
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
SCROLLBAR.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : SCROLLBAR.h 00040 Purpose : SCROLLBAR include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef SCROLLBAR_H 00045 #define SCROLLBAR_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "WIDGET.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * Defines 00060 * 00061 ********************************************************************** 00062 */ 00063 #define SCROLLBAR_CI_THUMB 0 00064 #define SCROLLBAR_CI_SHAFT 1 00065 #define SCROLLBAR_CI_ARROW 2 00066 00067 /********************************************************************* 00068 * 00069 * States 00070 */ 00071 #define SCROLLBAR_STATE_PRESSED WIDGET_STATE_USER0 00072 00073 /********************************************************************* 00074 * 00075 * Create / Status flags 00076 */ 00077 #define SCROLLBAR_CF_VERTICAL WIDGET_CF_VERTICAL 00078 #define SCROLLBAR_CF_FOCUSABLE WIDGET_STATE_FOCUSABLE 00079 00080 #define SCROLLBAR_CF_FOCUSSABLE SCROLLBAR_CF_FOCUSABLE 00081 00082 /************************************************************ 00083 * 00084 * Skinning property indices 00085 */ 00086 #define SCROLLBAR_SKINFLEX_PI_PRESSED 0 00087 #define SCROLLBAR_SKINFLEX_PI_UNPRESSED 1 00088 00089 /********************************************************************* 00090 * 00091 * Public Types 00092 * 00093 ********************************************************************** 00094 */ 00095 typedef WM_HMEM SCROLLBAR_Handle; 00096 00097 typedef struct { 00098 GUI_COLOR aColorFrame[3]; 00099 GUI_COLOR aColorUpper[2]; 00100 GUI_COLOR aColorLower[2]; 00101 GUI_COLOR aColorShaft[2]; 00102 GUI_COLOR ColorArrow; 00103 GUI_COLOR ColorGrasp; 00104 } SCROLLBAR_SKINFLEX_PROPS; 00105 00106 typedef struct { 00107 int IsVertical; 00108 int State; 00109 } SCROLLBAR_SKINFLEX_INFO; 00110 00111 /********************************************************************* 00112 * 00113 * Create functions 00114 * 00115 ********************************************************************** 00116 */ 00117 SCROLLBAR_Handle SCROLLBAR_Create (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int Id, int WinFlags, int SpecialFlags); 00118 SCROLLBAR_Handle SCROLLBAR_CreateAttached(WM_HWIN hParent, int SpecialFlags); 00119 SCROLLBAR_Handle SCROLLBAR_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00120 SCROLLBAR_Handle SCROLLBAR_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00121 SCROLLBAR_Handle SCROLLBAR_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00122 00123 /********************************************************************* 00124 * 00125 * The callback ... 00126 * 00127 * Do not call it directly ! It is only to be used from within an 00128 * overwritten callback. 00129 */ 00130 void SCROLLBAR_Callback(WM_MESSAGE * pMsg); 00131 00132 /********************************************************************* 00133 * 00134 * Member functions 00135 * 00136 ********************************************************************** 00137 */ 00138 00139 /* Methods changing properties */ 00140 00141 void SCROLLBAR_AddValue (SCROLLBAR_Handle hObj, int Add); 00142 void SCROLLBAR_Dec (SCROLLBAR_Handle hObj); 00143 void SCROLLBAR_Inc (SCROLLBAR_Handle hObj); 00144 int SCROLLBAR_GetUserData(SCROLLBAR_Handle hObj, void * pDest, int NumBytes); 00145 GUI_COLOR SCROLLBAR_SetColor (SCROLLBAR_Handle hObj, int Index, GUI_COLOR Color); 00146 void SCROLLBAR_SetNumItems(SCROLLBAR_Handle hObj, int NumItems); 00147 void SCROLLBAR_SetPageSize(SCROLLBAR_Handle hObj, int PageSize); 00148 void SCROLLBAR_SetValue (SCROLLBAR_Handle hObj, int v); 00149 int SCROLLBAR_SetWidth (SCROLLBAR_Handle hObj, int Width); 00150 void SCROLLBAR_SetState (SCROLLBAR_Handle hObj, const WM_SCROLL_STATE* pState); 00151 int SCROLLBAR_SetUserData(SCROLLBAR_Handle hObj, const void * pSrc, int NumBytes); 00152 00153 /********************************************************************* 00154 * 00155 * Member functions: Skinning 00156 * 00157 ********************************************************************** 00158 */ 00159 void SCROLLBAR_GetSkinFlexProps (SCROLLBAR_SKINFLEX_PROPS * pProps, int Index); 00160 void SCROLLBAR_SetSkinClassic (SCROLLBAR_Handle hObj); 00161 void SCROLLBAR_SetSkin (SCROLLBAR_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00162 int SCROLLBAR_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00163 void SCROLLBAR_SetSkinFlexProps (const SCROLLBAR_SKINFLEX_PROPS * pProps, int Index); 00164 void SCROLLBAR_SetDefaultSkinClassic(void); 00165 WIDGET_DRAW_ITEM_FUNC * SCROLLBAR_SetDefaultSkin(WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00166 00167 #define SCROLLBAR_SKIN_FLEX SCROLLBAR_DrawSkinFlex 00168 00169 /********************************************************************* 00170 * 00171 * Managing default values 00172 * 00173 ********************************************************************** 00174 */ 00175 int SCROLLBAR_GetDefaultWidth(void); 00176 GUI_COLOR SCROLLBAR_SetDefaultColor(GUI_COLOR Color, unsigned int Index); /* Not yet documented */ 00177 int SCROLLBAR_SetDefaultWidth(int DefaultWidth); 00178 00179 /********************************************************************* 00180 * 00181 * Global functions 00182 * 00183 ********************************************************************** 00184 */ 00185 int SCROLLBAR_GetThumbSizeMin(void); 00186 int SCROLLBAR_SetThumbSizeMin(int ThumbSizeMin); 00187 00188 /********************************************************************* 00189 * 00190 * Query state 00191 * 00192 ********************************************************************** 00193 */ 00194 GUI_COLOR SCROLLBAR_GetColor (SCROLLBAR_Handle hObj, int Index); 00195 int SCROLLBAR_GetNumItems(SCROLLBAR_Handle hObj); 00196 int SCROLLBAR_GetPageSize(SCROLLBAR_Handle hObj); 00197 int SCROLLBAR_GetValue (SCROLLBAR_Handle hObj); 00198 00199 /********************************************************************* 00200 * 00201 * Macros for compatibility 00202 * 00203 ********************************************************************** 00204 */ 00205 #define SCROLLBAR_BKCOLOR0_DEFAULT SCROLLBAR_COLOR_ARROW_DEFAULT 00206 #define SCROLLBAR_BKCOLOR1_DEFAULT SCROLLBAR_COLOR_SHAFT_DEFAULT 00207 #define SCROLLBAR_COLOR0_DEFAULT SCROLLBAR_COLOR_THUMB_DEFAULT 00208 00209 #if defined(__cplusplus) 00210 } 00211 #endif 00212 00213 #endif // GUI_WINSUPPORT 00214 #endif // SCROLLBAR_H 00215 00216 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
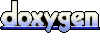