Library to handle SpaceBall, SpaceMouse and SpaceOrb on serial port. Gets access to 3D rotation and translation vector as well as button status. (USB is not supported)
SpaceBall Class Reference
SpaceBall class, based on serial connection. More...
#include <SpaceBall.h>
Public Member Functions | |
SpaceBall (PinName tx, PinName rx, bool bSpaceOrb=false) | |
Create a input object connected to the specified serial pin. | |
~SpaceBall () | |
destructor | |
void | Init () |
initializing the connection to the device | |
void | SetMapping (int mapping) |
Set mapping mode for function GetAxis() to adapt coordinate system. | |
void | GetTranslation (float *fValue[3]) const |
Gets the translation axis (push, pull) as 3D vector. | |
void | GetRotation (float *fValue[3]) const |
Gets the rotation axis as 3D vector. | |
float | GetAxis (int nAxis) const |
Gets a single axis value. | |
int | GetAxisRaw (int nAxis) const |
Gets a single axis value. | |
float | operator[] (eSpaceBallAxis nAxis) const |
Gets a single axis value as [] operator. | |
float | operator[] (int nAxis) const |
Gets a single axis value as [] operator. | |
int | GetButtons () const |
Gets the button states as an bit-combination. | |
operator int (void) const | |
Gets the button states as an bit-combination as int operator. | |
void | SetScale (float fScale=1.0f) |
Sets the additional scaling factor for function GetAxis() | |
float | GetScale (void) |
Gets the additional scaling factor for function GetAxis() |
Detailed Description
SpaceBall class, based on serial connection.
Library to handle SpaceBall, SpaceMouse and SpaceOrb on serial port. Gets access to 3D rotation and translation vector as well as button status
Supported devices:
- Spaceball 2003A
- Spaceball 2003B
- Spaceball 2003 FLX
- Spaceball 3003
- Spaceball 3003 FLX
- Spaceball 4000 FLX
- SpaceMouse
- SpaceOrb (Note: Flag has to be set in constructor)
Note: USB or wireless devices are NOT supported by this class
Serial connection (D-Sub 9p male):
- 3 <--- RS232 Driver <--- mbed TX
- 2 ---> RS232 Driver ---> mbed RX
- 5 ----| GND
- 4 <--- Power +9...+12 volt
- 7 <--- Power +9...+12 volt
Example: (illuminates LEDs dependent on force at the Spaceball)
#include "mbed.h" #include "SpaceBall.h" PwmOut led[] = {(LED1), (LED2), (LED3), (LED4) }; SpaceBall SBall(p9, p10); // tx, rx, bSOrb int main() { SBall.Init(); while(1) { led[0] = abs( SBall[TX] ) + abs( SBall[TY] ) + abs( SBall[TZ] ); led[1] = abs( SBall[RX] ); led[2] = abs( SBall[RY] ); led[3] = abs( SBall[RZ] ); wait_us(500); } }
Definition at line 118 of file SpaceBall.h.
Constructor & Destructor Documentation
SpaceBall | ( | PinName | tx, |
PinName | rx, | ||
bool | bSpaceOrb = false |
||
) |
Create a input object connected to the specified serial pin.
- Parameters:
-
tx Serial TX pin to connect to rx Serial RX pin to connect to bSpaceOrb Flag to select device. false = SpaceBall and SpaceMouse. true = SpaceOrb
Definition at line 8 of file SpaceBall.cpp.
~SpaceBall | ( | ) |
destructor
Definition at line 130 of file SpaceBall.h.
Member Function Documentation
float GetAxis | ( | int | nAxis ) | const |
Gets a single axis value.
Coordinate system is mapped according function SetMapping()
- Parameters:
-
nAxis Axis index/name
- Returns:
- Axis value as scaled float
Definition at line 75 of file SpaceBall.cpp.
int GetAxisRaw | ( | int | nAxis ) | const |
Gets a single axis value.
Coordinate system is mapped according function SetMapping()
- Parameters:
-
nAxis Axis index/name
- Returns:
- Axis value as unscaled int (as provided by the SpaceBall)
Definition at line 117 of file SpaceBall.cpp.
int GetButtons | ( | ) | const |
Gets the button states as an bit-combination.
See definitions of Spaceball Button bit-masks
- Returns:
- Buttons bit-combination
Definition at line 204 of file SpaceBall.h.
void GetRotation | ( | float * | fValue[3] ) | const |
Gets the rotation axis as 3D vector.
- Parameters:
-
fValue[] Return vector with Pitch, Roll and Yaw
Definition at line 159 of file SpaceBall.h.
float GetScale | ( | void | ) |
Gets the additional scaling factor for function GetAxis()
- Returns:
- Scaling factor
Definition at line 227 of file SpaceBall.h.
void GetTranslation | ( | float * | fValue[3] ) | const |
Gets the translation axis (push, pull) as 3D vector.
- Parameters:
-
fValue[] Return vector with X, Y and Z
Definition at line 148 of file SpaceBall.h.
void Init | ( | ) |
initializing the connection to the device
Definition at line 41 of file SpaceBall.cpp.
operator int | ( | void | ) | const |
Gets the button states as an bit-combination as int operator.
See definitions of Spaceball Button bit-masks
Usage: int b = spaceball;
- Returns:
- Buttons bit-combination
Definition at line 213 of file SpaceBall.h.
float operator[] | ( | int | nAxis ) | const |
Gets a single axis value as [] operator.
Coordinate system is mapped according function SetMapping()
Usage: float x = spaceball[3];
- Parameters:
-
nAxis Axis index/name
- Returns:
- Axis value as scaled float
Definition at line 197 of file SpaceBall.h.
float operator[] | ( | eSpaceBallAxis | nAxis ) | const |
Gets a single axis value as [] operator.
Coordinate system is mapped according function SetMapping()
Usage: float x = spaceball[RX];
- Parameters:
-
nAxis Axis index/name
- Returns:
- Axis value as scaled float
Definition at line 187 of file SpaceBall.h.
void SetMapping | ( | int | mapping ) |
Set mapping mode for function GetAxis() to adapt coordinate system.
- Parameters:
-
mapping Mapping mode
Definition at line 141 of file SpaceBall.h.
void SetScale | ( | float | fScale = 1.0f ) |
Sets the additional scaling factor for function GetAxis()
- Parameters:
-
fScale Scaling factor
Definition at line 220 of file SpaceBall.h.
Generated on Tue Jul 12 2022 23:18:04 by
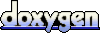