
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
WM_Intern.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : WM_Intern.h 00040 Purpose : Windows manager internal include 00041 ---------------------------------------------------------------------- 00042 */ 00043 00044 #ifndef WM_INTERN_H /* Make sure we only include it once */ 00045 #define WM_INTERN_H /* Make sure we only include it once */ 00046 00047 #include "WM.h" 00048 #include "GUI_Private.h" 00049 00050 00051 #if defined(__cplusplus) 00052 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00053 #endif 00054 00055 #if GUI_WINSUPPORT 00056 00057 /********************************************************************* 00058 * 00059 * defines 00060 * 00061 ********************************************************************** 00062 00063 The following could be placed in a file of its own as it is not 00064 used outside of the window manager 00065 00066 */ 00067 /* Basic Windows status flags. 00068 For module-internally use only ! 00069 */ 00070 #define WM_SF_HASTRANS WM_CF_HASTRANS 00071 #define WM_SF_MEMDEV WM_CF_MEMDEV 00072 #define WM_SF_MEMDEV_ON_REDRAW WM_CF_MEMDEV_ON_REDRAW 00073 #define WM_SF_DISABLED WM_CF_DISABLED /* Disabled: Does not receive PID (mouse & touch) input */ 00074 #define WM_SF_ISVIS WM_CF_SHOW /* Is visible flag */ 00075 00076 #define WM_SF_STAYONTOP WM_CF_STAYONTOP 00077 #define WM_SF_LATE_CLIP WM_CF_LATE_CLIP 00078 #define WM_SF_ANCHOR_RIGHT WM_CF_ANCHOR_RIGHT 00079 #define WM_SF_ANCHOR_BOTTOM WM_CF_ANCHOR_BOTTOM 00080 #define WM_SF_ANCHOR_LEFT WM_CF_ANCHOR_LEFT 00081 #define WM_SF_ANCHOR_TOP WM_CF_ANCHOR_TOP 00082 00083 #define WM_SF_INVALID WM_CF_ACTIVATE /* We reuse this flag, as it is create only and Invalid is status only */ 00084 00085 #define WM_SF_CONST_OUTLINE WM_CF_CONST_OUTLINE /* Constant outline.*/ 00086 00087 #define WM_H2P(hWin) ((WM_Obj*)GUI_ALLOC_h2p(hWin)) 00088 00089 00090 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_LOG_WARNINGS 00091 #define WM_ASSERT_NOT_IN_PAINT() { if (WM__PaintCallbackCnt) \ 00092 GUI_DEBUG_ERROROUT("Function may not be called from within a paint event"); \ 00093 } 00094 #else 00095 #define WM_ASSERT_NOT_IN_PAINT() 00096 #endif 00097 00098 /********************************************************************* 00099 * 00100 * Data types & structures 00101 * 00102 ********************************************************************** 00103 */ 00104 typedef struct { 00105 WM_HWIN hOld; 00106 WM_HWIN hNew; 00107 } WM_NOTIFY_CHILD_HAS_FOCUS_INFO; 00108 00109 typedef struct WM_CRITICAL_HANDLE { 00110 struct WM_CRITICAL_HANDLE * pNext; 00111 volatile WM_HWIN hWin; 00112 } WM_CRITICAL_HANDLE; 00113 00114 /********************************************************************* 00115 * 00116 * Data (extern) 00117 * 00118 ********************************************************************** 00119 */ 00120 extern U32 WM__CreateFlags; 00121 extern WM_HWIN WM__ahCapture[GUI_NUM_LAYERS]; 00122 extern WM_HWIN WM__ahWinFocus[GUI_NUM_LAYERS]; 00123 extern char WM__CaptureReleaseAuto; 00124 extern WM_tfPollPID * WM_pfPollPID; 00125 extern U8 WM__PaintCallbackCnt; /* Public for assertions only */ 00126 extern WM_HWIN WM__hCreateStatic; 00127 00128 #if WM_SUPPORT_TRANSPARENCY 00129 extern int WM__TransWindowCnt; 00130 extern WM_HWIN WM__hATransWindow; 00131 #endif 00132 00133 #if WM_SUPPORT_DIAG 00134 extern void (*WM__pfShowInvalid)(WM_HWIN hWin); 00135 #endif 00136 00137 extern WM_CRITICAL_HANDLE WM__aCHWinModal[GUI_NUM_LAYERS]; 00138 extern WM_CRITICAL_HANDLE WM__aCHWinLast[GUI_NUM_LAYERS]; 00139 extern int WM__ModalLayer; 00140 00141 #if GUI_SUPPORT_MOUSE 00142 extern WM_CRITICAL_HANDLE WM__aCHWinMouseOver[GUI_NUM_LAYERS]; 00143 #endif 00144 00145 #ifdef WM_C 00146 #define GUI_EXTERN 00147 #else 00148 #define GUI_EXTERN extern 00149 #endif 00150 00151 #if (GUI_NUM_LAYERS > 1) 00152 GUI_EXTERN U32 WM__InvalidLayerMask; 00153 GUI_EXTERN unsigned WM__TouchedLayer; 00154 #define WM__TOUCHED_LAYER WM__TouchedLayer 00155 #else 00156 #define WM__TOUCHED_LAYER GUI_CURSOR_LAYER 00157 #endif 00158 00159 GUI_EXTERN U16 WM__NumWindows; 00160 GUI_EXTERN U16 WM__NumInvalidWindows; 00161 GUI_EXTERN WM_HWIN WM__FirstWin; 00162 GUI_EXTERN WM_CRITICAL_HANDLE * WM__pFirstCriticalHandle; 00163 00164 GUI_EXTERN WM_HWIN WM__ahDesktopWin[GUI_NUM_LAYERS]; 00165 GUI_EXTERN GUI_COLOR WM__aBkColor[GUI_NUM_LAYERS]; 00166 00167 #undef GUI_EXTERN 00168 00169 /********************************************************************* 00170 * 00171 * Prototypes 00172 * 00173 ********************************************************************** 00174 */ 00175 void WM__ActivateClipRect (void); 00176 int WM__ClipAtParentBorders (GUI_RECT * pRect, WM_HWIN hWin); 00177 void WM__Client2Screen (const WM_Obj * pWin, GUI_RECT * pRect); 00178 void WM__DeleteAssocTimer (WM_HWIN hWin); 00179 void WM__DeleteSecure (WM_HWIN hWin); 00180 void WM__DetachWindow (WM_HWIN hChild); 00181 void WM__ForEachDesc (WM_HWIN hWin, WM_tfForEach * pcb, void * pData); 00182 void WM__GetClientRectWin (const WM_Obj * pWin, GUI_RECT * pRect); 00183 void WM__GetClientRectEx (WM_HWIN hWin, GUI_RECT * pRect); 00184 WM_HWIN WM__GetFirstSibling (WM_HWIN hWin); 00185 WM_HWIN WM__GetFocusedChild (WM_HWIN hWin); 00186 int WM__GetHasFocus (WM_HWIN hWin); 00187 WM_HWIN WM__GetLastSibling (WM_HWIN hWin); 00188 WM_HWIN WM__GetPrevSibling (WM_HWIN hWin); 00189 int WM__GetTopLevelLayer (WM_HWIN hWin); 00190 int WM__GetWindowSizeX (const WM_Obj * pWin); 00191 int WM__GetWindowSizeY (const WM_Obj * pWin); 00192 void WM__InsertWindowIntoList (WM_HWIN hWin, WM_HWIN hParent); 00193 void WM__Invalidate1Abs (WM_HWIN hWin, const GUI_RECT * pRect); 00194 void WM__InvalidateAreaBelow (const GUI_RECT * pRect, WM_HWIN StopWin); 00195 void WM__InvalidateRectEx (const GUI_RECT * pInvalidRect, WM_HWIN hParent, WM_HWIN hStop); 00196 void WM__InvalidateTransAreaAbove(const GUI_RECT * pRect, WM_HWIN StopWin); 00197 int WM__IntersectRect (GUI_RECT * pDest, const GUI_RECT * pr0, const GUI_RECT * pr1); 00198 int WM__IsAncestor (WM_HWIN hChild, WM_HWIN hParent); 00199 int WM__IsAncestorOrSelf (WM_HWIN hChild, WM_HWIN hParent); 00200 int WM__IsChild (WM_HWIN hWin, WM_HWIN hParent); 00201 int WM__IsEnabled (WM_HWIN hWin); 00202 int WM__IsInModalArea (WM_HWIN hWin); 00203 int WM__IsInWindow (WM_Obj * pWin, int x, int y); 00204 int WM__IsWindow (WM_HWIN hWin); 00205 void WM__LeaveIVRSearch (void); 00206 void WM__MoveTo (WM_HWIN hWin, int x, int y); 00207 void WM__MoveWindow (WM_HWIN hWin, int dx, int dy); 00208 void WM__NotifyVisChanged (WM_HWIN hWin, GUI_RECT * pRect); 00209 int WM__RectIsNZ (const GUI_RECT * pr); 00210 void WM__RemoveWindowFromList (WM_HWIN hWin); 00211 void WM__Screen2Client (const WM_Obj * pWin, GUI_RECT * pRect); 00212 void WM__SelectTopLevelLayer (WM_HWIN hWin); 00213 void WM__SendMsgNoData (WM_HWIN hWin, U8 MsgId); 00214 void WM__SendMessage (WM_HWIN hWin, WM_MESSAGE * pm); 00215 void WM__SendMessageIfEnabled (WM_HWIN hWin, WM_MESSAGE * pm); 00216 void WM__SendMessageNoPara (WM_HWIN hWin, int MsgId); 00217 void WM__SendPIDMessage (WM_HWIN hWin, WM_MESSAGE * pMsg); 00218 int WM__SetScrollbarH (WM_HWIN hWin, int OnOff); 00219 int WM__SetScrollbarV (WM_HWIN hWin, int OnOff); 00220 void WM__UpdateChildPositions (WM_Obj * pObj, int dx0, int dy0, int dx1, int dy1); 00221 void WM_PID__GetPrevState (GUI_PID_STATE * pPrevState, int Layer); 00222 void WM_PID__SetPrevState (GUI_PID_STATE * pPrevState, int Layer); 00223 void WM__SendTouchMessage (WM_HWIN hWin, WM_MESSAGE * pMsg); 00224 00225 U16 WM_GetFlags (WM_HWIN hWin); 00226 int WM__Paint (WM_HWIN hWin); 00227 void WM__Paint1 (WM_HWIN hWin); 00228 void WM__AddCriticalHandle (WM_CRITICAL_HANDLE * pCH); 00229 void WM__RemoveCriticalHandle (WM_CRITICAL_HANDLE * pCH); 00230 void WM__SetLastTouched (WM_HWIN hWin); 00231 00232 #if WM_SUPPORT_STATIC_MEMDEV 00233 void WM__InvalidateDrawAndDescs(WM_HWIN hWin); 00234 #else 00235 #define WM__InvalidateDrawAndDescs(hWin) 00236 #endif 00237 00238 /* Static memory devices */ 00239 #if (GUI_SUPPORT_MEMDEV) 00240 typedef struct { 00241 int xSize, ySize; // Size of bk window 00242 } EFFECT_CONTEXT; 00243 00244 int GUI_MEMDEV__CalcParaFadeIn (int Period, int TimeUsed); 00245 void GUI_MEMDEV__ClipBK (EFFECT_CONTEXT * pContext); 00246 void GUI_MEMDEV__RemoveStaticDevice(WM_HWIN hWin); 00247 void GUI_MEMDEV__UndoClipBK (EFFECT_CONTEXT * pContext); 00248 #endif 00249 00250 void WM__InvalidateParent(const GUI_RECT * pInvalidRect, WM_HWIN hParent, WM_HWIN hStop); 00251 void WM__InvalidateRect (const GUI_RECT * pInvalidRect, WM_HWIN hParent, WM_HWIN hStop, U16 Flags); 00252 00253 WM_tfInvalidateParent WM__SetInvalidateParentFunc(WM_tfInvalidateParent pfInvalidateParentFunc); 00254 WM_tfInvalidateDrawFunc WM__SetInvalidateDrawFunc (WM_tfInvalidateDrawFunc pfInvalidateDrawFunc); 00255 WM_tfPaint1Func WM__SetPaint1Func (WM_tfPaint1Func pfPaint1Func); 00256 00257 #endif /* GUI_WINSUPPORT */ 00258 00259 #if defined(__cplusplus) 00260 } 00261 #endif 00262 00263 #endif /* WM_INTERN_H */ 00264 00265 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
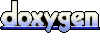