
NuMaker NuWicam sample on NUC472
Dependencies: Modbus nvt_rs485
main.cpp
00001 /* 00002 * The program is a sample code. 00003 * It needs run with NuMaker NuWicam board. 00004 */ 00005 00006 /* ----------------------- System includes --------------------------------*/ 00007 #include "mbed.h" 00008 #include "rtos.h" 00009 /*----------------------- Modbus includes ----------------------------------*/ 00010 #include "mb.h" 00011 #include "mbport.h" 00012 00013 /* ----------------------- Defines ------------------------------------------*/ 00014 // Sharing buffer index 00015 enum { 00016 eData_MBInCounter, 00017 eData_MBOutCounter, 00018 eData_MBError, 00019 eData_DI, 00020 eData_DO, 00021 eData_RGB, 00022 eData_MBResistorVar, 00023 eData_TemperatureSensor, 00024 eData_Cnt 00025 } E_DATA_TYPE; 00026 00027 #define REG_INPUT_START 1 00028 #define REG_INPUT_NREGS eData_Cnt 00029 #define SLAVE_ID 0x01 00030 /* ----------------------- Static variables ---------------------------------*/ 00031 static USHORT usRegInputStart = REG_INPUT_START; 00032 static USHORT usRegInputBuf[REG_INPUT_NREGS]; 00033 00034 DigitalOut led1(LED1); // For temperature worker. 00035 DigitalOut led2(LED2); // For Modbus worker. 00036 DigitalOut led3(LED3); // For Holder CB 00037 00038 AnalogIn LM35(A0); 00039 00040 #define DEF_LED_NUM 6 00041 #if defined(TARGET_NUMAKER_PFM_NUC472) 00042 DigitalOut LED[DEF_LED_NUM] = { PF_9, PF_10, PC_10, PC_11, PA_10, PA_9 } ; 00043 #elif defined(TARGET_NUMAKER_PFM_M453) 00044 DigitalOut LED[DEF_LED_NUM] = { PC_6, PC_7, PC_11, PC_12, PC_13, PC_14 } ; 00045 #elif defined(TARGET_NUMAKER_PFM_M487) 00046 DigitalOut LED[DEF_LED_NUM] = { PH_9, PH_8, PB_9, PF_11, PG_4, PC_11 } ; 00047 #endif 00048 00049 void light_leds() 00050 { 00051 int i=0; 00052 USHORT usOutValue = ~usRegInputBuf[eData_DO]; 00053 for ( i=0; i<DEF_LED_NUM ; i++) 00054 LED[i].write( (usOutValue&(0x1<<i)) >> i ); 00055 } 00056 00057 void get_temp(void) 00058 { 00059 float tempC, a[10], avg; 00060 int i; 00061 #define DEF_ADC_READTIMES 10 00062 avg=0; 00063 for(i=0;i<DEF_ADC_READTIMES;i++) 00064 { 00065 a[i] = LM35.read(); 00066 Thread::wait(1); 00067 } 00068 00069 for ( i=0; i<DEF_ADC_READTIMES; i++ ) 00070 avg += a[i]; 00071 00072 avg /= DEF_ADC_READTIMES; 00073 tempC=(avg*3.685503686*100); 00074 usRegInputBuf[eData_TemperatureSensor] = (USHORT)tempC; 00075 //printf("[%s %d] %f %d\r\n", __func__, __LINE__, avg, usRegInputBuf[eData_TemperatureSensor] ); 00076 } 00077 00078 void worker_get_temperature(void const *args) 00079 { 00080 // Poll temperature sensor per 1 second. 00081 while (true) { 00082 get_temp(); 00083 led1 = !led1; 00084 Thread::wait(1000); 00085 } 00086 } 00087 00088 #if 0 00089 void worker_uart(void const *args) 00090 { 00091 // For UART-SERIAL Tx/Rx Service. 00092 while (true) 00093 xMBPortSerialPolling(); 00094 } 00095 #endif 00096 00097 /* ----------------------- Start implementation -----------------------------*/ 00098 int 00099 main( void ) 00100 { 00101 eMBErrorCode eStatus; 00102 Thread temperature_thread(worker_get_temperature); 00103 //Thread uart_thread(worker_uart); 00104 00105 // Initialise some registers 00106 for (int i=0; i<REG_INPUT_NREGS; i++) 00107 usRegInputBuf[i] = 0x0; 00108 00109 light_leds(); // Control LEDs 00110 00111 /* Enable the Modbus Protocol Stack. */ 00112 if ( (eStatus = eMBInit( MB_RTU, SLAVE_ID, 0, 115200, MB_PAR_NONE )) != MB_ENOERR ) 00113 goto FAIL_MB; 00114 else if ( (eStatus = eMBEnable( ) ) != MB_ENOERR ) 00115 goto FAIL_MB_1; 00116 else { 00117 for( ;; ) 00118 { 00119 xMBPortSerialPolling(); 00120 if ( eMBPoll( ) != MB_ENOERR ) break; 00121 } 00122 } 00123 00124 FAIL_MB_1: 00125 eMBClose(); 00126 00127 FAIL_MB: 00128 for( ;; ) 00129 { 00130 led2 = !led2; 00131 Thread::wait(200); 00132 } 00133 } 00134 00135 00136 00137 00138 00139 00140 00141 00142 00143 00144 00145 00146 00147 eMBErrorCode 00148 eMBRegInputCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs ) 00149 { 00150 eMBErrorCode eStatus = MB_ENOERR; 00151 int iRegIndex; 00152 00153 if( ( usAddress >= REG_INPUT_START ) 00154 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00155 { 00156 iRegIndex = ( int )( usAddress - usRegInputStart ); 00157 while( usNRegs > 0 ) 00158 { 00159 *pucRegBuffer++ = 00160 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00161 *pucRegBuffer++ = 00162 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00163 iRegIndex++; 00164 usNRegs--; 00165 } 00166 } 00167 else 00168 { 00169 eStatus = MB_ENOREG; 00170 } 00171 00172 return eStatus; 00173 } 00174 00175 eMBErrorCode 00176 eMBRegHoldingCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNRegs, eMBRegisterMode eMode ) 00177 { 00178 eMBErrorCode eStatus = MB_ENOERR; 00179 int iRegIndex; 00180 00181 usRegInputBuf[eData_MBInCounter]++; 00182 usRegInputBuf[eData_MBOutCounter]++; 00183 00184 if (eMode == MB_REG_READ) 00185 { 00186 if( ( usAddress >= REG_INPUT_START ) 00187 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00188 { 00189 iRegIndex = ( int )( usAddress - usRegInputStart ); 00190 while( usNRegs > 0 ) 00191 { 00192 *pucRegBuffer++ = 00193 ( unsigned char )( usRegInputBuf[iRegIndex] >> 8 ); 00194 *pucRegBuffer++ = 00195 ( unsigned char )( usRegInputBuf[iRegIndex] & 0xFF ); 00196 iRegIndex++; 00197 usNRegs--; 00198 } 00199 } 00200 } 00201 00202 if (eMode == MB_REG_WRITE) 00203 { 00204 if( ( usAddress >= REG_INPUT_START ) 00205 && ( usAddress + usNRegs <= REG_INPUT_START + REG_INPUT_NREGS ) ) 00206 { 00207 iRegIndex = ( int )( usAddress - usRegInputStart ); 00208 while( usNRegs > 0 ) 00209 { 00210 usRegInputBuf[iRegIndex] = ((unsigned int) *pucRegBuffer << 8) | ((unsigned int) *(pucRegBuffer+1)); 00211 pucRegBuffer+=2; 00212 iRegIndex++; 00213 usNRegs--; 00214 } 00215 light_leds(); // Control LEDs 00216 } 00217 } 00218 00219 led3=!led3; 00220 00221 return eStatus; 00222 } 00223 00224 00225 eMBErrorCode 00226 eMBRegCoilsCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNCoils, 00227 eMBRegisterMode eMode ) 00228 { 00229 return MB_ENOREG; 00230 } 00231 00232 eMBErrorCode 00233 eMBRegDiscreteCB( UCHAR * pucRegBuffer, USHORT usAddress, USHORT usNDiscrete ) 00234 { 00235 return MB_ENOREG; 00236 }
Generated on Thu Jul 14 2022 03:54:53 by
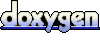