
NuMaker-PFM-NUC472: I2C1 LCD draw a moving circle
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // NuMaker-PFM-NUC472 : I2C1 LCD draw a moving circle 00002 #include "mbed.h" 00003 #include "math.h" 00004 #include "ssd1306.h" 00005 #include "draw2D.h" 00006 00007 #define PI 3.1415926535 00008 00009 #define X0 15 // Circle initial X 00010 #define Y0 10 // Circle initial Y 00011 00012 I2C i2c1(PD_12, PD_10); // I2C1_SDA, I2C1_SCL 00013 00014 SSD1306 LCD; // LCD connected on I2C1 00015 Draw2D D2D; // Draw2D library 00016 00017 int main() { 00018 00019 int dirX, dirY; 00020 int movX, movY; 00021 int r; 00022 int x, y; 00023 00024 i2c1.frequency(400000); 00025 00026 LCD.initialize(); 00027 LCD.clearscreen(); 00028 00029 x = X0; // circle center x 00030 y = Y0; // circle center y 00031 r = 3; // circle radius 00032 00033 movX = 3; // x movement 00034 movY = 3; // y movement 00035 dirX = 1; // x direction 00036 dirY = 1; // y direction 00037 00038 while(true) { 00039 D2D.drawCircle(x, y, r, FG_COLOR, BG_COLOR); // draw a circle 00040 00041 Thread::wait(1); // Delay for Vision 00042 00043 D2D.drawCircle(x, y, r, BG_COLOR, BG_COLOR); // erase a circle 00044 00045 x = x + dirX * movX; // change x of circle center 00046 y = y + dirY * movY; // change y of circle center 00047 00048 // boundary check for changing direction 00049 if ((x-r) <=0) {dirX= 1; x= x + dirX*movX;} 00050 else if ((x+r) >=LCD_Xmax) {dirX=-1; x= x + dirX*movX;} 00051 00052 if ((y-r) <=0) {dirY= 1; y= y + dirY*movY;} 00053 else if ((y+r) >=LCD_Ymax) {dirY=-1; y= y + dirY*movY;} 00054 } 00055 }
Generated on Wed Jul 20 2022 17:53:20 by
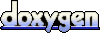