
Reference firmware for PixArt's ADBM-A350 sensor and evaluation board. "Hello World" and "Library" contain the exact same files. Please import just one of the two into your mBed compiler as a new program and not as a library.
main.cpp
00001 // ADBM-A350: Finger navigation chip. 00002 // Version: 1.1 00003 // Latest Revision Date: 18 July 2018 00004 // By PixArt Imaging Inc. 00005 // Primary Engineer: Vincent Yeh (PixArt USA) 00006 00007 // Copyright [2018] [Vincent Yeh] 00008 // Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with the License. You may obtain a copy of the License at: 00009 // http://www.apache.org/licenses/LICENSE-2.0 00010 00011 00012 /* 00013 //======================= 00014 //Revision History 00015 //======================= 00016 Version 1.1 -- 18 July 2018 00017 Added apache license notice. 00018 00019 Version 1.0 -- 14 June 2018 00020 First release. 00021 */ 00022 00023 #include "mbed.h" 00024 #include "registerArrays.h" 00025 //#include "I2CcommFunctions.h" 00026 #include "SPIcommFunctions.h" 00027 //Make sure you only have one of either SPIcommFunctions or I2CcommFunctions enabled. You cannot include both headers. 00028 00029 int main() 00030 { 00031 pc.baud(115200); // Set baud rate to 115200. Remember to sync serial terminal baud rate to the same value. 00032 00033 #ifdef SPImode 00034 IO_sel = 1; // Set IO_select pin to be HIGH for SPI. 00035 spi.format(8,3); // Set SPI to 8 bits with inverted polarity and phase-shifted to second edge. 00036 spi.frequency(100000); // Set frequency for SPI communication. 00037 cs = 1; // Initialize chip select as inactive. 00038 #endif 00039 00040 #ifdef I2Cmode 00041 IO_sel = 0; // Set IO_select pin to be LOW for I2C. 00042 i2c.frequency(400000); // Set frequency for I2C communication. 00043 cs = 1; // These two pins are used to determine the device's slave ID. 00044 MOSI = 1; 00045 #endif 00046 00047 shutdown = 0; 00048 writeRegister(0x3A, 0x5A); //Soft-reset the chip. 00049 00050 pc.printf("Program START\n\r"); 00051 00052 pc.printf("ID Check: %2X\n\r", readRegister(0x00)); //Checks product ID to make sure communication protocol is working properly. 00053 if(readRegister(0x00) != 0x88) 00054 { 00055 pc.printf("Communication protocol error! Terminating program.\n\r"); 00056 return 0; 00057 } 00058 00059 load(initialize, initialize_size); //Load register settings from the "initialize" array (see registerArrays.h) 00060 00061 while(1) 00062 { 00063 //pc.printf("MOTION bit: %2X\n\r", readRegister(0x02)); //Prints EVENT register for debugging. 00064 00065 if(readRegister(0x02) & 0x80) 00066 { 00067 grabData(); 00068 printData(); 00069 } 00070 } 00071 }
Generated on Sun Jul 17 2022 15:53:38 by
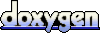