
NuMaker emWin HMI
Embed:
(wiki syntax)
Show/hide line numbers
CALENDAR.h
00001 /********************************************************************* 00002 * SEGGER Software GmbH * 00003 * Solutions for real time microcontroller applications * 00004 ********************************************************************** 00005 * * 00006 * (c) 1996 - 2018 SEGGER Microcontroller GmbH * 00007 * * 00008 * Internet: www.segger.com Support: support@segger.com * 00009 * * 00010 ********************************************************************** 00011 00012 ** emWin V5.48 - Graphical user interface for embedded applications ** 00013 All Intellectual Property rights in the Software belongs to SEGGER. 00014 emWin is protected by international copyright laws. Knowledge of the 00015 source code may not be used to write a similar product. This file may 00016 only be used in accordance with the following terms: 00017 00018 The software has been licensed by SEGGER Software GmbH to Nuvoton Technology Corporationat the address: No. 4, Creation Rd. III, Hsinchu Science Park, Taiwan 00019 for the purposes of creating libraries for its 00020 Arm Cortex-M and Arm9 32-bit microcontrollers, commercialized and distributed by Nuvoton Technology Corporation 00021 under the terms and conditions of an End User 00022 License Agreement supplied with the libraries. 00023 Full source code is available at: www.segger.com 00024 00025 We appreciate your understanding and fairness. 00026 ---------------------------------------------------------------------- 00027 Licensing information 00028 Licensor: SEGGER Software GmbH 00029 Licensed to: Nuvoton Technology Corporation, No. 4, Creation Rd. III, Hsinchu Science Park, 30077 Hsinchu City, Taiwan 00030 Licensed SEGGER software: emWin 00031 License number: GUI-00735 00032 License model: emWin License Agreement, signed February 27, 2018 00033 Licensed platform: Cortex-M and ARM9 32-bit series microcontroller designed and manufactured by or for Nuvoton Technology Corporation 00034 ---------------------------------------------------------------------- 00035 Support and Update Agreement (SUA) 00036 SUA period: 2018-03-26 - 2019-03-27 00037 Contact to extend SUA: sales@segger.com 00038 ---------------------------------------------------------------------- 00039 File : CALENDAR.h 00040 Purpose : Message box interface 00041 --------------------END-OF-HEADER------------------------------------- 00042 */ 00043 00044 #ifndef CALENDAR_H 00045 #define CALENDAR_H 00046 00047 #include "WM.h" 00048 00049 #if GUI_WINSUPPORT 00050 00051 #if defined(__cplusplus) 00052 extern "C" { /* Make sure we have C-declarations in C++ programs */ 00053 #endif 00054 00055 /********************************************************************* 00056 * 00057 * Defines 00058 * 00059 ********************************************************************** 00060 */ 00061 #define CALENDAR_CI_WEEKEND 0 00062 #define CALENDAR_CI_WEEKDAY 1 00063 #define CALENDAR_CI_SEL 2 00064 00065 #define CALENDAR_CI_HEADER 3 00066 #define CALENDAR_CI_MONTH 4 00067 #define CALENDAR_CI_LABEL 5 00068 #define CALENDAR_CI_FRAME 6 00069 00070 #define CALENDAR_FI_CONTENT 0 00071 #define CALENDAR_FI_HEADER 1 00072 00073 #define CALENDAR_SI_HEADER 0 00074 #define CALENDAR_SI_CELL_X 1 00075 #define CALENDAR_SI_CELL_Y 2 00076 00077 /********************************************************************* 00078 * 00079 * Notification codes 00080 * 00081 * The following is the list of notification codes specific to this widget, 00082 * Send with the WM_NOTIFY_PARENT message 00083 */ 00084 #define CALENDAR_NOTIFICATION_MONTH_CLICKED (WM_NOTIFICATION_WIDGET + 0) 00085 #define CALENDAR_NOTIFICATION_MONTH_RELEASED (WM_NOTIFICATION_WIDGET + 1) 00086 00087 /********************************************************************* 00088 * 00089 * Types 00090 * 00091 ********************************************************************** 00092 */ 00093 /********************************************************************* 00094 * 00095 * CALENDAR_DATE 00096 */ 00097 typedef struct { 00098 int Year; 00099 int Month; 00100 int Day; 00101 } CALENDAR_DATE; 00102 00103 /********************************************************************* 00104 * 00105 * CALENDAR_SKINFLEX_PROPS 00106 */ 00107 typedef struct { 00108 GUI_COLOR aColorFrame[3]; // Frame colors of buttons 00109 GUI_COLOR aColorUpper[2]; // Upper gradient colors of buttons 00110 GUI_COLOR aColorLower[2]; // Lower gradient colors of buttons 00111 GUI_COLOR ColorArrow; // Arrow colors 00112 } CALENDAR_SKINFLEX_PROPS; 00113 00114 /********************************************************************* 00115 * 00116 * Public code 00117 * 00118 ********************************************************************** 00119 */ 00120 WM_HWIN CALENDAR_Create (WM_HWIN hParent, int xPos, int yPos, unsigned Year, unsigned Month, unsigned Day, unsigned FirstDayOfWeek, int Id, int Flags); 00121 void CALENDAR_GetDate (WM_HWIN hWin, CALENDAR_DATE * pDate); 00122 int CALENDAR_GetDaysOfMonth (CALENDAR_DATE * pDate); 00123 void CALENDAR_GetSel (WM_HWIN hWin, CALENDAR_DATE * pDate); 00124 int CALENDAR_GetWeekday (CALENDAR_DATE * pDate); 00125 void CALENDAR_SetDate (WM_HWIN hWin, CALENDAR_DATE * pDate); 00126 void CALENDAR_SetSel (WM_HWIN hWin, CALENDAR_DATE * pDate); 00127 void CALENDAR_ShowDate (WM_HWIN hWin, CALENDAR_DATE * pDate); 00128 int CALENDAR_AddKey (WM_HWIN hWin, int Key); 00129 00130 /********************************************************************* 00131 * 00132 * Default related 00133 */ 00134 void CALENDAR_SetDefaultBkColor(unsigned Index, GUI_COLOR Color); 00135 void CALENDAR_SetDefaultColor (unsigned Index, GUI_COLOR Color); 00136 void CALENDAR_SetDefaultDays (const char ** apDays); 00137 void CALENDAR_SetDefaultFont (unsigned Index, const GUI_FONT * pFont); 00138 void CALENDAR_SetDefaultMonths (const char ** apMonths); 00139 void CALENDAR_SetDefaultSize (unsigned Index, unsigned Size); 00140 00141 /********************************************************************* 00142 * 00143 * Skinning related 00144 */ 00145 void CALENDAR_GetSkinFlexProps (CALENDAR_SKINFLEX_PROPS * pProps, int Index); 00146 void CALENDAR_SetSkinFlexProps (const CALENDAR_SKINFLEX_PROPS * pProps, int Index); 00147 00148 /********************************************************************* 00149 * 00150 * The callback ... 00151 * 00152 * Do not call it directly ! It is only to be used from within an 00153 * overwritten callback. 00154 */ 00155 void CALENDAR_Callback(WM_MESSAGE * pMsg); 00156 00157 #if defined(__cplusplus) 00158 } 00159 #endif 00160 00161 #endif // GUI_WINSUPPORT 00162 #endif // CALENDAR_H 00163 00164 /*************************** End of file ****************************/
Generated on Mon Mar 4 2024 07:48:13 by
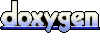