
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
LISTBOX.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : LISTBOX.h 00040 Purpose : LISTBOX widget include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef LISTBOX_H 00045 #define LISTBOX_H 00046 00047 #include "WM.h" 00048 #include "WIDGET.h" /* Req. for WIDGET_DRAW_ITEM_FUNC */ 00049 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * defines 00060 * 00061 ********************************************************************** 00062 */ 00063 00064 #define LISTBOX_ALL_ITEMS -1 00065 00066 /********************************************************************* 00067 * 00068 * Color indices 00069 */ 00070 #define LISTBOX_CI_UNSEL 0 00071 #define LISTBOX_CI_SEL 1 00072 #define LISTBOX_CI_SELFOCUS 2 00073 #define LISTBOX_CI_DISABLED 3 00074 00075 /************************************************************ 00076 * 00077 * States 00078 */ 00079 typedef WM_HMEM LISTBOX_Handle; 00080 00081 /********************************************************************* 00082 * 00083 * Notification codes 00084 * 00085 * The following is the list of notification codes specific to this widget, 00086 * Send with the WM_NOTIFY_PARENT message 00087 */ 00088 #define LISTBOX_NOTIFICATION_LOST_FOCUS (WM_NOTIFICATION_WIDGET + 0) 00089 00090 /************************************************************ 00091 * 00092 * Create / Status flags 00093 */ 00094 #define LISTBOX_CF_AUTOSCROLLBAR_H (1 << 0) 00095 #define LISTBOX_CF_AUTOSCROLLBAR_V (1 << 1) 00096 #define LISTBOX_CF_MULTISEL (1 << 2) 00097 #define LISTBOX_CF_WRAP (1 << 3) 00098 #define LISTBOX_CF_FIXEDSCROLLMODE (1 << 4) 00099 #define LISTBOX_SF_AUTOSCROLLBAR_H LISTBOX_CF_AUTOSCROLLBAR_H 00100 #define LISTBOX_SF_AUTOSCROLLBAR_V LISTBOX_CF_AUTOSCROLLBAR_V 00101 #define LISTBOX_SF_MULTISEL LISTBOX_CF_MULTISEL 00102 #define LISTBOX_SF_WRAP LISTBOX_CF_WRAP 00103 #define LISTBOX_SF_FIXEDSCROLLMODE LISTBOX_CF_FIXEDSCROLLMODE 00104 00105 /************************************************************ 00106 * 00107 * Fixed scroll mode flags 00108 */ 00109 #define LISTBOX_FM_OFF 0 // Turn fixed mode off 00110 #define LISTBOX_FM_ON 1 // Turn fixed mode on 00111 #define LISTBOX_FM_CENTER 2 // Set fixed mode to center 00112 00113 /********************************************************************* 00114 * 00115 * Create functions 00116 * 00117 ********************************************************************** 00118 */ 00119 00120 LISTBOX_Handle LISTBOX_Create (const GUI_ConstString * ppText, int x0, int y0, int xSize, int ySize, int Flags); 00121 LISTBOX_Handle LISTBOX_CreateAsChild (const GUI_ConstString * ppText, WM_HWIN hWinParent, int x0, int y0, int xSize, int ySize, int Flags); 00122 LISTBOX_Handle LISTBOX_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, const GUI_ConstString * ppText); 00123 LISTBOX_Handle LISTBOX_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, const GUI_ConstString * ppText, int NumExtraBytes); 00124 LISTBOX_Handle LISTBOX_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00125 00126 /********************************************************************* 00127 * 00128 * The callback ... 00129 * 00130 * Do not call it directly ! It is only to be used from within an 00131 * overwritten callback. 00132 */ 00133 void LISTBOX_Callback(WM_MESSAGE * pMsg); 00134 00135 /********************************************************************* 00136 * 00137 * Member functions 00138 * 00139 ********************************************************************** 00140 */ 00141 00142 int LISTBOX_AddKey (LISTBOX_Handle hObj, int Key); 00143 void LISTBOX_AddString (LISTBOX_Handle hObj, const char * s); 00144 void LISTBOX_AddStringH (LISTBOX_Handle hObj, WM_HMEM hString); /* Not to be documented!!! */ 00145 void LISTBOX_DecSel (LISTBOX_Handle hObj); 00146 void LISTBOX_DeleteItem (LISTBOX_Handle hObj, unsigned Index); 00147 void LISTBOX_EnableWrapMode (LISTBOX_Handle hObj, int OnOff); 00148 GUI_COLOR LISTBOX_GetBkColor (LISTBOX_Handle hObj, unsigned Index); 00149 const GUI_FONT * LISTBOX_GetFont (LISTBOX_Handle hObj); 00150 unsigned LISTBOX_GetItemSpacing (LISTBOX_Handle hObj); 00151 unsigned LISTBOX_GetNumItems (LISTBOX_Handle hObj); 00152 int LISTBOX_GetSel (LISTBOX_Handle hObj); 00153 GUI_COLOR LISTBOX_GetTextColor (LISTBOX_Handle hObj, unsigned Index); 00154 int LISTBOX_GetItemDisabled (LISTBOX_Handle hObj, unsigned Index); 00155 int LISTBOX_GetItemSel (LISTBOX_Handle hObj, unsigned Index); 00156 void LISTBOX_GetItemText (LISTBOX_Handle hObj, unsigned Index, char * pBuffer, int MaxSize); 00157 int LISTBOX_GetMulti (LISTBOX_Handle hObj); 00158 WM_HWIN LISTBOX_GetOwner (LISTBOX_Handle hObj); 00159 int LISTBOX_GetScrollStepH (LISTBOX_Handle hObj); 00160 int LISTBOX_GetTextAlign (LISTBOX_Handle hObj); 00161 int LISTBOX_GetUserData (LISTBOX_Handle hObj, void * pDest, int NumBytes); 00162 void LISTBOX_IncSel (LISTBOX_Handle hObj); 00163 void LISTBOX_InsertString (LISTBOX_Handle hObj, const char * s, unsigned Index); 00164 void LISTBOX_InvalidateItem (LISTBOX_Handle hObj, int Index); 00165 int LISTBOX_OwnerDraw (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00166 void LISTBOX_SetAutoScrollH (LISTBOX_Handle hObj, int OnOff); 00167 void LISTBOX_SetAutoScrollV (LISTBOX_Handle hObj, int OnOff); 00168 void LISTBOX_SetBkColor (LISTBOX_Handle hObj, unsigned Index, GUI_COLOR color); 00169 void LISTBOX_SetFixedScrollPos(LISTBOX_Handle hObj, U16 FixedScrollPos, U8 Mode); 00170 void LISTBOX_SetFont (LISTBOX_Handle hObj, const GUI_FONT * pFont); 00171 void LISTBOX_SetItemDisabled (LISTBOX_Handle hObj, unsigned Index, int OnOff); 00172 void LISTBOX_SetItemSel (LISTBOX_Handle hObj, unsigned Index, int OnOff); 00173 void LISTBOX_SetItemSpacing (LISTBOX_Handle hObj, unsigned Value); 00174 void LISTBOX_SetMulti (LISTBOX_Handle hObj, int Mode); 00175 void LISTBOX_SetOwner (LISTBOX_Handle hObj, WM_HWIN hOwner); 00176 void LISTBOX_SetOwnerDraw (LISTBOX_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawItem); 00177 void LISTBOX_SetScrollStepH (LISTBOX_Handle hObj, int Value); 00178 void LISTBOX_SetSel (LISTBOX_Handle hObj, int Sel); 00179 void LISTBOX_SetScrollbarColor(LISTBOX_Handle hObj, unsigned Index, GUI_COLOR Color); 00180 void LISTBOX_SetScrollbarWidth(LISTBOX_Handle hObj, unsigned Width); 00181 void LISTBOX_SetString (LISTBOX_Handle hObj, const char * s, unsigned Index); 00182 void LISTBOX_SetText (LISTBOX_Handle hObj, const GUI_ConstString * ppText); 00183 void LISTBOX_SetTextAlign (LISTBOX_Handle hObj, int Align); 00184 GUI_COLOR LISTBOX_SetTextColor (LISTBOX_Handle hObj, unsigned Index, GUI_COLOR Color); 00185 int LISTBOX_SetUserData (LISTBOX_Handle hObj, const void * pSrc, int NumBytes); 00186 int LISTBOX_UpdateScrollers (LISTBOX_Handle hObj); 00187 00188 /********************************************************************* 00189 * 00190 * Managing default values 00191 * 00192 ********************************************************************** 00193 */ 00194 00195 const GUI_FONT * LISTBOX_GetDefaultFont(void); 00196 int LISTBOX_GetDefaultScrollStepH (void); 00197 GUI_COLOR LISTBOX_GetDefaultBkColor (unsigned Index); 00198 int LISTBOX_GetDefaultTextAlign (void); 00199 GUI_COLOR LISTBOX_GetDefaultTextColor (unsigned Index); 00200 void LISTBOX_SetDefaultFont (const GUI_FONT * pFont); 00201 void LISTBOX_SetDefaultScrollStepH (int Value); 00202 void LISTBOX_SetDefaultBkColor (unsigned Index, GUI_COLOR Color); 00203 void LISTBOX_SetDefaultTextAlign (int Align); 00204 void LISTBOX_SetDefaultTextColor (unsigned Index, GUI_COLOR Color); 00205 00206 /********************************************************************* 00207 * 00208 * Compatibility to older versions 00209 * 00210 ********************************************************************** 00211 */ 00212 00213 #define LISTBOX_SetBackColor(hObj, Index, Color) LISTBOX_SetBkColor(hObj, Index, Color) 00214 #define LISTBOX_DeleteString LISTBOX_DeleteItem 00215 00216 #if defined(__cplusplus) 00217 } 00218 #endif 00219 00220 #endif // GUI_WINSUPPORT 00221 #endif // LISTBOX_H 00222 00223 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
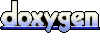