
Host API Example for the ADMW1001
Embed:
(wiki syntax)
Show/hide line numbers
ADMW1001_REGISTERS.h
00001 /* ================================================================================ 00002 00003 Project : ADMW1001_REGISTERS 00004 File : ADMW1001_REGISTERS.h 00005 Description : Register Definitions 00006 00007 Date : Jun 19, 2019 00008 00009 Copyright (c) 2019 Analog Devices, Inc. All Rights Reserved. 00010 This software is proprietary and confidential to Analog Devices, Inc. and 00011 its licensors. 00012 00013 This file was auto-generated. Do not make local changes to this file. 00014 00015 ================================================================================ */ 00016 00017 #ifndef _DEF_ADMW1001_REGISTERS_H 00018 #define _DEF_ADMW1001_REGISTERS_H 00019 00020 #if defined(_LANGUAGE_C) || (defined(__GNUC__) && !defined(__ASSEMBLER__)) 00021 #include <stdint.h> 00022 #endif /* _LANGUAGE_C */ 00023 00024 #ifndef __ADI_GENERATED_DEF_HEADERS__ 00025 #define __ADI_GENERATED_DEF_HEADERS__ 1 00026 #endif 00027 00028 #define __ADI_HAS_CORE__ 1 00029 #define __ADI_HAS_SPI__ 1 00030 #define __ADI_HAS_ADMW_TEST__ 1 00031 00032 /* ============================================================================================================================ 00033 00034 ============================================================================================================================ */ 00035 00036 /* ============================================================================================================================ 00037 SPI 00038 ============================================================================================================================ */ 00039 #define REG_SPI_INTERFACE_CONFIG_A_RESET 0x00000030 /* Reset Value for Interface_Config_A */ 00040 #define REG_SPI_INTERFACE_CONFIG_A 0x00000000 /* SPI Interface Configuration A */ 00041 #define REG_SPI_INTERFACE_CONFIG_B_RESET 0x00000000 /* Reset Value for Interface_Config_B */ 00042 #define REG_SPI_INTERFACE_CONFIG_B 0x00000001 /* SPI Interface Configuration B */ 00043 #define REG_SPI_DEVICE_CONFIG_RESET 0x00000000 /* Reset Value for Device_Config */ 00044 #define REG_SPI_DEVICE_CONFIG 0x00000002 /* SPI Device Configuration */ 00045 #define REG_SPI_CHIP_TYPE_RESET 0x00000007 /* Reset Value for Chip_Type */ 00046 #define REG_SPI_CHIP_TYPE 0x00000003 /* SPI Chip Type */ 00047 #define REG_SPI_PRODUCT_ID_L_RESET 0x00000020 /* Reset Value for Product_ID_L */ 00048 #define REG_SPI_PRODUCT_ID_L 0x00000004 /* SPI Product ID Low */ 00049 #define REG_SPI_PRODUCT_ID_H_RESET 0x00000000 /* Reset Value for Product_ID_H */ 00050 #define REG_SPI_PRODUCT_ID_H 0x00000005 /* SPI Product ID High */ 00051 #define REG_SPI_CHIP_GRADE_RESET 0x00000000 /* Reset Value for Chip_Grade */ 00052 #define REG_SPI_CHIP_GRADE 0x00000006 /* SPI Chip Grade */ 00053 #define REG_SPI_SCRATCH_PAD_RESET 0x00000000 /* Reset Value for Scratch_Pad */ 00054 #define REG_SPI_SCRATCH_PAD 0x0000000A /* SPI Scratch Pad */ 00055 #define REG_SPI_SPI_REVISION_RESET 0x00000082 /* Reset Value for SPI_Revision */ 00056 #define REG_SPI_SPI_REVISION 0x0000000B /* SPI SPI Revision */ 00057 #define REG_SPI_VENDOR_L_RESET 0x00000056 /* Reset Value for Vendor_L */ 00058 #define REG_SPI_VENDOR_L 0x0000000C /* SPI Vendor ID Low */ 00059 #define REG_SPI_VENDOR_H_RESET 0x00000004 /* Reset Value for Vendor_H */ 00060 #define REG_SPI_VENDOR_H 0x0000000D /* SPI Vendor ID High */ 00061 #define REG_SPI_STREAM_MODE_RESET 0x00000000 /* Reset Value for Stream_Mode */ 00062 #define REG_SPI_STREAM_MODE 0x0000000E /* SPI Stream Mode */ 00063 #define REG_SPI_TRANSFER_CONFIG_RESET 0x00000000 /* Reset Value for Transfer_Config */ 00064 #define REG_SPI_TRANSFER_CONFIG 0x0000000F /* SPI Transfer Config */ 00065 #define REG_SPI_INTERFACE_CONFIG_C_RESET 0x00000033 /* Reset Value for Interface_Config_C */ 00066 #define REG_SPI_INTERFACE_CONFIG_C 0x00000010 /* SPI Interface Configuration C */ 00067 #define REG_SPI_INTERFACE_STATUS_A_RESET 0x00000000 /* Reset Value for Interface_Status_A */ 00068 #define REG_SPI_INTERFACE_STATUS_A 0x00000011 /* SPI Interface Status A */ 00069 00070 /* ============================================================================================================================ 00071 SPI Register BitMasks, Positions & Enumerations 00072 ============================================================================================================================ */ 00073 /* ------------------------------------------------------------------------------------------------------------------------- 00074 SPI_INTERFACE_CONFIG_A Pos/Masks Description 00075 ------------------------------------------------------------------------------------------------------------------------- */ 00076 #define BITP_SPI_INTERFACE_CONFIG_A_SW_RESET 7 /* First of Two of SW_RESET Bits. */ 00077 #define BITP_SPI_INTERFACE_CONFIG_A_ADDR_ASCENSION 5 /* Determines Sequential Addressing Behavior */ 00078 #define BITP_SPI_INTERFACE_CONFIG_A_SDO_ENABLE 4 /* SDO Pin Enable */ 00079 #define BITP_SPI_INTERFACE_CONFIG_A_SW_RESETX 0 /* Second of Two of SW_RESET Bits. */ 00080 #define BITM_SPI_INTERFACE_CONFIG_A_SW_RESET 0x00000080 /* First of Two of SW_RESET Bits. */ 00081 #define BITM_SPI_INTERFACE_CONFIG_A_ADDR_ASCENSION 0x00000020 /* Determines Sequential Addressing Behavior */ 00082 #define BITM_SPI_INTERFACE_CONFIG_A_SDO_ENABLE 0x00000010 /* SDO Pin Enable */ 00083 #define BITM_SPI_INTERFACE_CONFIG_A_SW_RESETX 0x00000001 /* Second of Two of SW_RESET Bits. */ 00084 #define ENUM_SPI_INTERFACE_CONFIG_A_DESCEND 0x00000000 /* Addr_Ascension: Address accessed is decremented by one for each data byte when streaming */ 00085 #define ENUM_SPI_INTERFACE_CONFIG_A_ASCEND 0x00000020 /* Addr_Ascension: Address accessed is incremented by one for each data byte when streaming */ 00086 00087 /* ------------------------------------------------------------------------------------------------------------------------- 00088 SPI_INTERFACE_CONFIG_B Pos/Masks Description 00089 ------------------------------------------------------------------------------------------------------------------------- */ 00090 #define BITP_SPI_INTERFACE_CONFIG_B_SINGLE_INST 7 /* Select Streaming or Single Instruction Mode */ 00091 #define BITM_SPI_INTERFACE_CONFIG_B_SINGLE_INST 0x00000080 /* Select Streaming or Single Instruction Mode */ 00092 #define ENUM_SPI_INTERFACE_CONFIG_B_STREAMING_MODE 0x00000000 /* Single_Inst: Streaming mode is enabled */ 00093 #define ENUM_SPI_INTERFACE_CONFIG_B_SINGLE_INSTRUCTION_MODE 0x00000080 /* Single_Inst: Single Instruction mode is enabled */ 00094 00095 /* ------------------------------------------------------------------------------------------------------------------------- 00096 SPI_DEVICE_CONFIG Pos/Masks Description 00097 ------------------------------------------------------------------------------------------------------------------------- */ 00098 #define BITP_SPI_DEVICE_CONFIG_OPERATING_MODES 0 /* Power Modes */ 00099 #define BITM_SPI_DEVICE_CONFIG_OPERATING_MODES 0x00000003 /* Power Modes */ 00100 #define ENUM_SPI_DEVICE_CONFIG_NORMAL 0x00000000 /* Operating_Modes: Normal Operating Mode */ 00101 #define ENUM_SPI_DEVICE_CONFIG_SLEEP 0x00000003 /* Operating_Modes: Low Power Mode */ 00102 00103 /* ------------------------------------------------------------------------------------------------------------------------- 00104 SPI_CHIP_TYPE Pos/Masks Description 00105 ------------------------------------------------------------------------------------------------------------------------- */ 00106 #define BITP_SPI_CHIP_TYPE_CHIP_TYPE 0 /* Precision ADC */ 00107 #define BITM_SPI_CHIP_TYPE_CHIP_TYPE 0x0000000F /* Precision ADC */ 00108 00109 /* ------------------------------------------------------------------------------------------------------------------------- 00110 SPI_PRODUCT_ID_L Pos/Masks Description 00111 ------------------------------------------------------------------------------------------------------------------------- */ 00112 #define BITP_SPI_PRODUCT_ID_L_PRODUCT_ID 0 /* This is Device Chip Type/Family */ 00113 #define BITM_SPI_PRODUCT_ID_L_PRODUCT_ID 0x000000FF /* This is Device Chip Type/Family */ 00114 00115 /* ------------------------------------------------------------------------------------------------------------------------- 00116 SPI_PRODUCT_ID_H Pos/Masks Description 00117 ------------------------------------------------------------------------------------------------------------------------- */ 00118 #define BITP_SPI_PRODUCT_ID_H_PRODUCT_ID 0 /* This is Device Chip Type/Family */ 00119 #define BITM_SPI_PRODUCT_ID_H_PRODUCT_ID 0x000000FF /* This is Device Chip Type/Family */ 00120 00121 /* ------------------------------------------------------------------------------------------------------------------------- 00122 SPI_CHIP_GRADE Pos/Masks Description 00123 ------------------------------------------------------------------------------------------------------------------------- */ 00124 #define BITP_SPI_CHIP_GRADE_GRADE 4 /* This is the Device Performance Grade */ 00125 #define BITP_SPI_CHIP_GRADE_DEVICE_REVISION 0 /* This is the Device Hardware Revision */ 00126 #define BITM_SPI_CHIP_GRADE_GRADE 0x000000F0 /* This is the Device Performance Grade */ 00127 #define BITM_SPI_CHIP_GRADE_DEVICE_REVISION 0x0000000F /* This is the Device Hardware Revision */ 00128 00129 /* ------------------------------------------------------------------------------------------------------------------------- 00130 SPI_SCRATCH_PAD Pos/Masks Description 00131 ------------------------------------------------------------------------------------------------------------------------- */ 00132 #define BITP_SPI_SCRATCH_PAD_SCRATCH_VALUE 0 /* Software Scratchpad */ 00133 #define BITM_SPI_SCRATCH_PAD_SCRATCH_VALUE 0x000000FF /* Software Scratchpad */ 00134 00135 /* ------------------------------------------------------------------------------------------------------------------------- 00136 SPI_SPI_REVISION Pos/Masks Description 00137 ------------------------------------------------------------------------------------------------------------------------- */ 00138 #define BITP_SPI_SPI_REVISION_SPI_TYPE 6 /* Always Reads as 0x2 */ 00139 #define BITP_SPI_SPI_REVISION_VERSION 0 /* SPI Version */ 00140 #define BITM_SPI_SPI_REVISION_SPI_TYPE 0x000000C0 /* Always Reads as 0x2 */ 00141 #define BITM_SPI_SPI_REVISION_VERSION 0x0000003F /* SPI Version */ 00142 #define ENUM_SPI_SPI_REVISION_ADI_SPI 0x00000000 00143 #define ENUM_SPI_SPI_REVISION_LPT_SPI 0x00000080 00144 #define ENUM_SPI_SPI_REVISION_REV1_0 0x00000002 /* Version: Revision 1.0 */ 00145 00146 /* ------------------------------------------------------------------------------------------------------------------------- 00147 SPI_VENDOR_L Pos/Masks Description 00148 ------------------------------------------------------------------------------------------------------------------------- */ 00149 #define BITP_SPI_VENDOR_L_VID 0 /* Analog Devices Vendor ID */ 00150 #define BITM_SPI_VENDOR_L_VID 0x000000FF /* Analog Devices Vendor ID */ 00151 00152 /* ------------------------------------------------------------------------------------------------------------------------- 00153 SPI_VENDOR_H Pos/Masks Description 00154 ------------------------------------------------------------------------------------------------------------------------- */ 00155 #define BITP_SPI_VENDOR_H_VID 0 /* Analog Devices Vendor ID */ 00156 #define BITM_SPI_VENDOR_H_VID 0x000000FF /* Analog Devices Vendor ID */ 00157 00158 /* ------------------------------------------------------------------------------------------------------------------------- 00159 SPI_STREAM_MODE Pos/Masks Description 00160 ------------------------------------------------------------------------------------------------------------------------- */ 00161 #define BITP_SPI_STREAM_MODE_LOOP_COUNT 0 /* Sets the Data Byte Count Before Looping to Start Address */ 00162 #define BITM_SPI_STREAM_MODE_LOOP_COUNT 0x000000FF /* Sets the Data Byte Count Before Looping to Start Address */ 00163 00164 /* ------------------------------------------------------------------------------------------------------------------------- 00165 SPI_TRANSFER_CONFIG Pos/Masks Description 00166 ------------------------------------------------------------------------------------------------------------------------- */ 00167 #define BITP_SPI_TRANSFER_CONFIG_STREAM_MODE 1 /* When Streaming, Controls Master-Slave Transfer */ 00168 #define BITM_SPI_TRANSFER_CONFIG_STREAM_MODE 0x00000002 /* When Streaming, Controls Master-Slave Transfer */ 00169 #define ENUM_SPI_TRANSFER_CONFIG_UPDATE_ON_WRITE 0x00000000 /* Stream_Mode: Transfers after each byte/mulit-byte register */ 00170 #define ENUM_SPI_TRANSFER_CONFIG_UPDATE_ON_ADDRESS_LOOP 0x00000002 /* Stream_Mode: Transfers when address loops */ 00171 00172 /* ------------------------------------------------------------------------------------------------------------------------- 00173 SPI_INTERFACE_CONFIG_C Pos/Masks Description 00174 ------------------------------------------------------------------------------------------------------------------------- */ 00175 #define BITP_SPI_INTERFACE_CONFIG_C_CRC_ENABLE 6 /* CRC Enable */ 00176 #define BITP_SPI_INTERFACE_CONFIG_C_STRICT_REGISTER_ACCESS 5 /* Multi-byte Registers Must Be Read/Written in Full */ 00177 #define BITP_SPI_INTERFACE_CONFIG_C_SEND_STATUS 4 /* Enables Sending of Status in 4-wire Mode */ 00178 #define BITP_SPI_INTERFACE_CONFIG_C_CRC_ENABLEB 0 /* Inverted CRC Enable */ 00179 #define BITM_SPI_INTERFACE_CONFIG_C_CRC_ENABLE 0x000000C0 /* CRC Enable */ 00180 #define BITM_SPI_INTERFACE_CONFIG_C_STRICT_REGISTER_ACCESS 0x00000020 /* Multi-byte Registers Must Be Read/Written in Full */ 00181 #define BITM_SPI_INTERFACE_CONFIG_C_SEND_STATUS 0x00000010 /* Enables Sending of Status in 4-wire Mode */ 00182 #define BITM_SPI_INTERFACE_CONFIG_C_CRC_ENABLEB 0x00000003 /* Inverted CRC Enable */ 00183 #define ENUM_SPI_INTERFACE_CONFIG_C_DISABLED 0x00000000 /* CRC_Enable: CRC Disabled */ 00184 #define ENUM_SPI_INTERFACE_CONFIG_C_ENABLED 0x00000040 /* CRC_Enable: CRC Enabled */ 00185 #define ENUM_SPI_INTERFACE_CONFIG_C_NORMAL_ACCESS 0x00000000 /* Strict_Register_Access: Normal mode, no access restrictions */ 00186 #define ENUM_SPI_INTERFACE_CONFIG_C_STRICT_ACCESS 0x00000020 /* Strict_Register_Access: Strict mode, multi-byte registers require all bytes read/written */ 00187 00188 /* ------------------------------------------------------------------------------------------------------------------------- 00189 SPI_INTERFACE_STATUS_A Pos/Masks Description 00190 ------------------------------------------------------------------------------------------------------------------------- */ 00191 #define BITP_SPI_INTERFACE_STATUS_A_NOT_READY_ERROR 7 /* Device Not Ready for Transaction */ 00192 #define BITP_SPI_INTERFACE_STATUS_A_CLOCK_COUNT_ERROR 4 /* Incorrect Number of Clocks Detected in a Transaction */ 00193 #define BITP_SPI_INTERFACE_STATUS_A_CRC_ERROR 3 /* Invalid/No CRC Received */ 00194 #define BITP_SPI_INTERFACE_STATUS_A_WR_TO_RD_ONLY_REG_ERROR 2 /* Write to Read-Only Register Attempted */ 00195 #define BITP_SPI_INTERFACE_STATUS_A_REGISTER_PARTIAL_ACCESS_ERROR 1 /* Set When Fewer Than Expected Number of Bytes Read/Written */ 00196 #define BITP_SPI_INTERFACE_STATUS_A_ADDRESS_INVALID_ERROR 0 /* Attempt to Read/Write Non-existent Register Address */ 00197 #define BITM_SPI_INTERFACE_STATUS_A_NOT_READY_ERROR 0x00000080 /* Device Not Ready for Transaction */ 00198 #define BITM_SPI_INTERFACE_STATUS_A_CLOCK_COUNT_ERROR 0x00000010 /* Incorrect Number of Clocks Detected in a Transaction */ 00199 #define BITM_SPI_INTERFACE_STATUS_A_CRC_ERROR 0x00000008 /* Invalid/No CRC Received */ 00200 #define BITM_SPI_INTERFACE_STATUS_A_WR_TO_RD_ONLY_REG_ERROR 0x00000004 /* Write to Read-Only Register Attempted */ 00201 #define BITM_SPI_INTERFACE_STATUS_A_REGISTER_PARTIAL_ACCESS_ERROR 0x00000002 /* Set When Fewer Than Expected Number of Bytes Read/Written */ 00202 #define BITM_SPI_INTERFACE_STATUS_A_ADDRESS_INVALID_ERROR 0x00000001 /* Attempt to Read/Write Non-existent Register Address */ 00203 00204 00205 /* ============================================================================================================================ 00206 ADMW1001 Core Registers 00207 ============================================================================================================================ */ 00208 00209 /* ============================================================================================================================ 00210 CORE 00211 ============================================================================================================================ */ 00212 #define REG_CORE_COMMAND_RESET 0x00000000 /* Reset Value for Command */ 00213 #define REG_CORE_COMMAND 0x00000014 /* CORE Special Command */ 00214 #define REG_CORE_MODE_RESET 0x00000000 /* Reset Value for Mode */ 00215 #define REG_CORE_MODE 0x00000016 /* CORE Operating Mode and DRDY Control */ 00216 #define REG_CORE_POWER_CONFIG_RESET 0x00000000 /* Reset Value for Power_Config */ 00217 #define REG_CORE_POWER_CONFIG 0x00000017 /* CORE General Configuration */ 00218 #define REG_CORE_CYCLE_CONTROL_RESET 0x00000000 /* Reset Value for Cycle_Control */ 00219 #define REG_CORE_CYCLE_CONTROL 0x00000018 /* CORE Measurement Cycle */ 00220 #define REG_CORE_STATUS_RESET 0x00000000 /* Reset Value for Status */ 00221 #define REG_CORE_STATUS 0x00000020 /* CORE General Status */ 00222 #define REG_CORE_DIAGNOSTICS_STATUS_RESET 0x00000000 /* Reset Value for Diagnostics_Status */ 00223 #define REG_CORE_DIAGNOSTICS_STATUS 0x00000024 /* CORE Diagnostics Status */ 00224 #define REG_CORE_CHANNEL_ALERT_STATUS_RESET 0x00000000 /* Reset Value for Channel_Alert_Status */ 00225 #define REG_CORE_CHANNEL_ALERT_STATUS 0x00000026 /* CORE Alert Status Summary */ 00226 #define REG_CORE_ALERT_STATUS_2_RESET 0x00000000 /* Reset Value for Alert_Status_2 */ 00227 #define REG_CORE_ALERT_STATUS_2 0x00000028 /* CORE Additional Alert Status Information */ 00228 #define REG_CORE_ALERT_DETAIL_CHn_RESET 0x00000000 /* Reset Value for Alert_Detail_Ch[n] */ 00229 #define REG_CORE_ALERT_DETAIL_CH0_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH0 */ 00230 #define REG_CORE_ALERT_DETAIL_CH1_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH1 */ 00231 #define REG_CORE_ALERT_DETAIL_CH2_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH2 */ 00232 #define REG_CORE_ALERT_DETAIL_CH3_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH3 */ 00233 #define REG_CORE_ALERT_DETAIL_CH4_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH4 */ 00234 #define REG_CORE_ALERT_DETAIL_CH5_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH5 */ 00235 #define REG_CORE_ALERT_DETAIL_CH6_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH6 */ 00236 #define REG_CORE_ALERT_DETAIL_CH7_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH7 */ 00237 #define REG_CORE_ALERT_DETAIL_CH8_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH8 */ 00238 #define REG_CORE_ALERT_DETAIL_CH9_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH9 */ 00239 #define REG_CORE_ALERT_DETAIL_CH10_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH10 */ 00240 #define REG_CORE_ALERT_DETAIL_CH11_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH11 */ 00241 #define REG_CORE_ALERT_DETAIL_CH12_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH12 */ 00242 #define REG_CORE_ALERT_DETAIL_CH0 0x0000002A /* CORE Detailed Error Information */ 00243 #define REG_CORE_ALERT_DETAIL_CH1 0x0000002C /* CORE Detailed Error Information */ 00244 #define REG_CORE_ALERT_DETAIL_CH2 0x0000002E /* CORE Detailed Error Information */ 00245 #define REG_CORE_ALERT_DETAIL_CH3 0x00000030 /* CORE Detailed Error Information */ 00246 #define REG_CORE_ALERT_DETAIL_CH4 0x00000032 /* CORE Detailed Error Information */ 00247 #define REG_CORE_ALERT_DETAIL_CH5 0x00000034 /* CORE Detailed Error Information */ 00248 #define REG_CORE_ALERT_DETAIL_CH6 0x00000036 /* CORE Detailed Error Information */ 00249 #define REG_CORE_ALERT_DETAIL_CH7 0x00000038 /* CORE Detailed Error Information */ 00250 #define REG_CORE_ALERT_DETAIL_CH8 0x0000003A /* CORE Detailed Error Information */ 00251 #define REG_CORE_ALERT_DETAIL_CH9 0x0000003C /* CORE Detailed Error Information */ 00252 #define REG_CORE_ALERT_DETAIL_CH10 0x0000003E /* CORE Detailed Error Information */ 00253 #define REG_CORE_ALERT_DETAIL_CH11 0x00000040 /* CORE Detailed Error Information */ 00254 #define REG_CORE_ALERT_DETAIL_CH12 0x00000042 /* CORE Detailed Error Information */ 00255 #define REG_CORE_ALERT_DETAIL_CHn(i) (REG_CORE_ALERT_DETAIL_CH0 + ((i) * 2)) 00256 #define REG_CORE_ALERT_DETAIL_CHn_COUNT 13 00257 #define REG_CORE_ERROR_CODE_RESET 0x00000000 /* Reset Value for Error_Code */ 00258 #define REG_CORE_ERROR_CODE 0x0000004C /* CORE Code Indicating Source of Error */ 00259 #define REG_CORE_ALERT_CODE_RESET 0x00000000 /* Reset Value for Alert_Code */ 00260 #define REG_CORE_ALERT_CODE 0x0000004E /* CORE Code Indicating Source of Alert */ 00261 #define REG_CORE_EXTERNAL_REFERENCE_RESISTOR_RESET 0x00000000 /* Reset Value for External_Reference_Resistor */ 00262 #define REG_CORE_EXTERNAL_REFERENCE_RESISTOR 0x00000050 /* CORE External Reference Information */ 00263 #define REG_CORE_EXTERNAL_VOLTAGE_REFERENCE_RESET 0x00000000 /* Reset Value for External_Voltage_Reference */ 00264 #define REG_CORE_EXTERNAL_VOLTAGE_REFERENCE 0x00000054 /* CORE External Reference Information */ 00265 #define REG_CORE_DIAGNOSTICS_CONTROL_RESET 0x00000000 /* Reset Value for Diagnostics_Control */ 00266 #define REG_CORE_DIAGNOSTICS_CONTROL 0x0000005C /* CORE Diagnostic Control */ 00267 #define REG_CORE_DATA_FIFO_RESET 0x00000000 /* Reset Value for Data_FIFO */ 00268 #define REG_CORE_DATA_FIFO 0x00000060 /* CORE FIFO Buffer of Sensor Results */ 00269 #define REG_CORE_DEBUG_CODE_RESET 0x00000000 /* Reset Value for Debug_Code */ 00270 #define REG_CORE_DEBUG_CODE 0x00000064 /* CORE Additional Information on Source of Alert or Errors */ 00271 #define REG_CORE_ADVANCED_SENSOR_ACCESS_RESET 0x00000000 /* Reset Value for Advanced_Sensor_Access */ 00272 #define REG_CORE_ADVANCED_SENSOR_ACCESS 0x0000006E /* CORE Enables Access to Advanced Sensor Configuration */ 00273 #define REG_CORE_LUT_SELECT_RESET 0x00000000 /* Reset Value for LUT_Select */ 00274 #define REG_CORE_LUT_SELECT 0x00000070 /* CORE Read/Write Strobe */ 00275 #define REG_CORE_LUT_OFFSET_RESET 0x00000000 /* Reset Value for LUT_Offset */ 00276 #define REG_CORE_LUT_OFFSET 0x00000072 /* CORE Offset into Selected LUT */ 00277 #define REG_CORE_LUT_DATA_RESET 0x00000000 /* Reset Value for LUT_Data */ 00278 #define REG_CORE_LUT_DATA 0x00000074 /* CORE Data to Read/Write from Addressed LUT Entry */ 00279 #define REG_CORE_REVISION_RESET 0x00000000 /* Reset Value for Revision */ 00280 #define REG_CORE_REVISION 0x0000008C /* CORE Hardware, Firmware Revision */ 00281 #define REG_CORE_CHANNEL_COUNTn_RESET 0x00000000 /* Reset Value for Channel_Count[n] */ 00282 #define REG_CORE_CHANNEL_COUNT0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT0 */ 00283 #define REG_CORE_CHANNEL_COUNT1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT1 */ 00284 #define REG_CORE_CHANNEL_COUNT2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT2 */ 00285 #define REG_CORE_CHANNEL_COUNT3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT3 */ 00286 #define REG_CORE_CHANNEL_COUNT4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT4 */ 00287 #define REG_CORE_CHANNEL_COUNT5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT5 */ 00288 #define REG_CORE_CHANNEL_COUNT6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT6 */ 00289 #define REG_CORE_CHANNEL_COUNT7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT7 */ 00290 #define REG_CORE_CHANNEL_COUNT8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT8 */ 00291 #define REG_CORE_CHANNEL_COUNT9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT9 */ 00292 #define REG_CORE_CHANNEL_COUNT10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT10 */ 00293 #define REG_CORE_CHANNEL_COUNT11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT11 */ 00294 #define REG_CORE_CHANNEL_COUNT12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT12 */ 00295 #define REG_CORE_CHANNEL_COUNT0 0x00000090 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00296 #define REG_CORE_CHANNEL_COUNT1 0x000000D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00297 #define REG_CORE_CHANNEL_COUNT2 0x00000110 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00298 #define REG_CORE_CHANNEL_COUNT3 0x00000150 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00299 #define REG_CORE_CHANNEL_COUNT4 0x00000190 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00300 #define REG_CORE_CHANNEL_COUNT5 0x000001D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00301 #define REG_CORE_CHANNEL_COUNT6 0x00000210 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00302 #define REG_CORE_CHANNEL_COUNT7 0x00000250 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00303 #define REG_CORE_CHANNEL_COUNT8 0x00000290 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00304 #define REG_CORE_CHANNEL_COUNT9 0x000002D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00305 #define REG_CORE_CHANNEL_COUNT10 0x00000310 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00306 #define REG_CORE_CHANNEL_COUNT11 0x00000350 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00307 #define REG_CORE_CHANNEL_COUNT12 0x00000390 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00308 #define REG_CORE_CHANNEL_COUNTn(i) (REG_CORE_CHANNEL_COUNT0 + ((i) * 64)) 00309 #define REG_CORE_CHANNEL_COUNTn_COUNT 13 00310 #define REG_CORE_CHANNEL_OPTIONSn_RESET 0x00000000 /* Reset Value for Channel_Options[n] */ 00311 #define REG_CORE_CHANNEL_OPTIONS0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS0 */ 00312 #define REG_CORE_CHANNEL_OPTIONS1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS1 */ 00313 #define REG_CORE_CHANNEL_OPTIONS2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS2 */ 00314 #define REG_CORE_CHANNEL_OPTIONS3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS3 */ 00315 #define REG_CORE_CHANNEL_OPTIONS4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS4 */ 00316 #define REG_CORE_CHANNEL_OPTIONS5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS5 */ 00317 #define REG_CORE_CHANNEL_OPTIONS6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS6 */ 00318 #define REG_CORE_CHANNEL_OPTIONS7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS7 */ 00319 #define REG_CORE_CHANNEL_OPTIONS8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS8 */ 00320 #define REG_CORE_CHANNEL_OPTIONS9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS9 */ 00321 #define REG_CORE_CHANNEL_OPTIONS10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS10 */ 00322 #define REG_CORE_CHANNEL_OPTIONS11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS11 */ 00323 #define REG_CORE_CHANNEL_OPTIONS12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS12 */ 00324 #define REG_CORE_CHANNEL_OPTIONS0 0x00000091 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00325 #define REG_CORE_CHANNEL_OPTIONS1 0x000000D1 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00326 #define REG_CORE_CHANNEL_OPTIONS2 0x00000111 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00327 #define REG_CORE_CHANNEL_OPTIONS3 0x00000151 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00328 #define REG_CORE_CHANNEL_OPTIONS4 0x00000191 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00329 #define REG_CORE_CHANNEL_OPTIONS5 0x000001D1 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00330 #define REG_CORE_CHANNEL_OPTIONS6 0x00000211 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00331 #define REG_CORE_CHANNEL_OPTIONS7 0x00000251 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00332 #define REG_CORE_CHANNEL_OPTIONS8 0x00000291 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00333 #define REG_CORE_CHANNEL_OPTIONS9 0x000002D1 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00334 #define REG_CORE_CHANNEL_OPTIONS10 0x00000311 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00335 #define REG_CORE_CHANNEL_OPTIONS11 0x00000351 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00336 #define REG_CORE_CHANNEL_OPTIONS12 0x00000391 /* CORE Position of Channel Within Sequence and Enable for FFT */ 00337 #define REG_CORE_CHANNEL_OPTIONSn(i) (REG_CORE_CHANNEL_OPTIONS0 + ((i) * 64)) 00338 #define REG_CORE_CHANNEL_OPTIONSn_COUNT 13 00339 #define REG_CORE_SENSOR_TYPEn_RESET 0x00000000 /* Reset Value for Sensor_Type[n] */ 00340 #define REG_CORE_SENSOR_TYPE0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE0 */ 00341 #define REG_CORE_SENSOR_TYPE1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE1 */ 00342 #define REG_CORE_SENSOR_TYPE2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE2 */ 00343 #define REG_CORE_SENSOR_TYPE3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE3 */ 00344 #define REG_CORE_SENSOR_TYPE4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE4 */ 00345 #define REG_CORE_SENSOR_TYPE5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE5 */ 00346 #define REG_CORE_SENSOR_TYPE6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE6 */ 00347 #define REG_CORE_SENSOR_TYPE7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE7 */ 00348 #define REG_CORE_SENSOR_TYPE8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE8 */ 00349 #define REG_CORE_SENSOR_TYPE9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE9 */ 00350 #define REG_CORE_SENSOR_TYPE10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE10 */ 00351 #define REG_CORE_SENSOR_TYPE11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE11 */ 00352 #define REG_CORE_SENSOR_TYPE12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE12 */ 00353 #define REG_CORE_SENSOR_TYPE0 0x00000092 /* CORE Sensor Select */ 00354 #define REG_CORE_SENSOR_TYPE1 0x000000D2 /* CORE Sensor Select */ 00355 #define REG_CORE_SENSOR_TYPE2 0x00000112 /* CORE Sensor Select */ 00356 #define REG_CORE_SENSOR_TYPE3 0x00000152 /* CORE Sensor Select */ 00357 #define REG_CORE_SENSOR_TYPE4 0x00000192 /* CORE Sensor Select */ 00358 #define REG_CORE_SENSOR_TYPE5 0x000001D2 /* CORE Sensor Select */ 00359 #define REG_CORE_SENSOR_TYPE6 0x00000212 /* CORE Sensor Select */ 00360 #define REG_CORE_SENSOR_TYPE7 0x00000252 /* CORE Sensor Select */ 00361 #define REG_CORE_SENSOR_TYPE8 0x00000292 /* CORE Sensor Select */ 00362 #define REG_CORE_SENSOR_TYPE9 0x000002D2 /* CORE Sensor Select */ 00363 #define REG_CORE_SENSOR_TYPE10 0x00000312 /* CORE Sensor Select */ 00364 #define REG_CORE_SENSOR_TYPE11 0x00000352 /* CORE Sensor Select */ 00365 #define REG_CORE_SENSOR_TYPE12 0x00000392 /* CORE Sensor Select */ 00366 #define REG_CORE_SENSOR_TYPEn(i) (REG_CORE_SENSOR_TYPE0 + ((i) * 64)) 00367 #define REG_CORE_SENSOR_TYPEn_COUNT 13 00368 #define REG_CORE_SENSOR_DETAILSn_RESET 0x000000F0 /* Reset Value for Sensor_Details[n] */ 00369 #define REG_CORE_SENSOR_DETAILS0_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS0 */ 00370 #define REG_CORE_SENSOR_DETAILS1_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS1 */ 00371 #define REG_CORE_SENSOR_DETAILS2_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS2 */ 00372 #define REG_CORE_SENSOR_DETAILS3_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS3 */ 00373 #define REG_CORE_SENSOR_DETAILS4_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS4 */ 00374 #define REG_CORE_SENSOR_DETAILS5_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS5 */ 00375 #define REG_CORE_SENSOR_DETAILS6_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS6 */ 00376 #define REG_CORE_SENSOR_DETAILS7_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS7 */ 00377 #define REG_CORE_SENSOR_DETAILS8_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS8 */ 00378 #define REG_CORE_SENSOR_DETAILS9_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS9 */ 00379 #define REG_CORE_SENSOR_DETAILS10_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS10 */ 00380 #define REG_CORE_SENSOR_DETAILS11_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS11 */ 00381 #define REG_CORE_SENSOR_DETAILS12_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS12 */ 00382 #define REG_CORE_SENSOR_DETAILS0 0x00000094 /* CORE Sensor Details */ 00383 #define REG_CORE_SENSOR_DETAILS1 0x000000D4 /* CORE Sensor Details */ 00384 #define REG_CORE_SENSOR_DETAILS2 0x00000114 /* CORE Sensor Details */ 00385 #define REG_CORE_SENSOR_DETAILS3 0x00000154 /* CORE Sensor Details */ 00386 #define REG_CORE_SENSOR_DETAILS4 0x00000194 /* CORE Sensor Details */ 00387 #define REG_CORE_SENSOR_DETAILS5 0x000001D4 /* CORE Sensor Details */ 00388 #define REG_CORE_SENSOR_DETAILS6 0x00000214 /* CORE Sensor Details */ 00389 #define REG_CORE_SENSOR_DETAILS7 0x00000254 /* CORE Sensor Details */ 00390 #define REG_CORE_SENSOR_DETAILS8 0x00000294 /* CORE Sensor Details */ 00391 #define REG_CORE_SENSOR_DETAILS9 0x000002D4 /* CORE Sensor Details */ 00392 #define REG_CORE_SENSOR_DETAILS10 0x00000314 /* CORE Sensor Details */ 00393 #define REG_CORE_SENSOR_DETAILS11 0x00000354 /* CORE Sensor Details */ 00394 #define REG_CORE_SENSOR_DETAILS12 0x00000394 /* CORE Sensor Details */ 00395 #define REG_CORE_SENSOR_DETAILSn(i) (REG_CORE_SENSOR_DETAILS0 + ((i) * 64)) 00396 #define REG_CORE_SENSOR_DETAILSn_COUNT 13 00397 #define REG_CORE_CHANNEL_EXCITATIONn_RESET 0x00000000 /* Reset Value for Channel_Excitation[n] */ 00398 #define REG_CORE_CHANNEL_EXCITATION0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION0 */ 00399 #define REG_CORE_CHANNEL_EXCITATION1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION1 */ 00400 #define REG_CORE_CHANNEL_EXCITATION2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION2 */ 00401 #define REG_CORE_CHANNEL_EXCITATION3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION3 */ 00402 #define REG_CORE_CHANNEL_EXCITATION4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION4 */ 00403 #define REG_CORE_CHANNEL_EXCITATION5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION5 */ 00404 #define REG_CORE_CHANNEL_EXCITATION6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION6 */ 00405 #define REG_CORE_CHANNEL_EXCITATION7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION7 */ 00406 #define REG_CORE_CHANNEL_EXCITATION8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION8 */ 00407 #define REG_CORE_CHANNEL_EXCITATION9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION9 */ 00408 #define REG_CORE_CHANNEL_EXCITATION10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION10 */ 00409 #define REG_CORE_CHANNEL_EXCITATION11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION11 */ 00410 #define REG_CORE_CHANNEL_EXCITATION12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION12 */ 00411 #define REG_CORE_CHANNEL_EXCITATION0 0x00000098 /* CORE Excitation Current */ 00412 #define REG_CORE_CHANNEL_EXCITATION1 0x000000D8 /* CORE Excitation Current */ 00413 #define REG_CORE_CHANNEL_EXCITATION2 0x00000118 /* CORE Excitation Current */ 00414 #define REG_CORE_CHANNEL_EXCITATION3 0x00000158 /* CORE Excitation Current */ 00415 #define REG_CORE_CHANNEL_EXCITATION4 0x00000198 /* CORE Excitation Current */ 00416 #define REG_CORE_CHANNEL_EXCITATION5 0x000001D8 /* CORE Excitation Current */ 00417 #define REG_CORE_CHANNEL_EXCITATION6 0x00000218 /* CORE Excitation Current */ 00418 #define REG_CORE_CHANNEL_EXCITATION7 0x00000258 /* CORE Excitation Current */ 00419 #define REG_CORE_CHANNEL_EXCITATION8 0x00000298 /* CORE Excitation Current */ 00420 #define REG_CORE_CHANNEL_EXCITATION9 0x000002D8 /* CORE Excitation Current */ 00421 #define REG_CORE_CHANNEL_EXCITATION10 0x00000318 /* CORE Excitation Current */ 00422 #define REG_CORE_CHANNEL_EXCITATION11 0x00000358 /* CORE Excitation Current */ 00423 #define REG_CORE_CHANNEL_EXCITATION12 0x00000398 /* CORE Excitation Current */ 00424 #define REG_CORE_CHANNEL_EXCITATIONn(i) (REG_CORE_CHANNEL_EXCITATION0 + ((i) * 64)) 00425 #define REG_CORE_CHANNEL_EXCITATIONn_COUNT 13 00426 #define REG_CORE_SETTLING_TIMEn_RESET 0x00000000 /* Reset Value for Settling_Time[n] */ 00427 #define REG_CORE_SETTLING_TIME0_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME0 */ 00428 #define REG_CORE_SETTLING_TIME1_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME1 */ 00429 #define REG_CORE_SETTLING_TIME2_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME2 */ 00430 #define REG_CORE_SETTLING_TIME3_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME3 */ 00431 #define REG_CORE_SETTLING_TIME4_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME4 */ 00432 #define REG_CORE_SETTLING_TIME5_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME5 */ 00433 #define REG_CORE_SETTLING_TIME6_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME6 */ 00434 #define REG_CORE_SETTLING_TIME7_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME7 */ 00435 #define REG_CORE_SETTLING_TIME8_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME8 */ 00436 #define REG_CORE_SETTLING_TIME9_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME9 */ 00437 #define REG_CORE_SETTLING_TIME10_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME10 */ 00438 #define REG_CORE_SETTLING_TIME11_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME11 */ 00439 #define REG_CORE_SETTLING_TIME12_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME12 */ 00440 #define REG_CORE_SETTLING_TIME0 0x0000009A /* CORE Settling Time */ 00441 #define REG_CORE_SETTLING_TIME1 0x000000DA /* CORE Settling Time */ 00442 #define REG_CORE_SETTLING_TIME2 0x0000011A /* CORE Settling Time */ 00443 #define REG_CORE_SETTLING_TIME3 0x0000015A /* CORE Settling Time */ 00444 #define REG_CORE_SETTLING_TIME4 0x0000019A /* CORE Settling Time */ 00445 #define REG_CORE_SETTLING_TIME5 0x000001DA /* CORE Settling Time */ 00446 #define REG_CORE_SETTLING_TIME6 0x0000021A /* CORE Settling Time */ 00447 #define REG_CORE_SETTLING_TIME7 0x0000025A /* CORE Settling Time */ 00448 #define REG_CORE_SETTLING_TIME8 0x0000029A /* CORE Settling Time */ 00449 #define REG_CORE_SETTLING_TIME9 0x000002DA /* CORE Settling Time */ 00450 #define REG_CORE_SETTLING_TIME10 0x0000031A /* CORE Settling Time */ 00451 #define REG_CORE_SETTLING_TIME11 0x0000035A /* CORE Settling Time */ 00452 #define REG_CORE_SETTLING_TIME12 0x0000039A /* CORE Settling Time */ 00453 #define REG_CORE_SETTLING_TIMEn(i) (REG_CORE_SETTLING_TIME0 + ((i) * 64)) 00454 #define REG_CORE_SETTLING_TIMEn_COUNT 13 00455 #define REG_CORE_MEASUREMENT_SETUPn_RESET 0x00000000 /* Reset Value for Measurement_Setup[n] */ 00456 #define REG_CORE_MEASUREMENT_SETUP0_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP0 */ 00457 #define REG_CORE_MEASUREMENT_SETUP1_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP1 */ 00458 #define REG_CORE_MEASUREMENT_SETUP2_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP2 */ 00459 #define REG_CORE_MEASUREMENT_SETUP3_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP3 */ 00460 #define REG_CORE_MEASUREMENT_SETUP4_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP4 */ 00461 #define REG_CORE_MEASUREMENT_SETUP5_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP5 */ 00462 #define REG_CORE_MEASUREMENT_SETUP6_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP6 */ 00463 #define REG_CORE_MEASUREMENT_SETUP7_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP7 */ 00464 #define REG_CORE_MEASUREMENT_SETUP8_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP8 */ 00465 #define REG_CORE_MEASUREMENT_SETUP9_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP9 */ 00466 #define REG_CORE_MEASUREMENT_SETUP10_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP10 */ 00467 #define REG_CORE_MEASUREMENT_SETUP11_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP11 */ 00468 #define REG_CORE_MEASUREMENT_SETUP12_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP12 */ 00469 #define REG_CORE_MEASUREMENT_SETUP0 0x0000009C /* CORE ADC Digital Filter Selection */ 00470 #define REG_CORE_MEASUREMENT_SETUP1 0x000000DC /* CORE ADC Digital Filter Selection */ 00471 #define REG_CORE_MEASUREMENT_SETUP2 0x0000011C /* CORE ADC Digital Filter Selection */ 00472 #define REG_CORE_MEASUREMENT_SETUP3 0x0000015C /* CORE ADC Digital Filter Selection */ 00473 #define REG_CORE_MEASUREMENT_SETUP4 0x0000019C /* CORE ADC Digital Filter Selection */ 00474 #define REG_CORE_MEASUREMENT_SETUP5 0x000001DC /* CORE ADC Digital Filter Selection */ 00475 #define REG_CORE_MEASUREMENT_SETUP6 0x0000021C /* CORE ADC Digital Filter Selection */ 00476 #define REG_CORE_MEASUREMENT_SETUP7 0x0000025C /* CORE ADC Digital Filter Selection */ 00477 #define REG_CORE_MEASUREMENT_SETUP8 0x0000029C /* CORE ADC Digital Filter Selection */ 00478 #define REG_CORE_MEASUREMENT_SETUP9 0x000002DC /* CORE ADC Digital Filter Selection */ 00479 #define REG_CORE_MEASUREMENT_SETUP10 0x0000031C /* CORE ADC Digital Filter Selection */ 00480 #define REG_CORE_MEASUREMENT_SETUP11 0x0000035C /* CORE ADC Digital Filter Selection */ 00481 #define REG_CORE_MEASUREMENT_SETUP12 0x0000039C /* CORE ADC Digital Filter Selection */ 00482 #define REG_CORE_MEASUREMENT_SETUPn(i) (REG_CORE_MEASUREMENT_SETUP0 + ((i) * 64)) 00483 #define REG_CORE_MEASUREMENT_SETUPn_COUNT 13 00484 #define REG_CORE_HIGH_THRESHOLD_LIMITn_RESET 0x00000000 /* Reset Value for High_Threshold_Limit[n] */ 00485 #define REG_CORE_HIGH_THRESHOLD_LIMIT0_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT0 */ 00486 #define REG_CORE_HIGH_THRESHOLD_LIMIT1_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT1 */ 00487 #define REG_CORE_HIGH_THRESHOLD_LIMIT2_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT2 */ 00488 #define REG_CORE_HIGH_THRESHOLD_LIMIT3_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT3 */ 00489 #define REG_CORE_HIGH_THRESHOLD_LIMIT4_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT4 */ 00490 #define REG_CORE_HIGH_THRESHOLD_LIMIT5_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT5 */ 00491 #define REG_CORE_HIGH_THRESHOLD_LIMIT6_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT6 */ 00492 #define REG_CORE_HIGH_THRESHOLD_LIMIT7_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT7 */ 00493 #define REG_CORE_HIGH_THRESHOLD_LIMIT8_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT8 */ 00494 #define REG_CORE_HIGH_THRESHOLD_LIMIT9_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT9 */ 00495 #define REG_CORE_HIGH_THRESHOLD_LIMIT10_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT10 */ 00496 #define REG_CORE_HIGH_THRESHOLD_LIMIT11_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT11 */ 00497 #define REG_CORE_HIGH_THRESHOLD_LIMIT12_RESET 0x00000000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT12 */ 00498 #define REG_CORE_HIGH_THRESHOLD_LIMIT0 0x000000A0 /* CORE High Threshold */ 00499 #define REG_CORE_HIGH_THRESHOLD_LIMIT1 0x000000E0 /* CORE High Threshold */ 00500 #define REG_CORE_HIGH_THRESHOLD_LIMIT2 0x00000120 /* CORE High Threshold */ 00501 #define REG_CORE_HIGH_THRESHOLD_LIMIT3 0x00000160 /* CORE High Threshold */ 00502 #define REG_CORE_HIGH_THRESHOLD_LIMIT4 0x000001A0 /* CORE High Threshold */ 00503 #define REG_CORE_HIGH_THRESHOLD_LIMIT5 0x000001E0 /* CORE High Threshold */ 00504 #define REG_CORE_HIGH_THRESHOLD_LIMIT6 0x00000220 /* CORE High Threshold */ 00505 #define REG_CORE_HIGH_THRESHOLD_LIMIT7 0x00000260 /* CORE High Threshold */ 00506 #define REG_CORE_HIGH_THRESHOLD_LIMIT8 0x000002A0 /* CORE High Threshold */ 00507 #define REG_CORE_HIGH_THRESHOLD_LIMIT9 0x000002E0 /* CORE High Threshold */ 00508 #define REG_CORE_HIGH_THRESHOLD_LIMIT10 0x00000320 /* CORE High Threshold */ 00509 #define REG_CORE_HIGH_THRESHOLD_LIMIT11 0x00000360 /* CORE High Threshold */ 00510 #define REG_CORE_HIGH_THRESHOLD_LIMIT12 0x000003A0 /* CORE High Threshold */ 00511 #define REG_CORE_HIGH_THRESHOLD_LIMITn(i) (REG_CORE_HIGH_THRESHOLD_LIMIT0 + ((i) * 64)) 00512 #define REG_CORE_HIGH_THRESHOLD_LIMITn_COUNT 13 00513 #define REG_CORE_LOW_THRESHOLD_LIMITn_RESET 0x00000000 /* Reset Value for Low_Threshold_Limit[n] */ 00514 #define REG_CORE_LOW_THRESHOLD_LIMIT0_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT0 */ 00515 #define REG_CORE_LOW_THRESHOLD_LIMIT1_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT1 */ 00516 #define REG_CORE_LOW_THRESHOLD_LIMIT2_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT2 */ 00517 #define REG_CORE_LOW_THRESHOLD_LIMIT3_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT3 */ 00518 #define REG_CORE_LOW_THRESHOLD_LIMIT4_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT4 */ 00519 #define REG_CORE_LOW_THRESHOLD_LIMIT5_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT5 */ 00520 #define REG_CORE_LOW_THRESHOLD_LIMIT6_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT6 */ 00521 #define REG_CORE_LOW_THRESHOLD_LIMIT7_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT7 */ 00522 #define REG_CORE_LOW_THRESHOLD_LIMIT8_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT8 */ 00523 #define REG_CORE_LOW_THRESHOLD_LIMIT9_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT9 */ 00524 #define REG_CORE_LOW_THRESHOLD_LIMIT10_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT10 */ 00525 #define REG_CORE_LOW_THRESHOLD_LIMIT11_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT11 */ 00526 #define REG_CORE_LOW_THRESHOLD_LIMIT12_RESET 0x00000000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT12 */ 00527 #define REG_CORE_LOW_THRESHOLD_LIMIT0 0x000000A4 /* CORE Low Threshold */ 00528 #define REG_CORE_LOW_THRESHOLD_LIMIT1 0x000000E4 /* CORE Low Threshold */ 00529 #define REG_CORE_LOW_THRESHOLD_LIMIT2 0x00000124 /* CORE Low Threshold */ 00530 #define REG_CORE_LOW_THRESHOLD_LIMIT3 0x00000164 /* CORE Low Threshold */ 00531 #define REG_CORE_LOW_THRESHOLD_LIMIT4 0x000001A4 /* CORE Low Threshold */ 00532 #define REG_CORE_LOW_THRESHOLD_LIMIT5 0x000001E4 /* CORE Low Threshold */ 00533 #define REG_CORE_LOW_THRESHOLD_LIMIT6 0x00000224 /* CORE Low Threshold */ 00534 #define REG_CORE_LOW_THRESHOLD_LIMIT7 0x00000264 /* CORE Low Threshold */ 00535 #define REG_CORE_LOW_THRESHOLD_LIMIT8 0x000002A4 /* CORE Low Threshold */ 00536 #define REG_CORE_LOW_THRESHOLD_LIMIT9 0x000002E4 /* CORE Low Threshold */ 00537 #define REG_CORE_LOW_THRESHOLD_LIMIT10 0x00000324 /* CORE Low Threshold */ 00538 #define REG_CORE_LOW_THRESHOLD_LIMIT11 0x00000364 /* CORE Low Threshold */ 00539 #define REG_CORE_LOW_THRESHOLD_LIMIT12 0x000003A4 /* CORE Low Threshold */ 00540 #define REG_CORE_LOW_THRESHOLD_LIMITn(i) (REG_CORE_LOW_THRESHOLD_LIMIT0 + ((i) * 64)) 00541 #define REG_CORE_LOW_THRESHOLD_LIMITn_COUNT 13 00542 #define REG_CORE_SENSOR_OFFSETn_RESET 0x00000000 /* Reset Value for Sensor_Offset[n] */ 00543 #define REG_CORE_SENSOR_OFFSET0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET0 */ 00544 #define REG_CORE_SENSOR_OFFSET1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET1 */ 00545 #define REG_CORE_SENSOR_OFFSET2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET2 */ 00546 #define REG_CORE_SENSOR_OFFSET3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET3 */ 00547 #define REG_CORE_SENSOR_OFFSET4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET4 */ 00548 #define REG_CORE_SENSOR_OFFSET5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET5 */ 00549 #define REG_CORE_SENSOR_OFFSET6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET6 */ 00550 #define REG_CORE_SENSOR_OFFSET7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET7 */ 00551 #define REG_CORE_SENSOR_OFFSET8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET8 */ 00552 #define REG_CORE_SENSOR_OFFSET9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET9 */ 00553 #define REG_CORE_SENSOR_OFFSET10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET10 */ 00554 #define REG_CORE_SENSOR_OFFSET11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET11 */ 00555 #define REG_CORE_SENSOR_OFFSET12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET12 */ 00556 #define REG_CORE_SENSOR_OFFSET0 0x000000A8 /* CORE Sensor Offset Adjustment */ 00557 #define REG_CORE_SENSOR_OFFSET1 0x000000E8 /* CORE Sensor Offset Adjustment */ 00558 #define REG_CORE_SENSOR_OFFSET2 0x00000128 /* CORE Sensor Offset Adjustment */ 00559 #define REG_CORE_SENSOR_OFFSET3 0x00000168 /* CORE Sensor Offset Adjustment */ 00560 #define REG_CORE_SENSOR_OFFSET4 0x000001A8 /* CORE Sensor Offset Adjustment */ 00561 #define REG_CORE_SENSOR_OFFSET5 0x000001E8 /* CORE Sensor Offset Adjustment */ 00562 #define REG_CORE_SENSOR_OFFSET6 0x00000228 /* CORE Sensor Offset Adjustment */ 00563 #define REG_CORE_SENSOR_OFFSET7 0x00000268 /* CORE Sensor Offset Adjustment */ 00564 #define REG_CORE_SENSOR_OFFSET8 0x000002A8 /* CORE Sensor Offset Adjustment */ 00565 #define REG_CORE_SENSOR_OFFSET9 0x000002E8 /* CORE Sensor Offset Adjustment */ 00566 #define REG_CORE_SENSOR_OFFSET10 0x00000328 /* CORE Sensor Offset Adjustment */ 00567 #define REG_CORE_SENSOR_OFFSET11 0x00000368 /* CORE Sensor Offset Adjustment */ 00568 #define REG_CORE_SENSOR_OFFSET12 0x000003A8 /* CORE Sensor Offset Adjustment */ 00569 #define REG_CORE_SENSOR_OFFSETn(i) (REG_CORE_SENSOR_OFFSET0 + ((i) * 64)) 00570 #define REG_CORE_SENSOR_OFFSETn_COUNT 13 00571 #define REG_CORE_SENSOR_GAINn_RESET 0x00000000 /* Reset Value for Sensor_Gain[n] */ 00572 #define REG_CORE_SENSOR_GAIN0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN0 */ 00573 #define REG_CORE_SENSOR_GAIN1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN1 */ 00574 #define REG_CORE_SENSOR_GAIN2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN2 */ 00575 #define REG_CORE_SENSOR_GAIN3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN3 */ 00576 #define REG_CORE_SENSOR_GAIN4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN4 */ 00577 #define REG_CORE_SENSOR_GAIN5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN5 */ 00578 #define REG_CORE_SENSOR_GAIN6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN6 */ 00579 #define REG_CORE_SENSOR_GAIN7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN7 */ 00580 #define REG_CORE_SENSOR_GAIN8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN8 */ 00581 #define REG_CORE_SENSOR_GAIN9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN9 */ 00582 #define REG_CORE_SENSOR_GAIN10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN10 */ 00583 #define REG_CORE_SENSOR_GAIN11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN11 */ 00584 #define REG_CORE_SENSOR_GAIN12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_GAIN12 */ 00585 #define REG_CORE_SENSOR_GAIN0 0x000000AC /* CORE Sensor Gain Adjustment */ 00586 #define REG_CORE_SENSOR_GAIN1 0x000000EC /* CORE Sensor Gain Adjustment */ 00587 #define REG_CORE_SENSOR_GAIN2 0x0000012C /* CORE Sensor Gain Adjustment */ 00588 #define REG_CORE_SENSOR_GAIN3 0x0000016C /* CORE Sensor Gain Adjustment */ 00589 #define REG_CORE_SENSOR_GAIN4 0x000001AC /* CORE Sensor Gain Adjustment */ 00590 #define REG_CORE_SENSOR_GAIN5 0x000001EC /* CORE Sensor Gain Adjustment */ 00591 #define REG_CORE_SENSOR_GAIN6 0x0000022C /* CORE Sensor Gain Adjustment */ 00592 #define REG_CORE_SENSOR_GAIN7 0x0000026C /* CORE Sensor Gain Adjustment */ 00593 #define REG_CORE_SENSOR_GAIN8 0x000002AC /* CORE Sensor Gain Adjustment */ 00594 #define REG_CORE_SENSOR_GAIN9 0x000002EC /* CORE Sensor Gain Adjustment */ 00595 #define REG_CORE_SENSOR_GAIN10 0x0000032C /* CORE Sensor Gain Adjustment */ 00596 #define REG_CORE_SENSOR_GAIN11 0x0000036C /* CORE Sensor Gain Adjustment */ 00597 #define REG_CORE_SENSOR_GAIN12 0x000003AC /* CORE Sensor Gain Adjustment */ 00598 #define REG_CORE_SENSOR_GAINn(i) (REG_CORE_SENSOR_GAIN0 + ((i) * 64)) 00599 #define REG_CORE_SENSOR_GAINn_COUNT 13 00600 #define REG_CORE_ALERT_CODE_CHn_RESET 0x00000000 /* Reset Value for Alert_Code_Ch[n] */ 00601 #define REG_CORE_ALERT_CODE_CH0_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH0 */ 00602 #define REG_CORE_ALERT_CODE_CH1_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH1 */ 00603 #define REG_CORE_ALERT_CODE_CH2_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH2 */ 00604 #define REG_CORE_ALERT_CODE_CH3_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH3 */ 00605 #define REG_CORE_ALERT_CODE_CH4_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH4 */ 00606 #define REG_CORE_ALERT_CODE_CH5_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH5 */ 00607 #define REG_CORE_ALERT_CODE_CH6_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH6 */ 00608 #define REG_CORE_ALERT_CODE_CH7_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH7 */ 00609 #define REG_CORE_ALERT_CODE_CH8_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH8 */ 00610 #define REG_CORE_ALERT_CODE_CH9_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH9 */ 00611 #define REG_CORE_ALERT_CODE_CH10_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH10 */ 00612 #define REG_CORE_ALERT_CODE_CH11_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH11 */ 00613 #define REG_CORE_ALERT_CODE_CH12_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_CODE_CH12 */ 00614 #define REG_CORE_ALERT_CODE_CH0 0x000000B0 /* CORE Per-Channel Detailed Alert-Code Information */ 00615 #define REG_CORE_ALERT_CODE_CH1 0x000000F0 /* CORE Per-Channel Detailed Alert-Code Information */ 00616 #define REG_CORE_ALERT_CODE_CH2 0x00000130 /* CORE Per-Channel Detailed Alert-Code Information */ 00617 #define REG_CORE_ALERT_CODE_CH3 0x00000170 /* CORE Per-Channel Detailed Alert-Code Information */ 00618 #define REG_CORE_ALERT_CODE_CH4 0x000001B0 /* CORE Per-Channel Detailed Alert-Code Information */ 00619 #define REG_CORE_ALERT_CODE_CH5 0x000001F0 /* CORE Per-Channel Detailed Alert-Code Information */ 00620 #define REG_CORE_ALERT_CODE_CH6 0x00000230 /* CORE Per-Channel Detailed Alert-Code Information */ 00621 #define REG_CORE_ALERT_CODE_CH7 0x00000270 /* CORE Per-Channel Detailed Alert-Code Information */ 00622 #define REG_CORE_ALERT_CODE_CH8 0x000002B0 /* CORE Per-Channel Detailed Alert-Code Information */ 00623 #define REG_CORE_ALERT_CODE_CH9 0x000002F0 /* CORE Per-Channel Detailed Alert-Code Information */ 00624 #define REG_CORE_ALERT_CODE_CH10 0x00000330 /* CORE Per-Channel Detailed Alert-Code Information */ 00625 #define REG_CORE_ALERT_CODE_CH11 0x00000370 /* CORE Per-Channel Detailed Alert-Code Information */ 00626 #define REG_CORE_ALERT_CODE_CH12 0x000003B0 /* CORE Per-Channel Detailed Alert-Code Information */ 00627 #define REG_CORE_ALERT_CODE_CHn(i) (REG_CORE_ALERT_CODE_CH0 + ((i) * 64)) 00628 #define REG_CORE_ALERT_CODE_CHn_COUNT 13 00629 #define REG_CORE_CHANNEL_SKIPn_RESET 0x00000000 /* Reset Value for Channel_Skip[n] */ 00630 #define REG_CORE_CHANNEL_SKIP0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP0 */ 00631 #define REG_CORE_CHANNEL_SKIP1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP1 */ 00632 #define REG_CORE_CHANNEL_SKIP2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP2 */ 00633 #define REG_CORE_CHANNEL_SKIP3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP3 */ 00634 #define REG_CORE_CHANNEL_SKIP4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP4 */ 00635 #define REG_CORE_CHANNEL_SKIP5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP5 */ 00636 #define REG_CORE_CHANNEL_SKIP6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP6 */ 00637 #define REG_CORE_CHANNEL_SKIP7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP7 */ 00638 #define REG_CORE_CHANNEL_SKIP8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP8 */ 00639 #define REG_CORE_CHANNEL_SKIP9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP9 */ 00640 #define REG_CORE_CHANNEL_SKIP10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP10 */ 00641 #define REG_CORE_CHANNEL_SKIP11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP11 */ 00642 #define REG_CORE_CHANNEL_SKIP12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP12 */ 00643 #define REG_CORE_CHANNEL_SKIP0 0x000000B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00644 #define REG_CORE_CHANNEL_SKIP1 0x000000F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00645 #define REG_CORE_CHANNEL_SKIP2 0x00000132 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00646 #define REG_CORE_CHANNEL_SKIP3 0x00000172 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00647 #define REG_CORE_CHANNEL_SKIP4 0x000001B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00648 #define REG_CORE_CHANNEL_SKIP5 0x000001F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00649 #define REG_CORE_CHANNEL_SKIP6 0x00000232 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00650 #define REG_CORE_CHANNEL_SKIP7 0x00000272 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00651 #define REG_CORE_CHANNEL_SKIP8 0x000002B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00652 #define REG_CORE_CHANNEL_SKIP9 0x000002F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00653 #define REG_CORE_CHANNEL_SKIP10 0x00000332 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00654 #define REG_CORE_CHANNEL_SKIP11 0x00000372 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00655 #define REG_CORE_CHANNEL_SKIP12 0x000003B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00656 #define REG_CORE_CHANNEL_SKIPn(i) (REG_CORE_CHANNEL_SKIP0 + ((i) * 64)) 00657 #define REG_CORE_CHANNEL_SKIPn_COUNT 13 00658 #define REG_CORE_SENSOR_PARAMETERn_RESET 0x00000000 /* Reset Value for Sensor_Parameter[n] */ 00659 #define REG_CORE_SENSOR_PARAMETER0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER0 */ 00660 #define REG_CORE_SENSOR_PARAMETER1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER1 */ 00661 #define REG_CORE_SENSOR_PARAMETER2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER2 */ 00662 #define REG_CORE_SENSOR_PARAMETER3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER3 */ 00663 #define REG_CORE_SENSOR_PARAMETER4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER4 */ 00664 #define REG_CORE_SENSOR_PARAMETER5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER5 */ 00665 #define REG_CORE_SENSOR_PARAMETER6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER6 */ 00666 #define REG_CORE_SENSOR_PARAMETER7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER7 */ 00667 #define REG_CORE_SENSOR_PARAMETER8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER8 */ 00668 #define REG_CORE_SENSOR_PARAMETER9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER9 */ 00669 #define REG_CORE_SENSOR_PARAMETER10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER10 */ 00670 #define REG_CORE_SENSOR_PARAMETER11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER11 */ 00671 #define REG_CORE_SENSOR_PARAMETER12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_PARAMETER12 */ 00672 #define REG_CORE_SENSOR_PARAMETER0 0x000000B4 /* CORE Sensor Parameter Adjustment */ 00673 #define REG_CORE_SENSOR_PARAMETER1 0x000000F4 /* CORE Sensor Parameter Adjustment */ 00674 #define REG_CORE_SENSOR_PARAMETER2 0x00000134 /* CORE Sensor Parameter Adjustment */ 00675 #define REG_CORE_SENSOR_PARAMETER3 0x00000174 /* CORE Sensor Parameter Adjustment */ 00676 #define REG_CORE_SENSOR_PARAMETER4 0x000001B4 /* CORE Sensor Parameter Adjustment */ 00677 #define REG_CORE_SENSOR_PARAMETER5 0x000001F4 /* CORE Sensor Parameter Adjustment */ 00678 #define REG_CORE_SENSOR_PARAMETER6 0x00000234 /* CORE Sensor Parameter Adjustment */ 00679 #define REG_CORE_SENSOR_PARAMETER7 0x00000274 /* CORE Sensor Parameter Adjustment */ 00680 #define REG_CORE_SENSOR_PARAMETER8 0x000002B4 /* CORE Sensor Parameter Adjustment */ 00681 #define REG_CORE_SENSOR_PARAMETER9 0x000002F4 /* CORE Sensor Parameter Adjustment */ 00682 #define REG_CORE_SENSOR_PARAMETER10 0x00000334 /* CORE Sensor Parameter Adjustment */ 00683 #define REG_CORE_SENSOR_PARAMETER11 0x00000374 /* CORE Sensor Parameter Adjustment */ 00684 #define REG_CORE_SENSOR_PARAMETER12 0x000003B4 /* CORE Sensor Parameter Adjustment */ 00685 #define REG_CORE_SENSOR_PARAMETERn(i) (REG_CORE_SENSOR_PARAMETER0 + ((i) * 64)) 00686 #define REG_CORE_SENSOR_PARAMETERn_COUNT 13 00687 #define REG_CORE_CALIBRATION_PARAMETERn_RESET 0x00000000 /* Reset Value for Calibration_Parameter[n] */ 00688 #define REG_CORE_CALIBRATION_PARAMETER0_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER0 */ 00689 #define REG_CORE_CALIBRATION_PARAMETER1_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER1 */ 00690 #define REG_CORE_CALIBRATION_PARAMETER2_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER2 */ 00691 #define REG_CORE_CALIBRATION_PARAMETER3_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER3 */ 00692 #define REG_CORE_CALIBRATION_PARAMETER4_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER4 */ 00693 #define REG_CORE_CALIBRATION_PARAMETER5_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER5 */ 00694 #define REG_CORE_CALIBRATION_PARAMETER6_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER6 */ 00695 #define REG_CORE_CALIBRATION_PARAMETER7_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER7 */ 00696 #define REG_CORE_CALIBRATION_PARAMETER8_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER8 */ 00697 #define REG_CORE_CALIBRATION_PARAMETER9_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER9 */ 00698 #define REG_CORE_CALIBRATION_PARAMETER10_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER10 */ 00699 #define REG_CORE_CALIBRATION_PARAMETER11_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER11 */ 00700 #define REG_CORE_CALIBRATION_PARAMETER12_RESET 0x00000000 /* Reset Value for REG_CORE_CALIBRATION_PARAMETER12 */ 00701 #define REG_CORE_CALIBRATION_PARAMETER0 0x000000B8 /* CORE Calibration Parameter Value */ 00702 #define REG_CORE_CALIBRATION_PARAMETER1 0x000000F8 /* CORE Calibration Parameter Value */ 00703 #define REG_CORE_CALIBRATION_PARAMETER2 0x00000138 /* CORE Calibration Parameter Value */ 00704 #define REG_CORE_CALIBRATION_PARAMETER3 0x00000178 /* CORE Calibration Parameter Value */ 00705 #define REG_CORE_CALIBRATION_PARAMETER4 0x000001B8 /* CORE Calibration Parameter Value */ 00706 #define REG_CORE_CALIBRATION_PARAMETER5 0x000001F8 /* CORE Calibration Parameter Value */ 00707 #define REG_CORE_CALIBRATION_PARAMETER6 0x00000238 /* CORE Calibration Parameter Value */ 00708 #define REG_CORE_CALIBRATION_PARAMETER7 0x00000278 /* CORE Calibration Parameter Value */ 00709 #define REG_CORE_CALIBRATION_PARAMETER8 0x000002B8 /* CORE Calibration Parameter Value */ 00710 #define REG_CORE_CALIBRATION_PARAMETER9 0x000002F8 /* CORE Calibration Parameter Value */ 00711 #define REG_CORE_CALIBRATION_PARAMETER10 0x00000338 /* CORE Calibration Parameter Value */ 00712 #define REG_CORE_CALIBRATION_PARAMETER11 0x00000378 /* CORE Calibration Parameter Value */ 00713 #define REG_CORE_CALIBRATION_PARAMETER12 0x000003B8 /* CORE Calibration Parameter Value */ 00714 #define REG_CORE_CALIBRATION_PARAMETERn(i) (REG_CORE_CALIBRATION_PARAMETER0 + ((i) * 64)) 00715 #define REG_CORE_CALIBRATION_PARAMETERn_COUNT 13 00716 #define REG_CORE_DIGITAL_SENSOR_CONFIGn_RESET 0x00000000 /* Reset Value for Digital_Sensor_Config[n] */ 00717 #define REG_CORE_DIGITAL_SENSOR_CONFIG0_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG0 */ 00718 #define REG_CORE_DIGITAL_SENSOR_CONFIG1_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG1 */ 00719 #define REG_CORE_DIGITAL_SENSOR_CONFIG2_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG2 */ 00720 #define REG_CORE_DIGITAL_SENSOR_CONFIG3_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG3 */ 00721 #define REG_CORE_DIGITAL_SENSOR_CONFIG4_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG4 */ 00722 #define REG_CORE_DIGITAL_SENSOR_CONFIG5_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG5 */ 00723 #define REG_CORE_DIGITAL_SENSOR_CONFIG6_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG6 */ 00724 #define REG_CORE_DIGITAL_SENSOR_CONFIG7_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG7 */ 00725 #define REG_CORE_DIGITAL_SENSOR_CONFIG8_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG8 */ 00726 #define REG_CORE_DIGITAL_SENSOR_CONFIG9_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG9 */ 00727 #define REG_CORE_DIGITAL_SENSOR_CONFIG10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG10 */ 00728 #define REG_CORE_DIGITAL_SENSOR_CONFIG11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG11 */ 00729 #define REG_CORE_DIGITAL_SENSOR_CONFIG12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG12 */ 00730 #define REG_CORE_DIGITAL_SENSOR_CONFIG0 0x000000BC /* CORE Digital Sensor Data Coding */ 00731 #define REG_CORE_DIGITAL_SENSOR_CONFIG1 0x000000FC /* CORE Digital Sensor Data Coding */ 00732 #define REG_CORE_DIGITAL_SENSOR_CONFIG2 0x0000013C /* CORE Digital Sensor Data Coding */ 00733 #define REG_CORE_DIGITAL_SENSOR_CONFIG3 0x0000017C /* CORE Digital Sensor Data Coding */ 00734 #define REG_CORE_DIGITAL_SENSOR_CONFIG4 0x000001BC /* CORE Digital Sensor Data Coding */ 00735 #define REG_CORE_DIGITAL_SENSOR_CONFIG5 0x000001FC /* CORE Digital Sensor Data Coding */ 00736 #define REG_CORE_DIGITAL_SENSOR_CONFIG6 0x0000023C /* CORE Digital Sensor Data Coding */ 00737 #define REG_CORE_DIGITAL_SENSOR_CONFIG7 0x0000027C /* CORE Digital Sensor Data Coding */ 00738 #define REG_CORE_DIGITAL_SENSOR_CONFIG8 0x000002BC /* CORE Digital Sensor Data Coding */ 00739 #define REG_CORE_DIGITAL_SENSOR_CONFIG9 0x000002FC /* CORE Digital Sensor Data Coding */ 00740 #define REG_CORE_DIGITAL_SENSOR_CONFIG10 0x0000033C /* CORE Digital Sensor Data Coding */ 00741 #define REG_CORE_DIGITAL_SENSOR_CONFIG11 0x0000037C /* CORE Digital Sensor Data Coding */ 00742 #define REG_CORE_DIGITAL_SENSOR_CONFIG12 0x000003BC /* CORE Digital Sensor Data Coding */ 00743 #define REG_CORE_DIGITAL_SENSOR_CONFIGn(i) (REG_CORE_DIGITAL_SENSOR_CONFIG0 + ((i) * 64)) 00744 #define REG_CORE_DIGITAL_SENSOR_CONFIGn_COUNT 13 00745 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn_RESET 0x00000000 /* Reset Value for Digital_Sensor_Address[n] */ 00746 #define REG_CORE_DIGITAL_SENSOR_ADDRESS0_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS0 */ 00747 #define REG_CORE_DIGITAL_SENSOR_ADDRESS1_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS1 */ 00748 #define REG_CORE_DIGITAL_SENSOR_ADDRESS2_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS2 */ 00749 #define REG_CORE_DIGITAL_SENSOR_ADDRESS3_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS3 */ 00750 #define REG_CORE_DIGITAL_SENSOR_ADDRESS4_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS4 */ 00751 #define REG_CORE_DIGITAL_SENSOR_ADDRESS5_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS5 */ 00752 #define REG_CORE_DIGITAL_SENSOR_ADDRESS6_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS6 */ 00753 #define REG_CORE_DIGITAL_SENSOR_ADDRESS7_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS7 */ 00754 #define REG_CORE_DIGITAL_SENSOR_ADDRESS8_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS8 */ 00755 #define REG_CORE_DIGITAL_SENSOR_ADDRESS9_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS9 */ 00756 #define REG_CORE_DIGITAL_SENSOR_ADDRESS10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS10 */ 00757 #define REG_CORE_DIGITAL_SENSOR_ADDRESS11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS11 */ 00758 #define REG_CORE_DIGITAL_SENSOR_ADDRESS12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS12 */ 00759 #define REG_CORE_DIGITAL_SENSOR_ADDRESS0 0x000000BE /* CORE Sensor Address */ 00760 #define REG_CORE_DIGITAL_SENSOR_ADDRESS1 0x000000FE /* CORE Sensor Address */ 00761 #define REG_CORE_DIGITAL_SENSOR_ADDRESS2 0x0000013E /* CORE Sensor Address */ 00762 #define REG_CORE_DIGITAL_SENSOR_ADDRESS3 0x0000017E /* CORE Sensor Address */ 00763 #define REG_CORE_DIGITAL_SENSOR_ADDRESS4 0x000001BE /* CORE Sensor Address */ 00764 #define REG_CORE_DIGITAL_SENSOR_ADDRESS5 0x000001FE /* CORE Sensor Address */ 00765 #define REG_CORE_DIGITAL_SENSOR_ADDRESS6 0x0000023E /* CORE Sensor Address */ 00766 #define REG_CORE_DIGITAL_SENSOR_ADDRESS7 0x0000027E /* CORE Sensor Address */ 00767 #define REG_CORE_DIGITAL_SENSOR_ADDRESS8 0x000002BE /* CORE Sensor Address */ 00768 #define REG_CORE_DIGITAL_SENSOR_ADDRESS9 0x000002FE /* CORE Sensor Address */ 00769 #define REG_CORE_DIGITAL_SENSOR_ADDRESS10 0x0000033E /* CORE Sensor Address */ 00770 #define REG_CORE_DIGITAL_SENSOR_ADDRESS11 0x0000037E /* CORE Sensor Address */ 00771 #define REG_CORE_DIGITAL_SENSOR_ADDRESS12 0x000003BE /* CORE Sensor Address */ 00772 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn(i) (REG_CORE_DIGITAL_SENSOR_ADDRESS0 + ((i) * 64)) 00773 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn_COUNT 13 00774 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDSn_RESET 0x00000000 /* Reset Value for Digital_Sensor_Num_Cmds[n] */ 00775 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS0_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS0 */ 00776 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS1_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS1 */ 00777 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS2_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS2 */ 00778 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS3_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS3 */ 00779 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS4_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS4 */ 00780 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS5_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS5 */ 00781 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS6_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS6 */ 00782 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS7_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS7 */ 00783 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS8_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS8 */ 00784 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS9_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS9 */ 00785 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS10 */ 00786 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS11 */ 00787 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_NUM_CMDS12 */ 00788 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS0 0x000000BF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00789 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS1 0x000000FF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00790 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS2 0x0000013F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00791 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS3 0x0000017F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00792 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS4 0x000001BF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00793 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS5 0x000001FF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00794 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS6 0x0000023F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00795 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS7 0x0000027F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00796 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS8 0x000002BF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00797 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS9 0x000002FF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00798 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS10 0x0000033F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00799 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS11 0x0000037F /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00800 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDS12 0x000003BF /* CORE Number of Configuration, Read Commands for Digital Sensors */ 00801 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDSn(i) (REG_CORE_DIGITAL_SENSOR_NUM_CMDS0 + ((i) * 64)) 00802 #define REG_CORE_DIGITAL_SENSOR_NUM_CMDSn_COUNT 13 00803 #define REG_CORE_DIGITAL_SENSOR_COMMSn_RESET 0x00000006 /* Reset Value for Digital_Sensor_Comms[n] */ 00804 #define REG_CORE_DIGITAL_SENSOR_COMMS0_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS0 */ 00805 #define REG_CORE_DIGITAL_SENSOR_COMMS1_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS1 */ 00806 #define REG_CORE_DIGITAL_SENSOR_COMMS2_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS2 */ 00807 #define REG_CORE_DIGITAL_SENSOR_COMMS3_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS3 */ 00808 #define REG_CORE_DIGITAL_SENSOR_COMMS4_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS4 */ 00809 #define REG_CORE_DIGITAL_SENSOR_COMMS5_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS5 */ 00810 #define REG_CORE_DIGITAL_SENSOR_COMMS6_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS6 */ 00811 #define REG_CORE_DIGITAL_SENSOR_COMMS7_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS7 */ 00812 #define REG_CORE_DIGITAL_SENSOR_COMMS8_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS8 */ 00813 #define REG_CORE_DIGITAL_SENSOR_COMMS9_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS9 */ 00814 #define REG_CORE_DIGITAL_SENSOR_COMMS10_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS10 */ 00815 #define REG_CORE_DIGITAL_SENSOR_COMMS11_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS11 */ 00816 #define REG_CORE_DIGITAL_SENSOR_COMMS12_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS12 */ 00817 #define REG_CORE_DIGITAL_SENSOR_COMMS0 0x000000C0 /* CORE Digital Sensor Communication Clock Configuration */ 00818 #define REG_CORE_DIGITAL_SENSOR_COMMS1 0x00000100 /* CORE Digital Sensor Communication Clock Configuration */ 00819 #define REG_CORE_DIGITAL_SENSOR_COMMS2 0x00000140 /* CORE Digital Sensor Communication Clock Configuration */ 00820 #define REG_CORE_DIGITAL_SENSOR_COMMS3 0x00000180 /* CORE Digital Sensor Communication Clock Configuration */ 00821 #define REG_CORE_DIGITAL_SENSOR_COMMS4 0x000001C0 /* CORE Digital Sensor Communication Clock Configuration */ 00822 #define REG_CORE_DIGITAL_SENSOR_COMMS5 0x00000200 /* CORE Digital Sensor Communication Clock Configuration */ 00823 #define REG_CORE_DIGITAL_SENSOR_COMMS6 0x00000240 /* CORE Digital Sensor Communication Clock Configuration */ 00824 #define REG_CORE_DIGITAL_SENSOR_COMMS7 0x00000280 /* CORE Digital Sensor Communication Clock Configuration */ 00825 #define REG_CORE_DIGITAL_SENSOR_COMMS8 0x000002C0 /* CORE Digital Sensor Communication Clock Configuration */ 00826 #define REG_CORE_DIGITAL_SENSOR_COMMS9 0x00000300 /* CORE Digital Sensor Communication Clock Configuration */ 00827 #define REG_CORE_DIGITAL_SENSOR_COMMS10 0x00000340 /* CORE Digital Sensor Communication Clock Configuration */ 00828 #define REG_CORE_DIGITAL_SENSOR_COMMS11 0x00000380 /* CORE Digital Sensor Communication Clock Configuration */ 00829 #define REG_CORE_DIGITAL_SENSOR_COMMS12 0x000003C0 /* CORE Digital Sensor Communication Clock Configuration */ 00830 #define REG_CORE_DIGITAL_SENSOR_COMMSn(i) (REG_CORE_DIGITAL_SENSOR_COMMS0 + ((i) * 64)) 00831 #define REG_CORE_DIGITAL_SENSOR_COMMSn_COUNT 13 00832 #define REG_CORE_DIGITAL_SENSOR_COMMAND1n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command1[n] */ 00833 #define REG_CORE_DIGITAL_SENSOR_COMMAND10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND10 */ 00834 #define REG_CORE_DIGITAL_SENSOR_COMMAND11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND11 */ 00835 #define REG_CORE_DIGITAL_SENSOR_COMMAND12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND12 */ 00836 #define REG_CORE_DIGITAL_SENSOR_COMMAND13_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND13 */ 00837 #define REG_CORE_DIGITAL_SENSOR_COMMAND14_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND14 */ 00838 #define REG_CORE_DIGITAL_SENSOR_COMMAND15_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND15 */ 00839 #define REG_CORE_DIGITAL_SENSOR_COMMAND16_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND16 */ 00840 #define REG_CORE_DIGITAL_SENSOR_COMMAND17_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND17 */ 00841 #define REG_CORE_DIGITAL_SENSOR_COMMAND18_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND18 */ 00842 #define REG_CORE_DIGITAL_SENSOR_COMMAND19_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND19 */ 00843 #define REG_CORE_DIGITAL_SENSOR_COMMAND110_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND110 */ 00844 #define REG_CORE_DIGITAL_SENSOR_COMMAND111_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND111 */ 00845 #define REG_CORE_DIGITAL_SENSOR_COMMAND112_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND112 */ 00846 #define REG_CORE_DIGITAL_SENSOR_COMMAND10 0x000000C2 /* CORE Sensor Configuration Command1 */ 00847 #define REG_CORE_DIGITAL_SENSOR_COMMAND11 0x00000102 /* CORE Sensor Configuration Command1 */ 00848 #define REG_CORE_DIGITAL_SENSOR_COMMAND12 0x00000142 /* CORE Sensor Configuration Command1 */ 00849 #define REG_CORE_DIGITAL_SENSOR_COMMAND13 0x00000182 /* CORE Sensor Configuration Command1 */ 00850 #define REG_CORE_DIGITAL_SENSOR_COMMAND14 0x000001C2 /* CORE Sensor Configuration Command1 */ 00851 #define REG_CORE_DIGITAL_SENSOR_COMMAND15 0x00000202 /* CORE Sensor Configuration Command1 */ 00852 #define REG_CORE_DIGITAL_SENSOR_COMMAND16 0x00000242 /* CORE Sensor Configuration Command1 */ 00853 #define REG_CORE_DIGITAL_SENSOR_COMMAND17 0x00000282 /* CORE Sensor Configuration Command1 */ 00854 #define REG_CORE_DIGITAL_SENSOR_COMMAND18 0x000002C2 /* CORE Sensor Configuration Command1 */ 00855 #define REG_CORE_DIGITAL_SENSOR_COMMAND19 0x00000302 /* CORE Sensor Configuration Command1 */ 00856 #define REG_CORE_DIGITAL_SENSOR_COMMAND110 0x00000342 /* CORE Sensor Configuration Command1 */ 00857 #define REG_CORE_DIGITAL_SENSOR_COMMAND111 0x00000382 /* CORE Sensor Configuration Command1 */ 00858 #define REG_CORE_DIGITAL_SENSOR_COMMAND112 0x000003C2 /* CORE Sensor Configuration Command1 */ 00859 #define REG_CORE_DIGITAL_SENSOR_COMMAND1n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND10 + ((i) * 64)) 00860 #define REG_CORE_DIGITAL_SENSOR_COMMAND1n_COUNT 13 00861 #define REG_CORE_DIGITAL_SENSOR_COMMAND2n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command2[n] */ 00862 #define REG_CORE_DIGITAL_SENSOR_COMMAND20_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND20 */ 00863 #define REG_CORE_DIGITAL_SENSOR_COMMAND21_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND21 */ 00864 #define REG_CORE_DIGITAL_SENSOR_COMMAND22_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND22 */ 00865 #define REG_CORE_DIGITAL_SENSOR_COMMAND23_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND23 */ 00866 #define REG_CORE_DIGITAL_SENSOR_COMMAND24_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND24 */ 00867 #define REG_CORE_DIGITAL_SENSOR_COMMAND25_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND25 */ 00868 #define REG_CORE_DIGITAL_SENSOR_COMMAND26_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND26 */ 00869 #define REG_CORE_DIGITAL_SENSOR_COMMAND27_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND27 */ 00870 #define REG_CORE_DIGITAL_SENSOR_COMMAND28_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND28 */ 00871 #define REG_CORE_DIGITAL_SENSOR_COMMAND29_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND29 */ 00872 #define REG_CORE_DIGITAL_SENSOR_COMMAND210_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND210 */ 00873 #define REG_CORE_DIGITAL_SENSOR_COMMAND211_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND211 */ 00874 #define REG_CORE_DIGITAL_SENSOR_COMMAND212_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND212 */ 00875 #define REG_CORE_DIGITAL_SENSOR_COMMAND20 0x000000C3 /* CORE Sensor Configuration Command2 */ 00876 #define REG_CORE_DIGITAL_SENSOR_COMMAND21 0x00000103 /* CORE Sensor Configuration Command2 */ 00877 #define REG_CORE_DIGITAL_SENSOR_COMMAND22 0x00000143 /* CORE Sensor Configuration Command2 */ 00878 #define REG_CORE_DIGITAL_SENSOR_COMMAND23 0x00000183 /* CORE Sensor Configuration Command2 */ 00879 #define REG_CORE_DIGITAL_SENSOR_COMMAND24 0x000001C3 /* CORE Sensor Configuration Command2 */ 00880 #define REG_CORE_DIGITAL_SENSOR_COMMAND25 0x00000203 /* CORE Sensor Configuration Command2 */ 00881 #define REG_CORE_DIGITAL_SENSOR_COMMAND26 0x00000243 /* CORE Sensor Configuration Command2 */ 00882 #define REG_CORE_DIGITAL_SENSOR_COMMAND27 0x00000283 /* CORE Sensor Configuration Command2 */ 00883 #define REG_CORE_DIGITAL_SENSOR_COMMAND28 0x000002C3 /* CORE Sensor Configuration Command2 */ 00884 #define REG_CORE_DIGITAL_SENSOR_COMMAND29 0x00000303 /* CORE Sensor Configuration Command2 */ 00885 #define REG_CORE_DIGITAL_SENSOR_COMMAND210 0x00000343 /* CORE Sensor Configuration Command2 */ 00886 #define REG_CORE_DIGITAL_SENSOR_COMMAND211 0x00000383 /* CORE Sensor Configuration Command2 */ 00887 #define REG_CORE_DIGITAL_SENSOR_COMMAND212 0x000003C3 /* CORE Sensor Configuration Command2 */ 00888 #define REG_CORE_DIGITAL_SENSOR_COMMAND2n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND20 + ((i) * 64)) 00889 #define REG_CORE_DIGITAL_SENSOR_COMMAND2n_COUNT 13 00890 #define REG_CORE_DIGITAL_SENSOR_COMMAND3n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command3[n] */ 00891 #define REG_CORE_DIGITAL_SENSOR_COMMAND30_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND30 */ 00892 #define REG_CORE_DIGITAL_SENSOR_COMMAND31_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND31 */ 00893 #define REG_CORE_DIGITAL_SENSOR_COMMAND32_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND32 */ 00894 #define REG_CORE_DIGITAL_SENSOR_COMMAND33_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND33 */ 00895 #define REG_CORE_DIGITAL_SENSOR_COMMAND34_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND34 */ 00896 #define REG_CORE_DIGITAL_SENSOR_COMMAND35_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND35 */ 00897 #define REG_CORE_DIGITAL_SENSOR_COMMAND36_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND36 */ 00898 #define REG_CORE_DIGITAL_SENSOR_COMMAND37_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND37 */ 00899 #define REG_CORE_DIGITAL_SENSOR_COMMAND38_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND38 */ 00900 #define REG_CORE_DIGITAL_SENSOR_COMMAND39_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND39 */ 00901 #define REG_CORE_DIGITAL_SENSOR_COMMAND310_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND310 */ 00902 #define REG_CORE_DIGITAL_SENSOR_COMMAND311_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND311 */ 00903 #define REG_CORE_DIGITAL_SENSOR_COMMAND312_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND312 */ 00904 #define REG_CORE_DIGITAL_SENSOR_COMMAND30 0x000000C4 /* CORE Sensor Configuration Command3 */ 00905 #define REG_CORE_DIGITAL_SENSOR_COMMAND31 0x00000104 /* CORE Sensor Configuration Command3 */ 00906 #define REG_CORE_DIGITAL_SENSOR_COMMAND32 0x00000144 /* CORE Sensor Configuration Command3 */ 00907 #define REG_CORE_DIGITAL_SENSOR_COMMAND33 0x00000184 /* CORE Sensor Configuration Command3 */ 00908 #define REG_CORE_DIGITAL_SENSOR_COMMAND34 0x000001C4 /* CORE Sensor Configuration Command3 */ 00909 #define REG_CORE_DIGITAL_SENSOR_COMMAND35 0x00000204 /* CORE Sensor Configuration Command3 */ 00910 #define REG_CORE_DIGITAL_SENSOR_COMMAND36 0x00000244 /* CORE Sensor Configuration Command3 */ 00911 #define REG_CORE_DIGITAL_SENSOR_COMMAND37 0x00000284 /* CORE Sensor Configuration Command3 */ 00912 #define REG_CORE_DIGITAL_SENSOR_COMMAND38 0x000002C4 /* CORE Sensor Configuration Command3 */ 00913 #define REG_CORE_DIGITAL_SENSOR_COMMAND39 0x00000304 /* CORE Sensor Configuration Command3 */ 00914 #define REG_CORE_DIGITAL_SENSOR_COMMAND310 0x00000344 /* CORE Sensor Configuration Command3 */ 00915 #define REG_CORE_DIGITAL_SENSOR_COMMAND311 0x00000384 /* CORE Sensor Configuration Command3 */ 00916 #define REG_CORE_DIGITAL_SENSOR_COMMAND312 0x000003C4 /* CORE Sensor Configuration Command3 */ 00917 #define REG_CORE_DIGITAL_SENSOR_COMMAND3n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND30 + ((i) * 64)) 00918 #define REG_CORE_DIGITAL_SENSOR_COMMAND3n_COUNT 13 00919 #define REG_CORE_DIGITAL_SENSOR_COMMAND4n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command4[n] */ 00920 #define REG_CORE_DIGITAL_SENSOR_COMMAND40_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND40 */ 00921 #define REG_CORE_DIGITAL_SENSOR_COMMAND41_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND41 */ 00922 #define REG_CORE_DIGITAL_SENSOR_COMMAND42_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND42 */ 00923 #define REG_CORE_DIGITAL_SENSOR_COMMAND43_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND43 */ 00924 #define REG_CORE_DIGITAL_SENSOR_COMMAND44_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND44 */ 00925 #define REG_CORE_DIGITAL_SENSOR_COMMAND45_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND45 */ 00926 #define REG_CORE_DIGITAL_SENSOR_COMMAND46_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND46 */ 00927 #define REG_CORE_DIGITAL_SENSOR_COMMAND47_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND47 */ 00928 #define REG_CORE_DIGITAL_SENSOR_COMMAND48_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND48 */ 00929 #define REG_CORE_DIGITAL_SENSOR_COMMAND49_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND49 */ 00930 #define REG_CORE_DIGITAL_SENSOR_COMMAND410_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND410 */ 00931 #define REG_CORE_DIGITAL_SENSOR_COMMAND411_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND411 */ 00932 #define REG_CORE_DIGITAL_SENSOR_COMMAND412_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND412 */ 00933 #define REG_CORE_DIGITAL_SENSOR_COMMAND40 0x000000C5 /* CORE Sensor Configuration Command4 */ 00934 #define REG_CORE_DIGITAL_SENSOR_COMMAND41 0x00000105 /* CORE Sensor Configuration Command4 */ 00935 #define REG_CORE_DIGITAL_SENSOR_COMMAND42 0x00000145 /* CORE Sensor Configuration Command4 */ 00936 #define REG_CORE_DIGITAL_SENSOR_COMMAND43 0x00000185 /* CORE Sensor Configuration Command4 */ 00937 #define REG_CORE_DIGITAL_SENSOR_COMMAND44 0x000001C5 /* CORE Sensor Configuration Command4 */ 00938 #define REG_CORE_DIGITAL_SENSOR_COMMAND45 0x00000205 /* CORE Sensor Configuration Command4 */ 00939 #define REG_CORE_DIGITAL_SENSOR_COMMAND46 0x00000245 /* CORE Sensor Configuration Command4 */ 00940 #define REG_CORE_DIGITAL_SENSOR_COMMAND47 0x00000285 /* CORE Sensor Configuration Command4 */ 00941 #define REG_CORE_DIGITAL_SENSOR_COMMAND48 0x000002C5 /* CORE Sensor Configuration Command4 */ 00942 #define REG_CORE_DIGITAL_SENSOR_COMMAND49 0x00000305 /* CORE Sensor Configuration Command4 */ 00943 #define REG_CORE_DIGITAL_SENSOR_COMMAND410 0x00000345 /* CORE Sensor Configuration Command4 */ 00944 #define REG_CORE_DIGITAL_SENSOR_COMMAND411 0x00000385 /* CORE Sensor Configuration Command4 */ 00945 #define REG_CORE_DIGITAL_SENSOR_COMMAND412 0x000003C5 /* CORE Sensor Configuration Command4 */ 00946 #define REG_CORE_DIGITAL_SENSOR_COMMAND4n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND40 + ((i) * 64)) 00947 #define REG_CORE_DIGITAL_SENSOR_COMMAND4n_COUNT 13 00948 #define REG_CORE_DIGITAL_SENSOR_COMMAND5n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command5[n] */ 00949 #define REG_CORE_DIGITAL_SENSOR_COMMAND50_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND50 */ 00950 #define REG_CORE_DIGITAL_SENSOR_COMMAND51_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND51 */ 00951 #define REG_CORE_DIGITAL_SENSOR_COMMAND52_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND52 */ 00952 #define REG_CORE_DIGITAL_SENSOR_COMMAND53_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND53 */ 00953 #define REG_CORE_DIGITAL_SENSOR_COMMAND54_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND54 */ 00954 #define REG_CORE_DIGITAL_SENSOR_COMMAND55_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND55 */ 00955 #define REG_CORE_DIGITAL_SENSOR_COMMAND56_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND56 */ 00956 #define REG_CORE_DIGITAL_SENSOR_COMMAND57_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND57 */ 00957 #define REG_CORE_DIGITAL_SENSOR_COMMAND58_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND58 */ 00958 #define REG_CORE_DIGITAL_SENSOR_COMMAND59_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND59 */ 00959 #define REG_CORE_DIGITAL_SENSOR_COMMAND510_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND510 */ 00960 #define REG_CORE_DIGITAL_SENSOR_COMMAND511_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND511 */ 00961 #define REG_CORE_DIGITAL_SENSOR_COMMAND512_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND512 */ 00962 #define REG_CORE_DIGITAL_SENSOR_COMMAND50 0x000000C6 /* CORE Sensor Configuration Command5 */ 00963 #define REG_CORE_DIGITAL_SENSOR_COMMAND51 0x00000106 /* CORE Sensor Configuration Command5 */ 00964 #define REG_CORE_DIGITAL_SENSOR_COMMAND52 0x00000146 /* CORE Sensor Configuration Command5 */ 00965 #define REG_CORE_DIGITAL_SENSOR_COMMAND53 0x00000186 /* CORE Sensor Configuration Command5 */ 00966 #define REG_CORE_DIGITAL_SENSOR_COMMAND54 0x000001C6 /* CORE Sensor Configuration Command5 */ 00967 #define REG_CORE_DIGITAL_SENSOR_COMMAND55 0x00000206 /* CORE Sensor Configuration Command5 */ 00968 #define REG_CORE_DIGITAL_SENSOR_COMMAND56 0x00000246 /* CORE Sensor Configuration Command5 */ 00969 #define REG_CORE_DIGITAL_SENSOR_COMMAND57 0x00000286 /* CORE Sensor Configuration Command5 */ 00970 #define REG_CORE_DIGITAL_SENSOR_COMMAND58 0x000002C6 /* CORE Sensor Configuration Command5 */ 00971 #define REG_CORE_DIGITAL_SENSOR_COMMAND59 0x00000306 /* CORE Sensor Configuration Command5 */ 00972 #define REG_CORE_DIGITAL_SENSOR_COMMAND510 0x00000346 /* CORE Sensor Configuration Command5 */ 00973 #define REG_CORE_DIGITAL_SENSOR_COMMAND511 0x00000386 /* CORE Sensor Configuration Command5 */ 00974 #define REG_CORE_DIGITAL_SENSOR_COMMAND512 0x000003C6 /* CORE Sensor Configuration Command5 */ 00975 #define REG_CORE_DIGITAL_SENSOR_COMMAND5n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND50 + ((i) * 64)) 00976 #define REG_CORE_DIGITAL_SENSOR_COMMAND5n_COUNT 13 00977 #define REG_CORE_DIGITAL_SENSOR_COMMAND6n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command6[n] */ 00978 #define REG_CORE_DIGITAL_SENSOR_COMMAND60_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND60 */ 00979 #define REG_CORE_DIGITAL_SENSOR_COMMAND61_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND61 */ 00980 #define REG_CORE_DIGITAL_SENSOR_COMMAND62_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND62 */ 00981 #define REG_CORE_DIGITAL_SENSOR_COMMAND63_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND63 */ 00982 #define REG_CORE_DIGITAL_SENSOR_COMMAND64_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND64 */ 00983 #define REG_CORE_DIGITAL_SENSOR_COMMAND65_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND65 */ 00984 #define REG_CORE_DIGITAL_SENSOR_COMMAND66_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND66 */ 00985 #define REG_CORE_DIGITAL_SENSOR_COMMAND67_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND67 */ 00986 #define REG_CORE_DIGITAL_SENSOR_COMMAND68_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND68 */ 00987 #define REG_CORE_DIGITAL_SENSOR_COMMAND69_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND69 */ 00988 #define REG_CORE_DIGITAL_SENSOR_COMMAND610_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND610 */ 00989 #define REG_CORE_DIGITAL_SENSOR_COMMAND611_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND611 */ 00990 #define REG_CORE_DIGITAL_SENSOR_COMMAND612_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND612 */ 00991 #define REG_CORE_DIGITAL_SENSOR_COMMAND60 0x000000C7 /* CORE Sensor Configuration Command6 */ 00992 #define REG_CORE_DIGITAL_SENSOR_COMMAND61 0x00000107 /* CORE Sensor Configuration Command6 */ 00993 #define REG_CORE_DIGITAL_SENSOR_COMMAND62 0x00000147 /* CORE Sensor Configuration Command6 */ 00994 #define REG_CORE_DIGITAL_SENSOR_COMMAND63 0x00000187 /* CORE Sensor Configuration Command6 */ 00995 #define REG_CORE_DIGITAL_SENSOR_COMMAND64 0x000001C7 /* CORE Sensor Configuration Command6 */ 00996 #define REG_CORE_DIGITAL_SENSOR_COMMAND65 0x00000207 /* CORE Sensor Configuration Command6 */ 00997 #define REG_CORE_DIGITAL_SENSOR_COMMAND66 0x00000247 /* CORE Sensor Configuration Command6 */ 00998 #define REG_CORE_DIGITAL_SENSOR_COMMAND67 0x00000287 /* CORE Sensor Configuration Command6 */ 00999 #define REG_CORE_DIGITAL_SENSOR_COMMAND68 0x000002C7 /* CORE Sensor Configuration Command6 */ 01000 #define REG_CORE_DIGITAL_SENSOR_COMMAND69 0x00000307 /* CORE Sensor Configuration Command6 */ 01001 #define REG_CORE_DIGITAL_SENSOR_COMMAND610 0x00000347 /* CORE Sensor Configuration Command6 */ 01002 #define REG_CORE_DIGITAL_SENSOR_COMMAND611 0x00000387 /* CORE Sensor Configuration Command6 */ 01003 #define REG_CORE_DIGITAL_SENSOR_COMMAND612 0x000003C7 /* CORE Sensor Configuration Command6 */ 01004 #define REG_CORE_DIGITAL_SENSOR_COMMAND6n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND60 + ((i) * 64)) 01005 #define REG_CORE_DIGITAL_SENSOR_COMMAND6n_COUNT 13 01006 #define REG_CORE_DIGITAL_SENSOR_COMMAND7n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Command7[n] */ 01007 #define REG_CORE_DIGITAL_SENSOR_COMMAND70_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND70 */ 01008 #define REG_CORE_DIGITAL_SENSOR_COMMAND71_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND71 */ 01009 #define REG_CORE_DIGITAL_SENSOR_COMMAND72_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND72 */ 01010 #define REG_CORE_DIGITAL_SENSOR_COMMAND73_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND73 */ 01011 #define REG_CORE_DIGITAL_SENSOR_COMMAND74_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND74 */ 01012 #define REG_CORE_DIGITAL_SENSOR_COMMAND75_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND75 */ 01013 #define REG_CORE_DIGITAL_SENSOR_COMMAND76_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND76 */ 01014 #define REG_CORE_DIGITAL_SENSOR_COMMAND77_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND77 */ 01015 #define REG_CORE_DIGITAL_SENSOR_COMMAND78_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND78 */ 01016 #define REG_CORE_DIGITAL_SENSOR_COMMAND79_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND79 */ 01017 #define REG_CORE_DIGITAL_SENSOR_COMMAND710_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND710 */ 01018 #define REG_CORE_DIGITAL_SENSOR_COMMAND711_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND711 */ 01019 #define REG_CORE_DIGITAL_SENSOR_COMMAND712_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMAND712 */ 01020 #define REG_CORE_DIGITAL_SENSOR_COMMAND70 0x000000C8 /* CORE Sensor Configuration Command7 */ 01021 #define REG_CORE_DIGITAL_SENSOR_COMMAND71 0x00000108 /* CORE Sensor Configuration Command7 */ 01022 #define REG_CORE_DIGITAL_SENSOR_COMMAND72 0x00000148 /* CORE Sensor Configuration Command7 */ 01023 #define REG_CORE_DIGITAL_SENSOR_COMMAND73 0x00000188 /* CORE Sensor Configuration Command7 */ 01024 #define REG_CORE_DIGITAL_SENSOR_COMMAND74 0x000001C8 /* CORE Sensor Configuration Command7 */ 01025 #define REG_CORE_DIGITAL_SENSOR_COMMAND75 0x00000208 /* CORE Sensor Configuration Command7 */ 01026 #define REG_CORE_DIGITAL_SENSOR_COMMAND76 0x00000248 /* CORE Sensor Configuration Command7 */ 01027 #define REG_CORE_DIGITAL_SENSOR_COMMAND77 0x00000288 /* CORE Sensor Configuration Command7 */ 01028 #define REG_CORE_DIGITAL_SENSOR_COMMAND78 0x000002C8 /* CORE Sensor Configuration Command7 */ 01029 #define REG_CORE_DIGITAL_SENSOR_COMMAND79 0x00000308 /* CORE Sensor Configuration Command7 */ 01030 #define REG_CORE_DIGITAL_SENSOR_COMMAND710 0x00000348 /* CORE Sensor Configuration Command7 */ 01031 #define REG_CORE_DIGITAL_SENSOR_COMMAND711 0x00000388 /* CORE Sensor Configuration Command7 */ 01032 #define REG_CORE_DIGITAL_SENSOR_COMMAND712 0x000003C8 /* CORE Sensor Configuration Command7 */ 01033 #define REG_CORE_DIGITAL_SENSOR_COMMAND7n(i) (REG_CORE_DIGITAL_SENSOR_COMMAND70 + ((i) * 64)) 01034 #define REG_CORE_DIGITAL_SENSOR_COMMAND7n_COUNT 13 01035 #define REG_CORE_DIGITAL_SENSOR_READ_CMD1n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd1[n] */ 01036 #define REG_CORE_DIGITAL_SENSOR_READ_CMD10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD10 */ 01037 #define REG_CORE_DIGITAL_SENSOR_READ_CMD11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD11 */ 01038 #define REG_CORE_DIGITAL_SENSOR_READ_CMD12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD12 */ 01039 #define REG_CORE_DIGITAL_SENSOR_READ_CMD13_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD13 */ 01040 #define REG_CORE_DIGITAL_SENSOR_READ_CMD14_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD14 */ 01041 #define REG_CORE_DIGITAL_SENSOR_READ_CMD15_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD15 */ 01042 #define REG_CORE_DIGITAL_SENSOR_READ_CMD16_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD16 */ 01043 #define REG_CORE_DIGITAL_SENSOR_READ_CMD17_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD17 */ 01044 #define REG_CORE_DIGITAL_SENSOR_READ_CMD18_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD18 */ 01045 #define REG_CORE_DIGITAL_SENSOR_READ_CMD19_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD19 */ 01046 #define REG_CORE_DIGITAL_SENSOR_READ_CMD110_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD110 */ 01047 #define REG_CORE_DIGITAL_SENSOR_READ_CMD111_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD111 */ 01048 #define REG_CORE_DIGITAL_SENSOR_READ_CMD112_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD112 */ 01049 #define REG_CORE_DIGITAL_SENSOR_READ_CMD10 0x000000C9 /* CORE Sensor Read Command1 */ 01050 #define REG_CORE_DIGITAL_SENSOR_READ_CMD11 0x00000109 /* CORE Sensor Read Command1 */ 01051 #define REG_CORE_DIGITAL_SENSOR_READ_CMD12 0x00000149 /* CORE Sensor Read Command1 */ 01052 #define REG_CORE_DIGITAL_SENSOR_READ_CMD13 0x00000189 /* CORE Sensor Read Command1 */ 01053 #define REG_CORE_DIGITAL_SENSOR_READ_CMD14 0x000001C9 /* CORE Sensor Read Command1 */ 01054 #define REG_CORE_DIGITAL_SENSOR_READ_CMD15 0x00000209 /* CORE Sensor Read Command1 */ 01055 #define REG_CORE_DIGITAL_SENSOR_READ_CMD16 0x00000249 /* CORE Sensor Read Command1 */ 01056 #define REG_CORE_DIGITAL_SENSOR_READ_CMD17 0x00000289 /* CORE Sensor Read Command1 */ 01057 #define REG_CORE_DIGITAL_SENSOR_READ_CMD18 0x000002C9 /* CORE Sensor Read Command1 */ 01058 #define REG_CORE_DIGITAL_SENSOR_READ_CMD19 0x00000309 /* CORE Sensor Read Command1 */ 01059 #define REG_CORE_DIGITAL_SENSOR_READ_CMD110 0x00000349 /* CORE Sensor Read Command1 */ 01060 #define REG_CORE_DIGITAL_SENSOR_READ_CMD111 0x00000389 /* CORE Sensor Read Command1 */ 01061 #define REG_CORE_DIGITAL_SENSOR_READ_CMD112 0x000003C9 /* CORE Sensor Read Command1 */ 01062 #define REG_CORE_DIGITAL_SENSOR_READ_CMD1n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD10 + ((i) * 64)) 01063 #define REG_CORE_DIGITAL_SENSOR_READ_CMD1n_COUNT 13 01064 #define REG_CORE_DIGITAL_SENSOR_READ_CMD2n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd2[n] */ 01065 #define REG_CORE_DIGITAL_SENSOR_READ_CMD20_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD20 */ 01066 #define REG_CORE_DIGITAL_SENSOR_READ_CMD21_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD21 */ 01067 #define REG_CORE_DIGITAL_SENSOR_READ_CMD22_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD22 */ 01068 #define REG_CORE_DIGITAL_SENSOR_READ_CMD23_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD23 */ 01069 #define REG_CORE_DIGITAL_SENSOR_READ_CMD24_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD24 */ 01070 #define REG_CORE_DIGITAL_SENSOR_READ_CMD25_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD25 */ 01071 #define REG_CORE_DIGITAL_SENSOR_READ_CMD26_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD26 */ 01072 #define REG_CORE_DIGITAL_SENSOR_READ_CMD27_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD27 */ 01073 #define REG_CORE_DIGITAL_SENSOR_READ_CMD28_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD28 */ 01074 #define REG_CORE_DIGITAL_SENSOR_READ_CMD29_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD29 */ 01075 #define REG_CORE_DIGITAL_SENSOR_READ_CMD210_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD210 */ 01076 #define REG_CORE_DIGITAL_SENSOR_READ_CMD211_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD211 */ 01077 #define REG_CORE_DIGITAL_SENSOR_READ_CMD212_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD212 */ 01078 #define REG_CORE_DIGITAL_SENSOR_READ_CMD20 0x000000CA /* CORE Sensor Read Command2 */ 01079 #define REG_CORE_DIGITAL_SENSOR_READ_CMD21 0x0000010A /* CORE Sensor Read Command2 */ 01080 #define REG_CORE_DIGITAL_SENSOR_READ_CMD22 0x0000014A /* CORE Sensor Read Command2 */ 01081 #define REG_CORE_DIGITAL_SENSOR_READ_CMD23 0x0000018A /* CORE Sensor Read Command2 */ 01082 #define REG_CORE_DIGITAL_SENSOR_READ_CMD24 0x000001CA /* CORE Sensor Read Command2 */ 01083 #define REG_CORE_DIGITAL_SENSOR_READ_CMD25 0x0000020A /* CORE Sensor Read Command2 */ 01084 #define REG_CORE_DIGITAL_SENSOR_READ_CMD26 0x0000024A /* CORE Sensor Read Command2 */ 01085 #define REG_CORE_DIGITAL_SENSOR_READ_CMD27 0x0000028A /* CORE Sensor Read Command2 */ 01086 #define REG_CORE_DIGITAL_SENSOR_READ_CMD28 0x000002CA /* CORE Sensor Read Command2 */ 01087 #define REG_CORE_DIGITAL_SENSOR_READ_CMD29 0x0000030A /* CORE Sensor Read Command2 */ 01088 #define REG_CORE_DIGITAL_SENSOR_READ_CMD210 0x0000034A /* CORE Sensor Read Command2 */ 01089 #define REG_CORE_DIGITAL_SENSOR_READ_CMD211 0x0000038A /* CORE Sensor Read Command2 */ 01090 #define REG_CORE_DIGITAL_SENSOR_READ_CMD212 0x000003CA /* CORE Sensor Read Command2 */ 01091 #define REG_CORE_DIGITAL_SENSOR_READ_CMD2n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD20 + ((i) * 64)) 01092 #define REG_CORE_DIGITAL_SENSOR_READ_CMD2n_COUNT 13 01093 #define REG_CORE_DIGITAL_SENSOR_READ_CMD3n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd3[n] */ 01094 #define REG_CORE_DIGITAL_SENSOR_READ_CMD30_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD30 */ 01095 #define REG_CORE_DIGITAL_SENSOR_READ_CMD31_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD31 */ 01096 #define REG_CORE_DIGITAL_SENSOR_READ_CMD32_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD32 */ 01097 #define REG_CORE_DIGITAL_SENSOR_READ_CMD33_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD33 */ 01098 #define REG_CORE_DIGITAL_SENSOR_READ_CMD34_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD34 */ 01099 #define REG_CORE_DIGITAL_SENSOR_READ_CMD35_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD35 */ 01100 #define REG_CORE_DIGITAL_SENSOR_READ_CMD36_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD36 */ 01101 #define REG_CORE_DIGITAL_SENSOR_READ_CMD37_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD37 */ 01102 #define REG_CORE_DIGITAL_SENSOR_READ_CMD38_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD38 */ 01103 #define REG_CORE_DIGITAL_SENSOR_READ_CMD39_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD39 */ 01104 #define REG_CORE_DIGITAL_SENSOR_READ_CMD310_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD310 */ 01105 #define REG_CORE_DIGITAL_SENSOR_READ_CMD311_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD311 */ 01106 #define REG_CORE_DIGITAL_SENSOR_READ_CMD312_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD312 */ 01107 #define REG_CORE_DIGITAL_SENSOR_READ_CMD30 0x000000CB /* CORE Sensor Read Command3 */ 01108 #define REG_CORE_DIGITAL_SENSOR_READ_CMD31 0x0000010B /* CORE Sensor Read Command3 */ 01109 #define REG_CORE_DIGITAL_SENSOR_READ_CMD32 0x0000014B /* CORE Sensor Read Command3 */ 01110 #define REG_CORE_DIGITAL_SENSOR_READ_CMD33 0x0000018B /* CORE Sensor Read Command3 */ 01111 #define REG_CORE_DIGITAL_SENSOR_READ_CMD34 0x000001CB /* CORE Sensor Read Command3 */ 01112 #define REG_CORE_DIGITAL_SENSOR_READ_CMD35 0x0000020B /* CORE Sensor Read Command3 */ 01113 #define REG_CORE_DIGITAL_SENSOR_READ_CMD36 0x0000024B /* CORE Sensor Read Command3 */ 01114 #define REG_CORE_DIGITAL_SENSOR_READ_CMD37 0x0000028B /* CORE Sensor Read Command3 */ 01115 #define REG_CORE_DIGITAL_SENSOR_READ_CMD38 0x000002CB /* CORE Sensor Read Command3 */ 01116 #define REG_CORE_DIGITAL_SENSOR_READ_CMD39 0x0000030B /* CORE Sensor Read Command3 */ 01117 #define REG_CORE_DIGITAL_SENSOR_READ_CMD310 0x0000034B /* CORE Sensor Read Command3 */ 01118 #define REG_CORE_DIGITAL_SENSOR_READ_CMD311 0x0000038B /* CORE Sensor Read Command3 */ 01119 #define REG_CORE_DIGITAL_SENSOR_READ_CMD312 0x000003CB /* CORE Sensor Read Command3 */ 01120 #define REG_CORE_DIGITAL_SENSOR_READ_CMD3n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD30 + ((i) * 64)) 01121 #define REG_CORE_DIGITAL_SENSOR_READ_CMD3n_COUNT 13 01122 #define REG_CORE_DIGITAL_SENSOR_READ_CMD4n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd4[n] */ 01123 #define REG_CORE_DIGITAL_SENSOR_READ_CMD40_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD40 */ 01124 #define REG_CORE_DIGITAL_SENSOR_READ_CMD41_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD41 */ 01125 #define REG_CORE_DIGITAL_SENSOR_READ_CMD42_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD42 */ 01126 #define REG_CORE_DIGITAL_SENSOR_READ_CMD43_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD43 */ 01127 #define REG_CORE_DIGITAL_SENSOR_READ_CMD44_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD44 */ 01128 #define REG_CORE_DIGITAL_SENSOR_READ_CMD45_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD45 */ 01129 #define REG_CORE_DIGITAL_SENSOR_READ_CMD46_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD46 */ 01130 #define REG_CORE_DIGITAL_SENSOR_READ_CMD47_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD47 */ 01131 #define REG_CORE_DIGITAL_SENSOR_READ_CMD48_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD48 */ 01132 #define REG_CORE_DIGITAL_SENSOR_READ_CMD49_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD49 */ 01133 #define REG_CORE_DIGITAL_SENSOR_READ_CMD410_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD410 */ 01134 #define REG_CORE_DIGITAL_SENSOR_READ_CMD411_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD411 */ 01135 #define REG_CORE_DIGITAL_SENSOR_READ_CMD412_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD412 */ 01136 #define REG_CORE_DIGITAL_SENSOR_READ_CMD40 0x000000CC /* CORE Sensor Read Command4 */ 01137 #define REG_CORE_DIGITAL_SENSOR_READ_CMD41 0x0000010C /* CORE Sensor Read Command4 */ 01138 #define REG_CORE_DIGITAL_SENSOR_READ_CMD42 0x0000014C /* CORE Sensor Read Command4 */ 01139 #define REG_CORE_DIGITAL_SENSOR_READ_CMD43 0x0000018C /* CORE Sensor Read Command4 */ 01140 #define REG_CORE_DIGITAL_SENSOR_READ_CMD44 0x000001CC /* CORE Sensor Read Command4 */ 01141 #define REG_CORE_DIGITAL_SENSOR_READ_CMD45 0x0000020C /* CORE Sensor Read Command4 */ 01142 #define REG_CORE_DIGITAL_SENSOR_READ_CMD46 0x0000024C /* CORE Sensor Read Command4 */ 01143 #define REG_CORE_DIGITAL_SENSOR_READ_CMD47 0x0000028C /* CORE Sensor Read Command4 */ 01144 #define REG_CORE_DIGITAL_SENSOR_READ_CMD48 0x000002CC /* CORE Sensor Read Command4 */ 01145 #define REG_CORE_DIGITAL_SENSOR_READ_CMD49 0x0000030C /* CORE Sensor Read Command4 */ 01146 #define REG_CORE_DIGITAL_SENSOR_READ_CMD410 0x0000034C /* CORE Sensor Read Command4 */ 01147 #define REG_CORE_DIGITAL_SENSOR_READ_CMD411 0x0000038C /* CORE Sensor Read Command4 */ 01148 #define REG_CORE_DIGITAL_SENSOR_READ_CMD412 0x000003CC /* CORE Sensor Read Command4 */ 01149 #define REG_CORE_DIGITAL_SENSOR_READ_CMD4n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD40 + ((i) * 64)) 01150 #define REG_CORE_DIGITAL_SENSOR_READ_CMD4n_COUNT 13 01151 #define REG_CORE_DIGITAL_SENSOR_READ_CMD5n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd5[n] */ 01152 #define REG_CORE_DIGITAL_SENSOR_READ_CMD50_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD50 */ 01153 #define REG_CORE_DIGITAL_SENSOR_READ_CMD51_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD51 */ 01154 #define REG_CORE_DIGITAL_SENSOR_READ_CMD52_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD52 */ 01155 #define REG_CORE_DIGITAL_SENSOR_READ_CMD53_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD53 */ 01156 #define REG_CORE_DIGITAL_SENSOR_READ_CMD54_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD54 */ 01157 #define REG_CORE_DIGITAL_SENSOR_READ_CMD55_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD55 */ 01158 #define REG_CORE_DIGITAL_SENSOR_READ_CMD56_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD56 */ 01159 #define REG_CORE_DIGITAL_SENSOR_READ_CMD57_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD57 */ 01160 #define REG_CORE_DIGITAL_SENSOR_READ_CMD58_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD58 */ 01161 #define REG_CORE_DIGITAL_SENSOR_READ_CMD59_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD59 */ 01162 #define REG_CORE_DIGITAL_SENSOR_READ_CMD510_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD510 */ 01163 #define REG_CORE_DIGITAL_SENSOR_READ_CMD511_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD511 */ 01164 #define REG_CORE_DIGITAL_SENSOR_READ_CMD512_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD512 */ 01165 #define REG_CORE_DIGITAL_SENSOR_READ_CMD50 0x000000CD /* CORE Sensor Read Command5 */ 01166 #define REG_CORE_DIGITAL_SENSOR_READ_CMD51 0x0000010D /* CORE Sensor Read Command5 */ 01167 #define REG_CORE_DIGITAL_SENSOR_READ_CMD52 0x0000014D /* CORE Sensor Read Command5 */ 01168 #define REG_CORE_DIGITAL_SENSOR_READ_CMD53 0x0000018D /* CORE Sensor Read Command5 */ 01169 #define REG_CORE_DIGITAL_SENSOR_READ_CMD54 0x000001CD /* CORE Sensor Read Command5 */ 01170 #define REG_CORE_DIGITAL_SENSOR_READ_CMD55 0x0000020D /* CORE Sensor Read Command5 */ 01171 #define REG_CORE_DIGITAL_SENSOR_READ_CMD56 0x0000024D /* CORE Sensor Read Command5 */ 01172 #define REG_CORE_DIGITAL_SENSOR_READ_CMD57 0x0000028D /* CORE Sensor Read Command5 */ 01173 #define REG_CORE_DIGITAL_SENSOR_READ_CMD58 0x000002CD /* CORE Sensor Read Command5 */ 01174 #define REG_CORE_DIGITAL_SENSOR_READ_CMD59 0x0000030D /* CORE Sensor Read Command5 */ 01175 #define REG_CORE_DIGITAL_SENSOR_READ_CMD510 0x0000034D /* CORE Sensor Read Command5 */ 01176 #define REG_CORE_DIGITAL_SENSOR_READ_CMD511 0x0000038D /* CORE Sensor Read Command5 */ 01177 #define REG_CORE_DIGITAL_SENSOR_READ_CMD512 0x000003CD /* CORE Sensor Read Command5 */ 01178 #define REG_CORE_DIGITAL_SENSOR_READ_CMD5n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD50 + ((i) * 64)) 01179 #define REG_CORE_DIGITAL_SENSOR_READ_CMD5n_COUNT 13 01180 #define REG_CORE_DIGITAL_SENSOR_READ_CMD6n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd6[n] */ 01181 #define REG_CORE_DIGITAL_SENSOR_READ_CMD60_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD60 */ 01182 #define REG_CORE_DIGITAL_SENSOR_READ_CMD61_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD61 */ 01183 #define REG_CORE_DIGITAL_SENSOR_READ_CMD62_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD62 */ 01184 #define REG_CORE_DIGITAL_SENSOR_READ_CMD63_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD63 */ 01185 #define REG_CORE_DIGITAL_SENSOR_READ_CMD64_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD64 */ 01186 #define REG_CORE_DIGITAL_SENSOR_READ_CMD65_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD65 */ 01187 #define REG_CORE_DIGITAL_SENSOR_READ_CMD66_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD66 */ 01188 #define REG_CORE_DIGITAL_SENSOR_READ_CMD67_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD67 */ 01189 #define REG_CORE_DIGITAL_SENSOR_READ_CMD68_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD68 */ 01190 #define REG_CORE_DIGITAL_SENSOR_READ_CMD69_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD69 */ 01191 #define REG_CORE_DIGITAL_SENSOR_READ_CMD610_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD610 */ 01192 #define REG_CORE_DIGITAL_SENSOR_READ_CMD611_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD611 */ 01193 #define REG_CORE_DIGITAL_SENSOR_READ_CMD612_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD612 */ 01194 #define REG_CORE_DIGITAL_SENSOR_READ_CMD60 0x000000CE /* CORE Sensor Read Command6 */ 01195 #define REG_CORE_DIGITAL_SENSOR_READ_CMD61 0x0000010E /* CORE Sensor Read Command6 */ 01196 #define REG_CORE_DIGITAL_SENSOR_READ_CMD62 0x0000014E /* CORE Sensor Read Command6 */ 01197 #define REG_CORE_DIGITAL_SENSOR_READ_CMD63 0x0000018E /* CORE Sensor Read Command6 */ 01198 #define REG_CORE_DIGITAL_SENSOR_READ_CMD64 0x000001CE /* CORE Sensor Read Command6 */ 01199 #define REG_CORE_DIGITAL_SENSOR_READ_CMD65 0x0000020E /* CORE Sensor Read Command6 */ 01200 #define REG_CORE_DIGITAL_SENSOR_READ_CMD66 0x0000024E /* CORE Sensor Read Command6 */ 01201 #define REG_CORE_DIGITAL_SENSOR_READ_CMD67 0x0000028E /* CORE Sensor Read Command6 */ 01202 #define REG_CORE_DIGITAL_SENSOR_READ_CMD68 0x000002CE /* CORE Sensor Read Command6 */ 01203 #define REG_CORE_DIGITAL_SENSOR_READ_CMD69 0x0000030E /* CORE Sensor Read Command6 */ 01204 #define REG_CORE_DIGITAL_SENSOR_READ_CMD610 0x0000034E /* CORE Sensor Read Command6 */ 01205 #define REG_CORE_DIGITAL_SENSOR_READ_CMD611 0x0000038E /* CORE Sensor Read Command6 */ 01206 #define REG_CORE_DIGITAL_SENSOR_READ_CMD612 0x000003CE /* CORE Sensor Read Command6 */ 01207 #define REG_CORE_DIGITAL_SENSOR_READ_CMD6n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD60 + ((i) * 64)) 01208 #define REG_CORE_DIGITAL_SENSOR_READ_CMD6n_COUNT 13 01209 #define REG_CORE_DIGITAL_SENSOR_READ_CMD7n_RESET 0x00000000 /* Reset Value for Digital_Sensor_Read_Cmd7[n] */ 01210 #define REG_CORE_DIGITAL_SENSOR_READ_CMD70_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD70 */ 01211 #define REG_CORE_DIGITAL_SENSOR_READ_CMD71_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD71 */ 01212 #define REG_CORE_DIGITAL_SENSOR_READ_CMD72_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD72 */ 01213 #define REG_CORE_DIGITAL_SENSOR_READ_CMD73_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD73 */ 01214 #define REG_CORE_DIGITAL_SENSOR_READ_CMD74_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD74 */ 01215 #define REG_CORE_DIGITAL_SENSOR_READ_CMD75_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD75 */ 01216 #define REG_CORE_DIGITAL_SENSOR_READ_CMD76_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD76 */ 01217 #define REG_CORE_DIGITAL_SENSOR_READ_CMD77_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD77 */ 01218 #define REG_CORE_DIGITAL_SENSOR_READ_CMD78_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD78 */ 01219 #define REG_CORE_DIGITAL_SENSOR_READ_CMD79_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD79 */ 01220 #define REG_CORE_DIGITAL_SENSOR_READ_CMD710_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD710 */ 01221 #define REG_CORE_DIGITAL_SENSOR_READ_CMD711_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD711 */ 01222 #define REG_CORE_DIGITAL_SENSOR_READ_CMD712_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_READ_CMD712 */ 01223 #define REG_CORE_DIGITAL_SENSOR_READ_CMD70 0x000000CF /* CORE Sensor Read Command7 */ 01224 #define REG_CORE_DIGITAL_SENSOR_READ_CMD71 0x0000010F /* CORE Sensor Read Command7 */ 01225 #define REG_CORE_DIGITAL_SENSOR_READ_CMD72 0x0000014F /* CORE Sensor Read Command7 */ 01226 #define REG_CORE_DIGITAL_SENSOR_READ_CMD73 0x0000018F /* CORE Sensor Read Command7 */ 01227 #define REG_CORE_DIGITAL_SENSOR_READ_CMD74 0x000001CF /* CORE Sensor Read Command7 */ 01228 #define REG_CORE_DIGITAL_SENSOR_READ_CMD75 0x0000020F /* CORE Sensor Read Command7 */ 01229 #define REG_CORE_DIGITAL_SENSOR_READ_CMD76 0x0000024F /* CORE Sensor Read Command7 */ 01230 #define REG_CORE_DIGITAL_SENSOR_READ_CMD77 0x0000028F /* CORE Sensor Read Command7 */ 01231 #define REG_CORE_DIGITAL_SENSOR_READ_CMD78 0x000002CF /* CORE Sensor Read Command7 */ 01232 #define REG_CORE_DIGITAL_SENSOR_READ_CMD79 0x0000030F /* CORE Sensor Read Command7 */ 01233 #define REG_CORE_DIGITAL_SENSOR_READ_CMD710 0x0000034F /* CORE Sensor Read Command7 */ 01234 #define REG_CORE_DIGITAL_SENSOR_READ_CMD711 0x0000038F /* CORE Sensor Read Command7 */ 01235 #define REG_CORE_DIGITAL_SENSOR_READ_CMD712 0x000003CF /* CORE Sensor Read Command7 */ 01236 #define REG_CORE_DIGITAL_SENSOR_READ_CMD7n(i) (REG_CORE_DIGITAL_SENSOR_READ_CMD70 + ((i) * 64)) 01237 #define REG_CORE_DIGITAL_SENSOR_READ_CMD7n_COUNT 13 01238 01239 /* ============================================================================================================================ 01240 CORE Register BitMasks, Positions & Enumerations 01241 ============================================================================================================================ */ 01242 /* ------------------------------------------------------------------------------------------------------------------------- 01243 CORE_COMMAND Pos/Masks Description 01244 ------------------------------------------------------------------------------------------------------------------------- */ 01245 #define BITP_CORE_COMMAND_SPECIAL_COMMAND 0 /* Special Command */ 01246 #define BITM_CORE_COMMAND_SPECIAL_COMMAND 0x000000FF /* Special Command */ 01247 #define ENUM_CORE_COMMAND_NOP 0x00000000 /* Special_Command: No Command */ 01248 #define ENUM_CORE_COMMAND_CONVERT 0x00000001 /* Special_Command: Start ADC Conversions */ 01249 #define ENUM_CORE_COMMAND_CONVERT_WITH_RAW 0x00000002 /* Special_Command: Start Conversions with Added RAW ADC Data */ 01250 #define ENUM_CORE_COMMAND_LATCH_CONFIG 0x00000007 /* Special_Command: Latch Configuration. */ 01251 #define ENUM_CORE_COMMAND_LOAD_LUT 0x00000008 /* Special_Command: Load LUT from FLASH */ 01252 #define ENUM_CORE_COMMAND_SAVE_LUT 0x00000009 /* Special_Command: Save LUT to FLASH */ 01253 #define ENUM_CORE_COMMAND_POWER_DOWN 0x00000014 /* Special_Command: Enter Low Power State */ 01254 #define ENUM_CORE_COMMAND_LOAD_CONFIG_1 0x00000018 /* Special_Command: Load Registers with Configuration#1 from FLASH */ 01255 #define ENUM_CORE_COMMAND_SAVE_CONFIG_1 0x00000019 /* Special_Command: Store Current Registers to FLASH Configuration#1 */ 01256 01257 /* ------------------------------------------------------------------------------------------------------------------------- 01258 CORE_MODE Pos/Masks Description 01259 ------------------------------------------------------------------------------------------------------------------------- */ 01260 #define BITP_CORE_MODE_DRDY_MODE 2 /* Indicates Behavior of DRDY with Respect to FIFO State */ 01261 #define BITP_CORE_MODE_CONVERSION_MODE 0 /* Conversion Mode */ 01262 #define BITM_CORE_MODE_DRDY_MODE 0x0000000C /* Indicates Behavior of DRDY with Respect to FIFO State */ 01263 #define BITM_CORE_MODE_CONVERSION_MODE 0x00000003 /* Conversion Mode */ 01264 #define ENUM_CORE_MODE_DRDY_PER_CONVERSION 0x00000000 /* Drdy_Mode: Data Ready Per Conversion */ 01265 #define ENUM_CORE_MODE_DRDY_PER_CYCLE 0x00000004 /* Drdy_Mode: Data Ready Per Cycle */ 01266 #define ENUM_CORE_MODE_SINGLECYCLE 0x00000000 /* Conversion_Mode: Single Cycle */ 01267 #define ENUM_CORE_MODE_CONTINUOUS 0x00000002 /* Conversion_Mode: Continuous Conversion */ 01268 01269 /* ------------------------------------------------------------------------------------------------------------------------- 01270 CORE_POWER_CONFIG Pos/Masks Description 01271 ------------------------------------------------------------------------------------------------------------------------- */ 01272 #define BITP_CORE_POWER_CONFIG_POWER_MODE_MCU 0 /* MCU Power Mode */ 01273 #define BITM_CORE_POWER_CONFIG_POWER_MODE_MCU 0x00000001 /* MCU Power Mode */ 01274 #define ENUM_CORE_POWER_CONFIG_ACTIVE_MODE 0x00000000 /* Power_Mode_MCU: Part is fully powered up and either cycling through a sequence or awaiting a configuration */ 01275 #define ENUM_CORE_POWER_CONFIG_HIBERNATION 0x00000001 /* Power_Mode_MCU: module has entede hibernation mode. All analog circuitry is disabled. All peripherals disabled apart from the Wake-up pin functionality. */ 01276 01277 /* ------------------------------------------------------------------------------------------------------------------------- 01278 CORE_CYCLE_CONTROL Pos/Masks Description 01279 ------------------------------------------------------------------------------------------------------------------------- */ 01280 #define BITP_CORE_CYCLE_CONTROL_CYCLE_TIME_UNITS 14 /* Units for Cycle Time */ 01281 #define BITP_CORE_CYCLE_CONTROL_VBIAS 13 /* Voltage Bias Global Enable */ 01282 #define BITP_CORE_CYCLE_CONTROL_CYCLE_TYPE 12 /* Type of Measurement Cycle */ 01283 #define BITP_CORE_CYCLE_CONTROL_CYCLE_TIME 0 /* Duration of a Full Measurement Cycle */ 01284 #define BITM_CORE_CYCLE_CONTROL_CYCLE_TIME_UNITS 0x0000C000 /* Units for Cycle Time */ 01285 #define BITM_CORE_CYCLE_CONTROL_VBIAS 0x00002000 /* Voltage Bias Global Enable */ 01286 #define BITM_CORE_CYCLE_CONTROL_CYCLE_TYPE 0x00001000 /* Type of Measurement Cycle */ 01287 #define BITM_CORE_CYCLE_CONTROL_CYCLE_TIME 0x00000FFF /* Duration of a Full Measurement Cycle */ 01288 #define ENUM_CORE_CYCLE_CONTROL_MILLISECONDS 0x00000000 /* Cycle_Time_Units: Milli-Seconds */ 01289 #define ENUM_CORE_CYCLE_CONTROL_SECONDS 0x00004000 /* Cycle_Time_Units: Seconds */ 01290 #define ENUM_CORE_CYCLE_CONTROL_VBIAS_DISABLE 0x00000000 /* Vbias: Vbias Disabled */ 01291 #define ENUM_CORE_CYCLE_CONTROL_VBIAS_ENABLE 0x00002000 /* Vbias: Enable Vbias Output For the Duration of a Cycle */ 01292 #define ENUM_CORE_CYCLE_CONTROL_SWITCH 0x00000000 /* Cycle_Type: Switch Channels After Every Conversion */ 01293 #define ENUM_CORE_CYCLE_CONTROL_FULL 0x00001000 /* Cycle_Type: Perform Full Number Of Conversions On A Channel Consecutively */ 01294 01295 /* ------------------------------------------------------------------------------------------------------------------------- 01296 CORE_STATUS Pos/Masks Description 01297 ------------------------------------------------------------------------------------------------------------------------- */ 01298 #define BITP_CORE_STATUS_FIFO_ERROR 5 /* Indicates Error with FIFO */ 01299 #define BITP_CORE_STATUS_CMD_RUNNING 4 /* Indicates a Special Command is Active */ 01300 #define BITP_CORE_STATUS_DRDY 3 /* Indicates a New Sensor Result is Available to Be Read */ 01301 #define BITP_CORE_STATUS_ERROR 2 /* Indicates an Error */ 01302 #define BITP_CORE_STATUS_ALERT_ACTIVE 1 /* Indicates One or More Sensors Alerts are Active */ 01303 #define BITM_CORE_STATUS_FIFO_ERROR 0x00000020 /* Indicates Error with FIFO */ 01304 #define BITM_CORE_STATUS_CMD_RUNNING 0x00000010 /* Indicates a Special Command is Active */ 01305 #define BITM_CORE_STATUS_DRDY 0x00000008 /* Indicates a New Sensor Result is Available to Be Read */ 01306 #define BITM_CORE_STATUS_ERROR 0x00000004 /* Indicates an Error */ 01307 #define BITM_CORE_STATUS_ALERT_ACTIVE 0x00000002 /* Indicates One or More Sensors Alerts are Active */ 01308 01309 /* ------------------------------------------------------------------------------------------------------------------------- 01310 CORE_DIAGNOSTICS_STATUS Pos/Masks Description 01311 ------------------------------------------------------------------------------------------------------------------------- */ 01312 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_CALIBRATION_ERROR 13 /* Indicates Error During Internal Device Calibrations */ 01313 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_CONVERSION_ERROR 12 /* Indicates Error During Internal ADC Conversions */ 01314 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_AINP_OV_ERROR 11 /* Indicates Over-Voltage Error on Positive Analog Input */ 01315 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_AINP_UV_ERROR 10 /* Indicates Under-Voltage Error on Positive Analog Input */ 01316 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_AINM_OV_ERROR 9 /* Indicates Over-Voltage Error on Negative Analog Input */ 01317 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_AINM_UV_ERROR 8 /* Indicates Under-Voltage Error on Negative Analog Input */ 01318 #define BITP_CORE_DIAGNOSTICS_STATUS_DIAG_CHECKSUM_ERROR 0 /* Indicates Error on Internal Checksum Calculations */ 01319 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_CALIBRATION_ERROR 0x00002000 /* Indicates Error During Internal Device Calibrations */ 01320 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_CONVERSION_ERROR 0x00001000 /* Indicates Error During Internal ADC Conversions */ 01321 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_AINP_OV_ERROR 0x00000800 /* Indicates Over-Voltage Error on Positive Analog Input */ 01322 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_AINP_UV_ERROR 0x00000400 /* Indicates Under-Voltage Error on Positive Analog Input */ 01323 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_AINM_OV_ERROR 0x00000200 /* Indicates Over-Voltage Error on Negative Analog Input */ 01324 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_AINM_UV_ERROR 0x00000100 /* Indicates Under-Voltage Error on Negative Analog Input */ 01325 #define BITM_CORE_DIAGNOSTICS_STATUS_DIAG_CHECKSUM_ERROR 0x00000001 /* Indicates Error on Internal Checksum Calculations */ 01326 01327 /* ------------------------------------------------------------------------------------------------------------------------- 01328 CORE_CHANNEL_ALERT_STATUS Pos/Masks Description 01329 ------------------------------------------------------------------------------------------------------------------------- */ 01330 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH11 11 /* Indicates Channel Alert is Active */ 01331 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH10 10 /* Indicates Channel Alert is Active */ 01332 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH9 9 /* Indicates Channel Alert is Active */ 01333 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH8 8 /* Indicates Channel Alert is Active */ 01334 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH7 7 /* Indicates Channel Alert is Active */ 01335 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH6 6 /* Indicates Channel Alert is Active */ 01336 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH5 5 /* Indicates Channel Alert is Active */ 01337 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH4 4 /* Indicates Channel Alert is Active */ 01338 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH3 3 /* Indicates Channel Alert is Active */ 01339 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH2 2 /* Indicates Channel Alert is Active */ 01340 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH1 1 /* Indicates Channel Alert is Active */ 01341 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH0 0 /* Indicates Channel Alert is Active */ 01342 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH11 0x00000800 /* Indicates Channel Alert is Active */ 01343 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH10 0x00000400 /* Indicates Channel Alert is Active */ 01344 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH9 0x00000200 /* Indicates Channel Alert is Active */ 01345 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH8 0x00000100 /* Indicates Channel Alert is Active */ 01346 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH7 0x00000080 /* Indicates Channel Alert is Active */ 01347 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH6 0x00000040 /* Indicates Channel Alert is Active */ 01348 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH5 0x00000020 /* Indicates Channel Alert is Active */ 01349 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH4 0x00000010 /* Indicates Channel Alert is Active */ 01350 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH3 0x00000008 /* Indicates Channel Alert is Active */ 01351 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH2 0x00000004 /* Indicates Channel Alert is Active */ 01352 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH1 0x00000002 /* Indicates Channel Alert is Active */ 01353 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH0 0x00000001 /* Indicates Channel Alert is Active */ 01354 01355 /* ------------------------------------------------------------------------------------------------------------------------- 01356 CORE_ALERT_STATUS_2 Pos/Masks Description 01357 ------------------------------------------------------------------------------------------------------------------------- */ 01358 #define BITP_CORE_ALERT_STATUS_2_CONFIGURATION_ERROR 2 /* Indicates Error with Programmed Configuration */ 01359 #define BITP_CORE_ALERT_STATUS_2_LUT_ERROR 1 /* Indicates Error with One or More Look-Up-Tables */ 01360 #define BITM_CORE_ALERT_STATUS_2_CONFIGURATION_ERROR 0x00000004 /* Indicates Error with Programmed Configuration */ 01361 #define BITM_CORE_ALERT_STATUS_2_LUT_ERROR 0x00000002 /* Indicates Error with One or More Look-Up-Tables */ 01362 01363 /* ------------------------------------------------------------------------------------------------------------------------- 01364 CORE_ALERT_DETAIL_CH[n] Pos/Masks Description 01365 ------------------------------------------------------------------------------------------------------------------------- */ 01366 #define BITP_CORE_ALERT_DETAIL_CH_COMP_NOT_READY 15 /* Indicates Compensation Channel Not Ready When Required */ 01367 #define BITP_CORE_ALERT_DETAIL_CH_SENSOR_NOT_READY 14 /* Indicates Digital Sensor Not Ready When Read */ 01368 #define BITP_CORE_ALERT_DETAIL_CH_CORRECTION_OVERRANGE 13 /* Indicates Result Larger Than LUT/Equation Range */ 01369 #define BITP_CORE_ALERT_DETAIL_CH_CORRECTION_UNDERRANGE 12 /* Indicates Result Less Than LUT/Equation Range */ 01370 #define BITP_CORE_ALERT_DETAIL_CH_OVER_VOLTAGE 11 /* Indicates Channel Over-Voltage */ 01371 #define BITP_CORE_ALERT_DETAIL_CH_UNDER_VOLTAGE 10 /* Indicates Channel Under-Voltage */ 01372 #define BITP_CORE_ALERT_DETAIL_CH_LUT_ERROR_CH 9 /* Indicates Error with Channel Look-Up-Table */ 01373 #define BITP_CORE_ALERT_DETAIL_CH_CONFIG_ERR 8 /* Indicates Configuration Error on Channel */ 01374 #define BITP_CORE_ALERT_DETAIL_CH_CALIBRATION_INVALID 7 /* Indicates Problem During Calibration of Channel */ 01375 #define BITP_CORE_ALERT_DETAIL_CH_REF_DETECT 6 /* Indicates Whether ADC Reference is Valid */ 01376 #define BITP_CORE_ALERT_DETAIL_CH_SENSOR_OPEN 5 /* Indicates Sensor Input is Open Circuit */ 01377 #define BITP_CORE_ALERT_DETAIL_CH_HIGH_LIMIT 4 /* Indicates Sensor Result is Greater Than High Limit */ 01378 #define BITP_CORE_ALERT_DETAIL_CH_LOW_LIMIT 3 /* Indicates Sensor Result is Less Than Low Limit */ 01379 #define BITP_CORE_ALERT_DETAIL_CH_OVER_RANGE 2 /* Indicates Channel Over-Range */ 01380 #define BITP_CORE_ALERT_DETAIL_CH_UNDER_RANGE 1 /* Indicates Channel Under-Range */ 01381 #define BITP_CORE_ALERT_DETAIL_CH_TIME_OUT 0 /* Indicates Time-Out Error from Digital Sensor */ 01382 #define BITM_CORE_ALERT_DETAIL_CH_COMP_NOT_READY 0x00008000 /* Indicates Compensation Channel Not Ready When Required */ 01383 #define BITM_CORE_ALERT_DETAIL_CH_SENSOR_NOT_READY 0x00004000 /* Indicates Digital Sensor Not Ready When Read */ 01384 #define BITM_CORE_ALERT_DETAIL_CH_CORRECTION_OVERRANGE 0x00002000 /* Indicates Result Larger Than LUT/Equation Range */ 01385 #define BITM_CORE_ALERT_DETAIL_CH_CORRECTION_UNDERRANGE 0x00001000 /* Indicates Result Less Than LUT/Equation Range */ 01386 #define BITM_CORE_ALERT_DETAIL_CH_OVER_VOLTAGE 0x00000800 /* Indicates Channel Over-Voltage */ 01387 #define BITM_CORE_ALERT_DETAIL_CH_UNDER_VOLTAGE 0x00000400 /* Indicates Channel Under-Voltage */ 01388 #define BITM_CORE_ALERT_DETAIL_CH_LUT_ERROR_CH 0x00000200 /* Indicates Error with Channel Look-Up-Table */ 01389 #define BITM_CORE_ALERT_DETAIL_CH_CONFIG_ERR 0x00000100 /* Indicates Configuration Error on Channel */ 01390 #define BITM_CORE_ALERT_DETAIL_CH_CALIBRATION_INVALID 0x00000080 /* Indicates Problem During Calibration of Channel */ 01391 #define BITM_CORE_ALERT_DETAIL_CH_REF_DETECT 0x00000040 /* Indicates Whether ADC Reference is Valid */ 01392 #define BITM_CORE_ALERT_DETAIL_CH_SENSOR_OPEN 0x00000020 /* Indicates Sensor Input is Open Circuit */ 01393 #define BITM_CORE_ALERT_DETAIL_CH_HIGH_LIMIT 0x00000010 /* Indicates Sensor Result is Greater Than High Limit */ 01394 #define BITM_CORE_ALERT_DETAIL_CH_LOW_LIMIT 0x00000008 /* Indicates Sensor Result is Less Than Low Limit */ 01395 #define BITM_CORE_ALERT_DETAIL_CH_OVER_RANGE 0x00000004 /* Indicates Channel Over-Range */ 01396 #define BITM_CORE_ALERT_DETAIL_CH_UNDER_RANGE 0x00000002 /* Indicates Channel Under-Range */ 01397 #define BITM_CORE_ALERT_DETAIL_CH_TIME_OUT 0x00000001 /* Indicates Time-Out Error from Digital Sensor */ 01398 01399 /* ------------------------------------------------------------------------------------------------------------------------- 01400 CORE_ERROR_CODE Pos/Masks Description 01401 ------------------------------------------------------------------------------------------------------------------------- */ 01402 #define BITP_CORE_ERROR_CODE_ERROR_CODE 0 /* Code Indicating Type of Error */ 01403 #define BITM_CORE_ERROR_CODE_ERROR_CODE 0x0000FFFF /* Code Indicating Type of Error */ 01404 01405 /* ------------------------------------------------------------------------------------------------------------------------- 01406 CORE_ALERT_CODE Pos/Masks Description 01407 ------------------------------------------------------------------------------------------------------------------------- */ 01408 #define BITP_CORE_ALERT_CODE_ALERT_CODE 0 /* Code Indicating Type of Alert */ 01409 #define BITM_CORE_ALERT_CODE_ALERT_CODE 0x0000FFFF /* Code Indicating Type of Alert */ 01410 01411 /* ------------------------------------------------------------------------------------------------------------------------- 01412 CORE_EXTERNAL_REFERENCE_RESISTOR Pos/Masks Description 01413 ------------------------------------------------------------------------------------------------------------------------- */ 01414 #define BITP_CORE_EXTERNAL_REFERENCE_RESISTOR_EXT_REFIN1_VALUE 0 /* Refin1 Value */ 01415 #define BITM_CORE_EXTERNAL_REFERENCE_RESISTOR_EXT_REFIN1_VALUE 0x00000000 /* Refin1 Value */ 01416 01417 /* ------------------------------------------------------------------------------------------------------------------------- 01418 CORE_EXTERNAL_VOLTAGE_REFERENCE Pos/Masks Description 01419 ------------------------------------------------------------------------------------------------------------------------- */ 01420 #define BITP_CORE_EXTERNAL_VOLTAGE_REFERENCE_EXT_REFIN2_VALUE 0 /* Refin2 Value */ 01421 #define BITM_CORE_EXTERNAL_VOLTAGE_REFERENCE_EXT_REFIN2_VALUE 0x00000000 /* Refin2 Value */ 01422 01423 /* ------------------------------------------------------------------------------------------------------------------------- 01424 CORE_DIAGNOSTICS_CONTROL Pos/Masks Description 01425 ------------------------------------------------------------------------------------------------------------------------- */ 01426 #define BITP_CORE_DIAGNOSTICS_CONTROL_DIAG_OSD_FREQ 2 /* Diagnostics Open Sensor Detect Frequency */ 01427 #define BITP_CORE_DIAGNOSTICS_CONTROL_DIAG_MEAS_EN 1 /* Diagnostics Measure Enable */ 01428 #define BITP_CORE_DIAGNOSTICS_CONTROL_DIAG_GLOBAL_EN 0 /* Diagnostics Global Enable */ 01429 #define BITM_CORE_DIAGNOSTICS_CONTROL_DIAG_OSD_FREQ 0x0000000C /* Diagnostics Open Sensor Detect Frequency */ 01430 #define BITM_CORE_DIAGNOSTICS_CONTROL_DIAG_MEAS_EN 0x00000002 /* Diagnostics Measure Enable */ 01431 #define BITM_CORE_DIAGNOSTICS_CONTROL_DIAG_GLOBAL_EN 0x00000001 /* Diagnostics Global Enable */ 01432 #define ENUM_CORE_DIAGNOSTICS_CONTROL_OCD_OFF 0x00000000 /* Diag_OSD_Freq: No Open-Circuit Detection During Measurement */ 01433 #define ENUM_CORE_DIAGNOSTICS_CONTROL_OCD_PER_1_CYCLE 0x00000004 /* Diag_OSD_Freq: Open-Circuit Detection Performed Once Per Measurement Cycle */ 01434 #define ENUM_CORE_DIAGNOSTICS_CONTROL_OCD_PER_100_CYCLES 0x00000008 /* Diag_OSD_Freq: Open-Circuit Detection Performed Once Per Hundred Measurement Cycles */ 01435 #define ENUM_CORE_DIAGNOSTICS_CONTROL_OCD_PER_1000_CYCLES 0x0000000C /* Diag_OSD_Freq: Open-Circuit Detection Performed Once Per Thousand Measurement Cycles */ 01436 01437 /* ------------------------------------------------------------------------------------------------------------------------- 01438 CORE_DATA_FIFO Pos/Masks Description 01439 ------------------------------------------------------------------------------------------------------------------------- */ 01440 #define BITP_CORE_DATA_FIFO_DATA_FIFO 0 /* Fifo Buffer of Sensor Results */ 01441 #define BITM_CORE_DATA_FIFO_DATA_FIFO 0x000000FF /* Fifo Buffer of Sensor Results */ 01442 01443 /* ------------------------------------------------------------------------------------------------------------------------- 01444 CORE_DEBUG_CODE Pos/Masks Description 01445 ------------------------------------------------------------------------------------------------------------------------- */ 01446 #define BITP_CORE_DEBUG_CODE_DEBUG_CODE 0 /* Additional Information on Source of Alert or Errors */ 01447 #define BITM_CORE_DEBUG_CODE_DEBUG_CODE 0x00000000 /* Additional Information on Source of Alert or Errors */ 01448 01449 /* ------------------------------------------------------------------------------------------------------------------------- 01450 CORE_ADVANCED_SENSOR_ACCESS Pos/Masks Description 01451 ------------------------------------------------------------------------------------------------------------------------- */ 01452 #define BITP_CORE_ADVANCED_SENSOR_ACCESS_ADVANCED_SENSOR_ACCESS 0 /* Write Specific Key Value to Access Advanced Sensors */ 01453 #define BITM_CORE_ADVANCED_SENSOR_ACCESS_ADVANCED_SENSOR_ACCESS 0x0000FFFF /* Write Specific Key Value to Access Advanced Sensors */ 01454 01455 /* ------------------------------------------------------------------------------------------------------------------------- 01456 CORE_LUT_SELECT Pos/Masks Description 01457 ------------------------------------------------------------------------------------------------------------------------- */ 01458 #define BITP_CORE_LUT_SELECT_LUT_RW 7 /* Read or Write LUT Data */ 01459 #define BITM_CORE_LUT_SELECT_LUT_RW 0x00000080 /* Read or Write LUT Data */ 01460 #define ENUM_CORE_LUT_SELECT_LUT_READ 0x00000000 /* LUT_RW: Read Addressed LUT Data */ 01461 #define ENUM_CORE_LUT_SELECT_LUT_WRITE 0x00000080 /* LUT_RW: Write Addressed LUT Data */ 01462 01463 /* ------------------------------------------------------------------------------------------------------------------------- 01464 CORE_LUT_OFFSET Pos/Masks Description 01465 ------------------------------------------------------------------------------------------------------------------------- */ 01466 #define BITP_CORE_LUT_OFFSET_LUT_OFFSET 0 /* Offset into Look-Up-Table */ 01467 #define BITM_CORE_LUT_OFFSET_LUT_OFFSET 0x00003FFF /* Offset into Look-Up-Table */ 01468 01469 /* ------------------------------------------------------------------------------------------------------------------------- 01470 CORE_LUT_DATA Pos/Masks Description 01471 ------------------------------------------------------------------------------------------------------------------------- */ 01472 #define BITP_CORE_LUT_DATA_LUT_DATA 0 /* Data Byte to Write to / Read from Look-Up-Table */ 01473 #define BITM_CORE_LUT_DATA_LUT_DATA 0x000000FF /* Data Byte to Write to / Read from Look-Up-Table */ 01474 01475 /* ------------------------------------------------------------------------------------------------------------------------- 01476 CORE_REVISION Pos/Masks Description 01477 ------------------------------------------------------------------------------------------------------------------------- */ 01478 #define BITP_CORE_REVISION_REV_MAJOR 24 /* Major Revision Information */ 01479 #define BITP_CORE_REVISION_REV_MINOR 16 /* Minor Revision Information */ 01480 #define BITP_CORE_REVISION_REV_PATCH 0 /* Patch Revision Information */ 01481 #define BITM_CORE_REVISION_REV_MAJOR 0xFF000000 /* Major Revision Information */ 01482 #define BITM_CORE_REVISION_REV_MINOR 0x00FF0000 /* Minor Revision Information */ 01483 #define BITM_CORE_REVISION_REV_PATCH 0x0000FFFF /* Patch Revision Information */ 01484 01485 /* ------------------------------------------------------------------------------------------------------------------------- 01486 CORE_CHANNEL_COUNT[n] Pos/Masks Description 01487 ------------------------------------------------------------------------------------------------------------------------- */ 01488 #define BITP_CORE_CHANNEL_COUNT_CHANNEL_ENABLE 7 /* Enable Channel in Measurement Cycle */ 01489 #define BITP_CORE_CHANNEL_COUNT_CHANNEL_COUNT 0 /* How Many Times Channel Should Appear in One Cycle */ 01490 #define BITM_CORE_CHANNEL_COUNT_CHANNEL_ENABLE 0x00000080 /* Enable Channel in Measurement Cycle */ 01491 #define BITM_CORE_CHANNEL_COUNT_CHANNEL_COUNT 0x0000007F /* How Many Times Channel Should Appear in One Cycle */ 01492 01493 /* ------------------------------------------------------------------------------------------------------------------------- 01494 CORE_CHANNEL_OPTIONS[n] Pos/Masks Description 01495 ------------------------------------------------------------------------------------------------------------------------- */ 01496 #define BITP_CORE_CHANNEL_OPTIONS_CHANNEL_PRIORITY 0 /* Indicates Priority or Position of This Channel in Sequence */ 01497 #define BITM_CORE_CHANNEL_OPTIONS_CHANNEL_PRIORITY 0x0000000F /* Indicates Priority or Position of This Channel in Sequence */ 01498 01499 /* ------------------------------------------------------------------------------------------------------------------------- 01500 CORE_SENSOR_TYPE[n] Pos/Masks Description 01501 ------------------------------------------------------------------------------------------------------------------------- */ 01502 #define BITP_CORE_SENSOR_TYPE_SENSOR_TYPE 0 /* Sensor Type */ 01503 #define BITM_CORE_SENSOR_TYPE_SENSOR_TYPE 0x00000FFF /* Sensor Type */ 01504 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_T 0x00000000 /* Sensor_Type: Thermocouple T-Type Sensor Defined Level 1 */ 01505 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_J 0x00000001 /* Sensor_Type: Thermocouple J-Type Sensor Defined Level 1 */ 01506 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_K 0x00000002 /* Sensor_Type: Thermocouple K-Type Sensor Defined Level 1 */ 01507 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT100 0x00000020 /* Sensor_Type: RTD 2 Wire PT100 Sensor Defined Level 1 */ 01508 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT1000 0x00000021 /* Sensor_Type: RTD 2 Wire PT1000 Sensor Defined Level 1 */ 01509 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT100 0x00000040 /* Sensor_Type: RTD 3 Wire PT100 Sensor Defined Level 1 */ 01510 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT1000 0x00000041 /* Sensor_Type: RTD 3 Wire PT1000 Sensor Defined Level 1 */ 01511 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT100 0x00000060 /* Sensor_Type: RTD 4 Wire PT100 Sensor Defined Level 1 */ 01512 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT1000 0x00000061 /* Sensor_Type: RTD 4 Wire PT1000 Sensor Defined Level 1 */ 01513 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_A_10K 0x00000080 /* Sensor_Type: Thermistor Type A 10kOhm Sensor Defined Level 1 */ 01514 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_B_10K 0x00000081 /* Sensor_Type: Thermistor Type B 10kOhm Sensor Defined Level 1 */ 01515 #define ENUM_CORE_SENSOR_TYPE_BRIDGE_4W_2 0x000000A9 /* Sensor_Type: Bridge 4 Wire Sensor 2 Defined Level 2 */ 01516 #define ENUM_CORE_SENSOR_TYPE_BRIDGE_6W_1 0x000000C8 /* Sensor_Type: Bridge 6 Wire Sensor 1 Defined Level 2 */ 01517 #define ENUM_CORE_SENSOR_TYPE_BRIDGE_6W_2 0x000000C9 /* Sensor_Type: Bridge 6 Wire Sensor 2 Defined Level 2 */ 01518 #define ENUM_CORE_SENSOR_TYPE_DIODE_2C_TYPEA 0x000000E0 /* Sensor_Type: Diode 2 Current Type A Sensor Defined Level 1 */ 01519 #define ENUM_CORE_SENSOR_TYPE_DIODE_3C_TYPEA 0x000000E1 /* Sensor_Type: Diode 3 Current Type A Sensor Defined Level 1 */ 01520 #define ENUM_CORE_SENSOR_TYPE_DIODE_2C_1 0x000000E8 /* Sensor_Type: Diode 2 Current Sensor 1 Defined Level 2 */ 01521 #define ENUM_CORE_SENSOR_TYPE_DIODE_3C_1 0x000000E9 /* Sensor_Type: Diode 3 Current Sensor 1 Defined Level 2 */ 01522 #define ENUM_CORE_SENSOR_TYPE_VOLTAGE 0x00000200 /* Sensor_Type: Voltage Input */ 01523 #define ENUM_CORE_SENSOR_TYPE_VOLTAGE_PRESSURE_A 0x00000220 /* Sensor_Type: Voltage Output Pressure Sensor A Defined Level 1 */ 01524 #define ENUM_CORE_SENSOR_TYPE_VOLTAGE_PRESSURE_B 0x00000221 /* Sensor_Type: Voltage Output Pressure Sensor B Defined Level 1 */ 01525 #define ENUM_CORE_SENSOR_TYPE_CURRENT 0x00000300 /* Sensor_Type: Current Input */ 01526 #define ENUM_CORE_SENSOR_TYPE_I2C_PRESSURE_A 0x00000800 /* Sensor_Type: I2C Pressure Sensor A Defined Level 1 */ 01527 #define ENUM_CORE_SENSOR_TYPE_I2C_PRESSURE_B 0x00000801 /* Sensor_Type: I2C Pressure Sensor B Defined Level 1 */ 01528 #define ENUM_CORE_SENSOR_TYPE_I2C_HUMIDITY_A 0x00000840 /* Sensor_Type: I2C Humidity Sensor A Defined Level 1 */ 01529 #define ENUM_CORE_SENSOR_TYPE_I2C_HUMIDITY_B 0x00000841 /* Sensor_Type: I2C Humidity Sensor B Defined Level 1 */ 01530 #define ENUM_CORE_SENSOR_TYPE_SPI_ACCELEROMETER_A 0x00000C80 /* Sensor_Type: SPI Accelerometer Sensor A 3-Axis Defined Level 1 */ 01531 #define ENUM_CORE_SENSOR_TYPE_SPI_ACCELEROMETER_B 0x00000C81 /* Sensor_Type: SPI Accelerometer Sensor B 3-Axis Defined Level 1 */ 01532 #define ENUM_CORE_SENSOR_TYPE_CO2_A_DEF 0x00000E00 /* Sensor_Type: CO2 Sensor A Defined Level 1 */ 01533 01534 /* ------------------------------------------------------------------------------------------------------------------------- 01535 CORE_SENSOR_DETAILS[n] Pos/Masks Description 01536 ------------------------------------------------------------------------------------------------------------------------- */ 01537 #define BITP_CORE_SENSOR_DETAILS_COMPENSATION_DISABLE 31 /* Indicates Compensation Data Should Not Be Used */ 01538 #define BITP_CORE_SENSOR_DETAILS_RTD_CURVE 27 /* Select RTD Curve for Linearisation */ 01539 #define BITP_CORE_SENSOR_DETAILS_PGA_GAIN 24 /* PGA Gain */ 01540 #define BITP_CORE_SENSOR_DETAILS_REFERENCE_SELECT 20 /* Reference Selection */ 01541 #define BITP_CORE_SENSOR_DETAILS_DO_NOT_PUBLISH 17 /* Do Not Publish Channel Result */ 01542 #define BITP_CORE_SENSOR_DETAILS_LUT_SELECT 15 /* Lookup Table Select */ 01543 #define BITP_CORE_SENSOR_DETAILS_COMPENSATION_CHANNEL 4 /* Indicates Which Channel is Used to Compensate Sensor Result */ 01544 #define BITP_CORE_SENSOR_DETAILS_MEASUREMENT_UNITS 0 /* Units of Sensor Measurement */ 01545 #define BITM_CORE_SENSOR_DETAILS_COMPENSATION_DISABLE 0x80000000 /* Indicates Compensation Data Should Not Be Used */ 01546 #define BITM_CORE_SENSOR_DETAILS_RTD_CURVE 0x18000000 /* Select RTD Curve for Linearisation */ 01547 #define BITM_CORE_SENSOR_DETAILS_PGA_GAIN 0x07000000 /* PGA Gain */ 01548 #define BITM_CORE_SENSOR_DETAILS_REFERENCE_SELECT 0x00F00000 /* Reference Selection */ 01549 #define BITM_CORE_SENSOR_DETAILS_DO_NOT_PUBLISH 0x00020000 /* Do Not Publish Channel Result */ 01550 #define BITM_CORE_SENSOR_DETAILS_LUT_SELECT 0x00018000 /* Lookup Table Select */ 01551 #define BITM_CORE_SENSOR_DETAILS_COMPENSATION_CHANNEL 0x000000F0 /* Indicates Which Channel is Used to Compensate Sensor Result */ 01552 #define BITM_CORE_SENSOR_DETAILS_MEASUREMENT_UNITS 0x0000000F /* Units of Sensor Measurement */ 01553 #define ENUM_CORE_SENSOR_DETAILS_EUROPEAN_CURVE 0x00000000 /* RTD_Curve: European Curve */ 01554 #define ENUM_CORE_SENSOR_DETAILS_AMERICAN_CURVE 0x08000000 /* RTD_Curve: American Curve */ 01555 #define ENUM_CORE_SENSOR_DETAILS_JAPANESE_CURVE 0x10000000 /* RTD_Curve: Japanese Curve */ 01556 #define ENUM_CORE_SENSOR_DETAILS_ITS90_CURVE 0x18000000 /* RTD_Curve: ITS-90 Curve */ 01557 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_1 0x00000000 /* PGA_Gain: Gain of 1 */ 01558 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_2 0x01000000 /* PGA_Gain: Gain of 2 */ 01559 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_4 0x02000000 /* PGA_Gain: Gain of 4 */ 01560 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_8 0x03000000 /* PGA_Gain: Gain of 8 */ 01561 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_16 0x04000000 /* PGA_Gain: Gain of 16 */ 01562 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_32 0x05000000 /* PGA_Gain: Gain of 32 */ 01563 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_64 0x06000000 /* PGA_Gain: Gain of 64 */ 01564 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_128 0x07000000 /* PGA_Gain: Gain of 128 */ 01565 #define ENUM_CORE_SENSOR_DETAILS_REF_VINT 0x00000000 /* Reference_Select: Internal voltage reference (1.2V) */ 01566 #define ENUM_CORE_SENSOR_DETAILS_REF_VEXT1 0x00100000 /* Reference_Select: External Voltage reference applied to VERF+ and VREF- */ 01567 #define ENUM_CORE_SENSOR_DETAILS_REF_AVDD 0x00300000 /* Reference_Select: AVDD Supply Used as Excitation and Internally applied as Reference */ 01568 #define ENUM_CORE_SENSOR_DETAILS_LUT_DEFAULT 0x00000000 /* LUT_Select: Default Lookup Table for Selected Sensor Type */ 01569 #define ENUM_CORE_SENSOR_DETAILS_LUT_UNITY 0x00008000 /* LUT_Select: Unity Lookup Table. 1:1 Mapping From Input to Output */ 01570 #define ENUM_CORE_SENSOR_DETAILS_LUT_CUSTOM 0x00010000 /* LUT_Select: User Defined Custom Lookup Table. */ 01571 #define ENUM_CORE_SENSOR_DETAILS_LUT_RESERVED 0x00018000 /* LUT_Select: Reserved */ 01572 #define ENUM_CORE_SENSOR_DETAILS_UNITS_UNSPECIFIED 0x00000000 /* Measurement_Units: Not Specified */ 01573 #define ENUM_CORE_SENSOR_DETAILS_UNITS_RESERVED 0x00000001 /* Measurement_Units: Reserved */ 01574 #define ENUM_CORE_SENSOR_DETAILS_UNITS_DEGC 0x00000002 /* Measurement_Units: Degrees C */ 01575 #define ENUM_CORE_SENSOR_DETAILS_UNITS_DEGF 0x00000003 /* Measurement_Units: Degrees F */ 01576 01577 /* ------------------------------------------------------------------------------------------------------------------------- 01578 CORE_CHANNEL_EXCITATION[n] Pos/Masks Description 01579 ------------------------------------------------------------------------------------------------------------------------- */ 01580 #define BITP_CORE_CHANNEL_EXCITATION_IOUT_DIODE_RATIO 6 /* Modify Current Ratios Used for Diode Sensor */ 01581 #define BITP_CORE_CHANNEL_EXCITATION_IOUT_EXCITATION_CURRENT 0 /* Current Source Value */ 01582 #define BITM_CORE_CHANNEL_EXCITATION_IOUT_DIODE_RATIO 0x000001C0 /* Modify Current Ratios Used for Diode Sensor */ 01583 #define BITM_CORE_CHANNEL_EXCITATION_IOUT_EXCITATION_CURRENT 0x0000000F /* Current Source Value */ 01584 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_10UA_100UA 0x00000000 /* IOUT_Diode_Ratio: 2 Current measurement 10uA 100uA */ 01585 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_20UA_160UA 0x00000040 /* IOUT_Diode_Ratio: 2 Current measurement 20uA 160uA */ 01586 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_50UA_300UA 0x00000080 /* IOUT_Diode_Ratio: 2 Current measurement 50uA 300uA */ 01587 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_100UA_600UA 0x000000C0 /* IOUT_Diode_Ratio: 2 Current measurement 100uA 600uA */ 01588 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_10UA_50UA_100UA 0x00000100 /* IOUT_Diode_Ratio: 3 current measuremet 10uA 50uA 100uA */ 01589 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_20UA_100UA_160UA 0x00000140 /* IOUT_Diode_Ratio: 3 current measuremet 20uA 100uA 160uA */ 01590 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_50UA_150UA_300UA 0x00000180 /* IOUT_Diode_Ratio: 3 current measuremet 50uA 150uA 300uA */ 01591 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_100UA_300UA_600UA 0x000001C0 /* IOUT_Diode_Ratio: 3 current measuremet 100uA 300uA 600uA */ 01592 #define ENUM_CORE_CHANNEL_EXCITATION_EXTERNAL 0x00000000 /* IOUT_Excitation_Current: External Current Sourced */ 01593 #define ENUM_CORE_CHANNEL_EXCITATION_RESERVED 0x00000001 /* IOUT_Excitation_Current: Reserved */ 01594 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_10UA 0x00000002 /* IOUT_Excitation_Current: 10 \mu;A */ 01595 #define ENUM_CORE_CHANNEL_EXCITATION_RESERVED2 0x00000003 /* IOUT_Excitation_Current: Reserved */ 01596 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_50UA 0x00000004 /* IOUT_Excitation_Current: 50 \mu;A */ 01597 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_100UA 0x00000005 /* IOUT_Excitation_Current: 100 \mu;A */ 01598 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_250UA 0x00000006 /* IOUT_Excitation_Current: 250 \mu;A */ 01599 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_500UA 0x00000007 /* IOUT_Excitation_Current: 500 \mu;A */ 01600 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_1000UA 0x00000008 /* IOUT_Excitation_Current: 1000 \mu;A */ 01601 01602 /* ------------------------------------------------------------------------------------------------------------------------- 01603 CORE_SETTLING_TIME[n] Pos/Masks Description 01604 ------------------------------------------------------------------------------------------------------------------------- */ 01605 #define BITP_CORE_SETTLING_TIME_SETTLING_TIME_UNITS 14 /* Units for Settling Time */ 01606 #define BITP_CORE_SETTLING_TIME_SETTLING_TIME 0 /* Settling Time to Allow When Switching to Channel */ 01607 #define BITM_CORE_SETTLING_TIME_SETTLING_TIME_UNITS 0x0000C000 /* Units for Settling Time */ 01608 #define BITM_CORE_SETTLING_TIME_SETTLING_TIME 0x00003FFF /* Settling Time to Allow When Switching to Channel */ 01609 #define ENUM_CORE_SETTLING_TIME_MICROSECONDS 0x00000000 /* Settling_Time_Units: Micro-Seconds */ 01610 #define ENUM_CORE_SETTLING_TIME_MILLISECONDS 0x00004000 /* Settling_Time_Units: Milli-Seconds */ 01611 #define ENUM_CORE_SETTLING_TIME_SECONDS 0x00008000 /* Settling_Time_Units: Seconds */ 01612 01613 /* ------------------------------------------------------------------------------------------------------------------------- 01614 CORE_MEASUREMENT_SETUP[n] Pos/Masks Description 01615 ------------------------------------------------------------------------------------------------------------------------- */ 01616 #define BITP_CORE_MEASUREMENT_SETUP_BUFFER_BYPASS 15 /* Disable Buffers */ 01617 #define BITP_CORE_MEASUREMENT_SETUP_GND_SW 13 /* GND_SW */ 01618 #define BITP_CORE_MEASUREMENT_SETUP_ADC_FILTER_TYPE 12 /* ADC Digital Filter Type */ 01619 #define BITP_CORE_MEASUREMENT_SETUP_CHOP_MODE 10 /* Enabled and Disable Chop Mode */ 01620 #define BITP_CORE_MEASUREMENT_SETUP_PST_MEAS_EXC_CTRL 9 /* Disabled Current Sources After Measurement Has Been Complete */ 01621 #define BITP_CORE_MEASUREMENT_SETUP_NOTCH_EN_2 8 /* Enable Notch 2 Filter Mode */ 01622 #define BITP_CORE_MEASUREMENT_SETUP_CUSTOM_CALIBRATION 7 /* Enables Custom Calibration for Selected Sensor */ 01623 #define BITP_CORE_MEASUREMENT_SETUP_ADC_SF 0 /* ADC Digital Filter Select */ 01624 #define BITM_CORE_MEASUREMENT_SETUP_BUFFER_BYPASS 0x00008000 /* Disable Buffers */ 01625 #define BITM_CORE_MEASUREMENT_SETUP_GND_SW 0x00006000 /* GND_SW */ 01626 #define BITM_CORE_MEASUREMENT_SETUP_ADC_FILTER_TYPE 0x00001000 /* ADC Digital Filter Type */ 01627 #define BITM_CORE_MEASUREMENT_SETUP_CHOP_MODE 0x00000C00 /* Enabled and Disable Chop Mode */ 01628 #define BITM_CORE_MEASUREMENT_SETUP_PST_MEAS_EXC_CTRL 0x00000200 /* Disabled Current Sources After Measurement Has Been Complete */ 01629 #define BITM_CORE_MEASUREMENT_SETUP_NOTCH_EN_2 0x00000100 /* Enable Notch 2 Filter Mode */ 01630 #define BITM_CORE_MEASUREMENT_SETUP_CUSTOM_CALIBRATION 0x00000080 /* Enables Custom Calibration for Selected Sensor */ 01631 #define BITM_CORE_MEASUREMENT_SETUP_ADC_SF 0x0000007F /* ADC Digital Filter Select */ 01632 #define ENUM_CORE_MEASUREMENT_SETUP_BUFFERS_ENABLED 0x00000000 01633 #define ENUM_CORE_MEASUREMENT_SETUP_BUFFERS_DISABLED 0x00008000 01634 #define ENUM_CORE_MEASUREMENT_SETUP_GND_SW_OPEN 0x00000000 /* GND_SW: GND_SW Open. The GND SW is not enabled for the sensor measurement */ 01635 #define ENUM_CORE_MEASUREMENT_SETUP_GND_SW_CLOSED 0x00002000 /* GND_SW: GND_SW Closed. The GND SW is enabled for the sensor measurement, bit wiil Remain Closed After the Measurement */ 01636 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_SINC4 0x00000000 /* ADC_Filter_Type: Enabled SINC4 Filter */ 01637 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_SINC3 0x00001000 /* ADC_Filter_Type: Enabled SINC3 Filter */ 01638 #define ENUM_CORE_MEASUREMENT_SETUP_DISABLE_CHOP 0x00000000 /* Chop_Mode: Chop Mode Disabled */ 01639 #define ENUM_CORE_MEASUREMENT_SETUP_HW_CHOP 0x00000400 /* Chop_Mode: Chop Mode Enabled */ 01640 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_CHOP 0x00000800 /* Chop_Mode: Chop Mode Enabled */ 01641 #define ENUM_CORE_MEASUREMENT_SETUP_HW_SW_CHOP 0x00000C00 /* Chop_Mode: Chop Mode Enabled */ 01642 #define ENUM_CORE_MEASUREMENT_SETUP_POWERCYCLE 0x00000000 01643 #define ENUM_CORE_MEASUREMENT_SETUP_ALWAYSON 0x00000200 01644 #define ENUM_CORE_MEASUREMENT_SETUP_NOTCH_DIS 0x00000000 /* NOTCH_EN_2: Disable Notch Filter */ 01645 #define ENUM_CORE_MEASUREMENT_SETUP_NOTCH_EN 0x00000100 /* NOTCH_EN_2: Enable Notch 2 Filter option. Places a addtional notch at 1.2X ODR. Can be used for 50 and 60Hz rejection simultaneously */ 01646 #define ENUM_CORE_MEASUREMENT_SETUP_INTERNAL_CALIBRATION 0x00000000 01647 #define ENUM_CORE_MEASUREMENT_SETUP_CUSTOM_CALIBRATION 0x00000080 01648 01649 /* ------------------------------------------------------------------------------------------------------------------------- 01650 CORE_HIGH_THRESHOLD_LIMIT[n] Pos/Masks Description 01651 ------------------------------------------------------------------------------------------------------------------------- */ 01652 #define BITP_CORE_HIGH_THRESHOLD_LIMIT_HIGH_THRESHOLD 0 /* Upper Limit for Sensor Alert Comparison */ 01653 #define BITM_CORE_HIGH_THRESHOLD_LIMIT_HIGH_THRESHOLD 0x00000000 /* Upper Limit for Sensor Alert Comparison */ 01654 01655 /* ------------------------------------------------------------------------------------------------------------------------- 01656 CORE_LOW_THRESHOLD_LIMIT[n] Pos/Masks Description 01657 ------------------------------------------------------------------------------------------------------------------------- */ 01658 #define BITP_CORE_LOW_THRESHOLD_LIMIT_LOW_THRESHOLD 0 /* Lower Limit for Sensor Alert Comparison */ 01659 #define BITM_CORE_LOW_THRESHOLD_LIMIT_LOW_THRESHOLD 0x00000000 /* Lower Limit for Sensor Alert Comparison */ 01660 01661 /* ------------------------------------------------------------------------------------------------------------------------- 01662 CORE_SENSOR_OFFSET[n] Pos/Masks Description 01663 ------------------------------------------------------------------------------------------------------------------------- */ 01664 #define BITP_CORE_SENSOR_OFFSET_SENSOR_OFFSET 0 /* Sensor Offset Adjustment */ 01665 #define BITM_CORE_SENSOR_OFFSET_SENSOR_OFFSET 0x00000000 /* Sensor Offset Adjustment */ 01666 01667 /* ------------------------------------------------------------------------------------------------------------------------- 01668 CORE_SENSOR_GAIN[n] Pos/Masks Description 01669 ------------------------------------------------------------------------------------------------------------------------- */ 01670 #define BITP_CORE_SENSOR_GAIN_SENSOR_GAIN 0 /* Sensor Gain Adjustment */ 01671 #define BITM_CORE_SENSOR_GAIN_SENSOR_GAIN 0x00000000 /* Sensor Gain Adjustment */ 01672 01673 /* ------------------------------------------------------------------------------------------------------------------------- 01674 CORE_ALERT_CODE_CH[n] Pos/Masks Description 01675 ------------------------------------------------------------------------------------------------------------------------- */ 01676 #define BITP_CORE_ALERT_CODE_CH_ALERT_CODE_CH 0 /* Per-Channel Code Indicating Type of Alert */ 01677 #define BITM_CORE_ALERT_CODE_CH_ALERT_CODE_CH 0x0000FFFF /* Per-Channel Code Indicating Type of Alert */ 01678 01679 /* ------------------------------------------------------------------------------------------------------------------------- 01680 CORE_CHANNEL_SKIP[n] Pos/Masks Description 01681 ------------------------------------------------------------------------------------------------------------------------- */ 01682 #define BITP_CORE_CHANNEL_SKIP_CHANNEL_SKIP 0 /* Indicates If Channel Will Skip Some Measurement Cycles */ 01683 #define BITM_CORE_CHANNEL_SKIP_CHANNEL_SKIP 0x000000FF /* Indicates If Channel Will Skip Some Measurement Cycles */ 01684 01685 /* ------------------------------------------------------------------------------------------------------------------------- 01686 CORE_SENSOR_PARAMETER[n] Pos/Masks Description 01687 ------------------------------------------------------------------------------------------------------------------------- */ 01688 #define BITP_CORE_SENSOR_PARAMETER_SENSOR_PARAMETER 0 /* Sensor Parameter Adjustment */ 01689 #define BITM_CORE_SENSOR_PARAMETER_SENSOR_PARAMETER 0x00000000 /* Sensor Parameter Adjustment */ 01690 01691 /* ------------------------------------------------------------------------------------------------------------------------- 01692 CORE_CALIBRATION_PARAMETER[n] Pos/Masks Description 01693 ------------------------------------------------------------------------------------------------------------------------- */ 01694 #define BITP_CORE_CALIBRATION_PARAMETER_CALIBRATION_PARAMETER_ENABLE 24 /* Enables Use of Calibration_Parameter */ 01695 #define BITP_CORE_CALIBRATION_PARAMETER_CALIBRATION_PARAMETER 0 /* Calibration Parameter Value */ 01696 #define BITM_CORE_CALIBRATION_PARAMETER_CALIBRATION_PARAMETER_ENABLE 0x01000000 /* Enables Use of Calibration_Parameter */ 01697 #define BITM_CORE_CALIBRATION_PARAMETER_CALIBRATION_PARAMETER 0x00FFFFFF /* Calibration Parameter Value */ 01698 01699 /* ------------------------------------------------------------------------------------------------------------------------- 01700 CORE_DIGITAL_SENSOR_CONFIG[n] Pos/Masks Description 01701 ------------------------------------------------------------------------------------------------------------------------- */ 01702 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_DATA_BITS 11 /* Number of Relevant Data Bits */ 01703 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_READ_BYTES 8 /* Number of Bytes to Read from the Sensor */ 01704 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_BIT_OFFSET 4 /* Data Bit Offset, Relative to Alignment */ 01705 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LEFT_ALIGNED 3 /* Data Alignment Within the Data Frame */ 01706 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LITTLE_ENDIAN 2 /* Data Endianness of Sensor Result */ 01707 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_CODING 0 /* Data Encoding of Sensor Result */ 01708 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_DATA_BITS 0x0000F800 /* Number of Relevant Data Bits */ 01709 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_READ_BYTES 0x00000700 /* Number of Bytes to Read from the Sensor */ 01710 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_BIT_OFFSET 0x000000F0 /* Data Bit Offset, Relative to Alignment */ 01711 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LEFT_ALIGNED 0x00000008 /* Data Alignment Within the Data Frame */ 01712 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LITTLE_ENDIAN 0x00000004 /* Data Endianness of Sensor Result */ 01713 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_CODING 0x00000003 /* Data Encoding of Sensor Result */ 01714 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_NONE 0x00000000 /* Digital_Sensor_Coding: None/Invalid */ 01715 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_UNIPOLAR 0x00000001 /* Digital_Sensor_Coding: Unipolar */ 01716 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_TWOS_COMPL 0x00000002 /* Digital_Sensor_Coding: Twos Complement */ 01717 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_OFFSET_BINARY 0x00000003 /* Digital_Sensor_Coding: Offset Binary */ 01718 01719 /* ------------------------------------------------------------------------------------------------------------------------- 01720 CORE_DIGITAL_SENSOR_ADDRESS[n] Pos/Masks Description 01721 ------------------------------------------------------------------------------------------------------------------------- */ 01722 #define BITP_CORE_DIGITAL_SENSOR_ADDRESS_DIGITAL_SENSOR_ADDRESS 0 /* I2C Address or Write Address Command for SPI Sensor */ 01723 #define BITM_CORE_DIGITAL_SENSOR_ADDRESS_DIGITAL_SENSOR_ADDRESS 0x000000FF /* I2C Address or Write Address Command for SPI Sensor */ 01724 01725 /* ------------------------------------------------------------------------------------------------------------------------- 01726 CORE_DIGITAL_SENSOR_NUM_CMDS[n] Pos/Masks Description 01727 ------------------------------------------------------------------------------------------------------------------------- */ 01728 #define BITP_CORE_DIGITAL_SENSOR_NUM_CMDS_DIGITAL_SENSOR_NUM_READ_CMDS 4 /* Number of Read Commands for Digital Sensor */ 01729 #define BITP_CORE_DIGITAL_SENSOR_NUM_CMDS_DIGITAL_SENSOR_NUM_CFG_CMDS 0 /* Number of Configuration Commands for Digital Sensor */ 01730 #define BITM_CORE_DIGITAL_SENSOR_NUM_CMDS_DIGITAL_SENSOR_NUM_READ_CMDS 0x00000070 /* Number of Read Commands for Digital Sensor */ 01731 #define BITM_CORE_DIGITAL_SENSOR_NUM_CMDS_DIGITAL_SENSOR_NUM_CFG_CMDS 0x00000007 /* Number of Configuration Commands for Digital Sensor */ 01732 01733 /* ------------------------------------------------------------------------------------------------------------------------- 01734 CORE_DIGITAL_SENSOR_COMMS[n] Pos/Masks Description 01735 ------------------------------------------------------------------------------------------------------------------------- */ 01736 #define BITP_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE 10 /* Configuration for Sensor SPI Protocol */ 01737 #define BITP_CORE_DIGITAL_SENSOR_COMMS_I2C_CLOCK 5 /* Controls SCLK Frequency for I2C Sensors */ 01738 #define BITP_CORE_DIGITAL_SENSOR_COMMS_SPI_CLOCK 1 /* Controls Clock Frequency for SPI Sensors */ 01739 #define BITP_CORE_DIGITAL_SENSOR_COMMS_DIGITAL_SENSOR_COMMS_EN 0 /* Enable Digital Sensor Comms Register Parameters */ 01740 #define BITM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE 0x00000C00 /* Configuration for Sensor SPI Protocol */ 01741 #define BITM_CORE_DIGITAL_SENSOR_COMMS_I2C_CLOCK 0x00000060 /* Controls SCLK Frequency for I2C Sensors */ 01742 #define BITM_CORE_DIGITAL_SENSOR_COMMS_SPI_CLOCK 0x0000001E /* Controls Clock Frequency for SPI Sensors */ 01743 #define BITM_CORE_DIGITAL_SENSOR_COMMS_DIGITAL_SENSOR_COMMS_EN 0x00000001 /* Enable Digital Sensor Comms Register Parameters */ 01744 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_0 0x00000000 /* SPI_Mode: Clock Polarity = 0 Clock Phase = 0 */ 01745 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_1 0x00000400 /* SPI_Mode: Clock Polarity = 0 Clock Phase = 1 */ 01746 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_2 0x00000800 /* SPI_Mode: Clock Polarity = 1 Clock Phase = 0 */ 01747 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_3 0x00000C00 /* SPI_Mode: Clock Polarity = 1 Clock Phase = 1 */ 01748 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_100K 0x00000000 /* I2C_Clock: 100kHz SCL */ 01749 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_400K 0x00000020 /* I2C_Clock: 400kHz SCL */ 01750 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED1 0x00000040 /* I2C_Clock: Reserved */ 01751 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED2 0x00000060 /* I2C_Clock: Reserved */ 01752 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_8MHZ 0x00000000 /* SPI_Clock: 8MHz */ 01753 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_4MHZ 0x00000002 /* SPI_Clock: 4MHz */ 01754 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_2MHZ 0x00000004 /* SPI_Clock: 2MHz */ 01755 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_1MHZ 0x00000006 /* SPI_Clock: 1MHz */ 01756 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_500KHZ 0x00000008 /* SPI_Clock: 500kHz */ 01757 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_250KHZ 0x0000000A /* SPI_Clock: 250kHz */ 01758 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_125KHZ 0x0000000C /* SPI_Clock: 125kHz */ 01759 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_62P5KHZ 0x0000000E /* SPI_Clock: 62.5kHz */ 01760 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_31P3KHZ 0x00000010 /* SPI_Clock: 31.25kHz */ 01761 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_15P6KHZ 0x00000012 /* SPI_Clock: 15.625kHz */ 01762 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_7P8KHZ 0x00000014 /* SPI_Clock: 7.8kHz */ 01763 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_3P9KHZ 0x00000016 /* SPI_Clock: 3.9kHz */ 01764 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_1P9KHZ 0x00000018 /* SPI_Clock: 1.95kHz */ 01765 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_977HZ 0x0000001A /* SPI_Clock: 977Hz */ 01766 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_488HZ 0x0000001C /* SPI_Clock: 488Hz */ 01767 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_244HZ 0x0000001E /* SPI_Clock: 244Hz */ 01768 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_DIGITAL_COMMS_DEFAULT 0x00000000 /* Digital_Sensor_Comms_En: Default Parameters Used for Digital Sensor Communications */ 01769 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_DIGITAL_COMMS_USER 0x00000001 /* Digital_Sensor_Comms_En: User Supplied Parameters Used for Digital Sensor Communications */ 01770 01771 /* ------------------------------------------------------------------------------------------------------------------------- 01772 CORE_DIGITAL_SENSOR_COMMAND1[n] Pos/Masks Description 01773 ------------------------------------------------------------------------------------------------------------------------- */ 01774 #define BITP_CORE_DIGITAL_SENSOR_COMMAND1_DIGITAL_SENSOR_COMMAND1 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01775 #define BITM_CORE_DIGITAL_SENSOR_COMMAND1_DIGITAL_SENSOR_COMMAND1 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01776 01777 /* ------------------------------------------------------------------------------------------------------------------------- 01778 CORE_DIGITAL_SENSOR_COMMAND2[n] Pos/Masks Description 01779 ------------------------------------------------------------------------------------------------------------------------- */ 01780 #define BITP_CORE_DIGITAL_SENSOR_COMMAND2_DIGITAL_SENSOR_COMMAND2 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01781 #define BITM_CORE_DIGITAL_SENSOR_COMMAND2_DIGITAL_SENSOR_COMMAND2 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01782 01783 /* ------------------------------------------------------------------------------------------------------------------------- 01784 CORE_DIGITAL_SENSOR_COMMAND3[n] Pos/Masks Description 01785 ------------------------------------------------------------------------------------------------------------------------- */ 01786 #define BITP_CORE_DIGITAL_SENSOR_COMMAND3_DIGITAL_SENSOR_COMMAND3 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01787 #define BITM_CORE_DIGITAL_SENSOR_COMMAND3_DIGITAL_SENSOR_COMMAND3 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01788 01789 /* ------------------------------------------------------------------------------------------------------------------------- 01790 CORE_DIGITAL_SENSOR_COMMAND4[n] Pos/Masks Description 01791 ------------------------------------------------------------------------------------------------------------------------- */ 01792 #define BITP_CORE_DIGITAL_SENSOR_COMMAND4_DIGITAL_SENSOR_COMMAND4 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01793 #define BITM_CORE_DIGITAL_SENSOR_COMMAND4_DIGITAL_SENSOR_COMMAND4 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01794 01795 /* ------------------------------------------------------------------------------------------------------------------------- 01796 CORE_DIGITAL_SENSOR_COMMAND5[n] Pos/Masks Description 01797 ------------------------------------------------------------------------------------------------------------------------- */ 01798 #define BITP_CORE_DIGITAL_SENSOR_COMMAND5_DIGITAL_SENSOR_COMMAND5 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01799 #define BITM_CORE_DIGITAL_SENSOR_COMMAND5_DIGITAL_SENSOR_COMMAND5 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01800 01801 /* ------------------------------------------------------------------------------------------------------------------------- 01802 CORE_DIGITAL_SENSOR_COMMAND6[n] Pos/Masks Description 01803 ------------------------------------------------------------------------------------------------------------------------- */ 01804 #define BITP_CORE_DIGITAL_SENSOR_COMMAND6_DIGITAL_SENSOR_COMMAND6 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01805 #define BITM_CORE_DIGITAL_SENSOR_COMMAND6_DIGITAL_SENSOR_COMMAND6 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01806 01807 /* ------------------------------------------------------------------------------------------------------------------------- 01808 CORE_DIGITAL_SENSOR_COMMAND7[n] Pos/Masks Description 01809 ------------------------------------------------------------------------------------------------------------------------- */ 01810 #define BITP_CORE_DIGITAL_SENSOR_COMMAND7_DIGITAL_SENSOR_COMMAND7 0 /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01811 #define BITM_CORE_DIGITAL_SENSOR_COMMAND7_DIGITAL_SENSOR_COMMAND7 0x000000FF /* Configuration Command to Send to Digital I2C/SPI Sensor */ 01812 01813 /* ------------------------------------------------------------------------------------------------------------------------- 01814 CORE_DIGITAL_SENSOR_READ_CMD1[n] Pos/Masks Description 01815 ------------------------------------------------------------------------------------------------------------------------- */ 01816 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD1_DIGITAL_SENSOR_READ_CMD1 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01817 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD1_DIGITAL_SENSOR_READ_CMD1 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01818 01819 /* ------------------------------------------------------------------------------------------------------------------------- 01820 CORE_DIGITAL_SENSOR_READ_CMD2[n] Pos/Masks Description 01821 ------------------------------------------------------------------------------------------------------------------------- */ 01822 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD2_DIGITAL_SENSOR_READ_CMD2 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01823 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD2_DIGITAL_SENSOR_READ_CMD2 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01824 01825 /* ------------------------------------------------------------------------------------------------------------------------- 01826 CORE_DIGITAL_SENSOR_READ_CMD3[n] Pos/Masks Description 01827 ------------------------------------------------------------------------------------------------------------------------- */ 01828 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD3_DIGITAL_SENSOR_READ_CMD3 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01829 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD3_DIGITAL_SENSOR_READ_CMD3 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01830 01831 /* ------------------------------------------------------------------------------------------------------------------------- 01832 CORE_DIGITAL_SENSOR_READ_CMD4[n] Pos/Masks Description 01833 ------------------------------------------------------------------------------------------------------------------------- */ 01834 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD4_DIGITAL_SENSOR_READ_CMD4 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01835 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD4_DIGITAL_SENSOR_READ_CMD4 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01836 01837 /* ------------------------------------------------------------------------------------------------------------------------- 01838 CORE_DIGITAL_SENSOR_READ_CMD5[n] Pos/Masks Description 01839 ------------------------------------------------------------------------------------------------------------------------- */ 01840 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD5_DIGITAL_SENSOR_READ_CMD5 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01841 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD5_DIGITAL_SENSOR_READ_CMD5 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01842 01843 /* ------------------------------------------------------------------------------------------------------------------------- 01844 CORE_DIGITAL_SENSOR_READ_CMD6[n] Pos/Masks Description 01845 ------------------------------------------------------------------------------------------------------------------------- */ 01846 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD6_DIGITAL_SENSOR_READ_CMD6 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01847 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD6_DIGITAL_SENSOR_READ_CMD6 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01848 01849 /* ------------------------------------------------------------------------------------------------------------------------- 01850 CORE_DIGITAL_SENSOR_READ_CMD7[n] Pos/Masks Description 01851 ------------------------------------------------------------------------------------------------------------------------- */ 01852 #define BITP_CORE_DIGITAL_SENSOR_READ_CMD7_DIGITAL_SENSOR_READ_CMD7 0 /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01853 #define BITM_CORE_DIGITAL_SENSOR_READ_CMD7_DIGITAL_SENSOR_READ_CMD7 0x000000FF /* Per Conversion Command to Send to Digital I2C/SPI Sensor */ 01854 01855 01856 #endif /* end ifndef _DEF_ADMW1001_REGISTERS_H */
Generated on Wed Jul 13 2022 03:04:54 by
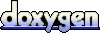