x10000000UL 00029 #define SPI_FREQUENCY_2M 0x20000000UL 00030 #define SPI_FREQUENCY_4M 0x40000000UL 00031 #define SPI_FREQUENCY_8M 0x80000000UL 00032 00033 #define SPI_125K 0 00034 #define SPI_250K 1 00035 #define SPI_500K 2 00036 #define SPI_1M 3 00037 #define SPI_2M 4 00038 #define SPI_4M 5 00039 #define SPI_8M 6 00040 00041 #define SPI_MODE0 0 00042 #define SPI_MODE1 1 00043 #define SPI_MODE2 2 00044 #define SPI_MODE3 3 00045 00046 #define CS P0_14 00047 #define SCK P0_25 00048 #define MOSI P0_20 00049 #define MISO P0_22 00050 00051 typedef enum{ 00052 00053 MSBFIRST = 0, 00054 LSBFIRST = 1 00055 00056 }BitOrder; 00057 00058 class SPIClass 00059 { 00060 public: 00061 SPIClass(NRF_SPI_Type *_spi); 00062 00063 void begin(); 00064 void begin(uint32_t sck, uint32_t mosi, uint32_t miso); 00065 uint8_t transfer(uint8_t data); 00066 void endTransfer(); 00067 00068 void setSPIMode( uint8_t mode ); 00069 void setFrequency(uint8_t speed ); 00070 void setBitORDER( BitOrder bit); 00071 void setCPHA( bool trailing); 00072 void setCPOL( bool active_low); 00073 00074 00075 private: 00076 NRF_SPI_Type *spi; 00077 00078 uint32_t SCK_Pin; 00079 uint32_t MOSI_Pin; 00080 uint32_t MISO_Pin; 00081 00082 }; 00083 00084 #endif
Generated on Thu Jul 14 2022 03:04:28 by
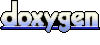