
POC1.5 prototype 2 x color sensor 2 x LM75B 3 x AnalogIn 1 x accel
LM75B Class Reference
NXP LM75B Digital temperature sensor and thermal watchdog. More...
#include <LM75B.h>
Public Member Functions | |
LM75B (I2C *i2c, int addr) | |
LM75B constructor. | |
~LM75B () | |
LM75B destructor. | |
int8_t | temp (void) |
get temperature as one byte (signed) | |
void | getTemp (float *temp) |
get temperature as 11 bit (float) | |
uint8_t | getConfig (uint8_t ptr_byte) |
get configuration register | |
int | setConfig (uint8_t ptr_byte, uint8_t config_data) |
set configuration register |
Detailed Description
NXP LM75B Digital temperature sensor and thermal watchdog.
#include "mbed.h" #include "LM75B.h" #define LM75B_I2C_ADDRESS (0x48) #if defined (TARGET_KL25Z) #define PIN_SCL PTE1 #define PIN_SDA PTE0 #elif defined (TARGET_KL46Z) #define PIN_SCL PTE1 #define PIN_SDA PTE0 #elif defined (TARGET_K64F) #define PIN_SCL PTE24 #define PIN_SDA PTE25 #elif defined (TARGET_K22F) #define PIN_SCL PTE1 #define PIN_SDA PTE0 #elif defined (TARGET_KL05Z) #define PIN_SCL PTB3 #define PIN_SDA PTB4 #elif defined (TARGET_NUCLEO_F411RE) #define PIN_SCL PB_8 #define PIN_SDA PB_9 #else #error TARGET NOT DEFINED #endif int main() { int8_t itemp = 0 ; float ftemp = 0.0 ; LM75B lm75b(PIN_SDA, PIN_SCL, LM75B_I2C_ADDRESS) ; while(1) { itemp = lm75b.temp() ; lm75b.getTemp(&ftemp) ; printf("Temp = %d C degree, %.3f C degree\n", itemp, ftemp) ; wait(1) ; } }
Definition at line 50 of file LM75B.h.
Constructor & Destructor Documentation
LM75B | ( | I2C * | i2c, |
int | addr | ||
) |
Member Function Documentation
uint8_t getConfig | ( | uint8_t | ptr_byte ) |
void getTemp | ( | float * | temp ) |
int setConfig | ( | uint8_t | ptr_byte, |
uint8_t | config_data | ||
) |
Generated on Tue Jul 12 2022 21:13:41 by
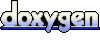