
Reference firmware for PixArt's ADBM-A350 sensor and evaluation board. "Hello World" and "Library" contain the exact same files. Please import just one of the two into your mBed compiler as a new program and not as a library.
I2CcommFunctions.h
00001 #define I2Cmode //Used to check if the sensor is in I2C mode or SPI mode. 00002 #define I2C_Slave_ID 0x57 //Slave ID is 0x57 only if p22 and p23 are both HIGH. 00003 00004 //========================================================================= 00005 //Communication pinouts for serial COM port, I2C, and interrupts 00006 //========================================================================= 00007 static Serial pc(USBTX, USBRX); //PC comm 00008 static I2C i2c(p26, p27); //SDA, SCL 00009 static DigitalOut cs(p22); //CS pin for selecting slave address 00010 static DigitalOut MOSI(p23); //MOSI pin for selecting slave address 00011 static DigitalOut shutdown(p20); //Shutdown pin 00012 static DigitalOut IO_sel(p19); //IO interface selection pin (I2C and SPI) 00013 00014 00015 //========================================================================= 00016 //Variables and arrays used for communications and data storage 00017 //========================================================================= 00018 int8_t deltaX, deltaY; //Stores the value of one individual motion report. 00019 int totalX, totalY = 0; //Stores the total deltaX and deltaY moved during runtime. 00020 00021 00022 //========================================================================= 00023 //Functions used to communicate with the sensor and grab/print data 00024 //========================================================================= 00025 uint8_t readRegister(uint8_t addr); 00026 //This function takes an 8-bit address in the form 0x00 and returns an 8-bit value in the form 0x00. 00027 00028 void writeRegister(uint8_t addr, uint8_t data); 00029 //This function takes an 8-bit address and 8-bit data. Writes the given data to the given address. 00030 00031 void load(const uint8_t array[][2], uint8_t arraySize); 00032 //Takes an array of registers/data (found in registerArrays.h) and their size and writes in all the values. 00033 00034 void grabData(void); 00035 //Grabs the deltaX and deltaY information from the proper registers and formats it into the proper format. 00036 00037 void printData(void); 00038 //Prints the data out to a serial terminal. 00039 00040 00041 00042 00043 00044 //========================================================================= 00045 //Functions definitions 00046 //========================================================================= 00047 uint8_t readRegister(uint8_t addr) 00048 { 00049 uint8_t data; 00050 i2c.write((I2C_Slave_ID << 1), (const char*)&addr, 1, 0); //Send the address to the chip 00051 i2c.read((I2C_Slave_ID << 1), (char*)&data, 1, 0); //Send the memory address where you want to store the read data 00052 return(data); 00053 } 00054 00055 00056 //========================================================================= 00057 void writeRegister(uint8_t addr, uint8_t data) 00058 { 00059 char data_write[2]; //Create an array to store the address/data to pass them at the same time. 00060 data_write[0] = addr; //Store the address in the first byte 00061 data_write[1] = data; //Store the data in the second byte 00062 i2c.write((I2C_Slave_ID << 1), data_write, 2, 0); //Send both over at once 00063 00064 pc.printf("R:%2X, D:%2X\n\r", addr, readRegister(addr)); 00065 //Uncomment this line for debugging. Prints every register write operation. 00066 } 00067 00068 00069 //========================================================================= 00070 void load(const uint8_t array[][2], uint8_t arraySize) 00071 { 00072 for(uint8_t q = 0; q < arraySize; q++) 00073 { 00074 writeRegister(array[q][0], array[q][1]); //Writes the given array of registers/data. 00075 } 00076 } 00077 00078 00079 //========================================================================= 00080 void grabData(void) 00081 { 00082 deltaX = readRegister(0x03); //Grabs data from the proper registers. 00083 deltaY = readRegister(0x04); 00084 writeRegister(0x02, 0x00); //Clear EVENT and motion registers. 00085 } 00086 00087 00088 //========================================================================= 00089 void printData(void) 00090 { 00091 if((deltaX != 0) || (deltaY != 0)) //If there is deltaX or deltaY movement, print the data. 00092 { 00093 totalX += deltaX; 00094 totalY += deltaY; 00095 00096 pc.printf("deltaX: %d\t\t\tdeltaY: %d\n\r", deltaX, deltaY); //Prints each individual count of deltaX and deltaY. 00097 pc.printf("X-axis Counts: %d\t\tY-axis Counts: %d\n\r", totalX, totalY); //Prints the total movement made during runtime. 00098 } 00099 00100 deltaX = 0; //Resets deltaX and Y values to zero, otherwise previous data is stored until overwritten. 00101 deltaY = 0; 00102 }
Generated on Sun Jul 17 2022 15:53:38 by
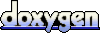