
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
ICONVIEW.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : ICONVIEW.h 00040 Purpose : ICONVIEW include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef ICONVIEW_H 00045 #define ICONVIEW_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "WIDGET.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * Defines 00060 * 00061 ********************************************************************** 00062 */ 00063 // 00064 // Status- and create flags 00065 // 00066 #define ICONVIEW_CF_AUTOSCROLLBAR_V (1 << 1) 00067 #define ICONVIEW_SF_AUTOSCROLLBAR_V ICONVIEW_CF_AUTOSCROLLBAR_V 00068 00069 // 00070 // Color indices 00071 // 00072 #define ICONVIEW_CI_BK 0 00073 #define ICONVIEW_CI_UNSEL 0 00074 #define ICONVIEW_CI_SEL 1 00075 #define ICONVIEW_CI_DISABLED 2 00076 00077 // 00078 // Icon alignment flags, horizontal 00079 // 00080 #define ICONVIEW_IA_HCENTER (0 << 0) 00081 #define ICONVIEW_IA_LEFT (1 << 0) 00082 #define ICONVIEW_IA_RIGHT (2 << 0) 00083 00084 // 00085 // Icon alignment flags, vertical 00086 // 00087 #define ICONVIEW_IA_VCENTER (0 << 2) 00088 #define ICONVIEW_IA_BOTTOM (1 << 2) 00089 #define ICONVIEW_IA_TOP (2 << 2) 00090 00091 /********************************************************************* 00092 * 00093 * Types 00094 * 00095 ********************************************************************** 00096 */ 00097 typedef WM_HMEM ICONVIEW_Handle; 00098 00099 /********************************************************************* 00100 * 00101 * Public functions 00102 * 00103 ********************************************************************** 00104 */ 00105 ICONVIEW_Handle ICONVIEW_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int xSizeItems, int ySizeItems); 00106 ICONVIEW_Handle ICONVIEW_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int xSizeItems, int ySizeItems, int NumExtraBytes); 00107 ICONVIEW_Handle ICONVIEW_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00108 00109 int ICONVIEW_AddBitmapItem (ICONVIEW_Handle hObj, const GUI_BITMAP * pBitmap, const char * pText); 00110 int ICONVIEW_AddBMPItem (ICONVIEW_Handle hObj, const U8 * pBMP, const char * pText); 00111 int ICONVIEW_AddBMPItemEx (ICONVIEW_Handle hObj, const void * pBMP, GUI_GET_DATA_FUNC * pfGetData, const char * pText); 00112 int ICONVIEW_AddStreamedBitmapItem (ICONVIEW_Handle hObj, const void * pStreamedBitmap, const char * pText); 00113 void ICONVIEW_DeleteItem (ICONVIEW_Handle hObj, unsigned Index); 00114 //void ICONVIEW_EnableStreamAuto (void); 00115 GUI_COLOR ICONVIEW_GetBkColor (ICONVIEW_Handle hObj, int Index); 00116 const GUI_FONT * ICONVIEW_GetFont (ICONVIEW_Handle hObj); 00117 U32 ICONVIEW_GetItemUserData (ICONVIEW_Handle hObj, int Index); 00118 int ICONVIEW_GetNumItems (ICONVIEW_Handle hObj); 00119 int ICONVIEW_GetItemText (ICONVIEW_Handle hObj, int Index, char * pBuffer, int MaxSize); 00120 int ICONVIEW_GetSel (ICONVIEW_Handle hObj); 00121 GUI_COLOR ICONVIEW_GetTextColor (ICONVIEW_Handle hObj, int Index); 00122 int ICONVIEW_GetUserData (ICONVIEW_Handle hObj, void * pDest, int NumBytes); 00123 GUI_BITMAP * ICONVIEW_GetItemBitmap (ICONVIEW_Handle hObj, int ItemIndex); 00124 int ICONVIEW_GetReleasedItem (ICONVIEW_Handle hObj); 00125 int ICONVIEW_InsertBitmapItem (ICONVIEW_Handle hObj, const GUI_BITMAP * pBitmap, const char * pText, int Index); 00126 int ICONVIEW_InsertBMPItem (ICONVIEW_Handle hObj, const U8 * pBMP, const char * pText, int Index); 00127 int ICONVIEW_InsertBMPItemEx (ICONVIEW_Handle hObj, const void * pBMP, GUI_GET_DATA_FUNC * pfGetData, const char * pText, int Index); 00128 int ICONVIEW_InsertStreamedBitmapItem(ICONVIEW_Handle hObj, const void * pStreamedBitmap, const char * pText, int Index); 00129 int ICONVIEW_OwnerDraw (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00130 int ICONVIEW_SetBitmapItem (ICONVIEW_Handle hObj, int Index, const GUI_BITMAP * pBitmap); 00131 void ICONVIEW_SetBkColor (ICONVIEW_Handle hObj, int Index, GUI_COLOR Color); 00132 int ICONVIEW_SetBMPItem (ICONVIEW_Handle hObj, const U8 * pBMP, int Index); 00133 int ICONVIEW_SetBMPItemEx (ICONVIEW_Handle hObj, const void * pBMP, GUI_GET_DATA_FUNC * pfGetData, int Index); 00134 void ICONVIEW_SetFont (ICONVIEW_Handle hObj, const GUI_FONT * pFont); 00135 void ICONVIEW_SetFrame (ICONVIEW_Handle hObj, int Coord, int Value); 00136 void ICONVIEW_SetItemText (ICONVIEW_Handle hObj, int Index, const char * pText); 00137 void ICONVIEW_SetItemUserData (ICONVIEW_Handle hObj, int Index, U32 UserData); 00138 void ICONVIEW_SetOwnerDraw (ICONVIEW_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawItem); 00139 void ICONVIEW_SetSel (ICONVIEW_Handle hObj, int Sel); 00140 void ICONVIEW_SetSpace (ICONVIEW_Handle hObj, int Coord, int Value); 00141 int ICONVIEW_SetStreamedBitmapItem (ICONVIEW_Handle hObj, int Index, const void * pStreamedBitmap); 00142 void ICONVIEW_SetIconAlign (ICONVIEW_Handle hObj, int IconAlign); 00143 void ICONVIEW_SetTextAlign (ICONVIEW_Handle hObj, int TextAlign); 00144 void ICONVIEW_SetTextColor (ICONVIEW_Handle hObj, int Index, GUI_COLOR Color); 00145 int ICONVIEW_SetUserData (ICONVIEW_Handle hObj, const void * pSrc, int NumBytes); 00146 void ICONVIEW_SetWrapMode (ICONVIEW_Handle hObj, GUI_WRAPMODE WrapMode); 00147 00148 void ICONVIEW_Callback (WM_MESSAGE * pMsg); 00149 00150 // 00151 // Compatibility macro 00152 // 00153 #define ICONVIEW_EnableStreamAuto() GUI_DrawStreamedEnableAuto() 00154 00155 #if defined(__cplusplus) 00156 } 00157 #endif 00158 00159 #endif // GUI_WINSUPPORT 00160 #endif // ICONVIEW_H 00161 00162 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
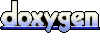