
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
fbutils.c
00001 /* 00002 * fbutils.c 00003 * 00004 * Utility routines for framebuffer interaction 00005 * 00006 * Copyright 2002 Russell King and Doug Lowder 00007 * 00008 * This file is placed under the GPL. Please see the 00009 * file COPYING for details. 00010 * 00011 */ 00012 #if 0 00013 //#include "config.h" 00014 00015 #include <stdio.h> 00016 #include <stdlib.h> 00017 #include <string.h> 00018 //#include <unistd.h> 00019 //#include <sys/fcntl.h> 00020 //#include <sys/ioctl.h> 00021 //#include <sys/mman.h> 00022 //#include <sys/time.h> 00023 #include "fbutils.h" 00024 //#include "wbtypes.h" 00025 //#include "LCDconf.h" 00026 #include "TouchPanel.h" 00027 #include "GUI.h" 00028 #if 0 00029 union multiptr 00030 { 00031 unsigned char *p8; 00032 unsigned short *p16; 00033 unsigned long *p32; 00034 }; 00035 #endif 00036 //static int con_fd, fb_fd, last_vt = -1; 00037 //static unsigned char *line_addr; 00038 //static int fb_fd=0; 00039 static int bytes_per_pixel=2; 00040 static unsigned colormap [13]; 00041 static unsigned colormap2[13]; 00042 unsigned int xres=__DEMO_TS_WIDTH__, yres=__DEMO_TS_HEIGHT__; 00043 00044 int red_length = 5; 00045 int green_length = 6; 00046 int blue_length = 5; 00047 00048 int red_offset = 11; 00049 int green_offset = 5; 00050 int blue_offset = 0; 00051 00052 //extern void * g_VAFrameBuf; 00053 00054 void line(int x1, int y1, int x2, int y2, unsigned colidx); 00055 00056 void put_cross(int x, int y, unsigned colidx) 00057 { 00058 line (x - 10, y, x - 2, y, colidx); 00059 line (x + 2, y, x + 10, y, colidx); 00060 line (x, y - 10, x, y - 2, colidx); 00061 line (x, y + 2, x, y + 10, colidx); 00062 00063 #if 1 00064 line (x - 6, y - 9, x - 9, y - 9, colidx + 1); 00065 line (x - 9, y - 8, x - 9, y - 6, colidx + 1); 00066 line (x - 9, y + 6, x - 9, y + 9, colidx + 1); 00067 line (x - 8, y + 9, x - 6, y + 9, colidx + 1); 00068 line (x + 6, y + 9, x + 9, y + 9, colidx + 1); 00069 line (x + 9, y + 8, x + 9, y + 6, colidx + 1); 00070 line (x + 9, y - 6, x + 9, y - 9, colidx + 1); 00071 line (x + 8, y - 9, x + 6, y - 9, colidx + 1); 00072 #else 00073 line (x - 7, y - 7, x - 4, y - 4, colidx + 1); 00074 line (x - 7, y + 7, x - 4, y + 4, colidx + 1); 00075 line (x + 4, y - 4, x + 7, y - 7, colidx + 1); 00076 line (x + 4, y + 4, x + 7, y + 7, colidx + 1); 00077 #endif 00078 } 00079 #if 0 00080 void put_char(int x, int y, int c, int colidx) 00081 { 00082 int i,j,bits; 00083 00084 for (i = 0; i < font_vga_8x8.height; i++) 00085 { 00086 bits = font_vga_8x8.data [font_vga_8x8.height * c + i]; 00087 for (j = 0; j < font_vga_8x8.width; j++, bits <<= 1) 00088 if (bits & 0x80) 00089 pixel (x + j, y + i, colidx); 00090 } 00091 } 00092 00093 void put_string(int x, int y, char *s, unsigned colidx) 00094 { 00095 int i; 00096 for (i = 0; *s; i++, x += font_vga_8x8.width, s++) 00097 put_char (x, y, *s, colidx); 00098 } 00099 00100 void put_string_center(int x, int y, char *s, unsigned colidx) 00101 { 00102 size_t sl = strlen (s); 00103 put_string (x - (sl / 2) * font_vga_8x8.width, 00104 y - font_vga_8x8.height / 2, s, colidx); 00105 } 00106 #endif 00107 void setcolor(unsigned colidx, unsigned value) 00108 { 00109 unsigned res; 00110 //unsigned short red, green, blue; 00111 // struct fb_cmap cmap; 00112 00113 #ifdef DEBUG 00114 if (colidx > 255) 00115 { 00116 fprintf (stderr, "WARNING: color index = %u, must be <256\n", 00117 colidx); 00118 return; 00119 } 00120 #endif 00121 00122 switch (bytes_per_pixel) 00123 { 00124 default: 00125 case 1: 00126 #if 0 00127 res = colidx; 00128 red = (value >> 8) & 0xff00; 00129 green = value & 0xff00; 00130 blue = (value << 8) & 0xff00; 00131 cmap.start = colidx; 00132 cmap.len = 1; 00133 cmap.red = &red; 00134 cmap.green = &green; 00135 cmap.blue = &blue; 00136 cmap.transp = NULL; 00137 #endif 00138 break; 00139 case 2: 00140 #if 0 00141 red = (value >> 16) & 0xff; 00142 green = (value >> 8) & 0xff; 00143 blue = value & 0xff; 00144 res = ((red >> (8 - red_length)) << red_offset) | 00145 ((green >> (8 - green_length)) << green_offset) | 00146 ((blue >> (8 - blue_length)) << blue_offset); 00147 #endif 00148 case 4: 00149 res = value; 00150 } 00151 colormap [colidx] = value; 00152 GUI_SetColor(value); 00153 GUI_DrawPixel(0, 0); 00154 res = GUI_GetPixelIndex(0, 0); 00155 colormap2[colidx] = res; 00156 GUI_SetColor(0x00); 00157 GUI_DrawPixel(0, 0); 00158 } 00159 #if 0 00160 static void __setpixel (union multiptr loc, unsigned xormode, unsigned color) 00161 { 00162 switch(bytes_per_pixel) 00163 { 00164 case 1: 00165 default: 00166 if (xormode) 00167 *loc.p8 ^= color; 00168 else 00169 *loc.p8 = color; 00170 break; 00171 case 2: 00172 if (xormode) 00173 *loc.p16 ^= color; 00174 else 00175 *loc.p16 = color; 00176 break; 00177 case 4: 00178 if (xormode) 00179 *loc.p32 ^= color; 00180 else 00181 *loc.p32 = color; 00182 break; 00183 } 00184 } 00185 #endif 00186 void pixel (int x, int y, unsigned colidx) 00187 { 00188 unsigned xormode; 00189 unsigned color, color2; 00190 //union multiptr loc; 00191 00192 if ((x < 0) || (x >= __DEMO_TS_WIDTH__) || 00193 (y < 0) || (y >= __DEMO_TS_HEIGHT__)) 00194 return; 00195 00196 xormode = colidx & XORMODE; 00197 colidx &= ~XORMODE; 00198 00199 #ifdef DEBUG 00200 if (colidx > 255) 00201 { 00202 fprintf (stderr, "WARNING: color value = %u, must be <256\n", 00203 colidx); 00204 return; 00205 } 00206 #endif 00207 00208 // loc.p8 = line_addr [y] + x * bytes_per_pixel; 00209 // line_addr = (unsigned char *)g_VAFrameBuf+ y*(LCD_XSIZE*bytes_per_pixel); 00210 color = GUI_GetPixelIndex(x, y); 00211 if ( color == colormap2[0] ) 00212 color = colormap[0]; 00213 else if ( color == colormap2[1] ) 00214 color = colormap[1]; 00215 else if ( color == colormap2[2] ) 00216 color = colormap[2]; 00217 else if ( color == colormap2[3] ) 00218 color = colormap[3]; 00219 else if ( color == colormap2[4] ) 00220 color = colormap[4]; 00221 else if ( color == colormap2[5] ) 00222 color = colormap[5]; 00223 else if ( color == colormap2[6] ) 00224 color = colormap[6]; 00225 else if ( color == colormap2[7] ) 00226 color = colormap[7]; 00227 else if ( color == colormap2[8] ) 00228 color = colormap[8]; 00229 else if ( color == colormap2[9] ) 00230 color = colormap[9]; 00231 else if ( color == colormap2[10] ) 00232 color = colormap[10]; 00233 else if ( color == colormap2[11] ) 00234 color = colormap[11]; 00235 else if ( color == colormap2[12] ) 00236 color = colormap[12]; 00237 color2 = colormap [colidx]; 00238 if (xormode) 00239 color ^= color2; 00240 else 00241 color = color2; 00242 GUI_SetColor(color); 00243 GUI_DrawPixel(x, y); 00244 00245 00246 //loc.p8 = line_addr + x*bytes_per_pixel; 00247 //__setpixel(loc, xormode, colormap [colidx]); 00248 } 00249 00250 void line(int x1, int y1, int x2, int y2, unsigned colidx) 00251 { 00252 int tmp; 00253 int dx = x2 - x1; 00254 int dy = y2 - y1; 00255 00256 if (abs (dx) < abs (dy)) 00257 { 00258 if (y1 > y2) 00259 { 00260 tmp = x1; 00261 x1 = x2; 00262 x2 = tmp; 00263 tmp = y1; 00264 y1 = y2; 00265 y2 = tmp; 00266 dx = -dx; 00267 dy = -dy; 00268 } 00269 x1 <<= 16; 00270 /* dy is apriori >0 */ 00271 dx = (dx << 16) / dy; 00272 while (y1 <= y2) 00273 { 00274 pixel (x1 >> 16, y1, colidx); 00275 x1 += dx; 00276 y1++; 00277 } 00278 } 00279 else 00280 { 00281 if (x1 > x2) 00282 { 00283 tmp = x1; 00284 x1 = x2; 00285 x2 = tmp; 00286 tmp = y1; 00287 y1 = y2; 00288 y2 = tmp; 00289 dx = -dx; 00290 dy = -dy; 00291 } 00292 y1 <<= 16; 00293 dy = dx ? (dy << 16) / dx : 0; 00294 while (x1 <= x2) 00295 { 00296 pixel (x1, y1 >> 16, colidx); 00297 y1 += dy; 00298 x1++; 00299 } 00300 } 00301 } 00302 #if 1 00303 void rect (int x1, int y1, int x2, int y2, unsigned colidx) 00304 { 00305 line (x1, y1, x2, y1, colidx); 00306 line (x2, y1, x2, y2, colidx); 00307 line (x2, y2, x1, y2, colidx); 00308 line (x1, y2, x1, y1, colidx); 00309 } 00310 00311 void fillrect (int x1, int y1, int x2, int y2, unsigned colidx) 00312 { 00313 int tmp; 00314 unsigned xormode; 00315 unsigned color, color2; 00316 //union multiptr loc; 00317 00318 /* Clipping and sanity checking */ 00319 if (x1 > x2) 00320 { 00321 tmp = x1; 00322 x1 = x2; 00323 x2 = tmp; 00324 } 00325 if (y1 > y2) 00326 { 00327 tmp = y1; 00328 y1 = y2; 00329 y2 = tmp; 00330 } 00331 if (x1 < 0) x1 = 0; 00332 if (x1 >= xres) x1 = xres - 1; 00333 if (x2 < 0) x2 = 0; 00334 if (x2 >= xres) x2 = xres - 1; 00335 if (y1 < 0) y1 = 0; 00336 if (y1 >= yres) y1 = yres - 1; 00337 if (y2 < 0) y2 = 0; 00338 if (y2 >= yres) y2 = yres - 1; 00339 00340 if ((x1 > x2) || (y1 > y2)) 00341 return; 00342 00343 xormode = colidx & XORMODE; 00344 colidx &= ~XORMODE; 00345 00346 #ifdef DEBUG 00347 if (colidx > 255) 00348 { 00349 fprintf (stderr, "WARNING: color value = %u, must be <256\n", 00350 colidx); 00351 return; 00352 } 00353 #endif 00354 00355 color2 = colormap [colidx]; 00356 00357 for (; y1 <= y2; y1++) 00358 { 00359 // loc.p8 = line_addr [y1] + x1 * bytes_per_pixel; 00360 //line_addr = (unsigned char *)g_VAFrameBuf+ y1*(LCD_XSIZE*bytes_per_pixel); 00361 //loc.p8 = line_addr + x1* bytes_per_pixel; 00362 for (tmp = x1; tmp <= x2; tmp++) 00363 { 00364 //__setpixel (loc, xormode, colidx); 00365 //loc.p8 += bytes_per_pixel; 00366 color = GUI_GetPixelIndex(tmp, y1); 00367 if ( color == colormap2[0] ) 00368 color = colormap[0]; 00369 else if ( color == colormap2[1] ) 00370 color = colormap[1]; 00371 else if ( color == colormap2[2] ) 00372 color = colormap[2]; 00373 else if ( color == colormap2[3] ) 00374 color = colormap[3]; 00375 else if ( color == colormap2[4] ) 00376 color = colormap[4]; 00377 else if ( color == colormap2[5] ) 00378 color = colormap[5]; 00379 else if ( color == colormap2[6] ) 00380 color = colormap[6]; 00381 else if ( color == colormap2[7] ) 00382 color = colormap[7]; 00383 else if ( color == colormap2[8] ) 00384 color = colormap[8]; 00385 else if ( color == colormap2[9] ) 00386 color = colormap[9]; 00387 else if ( color == colormap2[10] ) 00388 color = colormap[10]; 00389 else if ( color == colormap2[11] ) 00390 color = colormap[11]; 00391 else if ( color == colormap2[12] ) 00392 color = colormap[12]; 00393 color2 = colormap [colidx]; 00394 if (xormode) 00395 color ^= color2; 00396 else 00397 color = color2; 00398 GUI_SetColor(color); 00399 GUI_DrawPixel(tmp, y1); 00400 } 00401 } 00402 } 00403 #endif 00404 #endif
Generated on Mon Mar 4 2024 07:48:13 by
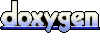