
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
HEADER.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : HEADER.h 00040 Purpose : HEADER include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef HEADER_H 00045 #define HEADER_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "WIDGET.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /********************************************************************* 00058 * 00059 * Public Types 00060 * 00061 ********************************************************************** 00062 */ 00063 00064 typedef WM_HMEM HEADER_Handle; 00065 00066 typedef struct { 00067 GUI_COLOR aColorFrame[2]; 00068 GUI_COLOR aColorUpper[2]; 00069 GUI_COLOR aColorLower[2]; 00070 GUI_COLOR ColorArrow; 00071 } HEADER_SKINFLEX_PROPS; 00072 00073 /********************************************************************* 00074 * 00075 * Create functions 00076 * 00077 ********************************************************************** 00078 */ 00079 00080 HEADER_Handle HEADER_Create (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int Id, int Flags, int SpecialFlags); 00081 HEADER_Handle HEADER_CreateAttached(WM_HWIN hParent, int Id, int SpecialFlags); 00082 HEADER_Handle HEADER_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00083 HEADER_Handle HEADER_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00084 HEADER_Handle HEADER_CreateIndirect(const GUI_WIDGET_CREATE_INFO * pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK * cb); 00085 00086 /********************************************************************* 00087 * 00088 * The callback ... 00089 * 00090 * Do not call it directly ! It is only to be used from within an 00091 * overwritten callback. 00092 */ 00093 void HEADER_Callback(WM_MESSAGE * pMsg); 00094 00095 /********************************************************************* 00096 * 00097 * Managing default values 00098 * 00099 ********************************************************************** 00100 */ 00101 /* Set defaults */ 00102 GUI_COLOR HEADER_SetDefaultArrowColor(GUI_COLOR Color); 00103 GUI_COLOR HEADER_SetDefaultBkColor (GUI_COLOR Color); 00104 const GUI_CURSOR * HEADER_SetDefaultCursor (const GUI_CURSOR * pCursor); 00105 const GUI_FONT * HEADER_SetDefaultFont (const GUI_FONT * pFont); 00106 int HEADER_SetDefaultBorderH (int Spacing); 00107 int HEADER_SetDefaultBorderV (int Spacing); 00108 GUI_COLOR HEADER_SetDefaultTextColor (GUI_COLOR Color); 00109 00110 /* Get defaults */ 00111 GUI_COLOR HEADER_GetDefaultArrowColor(void); 00112 GUI_COLOR HEADER_GetDefaultBkColor (void); 00113 const GUI_CURSOR * HEADER_GetDefaultCursor (void); 00114 const GUI_FONT * HEADER_GetDefaultFont (void); 00115 int HEADER_GetDefaultBorderH (void); 00116 int HEADER_GetDefaultBorderV (void); 00117 GUI_COLOR HEADER_GetDefaultTextColor (void); 00118 00119 /********************************************************************* 00120 * 00121 * Member functions 00122 * 00123 ********************************************************************** 00124 */ 00125 void HEADER_AddItem (HEADER_Handle hObj, int Width, const char * s, int Align); 00126 void HEADER_DeleteItem (HEADER_Handle hObj, unsigned Index); 00127 GUI_COLOR HEADER_GetArrowColor (HEADER_Handle hObj); 00128 GUI_COLOR HEADER_GetBkColor (HEADER_Handle hObj); 00129 const GUI_FONT * HEADER_GetFont (HEADER_Handle hObj); 00130 int HEADER_GetHeight (HEADER_Handle hObj); 00131 int HEADER_GetItemText (HEADER_Handle hObj, unsigned Index, char * pBuffer, int MaxSize); 00132 int HEADER_GetItemWidth (HEADER_Handle hObj, unsigned Index); 00133 int HEADER_GetNumItems (HEADER_Handle hObj); 00134 int HEADER_GetSel (HEADER_Handle hObj); 00135 GUI_COLOR HEADER_GetTextColor (HEADER_Handle hObj); 00136 int HEADER_GetUserData (HEADER_Handle hObj, void * pDest, int NumBytes); 00137 void HEADER_SetArrowColor (HEADER_Handle hObj, GUI_COLOR Color); 00138 void HEADER_SetBitmap (HEADER_Handle hObj, unsigned Index, const GUI_BITMAP * pBitmap); 00139 void HEADER_SetBitmapEx (HEADER_Handle hObj, unsigned Index, const GUI_BITMAP * pBitmap, int x, int y); 00140 void HEADER_SetBkColor (HEADER_Handle hObj, GUI_COLOR Color); 00141 void HEADER_SetBMP (HEADER_Handle hObj, unsigned Index, const void * pBitmap); 00142 void HEADER_SetBMPEx (HEADER_Handle hObj, unsigned Index, const void * pBitmap, int x, int y); 00143 void HEADER_SetDirIndicator (HEADER_Handle hObj, int Column, int Reverse); /* !!!Not to be documented!!! */ 00144 void HEADER_SetDragLimit (HEADER_Handle hObj, unsigned DragLimit); 00145 unsigned HEADER_SetFixed (HEADER_Handle hObj, unsigned Fixed); 00146 void HEADER_SetFont (HEADER_Handle hObj, const GUI_FONT * pFont); 00147 void HEADER_SetHeight (HEADER_Handle hObj, int Height); 00148 void HEADER_SetTextAlign (HEADER_Handle hObj, unsigned Index, int Align); 00149 void HEADER_SetItemText (HEADER_Handle hObj, unsigned Index, const char * s); 00150 void HEADER_SetItemWidth (HEADER_Handle hObj, unsigned Index, int Width); 00151 void HEADER_SetScrollPos (HEADER_Handle hObj, int ScrollPos); 00152 void HEADER_SetStreamedBitmap (HEADER_Handle hObj, unsigned Index, const GUI_BITMAP_STREAM * pBitmap); 00153 void HEADER_SetStreamedBitmapEx(HEADER_Handle hObj, unsigned Index, const GUI_BITMAP_STREAM * pBitmap, int x, int y); 00154 void HEADER_SetTextColor (HEADER_Handle hObj, GUI_COLOR Color); 00155 int HEADER_SetUserData (HEADER_Handle hObj, const void * pSrc, int NumBytes); 00156 00157 /********************************************************************* 00158 * 00159 * Member functions: Skinning 00160 * 00161 ********************************************************************** 00162 */ 00163 void HEADER_GetSkinFlexProps (HEADER_SKINFLEX_PROPS * pProps, int Index); 00164 void HEADER_SetSkinClassic (HEADER_Handle hObj); 00165 void HEADER_SetSkin (HEADER_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00166 int HEADER_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00167 void HEADER_SetSkinFlexProps (const HEADER_SKINFLEX_PROPS * pProps, int Index); 00168 void HEADER_SetDefaultSkinClassic(void); 00169 WIDGET_DRAW_ITEM_FUNC * HEADER_SetDefaultSkin(WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00170 00171 #define HEADER_SKIN_FLEX HEADER_DrawSkinFlex 00172 00173 /********************************************************************* 00174 * 00175 * Macros for compatibility with older versions 00176 * 00177 ********************************************************************** 00178 */ 00179 00180 #ifdef HEADER_SPACING_H 00181 #define HEADER_BORDER_H_DEFAULT HEADER_SPACING_H 00182 #endif 00183 #ifdef HEADER_SPACING_V 00184 #define HEADER_BORDER_V_DEFAULT HEADER_SPACING_V 00185 #endif 00186 #define HEADER_SetDefaultSpacingH(Value) HEADER_SetDefaultBorderH(Value) 00187 #define HEADER_SetDefaultSpacingV(Value) HEADER_SetDefaultBorderV(Value) 00188 #define HEADER_GetDefaultSpacingH() HEADER_GetDefaultBorderH() 00189 #define HEADER_GetDefaultSpacingV() HEADER_GetDefaultBorderV() 00190 00191 #if defined(__cplusplus) 00192 } 00193 #endif 00194 00195 #endif // GUI_WINSUPPORT 00196 #endif // HEADER_H 00197 00198 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
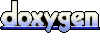