
PixArt Optical Track Sensor, OTS, demo program for P9126 sensor with library. Alternative porting style in C++. Initial release v1.0.
Dependencies: Pixart_OTS
Fork of OTS_P9126_Demo by
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 /* PAT9126JA: Optical Tracking Sensor. 00007 * By PixArt Imaging Inc. 00008 * Primary Engineer: Hill Chen (PixArt USA) 00009 * 00010 * License: Apache-2.0; http://www.apache.org/licenses/LICENSE-2.0 00011 */ 00012 00013 /* Demo Code Revision History 00014 * V1.0: March 6, 2019 00015 * First release. 00016 */ 00017 00018 #include "mbed.h" 00019 #include "Pixart_OTS.h" 00020 00021 Serial pc(USBTX, USBRX); 00022 I2C i2c(I2C_SDA0, I2C_SCL0); 00023 00024 static const Pixart_OTS_Model OTS_MODEL = PIXART_OTS_MODEL_9126; 00025 00026 int main() 00027 { 00028 pc.baud(115200); 00029 00030 Pixart_OTS *pixart_ots = create_pixart_ots(OTS_MODEL, pc, i2c); 00031 00032 if (!pixart_ots) 00033 { 00034 pc.printf("\r\n\n Not on library support list %d", OTS_MODEL); 00035 while (true); 00036 } 00037 00038 bool result = pixart_ots->sensor_init(); 00039 00040 if (result) 00041 { 00042 pc.printf("\r\n\n %s %s initialization successfully\r\n", PRODUCT, pixart_ots->get_model().c_str()); 00043 } 00044 else 00045 { 00046 pc.printf("\r\n\n %s %s fail on initialization", PRODUCT, pixart_ots->get_model().c_str()); 00047 while (true); 00048 } 00049 00050 while (true) { 00051 #ifndef USE_CALLBACK 00052 pixart_ots->periodic_callback(); 00053 wait_ms(250); 00054 #endif 00055 } 00056 } 00057
Generated on Mon Jul 18 2022 18:23:26 by
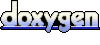