
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
GUI_Private.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : GUI_Private.h 00040 Purpose : GUI internal declarations 00041 ---------------------------END-OF-HEADER------------------------------ 00042 */ 00043 00044 #ifndef GUI_PRIVATE_H 00045 #define GUI_PRIVATE_H 00046 00047 #include "GUI.h" 00048 #include "LCD_Protected.h" 00049 #include "GUI_Debug.h" 00050 #if GUI_WINSUPPORT 00051 #include "WM_GUI.h" 00052 #endif 00053 00054 #if defined(__cplusplus) 00055 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00056 #endif 00057 00058 /********************************************************************* 00059 * 00060 * Defaults for config switches 00061 * 00062 ********************************************************************** 00063 00064 The config switches below do not affect the interface in GUI.h and 00065 are therefor not required to be in GUI.h. 00066 */ 00067 00068 /* Short address area. 00069 For most compilers, this is "near" or "__near" 00070 We do not use this except for some CPUs which we know to always have some 00071 near memory, because the GUI_Context and some other data will be declared 00072 to be in this short address (near) memory area as it has a major effect 00073 on performance. 00074 Please define in GUIConf.h (if you want to use it) 00075 */ 00076 #ifndef GUI_SADDR 00077 #define GUI_SADDR 00078 #endif 00079 00080 #ifndef GUI_DEFAULT_FONT 00081 #define GUI_DEFAULT_FONT &GUI_Font6x8 00082 #endif 00083 00084 #ifndef GUI_DEFAULT_CURSOR 00085 #define GUI_DEFAULT_CURSOR &GUI_CursorArrowM 00086 #endif 00087 00088 #ifndef GUI_DEFAULT_BKCOLOR 00089 #define GUI_DEFAULT_BKCOLOR GUI_BLACK 00090 #endif 00091 00092 #ifndef GUI_DEFAULT_COLOR 00093 #define GUI_DEFAULT_COLOR GUI_WHITE 00094 #endif 00095 00096 /********************************************************************* 00097 * 00098 * Angles 00099 * 00100 ********************************************************************** 00101 */ 00102 #define GUI_45DEG 512 00103 #define GUI_90DEG (2 * GUI_45DEG) 00104 #define GUI_180DEG (4 * GUI_45DEG) 00105 #define GUI_360DEG (8 * GUI_45DEG) 00106 00107 /********************************************************************* 00108 * 00109 * Locking checks 00110 * 00111 ********************************************************************** 00112 */ 00113 #if defined (WIN32) && defined (_DEBUG) && GUI_OS 00114 #define GUI_ASSERT_LOCK() GUITASK_AssertLock() 00115 #define GUI_ASSERT_NO_LOCK() GUITASK_AssertNoLock() 00116 void GUITASK_AssertLock(void); 00117 void GUITASK_AssertNoLock(void); 00118 #else 00119 #define GUI_ASSERT_LOCK() 00120 #define GUI_ASSERT_NO_LOCK() 00121 #endif 00122 00123 /********************************************************************* 00124 * 00125 * Division tables 00126 * 00127 ********************************************************************** 00128 */ 00129 extern const U8 GUI__aConvert_15_255[(1 << 4)]; 00130 extern const U8 GUI__aConvert_31_255[(1 << 5)]; 00131 extern const U8 GUI__aConvert_63_255[(1 << 6)]; 00132 extern const U8 GUI__aConvert_255_15[(1 << 8)]; 00133 extern const U8 GUI__aConvert_255_31[(1 << 8)]; 00134 extern const U8 GUI__aConvert_255_63[(1 << 8)]; 00135 00136 /********************************************************************* 00137 * 00138 * Usage internals 00139 * 00140 ********************************************************************** 00141 */ 00142 typedef GUI_HMEM GUI_USAGE_Handle; 00143 typedef struct tsUSAGE_APIList tUSAGE_APIList; 00144 typedef struct GUI_Usage GUI_USAGE; 00145 #define GUI_USAGE_h GUI_USAGE_Handle 00146 00147 typedef GUI_USAGE_h tUSAGE_CreateCompatible(GUI_USAGE * p); 00148 typedef void tUSAGE_AddPixel (GUI_USAGE * p, int x, int y); 00149 typedef void tUSAGE_AddHLine (GUI_USAGE * p, int x0, int y0, int len); 00150 typedef void tUSAGE_Clear (GUI_USAGE * p); 00151 typedef void tUSAGE_Delete (GUI_USAGE_h h); 00152 typedef int tUSAGE_GetNextDirty (GUI_USAGE * p, int * pxOff, int yOff); 00153 #define GUI_USAGE_LOCK_H(h) ((GUI_USAGE *)GUI_LOCK_H(h)) 00154 00155 void GUI_USAGE_DecUseCnt(GUI_USAGE_Handle hUsage); 00156 00157 GUI_USAGE_Handle GUI_USAGE_BM_Create(int x0, int y0, int xsize, int ysize, int Flags); 00158 void GUI_USAGE_Select(GUI_USAGE_Handle hUsage); 00159 void GUI_USAGE_AddRect(GUI_USAGE * pUsage, int x0, int y0, int xSize, int ySize); 00160 #define GUI_USAGE_AddPixel(p, x,y) p->pAPI->pfAddPixel(p,x,y) 00161 #define GUI_USAGE_AddHLine(p,x,y,len) p->pAPI->pfAddHLine(p,x,y,len) 00162 #define GUI_USAGE_Clear(p) p->pAPI->pfClear(p) 00163 #define GUI_USAGE_Delete(p) p->pAPI->pfDelete(p) 00164 #define GUI_USAGE_GetNextDirty(p,pxOff, yOff) p->pAPI->pfGetNextDirty(p,pxOff, yOff) 00165 00166 struct tsUSAGE_APIList { 00167 tUSAGE_AddPixel * pfAddPixel; 00168 tUSAGE_AddHLine * pfAddHLine; 00169 tUSAGE_Clear * pfClear; 00170 tUSAGE_CreateCompatible * pfCreateCompatible; 00171 tUSAGE_Delete * pfDelete; 00172 tUSAGE_GetNextDirty * pfGetNextDirty; 00173 } ; 00174 00175 struct GUI_Usage { 00176 I16P x0, y0, XSize, YSize; 00177 const tUSAGE_APIList * pAPI; 00178 I16 UseCnt; 00179 }; 00180 00181 /********************************************************************* 00182 * 00183 * GUI_MEMDEV 00184 * 00185 ********************************************************************** 00186 */ 00187 #if GUI_SUPPORT_MEMDEV 00188 00189 typedef struct { 00190 GUI_DEVICE * pDevice; 00191 I16P x0, y0, XSize, YSize; 00192 unsigned BytesPerLine; 00193 unsigned BitsPerPixel; 00194 GUI_HMEM hUsage; 00195 } GUI_MEMDEV; 00196 00197 #define GUI_MEMDEV_LOCK_H(h) ((GUI_MEMDEV *)GUI_LOCK_H(h)) 00198 00199 void GUI_MEMDEV__CopyFromLCD (GUI_MEMDEV_Handle hMem); 00200 void GUI_MEMDEV__GetRect (GUI_RECT * pRect); 00201 unsigned GUI_MEMDEV__Color2Index (LCD_COLOR Color); 00202 LCD_COLOR GUI_MEMDEV__Index2Color (int Index); 00203 unsigned int GUI_MEMDEV__GetIndexMask(void); 00204 void GUI_MEMDEV__SetAlphaCallback(unsigned(* pcbSetAlpha)(U8)); 00205 00206 GUI_MEMDEV_Handle GUI_MEMDEV__CreateFixed(int x0, int y0, int xSize, int ySize, int Flags, 00207 const GUI_DEVICE_API * pDeviceAPI, 00208 const LCD_API_COLOR_CONV * pColorConvAPI); 00209 00210 void GUI_MEMDEV__DrawSizedAt (GUI_MEMDEV_Handle hMem, int xPos, int yPos, int xSize, int ySize); 00211 GUI_MEMDEV_Handle GUI_MEMDEV__GetEmptyCopy32 (GUI_MEMDEV_Handle hMem, int * pxSize, int * pySize, int * pxPos, int * pyPos); 00212 void GUI_MEMDEV__ReadLine (int x0, int y, int x1, LCD_PIXELINDEX * pBuffer); 00213 void GUI_MEMDEV__WriteToActiveAlpha (GUI_MEMDEV_Handle hMem,int x, int y); 00214 void GUI_MEMDEV__WriteToActiveAt (GUI_MEMDEV_Handle hMem,int x, int y); 00215 void GUI_MEMDEV__WriteToActiveOpaque(GUI_MEMDEV_Handle hMem,int x, int y); 00216 void * GUI_MEMDEV__XY2PTR (int x,int y); 00217 void * GUI_MEMDEV__XY2PTREx (GUI_MEMDEV * pDev, int x,int y); 00218 void GUI_MEMDEV__BlendColor32 (GUI_MEMDEV_Handle hMem, U32 BlendColor, U8 BlendIntens); 00219 00220 unsigned GUI__AlphaPreserveTrans(int OnOff); 00221 00222 extern unsigned GUI_MEMDEV__TimePerFrame; 00223 00224 #define GUI_TIME_PER_FRAME (GUI_TIMER_TIME)GUI_MEMDEV__TimePerFrame 00225 00226 #define GUI_POS_AUTO -4095 /* Position value for auto-pos */ 00227 00228 #endif 00229 00230 /********************************************************************* 00231 * 00232 * LCD_HL_ level defines 00233 * 00234 ********************************************************************** 00235 */ 00236 #define LCD_HL_DrawHLine GUI_pContext->pLCD_HL->pfDrawHLine 00237 #define LCD_HL_DrawPixel GUI_pContext->pLCD_HL->pfDrawPixel 00238 00239 /********************************************************************* 00240 * 00241 * Helper functions 00242 * 00243 ********************************************************************** 00244 */ 00245 #define GUI_ZEROINIT(Obj) GUI__MEMSET(Obj, 0, sizeof(Obj)) 00246 int GUI_cos(int angle); 00247 int GUI_sin(int angle); 00248 extern const U32 GUI_Pow10[10]; 00249 00250 /* Multi-touch */ 00251 void GUI_MTOUCH__ManagePID(int OnOff); 00252 00253 /* Anti-aliased drawing */ 00254 int GUI_AA_Init (int x0, int x1); 00255 int GUI_AA_Init_HiRes (int x0, int x1); 00256 void GUI_AA_Exit (void); 00257 I16 GUI_AA_HiRes2Pixel(int HiRes); 00258 00259 void GL_DrawCircleAA_HiRes(int x0, int y0, int r); 00260 void GL_FillCircleAA_HiRes (int x0, int y0, int r); 00261 void GL_FillEllipseAA_HiRes(int x0, int y0, int rx, int ry); 00262 00263 void GUI_AA__DrawCharAA2(int x0, int y0, int XSize, int YSize, int BytesPerLine, const U8 * pData); 00264 void GUI_AA__DrawCharAA4(int x0, int y0, int XSize, int YSize, int BytesPerLine, const U8 * pData); 00265 void GUI_AA__DrawCharAA8(int x0, int y0, int XSize, int YSize, int BytesPerLine, const U8 * pData); 00266 00267 /* Alpha blending helper functions */ 00268 #define GUI_ALPHABLENDING_DONE (1 << 0) 00269 00270 int GUI__GetAlphaBuffer (U32 ** ppCurrent, U32 ** ppConvert, U32 ** ppData, int * pVXSizeMax); 00271 int GUI__AllocAlphaBuffer (int AllocDataBuffer); 00272 U32 * GUI__DoAlphaBlending (int x, int y, U32 * pData, int xSize, tLCDDEV_Index2Color * pfIndex2Color_DEV, int * pDone); 00273 unsigned GUI__SetAlphaBufferSize(int xSize); 00274 00275 /* System independent font routines */ 00276 int GUI_SIF__GetCharDistX (U16P c, int * pSizeX); 00277 void GUI_SIF__GetFontInfo (const GUI_FONT * pFont, GUI_FONTINFO * pfi); 00278 char GUI_SIF__IsInFont (const GUI_FONT * pFont, U16 c); 00279 const U8 * GUI_SIF__GetpCharInfo (const GUI_FONT * pFont, U16P c, unsigned SizeOfCharInfo); 00280 int GUI_SIF__GetNumCharAreas (const GUI_FONT * pFont); 00281 int GUI_SIF__GetCharDistX_ExtFrm(U16P c, int * pSizeX); 00282 void GUI_SIF__GetFontInfo_ExtFrm (const GUI_FONT * pFont, GUI_FONTINFO * pfi); 00283 char GUI_SIF__IsInFont_ExtFrm (const GUI_FONT * pFont, U16 c); 00284 int GUI_SIF__GetCharInfo_ExtFrm (U16P c, GUI_CHARINFO_EXT * pInfo); 00285 void GUI_SIF__ClearLine_ExtFrm (const char * s, int Len); 00286 00287 /* External binary font routines */ 00288 int GUI_XBF__GetOff (const GUI_XBF_DATA * pXBF_Data, unsigned c, U32 * pOff); 00289 int GUI_XBF__GetOffAndSize(const GUI_XBF_DATA * pXBF_Data, unsigned c, U32 * pOff, U16 * pSize); 00290 int GUI_XBF__GetCharDistX (U16P c, int * pSizeX); 00291 void GUI_XBF__GetFontInfo (const GUI_FONT * pFont, GUI_FONTINFO * pInfo); 00292 char GUI_XBF__IsInFont (const GUI_FONT * pFont, U16 c); 00293 int GUI_XBF__GetCharInfo (U16P c, GUI_CHARINFO_EXT * pInfo); 00294 void GUI_XBF__ClearLine (const char * s, int Len); 00295 00296 /* Conversion routines */ 00297 void GUI_AddHex (U32 v, U8 Len, char ** ps); 00298 void GUI_AddBin (U32 v, U8 Len, char ** ps); 00299 void GUI_AddDecMin (I32 v, char ** ps); 00300 void GUI_AddDecShift(I32 v, U8 Len, U8 Shift, char ** ps); 00301 long GUI_AddSign (long v, char ** ps); 00302 int GUI_Long2Len (I32 v); 00303 00304 #define GUI_UC__GetCharSize(sText) GUI_pUC_API->pfGetCharSize(sText) 00305 #define GUI_UC__GetCharCode(sText) GUI_pUC_API->pfGetCharCode(sText) 00306 00307 int GUI_UC__CalcSizeOfChar (U16 Char); 00308 U16 GUI_UC__GetCharCodeInc (const char ** ps); 00309 int GUI_UC__NumChars2NumBytes(const char * s, int NumChars); 00310 int GUI_UC__NumBytes2NumChars(const char * s, int NumBytes); 00311 00312 int GUI__GetLineNumChars (const char * s, int MaxNumChars); 00313 int GUI__GetNumChars (const char * s); 00314 int GUI__GetOverlap (U16 Char); 00315 int GUI__GetLineDistX (const char * s, int Len); 00316 int GUI__GetFontSizeY (void); 00317 int GUI__HandleEOLine (const char ** ps); 00318 void GUI__InvertRectColors (int x0, int y0, int x1, int y1); 00319 void GUI__DispLine (const char * s, int Len, const GUI_RECT * pr); 00320 void GUI__AddSpaceHex (U32 v, U8 Len, char ** ps); 00321 void GUI__CalcTextRect (const char * pText, const GUI_RECT * pTextRectIn, GUI_RECT * pTextRectOut, int TextAlign); 00322 00323 void GUI__ClearTextBackground(int xDist, int yDist); 00324 00325 int GUI__WrapGetNumCharsDisp (const char * pText, int xSize, GUI_WRAPMODE WrapMode); 00326 int GUI__WrapGetNumCharsToNextLine (const char * pText, int xSize, GUI_WRAPMODE WrapMode); 00327 int GUI__WrapGetNumBytesToNextLine (const char * pText, int xSize, GUI_WRAPMODE WrapMode); 00328 //void GUI__memset (U8 * p, U8 Fill, int NumBytes); 00329 void GUI__memset16 (U16 * p, U16 Fill, int NumWords); 00330 int GUI__strlen (const char * s); 00331 int GUI__strcmp (const char * s0, const char * s1); 00332 int GUI__strcmp_hp (GUI_HMEM hs0, const char * s1); 00333 00334 /* Get cursor position */ 00335 int GUI__GetCursorPosX (const char * s, int Index, int MaxNumChars); 00336 int GUI__GetCursorPosChar (const char * s, int x, int NumCharsToNextLine); 00337 U16 GUI__GetCursorCharacter(const char * s, int Index, int MaxNumChars, int * pIsRTL); 00338 00339 /* Arabic support (tbd) */ 00340 U16 GUI__GetPresentationForm (U16 Char, U16 Next, U16 Prev, int * pIgnoreNext, const char * s); 00341 int GUI__IsArabicCharacter (U16 c); 00342 00343 /* BiDi support */ 00344 int GUI__BIDI_Log2Vis (const char * s, int NumChars, char * pBuffer, int BufferSize); 00345 int GUI__BIDI_GetCursorPosX (const char * s, int NumChars, int Index); 00346 int GUI__BIDI_GetCursorPosChar (const char * s, int NumChars, int x); 00347 U16 GUI__BIDI_GetLogChar (const char * s, int NumChars, int Index); 00348 int GUI__BIDI_GetCharDir (const char * s, int NumChars, int Index); 00349 int GUI__BIDI_IsNSM (U16 Char); 00350 U16 GUI__BIDI_GetCursorCharacter(const char * s, int Index, int MaxNumChars, int * pIsRTL); 00351 int GUI__BIDI_GetWordWrap (const char * s, int xSize, int * pxDist); 00352 int GUI__BIDI_GetCharWrap (const char * s, int xSize); 00353 00354 #if (GUI_USE_BIDI2) 00355 00356 #define GUI__BIDI_Log2Vis GUI__BIDI2_Log2Vis 00357 #define GUI__BIDI_GetCursorPosX GUI__BIDI2_GetCursorPosX 00358 #define GUI__BIDI_GetCursorPosChar GUI__BIDI2_GetCursorPosChar 00359 #define GUI__BIDI_GetLogChar GUI__BIDI2_GetLogChar 00360 #define GUI__BIDI_GetCharDir GUI__BIDI2_GetCharDir 00361 #define GUI__BIDI_IsNSM GUI__BIDI2_IsNSM 00362 #define GUI__BIDI_GetCursorCharacter GUI__BIDI2_GetCursorCharacter 00363 #define GUI__BIDI_GetWordWrap GUI__BIDI2_GetWordWrap 00364 #define GUI__BIDI_GetCharWrap GUI__BIDI2_GetCharWrap 00365 #define GUI__BIDI_SetBaseDir GUI__BIDI2_SetBaseDir 00366 #define GUI__BIDI_GetBaseDir GUI__BIDI2_GetBaseDir 00367 00368 int GUI__BIDI_Log2Vis (const char * s, int NumChars, char * pBuffer, int BufferSize); 00369 int GUI__BIDI_GetCursorPosX (const char * s, int NumChars, int Index); 00370 int GUI__BIDI_GetCursorPosChar (const char * s, int NumChars, int x); 00371 U16 GUI__BIDI_GetLogChar (const char * s, int NumChars, int Index); 00372 int GUI__BIDI_GetCharDir (const char * s, int NumChars, int Index); 00373 int GUI__BIDI_IsNSM (U16 Char); 00374 U16 GUI__BIDI_GetCursorCharacter(const char * s, int Index, int MaxNumChars, int * pIsRTL); 00375 int GUI__BIDI_GetWordWrap (const char * s, int xSize, int * pxDist); 00376 int GUI__BIDI_GetCharWrap (const char * s, int xSize); 00377 void GUI__BIDI_SetBaseDir (int Dir); 00378 int GUI__BIDI_GetBaseDir (void); 00379 00380 #else 00381 00382 #define GUI__BIDI_SetBaseDir 00383 #define GUI__BIDI_GetBaseDir 00384 00385 #endif 00386 00387 const char * GUI__BIDI_Log2VisBuffered(const char * s, int * pMaxNumChars, int Mode); 00388 00389 extern int GUI__BIDI_Enabled; 00390 00391 extern int (* _pfGUI__BIDI_Log2Vis )(const char * s, int NumChars, char * pBuffer, int BufferSize); 00392 extern int (* _pfGUI__BIDI_GetCursorPosX )(const char * s, int NumChars, int Index); 00393 extern int (* _pfGUI__BIDI_GetCursorPosChar)(const char * s, int NumChars, int x); 00394 extern U16 (* _pfGUI__BIDI_GetLogChar )(const char * s, int NumChars, int Index); 00395 extern int (* _pfGUI__BIDI_GetCharDir )(const char * s, int NumChars, int Index); 00396 extern int (* _pfGUI__BIDI_IsNSM )(U16 Char); 00397 00398 /* BiDi-related function pointers */ 00399 extern const char * (* GUI_CharLine_pfLog2Vis)(const char * s, int * pMaxNumChars, int Mode); 00400 00401 extern int (* GUI__GetCursorPos_pfGetPosX) (const char * s, int MaxNumChars, int Index); 00402 extern int (* GUI__GetCursorPos_pfGetPosChar) (const char * s, int MaxNumChars, int x); 00403 extern U16 (* GUI__GetCursorPos_pfGetCharacter)(const char * s, int MaxNumChars, int Index, int * pIsRTL); 00404 00405 extern int (* GUI__Wrap_pfGetWordWrap)(const char * s, int xSize, int * pxDist); 00406 extern int (* GUI__Wrap_pfGetCharWrap)(const char * s, int xSize); 00407 00408 /* Proportional font support */ 00409 const GUI_FONT_PROP * GUIPROP__FindChar(const GUI_FONT_PROP * pProp, U16P c); 00410 00411 /* Extended proportional font support */ 00412 const GUI_FONT_PROP_EXT * GUIPROP_EXT__FindChar(const GUI_FONT_PROP_EXT * pPropExt, U16P c); 00413 void GUIPROP_EXT__DispLine (const char * s, int Len); 00414 void GUIPROP_EXT__ClearLine (const char * s, int Len); 00415 void GUIPROP_EXT__SetfpClearLine(void (* fpClearLine)(const char * s, int Len)); 00416 00417 /* Reading data routines */ 00418 U16 GUI__Read16(const U8 ** ppData); 00419 U32 GUI__Read32(const U8 ** ppData); 00420 00421 /* Virtual screen support */ 00422 void GUI__GetOrg(int * px, int * py); 00423 void GUI__SetOrgHook(void(* pfHook)(int x, int y)); 00424 00425 /* Timer support */ 00426 int GUI_TIMER__IsActive (void); 00427 GUI_TIMER_TIME GUI_TIMER__GetPeriod (void); 00428 GUI_TIMER_HANDLE GUI_TIMER__GetFirstTimer (PTR_ADDR * pContext); 00429 GUI_TIMER_HANDLE GUI_TIMER__GetNextTimerLin(GUI_TIMER_HANDLE hTimer, PTR_ADDR * pContext); 00430 00431 /* Get function pointers for color conversion */ 00432 tLCDDEV_Index2Color * GUI_GetpfIndex2ColorEx(int LayerIndex); 00433 tLCDDEV_Color2Index * GUI_GetpfColor2IndexEx(int LayerIndex); 00434 00435 int GUI_GetBitsPerPixelEx(int LayerIndex); 00436 00437 LCD_PIXELINDEX * LCD_GetpPalConvTable (const LCD_LOGPALETTE * pLogPal); 00438 LCD_PIXELINDEX * LCD_GetpPalConvTableUncached(const LCD_LOGPALETTE * pLogPal); 00439 LCD_PIXELINDEX * LCD_GetpPalConvTableBM (const LCD_LOGPALETTE * pLogPal, const GUI_BITMAP * pBitmap, int LayerIndex); 00440 00441 /* Setting a function for converting a color palette to an array of index values */ 00442 void GUI_SetFuncGetpPalConvTable(LCD_PIXELINDEX * (* pFunc)(const LCD_LOGPALETTE * pLogPal, const GUI_BITMAP * pBitmap, int LayerIndex)); 00443 00444 /********************************************************************* 00445 * 00446 * Format definitions used by streamed bitmaps 00447 * 00448 * IMPORTANT: DO NOT CHANGE THESE VALUES! 00449 * THEY HAVE TO CORRESPOND TO THE DEFINITIONS WITHIN THE CODE OF THE BITMAPCONVERTER! 00450 */ 00451 #define GUI_STREAM_FORMAT_INDEXED 100 /* DO NOT CHANGE */ 00452 #define GUI_STREAM_FORMAT_RLE4 6 /* DO NOT CHANGE */ 00453 #define GUI_STREAM_FORMAT_RLE8 7 /* DO NOT CHANGE */ 00454 #define GUI_STREAM_FORMAT_565 8 /* DO NOT CHANGE */ 00455 #define GUI_STREAM_FORMAT_M565 9 /* DO NOT CHANGE */ 00456 #define GUI_STREAM_FORMAT_555 10 /* DO NOT CHANGE */ 00457 #define GUI_STREAM_FORMAT_M555 11 /* DO NOT CHANGE */ 00458 #define GUI_STREAM_FORMAT_RLE16 12 /* DO NOT CHANGE */ 00459 #define GUI_STREAM_FORMAT_RLEM16 13 /* DO NOT CHANGE */ 00460 #define GUI_STREAM_FORMAT_8888 16 /* DO NOT CHANGE */ 00461 #define GUI_STREAM_FORMAT_RLE32 15 /* DO NOT CHANGE */ 00462 #define GUI_STREAM_FORMAT_24 17 /* DO NOT CHANGE */ 00463 #define GUI_STREAM_FORMAT_RLEALPHA 18 /* DO NOT CHANGE */ 00464 #define GUI_STREAM_FORMAT_444_12 19 /* DO NOT CHANGE */ 00465 #define GUI_STREAM_FORMAT_M444_12 20 /* DO NOT CHANGE */ 00466 #define GUI_STREAM_FORMAT_444_12_1 21 /* DO NOT CHANGE */ 00467 #define GUI_STREAM_FORMAT_M444_12_1 22 /* DO NOT CHANGE */ 00468 #define GUI_STREAM_FORMAT_444_16 23 /* DO NOT CHANGE */ 00469 #define GUI_STREAM_FORMAT_M444_16 24 /* DO NOT CHANGE */ 00470 #define GUI_STREAM_FORMAT_A555 25 /* DO NOT CHANGE */ 00471 #define GUI_STREAM_FORMAT_AM555 26 /* DO NOT CHANGE */ 00472 #define GUI_STREAM_FORMAT_A565 27 /* DO NOT CHANGE */ 00473 #define GUI_STREAM_FORMAT_AM565 28 /* DO NOT CHANGE */ 00474 #define GUI_STREAM_FORMAT_M8888I 29 /* DO NOT CHANGE */ 00475 00476 void GUI__ReadHeaderFromStream (GUI_BITMAP_STREAM * pBitmapHeader, const U8 * pData); 00477 void GUI__CreateBitmapFromStream(const GUI_BITMAP_STREAM * pBitmapHeader, const void * pData, GUI_BITMAP * pBMP, GUI_LOGPALETTE * pPAL, const GUI_BITMAP_METHODS * pMethods); 00478 00479 /* Cache management */ 00480 int GUI__ManageCache (int Cmd); 00481 int GUI__ManageCacheEx(int LayerIndex, int Cmd); 00482 00483 /********************************************************************* 00484 * 00485 * 2d - GL 00486 * 00487 ********************************************************************** 00488 */ 00489 void GL_DispChar (U16 c); 00490 void GL_DrawArc (int x0, int y0, int rx, int ry, int a0, int a1); 00491 void GL_DrawBitmap (const GUI_BITMAP * pBM, int x0, int y0); 00492 void GL_DrawCircle (int x0, int y0, int r); 00493 void GL_DrawEllipse (int x0, int y0, int rx, int ry, int w); 00494 void GL_DrawHLine (int y0, int x0, int x1); 00495 void GL_DrawPolygon (const GUI_POINT * pPoints, int NumPoints, int x0, int y0); 00496 void GL_DrawPoint (int x, int y); 00497 void GL_DrawLine1 (int x0, int y0, int x1, int y1); 00498 void GL_DrawLine1Ex (int x0, int y0, int x1, int y1, unsigned * pPixelCnt); 00499 void GL_DrawLineRel (int dx, int dy); 00500 void GL_DrawLineTo (int x, int y); 00501 void GL_DrawLineToEx (int x, int y, unsigned * pPixelCnt); 00502 void GL_DrawLine (int x0, int y0, int x1, int y1); 00503 void GL_DrawLineEx (int x0, int y0, int x1, int y1, unsigned * pPixelCnt); 00504 void GL_MoveTo (int x, int y); 00505 void GL_FillCircle (int x0, int y0, int r); 00506 void GL_FillCircleAA (int x0, int y0, int r); 00507 void GL_FillEllipse (int x0, int y0, int rx, int ry); 00508 void GL_FillPolygon (const GUI_POINT * pPoints, int NumPoints, int x0, int y0); 00509 void GL_SetDefault (void); 00510 00511 /********************************************************************* 00512 * 00513 * Replacement of memcpy() and memset() 00514 * 00515 ********************************************************************** 00516 */ 00517 // 00518 // Configurable function pointers 00519 // 00520 extern void * (* GUI__pfMemset)(void * pDest, int Fill, size_t Cnt); 00521 extern void * (* GUI__pfMemcpy)(void * pDest, const void * pSrc, size_t Cnt); 00522 00523 extern int (* GUI__pfStrcmp)(const char *, const char *); 00524 extern size_t (* GUI__pfStrlen)(const char *); 00525 extern char * (* GUI__pfStrcpy)(char *, const char *); 00526 // 00527 // Macros for typesave use of function pointers 00528 // 00529 #define GUI__MEMSET(pDest, Fill, Cnt) GUI__pfMemset((void *)(pDest), (int)(Fill), (size_t)(Cnt)) 00530 #define GUI__MEMCPY(pDest, pSrc, Cnt) GUI__pfMemcpy((void *)(pDest), (const void *)(pSrc), (size_t)(Cnt)) 00531 00532 /********************************************************************* 00533 * 00534 * Callback pointers for dynamic linkage 00535 * 00536 ********************************************************************** 00537 Dynamic linkage pointers reduces configuration hassles. 00538 */ 00539 typedef int GUI_tfTimer(void); 00540 typedef int WM_tfHandlePID(void); 00541 00542 /********************************************************************* 00543 * 00544 * Text rotation 00545 * 00546 ********************************************************************** 00547 */ 00548 extern GUI_RECT GUI_RectDispString; /* Used by LCD_Rotate...() and GUI_DispStringInRect() */ 00549 00550 /********************************************************************* 00551 * 00552 * Flag for setting transparency for 'EXT' fonts 00553 * 00554 ********************************************************************** 00555 */ 00556 extern U8 GUI__CharHasTrans; 00557 00558 /********************************************************************* 00559 * 00560 * Multitasking support 00561 * 00562 ********************************************************************** 00563 */ 00564 extern int GUITASK__EntranceCnt; 00565 00566 /********************************************************************* 00567 * 00568 * Bitmap related functions 00569 * 00570 ********************************************************************** 00571 */ 00572 00573 int GUI_GetBitmapPixelIndex(const GUI_BITMAP * pBMP, unsigned x, unsigned y); 00574 GUI_COLOR GUI_GetBitmapPixelColor(const GUI_BITMAP * pBMP, unsigned x, unsigned y); 00575 int GUI_GetBitmapPixelIndexEx(int BitsPerPixel, int BytesPerLine, const U8 * pData, unsigned x, unsigned y); 00576 00577 void GUI__DrawBitmap16bpp (int x0, int y0, int xsize, int ysize, const U8 * pPixel, const LCD_LOGPALETTE * pLogPal, int xMag, int yMag, tLCDDEV_Index2Color * pfIndex2Color, const LCD_API_COLOR_CONV * pColorConvAPI); 00578 void GUI__DrawBitmapA16bpp(int x0, int y0, int xSize, int ySize, const U8 * pPixel, const LCD_LOGPALETTE * pLogPal, int xMag, int yMag, tLCDDEV_Index2Color * pfIndex2Color); 00579 void GUI__SetPixelAlpha (int x, int y, U8 Alpha, LCD_COLOR Color); 00580 LCD_COLOR GUI__MixColors (LCD_COLOR Color, LCD_COLOR BkColor, U8 Intens); 00581 void GUI__MixColorsBulk (U32 * pFG, U32 * pBG, U32 * pDst, unsigned OffFG, unsigned OffBG, unsigned OffDest, unsigned xSize, unsigned ySize, U8 Intens); 00582 00583 extern const GUI_UC_ENC_APILIST GUI_UC_None; 00584 00585 /********************************************************************* 00586 * 00587 * LCDDEV_L0_xxx 00588 * 00589 ********************************************************************** 00590 */ 00591 #define LCDDEV_L0_Color2Index GUI__apDevice[GUI_pContext->SelLayer]->pColorConvAPI->pfColor2Index 00592 #define LCDDEV_L0_Index2Color GUI__apDevice[GUI_pContext->SelLayer]->pColorConvAPI->pfIndex2Color 00593 00594 #define LCDDEV_L0_DrawBitmap GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfDrawBitmap 00595 #define LCDDEV_L0_DrawHLine GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfDrawHLine 00596 #define LCDDEV_L0_DrawVLine GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfDrawVLine 00597 #define LCDDEV_L0_DrawPixel GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfDrawPixel 00598 #define LCDDEV_L0_FillRect GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfFillRect 00599 #define LCDDEV_L0_GetPixel GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfGetPixel 00600 #define LCDDEV_L0_GetRect GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfGetRect 00601 #define LCDDEV_L0_GetPixelIndex GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfGetPixelIndex 00602 #define LCDDEV_L0_SetPixelIndex GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfSetPixelIndex 00603 #define LCDDEV_L0_XorPixel GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfXorPixel 00604 #define LCDDEV_L0_GetDevFunc GUI__apDevice[GUI_pContext->SelLayer]->pDeviceAPI->pfGetDevFunc 00605 00606 void LCD_ReadRect (int x0, int y0, int x1, int y1, LCD_PIXELINDEX * pBuffer, GUI_DEVICE * pDevice); 00607 void GUI_ReadRect (int x0, int y0, int x1, int y1, LCD_PIXELINDEX * pBuffer, GUI_DEVICE * pDevice); 00608 void GUI_ReadRectEx(int x0, int y0, int x1, int y1, LCD_PIXELINDEX * pBuffer, GUI_DEVICE * pDevice); 00609 00610 void LCD_ReadRectNoClip(int x0, int y0, int x1, int y1, LCD_PIXELINDEX * pBuffer, GUI_DEVICE * pDevice); 00611 00612 /********************************************************************* 00613 * 00614 * Internal color management 00615 * 00616 ********************************************************************** 00617 */ 00618 typedef struct { 00619 void (* pfSetColor) (LCD_COLOR Index); 00620 void (* pfSetBkColor) (LCD_COLOR Index); 00621 LCD_DRAWMODE (* pfSetDrawMode)(LCD_DRAWMODE dm); 00622 } LCD_SET_COLOR_API; 00623 00624 extern const LCD_SET_COLOR_API * LCD__pSetColorAPI; 00625 00626 #define LCD__SetBkColorIndex(Index) (*GUI_pContext->LCD_pBkColorIndex = Index) 00627 #define LCD__SetColorIndex(Index) (*GUI_pContext->LCD_pColorIndex = Index) 00628 #define LCD__GetBkColorIndex() (*GUI_pContext->LCD_pBkColorIndex) 00629 #define LCD__GetColorIndex() (*GUI_pContext->LCD_pColorIndex) 00630 00631 /* The following 2 defines are only required for compatibility to older versions of the TTF library */ 00632 #define LCD_BKCOLORINDEX (*GUI_pContext->LCD_pBkColorIndex) 00633 #define LCD_COLORINDEX (*GUI_pContext->LCD_pColorIndex) 00634 00635 /********************************************************************* 00636 * 00637 * EXTERNs for GL_CORE 00638 * 00639 ********************************************************************** 00640 */ 00641 extern const GUI_FONT * GUI__pFontDefault; 00642 extern GUI_COLOR GUI__ColorDefault; 00643 extern GUI_COLOR GUI__BkColorDefault; 00644 00645 extern GUI_SADDR GUI_CONTEXT * GUI_pContext; 00646 00647 extern GUI_DEVICE * GUI__apDevice[GUI_NUM_LAYERS]; 00648 00649 // 00650 // Function pointer for converting a palette containing a color array into an index array 00651 // 00652 extern LCD_PIXELINDEX * (* GUI_pfGetpPalConvTable)(const LCD_LOGPALETTE * pLogPal, const GUI_BITMAP * pBitmap, int LayerIndex); 00653 00654 // 00655 // Function pointer for mixing up 2 colors 00656 // 00657 extern LCD_COLOR (* GUI__pfMixColors)(LCD_COLOR Color, LCD_COLOR BkColor, U8 Intens); 00658 00659 // 00660 // Function pointer for mixing up arrays of colors 00661 // 00662 extern void (* GUI__pfMixColorsBulk)(U32 * pFG, U32 * pBG, U32 * pDst, unsigned OffFG, unsigned OffBG, unsigned OffDest, unsigned xSize, unsigned ySize, U8 Intens); 00663 00664 // 00665 // Function pointer for mixing color and gamma values 00666 // 00667 extern LCD_COLOR (* LCD_AA_pfMixColors16)(LCD_COLOR Color, LCD_COLOR BkColor, U8 Intens); 00668 00669 // 00670 // Function pointer for drawing alpha memory devices 00671 // 00672 extern GUI_DRAWMEMDEV_FUNC * GUI__pfDrawAlphaMemdevFunc; 00673 extern GUI_DRAWMEMDEV_FUNC * GUI__pfDrawM565MemdevFunc; 00674 00675 // 00676 // Function pointer for drawing alpha bitmaps 00677 // 00678 extern GUI_DRAWBITMAP_FUNC * GUI__pfDrawAlphaBitmapFunc; 00679 extern GUI_DRAWBITMAP_FUNC * GUI__pfDrawM565BitmapFunc; 00680 00681 extern U8 GUI__DrawStreamedBitmap; 00682 00683 // 00684 // API list to be used for MultiBuffering 00685 // 00686 extern const GUI_MULTIBUF_API GUI_MULTIBUF_APIList; 00687 extern const GUI_MULTIBUF_API GUI_MULTIBUF_APIListMasked; 00688 extern const GUI_MULTIBUF_API_EX GUI_MULTIBUF_APIListEx; 00689 00690 #ifdef GL_CORE_C 00691 #define GUI_EXTERN 00692 #else 00693 #define GUI_EXTERN extern 00694 #endif 00695 00696 GUI_EXTERN void (* GUI_pfExecAnimations)(void); 00697 GUI_EXTERN int (* GUI_pfUpdateSoftLayer)(void); 00698 00699 #ifdef WIN32 00700 GUI_EXTERN void (* GUI_pfSoftlayerGetPixel)(int x, int y, void * p); 00701 #endif 00702 00703 GUI_EXTERN void (* GUI_pfHookMTOUCH)(const GUI_MTOUCH_STATE * pState); 00704 00705 GUI_EXTERN const GUI_UC_ENC_APILIST * GUI_pUC_API; /* Unicode encoding API */ 00706 00707 GUI_EXTERN GUI_SADDR char GUI_DecChar; 00708 GUI_EXTERN GUI_tfTimer * GUI_pfTimerExec; 00709 GUI_EXTERN WM_tfHandlePID * WM_pfHandlePID; 00710 GUI_EXTERN void (* GUI_pfDispCharStyle)(U16 Char); 00711 GUI_EXTERN void (* GUI_pfDispCharLine)(int x0); 00712 00713 GUI_EXTERN int GUI__BufferSize; // Required buffer size in pixels for alpha blending and/or antialiasing 00714 GUI_EXTERN int GUI_AA__ClipX0; // x0-clipping value for AA module 00715 00716 GUI_EXTERN I8 GUI__aNumBuffers[GUI_NUM_LAYERS]; // Number of buffers used per layer 00717 GUI_EXTERN U8 GUI__PreserveTrans; 00718 GUI_EXTERN U8 GUI__IsInitialized; 00719 00720 GUI_EXTERN U8 GUI__NumLayersInUse; 00721 GUI_EXTERN U32 GUI__LayerMask; 00722 00723 #if GUI_SUPPORT_ROTATION 00724 GUI_EXTERN const tLCD_APIList * GUI_pLCD_APIList; /* Used for rotating text */ 00725 #endif 00726 00727 GUI_EXTERN I16 GUI_OrgX, GUI_OrgY; 00728 00729 #undef GUI_EXTERN 00730 00731 #if defined(__cplusplus) 00732 } 00733 #endif 00734 00735 #endif /* GUI_PRIVATE_H */ 00736 00737 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
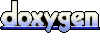