
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
CHOOSECOLOR.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : CHOOSECOLOR.h 00040 Purpose : Message box interface 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef CHOOSECOLOR_H 00045 #define CHOOSECOLOR_H 00046 00047 #include "WM.h" 00048 00049 #if GUI_WINSUPPORT 00050 00051 #if defined(__cplusplus) 00052 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00053 #endif 00054 00055 /********************************************************************* 00056 * 00057 * Defines 00058 * 00059 ********************************************************************** 00060 */ 00061 #define CHOOSECOLOR_CF_MOVEABLE FRAMEWIN_CF_MOVEABLE 00062 00063 #define CHOOSECOLOR_CI_FRAME 0 00064 #define CHOOSECOLOR_CI_FOCUS 1 00065 00066 /********************************************************************* 00067 * 00068 * Types 00069 * 00070 ********************************************************************** 00071 */ 00072 /********************************************************************* 00073 * 00074 * CHOOSECOLOR_PROPS 00075 */ 00076 typedef struct { 00077 unsigned aBorder[2]; 00078 unsigned aSpace[2]; 00079 unsigned aButtonSize[2]; 00080 GUI_COLOR aColor[2]; 00081 } CHOOSECOLOR_PROPS; 00082 00083 /********************************************************************* 00084 * 00085 * CHOOSECOLOR_CONTEXT 00086 */ 00087 typedef struct { 00088 U32 LastColor; 00089 const GUI_COLOR * pColor; 00090 unsigned NumColors; 00091 unsigned NumColorsPerLine; 00092 int SelOld; 00093 int Sel; 00094 WM_HWIN hParent; 00095 CHOOSECOLOR_PROPS Props; 00096 } CHOOSECOLOR_CONTEXT; 00097 00098 /********************************************************************* 00099 * 00100 * Public code 00101 * 00102 ********************************************************************** 00103 */ 00104 WM_HWIN CHOOSECOLOR_Create(WM_HWIN hParent, 00105 int xPos, 00106 int yPos, 00107 int xSize, 00108 int ySize, 00109 const GUI_COLOR * pColor, 00110 unsigned NumColors, 00111 unsigned NumColorsPerLine, 00112 int Sel, 00113 const char * sCaption, 00114 int Flags); 00115 00116 int CHOOSECOLOR_GetSel(WM_HWIN hObj); 00117 void CHOOSECOLOR_SetSel(WM_HWIN hObj, int Sel); 00118 00119 void CHOOSECOLOR_SetDefaultColor (unsigned Index, GUI_COLOR Color); 00120 void CHOOSECOLOR_SetDefaultSpace (unsigned Index, unsigned Space); 00121 void CHOOSECOLOR_SetDefaultBorder (unsigned Index, unsigned Border); 00122 void CHOOSECOLOR_SetDefaultButtonSize(unsigned Index, unsigned ButtonSize); 00123 00124 /********************************************************************* 00125 * 00126 * The callback ... 00127 * 00128 * Do not call it directly ! It is only to be used from within an 00129 * overwritten callback. 00130 */ 00131 void CHOOSECOLOR_Callback(WM_MESSAGE * pMsg); 00132 00133 #if defined(__cplusplus) 00134 } 00135 #endif 00136 00137 #endif /* GUI_WINSUPPORT */ 00138 00139 #endif /* CHOOSECOLOR_H */ 00140 00141 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
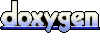