
I messed up the merge, so pushing it over to another repo so I don't lose it. Will tidy up and remove later
Dependencies: BufferedSerial FatFileSystemCpp mbed
frameclock.h
00001 #ifndef __frameclock_h__ 00002 #define __frameclock_h__ 00003 00004 #include "LTCApp.h" 00005 00006 class frameclock 00007 { 00008 public: 00009 frameclock(); 00010 frameclock(int hour, int minute, int second, int frame, int rate, bool drop); 00011 00012 void setMode(int rate, bool drop); 00013 void setTime(int hour, int minute, int second, int frame); 00014 00015 inline void getTime(int *hour, int *minute, int *second, int *frame) 00016 { 00017 *hour = _hour; 00018 *minute = _minute; 00019 *second = _second; 00020 *frame = _frame; 00021 } 00022 00023 uint32_t getTimeMS() { 00024 return (_hour*3600+_minute*60+_second)*1000 + (1000*_frame)/_rate; 00025 } 00026 00027 void nextFrame(); 00028 inline int hours(){return _hour;} 00029 inline int minutes(){return _minute;} 00030 inline int seconds(){return _second;} 00031 inline int frame() {return _frame;} 00032 00033 private: 00034 00035 int _hour; 00036 int _minute; 00037 int _second; 00038 int _frame; 00039 int _rate; 00040 bool _drop; 00041 00042 00043 }; 00044 00045 00046 00047 #endif
Generated on Thu Dec 15 2022 06:07:04 by
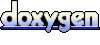