
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
FramewinDLG.cpp
00001 /********************************************************************* 00002 * * 00003 * SEGGER Microcontroller GmbH & Co. KG * 00004 * Solutions for real time microcontroller applications * 00005 * * 00006 ********************************************************************** 00007 * * 00008 * C-file generated by: * 00009 * * 00010 * GUI_Builder for emWin version 5.46 * 00011 * Compiled Dec 12 2017, 16:38:40 * 00012 * (c) 2017 Segger Microcontroller GmbH & Co. KG * 00013 * * 00014 ********************************************************************** 00015 * * 00016 * Internet: www.segger.com Support: support@segger.com * 00017 * * 00018 ********************************************************************** 00019 */ 00020 00021 // USER START (Optionally insert additional includes) 00022 // USER END 00023 #include "stdio.h" 00024 #include "DIALOG.h" 00025 00026 #include "NuMicro.h" 00027 /* Scheduler include files. */ 00028 //#include "FreeRTOS.h" 00029 //#include "task.h" 00030 //#include "semphr.h" 00031 00032 //extern xSemaphoreHandle psGUISemaphore; 00033 /********************************************************************* 00034 * 00035 * Defines 00036 * 00037 ********************************************************************** 00038 */ 00039 #define ID_FRAMEWIN_0 (GUI_ID_USER + 0x00) 00040 #define ID_BUTTON_0 (GUI_ID_USER + 0x05) 00041 #define ID_BUTTON_1 (GUI_ID_USER + 0x06) 00042 #define ID_BUTTON_2 (GUI_ID_USER + 0x07) 00043 #define ID_BUTTON_3 (GUI_ID_USER + 0x08) 00044 #define ID_EDIT_0 (GUI_ID_USER + 0x09) 00045 #define ID_TEXT_0 (GUI_ID_USER + 0x0B) 00046 00047 00048 // USER START (Optionally insert additional defines) 00049 // USER END 00050 00051 /********************************************************************* 00052 * 00053 * Static data 00054 * 00055 ********************************************************************** 00056 */ 00057 00058 // USER START (Optionally insert additional static data) 00059 // USER END 00060 00061 /********************************************************************* 00062 * 00063 * _aDialogCreate 00064 */ 00065 static const GUI_WIDGET_CREATE_INFO _aDialogCreate[7] = 00066 { 00067 { FRAMEWIN_CreateIndirect, "Framewin", ID_FRAMEWIN_0, 0, 0, 320, 240, 0, 0x0 }, 00068 { BUTTON_CreateIndirect, "+ 1", ID_BUTTON_0, 43, 38, 80, 20, 0, 0x0 }, 00069 { BUTTON_CreateIndirect, "+ 10", ID_BUTTON_1, 43, 77, 80, 20, 0, 0x0 }, 00070 { BUTTON_CreateIndirect, "- 1", ID_BUTTON_2, 45, 116, 80, 20, 0, 0x0 }, 00071 { BUTTON_CreateIndirect, "- 10", ID_BUTTON_3, 46, 158, 80, 20, 0, 0x0 }, 00072 { EDIT_CreateIndirect, "Edit", ID_EDIT_0, 204, 72, 80, 20, 0, 0x64 }, 00073 { TEXT_CreateIndirect, "Result :", ID_TEXT_0, 151, 74, 50, 20, 0, 0x0 }, 00074 // USER START (Optionally insert additional widgets) 00075 // USER END 00076 }; 00077 00078 /********************************************************************* 00079 * 00080 * Static code 00081 * 00082 ********************************************************************** 00083 */ 00084 00085 // USER START (Optionally insert additional static code) 00086 // USER END 00087 00088 int value; 00089 char sBuf[20]; 00090 /********************************************************************* 00091 * 00092 * _cbDialog 00093 */ 00094 static void _cbDialog(WM_MESSAGE * pMsg) 00095 { 00096 WM_HWIN hItem; 00097 int NCode; 00098 int Id; 00099 // USER START (Optionally insert additional variables) 00100 // USER END 00101 00102 switch (pMsg->MsgId) 00103 { 00104 case WM_INIT_DIALOG: 00105 // 00106 // Initialization of 'Edit' 00107 // 00108 hItem = WM_GetDialogItem(pMsg->hWin, ID_EDIT_0); 00109 EDIT_SetText(hItem, "123"); 00110 value = 123; 00111 // USER START (Optionally insert additional code for further widget initialization) 00112 // USER END 00113 break; 00114 case WM_NOTIFY_PARENT: 00115 Id = WM_GetId(pMsg->hWinSrc); 00116 NCode = pMsg->Data.v; 00117 switch(Id) 00118 { 00119 case ID_BUTTON_0: // Notifications sent by '+ 1' 00120 switch(NCode) 00121 { 00122 case WM_NOTIFICATION_CLICKED: 00123 // USER START (Optionally insert code for reacting on notification message) 00124 // USER END 00125 value += 1; 00126 sprintf(sBuf,"%d ", value); 00127 hItem = WM_GetDialogItem(pMsg->hWin, ID_EDIT_0); 00128 EDIT_SetText(hItem, sBuf); 00129 break; 00130 case WM_NOTIFICATION_RELEASED: 00131 // USER START (Optionally insert code for reacting on notification message) 00132 // USER END 00133 break; 00134 // USER START (Optionally insert additional code for further notification handling) 00135 // USER END 00136 } 00137 break; 00138 case ID_BUTTON_1: // Notifications sent by '+ 10' 00139 switch(NCode) 00140 { 00141 case WM_NOTIFICATION_CLICKED: 00142 // USER START (Optionally insert code for reacting on notification message) 00143 // USER END 00144 value += 10; 00145 sprintf(sBuf,"%d ", value); 00146 hItem = WM_GetDialogItem(pMsg->hWin, ID_EDIT_0); 00147 EDIT_SetText(hItem, sBuf); 00148 break; 00149 case WM_NOTIFICATION_RELEASED: 00150 // USER START (Optionally insert code for reacting on notification message) 00151 // USER END 00152 break; 00153 // USER START (Optionally insert additional code for further notification handling) 00154 // USER END 00155 } 00156 break; 00157 case ID_BUTTON_2: // Notifications sent by '- 1' 00158 switch(NCode) 00159 { 00160 case WM_NOTIFICATION_CLICKED: 00161 // USER START (Optionally insert code for reacting on notification message) 00162 // USER END 00163 value -= 1; 00164 sprintf(sBuf,"%d ", value); 00165 hItem = WM_GetDialogItem(pMsg->hWin, ID_EDIT_0); 00166 EDIT_SetText(hItem, sBuf); 00167 break; 00168 case WM_NOTIFICATION_RELEASED: 00169 // USER START (Optionally insert code for reacting on notification message) 00170 // USER END 00171 break; 00172 // USER START (Optionally insert additional code for further notification handling) 00173 // USER END 00174 } 00175 break; 00176 case ID_BUTTON_3: // Notifications sent by '- 10' 00177 switch(NCode) 00178 { 00179 case WM_NOTIFICATION_CLICKED: 00180 // USER START (Optionally insert code for reacting on notification message) 00181 value -= 10; 00182 sprintf(sBuf,"%d ", value); 00183 hItem = WM_GetDialogItem(pMsg->hWin, ID_EDIT_0); 00184 EDIT_SetText(hItem, sBuf); 00185 // USER END 00186 break; 00187 case WM_NOTIFICATION_RELEASED: 00188 // USER START (Optionally insert code for reacting on notification message) 00189 // USER END 00190 break; 00191 // USER START (Optionally insert additional code for further notification handling) 00192 // USER END 00193 } 00194 break; 00195 case ID_EDIT_0: // Notifications sent by 'Edit' 00196 switch(NCode) 00197 { 00198 case WM_NOTIFICATION_CLICKED: 00199 // USER START (Optionally insert code for reacting on notification message) 00200 // USER END 00201 break; 00202 case WM_NOTIFICATION_RELEASED: 00203 // USER START (Optionally insert code for reacting on notification message) 00204 // USER END 00205 break; 00206 case WM_NOTIFICATION_VALUE_CHANGED: 00207 // USER START (Optionally insert code for reacting on notification message) 00208 // USER END 00209 break; 00210 // USER START (Optionally insert additional code for further notification handling) 00211 // USER END 00212 } 00213 break; 00214 // USER START (Optionally insert additional code for further Ids) 00215 // USER END 00216 } 00217 break; 00218 // USER START (Optionally insert additional message handling) 00219 // USER END 00220 default: 00221 WM_DefaultProc(pMsg); 00222 // xSemaphoreTake(psGUISemaphore, 10/portTICK_RATE_MS); 00223 break; 00224 } 00225 } 00226 00227 /********************************************************************* 00228 * 00229 * Public code 00230 * 00231 ********************************************************************** 00232 */ 00233 /********************************************************************* 00234 * 00235 * CreateFramewin 00236 */ 00237 WM_HWIN CreateFramewin(void); 00238 WM_HWIN CreateFramewin(void) 00239 { 00240 WM_HWIN hWin; 00241 00242 hWin = GUI_CreateDialogBox(_aDialogCreate, GUI_COUNTOF(_aDialogCreate), _cbDialog, WM_HBKWIN, 0, 0); 00243 return hWin; 00244 } 00245 00246 // USER START (Optionally insert additional public code) 00247 // USER END 00248 00249 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
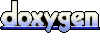