mbedos senza corrente
Embed:
(wiki syntax)
Show/hide line numbers
UARTSerial_half.h
00001 #ifndef MBED_UARTSERIAL_HALF_H 00002 00003 #define MBED_UARTSERIAL_HALF_H 00004 00005 #include "platform/platform.h" 00006 00007 #if (DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00008 00009 #include "platform/FileHandle.h" 00010 #include "SerialBase.h" 00011 #include "InterruptIn.h" 00012 #include "platform/PlatformMutex.h" 00013 #include "hal/serial_api.h" 00014 #include "platform/CircularBuffer.h" 00015 #include "platform/NonCopyable.h" 00016 00017 #include "mbed.h" 00018 00019 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 00020 #define MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE 256 00021 #endif 00022 00023 #ifndef MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 00024 #define MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE 256 00025 #endif 00026 00027 namespace mbed { 00028 00029 /** \addtogroup drivers */ 00030 00031 /** Class providing buffered UART communication functionality using separate circular buffer for send and receive channels 00032 * 00033 * @ingroup drivers 00034 */ 00035 00036 class UARTSerial_half : private SerialBase, public FileHandle, private NonCopyable<UARTSerial_half> { 00037 00038 public: 00039 00040 /** Create a UARTSerial_half port, connected to the specified transmit and receive pins, with a particular baud rate. 00041 * @param tx Transmit pin 00042 * @param rx Receive pin 00043 * @param baud The baud rate of the serial port (optional, defaults to MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE) 00044 */ 00045 UARTSerial_half(PinName tx, PinName rx, PinName dir, int UARTbaud = MBED_CONF_PLATFORM_DEFAULT_SERIAL_BAUD_RATE); 00046 virtual ~UARTSerial_half(); 00047 00048 /** Equivalent to POSIX poll(). Derived from FileHandle. 00049 * Provides a mechanism to multiplex input/output over a set of file handles. 00050 */ 00051 virtual short poll(short events) const; 00052 00053 /* Resolve ambiguities versus our private SerialBase 00054 * (for writable, spelling differs, but just in case) 00055 */ 00056 using FileHandle::readable; 00057 using FileHandle::writable; 00058 00059 /** Write the contents of a buffer to a file 00060 * 00061 * Follows POSIX semantics: 00062 * 00063 * * if blocking, block until all data is written 00064 * * if no data can be written, and non-blocking set, return -EAGAIN 00065 * * if some data can be written, and non-blocking set, write partial 00066 * 00067 * @param buffer The buffer to write from 00068 * @param length The number of bytes to write 00069 * @return The number of bytes written, negative error on failure 00070 */ 00071 virtual ssize_t write(const void *buffer, size_t length); 00072 00073 /** Read the contents of a file into a buffer 00074 * 00075 * Follows POSIX semantics: 00076 * 00077 * * if no data is available, and non-blocking set return -EAGAIN 00078 * * if no data is available, and blocking set, wait until data is available 00079 * * If any data is available, call returns immediately 00080 * 00081 * @param buffer The buffer to read in to 00082 * @param length The number of bytes to read 00083 * @return The number of bytes read, 0 at end of file, negative error on failure 00084 */ 00085 virtual ssize_t read(void *buffer, size_t length); 00086 virtual ssize_t read_timeout(void *buffer, size_t length, double _timeOut); 00087 00088 /** Close a file 00089 * 00090 * @return 0 on success, negative error code on failure 00091 */ 00092 virtual int close(); 00093 00094 /** Check if the file in an interactive terminal device 00095 * 00096 * @return True if the file is a terminal 00097 * @return False if the file is not a terminal 00098 * @return Negative error code on failure 00099 */ 00100 virtual int isatty(); 00101 00102 /** Move the file position to a given offset from from a given location 00103 * 00104 * Not valid for a device type FileHandle like UARTSerial_half. 00105 * In case of UARTSerial_half, returns ESPIPE 00106 * 00107 * @param offset The offset from whence to move to 00108 * @param whence The start of where to seek 00109 * SEEK_SET to start from beginning of file, 00110 * SEEK_CUR to start from current position in file, 00111 * SEEK_END to start from end of file 00112 * @return The new offset of the file, negative error code on failure 00113 */ 00114 virtual off_t seek(off_t offset, int whence); 00115 00116 00117 /** Flush any buffers associated with the file 00118 * 00119 * @return 0 on success, negative error code on failure 00120 */ 00121 virtual int sync(); 00122 00123 00124 /** Set blocking or non-blocking mode 00125 * The default is blocking. 00126 * 00127 * @param blocking true for blocking mode, false for non-blocking mode. 00128 */ 00129 virtual int flush(); 00130 00131 00132 /** Set blocking or non-blocking mode 00133 * The default is blocking. 00134 * 00135 * @param blocking true for blocking mode, false for non-blocking mode. 00136 */ 00137 virtual int set_blocking(bool blocking) 00138 { 00139 _blocking = blocking; 00140 return 0; 00141 } 00142 00143 00144 /** Check current blocking or non-blocking mode for file operations. 00145 * 00146 * @return true for blocking mode, false for non-blocking mode. 00147 */ 00148 virtual bool is_blocking() const 00149 { 00150 return _blocking; 00151 } 00152 00153 00154 /** Enable or disable input 00155 * 00156 * Control enabling of device for input. This is primarily intended 00157 * for temporary power-saving; the overall ability of the device to operate for 00158 * input and/or output may be fixed at creation time, but this call can 00159 * allow input to be temporarily disabled to permit power saving without 00160 * losing device state. 00161 * 00162 * @param enabled true to enable input, false to disable. 00163 * 00164 * @return 0 on success 00165 * @return Negative error code on failure 00166 */ 00167 virtual int enable_input(bool enabled); 00168 00169 00170 /** Enable or disable output 00171 * 00172 * Control enabling of device for output. This is primarily intended 00173 * for temporary power-saving; the overall ability of the device to operate for 00174 * input and/or output may be fixed at creation time, but this call can 00175 * allow output to be temporarily disabled to permit power saving without 00176 * losing device state. 00177 * 00178 * @param enabled true to enable output, false to disable. 00179 * 00180 * @return 0 on success 00181 * @return Negative error code on failure 00182 */ 00183 virtual int enable_output(bool enabled); 00184 00185 00186 /** Register a callback on state change of the file. 00187 * 00188 * The specified callback will be called on state changes such as when 00189 * the file can be written to or read from. 00190 * 00191 * The callback may be called in an interrupt context and should not 00192 * perform expensive operations. 00193 * 00194 * Note! This is not intended as an attach-like asynchronous api, but rather 00195 * as a building block for constructing such functionality. 00196 * 00197 * The exact timing of when the registered function 00198 * is called is not guaranteed and susceptible to change. It should be used 00199 * as a cue to make read/write/poll calls to find the current state. 00200 * 00201 * @param func Function to call on state change 00202 */ 00203 virtual void sigio(Callback<void()> func); 00204 00205 00206 /** Setup interrupt handler for DCD line 00207 * 00208 * If DCD line is connected, an IRQ handler will be setup. 00209 * Does nothing if DCD is NC, i.e., not connected. 00210 * 00211 * @param dcd_pin Pin-name for DCD 00212 * @param active_high a boolean set to true if DCD polarity is active low 00213 */ 00214 void set_data_carrier_detect(PinName dcd_pin, bool active_high = false); 00215 00216 00217 /** Set the baud rate 00218 * 00219 * @param baud The baud rate 00220 */ 00221 void set_baud(int UARTbaud); 00222 00223 00224 // Expose private SerialBase::Parity as UARTSerial_half::Parity 00225 using SerialBase::Parity; 00226 // In C++11, we wouldn't need to also have using directives for each value 00227 using SerialBase::None; 00228 using SerialBase::Odd; 00229 using SerialBase::Even; 00230 using SerialBase::Forced1; 00231 using SerialBase::Forced0; 00232 00233 00234 /** Set the transmission format used by the serial port 00235 * 00236 * @param bits The number of bits in a word (5-8; default = 8) 00237 * @param parity The parity used (None, Odd, Even, Forced1, Forced0; default = None) 00238 * @param stop_bits The number of stop bits (1 or 2; default = 1) 00239 */ 00240 void set_format(int bits = 8, Parity parity = UARTSerial_half::None, int stop_bits = 1); 00241 00242 00243 #if DEVICE_SERIAL_FC 00244 // For now use the base enum - but in future we may have extra options 00245 // such as XON/XOFF or manual GPIO RTSCTS. 00246 using SerialBase::Flow; 00247 // In C++11, we wouldn't need to also have using directives for each value 00248 using SerialBase::Disabled; 00249 using SerialBase::RTS; 00250 using SerialBase::CTS; 00251 using SerialBase::RTSCTS; 00252 00253 00254 /** Set the flow control type on the serial port 00255 * 00256 * @param type the flow control type (Disabled, RTS, CTS, RTSCTS) 00257 * @param flow1 the first flow control pin (RTS for RTS or RTSCTS, CTS for CTS) 00258 * @param flow2 the second flow control pin (CTS for RTSCTS) 00259 */ 00260 void set_flow_control(Flow type, PinName flow1 = NC, PinName flow2 = NC); 00261 #endif 00262 00263 00264 private: 00265 void wait_ms(uint32_t millisec); 00266 void wait_us(uint32_t microsec); 00267 00268 /** SerialBase lock override */ 00269 virtual void lock(void); 00270 00271 /** SerialBase unlock override */ 00272 virtual void unlock(void); 00273 00274 /** Acquire mutex */ 00275 virtual void api_lock(void); 00276 00277 /** Release mutex */ 00278 virtual void api_unlock(void); 00279 00280 /** Unbuffered write - invoked when write called from critical section */ 00281 ssize_t write_unbuffered(const char *buf_ptr, size_t length); 00282 00283 void enable_rx_irq(); 00284 void disable_rx_irq(); 00285 void enable_tx_irq(); 00286 void disable_tx_irq(); 00287 00288 00289 /** Software serial buffers 00290 * By default buffer size is 256 for TX and 256 for RX. Configurable through mbed_app.json 00291 */ 00292 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_RXBUF_SIZE> _rxbuf; 00293 CircularBuffer<char, MBED_CONF_DRIVERS_UART_SERIAL_TXBUF_SIZE> _txbuf; 00294 00295 PlatformMutex _mutex; 00296 00297 Callback<void()> _sigio_cb; 00298 00299 bool _blocking; 00300 bool _tx_irq_enabled; 00301 bool _rx_irq_enabled; 00302 bool _tx_enabled; 00303 bool _rx_enabled; 00304 int baudRate; 00305 InterruptIn *_dcd_irq; 00306 00307 00308 /** Device Hanged up 00309 * Determines if the device hanged up on us. 00310 * 00311 * @return True, if hanged up 00312 */ 00313 bool hup() const; 00314 00315 00316 /** ISRs for serial 00317 * Routines to handle interrupts on serial pins. 00318 * Copies data into Circular Buffer. 00319 * Reports the state change to File handle. 00320 */ 00321 void tx_irq(void); 00322 void rx_irq(void); 00323 00324 void wake(void); 00325 00326 void dcd_irq(void); 00327 DigitalOut *dirOut; 00328 00329 }; 00330 } //namespace mbed 00331 00332 #endif //(DEVICE_SERIAL && DEVICE_INTERRUPTIN) || defined(DOXYGEN_ONLY) 00333 #endif //MBED_UARTSERIAL_H
Generated on Mon Jul 18 2022 22:37:29 by
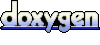