
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
LCDConf.c
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : LCDConf.c 00040 Purpose : Display controller configuration (single layer) 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 #if 0 // moved to the related target folders 00044 #include <stddef.h> 00045 #include <stdio.h> 00046 00047 #include "GUI.h" 00048 #include "GUIDRV_FlexColor.h" 00049 00050 #include "M480.h" 00051 00052 #include "M48XTouchPanel.h" 00053 00054 /********************************************************************* 00055 * 00056 * Layer configuration 00057 * 00058 ********************************************************************** 00059 */ 00060 // 00061 // Physical display size 00062 // 00063 #define XSIZE_PHYS 240 00064 #define YSIZE_PHYS 320 00065 00066 // 00067 // Color conversion 00068 // 00069 #define COLOR_CONVERSION GUICC_565 00070 00071 // 00072 // Display driver 00073 // 00074 #define DISPLAY_DRIVER GUIDRV_FLEXCOLOR 00075 00076 // 00077 // Orientation 00078 // 00079 #define DISPLAY_ORIENTATION (0) 00080 00081 // 00082 // Hardware related 00083 // 00084 /* LCD Module "RS" */ 00085 #define PH_CTRL_RS 1 00086 #if PH_CTRL_RS 00087 #define SET_RS PH3 = 1; 00088 #define CLR_RS PH3 = 0; 00089 #endif 00090 00091 /* LCD Module "RESET" */ 00092 #define SET_RST PB6 = 1; 00093 #define CLR_RST PB6 = 0; 00094 00095 /********************************************************************* 00096 * 00097 * Configuration checking 00098 * 00099 ********************************************************************** 00100 */ 00101 #ifndef VXSIZE_PHYS 00102 #define VXSIZE_PHYS XSIZE_PHYS 00103 #endif 00104 #ifndef VYSIZE_PHYS 00105 #define VYSIZE_PHYS YSIZE_PHYS 00106 #endif 00107 #ifndef XSIZE_PHYS 00108 #error Physical X size of display is not defined! 00109 #endif 00110 #ifndef YSIZE_PHYS 00111 #error Physical Y size of display is not defined! 00112 #endif 00113 #ifndef COLOR_CONVERSION 00114 #error Color conversion not defined! 00115 #endif 00116 #ifndef DISPLAY_DRIVER 00117 #error No display driver defined! 00118 #endif 00119 #ifndef DISPLAY_ORIENTATION 00120 #define DISPLAY_ORIENTATION 0 00121 #endif 00122 00123 /********************************************************************* 00124 * 00125 * Static code 00126 * 00127 **********************************************************************/ 00128 /*-----------------------------------------------*/ 00129 // Write control registers of LCD module 00130 // 00131 /*-----------------------------------------------*/ 00132 #if PH_CTRL_RS 00133 void LCD_WR_REG(uint16_t cmd) 00134 { 00135 CLR_RS 00136 EBI0_WRITE_DATA16(0x00000000, cmd); 00137 SET_RS 00138 00139 } 00140 00141 #else 00142 void LCD_WR_REG(uint16_t cmd) 00143 { 00144 EBI0_WRITE_DATA16(0x00000000, cmd); 00145 } 00146 00147 #endif 00148 00149 00150 /*-----------------------------------------------*/ 00151 // Write data to SRAM of LCD module 00152 // 00153 /*-----------------------------------------------*/ 00154 void LCD_WR_DATA(uint16_t dat) 00155 { 00156 EBI0_WRITE_DATA16(0x00030000, dat); 00157 00158 } 00159 00160 00161 /*-----------------------------------------------*/ 00162 // Read data from SRAM of LCD module 00163 // 00164 /*-----------------------------------------------*/ 00165 uint16_t LCD_RD_DATA(void) 00166 { 00167 return EBI0_READ_DATA16(0x00030000); 00168 00169 } 00170 00171 /******************************************************************** 00172 * 00173 * LcdWriteDataMultiple 00174 * 00175 * Function description: 00176 * Writes multiple values to a display register. 00177 */ 00178 static void LcdWriteDataMultiple(uint16_t * pData, int NumItems) 00179 { 00180 while (NumItems--) { 00181 EBI0_WRITE_DATA16(0x00030000,*pData++); 00182 } 00183 } 00184 00185 /******************************************************************** 00186 * 00187 * LcdReadDataMultiple 00188 * 00189 * Function description: 00190 * Reads multiple values from a display register. 00191 */ 00192 static void LcdReadDataMultiple(uint16_t * pData, int NumItems) 00193 { 00194 while (NumItems--) { 00195 *pData++ = EBI0_READ_DATA16(0x00030000); 00196 } 00197 } 00198 00199 /*-----------------------------------------------*/ 00200 // Set cursor positon on the LCD screen 00201 // 00202 /*-----------------------------------------------*/ 00203 #if 0 00204 void LCD_SetCursor(uint16_t x_s, uint16_t y_s) 00205 { 00206 uint16_t x_e = x_s+1; 00207 uint16_t y_e = y_s+1; 00208 00209 /* X range */ 00210 LCD_WR_REG(0x2A); 00211 LCD_WR_DATA(x_s>>8); 00212 LCD_WR_DATA(x_s); 00213 LCD_WR_DATA(x_e>>8); 00214 LCD_WR_DATA(x_e); 00215 00216 /* Y range */ 00217 LCD_WR_REG(0x2B); 00218 LCD_WR_DATA(y_s>>8); 00219 LCD_WR_DATA(y_s); 00220 LCD_WR_DATA(y_e>>8); 00221 LCD_WR_DATA(y_e); 00222 00223 /* Memory write */ 00224 LCD_WR_REG(0x2C); 00225 00226 } 00227 #endif 00228 00229 /*-----------------------------------------------*/ 00230 // Initial LIL9341 LCD driver chip 00231 // 00232 /*-----------------------------------------------*/ 00233 void ILI9341_Initial(void) 00234 { 00235 uint16_t Reg = 0; 00236 00237 /* Hardware reset */ 00238 SET_RST; 00239 GUI_X_Delay(5); // Delay 5ms 00240 00241 CLR_RST; 00242 GUI_X_Delay(20); // Delay 20ms 00243 00244 SET_RST; 00245 GUI_X_Delay(40); // Delay 40ms 00246 00247 /* Initial control registers */ 00248 LCD_WR_REG(0xCB); 00249 LCD_WR_DATA(0x39); 00250 LCD_WR_DATA(0x2C); 00251 LCD_WR_DATA(0x00); 00252 LCD_WR_DATA(0x34); 00253 LCD_WR_DATA(0x02); 00254 00255 LCD_WR_REG(0xCF); 00256 LCD_WR_DATA(0x00); 00257 LCD_WR_DATA(0xC1); 00258 LCD_WR_DATA(0x30); 00259 00260 LCD_WR_REG(0xE8); 00261 LCD_WR_DATA(0x85); 00262 LCD_WR_DATA(0x00); 00263 LCD_WR_DATA(0x78); 00264 00265 LCD_WR_REG(0xEA); 00266 LCD_WR_DATA(0x00); 00267 LCD_WR_DATA(0x00); 00268 00269 LCD_WR_REG(0xED); 00270 LCD_WR_DATA(0x64); 00271 LCD_WR_DATA(0x03); 00272 LCD_WR_DATA(0x12); 00273 LCD_WR_DATA(0x81); 00274 00275 LCD_WR_REG(0xF7); 00276 LCD_WR_DATA(0x20); 00277 00278 LCD_WR_REG(0xC0); 00279 LCD_WR_DATA(0x23); 00280 00281 LCD_WR_REG(0xC1); 00282 LCD_WR_DATA(0x10); 00283 00284 LCD_WR_REG(0xC5); 00285 LCD_WR_DATA(0x3e); 00286 LCD_WR_DATA(0x28); 00287 00288 LCD_WR_REG(0xC7); 00289 LCD_WR_DATA(0x86); 00290 00291 LCD_WR_REG(0x36); 00292 LCD_WR_DATA(0x48); 00293 00294 LCD_WR_REG(0x3A); 00295 LCD_WR_DATA(0x55); 00296 00297 LCD_WR_REG(0xB1); 00298 LCD_WR_DATA(0x00); 00299 LCD_WR_DATA(0x18); 00300 00301 LCD_WR_REG(0xB6); 00302 LCD_WR_DATA(0x08); 00303 LCD_WR_DATA(0x82); 00304 LCD_WR_DATA(0x27); 00305 00306 LCD_WR_REG(0xF2); 00307 LCD_WR_DATA(0x00); 00308 00309 LCD_WR_REG(0x26); 00310 LCD_WR_DATA(0x01); 00311 00312 LCD_WR_REG(0xE0); 00313 LCD_WR_DATA(0x0F); 00314 LCD_WR_DATA(0x31); 00315 LCD_WR_DATA(0x2B); 00316 LCD_WR_DATA(0x0C); 00317 LCD_WR_DATA(0x0E); 00318 LCD_WR_DATA(0x08); 00319 LCD_WR_DATA(0x4E); 00320 LCD_WR_DATA(0xF1); 00321 LCD_WR_DATA(0x37); 00322 LCD_WR_DATA(0x07); 00323 LCD_WR_DATA(0x10); 00324 LCD_WR_DATA(0x03); 00325 LCD_WR_DATA(0x0E); 00326 LCD_WR_DATA(0x09); 00327 LCD_WR_DATA(0x00); 00328 00329 LCD_WR_REG(0xE1); 00330 LCD_WR_DATA(0x00); 00331 LCD_WR_DATA(0x0E); 00332 LCD_WR_DATA(0x14); 00333 LCD_WR_DATA(0x03); 00334 LCD_WR_DATA(0x11); 00335 LCD_WR_DATA(0x07); 00336 LCD_WR_DATA(0x31); 00337 LCD_WR_DATA(0xC1); 00338 LCD_WR_DATA(0x48); 00339 LCD_WR_DATA(0x08); 00340 LCD_WR_DATA(0x0F); 00341 LCD_WR_DATA(0x0C); 00342 LCD_WR_DATA(0x31); 00343 LCD_WR_DATA(0x36); 00344 LCD_WR_DATA(0x0F); 00345 00346 LCD_WR_REG(0x11); 00347 GUI_X_Delay(200); // Delay 200ms 00348 00349 LCD_WR_REG(0x29); //Display on 00350 00351 LCD_WR_REG(0x0A); 00352 Reg = LCD_RD_DATA(); 00353 Reg = LCD_RD_DATA(); 00354 printf("0Ah = %02x.\n", Reg); 00355 00356 LCD_WR_REG(0x0B); 00357 Reg = LCD_RD_DATA(); 00358 Reg = LCD_RD_DATA(); 00359 printf("0Bh = %02x.\n", Reg); 00360 00361 LCD_WR_REG(0x0C); 00362 Reg = LCD_RD_DATA(); 00363 Reg = LCD_RD_DATA(); 00364 printf("0Ch = %02x.\n", Reg); 00365 00366 printf("Initial ILI9341 LCD Module done.\n\n"); 00367 00368 } 00369 00370 //void I2C2_Init(void) 00371 //{ 00372 /* Open I2C2 and set clock to 100k */ 00373 //I2C_Open(I2C2, 100000); 00374 //} 00375 00376 //void EINT7_IRQHandler(void) 00377 //{ 00378 // /* To check if PB.9 external interrupt occurred */ 00379 // if(GPIO_GET_INT_FLAG(PB, BIT9)) { 00380 // GPIO_CLR_INT_FLAG(PB, BIT9); 00381 // printf("PB.9 EINT7 occurred.\n"); 00382 // } 00383 00384 //} 00385 00386 00387 void EBI_FuncPinInit(void) 00388 { 00389 /*=== EBI (LCD module) mult-function pins ===*/ 00390 /* EBI AD0~5 pins on PG.9~14 */ 00391 SYS->GPG_MFPH &= ~(SYS_GPG_MFPH_PG9MFP_Msk | SYS_GPG_MFPH_PG10MFP_Msk | 00392 SYS_GPG_MFPH_PG11MFP_Msk | SYS_GPG_MFPH_PG12MFP_Msk | 00393 SYS_GPG_MFPH_PG13MFP_Msk | SYS_GPG_MFPH_PG14MFP_Msk); 00394 SYS->GPG_MFPH |= (SYS_GPG_MFPH_PG9MFP_EBI_AD0 | SYS_GPG_MFPH_PG10MFP_EBI_AD1 | 00395 SYS_GPG_MFPH_PG11MFP_EBI_AD2 | SYS_GPG_MFPH_PG12MFP_EBI_AD3 | 00396 SYS_GPG_MFPH_PG13MFP_EBI_AD4 | SYS_GPG_MFPH_PG14MFP_EBI_AD5); 00397 00398 /* EBI AD6, AD7 pins on PD.8, PD.9 */ 00399 SYS->GPD_MFPH &= ~(SYS_GPD_MFPH_PD8MFP_Msk | SYS_GPD_MFPH_PD9MFP_Msk); 00400 SYS->GPD_MFPH |= (SYS_GPD_MFPH_PD8MFP_EBI_AD6 | SYS_GPD_MFPH_PD9MFP_EBI_AD7); 00401 00402 /* EBI AD8, AD9 pins on PE.14, PE.15 */ 00403 SYS->GPE_MFPH &= ~(SYS_GPE_MFPH_PE14MFP_Msk | SYS_GPE_MFPH_PE15MFP_Msk); 00404 SYS->GPE_MFPH |= (SYS_GPE_MFPH_PE14MFP_EBI_AD8 | SYS_GPE_MFPH_PE15MFP_EBI_AD9); 00405 00406 /* EBI AD10, AD11 pins on PE.1, PE.0 */ 00407 SYS->GPE_MFPL &= ~(SYS_GPE_MFPL_PE1MFP_Msk | SYS_GPE_MFPL_PE0MFP_Msk); 00408 SYS->GPE_MFPL |= (SYS_GPE_MFPL_PE1MFP_EBI_AD10 | SYS_GPE_MFPL_PE0MFP_EBI_AD11); 00409 00410 /* EBI AD12~15 pins on PH.8~11 */ 00411 SYS->GPH_MFPH &= ~(SYS_GPH_MFPH_PH8MFP_Msk | SYS_GPH_MFPH_PH9MFP_Msk | 00412 SYS_GPH_MFPH_PH10MFP_Msk | SYS_GPH_MFPH_PH11MFP_Msk); 00413 SYS->GPH_MFPH |= (SYS_GPH_MFPH_PH8MFP_EBI_AD12 | SYS_GPH_MFPH_PH9MFP_EBI_AD13 | 00414 SYS_GPH_MFPH_PH10MFP_EBI_AD14 | SYS_GPH_MFPH_PH11MFP_EBI_AD15); 00415 00416 /* Configure PH.3 as Output mode for LCD_RS (use GPIO PH.3 to control LCD_RS) */ 00417 GPIO_SetMode(PH, BIT3, GPIO_MODE_OUTPUT); 00418 PH3 = 1; 00419 00420 /* EBI RD and WR pins on PE.4 and PE.5 */ 00421 SYS->GPE_MFPL &= ~(SYS_GPE_MFPL_PE4MFP_Msk | SYS_GPE_MFPL_PE5MFP_Msk); 00422 SYS->GPE_MFPL |= (SYS_GPE_MFPL_PE4MFP_EBI_nWR | SYS_GPE_MFPL_PE5MFP_EBI_nRD); 00423 00424 /* EBI CS0 pin on PD.14 */ 00425 SYS->GPD_MFPH &= ~SYS_GPD_MFPH_PD14MFP_Msk; 00426 SYS->GPD_MFPH |= SYS_GPD_MFPH_PD14MFP_EBI_nCS0; 00427 00428 /* Configure PB.6 and PB.7 as Output mode for LCD_RST and LCD_Backlight */ 00429 GPIO_SetMode(PB, BIT6, GPIO_MODE_OUTPUT); 00430 GPIO_SetMode(PB, BIT7, GPIO_MODE_OUTPUT); 00431 PB6 = 1; 00432 PB7 = 0; 00433 00434 //GPIO_SetMode(PH, BIT5, GPIO_MODE_INPUT); 00435 //GPIO_SetMode(PH, BIT6, GPIO_MODE_INPUT); 00436 //GPIO_SetMode(PH, BIT7, GPIO_MODE_INPUT); 00437 00438 } 00439 00440 /********************************************************************* 00441 * 00442 * _InitController 00443 * 00444 * Purpose: 00445 * Initializes the display controller 00446 */ 00447 static void _InitController(void) 00448 { 00449 static uint8_t s_InitOnce = 0; 00450 00451 if (s_InitOnce == 0) 00452 s_InitOnce = 1; 00453 else 00454 return; 00455 00456 CLK_EnableModuleClock(EBI_MODULE); 00457 00458 /* Configure DC/RESET/LED pins */ 00459 EBI_FuncPinInit(); 00460 00461 /* Initialize EBI bank0 to access external LCD Module */ 00462 EBI_Open(EBI_BANK0, EBI_BUSWIDTH_16BIT, EBI_TIMING_NORMAL, 0, EBI_CS_ACTIVE_LOW); 00463 EBI->CTL0 |= EBI_CTL0_CACCESS_Msk; 00464 EBI->TCTL0 |= (EBI_TCTL0_WAHDOFF_Msk | EBI_TCTL0_RAHDOFF_Msk); 00465 printf("\n[EBI CTL0:0x%08X, TCLT0:0x%08X]\n\n", EBI->CTL0, EBI->TCTL0); 00466 00467 /* Init LCD Module */ 00468 ILI9341_Initial(); 00469 00470 /* PB.7 BL_CTRL pin */ 00471 PB7 = 1; 00472 00473 /* Init I2C2 to TP of LCD module */ 00474 //I2C2_Init(); 00475 00476 // /* Configure PB.9 as Quasi-bidirection mode and enable interrupt by falling edge trigger */ 00477 // GPIO_SetMode(PB, BIT9, GPIO_MODE_QUASI); 00478 // GPIO_EnableInt(PB, 9, GPIO_INT_FALLING); 00479 // NVIC_EnableIRQ(EINT7_IRQn); 00480 } 00481 00482 /********************************************************************* 00483 * 00484 * Public code 00485 * 00486 ********************************************************************** 00487 */ 00488 #if GUI_SUPPORT_TOUCH 00489 extern int ts_phy2log(int *sumx, int *sumy); 00490 00491 void GUI_TOUCH_X_ActivateX(void) { 00492 } 00493 00494 void GUI_TOUCH_X_ActivateY(void) { 00495 } 00496 00497 00498 00499 int GUI_TOUCH_X_MeasureX(void) { 00500 int sumx; 00501 int sumy; 00502 if (Read_TouchPanel(&sumx, &sumy)) 00503 { 00504 // sysprintf("X = %d\n", sumx); 00505 ts_phy2log(&sumx, &sumy); 00506 return sumx; 00507 } 00508 return -1; 00509 } 00510 00511 int GUI_TOUCH_X_MeasureY(void) { 00512 int sumx; 00513 int sumy; 00514 if ( Read_TouchPanel(&sumx, &sumy) ) 00515 { 00516 // sysprintf("Y = %d\n", sumy); 00517 ts_phy2log(&sumx, &sumy); 00518 return sumy; 00519 } 00520 return -1; 00521 } 00522 #endif 00523 /********************************************************************* 00524 * 00525 * LCD_X_Config 00526 * 00527 * Purpose: 00528 * Called during the initialization process in order to set up the 00529 * display driver configuration. 00530 * 00531 */ 00532 void LCD_X_Config(void) 00533 { 00534 GUI_DEVICE * pDevice; 00535 CONFIG_FLEXCOLOR Config = {0}; 00536 GUI_PORT_API PortAPI = {0}; 00537 // 00538 // Set display driver and color conversion 00539 // 00540 pDevice = GUI_DEVICE_CreateAndLink(DISPLAY_DRIVER, COLOR_CONVERSION, 0, 0); 00541 00542 // 00543 // Display driver configuration 00544 // 00545 LCD_SetSizeEx (0, XSIZE_PHYS, YSIZE_PHYS); 00546 LCD_SetVSizeEx(0, VXSIZE_PHYS, VYSIZE_PHYS); 00547 // 00548 // Orientation 00549 // 00550 Config.Orientation = GUI_MIRROR_X | GUI_MIRROR_Y | GUI_SWAP_XY; 00551 GUIDRV_FlexColor_Config(pDevice, &Config); 00552 // 00553 // Set controller and operation mode 00554 // 00555 PortAPI.pfWrite16_A0 = LCD_WR_REG; 00556 PortAPI.pfWrite16_A1 = LCD_WR_DATA; 00557 PortAPI.pfWriteM16_A0 = LcdWriteDataMultiple; 00558 PortAPI.pfWriteM16_A1 = LcdWriteDataMultiple; 00559 PortAPI.pfRead16_A0 = LCD_RD_DATA; 00560 PortAPI.pfRead16_A1 = LCD_RD_DATA; 00561 PortAPI.pfReadM16_A0 = LcdReadDataMultiple; 00562 PortAPI.pfReadM16_A1 = LcdReadDataMultiple; 00563 GUIDRV_FlexColor_SetReadFunc66709_B16(pDevice, GUIDRV_FLEXCOLOR_READ_FUNC_III); 00564 GUIDRV_FlexColor_SetFunc(pDevice, &PortAPI, GUIDRV_FLEXCOLOR_F66709, GUIDRV_FLEXCOLOR_M16C0B16); 00565 00566 #if GUI_SUPPORT_TOUCH 00567 // LCD calibration 00568 // 00569 // Calibrate touch screen 00570 // 00571 GUI_TOUCH_Calibrate(GUI_COORD_X, 0, (__DEMO_TS_WIDTH__ - 1), 0, (__DEMO_TS_WIDTH__ - 1)); 00572 GUI_TOUCH_Calibrate(GUI_COORD_Y, 0, (__DEMO_TS_HEIGHT__- 1), 0, (__DEMO_TS_HEIGHT__- 1)); 00573 #endif 00574 } 00575 /********************************************************************* 00576 * 00577 * LCD_X_DisplayDriver 00578 * 00579 * Purpose: 00580 * This function is called by the display driver for several purposes. 00581 * To support the according task the routine needs to be adapted to 00582 * the display controller. Please note that the commands marked with 00583 * 'optional' are not cogently required and should only be adapted if 00584 * the display controller supports these features. 00585 * 00586 * Parameter: 00587 * LayerIndex - Index of layer to be configured 00588 * Cmd - Please refer to the details in the switch statement below 00589 * pData - Pointer to a LCD_X_DATA structure 00590 */ 00591 int LCD_X_DisplayDriver(unsigned LayerIndex, unsigned Cmd, void * pData) 00592 { 00593 int r; 00594 00595 GUI_USE_PARA(LayerIndex); 00596 GUI_USE_PARA(pData); 00597 switch (Cmd) { 00598 // 00599 // Required 00600 // 00601 case LCD_X_INITCONTROLLER: { 00602 // 00603 // Called during the initialization process in order to set up the 00604 // display controller and put it into operation. If the display 00605 // controller is not initialized by any external routine this needs 00606 // to be adapted by the customer... 00607 // 00608 _InitController(); 00609 //printf("\n************ _InitController \n\n"); 00610 return 0; 00611 } 00612 default: 00613 r = -1; 00614 } 00615 return r; 00616 } 00617 #endif 00618 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
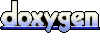