
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #include "GUI.h" 00004 #include "WM.h" 00005 #include "FRAMEWIN.h" 00006 00007 #include "TouchPanel.h" 00008 // 00009 // Enable emWin touch feature after calibration 00010 // 00011 volatile int g_enable_Touch; 00012 // 00013 // For touch sampling timer 00014 // 00015 Ticker s_ticker_emWinloop; 00016 // 00017 // emWin timer count 00018 // 00019 extern GUI_TIMER_TIME OS_TimeMS; 00020 // 00021 // Tick loop for emWin 00022 // 00023 void task_emWinloop(void) 00024 { 00025 OS_TimeMS++; 00026 } 00027 // 00028 // loop for emWin touch 00029 // 00030 Thread touch_thread; 00031 00032 void gui_touch_thread() 00033 { 00034 while (true) 00035 { 00036 #if GUI_SUPPORT_TOUCH 00037 if ( g_enable_Touch == 1 ) 00038 { 00039 GUI_TOUCH_Exec(); 00040 } 00041 #endif 00042 ThisThread::sleep_for(20); 00043 } 00044 } 00045 // 00046 // Extern emWin GUI layout 00047 // 00048 extern WM_HWIN CreateFramewin(void); 00049 // 00050 // emWin MainTask to create a GUI layout 00051 // 00052 void MainTask(void) 00053 { 00054 WM_HWIN hWin; 00055 char acVersion[40] = "Framewin: Version of emWin: "; 00056 00057 printf("Main Task -> \n"); 00058 00059 strcat(acVersion, GUI_GetVersionString()); 00060 hWin = CreateFramewin(); 00061 FRAMEWIN_SetText(hWin, acVersion); 00062 while (1) 00063 { 00064 GUI_Delay(500); 00065 } 00066 } 00067 // 00068 // Main 00069 // 00070 int main(void) 00071 { 00072 #ifdef MBED_MAJOR_VERSION 00073 printf("Mbed OS version %d.%d.%d\r\n\n", MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00074 #endif 00075 // 00076 // Before touch calibration 00077 // 00078 g_enable_Touch = 0; 00079 // 00080 // Attach Mbed tick 00081 // 00082 s_ticker_emWinloop.attach(&task_emWinloop, 0.001); 00083 00084 #if GUI_SUPPORT_TOUCH 00085 // 00086 // Init emWin and touch calibration 00087 // 00088 GUI_Init(); 00089 Init_TouchPanel(); 00090 ts_calibrate(__DEMO_TS_WIDTH__, __DEMO_TS_HEIGHT__); 00091 #endif 00092 // 00093 // After touch calibration 00094 // 00095 g_enable_Touch = 1; 00096 touch_thread.start(gui_touch_thread); 00097 // 00098 // Create GUI layout 00099 // 00100 MainTask(); 00101 00102 while(1); 00103 }
Generated on Mon Mar 4 2024 07:48:13 by
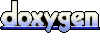