
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
MULTIPAGE.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : MULTIPAGE.h 00040 Purpose : MULTIPAGE include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef MULTIPAGE_H 00045 #define MULTIPAGE_H 00046 00047 #include "WM.h" 00048 #include "DIALOG.h" // Required for Create indirect data structure 00049 00050 #if GUI_WINSUPPORT 00051 00052 #if defined(__cplusplus) 00053 extern "C" { // Make sure we have C-declarations in C++ programs 00054 #endif 00055 00056 /********************************************************************* 00057 * 00058 * Defines 00059 * 00060 ********************************************************************** 00061 */ 00062 /********************************************************************* 00063 * 00064 * Create / Status flags 00065 */ 00066 #define MULTIPAGE_ALIGN_LEFT (0 << 0) 00067 #define MULTIPAGE_ALIGN_RIGHT (1 << 0) 00068 #define MULTIPAGE_ALIGN_TOP (0 << 2) 00069 #define MULTIPAGE_ALIGN_BOTTOM (1 << 2) 00070 00071 #define MULTIPAGE_CF_ROTATE_CW WIDGET_CF_VERTICAL 00072 00073 #define MULTIPAGE_CI_DISABLED 0 00074 #define MULTIPAGE_CI_ENABLED 1 00075 00076 #define MULTIPAGE_SKIN_FRAME_LEFT (1 << 0) 00077 #define MULTIPAGE_SKIN_FRAME_RIGHT (1 << 1) 00078 #define MULTIPAGE_SKIN_FRAME_TOP (1 << 2) 00079 #define MULTIPAGE_SKIN_FRAME_BOTTOM (1 << 3) 00080 #define MULTIPAGE_SKIN_FRAME_ALL (MULTIPAGE_SKIN_FRAME_LEFT | MULTIPAGE_SKIN_FRAME_RIGHT | MULTIPAGE_SKIN_FRAME_TOP | MULTIPAGE_SKIN_FRAME_BOTTOM) 00081 00082 #define MULTIPAGE_SKINFLEX_PI_ENABLED 0 00083 #define MULTIPAGE_SKINFLEX_PI_SELECTED 1 00084 #define MULTIPAGE_SKINFLEX_PI_DISABLED 2 00085 00086 #define SCROLLBAR_SIZE 32 // Defines the space for the scrollbar arrows 00087 00088 #define MULTIPAGE_BI_SELECTED 0 00089 #define MULTIPAGE_BI_UNSELECTED 1 00090 #define MULTIPAGE_BI_DISABLED 2 00091 #define MULTIPAGE_BI_MAX 3 // The defines above are used as array indices. 00092 00093 /********************************************************************* 00094 * 00095 * Public Types 00096 * 00097 ********************************************************************** 00098 */ 00099 typedef WM_HMEM MULTIPAGE_Handle; 00100 00101 typedef struct { 00102 GUI_COLOR BkColor; 00103 GUI_COLOR aBkUpper[2]; 00104 GUI_COLOR aBkLower[2]; 00105 GUI_COLOR FrameColor; 00106 GUI_COLOR TextColor; 00107 } MULTIPAGE_SKINFLEX_PROPS; 00108 00109 typedef struct { 00110 U8 SelSideBorderInc; // Number of pixels to add on both sides when drawing the selected item. 00111 U8 SelTopBorderInc; // Number of pixels to add on top of selected items. 00112 } MULTIPAGE_SKIN_PROPS; 00113 00114 typedef struct { 00115 #if GUI_SUPPORT_ROTATION 00116 GUI_ROTATION * pRotation; 00117 #endif 00118 unsigned Align; 00119 int Sel; 00120 U16 State; 00121 U8 FrameFlags; // Flags to let the drawing function know which parts of the frame to display. 00122 U8 PageStatus; 00123 GUI_DRAW_HANDLE * pDrawObj; 00124 } MULTIPAGE_SKIN_INFO; 00125 00126 /********************************************************************* 00127 * 00128 * Create functions 00129 * 00130 ********************************************************************** 00131 */ 00132 MULTIPAGE_Handle MULTIPAGE_Create (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int Id, int Flags, int SpecialFlags); 00133 MULTIPAGE_Handle MULTIPAGE_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00134 MULTIPAGE_Handle MULTIPAGE_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00135 MULTIPAGE_Handle MULTIPAGE_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00136 00137 /********************************************************************* 00138 * 00139 * The callback ... 00140 * 00141 * Do not call it directly ! It is only to be used from within an 00142 * overwritten callback. 00143 */ 00144 void MULTIPAGE_Callback(WM_MESSAGE * pMsg); 00145 00146 /********************************************************************* 00147 * 00148 * Member functions 00149 * 00150 ********************************************************************** 00151 */ 00152 void MULTIPAGE_AddEmptyPage (MULTIPAGE_Handle hObj, WM_HWIN hWin ,const char * pText); 00153 void MULTIPAGE_AddPage (MULTIPAGE_Handle hObj, WM_HWIN hWin ,const char * pText); 00154 WM_HWIN MULTIPAGE_AttachWindow (MULTIPAGE_Handle hObj, unsigned Index, WM_HWIN hWin); 00155 void MULTIPAGE_DeletePage (MULTIPAGE_Handle hObj, unsigned Index, int Delete); 00156 void MULTIPAGE_DisablePage (MULTIPAGE_Handle hObj, unsigned Index); 00157 void MULTIPAGE_EnablePage (MULTIPAGE_Handle hObj, unsigned Index); 00158 void MULTIPAGE_EnableScrollbar(MULTIPAGE_Handle hObj, unsigned OnOff); 00159 GUI_COLOR MULTIPAGE_GetBkColor (MULTIPAGE_Handle hObj, unsigned Index); 00160 const GUI_FONT * MULTIPAGE_GetFont (MULTIPAGE_Handle hObj); 00161 int MULTIPAGE_GetSelection (MULTIPAGE_Handle hObj); 00162 int MULTIPAGE_GetPageText (MULTIPAGE_Handle hObj, unsigned Index, char * pBuffer, int MaxLen); 00163 GUI_COLOR MULTIPAGE_GetTextColor (MULTIPAGE_Handle hObj, unsigned Index); 00164 int MULTIPAGE_GetUserData (MULTIPAGE_Handle hObj, void * pDest, int NumBytes); 00165 WM_HWIN MULTIPAGE_GetWindow (MULTIPAGE_Handle hObj, unsigned Index); 00166 int MULTIPAGE_IsPageEnabled (MULTIPAGE_Handle hObj, unsigned Index); 00167 void MULTIPAGE_SelectPage (MULTIPAGE_Handle hObj, unsigned Index); 00168 void MULTIPAGE_SetAlign (MULTIPAGE_Handle hObj, unsigned Align); 00169 int MULTIPAGE_SetBitmapEx (MULTIPAGE_Handle hObj, const GUI_BITMAP * pBitmap, int x, int y, int Index, int State); 00170 int MULTIPAGE_SetBitmap (MULTIPAGE_Handle hObj, const GUI_BITMAP * pBitmap, int Index, int State); 00171 void MULTIPAGE_SetBkColor (MULTIPAGE_Handle hObj, GUI_COLOR Color, unsigned Index); 00172 void MULTIPAGE_SetFont (MULTIPAGE_Handle hObj, const GUI_FONT * pFont); 00173 void MULTIPAGE_SetRotation (MULTIPAGE_Handle hObj, unsigned Rotation); 00174 void MULTIPAGE_SetTabWidth (MULTIPAGE_Handle hObj, int Width, int Index); 00175 void MULTIPAGE_SetTabHeight (MULTIPAGE_Handle hObj, int Height); 00176 void MULTIPAGE_SetTextAlign (MULTIPAGE_Handle hObj, unsigned Align); 00177 void MULTIPAGE_SetText (MULTIPAGE_Handle hObj, const char * pText, unsigned Index); 00178 void MULTIPAGE_SetTextColor (MULTIPAGE_Handle hObj, GUI_COLOR Color, unsigned Index); 00179 int MULTIPAGE_SetUserData (MULTIPAGE_Handle hObj, const void * pSrc, int NumBytes); 00180 00181 /********************************************************************* 00182 * 00183 * Managing default values 00184 * 00185 ********************************************************************** 00186 */ 00187 unsigned MULTIPAGE_GetDefaultAlign (void); 00188 GUI_COLOR MULTIPAGE_GetDefaultBkColor (unsigned Index); 00189 const GUI_FONT * MULTIPAGE_GetDefaultFont (void); 00190 GUI_COLOR MULTIPAGE_GetDefaultTextColor (unsigned Index); 00191 00192 void MULTIPAGE_SetDefaultAlign (unsigned Align); 00193 void MULTIPAGE_SetDefaultBkColor (GUI_COLOR Color, unsigned Index); 00194 void MULTIPAGE_SetDefaultBorderSizeX(unsigned Size); 00195 void MULTIPAGE_SetDefaultBorderSizeY(unsigned Size); 00196 void MULTIPAGE_SetDefaultFont (const GUI_FONT * pFont); 00197 void MULTIPAGE_SetDefaultTextColor (GUI_COLOR Color, unsigned Index); 00198 00199 void MULTIPAGE_SetEffectColor (unsigned Index, GUI_COLOR Color); 00200 GUI_COLOR MULTIPAGE_GetEffectColor (unsigned Index); 00201 int MULTIPAGE_GetNumEffectColors (void); 00202 00203 /********************************************************************* 00204 * 00205 * Member functions: Skinning 00206 * 00207 ********************************************************************** 00208 */ 00209 int MULTIPAGE_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00210 void MULTIPAGE_GetSkinFlexProps (MULTIPAGE_SKINFLEX_PROPS * pProps, int Index); 00211 WIDGET_DRAW_ITEM_FUNC * MULTIPAGE_SetDefaultSkin (WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00212 void MULTIPAGE_SetDefaultSkinClassic(void); 00213 void MULTIPAGE_SetSkinClassic (MULTIPAGE_Handle hObj); 00214 void MULTIPAGE_SetSkin (MULTIPAGE_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00215 void MULTIPAGE_SetSkinFlexProps (const MULTIPAGE_SKINFLEX_PROPS * pProps, int Index); 00216 00217 #define MULTIPAGE_SKIN_FLEX MULTIPAGE_DrawSkinFlex 00218 00219 #if defined(__cplusplus) 00220 } 00221 #endif 00222 00223 #endif // GUI_WINSUPPORT 00224 #endif // MULTIPAGE_H 00225 00226 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
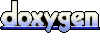