
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
DROPDOWN.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : DROPDOWN.h 00040 Purpose : Multiple choice object include 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef DROPDOWN_H 00045 #define DROPDOWN_H 00046 00047 #include "WM.h" 00048 #include "DIALOG_Intern.h" /* Req. for Create indirect data structure */ 00049 #include "LISTBOX.h" 00050 00051 #if GUI_WINSUPPORT 00052 00053 #if defined(__cplusplus) 00054 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00055 #endif 00056 00057 /************************************************************ 00058 * 00059 * Create flags 00060 */ 00061 #define DROPDOWN_CF_AUTOSCROLLBAR (1 << 0) 00062 #define DROPDOWN_CF_UP (1 << 1) 00063 00064 /********************************************************************* 00065 * 00066 * Color indices 00067 */ 00068 #define DROPDOWN_CI_UNSEL 0 00069 #define DROPDOWN_CI_SEL 1 00070 #define DROPDOWN_CI_SELFOCUS 2 00071 00072 #define DROPDOWN_CI_ARROW 0 00073 #define DROPDOWN_CI_BUTTON 1 00074 00075 /********************************************************************* 00076 * 00077 * Skinning property indices 00078 */ 00079 #define DROPDOWN_SKINFLEX_PI_EXPANDED 0 00080 #define DROPDOWN_SKINFLEX_PI_FOCUSED 1 00081 #define DROPDOWN_SKINFLEX_PI_ENABLED 2 00082 #define DROPDOWN_SKINFLEX_PI_DISABLED 3 00083 00084 /********************************************************************* 00085 * 00086 * Types 00087 * 00088 ********************************************************************** 00089 */ 00090 typedef WM_HMEM DROPDOWN_Handle; 00091 00092 typedef struct { 00093 GUI_COLOR aColorFrame[3]; 00094 GUI_COLOR aColorUpper[2]; 00095 GUI_COLOR aColorLower[2]; 00096 GUI_COLOR ColorArrow; 00097 GUI_COLOR ColorText; 00098 GUI_COLOR ColorSep; 00099 int Radius; 00100 } DROPDOWN_SKINFLEX_PROPS; 00101 00102 /********************************************************************* 00103 * 00104 * Create functions 00105 * 00106 ********************************************************************** 00107 */ 00108 DROPDOWN_Handle DROPDOWN_Create (WM_HWIN hWinParent, int x0, int y0, int xSize, int ySize, int Flags); 00109 DROPDOWN_Handle DROPDOWN_CreateEx (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id); 00110 DROPDOWN_Handle DROPDOWN_CreateUser (int x0, int y0, int xSize, int ySize, WM_HWIN hParent, int WinFlags, int ExFlags, int Id, int NumExtraBytes); 00111 DROPDOWN_Handle DROPDOWN_CreateIndirect(const GUI_WIDGET_CREATE_INFO* pCreateInfo, WM_HWIN hWinParent, int x0, int y0, WM_CALLBACK* cb); 00112 00113 /********************************************************************* 00114 * 00115 * The callback ... 00116 * 00117 * Do not call it directly ! It is only to be used from within an 00118 * overwritten callback. 00119 */ 00120 void DROPDOWN_Callback(WM_MESSAGE * pMsg); 00121 00122 /********************************************************************* 00123 * 00124 * Member functions 00125 * 00126 ********************************************************************** 00127 */ 00128 void DROPDOWN_AddKey (DROPDOWN_Handle hObj, int Key); 00129 void DROPDOWN_AddString (DROPDOWN_Handle hObj, const char* s); 00130 void DROPDOWN_Collapse (DROPDOWN_Handle hObj); 00131 void DROPDOWN_DecSel (DROPDOWN_Handle hObj); 00132 void DROPDOWN_DecSelExp (DROPDOWN_Handle hObj); 00133 void DROPDOWN_DeleteItem (DROPDOWN_Handle hObj, unsigned int Index); 00134 void DROPDOWN_Expand (DROPDOWN_Handle hObj); 00135 GUI_COLOR DROPDOWN_GetBkColor (DROPDOWN_Handle hObj, unsigned int Index); 00136 GUI_COLOR DROPDOWN_GetColor (DROPDOWN_Handle hObj, unsigned int Index); 00137 const GUI_FONT * DROPDOWN_GetFont (DROPDOWN_Handle hObj); 00138 unsigned DROPDOWN_GetItemDisabled (DROPDOWN_Handle hObj, unsigned Index); 00139 unsigned DROPDOWN_GetItemSpacing (DROPDOWN_Handle hObj); 00140 int DROPDOWN_GetItemText (DROPDOWN_Handle hObj, unsigned Index, char * pBuffer, int MaxSize); 00141 LISTBOX_Handle DROPDOWN_GetListbox (DROPDOWN_Handle hObj); 00142 int DROPDOWN_GetNumItems (DROPDOWN_Handle hObj); 00143 int DROPDOWN_GetSel (DROPDOWN_Handle hObj); 00144 int DROPDOWN_GetSelExp (DROPDOWN_Handle hObj); 00145 GUI_COLOR DROPDOWN_GetTextColor (DROPDOWN_Handle hObj, unsigned int Index); 00146 int DROPDOWN_GetUserData (DROPDOWN_Handle hObj, void * pDest, int NumBytes); 00147 void DROPDOWN_IncSel (DROPDOWN_Handle hObj); 00148 void DROPDOWN_IncSelExp (DROPDOWN_Handle hObj); 00149 void DROPDOWN_InsertString (DROPDOWN_Handle hObj, const char* s, unsigned int Index); 00150 void DROPDOWN_SetAutoScroll (DROPDOWN_Handle hObj, int OnOff); 00151 void DROPDOWN_SetBkColor (DROPDOWN_Handle hObj, unsigned int Index, GUI_COLOR color); 00152 void DROPDOWN_SetColor (DROPDOWN_Handle hObj, unsigned int Index, GUI_COLOR Color); 00153 void DROPDOWN_SetFont (DROPDOWN_Handle hObj, const GUI_FONT * pfont); 00154 void DROPDOWN_SetItemDisabled (DROPDOWN_Handle hObj, unsigned Index, int OnOff); 00155 void DROPDOWN_SetItemSpacing (DROPDOWN_Handle hObj, unsigned Value); 00156 int DROPDOWN_SetListHeight (DROPDOWN_Handle hObj, unsigned Height); 00157 void DROPDOWN_SetScrollbarColor(DROPDOWN_Handle hObj, unsigned Index, GUI_COLOR Color); 00158 void DROPDOWN_SetScrollbarWidth(DROPDOWN_Handle hObj, unsigned Width); 00159 void DROPDOWN_SetSel (DROPDOWN_Handle hObj, int Sel); 00160 void DROPDOWN_SetSelExp (DROPDOWN_Handle hObj, int Sel); 00161 void DROPDOWN_SetTextAlign (DROPDOWN_Handle hObj, int Align); 00162 void DROPDOWN_SetTextColor (DROPDOWN_Handle hObj, unsigned int index, GUI_COLOR color); 00163 void DROPDOWN_SetTextHeight (DROPDOWN_Handle hObj, unsigned TextHeight); 00164 int DROPDOWN_SetUpMode (DROPDOWN_Handle hObj, int OnOff); 00165 int DROPDOWN_SetUserData (DROPDOWN_Handle hObj, const void * pSrc, int NumBytes); 00166 00167 /********************************************************************* 00168 * 00169 * Member functions: Skinning 00170 * 00171 ********************************************************************** 00172 */ 00173 void DROPDOWN_GetSkinFlexProps (DROPDOWN_SKINFLEX_PROPS * pProps, int Index); 00174 void DROPDOWN_SetSkinClassic (DROPDOWN_Handle hObj); 00175 void DROPDOWN_SetSkin (DROPDOWN_Handle hObj, WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00176 int DROPDOWN_DrawSkinFlex (const WIDGET_ITEM_DRAW_INFO * pDrawItemInfo); 00177 void DROPDOWN_SetSkinFlexProps (const DROPDOWN_SKINFLEX_PROPS * pProps, int Index); 00178 void DROPDOWN_SetDefaultSkinClassic(void); 00179 WIDGET_DRAW_ITEM_FUNC * DROPDOWN_SetDefaultSkin(WIDGET_DRAW_ITEM_FUNC * pfDrawSkin); 00180 00181 #define DROPDOWN_SKIN_FLEX DROPDOWN_DrawSkinFlex 00182 00183 /********************************************************************* 00184 * 00185 * Managing default values 00186 * 00187 ********************************************************************** 00188 */ 00189 GUI_COLOR DROPDOWN_GetDefaultBkColor (int Index); 00190 GUI_COLOR DROPDOWN_GetDefaultColor (int Index); 00191 const GUI_FONT * DROPDOWN_GetDefaultFont (void); 00192 GUI_COLOR DROPDOWN_GetDefaultScrollbarColor(int Index); 00193 void DROPDOWN_SetDefaultFont (const GUI_FONT * pFont); 00194 GUI_COLOR DROPDOWN_SetDefaultBkColor (int Index, GUI_COLOR Color); 00195 GUI_COLOR DROPDOWN_SetDefaultColor (int Index, GUI_COLOR Color); 00196 GUI_COLOR DROPDOWN_SetDefaultScrollbarColor(int Index, GUI_COLOR Color); 00197 00198 #if defined(__cplusplus) 00199 } 00200 #endif 00201 00202 #endif // GUI_WINSUPPORT 00203 #endif // DROPDOWN_H 00204 00205 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
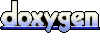