
Host API Example for the ADMW1001
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_config.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw1001_config.h 00039 * @brief: Configuration type definitions for ADMW1001. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW1001_CONFIG_H__ 00044 #define __ADMW1001_CONFIG_H__ 00045 00046 #include "admw_platform.h" 00047 #include "admw1001_sensor_types.h" 00048 00049 /*! @addtogroup ADMW1001_Api 00050 * @{ 00051 */ 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /*! Maximum length allowed for a digital sensor command */ 00058 #define ADMW1001_SENSOR_COMMAND_MAX_LENGTH 7 00059 00060 /*! ADMW1001 channel priority options */ 00061 typedef enum 00062 { 00063 ADMW1001_CHANNEL_PRIORITY_0 = 0, 00064 ADMW1001_CHANNEL_PRIORITY_1, 00065 ADMW1001_CHANNEL_PRIORITY_2, 00066 ADMW1001_CHANNEL_PRIORITY_3, 00067 ADMW1001_CHANNEL_PRIORITY_4, 00068 ADMW1001_CHANNEL_PRIORITY_5, 00069 ADMW1001_CHANNEL_PRIORITY_6, 00070 ADMW1001_CHANNEL_PRIORITY_7, 00071 ADMW1001_CHANNEL_PRIORITY_8, 00072 ADMW1001_CHANNEL_PRIORITY_9, 00073 ADMW1001_CHANNEL_PRIORITY_10, 00074 ADMW1001_CHANNEL_PRIORITY_11, 00075 ADMW1001_CHANNEL_PRIORITY_12, 00076 00077 ADMW1001_CHANNEL_PRIORITY_HIGHEST = ADMW1001_CHANNEL_PRIORITY_0, 00078 ADMW1001_CHANNEL_PRIORITY_LOWEST = ADMW1001_CHANNEL_PRIORITY_12, 00079 00080 } ADMW1001_CHANNEL_PRIORITY ; 00081 00082 /*! ADMW1001 operating mode options */ 00083 typedef enum 00084 { 00085 ADMW1001_OPERATING_MODE_SINGLECYCLE = 0, 00086 /*!< Executes a single measurement cycle and stops */ 00087 ADMW1001_OPERATING_MODE_CONTINUOUS , 00088 /*!< Continuously executes measurement cycles */ 00089 ADMW1001_OPERATING_MODE_MULTICYCLE , 00090 /*!< Executes a burst of measurement cycles, repeated at defined intervals */ 00091 00092 } ADMW1001_OPERATING_MODE ; 00093 00094 /*! ADMW1001 data ready mode options */ 00095 typedef enum 00096 { 00097 ADMW1001_DATAREADY_PER_CONVERSION = 0, 00098 /*!< The DATAREADY signal is asserted after completion of each conversion 00099 * - a single data sample only from the latest completed conversion is 00100 * stored in this mode 00101 */ 00102 ADMW1001_DATAREADY_PER_CYCLE , 00103 /*!< The DATAREADY signal is asserted after completion of each measurement 00104 * cycle 00105 * - data samples only from the lastest completed measurement cycle are 00106 * stored in this mode 00107 */ 00108 ADMW1001_DATAREADY_PER_FIFO_FILL , 00109 /*!< The DATAREADY signal is asserted after each fill of the data FIFO 00110 * - applicable only when @ref ADMW1001_OPERATING_MODE_CONTINUOUS or 00111 * @ref ADMW1001_OPERATING_MODE_MULTICYCLE is also selected 00112 */ 00113 00114 } ADMW1001_DATAREADY_MODE ; 00115 00116 /*! ADMW1001 power mode options */ 00117 typedef enum 00118 { 00119 ADMW1001_POWER_MODE_HIBERNATION = 0, 00120 /*!< module has entede hibernation mode. All analog circuitry is disabled. All peripherals disabled apart from the Wake-up pin functionality. */ 00121 ADMW1001_POWER_MODE_ACTIVE , 00122 /*!< Part is fully powered up and either cycling through a sequence or awaiting a configuration */ 00123 00124 } ADMW1001_POWER_MODE ; 00125 00126 /*! ADMW1001 measurement analog filter settling options */ 00127 typedef enum 00128 { 00129 ADMW1001_FILTER_SETTLING_ALWAYS = 0, 00130 /*!< Allow full settling time to elapse between every measurement from an analog sensor */ 00131 ADMW1001_FILTER_SETTLING_FAST , 00132 /*!< Skip settling time between consecutive measurements from an analog sensor */ 00133 00134 } ADMW1001_FILTER_SETTLING ; 00135 00136 /*! ADMW1001 measurement unit options 00137 * 00138 * Optionally select a measurement unit for final conversion results. 00139 * Currently applicable only to specific temperature sensor types. 00140 */ 00141 typedef enum 00142 { 00143 ADMW1001_MEASUREMENT_UNIT_UNSPECIFIED = 0, 00144 /*!< No measurement unit specified */ 00145 ADMW1001_MEASUREMENT_UNIT_CELSIUS , 00146 /*!< Celsius temperature unit - applicable to temperature sensors only */ 00147 ADMW1001_MEASUREMENT_UNIT_FAHRENHEIT , 00148 /*!< Fahrenheit temperature unit - applicable to temperature sensors only */ 00149 00150 } ADMW1001_MEASUREMENT_UNIT ; 00151 00152 /*! ADMW1001 Open-Sensor Diagnostics frequency 00153 * 00154 * Select the per-cycle frequency at which open-sensor diagnostic 00155 * checks should be performed. Open-sensor diagnostic checks typically require 00156 * specific or time-consuming processing which cannot be executed while a 00157 * measurement cycle is running. 00158 * 00159 * @note Open-sensor diagnostic checks, when performed, will add a delay to the 00160 * start of the next measurement cycle. 00161 */ 00162 typedef enum 00163 { 00164 ADMW1001_OPEN_SENSOR_DIAGNOSTICS_DISABLED = 0, 00165 /*!< No Open-Sensor Detection is performed */ 00166 ADMW1001_OPEN_SENSOR_DIAGNOSTICS_PER_CYCLE , 00167 /*!< No Open-Sensor Detection is performed prior to each cycle */ 00168 ADMW1001_OPEN_SENSOR_DIAGNOSTICS_PER_100_CYCLES , 00169 /*!< No Open-Sensor Detection is performed at intervals of 100 cycles */ 00170 ADMW1001_OPEN_SENSOR_DIAGNOSTICS_PER_1000_CYCLES , 00171 /*!< No Open-Sensor Detection is performed at intervals of 1001 cycles */ 00172 00173 } ADMW1001_OPEN_SENSOR_DIAGNOSTICS ; 00174 00175 /*! ADMW1001 analog input signal amplification gain options 00176 * 00177 * @note applicable only to ADC analog sensor channels 00178 */ 00179 typedef enum 00180 { 00181 ADMW1001_ADC_RTD_CURVE_EUROPEAN = 0, 00182 /*!< EUROPEAN RTD curve used. */ 00183 ADMW1001_ADC_RTD_CURVE_AMERICAN , 00184 /*!< AMERICAN RTD curve used. */ 00185 ADMW1001_ADC_RTD_CURVE_JAPANESE , 00186 /*!< JAPANESE RTD curve used. */ 00187 ADMW1001_ADC_RTD_CURVE_ITS90 , 00188 /*!< ITS90 RTD curve used. */ 00189 00190 } ADMW1001_ADC_RTD_CURVE ; 00191 /*! ADMW1001 analog input signal amplification gain options 00192 * 00193 * @note applicable only to ADC analog sensor channels 00194 */ 00195 typedef enum 00196 { 00197 ADMW1001_ADC_GAIN_1X = 0, 00198 /*!< no amplification gain */ 00199 ADMW1001_ADC_GAIN_2X , 00200 /*!< x2 amplification gain */ 00201 ADMW1001_ADC_GAIN_4X , 00202 /*!< x4 amplification gain */ 00203 ADMW1001_ADC_GAIN_8X , 00204 /*!< x8 amplification gain */ 00205 ADMW1001_ADC_GAIN_16X , 00206 /*!< x16 amplification gain */ 00207 ADMW1001_ADC_GAIN_32X , 00208 /*!< x32 amplification gain */ 00209 ADMW1001_ADC_GAIN_64X , 00210 /*!< x64 amplification gain */ 00211 ADMW1001_ADC_GAIN_128X , 00212 /*!< x128 amplification gain */ 00213 00214 } ADMW1001_ADC_GAIN ; 00215 00216 /*! ADMW1001 analog sensor excitation state options 00217 * 00218 * @note applicable only to ADC analog sensor channels, and 00219 * specific sensor types 00220 */ 00221 typedef enum 00222 { 00223 ADMW1001_ADC_EXC_STATE_OFF =-1, 00224 /*!< Excitation for measurement is off*/ 00225 ADMW1001_ADC_EXC_STATE_ALWAYS_ON , 00226 /*!< Excitation for measurement is always on */ 00227 ADMW1001_ADC_EXC_STATE_CYCLE_POWER , 00228 /*!< Excitation for measurement is active only during measurement */ 00229 00230 } ADMW1001_ADC_EXC_STATE ; 00231 00232 /*! ADMW1001 analog sensor excitation current output level options 00233 * 00234 * @note applicable only to ADC analog sensor channels, and 00235 * specific sensor types 00236 */ 00237 typedef enum 00238 { 00239 ADMW1001_ADC_NO_EXTERNAL_EXC_CURRENT = -1, 00240 /*!< NO External excitation is provided */ 00241 ADMW1001_ADC_EXC_CURRENT_EXTERNAL = 0, 00242 /*!< External excitation is provided */ 00243 ADMW1001_ADC_EXC_CURRENT_50uA , 00244 /*!< 50uA excitation current enabled */ 00245 ADMW1001_ADC_EXC_CURRENT_100uA , 00246 /*!< 100uA excitation current */ 00247 ADMW1001_ADC_EXC_CURRENT_250uA , 00248 /*!< 250uA excitation current enabled */ 00249 ADMW1001_ADC_EXC_CURRENT_500uA , 00250 /*!< 500uA excitation current enabled */ 00251 ADMW1001_ADC_EXC_CURRENT_1000uA , 00252 /*!< 1mA excitation current enabled */ 00253 00254 } ADMW1001_ADC_EXC_CURRENT ; 00255 00256 /*! ADMW1001 analog sensor excitation current ratios used for diode sensor 00257 * 00258 * @note applicable only to a diode sensor 00259 */ 00260 typedef enum 00261 { 00262 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_10UA_100UA = 0, 00263 /**< 2 Current measurement 10uA 100uA */ 00264 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_20UA_160UA, 00265 /**< 2 Current measurement 20uA 160uA */ 00266 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_50UA_300UA, 00267 /**< 2 Current measurement 50uA 300uA */ 00268 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_100UA_600UA, 00269 /**< 2 Current measurement 100uA 600uA */ 00270 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_10UA_50UA_100UA, 00271 /**< 3 current measuremetn 10uA 50uA 100uA */ 00272 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_20UA_100UA_160UA, 00273 /**< 3 current measuremetn 20uA 100uA 160uA */ 00274 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_50UA_150UA_300UA, 00275 /**< 3 current measuremetn 50uA 150uA 300uA */ 00276 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_100UA_300UA_600UA, 00277 /**< 3 current measuremetn 100uA 300uA 600uA */ 00278 00279 } ADMW1001_ADC_EXC_CURRENT_DIODE_RATIO ; 00280 00281 /*! ADMW1001 analog reference selection options 00282 * 00283 * @note applicable only to ADC analog sensor channels, and 00284 * specific sensor types 00285 */ 00286 typedef enum 00287 { 00288 ADMW1001_ADC_REFERENCE_VOLTAGE_INTERNAL = 0, 00289 /*!< Internal VRef - 1.2V */ 00290 ADMW1001_ADC_REFERENCE_VOLTAGE_EXTERNAL_1 , 00291 /*!< External reference voltage #1 */ 00292 ADMW1001_ADC_REFERENCE_VOLTAGE_EXTERNAL_2 , 00293 /*!< External reference voltage #2 */ 00294 ADMW1001_ADC_REFERENCE_VOLTAGE_AVDD , 00295 /*!< Analag Supply Voltage AVDD reference (typically 3.3V) is selected */ 00296 00297 } ADMW1001_ADC_REFERENCE_TYPE ; 00298 00299 /*! ADMW1001 ADC Reference configuration 00300 * 00301 * @note applicable only to ADC analog sensor channels 00302 */ 00303 typedef enum 00304 { 00305 ADMW1001_ADC_GND_SW_OPEN = 0, 00306 /*!< Ground switch not enabled for measurement. */ 00307 ADMW1001_ADC_GND_SW_CLOSED , 00308 /*!< Ground switch enabled for measurement. */ 00309 00310 } ADMW1001_ADC_GND_SW ; 00311 00312 /*! ADMW1001 analog filter chop mode 00313 * 00314 * @note applicable only to ADC analog sensor channels 00315 */ 00316 typedef enum 00317 { 00318 ADMW1001_CHOP_MD_NONE = 0, 00319 /*!< No chop performed. */ 00320 ADMW1001_CHOP_MD_HW , 00321 /*!< Hardware only chop performed. */ 00322 ADMW1001_CHOP_MD_SW , 00323 /*!< Software only chop performed. */ 00324 ADMW1001_CHOP_MD_HWSW , 00325 /*!< Hardware and software chop performed. */ 00326 00327 } ADMW1001_CHOP_MD ; 00328 00329 /*! ADMW1001 analog filter selection options 00330 * 00331 * @note applicable only to ADC analog sensor channels 00332 */ 00333 typedef enum 00334 { 00335 ADMW1001_ADC_FILTER_SINC4 = 0, 00336 /*!< SINC4 - 4th order sinc response filter */ 00337 ADMW1001_ADC_FILTER_SINC3 , 00338 /*!< SINC3 - 3rd order sinc response filter */ 00339 00340 } ADMW1001_ADC_FILTER_TYPE ; 00341 00342 /*! ADMW1001 Sinc Filter range (SF) 00343 * 00344 * @note applicable only to ADC analog sensor channels 00345 * @note SF must be set in conjunction with chop mode 00346 * and sinc filter type to achieve the desired sampling rate. 00347 */ 00348 typedef enum 00349 { 00350 ADMW1001_SF_976HZ = 0, 00351 /*!< SF setting for 976Hz sample rate. */ 00352 ADMW1001_SF_488HZ = 1, 00353 /*!< SF setting for 488Hz sample rate. */ 00354 ADMW1001_SF_244HZ = 3, 00355 /*!< SF setting for 244Hz sample rate. */ 00356 ADMW1001_SF_122HZ = 7, 00357 /*!< SF setting for 122Hz sample rate. */ 00358 ADMW1001_SF_61HZ = 31, 00359 /*!< SF setting for 61Hz sample rate. */ 00360 ADMW1001_SF_30P5HZ = 51, 00361 /*!< SF setting for 61Hz sample rate. */ 00362 ADMW1001_SF_10HZ = 124, 00363 /*!< SF setting for 10Hz sample rate. */ 00364 ADMW1001_SF_8P24HZ = 125, 00365 /*!< SF setting for 8.24Hz sample rate. */ 00366 ADMW1001_SF_5HZ = 127, 00367 /*!< SF setting for 5Hz sample rate. */ 00368 00369 } ADMW1001_SINC_FILTER_RANGE ; 00370 00371 /*! ADMW1001 I2C clock speed options 00372 * 00373 * @note applicable only for I2C sensors 00374 */ 00375 typedef enum 00376 { 00377 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED_100K = 0, 00378 /*!< 100kHz I2C clock speed */ 00379 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED_400K , 00380 /*!< 400kHz I2C clock speed */ 00381 00382 } ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED ; 00383 00384 /*! ADMW1001 SPI mode options 00385 * 00386 * @note applicable only for SPI sensors 00387 */ 00388 typedef enum 00389 { 00390 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE_0 = 0, 00391 /*!< SPI mode 0 Clock Polarity = 0 Clock Phase = 0 */ 00392 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE_1 , 00393 /*!< SPI mode 0 Clock Polarity = 0 Clock Phase = 1 */ 00394 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE_2 , 00395 /*!< SPI mode 0 Clock Polarity = 1 Clock Phase = 0 */ 00396 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE_3 , 00397 /*!< SPI mode 0 Clock Polarity = 1 Clock Phase = 1 */ 00398 00399 } ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE ; 00400 00401 /*! ADMW1001 SPI clock speed options 00402 * 00403 * @note applicable only for SPI sensors 00404 */ 00405 typedef enum 00406 { 00407 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_8MHZ = 0, 00408 /*!< SPI Clock Speed configured to 8MHz */ 00409 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_4MHZ , 00410 /*!< SPI Clock Speed configured to 4MHz */ 00411 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_2MHZ , 00412 /*!< SPI Clock Speed configured to 2MHz */ 00413 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_1MHZ , 00414 /*!< SPI Clock Speed configured to 1MHz */ 00415 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_500KHZ , 00416 /*!< SPI Clock Speed configured to 500kHz */ 00417 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_250KHZ , 00418 /*!< SPI Clock Speed configured to 250kHz */ 00419 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_125KHZ , 00420 /*!< SPI Clock Speed configured to 125kHz */ 00421 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_62P5KHZ , 00422 /*!< SPI Clock Speed configured to 62.5kHz */ 00423 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_31P3KHZ , 00424 /*!< SPI Clock Speed configured to 31.3kHz */ 00425 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_15P6KHZ , 00426 /*!< SPI Clock Speed configured to 15.6kHz */ 00427 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_7P8KHZ , 00428 /*!< SPI Clock Speed configured to 7.8kHz */ 00429 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_3P9KHZ , 00430 /*!< SPI Clock Speed configured to 3.9kHz */ 00431 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_1P9KHZ , 00432 /*!< SPI Clock Speed configured to 1.9kHz */ 00433 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_977HZ , 00434 /*!< SPI Clock Speed configured to 977Hz */ 00435 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_488HZ , 00436 /*!< SPI Clock Speed configured to 488Hz */ 00437 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK_244HZ , 00438 /*!< SPI Clock Speed configured to 244Hz */ 00439 00440 } ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK ; 00441 00442 /*! ADMW1001 Power Configuration options */ 00443 typedef struct 00444 { 00445 ADMW1001_POWER_MODE powerMode; 00446 /*!< Power mode selection */ 00447 00448 } ADMW1001_POWER_CONFIG ; 00449 00450 /*! ADMW1001 Multi-Cycle Configuration options 00451 * 00452 * @note required only when ADMW1001_OPERATING_MODE_MULTICYCLE is selected 00453 * as the operatingMode (@ref ADMW1001_MEASUREMENT_CONFIG) 00454 */ 00455 typedef struct 00456 { 00457 uint32_t cyclesPerBurst; 00458 /*!< Number of cycles to complete for a single burst */ 00459 uint32_t burstInterval; 00460 /*!< Interval, in seconds, between each successive burst of cycles */ 00461 00462 } ADMW1001_MULTICYCLE_CONFIG ; 00463 00464 /*! ADMW1001 Measurement Configuration options */ 00465 typedef struct 00466 { 00467 ADMW1001_OPERATING_MODE operatingMode; 00468 /*!< Operating mode - specifies how measurement cycles are scheduled */ 00469 ADMW1001_DATAREADY_MODE dataReadyMode; 00470 /*!< Data read mode - specifies how output samples are stored for reading */ 00471 ADMW1001_MULTICYCLE_CONFIG multiCycleConfig; 00472 /*!< Multi-Cycle configuration - specifies how bursts of measurement cycles 00473 * are scheduled. Applicable only when operatingMode is 00474 * ADMW1001_OPERATING_MODE_MULTICYCLE 00475 */ 00476 ADMW1001_FILTER_SETTLING filterSettling; 00477 /*!< Analog filter settling - specifies the policy for settling time 00478 * between consecutive measurements from an analog channel in a multi- 00479 * channel configuration. Note that, in single analog channel 00480 * configurations, settling time is always skipped between consecutive 00481 * measurements in a measurement cycle. 00482 */ 00483 uint8_t reserved0[3]; 00484 /*!< Reserved for future use and ensure word alignment. 00485 */ 00486 uint32_t cycleInterval; 00487 /*!< Cycle interval - specifies the time interval between the start of each 00488 * successive measurement cycle. Applicable only when operatingMode is 00489 * not ADMW1001_OPERATING_MODE_SINGLECYCLE 00490 */ 00491 bool vBiasEnable; 00492 /*!< Enable voltage Bias output of ADC 00493 */ 00494 float32_t externalRef1Value; 00495 /*!< Voltage value connected to external reference input #1. 00496 * Applicable only if the selected reference type is 00497 * voltage. 00498 * (see @ref ADMW1001_ADC_REFERENCE_TYPE) 00499 */ 00500 uint32_t reserved1[4]; 00501 /*!< Reserved for future use and ensure word alignment. 00502 */ 00503 00504 } ADMW1001_MEASUREMENT_CONFIG ; 00505 00506 /*! ADMW1001 ADC Excitation Current output configuration 00507 * 00508 * @note applicable only to ADC analog sensor channels, and 00509 * specific sensor types 00510 */ 00511 typedef struct 00512 { 00513 ADMW1001_ADC_EXC_STATE excitationState; 00514 /*!< Excitation current state */ 00515 ADMW1001_ADC_EXC_CURRENT outputLevel; 00516 /*!< Excitation current output level */ 00517 ADMW1001_ADC_EXC_CURRENT_DIODE_RATIO diodeRatio; 00518 /*!< Excitation current output diode ratio */ 00519 00520 } ADMW1001_ADC_EXC_CURRENT_CONFIG ; 00521 00522 /*! ADMW1001 ADC Filter configuration 00523 * 00524 * @note applicable only to ADC analog sensor channels 00525 */ 00526 typedef struct 00527 { 00528 ADMW1001_ADC_FILTER_TYPE type; 00529 /*!< Filter type selection */ 00530 ADMW1001_SINC_FILTER_RANGE sf; 00531 /*!< SF value used along with filter type and chop mode to determine speed */ 00532 ADMW1001_CHOP_MD chopMode; 00533 /*!< Enable filter chop */ 00534 bool notch1p2; 00535 /*!< Enable Notch 2 Filter Mode */ 00536 ADMW1001_ADC_GND_SW groundSwitch; 00537 /*!< Option to open or close sensor ground switch */ 00538 00539 } ADMW1001_ADC_FILTER_CONFIG ; 00540 00541 /*! ADMW1001 ADC analog channel configuration details 00542 * 00543 * @note applicable only to ADC analog sensor channels 00544 */ 00545 typedef struct 00546 { 00547 ADMW1001_ADC_SENSOR_TYPE sensor; 00548 /*!< Sensor type selection */ 00549 ADMW1001_ADC_RTD_CURVE rtdCurve; 00550 /*!< Rtd curve selection */ 00551 ADMW1001_ADC_GAIN gain; 00552 /*!< ADC Gain selection */ 00553 ADMW1001_ADC_EXC_CURRENT_CONFIG current; 00554 /*!< ADC Excitation Current configuration */ 00555 ADMW1001_ADC_FILTER_CONFIG filter; 00556 /*!< ADC Filter configuration */ 00557 ADMW1001_ADC_REFERENCE_TYPE reference; 00558 bool vBiasEnable; 00559 /*!< ADC Reference configuration */ 00560 uint8_t reserved0[3]; 00561 /*!< Reserved for future use and ensure word alignment. */ 00562 uint32_t reserved1[6]; 00563 /*!< Reserved for future use and ensure word alignment. */ 00564 00565 } ADMW1001_ADC_CHANNEL_CONFIG ; 00566 00567 /*! ADMW1001 look-up table selection 00568 * Select table used to linearise the measurement. 00569 */ 00570 typedef enum 00571 { 00572 ADMW1001_LUT_DEFAULT = 0, 00573 /*!< Default LUT */ 00574 ADMW1001_LUT_UNITY = 1, 00575 /*!< Unity LUT */ 00576 ADMW1001_LUT_CUSTOM = 2, 00577 /*!< User defined custom LUT */ 00578 ADMW1001_LUT_RESERVED = 3, 00579 /*!< Reserved for future use */ 00580 00581 } ADMW1001_LUT_SELECT ; 00582 00583 /*! ADMW1001 digital sensor data encoding 00584 * 00585 * @note applicable only to SPI and I2C digital sensor channels 00586 */ 00587 typedef enum 00588 { 00589 ADMW1001_DIGITAL_SENSOR_DATA_CODING_NONE = 0, 00590 /**< None/Invalid - data format is ignored if coding is set to this value */ 00591 ADMW1001_DIGITAL_SENSOR_DATA_CODING_UNIPOLAR, 00592 /**< Unipolar - unsigned integer values */ 00593 ADMW1001_DIGITAL_SENSOR_DATA_CODING_TWOS_COMPLEMENT, 00594 /**< Twos-complement - signed integer values */ 00595 ADMW1001_DIGITAL_SENSOR_DATA_CODING_OFFSET_BINARY, 00596 /**< Offset Binary - used to represent signed values with unsigned integers, 00597 * with the mid-range value representing 0 */ 00598 00599 } ADMW1001_DIGITAL_SENSOR_DATA_CODING ; 00600 00601 /*! ADMW1001 digital sensor data format configuration 00602 * 00603 * @note applicable only to SPI and I2C digital sensor channels 00604 */ 00605 typedef struct 00606 { 00607 ADMW1001_DIGITAL_SENSOR_DATA_CODING coding; 00608 /**< Data Encoding of Sensor Result */ 00609 bool littleEndian; 00610 /**< Set as true if data format is little-endian, false otherwise */ 00611 bool leftJustified; 00612 /**< Set as true if data is left-justified in the data frame, false otherwise */ 00613 uint8_t frameLength; 00614 /**< Data frame length (number of bytes to read from the sensor) */ 00615 uint8_t numDataBits; 00616 /**< Number of relevant data bits to extract from the data frame */ 00617 uint8_t bitOffset; 00618 /**< Data bit offset, relative to data alignment within the data frame */ 00619 uint8_t reserved[2]; 00620 /*!< Reserved for future use and ensure word alignment. */ 00621 00622 } ADMW1001_DIGITAL_SENSOR_DATA_FORMAT ; 00623 00624 /*! ADMW1001 digital sensor command 00625 * 00626 * @note applicable only to SPI and I2C digital sensor channels 00627 */ 00628 typedef struct 00629 { 00630 uint8_t command[ADMW1001_SENSOR_COMMAND_MAX_LENGTH]; 00631 /*!< Optional command bytes to send to the device */ 00632 uint8_t commandLength; 00633 /*!< Number of valid command bytes. Set to 0 if unused */ 00634 00635 } ADMW1001_DIGITAL_SENSOR_COMMAND ; 00636 00637 /*! ADMW1001 digital sensor calibration param 00638 * 00639 * @note applicable only to digital sensor channels 00640 */ 00641 typedef struct 00642 { 00643 uint32_t calibrationParam; 00644 /*!< Independently established environmental variable used during calibration 00645 * of a digital sensor. Used only if the sensor supports calibration 00646 * and expects an environmental parameter 00647 */ 00648 bool enableCalibrationParam; 00649 /*!< Allow Calibration_Parameter to be used during calibration of any digital sensor */ 00650 uint8_t reserved[3]; 00651 /*!< Reserved for future use and ensure word alignment. */ 00652 00653 } ADMW1001_DIGITAL_CALIBRATION_COMMAND ; 00654 00655 /*! ADMW1001 digital sensor communication config 00656 * 00657 * @note applicable only to digital sensor channels 00658 */ 00659 typedef struct 00660 { 00661 bool useCustomCommsConfig; 00662 /*!< Optional parameter to enable user digital communication settings */ 00663 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED i2cClockSpeed; 00664 /*!< Optional parameter to configure specific i2c speed for i2c sensor */ 00665 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_MODE spiMode; 00666 /*!< Optional parameter to configure specific spi mode for spi sensor */ 00667 ADMW1001_DIGITAL_SENSOR_COMMS_SPI_CLOCK spiClock; 00668 /*!< Optional parameter to configure specific spi clock for spi sensor */ 00669 00670 } ADMW1001_DIGITAL_SENSOR_COMMS ; 00671 00672 /*! ADMW1001 I2C digital channel configuration details 00673 * 00674 * @note applicable only to I2C digital sensor channels 00675 */ 00676 typedef struct 00677 { 00678 ADMW1001_I2C_SENSOR_TYPE sensor; 00679 /*!< Sensor type selection */ 00680 uint8_t deviceAddress; 00681 /*!< I2C device address (7-bit) */ 00682 uint8_t reserved; 00683 /*!< Reserved for future use and ensure word alignment. */ 00684 ADMW1001_DIGITAL_SENSOR_COMMAND configurationCommand; 00685 /*!< Optional configuration command to send to the device at start-up. 00686 * A default configuration command will be used if this is not specified. 00687 * Applicable only to specific I2C sensor types. 00688 */ 00689 ADMW1001_DIGITAL_SENSOR_COMMAND dataRequestCommand; 00690 /*!< Optional data request command to send to the device for each sample. 00691 * A default data request command will be used if this is not specified. 00692 * Applicable only to specific I2C sensor types. 00693 */ 00694 ADMW1001_DIGITAL_SENSOR_DATA_FORMAT dataFormat; 00695 /*!< Optional data format configuration to parse/extract data from the device. 00696 * A default data format will be used if this is not specified. 00697 * Applicable only to specific I2C sensor types 00698 */ 00699 ADMW1001_DIGITAL_CALIBRATION_COMMAND digitalCalibrationParam; 00700 /*!< This is used to supply an independently established environmental variable 00701 * that must be used during calibration of a [digital] sensor which a) 00702 * supports calibration and b) expects an environmental parameter. 00703 * An example is a CO2 sensor, which may require the CO2 concentration level 00704 * when performing a calibration 00705 */ 00706 ADMW1001_DIGITAL_SENSOR_COMMS configureComms; 00707 /*!< Optional configuration to setup a user communication config. 00708 * A default configuration will be used if this is not specified. 00709 * Applicable only to specific I2C sensor types. 00710 */ 00711 00712 } ADMW1001_I2C_CHANNEL_CONFIG ; 00713 00714 /*! ADMW1001 SPI digital channel configuration details 00715 * 00716 * @note applicable only to SPI digital sensor channels 00717 */ 00718 typedef struct 00719 { 00720 ADMW1001_SPI_SENSOR_TYPE sensor; 00721 /*!< Sensor type selection */ 00722 uint8_t reserved[2]; 00723 /*!< Reserved for future use and ensure word alignment. */ 00724 ADMW1001_DIGITAL_SENSOR_COMMAND configurationCommand; 00725 /*!< Optional configuration command to send to the device at start-up. 00726 * A default configuration command will be used if this is not specified. 00727 * Applicable only to specific SPI sensor types. 00728 */ 00729 ADMW1001_DIGITAL_SENSOR_COMMAND dataRequestCommand; 00730 /*!< Optional data request command to send to the device for each sample. 00731 * A default data request command will be used if this is not specified. 00732 * Applicable only to specific SPI sensor types. 00733 */ 00734 ADMW1001_DIGITAL_SENSOR_DATA_FORMAT dataFormat; 00735 /*!< Optional data format configuration to parse/extract data from the device. 00736 * A default data format will be used if this is not specified. 00737 * Applicable only to specific SPI sensor types 00738 */ 00739 ADMW1001_DIGITAL_CALIBRATION_COMMAND digitalCalibrationParam; 00740 /*!< This is used to supply an independently established environmental variable 00741 * that must be used during calibration of a [digital] sensor which a) 00742 * supports calibration and b) expects an environmental parameter. 00743 * An example is a CO2 sensor, which may require the CO2 concentration level 00744 * when performing a calibration 00745 */ 00746 ADMW1001_DIGITAL_SENSOR_COMMS configureComms; 00747 /*!< Optional configuration to setup a user communication config. 00748 * A default configuration will be used if this is not specified. 00749 * Applicable only to specific SPI sensor types. 00750 */ 00751 00752 } ADMW1001_SPI_CHANNEL_CONFIG ; 00753 00754 /*! ADMW1001 Measurement Channel configuration details */ 00755 typedef struct 00756 { 00757 bool enableChannel; 00758 /*!< Option to include this channel in normal measurement cycles */ 00759 bool disablePublishing; 00760 /*!< Option to disable publishing of data samples from this channel. The 00761 * channel may still be included in measurement cycles, but data samples 00762 * obtained from this channel will not be published. This is typically 00763 * used for channels which are required only as a compensation reference 00764 * for another channel (e.g. Cold-Junction Compensation channels). 00765 */ 00766 ADMW1001_CH_ID compensationChannel; 00767 /*!< Optional compensation channel. Set to ADMW1001_CH_ID_NONE if not 00768 * required. Typically used for thermocouple sensors that require a 00769 * separate measurement of the "cold-junction" temperature, which can be 00770 * be provided by an RTD temperature sensor connected on a separate 00771 * "compensation channel" */ 00772 ADMW1001_LUT_SELECT lutSelect; 00773 /*!<Select Look Up Table LUT for calculations, this implies that the 00774 * fundamental measurement for the sensor (typically mV or Ohms) 00775 * 0 = default, 1= unity, 2 = custom 00776 */ 00777 ADMW1001_MEASUREMENT_UNIT measurementUnit; 00778 /*!< Optional measurement unit selection for conversion results. Applicable 00779 * only for certain sensor types. Set to 00780 * ADMW1001_MEASUREMENT_UNIT_DEFAULT if not applicable. 00781 */ 00782 float32_t lowThreshold; 00783 /*!< Optional minimum threshold value for each processed sample, to be 00784 * checked prior to publishing. A channel ALERT condition is raised 00785 * if the processed value is lower than this threshold. Set to NaN 00786 * if not required. 00787 */ 00788 float32_t highThreshold; 00789 /*!< Optional maximum threshold value for each processed sample, to be 00790 * checked prior to publishing. A channel ALERT condition is raised 00791 * if the processed value is higher than this threshold. Set to NaN 00792 * if not required. 00793 */ 00794 float32_t offsetAdjustment; 00795 /*!< Optional offset adjustment value applied to each processed sample. 00796 * Set to NaN or 0.0 if not required. 00797 */ 00798 float32_t gainAdjustment; 00799 /*!< Optional gain adjustment value applied to each processed sample. 00800 * Set to NaN or 1.0 if not required. 00801 */ 00802 float32_t sensorParameter; 00803 /*!< Optional sensor parameter adjustment. 00804 * Set to NaN or 0 if not required. 00805 */ 00806 uint32_t measurementsPerCycle; 00807 /*!< The number of measurements to obtain from this channel within each 00808 * cycle. Each enabled channel is measured in turn, until the number of 00809 * measurements requested for the channel has been reached. A different 00810 * number of measurements-per-cycle may be specified for each channel. 00811 */ 00812 uint32_t cycleSkipCount; 00813 /*!< Optional number of cycles to skip, such that this channel is included 00814 * in the sequence in only one of every (cycleSkipCount + 1) cycles that 00815 * occur. If set to 0 (default), this channel is included in every cycle; 00816 * if set to 1, this channel is included in every 2nd cycle; if set to 2, 00817 * this channel is included in every 3rd cycle, and so on. 00818 */ 00819 uint32_t extraSettlingTime; 00820 /*!< A minimum settling time is applied internally for each channel, based 00821 * on the sensor type. However, additional settling time (microseconds) 00822 * can optionally be specified. Set to 0 if not required. 00823 */ 00824 ADMW1001_CHANNEL_PRIORITY priority; 00825 /*!< By default, channels are arranged in the measurement sequence based on 00826 * ascending order of channel ID. However, a priority-level may be 00827 * specified per channel to force a different ordering of the channels, 00828 * with higher-priority channels appearing before lower-priority channels. 00829 * Channels with equal priority are ordered by ascending order of channel 00830 * ID. Lower numbers indicate higher priority, with 0 being the highest. 00831 * Set to 0 if not required. 00832 */ 00833 union 00834 { 00835 ADMW1001_ADC_CHANNEL_CONFIG adcChannelConfig; 00836 /*!< ADC channel configuration - applicable only to ADC channels */ 00837 ADMW1001_I2C_CHANNEL_CONFIG i2cChannelConfig; 00838 /*!< I2C channel configuration - applicable only to I2C channels */ 00839 ADMW1001_SPI_CHANNEL_CONFIG spiChannelConfig; 00840 /*!< SPI channel configuration - applicable only to SPI channels */ 00841 }; 00842 /*!< Only one of adcChannelConfig, i2cChannelConfig, spiChannelConfig 00843 * is required, depending on the channel designation 00844 * (analog, I2C, SPI) 00845 */ 00846 00847 } ADMW1001_CHANNEL_CONFIG ; 00848 00849 /*! ADMW1001 Diagnostics configuration details */ 00850 typedef struct 00851 { 00852 bool disableGlobalDiag; 00853 /*!< Option to disable the following diagnostic checks on the ADC: 00854 * - Reference Detection errors 00855 * - Input under-/over-voltage errors 00856 * - Calibration, Conversion and Saturation errors 00857 */ 00858 bool disableMeasurementDiag; 00859 /*!< Option to disable additional checks per measurement channel: 00860 * - High/low threshold limit violation 00861 */ 00862 00863 bool disableCriticalTempAbort; 00864 /*!< Option to disable abort of measurement cycle if the operating 00865 * temperature of the ADMW1001 has exceeded critical limits 00866 */ 00867 00868 ADMW1001_OPEN_SENSOR_DIAGNOSTICS osdFrequency; 00869 /*!< Option to enable Open-Circuit Detection at a selected cycle interval */ 00870 00871 } ADMW1001_DIAGNOSTICS_CONFIG ; 00872 00873 typedef uint16_t ADMW1001_ADVANCED_ACCESS_KEY; 00874 00875 /*! ADMW1001 Device configuration details */ 00876 typedef struct 00877 { 00878 ADMW1001_POWER_CONFIG power; 00879 /*!< Power configuration details */ 00880 ADMW1001_MEASUREMENT_CONFIG measurement; 00881 /*!< Measurement configuration details */ 00882 ADMW1001_DIAGNOSTICS_CONFIG diagnostics; 00883 /*!< FFT configuration details */ 00884 ADMW1001_CHANNEL_CONFIG channels[ADMW1001_MAX_CHANNELS ]; 00885 /*!< Channel-specific configuration details */ 00886 ADMW1001_ADVANCED_ACCESS_KEY advancedAccessKey; 00887 /*!< Key to enable access to advanced sensor configuration options */ 00888 00889 } ADMW1001_CONFIG ; 00890 00891 #ifdef __cplusplus 00892 } 00893 #endif 00894 00895 /*! 00896 * @} 00897 */ 00898 00899 #endif /* __ADMW1001_CONFIG_H__ */
Generated on Wed Jul 13 2022 03:04:54 by
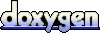