
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
TREEVIEW_Private.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : TREEVIEW_Private.h 00040 Purpose : TREEVIEW private header file 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef TREEVIEW_PRIVATE_H 00045 #define TREEVIEW_PRIVATE_H 00046 00047 #include "WM.h" 00048 #include "TREEVIEW.h" 00049 00050 #if GUI_WINSUPPORT 00051 00052 /********************************************************************* 00053 * 00054 * Defines 00055 * 00056 ********************************************************************** 00057 */ 00058 #define TREEVIEW_ITEM_STATE_EXPANDED (1 << 1) 00059 #define TREEVIEW_ITEM_STATE_ISLAST (1 << 2) 00060 00061 #define TREEVIEW_STATE_HASLINES (1 << 0) 00062 00063 #define TREEVIEW_COLORS_BK 0 00064 #define TREEVIEW_COLORS_TEXT 1 00065 #define TREEVIEW_COLORS_LINE 2 00066 00067 /********************************************************************* 00068 * 00069 * Types 00070 * 00071 ********************************************************************** 00072 */ 00073 typedef struct { 00074 const GUI_BITMAP * apBm[3]; /* Closed, Open, Leaf */ 00075 } TREEVIEW_ITEM_DATA; 00076 00077 typedef struct { 00078 WM_HMEM hParent; /* Handle to treeview object */ 00079 TREEVIEW_ITEM_Handle hNext; 00080 TREEVIEW_ITEM_Handle hPrev; 00081 U32 UserData; 00082 WM_HMEM hData; /* Handle of TREEVIEW_ITEM_DATA structure */ 00083 U16 xSize; 00084 U16 ySize; 00085 U16 Flags; 00086 U16 Connectors; 00087 U8 Level; /* 0...15 */ 00088 char acText[1]; 00089 } TREEVIEW_ITEM_OBJ; 00090 00091 typedef struct { 00092 const GUI_FONT * pFont; 00093 GUI_COLOR aBkColor[3]; 00094 GUI_COLOR aTextColor[3]; 00095 GUI_COLOR aLineColor[3]; 00096 GUI_COLOR FocusColor; 00097 const GUI_BITMAP * apBm[5]; /* Closed, Open, Leaf, Plus, Minus */ 00098 int Indent; 00099 int TextIndent; 00100 int MinItemHeight; 00101 } TREEVIEW_PROPS; 00102 00103 typedef struct { 00104 WIDGET Widget; 00105 WIDGET_DRAW_ITEM_FUNC * pfDrawItem; 00106 WM_SCROLL_STATE ScrollStateV; 00107 WM_SCROLL_STATE ScrollStateH; 00108 TREEVIEW_PROPS Props; 00109 U16 Flags; 00110 TREEVIEW_ITEM_Handle hFirst; 00111 TREEVIEW_ITEM_Handle hLast; 00112 TREEVIEW_ITEM_Handle hSel; 00113 GUI_TIMER_HANDLE hTimer; 00114 /* Cache variables */ 00115 int NumItems; 00116 int NumVisItems; 00117 int xSizeItems; /* xSize in pixel used for all visible items */ 00118 int ySizeItems; /* ySize in pixel used for all visible items */ 00119 I16 xOffPM, yOffPM; /* x/y offset of PM bitmap */ 00120 U16 xOverlapHLine; 00121 } TREEVIEW_OBJ; 00122 00123 /********************************************************************* 00124 * 00125 * Macros for internal use 00126 * 00127 ********************************************************************** 00128 */ 00129 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00130 #define TREEVIEW_INIT_ID(p) p->Widget.DebugId = TREEVIEW_ID 00131 #else 00132 #define TREEVIEW_INIT_ID(p) 00133 #endif 00134 00135 #if GUI_DEBUG_LEVEL >= GUI_DEBUG_LEVEL_CHECK_ALL 00136 TREEVIEW_OBJ * TREEVIEW_LockH(TREEVIEW_Handle h); 00137 #define TREEVIEW_LOCK_H(h) TREEVIEW_LockH(h) 00138 #else 00139 #define TREEVIEW_LOCK_H(h) (TREEVIEW_OBJ *)GUI_LOCK_H(h) 00140 #endif 00141 00142 /********************************************************************* 00143 * 00144 * Module internal data 00145 * 00146 ********************************************************************** 00147 */ 00148 extern TREEVIEW_PROPS TREEVIEW__DefaultProps; 00149 extern GUI_CONST_STORAGE GUI_BITMAP TREEVIEW__bmOpen; 00150 extern GUI_CONST_STORAGE GUI_BITMAP TREEVIEW__bmClosed; 00151 extern GUI_CONST_STORAGE GUI_BITMAP TREEVIEW__bmLeaf; 00152 extern GUI_CONST_STORAGE GUI_BITMAP TREEVIEW__bmPlus; 00153 extern GUI_CONST_STORAGE GUI_BITMAP TREEVIEW__bmMinus; 00154 00155 #endif /* GUI_WINSUPPORT */ 00156 #endif /* TREEVIEW_H */ 00157 00158 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
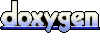